Dagster Data Engineering Glossary:
Data Rebalancing
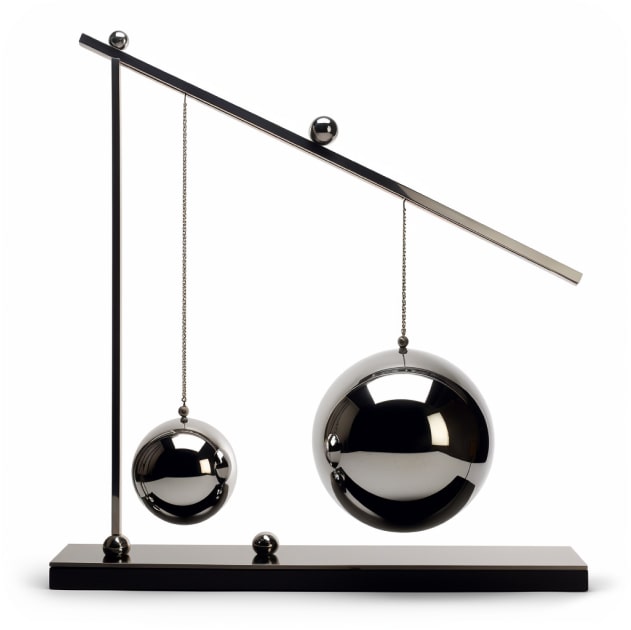
Data rebalancing definition:
Data rebalancing refers to the process of redistributing data across nodes or partitions in a distributed system to ensure optimal utilization of resources and balanced load. As data is added, removed, or updated, or as nodes are added or removed, imbalances can emerge, which might lead to hotspots (some nodes being heavily used while others are under-utilized) or inefficient data access patterns.
Why is data rebalancing important?
Performance Optimization: Without rebalancing, some nodes can become overloaded while others are under-utilized, leading to performance bottlenecks.
Fault Tolerance: In distributed storage systems like Hadoop's HDFS or Apache Kafka, data is often replicated across multiple nodes for fault tolerance. Proper rebalancing ensures that data replicas are well-distributed, enhancing the system's resilience to node failures.
Scalability: As a cluster grows or shrinks, rebalancing helps in efficiently integrating new nodes or decommissioning old ones.
Storage Efficiency: Ensuring data is evenly distributed helps in making the best use of available storage capacity across the cluster.
How is data rebalancing done?
Manual Rebalancing: Some systems offer tools or commands that allow administrators to manually trigger a rebalancing operation.
Automatic Rebalancing: Some distributed systems automatically detect imbalances and trigger rebalancing without human intervention.
Consistent Hashing: This is a technique often used in distributed caching systems (like Cassandra). Instead of directly hashing an item to a specific node, the item is hashed to a point on a continuum or "ring". As nodes are added or removed, only a fraction of the keys need to be remapped and moved, making rebalancing more efficient.
Examples in distributed systems:
Apache Kafka: Kafka is a distributed streaming platform where data is stored in topics that are split into partitions. These partitions are distributed across different broker nodes. As data grows, or when brokers are added or removed, Kafka might need to rebalance these partitions for load distribution.
Hadoop's HDFS: Hadoop’s distributed filesystem creates replicas of data blocks. When new nodes are added, or data grows disproportionately, HDFS can redistribute blocks to ensure that each node in the cluster is utilized effectively.
GlusterFS: GlusterFS supports adding new bricks (storage units) to a volume. When this happens, data might need to be rebalanced across the bricks.
Ceph: As an object storage system, Ceph uses the CRUSH algorithm and CRUSH maps to decide where to store data. When new nodes or storage devices are added, Ceph may rebalance data to ensure efficient utilization.
Swift: In OpenStack Swift, data is stored as replicas across nodes. When a new node is added or an existing one fails, Swift rebalances the data to ensure the desired replication factor is maintained.
Riak: Riak also requires rebalancing when adding or removing nodes from the cluster. It redistributes the data to maintain an even distribution.
