Data Backpressure
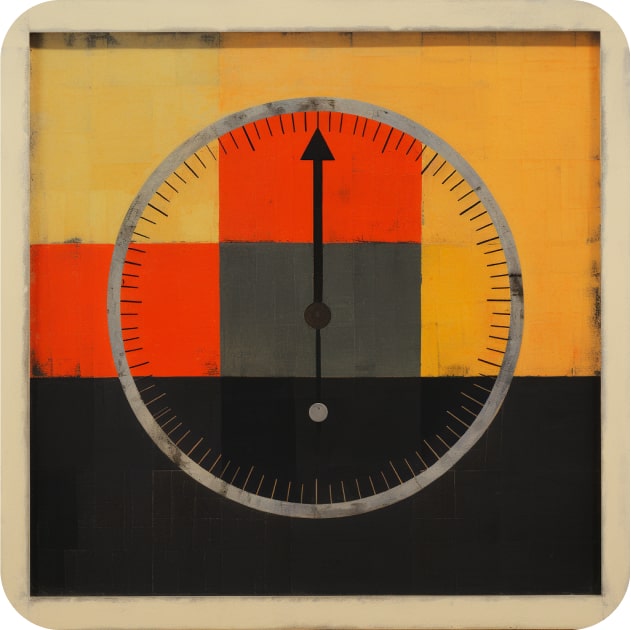
Definition of backpressure in data
In the context of data engineering and particularly in stream processing and message passing systems, the term "backpressure" refers to a mechanism to handle situations where data is produced faster than it can be consumed.
Some areas and systems where you will see backpressure mechanisms deployed include:
- Reactive Streams: This is a standard for asynchronous stream processing with non-blocking backpressure. Popular libraries/frameworks like Akka Streams, Project Reactor, and RxJava have incorporated this.
- Apache Kafka provides a form of backpressure by allowing consumers to pull data at their own pace, rather than having data pushed to them.
- Apache Flink & Apache Spark Streaming both have mechanisms to handle backpressure to ensure that the streaming jobs can cope with large amounts of data.
Why is backpressure needed?
Imagine a scenario where you have a producer (or multiple producers) sending data to a consumer for processing. If the producer sends data faster than the consumer can handle, without a controlling mechanism in place, the consumer might be overwhelmed. This could lead to data loss, system crashes, or other undesirable effects.
Backpressure is essentially a feedback mechanism where the consumer can signal the producer about its current capacity. If the consumer is overwhelmed, it can slow down the producer or even stop it temporarily to prevent overloading.
The benefites and challenges of building backpressure into your pipelines:
Building a backpressure mechanism into your pipeline provides several key benefits:
- Avoids Overloading: Backpressure prevents downstream systems (consumers) from being overloaded by upstream systems (producers).
- Resource Efficiency: Instead of wasting resources (like memory) on data that can't be processed immediately, systems can utilize resources more efficiently.
- Enhanced Stability: It makes the data processing pipeline more resilient and stable, reducing the chances of failures.
This said, there are also challenges associated with this approach:
- Balancing: Striking the right balance is key. Too much backpressure can make the system slow and underutilized, while too little can lead to overloading and potential data loss.
- Propagation: In complex systems with multiple stages, backpressure needs to be propagated correctly through all components to be effective.
- Latency: Introducing backpressure can add latency, especially if data has to wait before being processed.
Approaches to implementing ("applying") backpressure:
There are a number of techniques for implementing a backpressure mechanism in data flows:
- Buffering: Introduce buffers (or queues) between the producer and consumer. When the buffer reaches a threshold, it can signal the producer to slow down.
- Rate Limiting: Limit the rate at which a producer can send data to the consumer.
- Dynamic Adjustment: Some systems can dynamically adjust the rate of data flow based on the current state of the consumer.
- Acknowledgements: The consumer sends an acknowledgment after processing a piece of data. The producer waits for this acknowledgment before sending more data.
