Data replication definition:
Data replication in the context of modern data pipelines involves creating multiple copies of data and distributing them across multiple nodes or clusters to ensure high availability and fault tolerance. This is typically done to minimize the risk of data loss due to hardware failure or other issues.
Data replication example using Python:
Please note that you need to have the necessary Python libraries installed in your Python environment to run this code.
In Python, data replication can be achieved using various distributed computing frameworks such as Apache Hadoop, Apache Spark, or Dask. These frameworks provide mechanisms for replicating data across multiple nodes or clusters.
For example, using Apache Spark, we can create a replicated RDD (Resilient Distributed Dataset) by calling the `repartition()`` function with a desired number of partitions:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("Data Replication Example").getOrCreate()
# Load data from a CSV file
data = spark.read.csv("data.csv", header=True, inferSchema=True)
# Replicate the data across two nodes
replicated_data = data.repartition(2)
# Do some processing on the replicated data
result = replicated_data.groupBy("column1").count()
# Save the result to a CSV file
result.write.csv("replicate_result", mode="overwrite", header=True)
In this example, we load data from a CSV file using Spark and then replicate the data across two nodes using the repartition()
function. We then perform some processing on the replicated data and save the result to a CSV file in the folder replicate_result
. The replicated data ensures high availability and fault tolerance in case of hardware failure or other issues.
Append
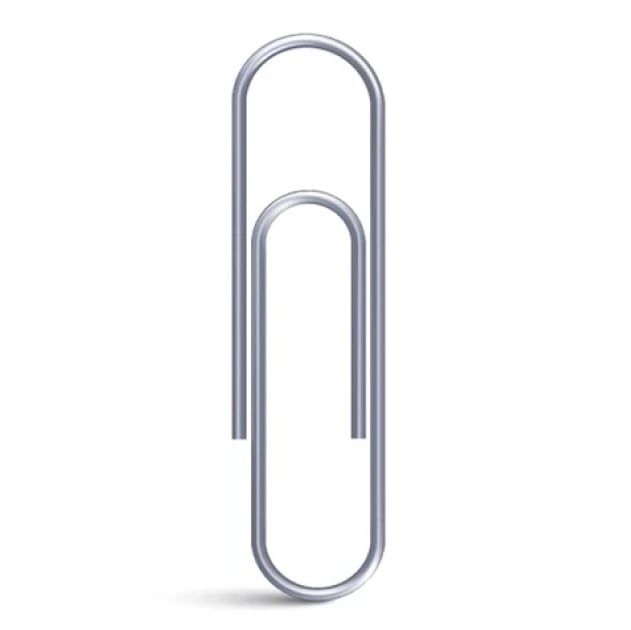
Archive
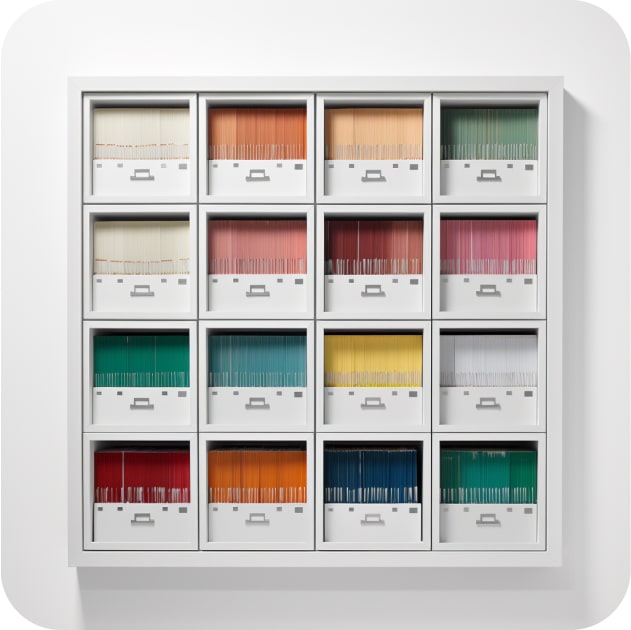
Augment
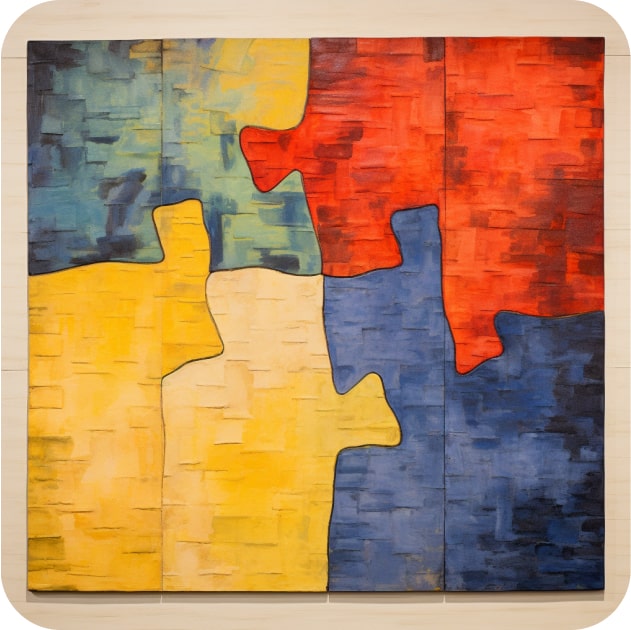
Auto-materialize
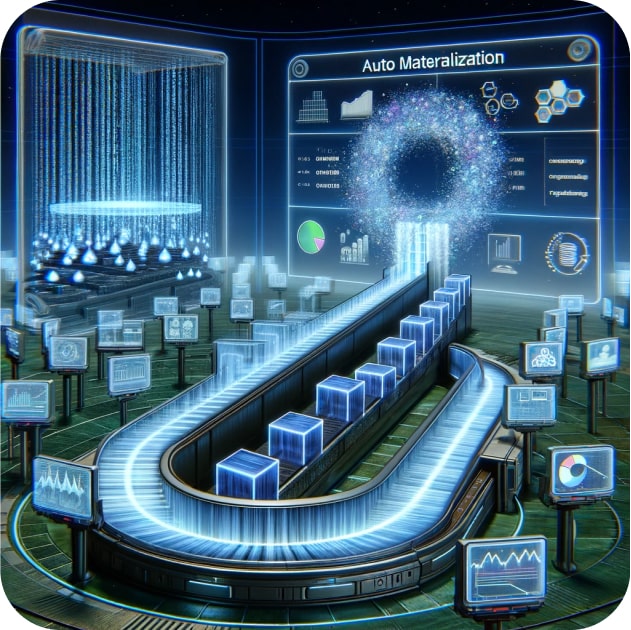
Backup
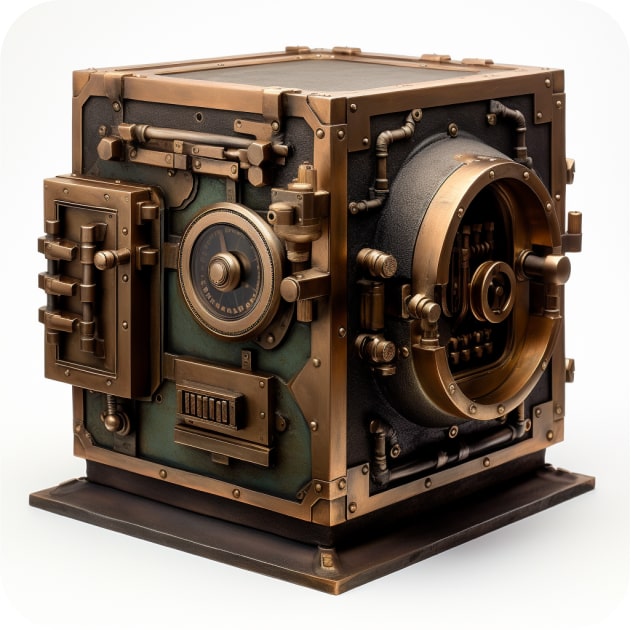
Batch Processing
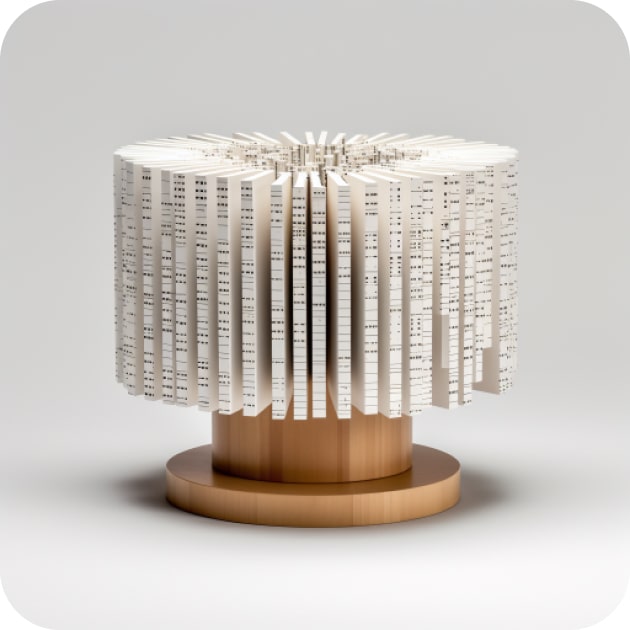