CockroachDB: As a distributed SQL database, CockroachDB automatically rebalances data as nodes are added or removed to ensure that each node has roughly the same amount of data.
MinIO: MinIO's erasure-coded setup can be expanded by adding new nodes. When this occurs, data might need to be rebalanced to make use of the new storage capacity.
The role of data orchestration in data rebalancing
Data Orchestration in Data Rebalancing
Data orchestration plays a pivotal role in data rebalancing by automating, coordinating, and optimizing the data movement processes across disparate storage resources. It is responsible for making decisions on when to rebalance data, which data to move, and where to place it. This involves:
Monitoring Workloads: By continually monitoring data access patterns and node workloads, orchestration systems can detect imbalances early and respond proactively.
Intelligent Movement: Instead of rudimentary data transfers, orchestration ensures data is moved intelligently, considering factors like network bandwidth, storage capacity, and anticipated future workloads.
Minimizing Disruption: A key aspect of data orchestration is to perform rebalancing with minimal disruption to ongoing operations. This requires smart algorithms and strategies to transfer data without significantly affecting system performance or availability.
Automation and Policies: Data orchestration tools allow administrators to set policies for rebalancing, ensuring that the system adheres to predefined guidelines about data locality, redundancy, and other factors. Automation aids in minimizing manual intervention, thus reducing potential human-induced errors.
Fault Tolerance and Recovery: In case of node failures, data orchestration plays a role in restoring data distribution by triggering rebalancing actions, ensuring that the system maintains its resilience and service levels.
In essence, data orchestration provides the tools and frameworks needed to carry out rebalancing seamlessly. As data grows and systems evolve, data orchestration becomes the linchpin, ensuring that data is always optimally placed, accessible, and resilient.
For many platforms the cloud providers (such as Amazon or Google) or the technology platform itself will handle data distribution and rebalancing for us behind the scenes in their storage services, abstracting those details from the end-users. In other circumstances where we want greater control, we can orchestrate rebalancing tasks ourselves.
Challenges:
Service Impact: Rebalancing can be resource-intensive, which might impact the performance of the system during the operation.
Data Transfer Costs: Especially in cloud environments, transferring large amounts of data during rebalancing can lead to increased costs.
Complexity: Rebalancing algorithms need to be efficient, and ensuring optimal distribution without frequent unnecessary data movement can be complex.
An example of data rebalancing in Python:
To keep our example simple we will simulate nodes on a network using a basic local program. We'll represent each node as a list in Python, and our distributed system will be a list of these nodes (lists).
Suppose we have a distributed system with multiple nodes that store data. Over time, data can be added to these nodes, leading to imbalances. Our goal is to rebalance the data such that each node has an approximately equal number of data items.
Certainly! Let's build an example that delves into how a distributed key-value store with hash partitioning can be rebalanced when a node is added.
Initialization:
- We start by creating a basic distributed key-value store with a single node, then we add nodes and fresh data.
- Each node will be responsible for a range of hash values.
- Each key-value pair added to the store is hashed and directed to a node based on its hash.
Rebalancing:
- When a new node is added, the system will need to redistribute keys so that each node still handles roughly the same amount of data.
- We'll compute which keys need to move to the new node and then transfer them.
Hashing:
- We'll use a simple modulo-based hash partitioning for this example.
