A definition of spilling to disk in data processing
"Spilling to disk" in the context of data engineering refers to a process where data that cannot fit entirely in a computer's memory (RAM) is temporarily written or stored onto the computer's hard disk or other storage media. This is typically done to prevent memory exhaustion and maintain the overall stability of a data processing system.
When working with large datasets or performing complex data transformations, there might be instances where the amount of data being processed exceeds the available memory. In such cases, the system needs a way to handle this excess data without causing errors or crashes. This is where spilling to disk comes into play.
Here's a simplified explanation of how spilling to disk works:
Memory Usage: When a data processing task begins, data is loaded into memory for faster access and processing. However, if the dataset is too large to fit entirely in memory, the system needs a strategy to handle this situation.
Spill to Disk: When the available memory is nearing its limit, the system will start moving excess data from memory to the hard disk. This data is written to temporary files on the disk. This frees up memory for new data to be processed without causing out-of-memory errors.
Processing: The data processing continues using the available memory. As new data is processed, it might again fill up the memory, leading to further spilling of excess data to disk as needed.
Disk I/O: Reading and writing data to and from the disk is generally slower compared to memory access. Therefore, while spilling to disk prevents memory exhaustion, it can also lead to a performance bottleneck due to the increased disk input/output (I/O) operations.
Disk Cleanup: Once the data processing task is complete, or when memory becomes more available, the system can clean up the temporary files on the disk. These temporary files are no longer needed, as the original data might already have been transformed or analyzed.
Spilling to disk is a mechanism that helps manage large datasets efficiently within the constraints of available memory. It's commonly used in data processing frameworks, database management systems, and other software tools where working with large volumes of data is a routine requirement. The goal is to strike a balance between utilizing memory for faster processing and leveraging disk storage to handle data that cannot fit in memory at once.
An example of spilling to disk using Python
Like many self-contained examples, this one is a bit contrived, but we hope it helps illustrate the concept of "spilling to disk"".
The following Python code uses a hypothetical scenario of processing a large dataset in chunks. In this example, we'll generate random numbers as our dataset and simulate the spilling process by writing chunks of data to temporary files on the disk.
import os
import tempfile
# Parameters
total_data_points = 1000
memory_capacity = 100 # Simulated memory capacity
chunk_size = 50 # Size of each chunk to process
# Generate random data points
data = [i for i in range(total_data_points)]
# Simulated memory
memory = []
# Simulated spilling process
def process_chunk(chunk):
# Simulated data processing
processed_chunk = [x * 2 for x in chunk]
return processed_chunk
# Process the data in chunks
for i in range(0, total_data_points, chunk_size):
chunk = data[i:i+chunk_size]
# Check if chunk fits in memory
if len(memory) + len(chunk) > memory_capacity:
# Spill the excess data to a temporary file on disk
with tempfile.NamedTemporaryFile(delete=False) as temp_file:
temp_file.write(bytes(str(process_chunk(memory)), 'utf-8'))
spilled_filename = temp_file.name
print(f"Spilled {len(memory)} data points to disk: {spilled_filename}")
# Reset memory for the next chunk
memory = []
# Process the chunk in memory
processed_chunk = process_chunk(chunk)
memory.extend(processed_chunk)
# Process any remaining data in memory
if memory:
processed_remaining_data = process_chunk(memory)
print("Processed remaining data:", processed_remaining_data)
# Clean up spilled files (in a real scenario, you would handle this more robustly)
if spilled_filename:
os.remove(spilled_filename)
print("Processing complete!")
The output you generate form the above code will look something like:
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmpi195o90z
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmpippjkr0p
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmpq9xdjpdm
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmptnoway00
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmp13_tivh3
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmpsuizyjzx
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmpus6utws7
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmpyliozzaz
Spilled 100 data points to disk: /var/folders/yz/6a7b9cdef74h3ij9kl1mnopq40000rs/T/tmpn0y68wqy
Processed remaining data: [3600, 3604, 3608, 3612, 3616, 3620, 3624, 3628, 3632, 3636, 3640, 3644, 3648, 3652, 3656, 3660, 3664, 3668, 3672, 3676, 3680, 3684, 3688, 3692, 3696, 3700, 3704, 3708, 3712, 3716, 3720, 3724, 3728, 3732, 3736, 3740, 3744, 3748, 3752, 3756, 3760, 3764, 3768, 3772, 3776, 3780, 3784, 3788, 3792, 3796, 3800, 3804, 3808, 3812, 3816, 3820, 3824, 3828, 3832, 3836, 3840, 3844, 3848, 3852, 3856, 3860, 3864, 3868, 3872, 3876, 3880, 3884, 3888, 3892, 3896, 3900, 3904, 3908, 3912, 3916, 3920, 3924, 3928, 3932, 3936, 3940, 3944, 3948, 3952, 3956, 3960, 3964, 3968, 3972, 3976, 3980, 3984, 3988, 3992, 3996]
Processing complete!