In conclusion, backpressure is a fundamental concept in data engineering, especially in real-time processing systems. It helps in ensuring that systems are resilient, efficient, and that they can handle variable data loads effectively.
An example of backpressure in data pipelines using Python
Here's a simple example using Python to demonstrate a backpressure mechanism. In this example, we'll use Python's queue
for buffering and threading
to simulate data producers and data consumers. In this example:
- We have a shared
buffer
(of size 5) between the producer and consumer. - The producer generates a random number and tries to put it into the buffer.
- If the buffer is full, the producer waits.
- The consumer tries to consume data from the buffer.
- If the buffer is empty, the consumer waits.
- The consumer sleeps for a slightly longer random time to simulate backpressure, i.e., the consumer processing items slower than the producer produces them.
import queue
import threading
import time
import random
class Producer(threading.Thread):
def __init__(self, buffer):
super().__init__()
self.buffer = buffer
def run(self):
while True:
item = random.randint(1, 100)
print(f"Producing {item}")
# If the buffer is full, wait before producing more
while self.buffer.full():
print("Buffer full, waiting...")
time.sleep(1)
self.buffer.put(item)
time.sleep(random.random()) # Sleep for a random time
class Consumer(threading.Thread):
def __init__(self, buffer):
super().__init__()
self.buffer = buffer
def run(self):
while True:
# If the buffer is empty, wait
while self.buffer.empty():
print("Buffer empty, waiting for items...")
time.sleep(1)
item = self.buffer.get()
print(f"Consuming {item}")
time.sleep(1 + random.random()) # Sleep for a slightly longer random time to simulate backpressure
if __name__ == "__main__":
buffer = queue.Queue(maxsize=5) # Limited size buffer to store data items
producer = Producer(buffer)
consumer = Consumer(buffer)
producer.start()
consumer.start()
producer.join()
consumer.join()
When you run the program, you'll notice periods where the producer has to wait because the buffer is full, illustrating the backpressure mechanism in action. The output will look something like this:
Producing 82
Buffer full, waiting...
Consuming 24
Producing 97
Buffer full, waiting...
Consuming 99
Producing 100
Compact
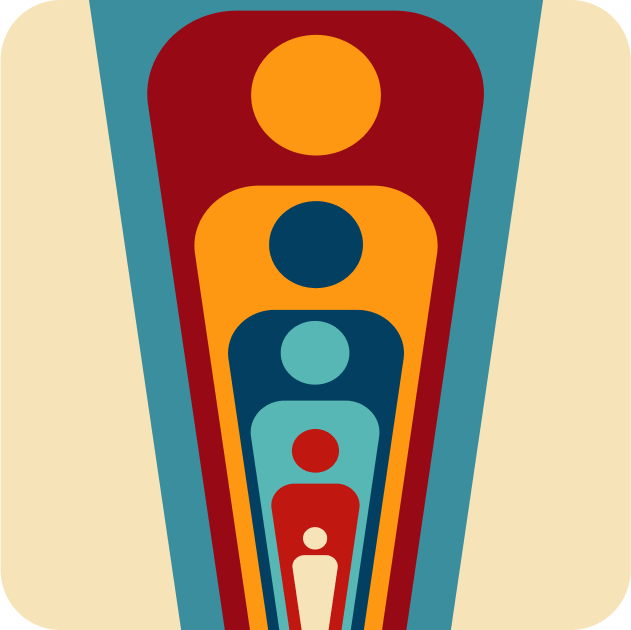
Compress
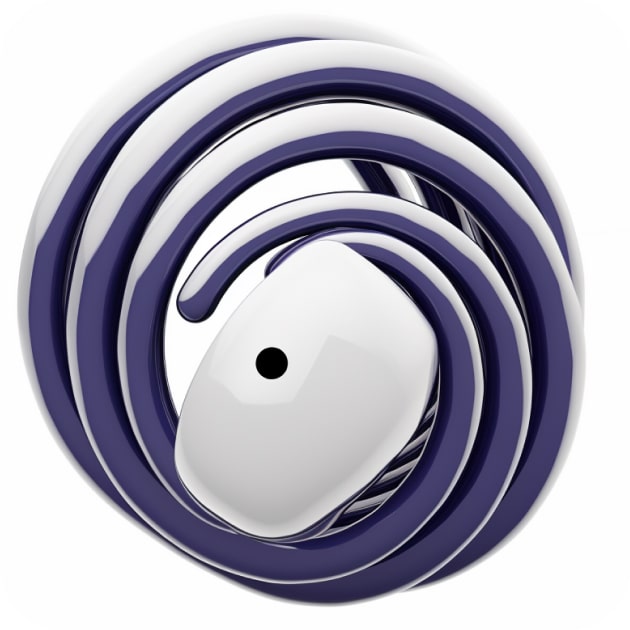
Ingest
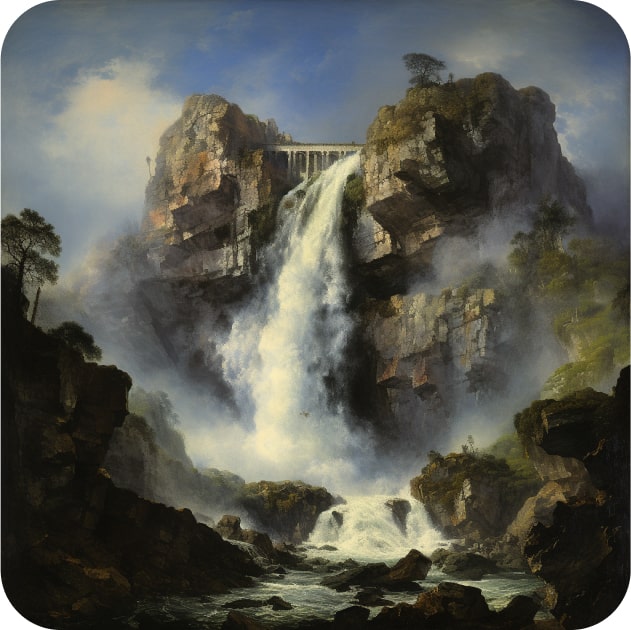
Load
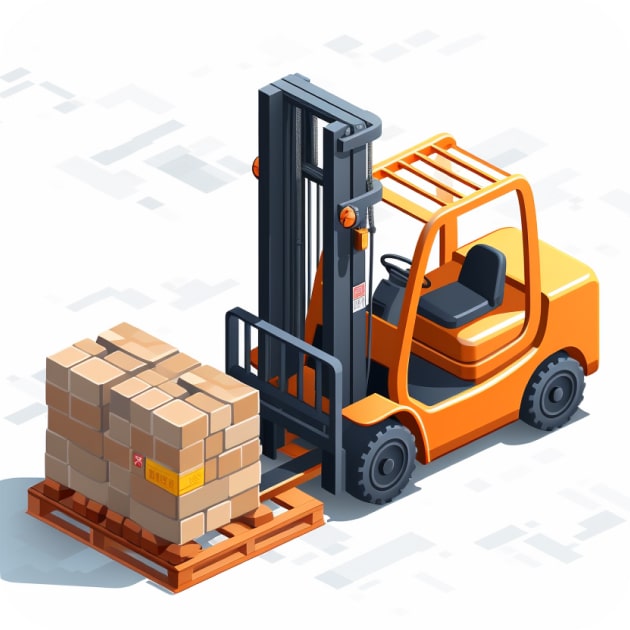
NoSQL
Pickle
Rebalance
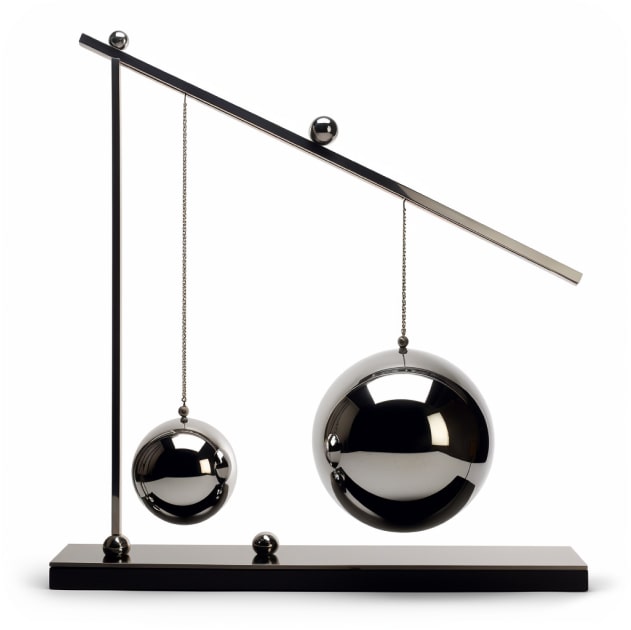
Repartition
Scrape
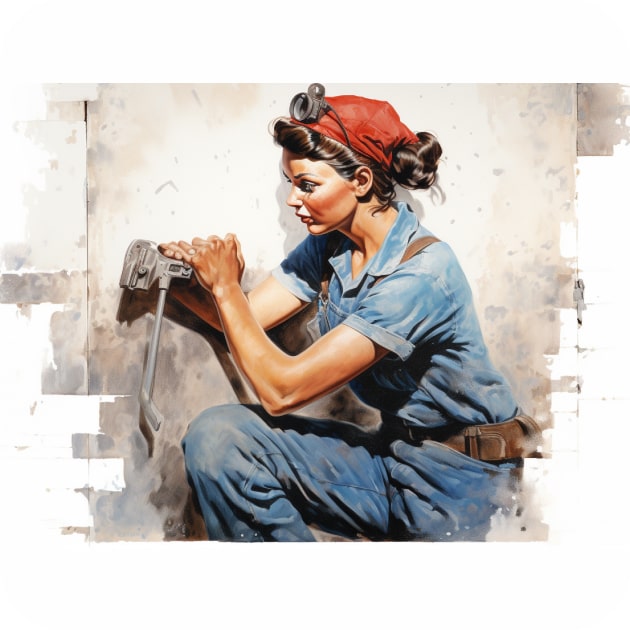
Shard
Shuffle
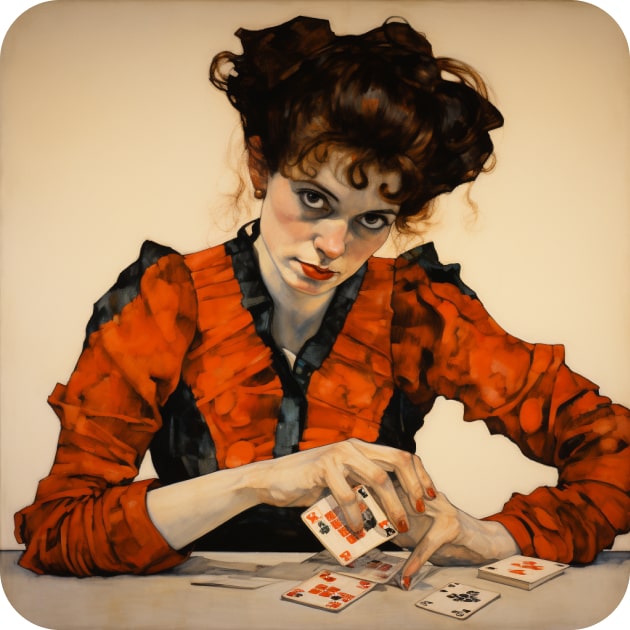
Spill
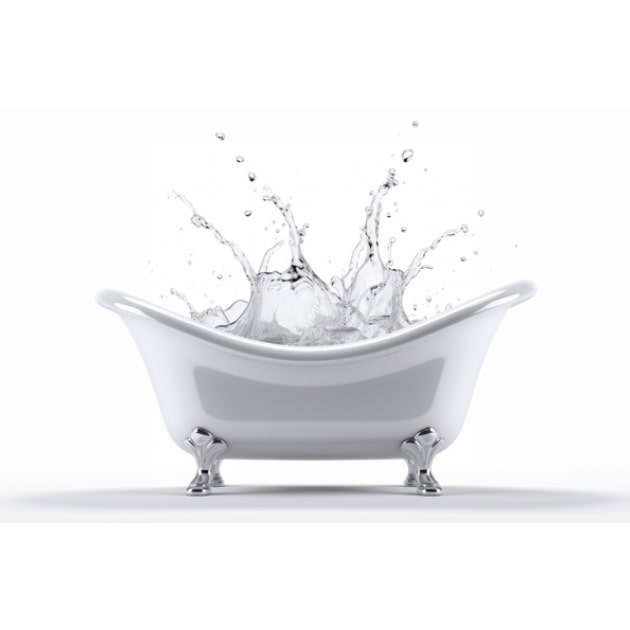
Stored Procedure
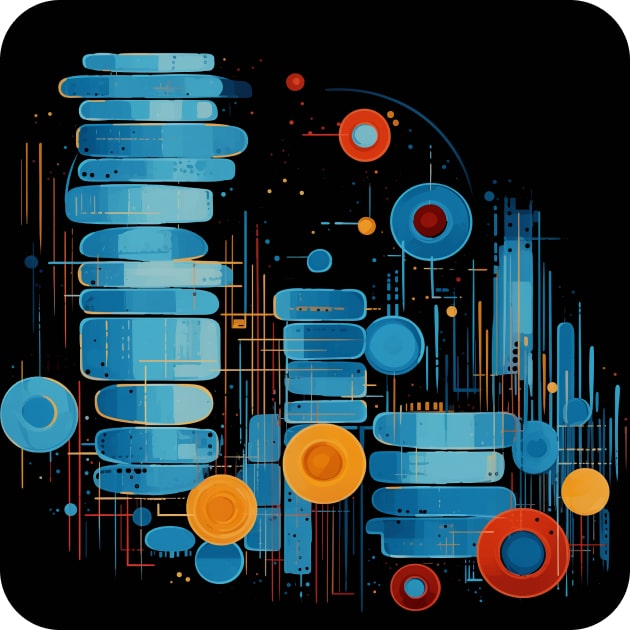
Upsert
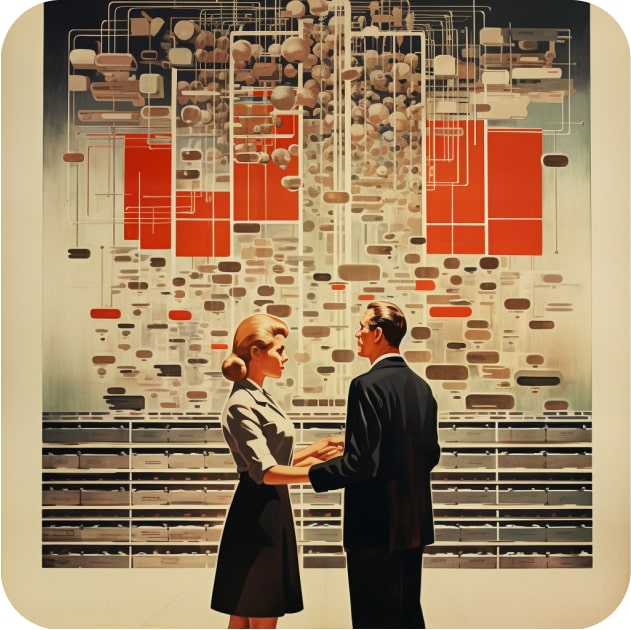