Let's get started:
import hashlib
class Node:
def __init__(self, node_id):
self.node_id = node_id
self.data = {}
def __repr__(self):
return f"Node({self.node_id})"
class KeyValueStore:
def __init__(self):
self.nodes = []
self.node_count = 0
def add_node(self):
new_node = Node(self.node_count)
self.nodes.append(new_node)
self.node_count += 1
self.rebalance()
def hash_key(self, key):
hashed = int(hashlib.md5(key.encode()).hexdigest(), 16)
return hashed % self.node_count
def insert(self, key, value):
node_idx = self.hash_key(key)
self.nodes[node_idx].data[key] = value
def add_data(self, grouping, count):
for i in range(count):
key= f"key{grouping}{i}"
node_idx = self.hash_key(key)
self.nodes[node_idx].data[key] = f"value{grouping}{i}"
def get(self, key):
node_idx = self.hash_key(key)
return self.nodes[node_idx].data.get(key, None)
def rebalance(self):
if self.node_count <= 1:
return
# Identify keys that need to move
for node in self.nodes[:-1]: # Exclude the last node (new one)
keys_to_move = [k for k in node.data if self.hash_key(k) != node.node_id]
for k in keys_to_move:
value = node.data.pop(k)
self.insert(k, value)
store = KeyValueStore()
# Inserting some data
store.add_node()
store.add_data("A", 15000)
print("\nStep 1: Data distribution with a single node:")
for node in store.nodes:
print(node, len(node.data))
# Adding a new node and triggering rebalancing
store.add_node()
# Display nodes and their data
print("\nStep 2: Data distribution with an added node:")
for node in store.nodes:
print(node, len(node.data))
# Inserting some data
store.add_node()
print("\nStep 3: Data distribution with an added node:")
for node in store.nodes:
print(node, len(node.data))
store.add_data("B", 5000)
# Display nodes and their data
print("\nStep 4: Data distribution with new data and an extra node added:")
for node in store.nodes:
print(node, len(node.data))
print("\nStep 5: Rebalancing with no changes")
store.rebalance()
for node in store.nodes:
print(node, len(node.data))