Please note that this code is a simplified example for illustration purposes and is not recommended for actual production scenarios. In a real-world setting, you would likely use libraries like pandas
or other data processing frameworks to handle large datasets more efficiently.
Backpressure
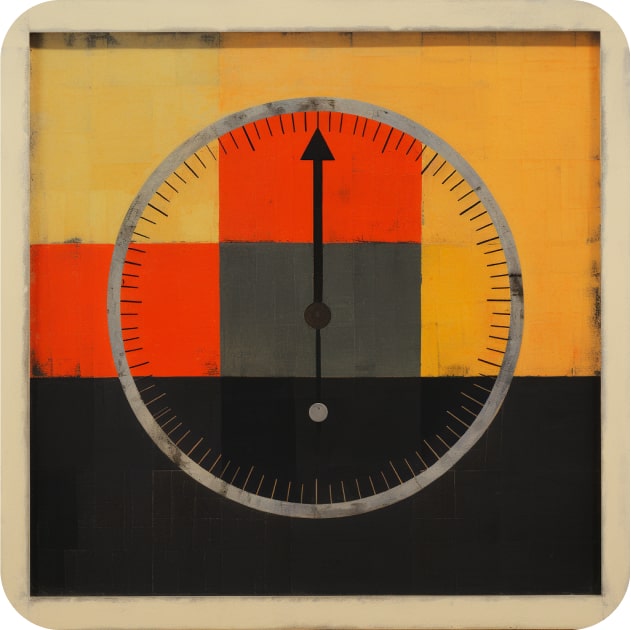
Compact
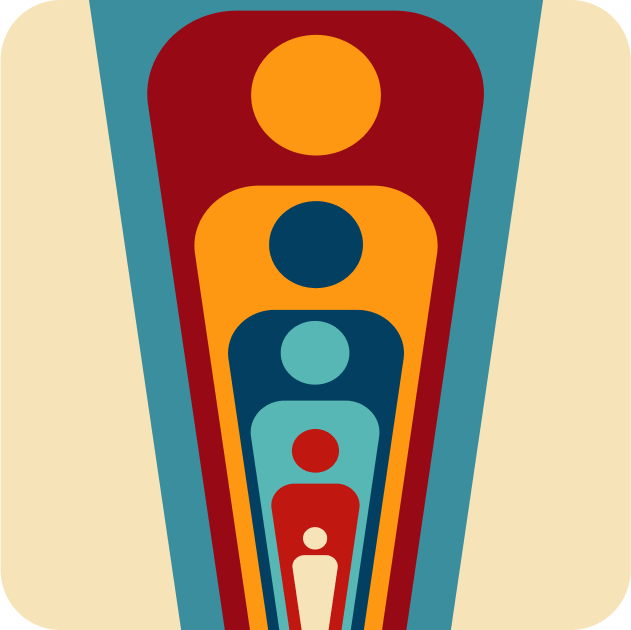
Compress
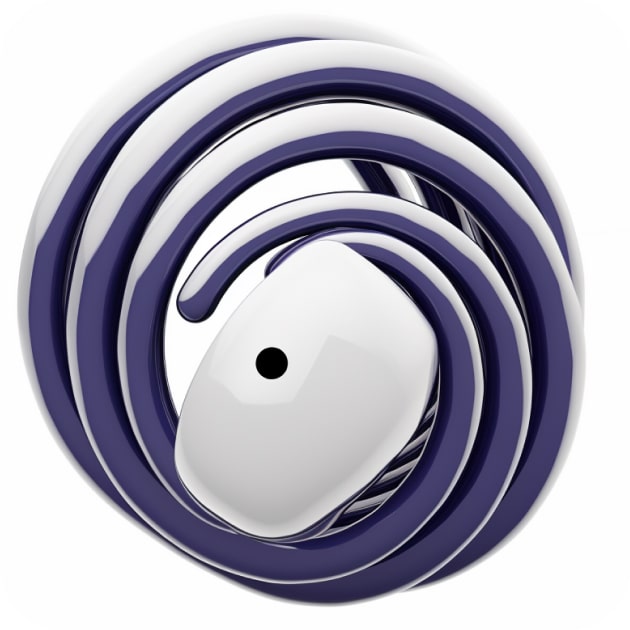
Ingest
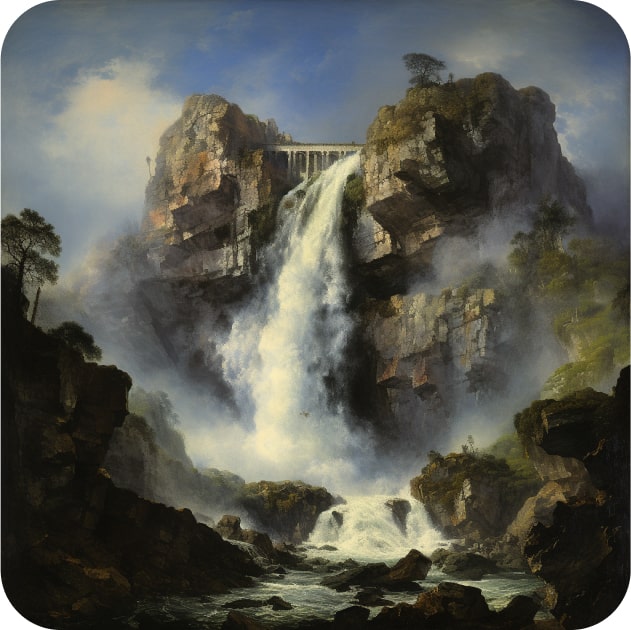
Load
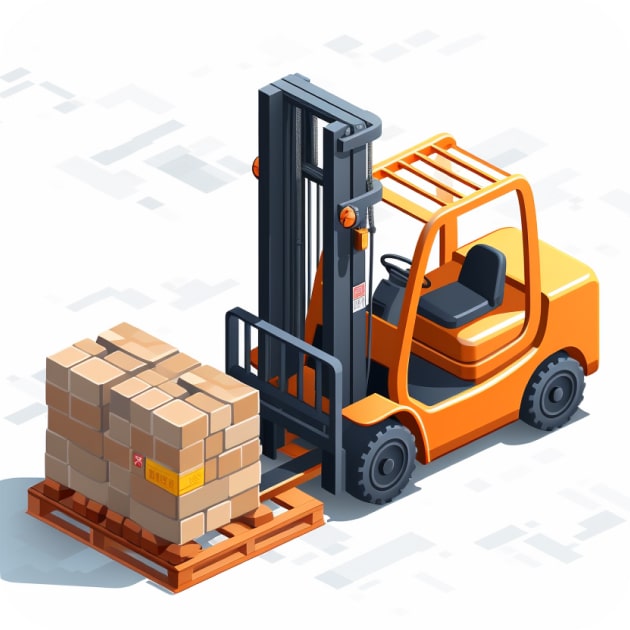
NoSQL
Pickle
