Dagster Data Engineering Glossary:
Feature Selection
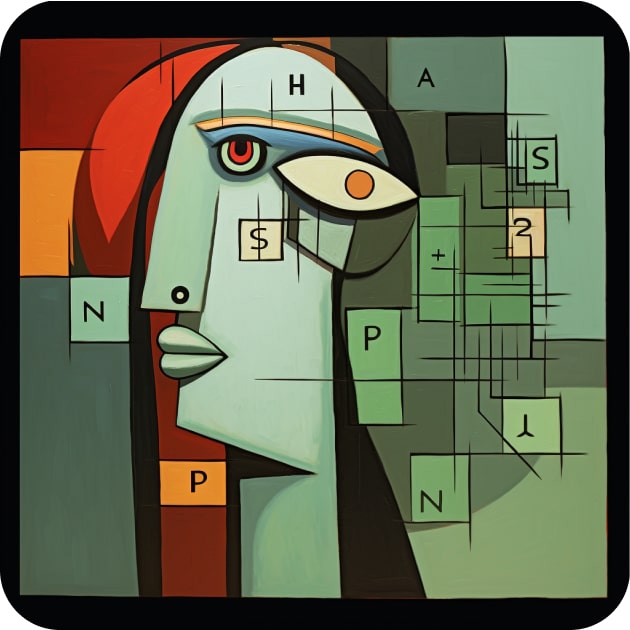
Feature selection definition:
Feature selection is the process of selecting a subset of relevant features (variables or columns) from a larger set of features to use in a model or analysis. This process can help reduce the dimensionality of the data and improve the accuracy and efficiency of the model. In the context of modern data pipelines, feature selection is an important step in preparing data for machine learning algorithms.
Feature selection isn’t always necessary or beneficial for every dataset or model. It's important to evaluate the impact of feature selection on model performance and to carefully consider the trade-off between reducing dimensionality and potentially losing important information.
Python techniques for feature selection
Please note that you need to have the necessary Python libraries installed in your Python environment to run the following code examples.
There are many techniques for feature selection, ranging from simple threshold-based methods to more complex methods like wrapper and embedded methods. Some popular methods for feature selection include:
- Filter methods: These methods select features based on their statistical properties, such as correlation with the target variable or variance. Examples of filter methods in Python include
SelectKBest
andSelectPercentile
from scikit-learn. - Wrapper methods: These methods evaluate the performance of a model using a particular subset of features, and select the best subset based on the model performance. Examples of wrapper methods in Python include recursive feature elimination (RFE) and forward selection.
- Embedded methods: These methods perform feature selection as part of the model training process. Examples of embedded methods in Python include Lasso and Ridge regression, which perform regularization to select relevant features.
Here's an example of using SelectKBest
from scikit-learn to select the top 5 features based on their correlation with the target variable:
import numpy as np
from sklearn.feature_selection import SelectKBest, f_regression
np.random.seed(42)
X = np.random.rand(100, 10)
y = np.random.rand(100)
# select top 5 features based on correlation with y
selector = SelectKBest(score_func=f_regression, k=5)
X_new = selector.fit_transform(X, y)
# get indices of selected features
mask = selector.get_support()
selected_features = np.arange(X.shape[1])[mask]
print(selected_features)
In this example, we generate some sample data - a matrix X
with 10 features and 100 samples, and a vector y
with the target variable.
We then use SelectKBest
to select the top 5 features based on their correlation with y
. We use the f_regression
scoring function, which computes the correlation between each feature and y
using an F-test.
The fit_transform
method of the SelectKBest
object returns a new matrix X_new
with only the top 5 features selected. We can also access the selected features using the get_support
method:
The above code will yield this output:
[0 1 3 6 8]
So the top 5 features based on their correlation with y are the 0th, 1st, 3rd, 6th, and 8th features.