In this example:
This example is an oversimplification, and real-world data rebalancing would involve a lot more complexities, including considerations for data locality, fault tolerance, minimizing data transfers, and dealing with real-time data access needs. However, this example should provide a basic idea of what data rebalancing means in the context of distributed systems.
The output will look something like this:
Step 1: Data distribution with a single node:
Node(0) 15000
Step 2: Data distribution with an added node:
Node(0) 7555
Node(1) 7445
Step 3: Data distribution with an added node:
Node(0) 4998
Node(1) 4978
Node(2) 5024
Step 4: Data distribution with new data and an extra node added:
Node(0) 6628
Node(1) 6671
Node(2) 6701
Step 5: Rebalancing with no changes
Node(0) 6628
Node(1) 6671
Node(2) 6701
Note that when rebalancing, the distribution of the data across nodes is not perfectly equal, and we would expect a certain amount of variance. Also note that in step 5 no rebalancing takes place. Remember that rebalancing data on large distributed systems is expensive, so we need to only rebalance the distributed system when there is significant business benefit in doing so.
In conclusion, data rebalancing is a crucial aspect of maintaining the health, performance, and resilience of distributed systems in data engineering. Proper tools and strategies are needed to execute rebalancing efficiently and with minimal service impact.
Backpressure
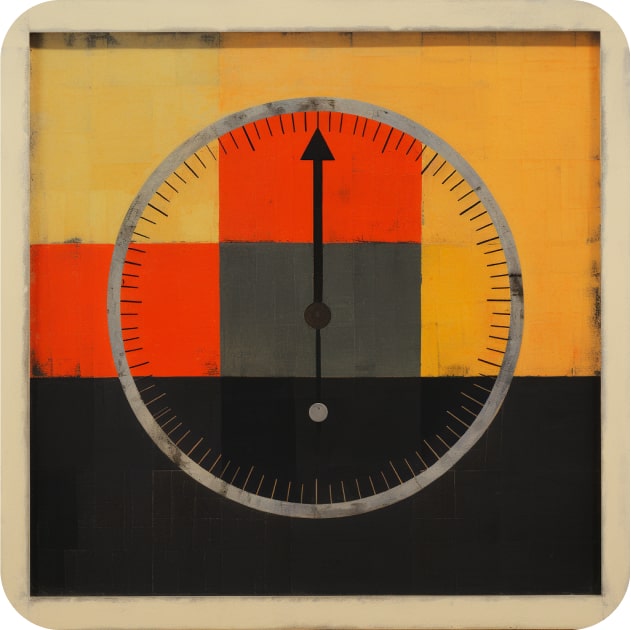
Compact
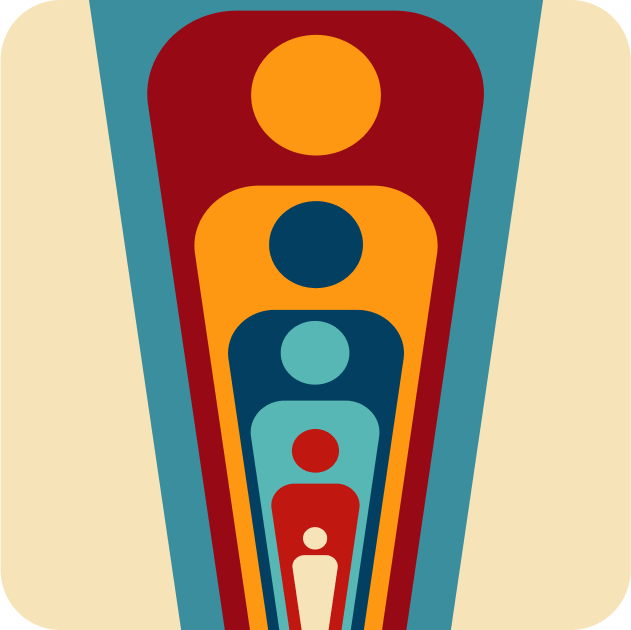
Compress
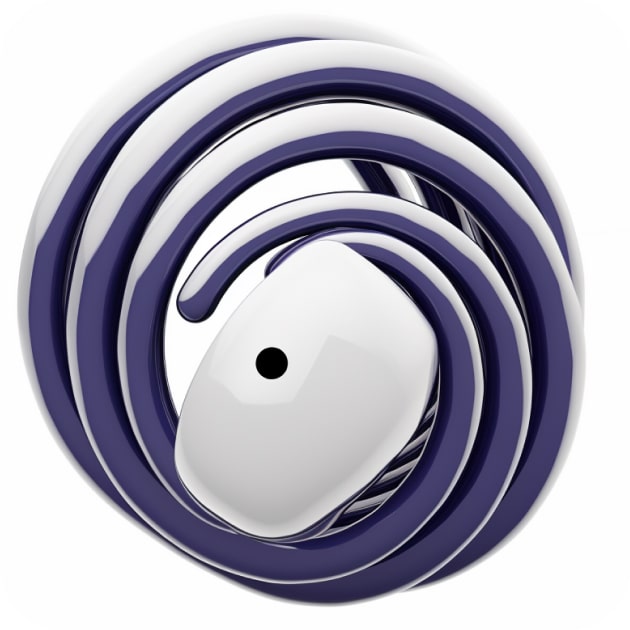
Ingest
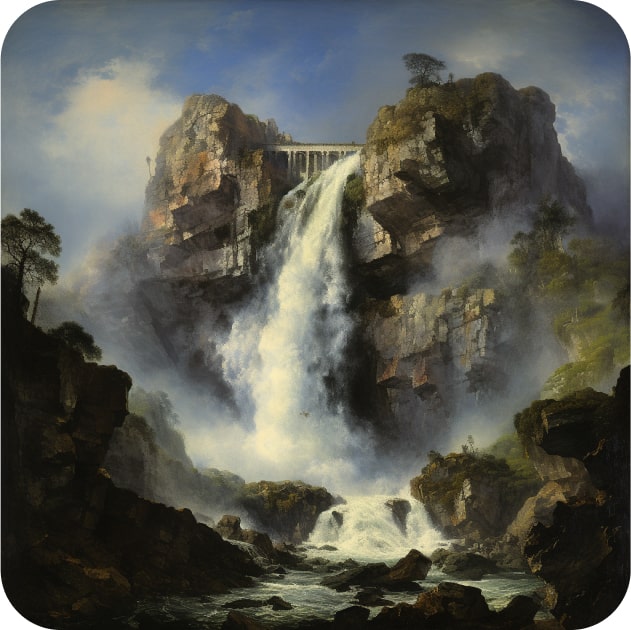
Load
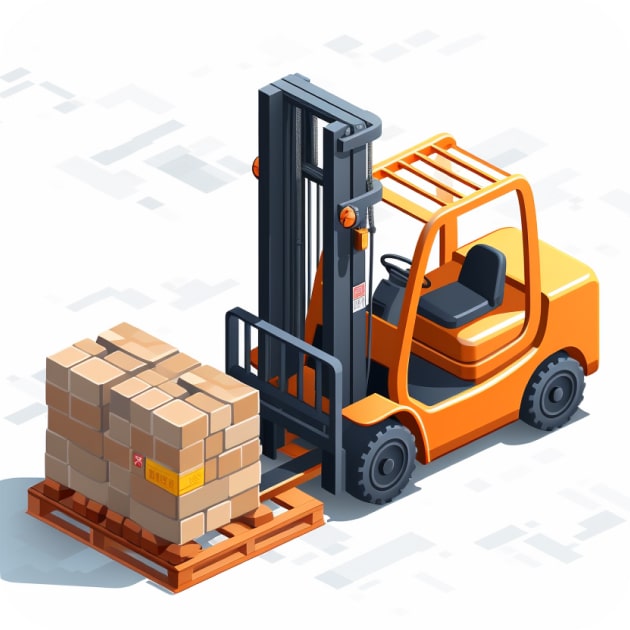
NoSQL
Pickle
Repartition
Scrape
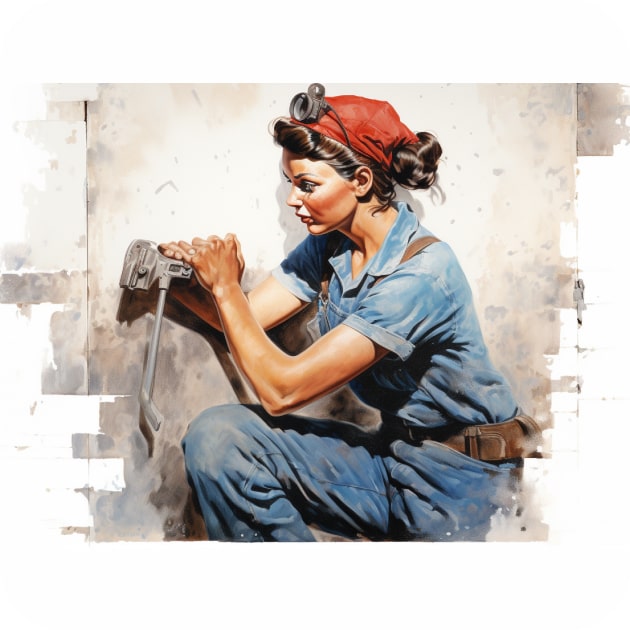
Shard
Shuffle
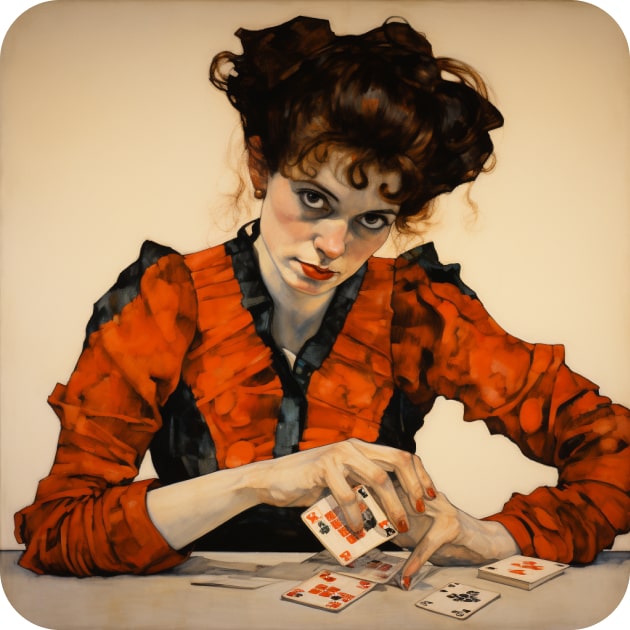
Spill
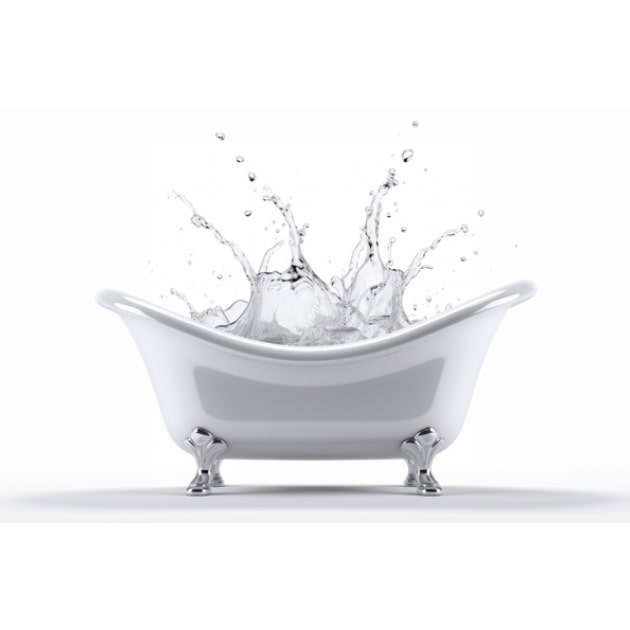
Stored Procedure
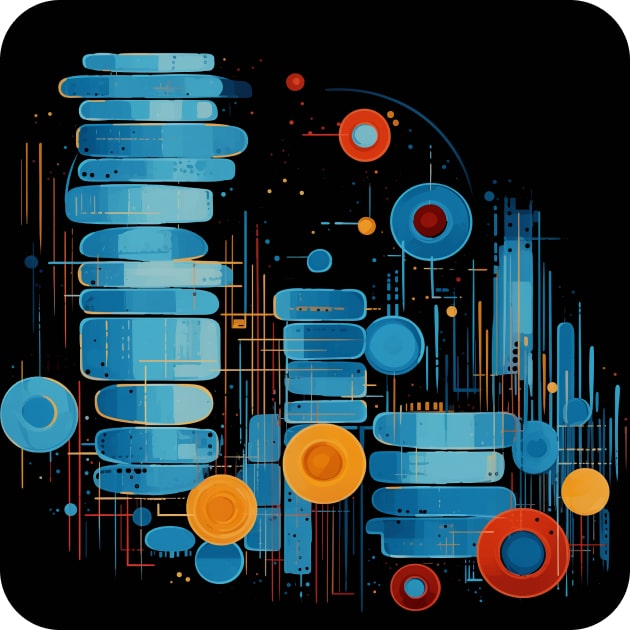
Upsert
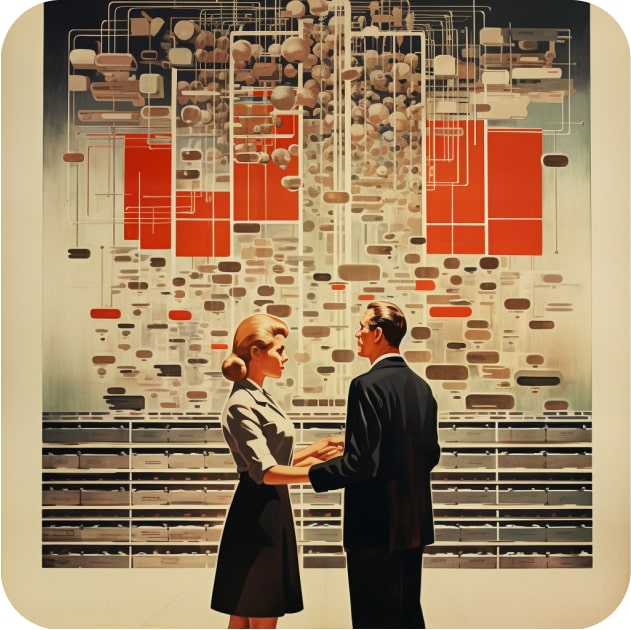