Rebalance
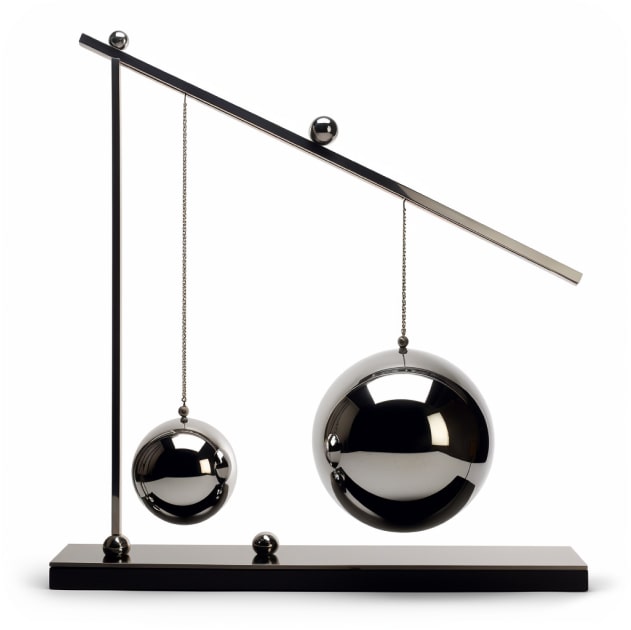
Repartition
Scrape
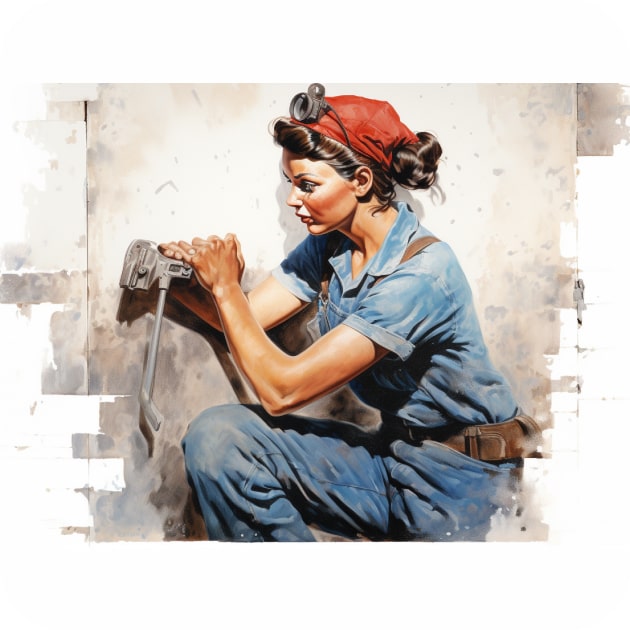
Shard
Shuffle
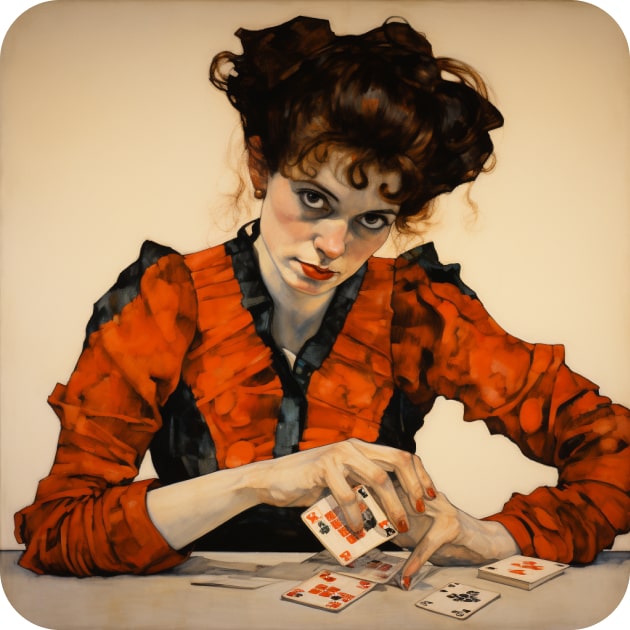
Stored Procedure
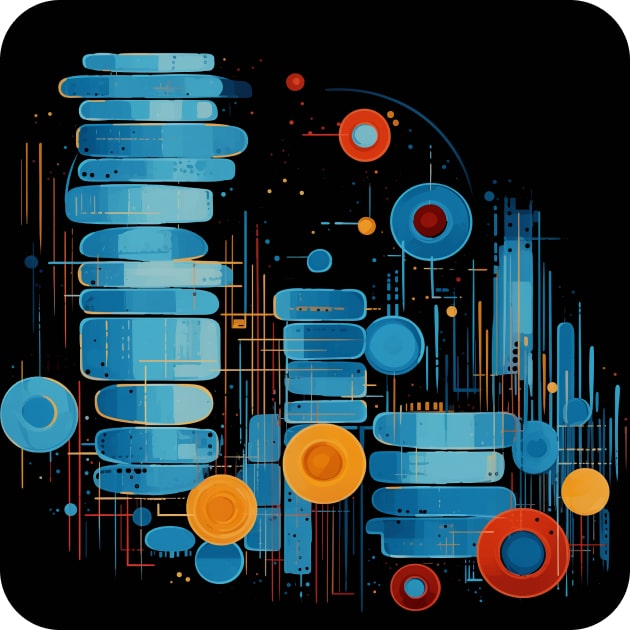
Upsert
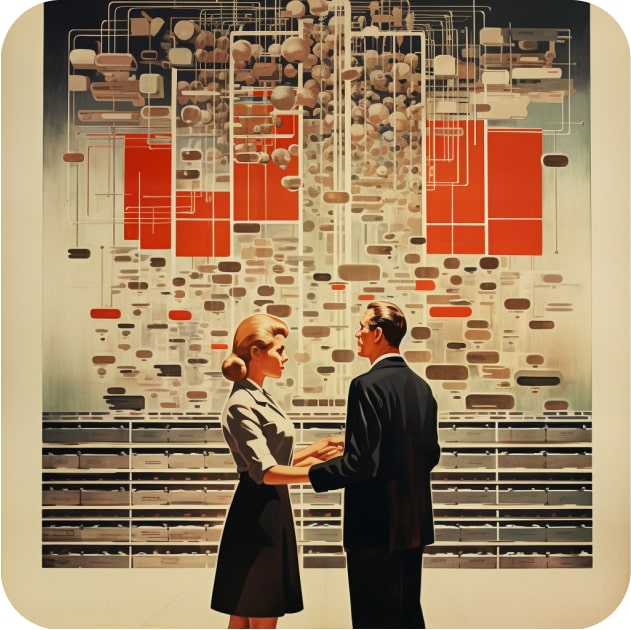