Cache
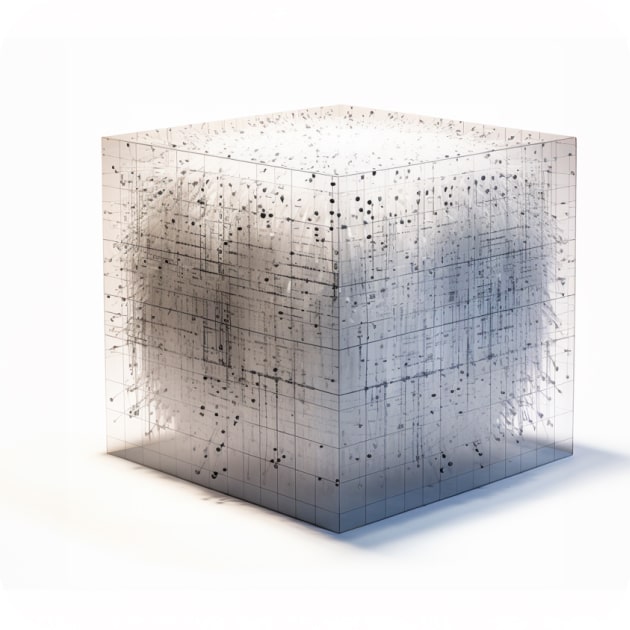
Categorize
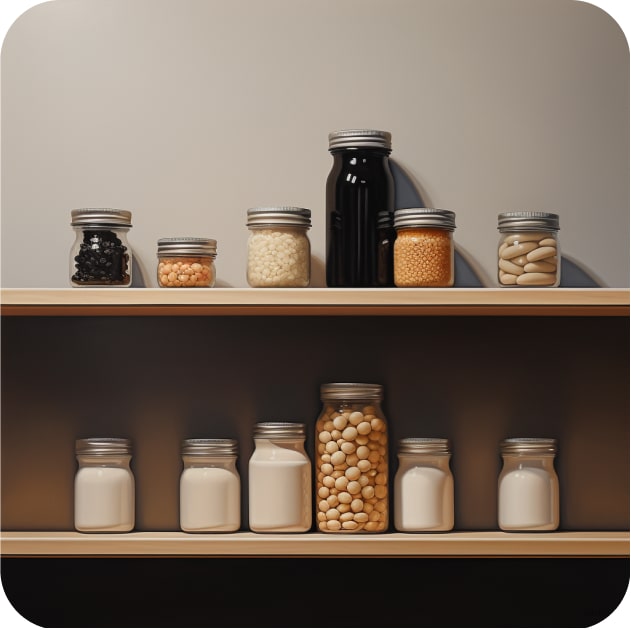
Checkpointing
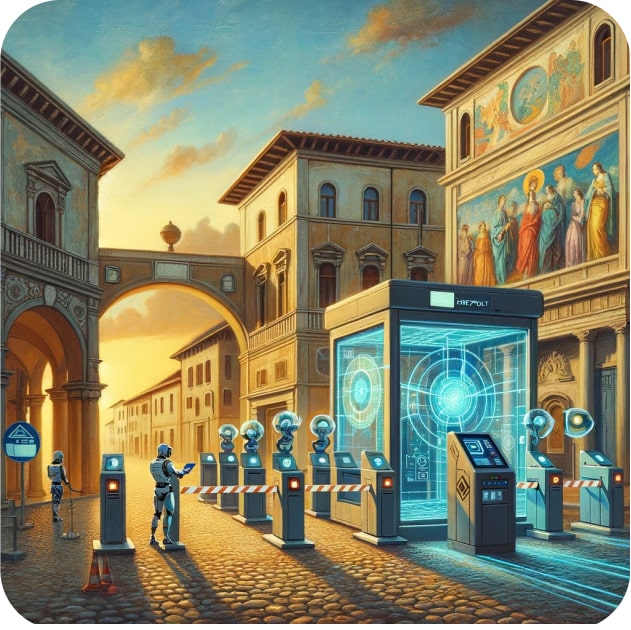
Deduplicate
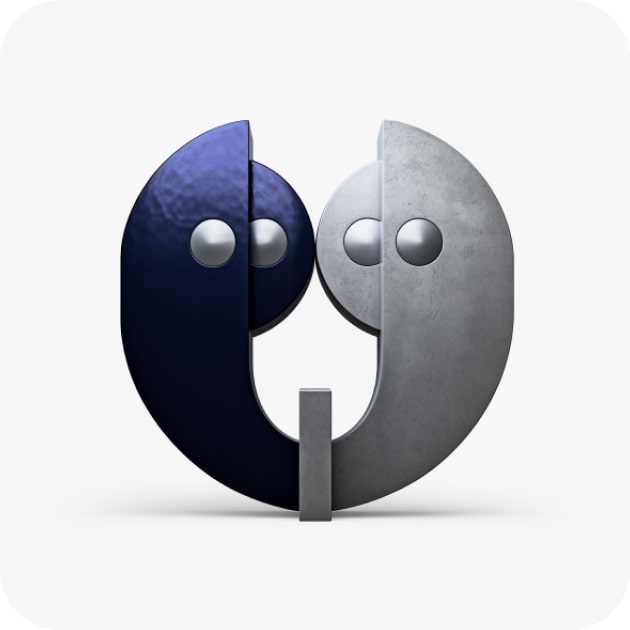
Deserialize
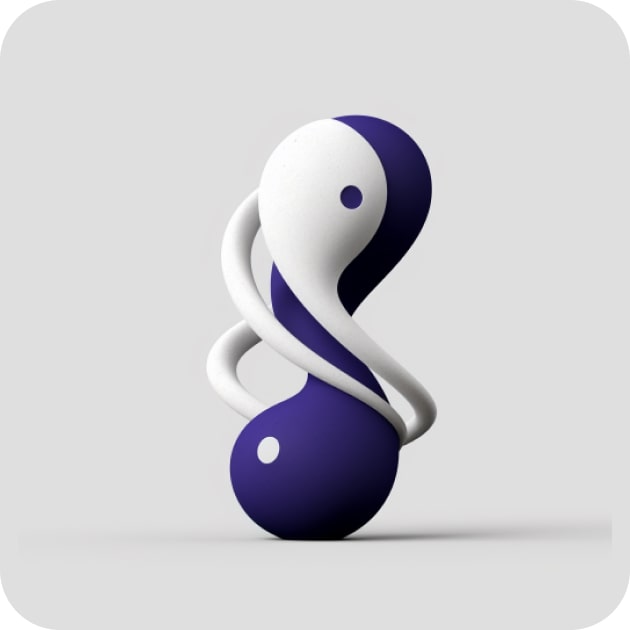
Dimensionality
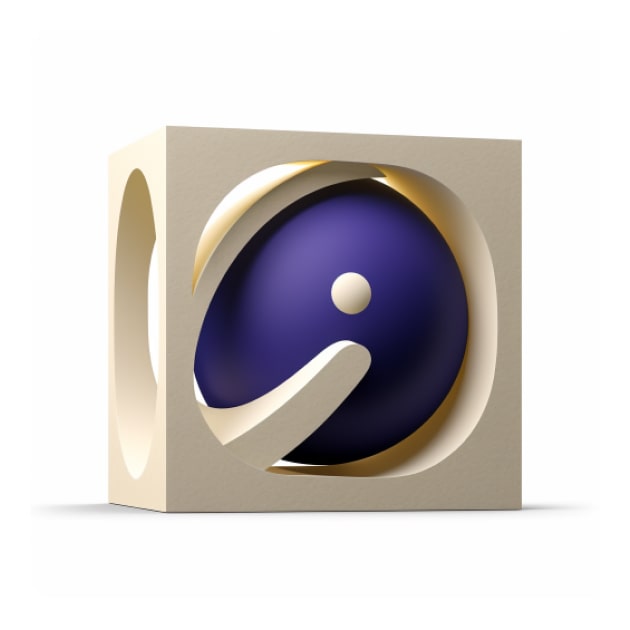
Encapsulate
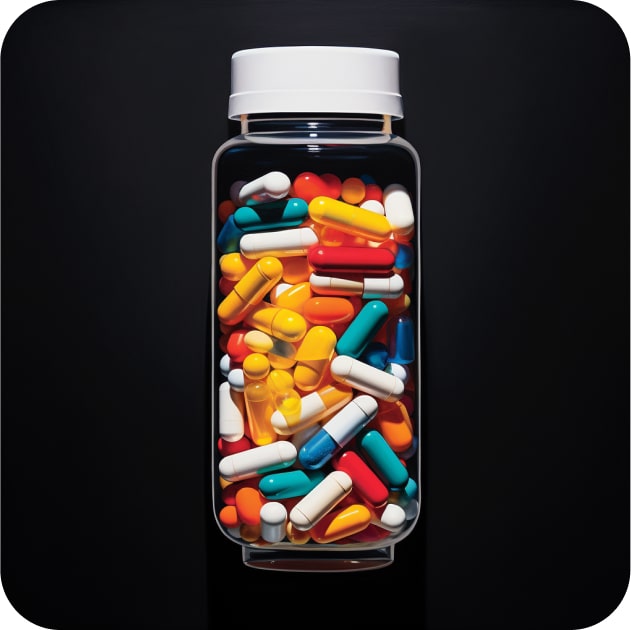
Enrich