Aggregate
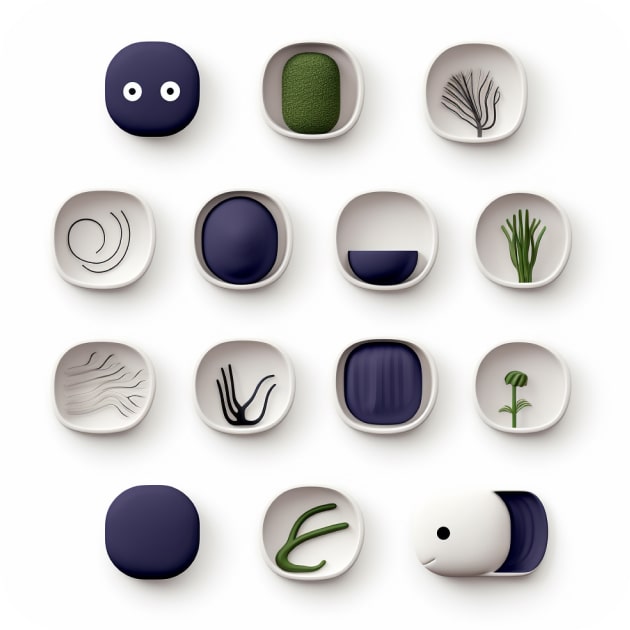
Anomaly Detection
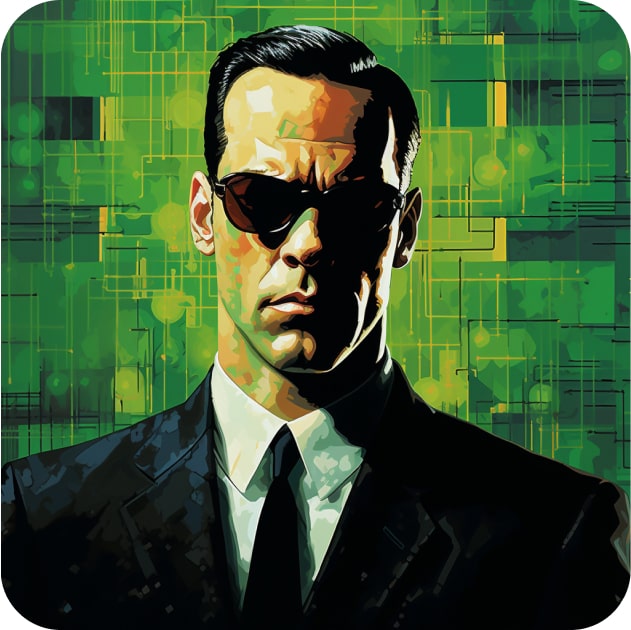
Consolidate
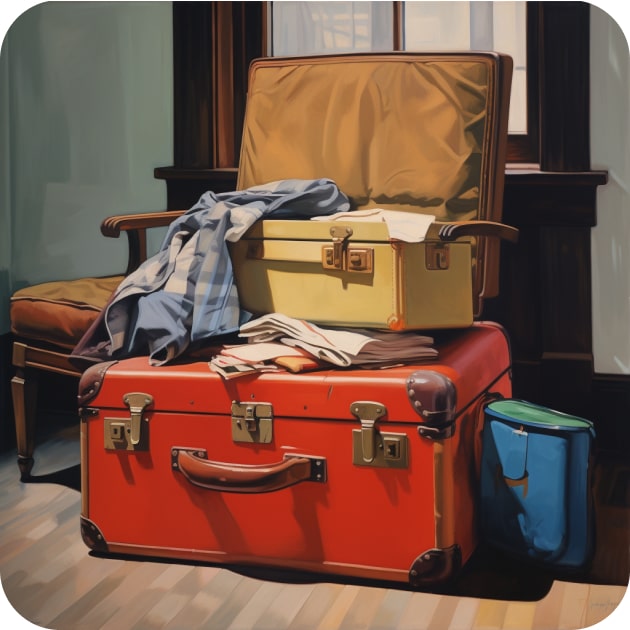
Downsample
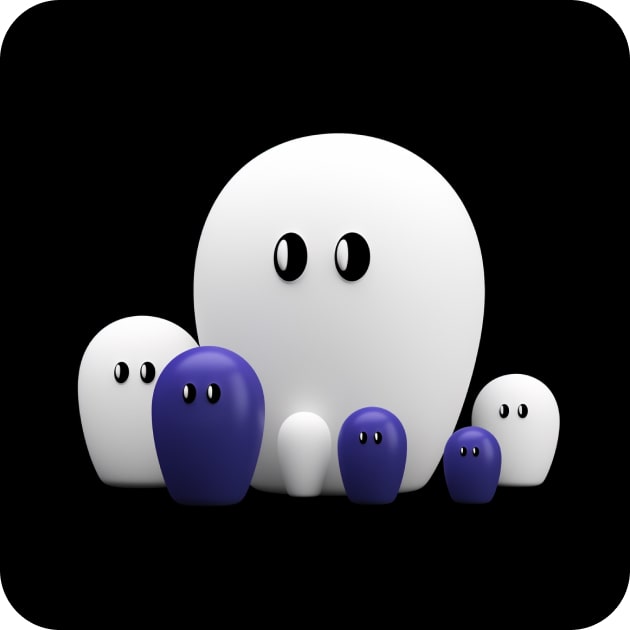
Feature Extraction
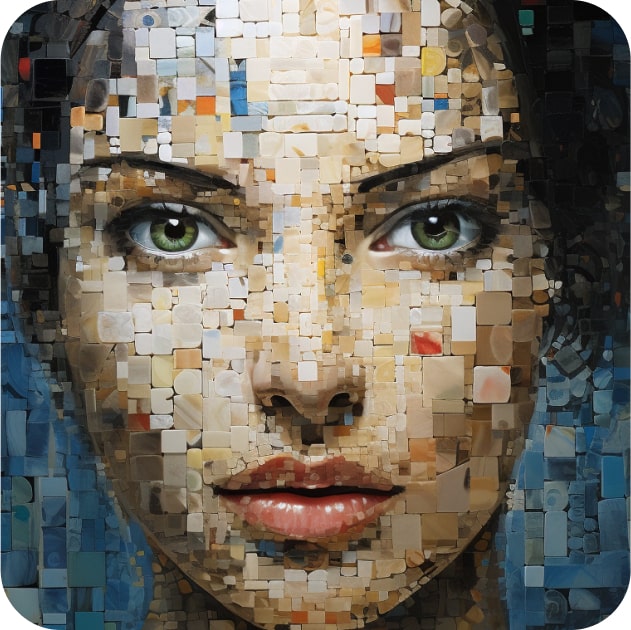