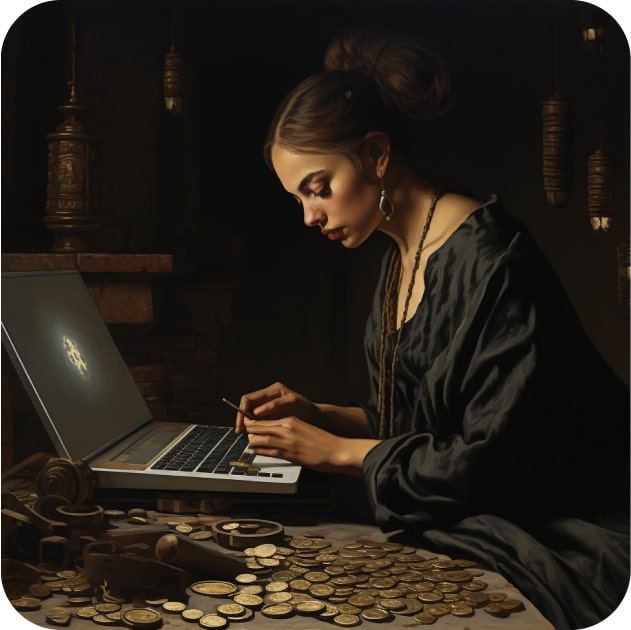
Export
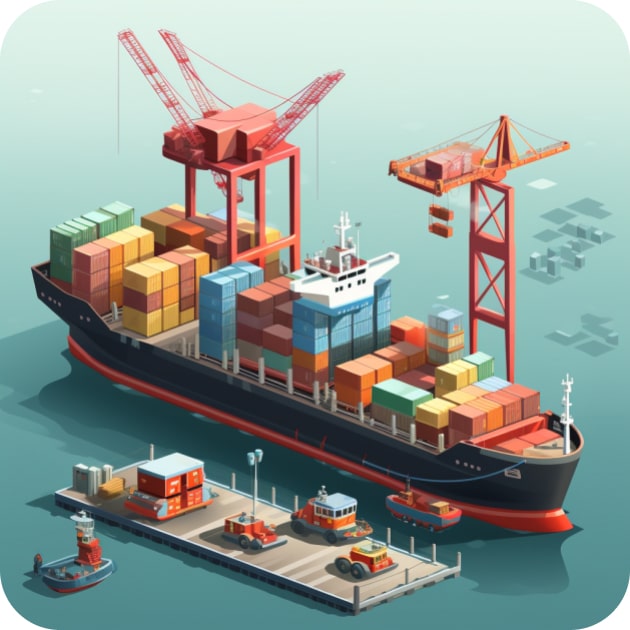
Graph Theory
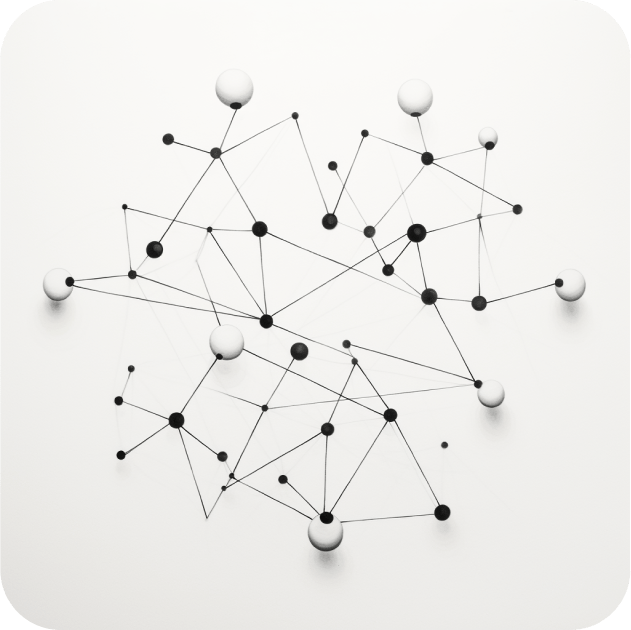
Idempotent
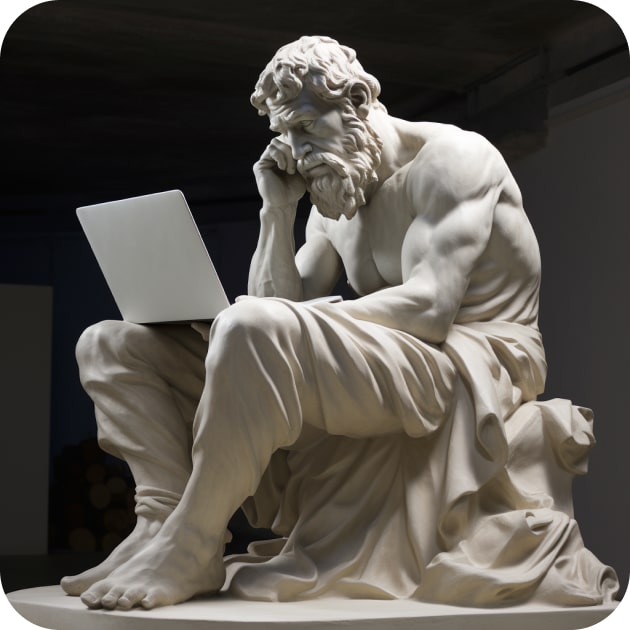
Index
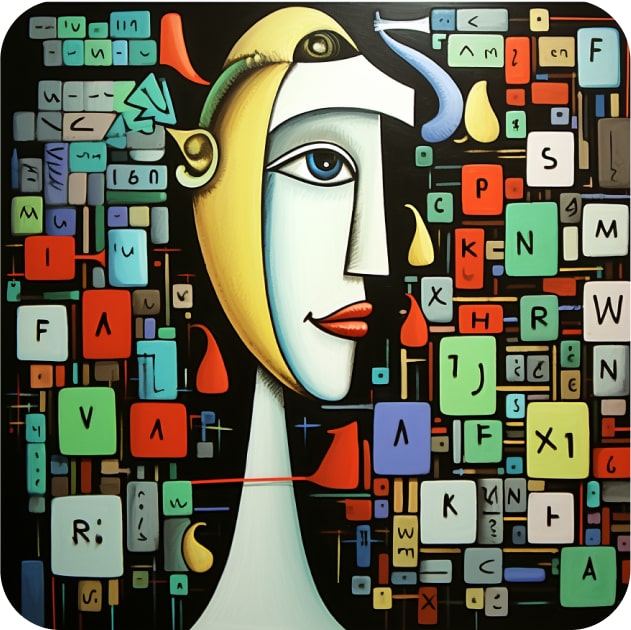
Integrate
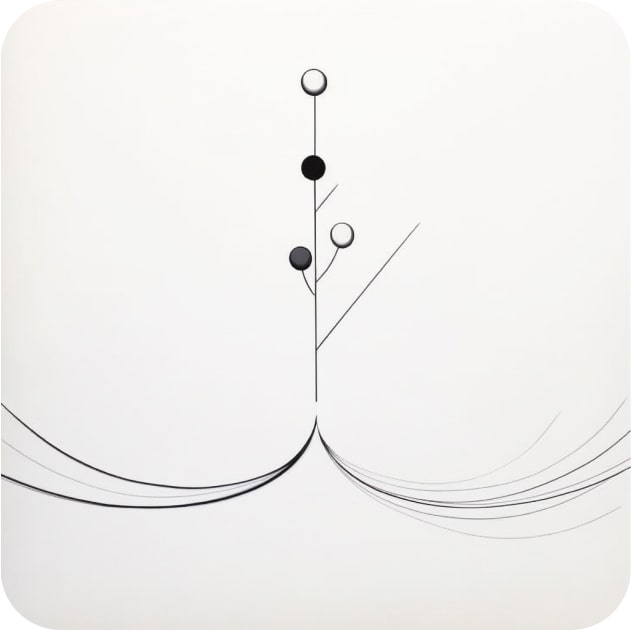
Lineage
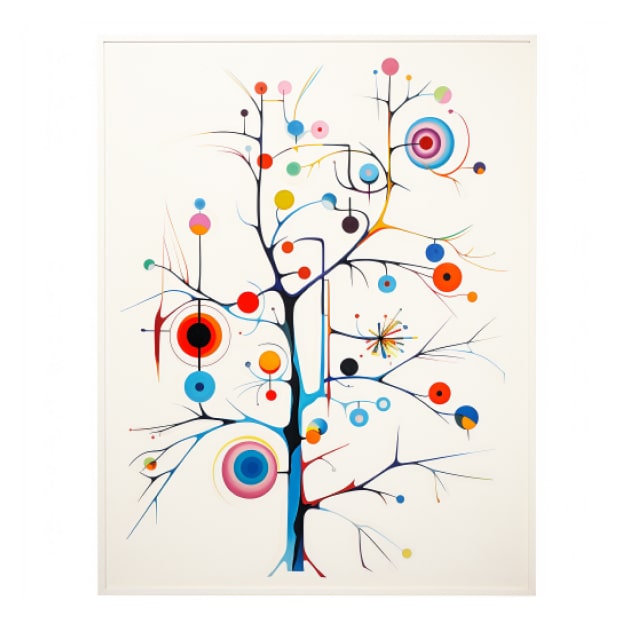
Linearizability
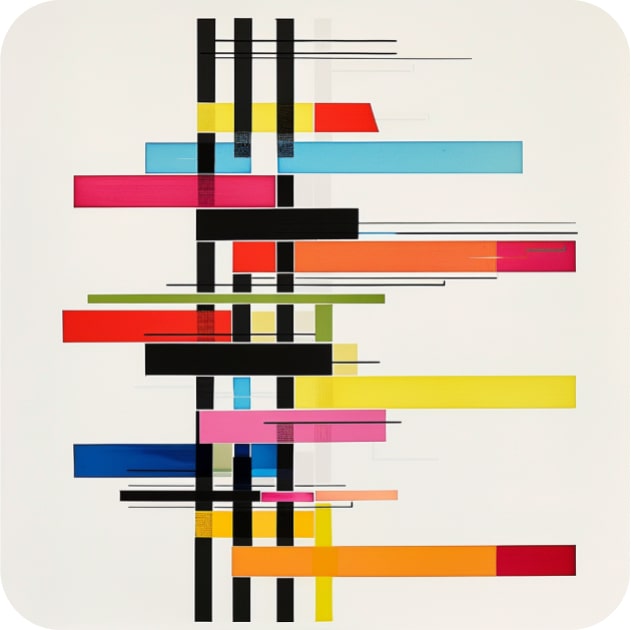
Materialize
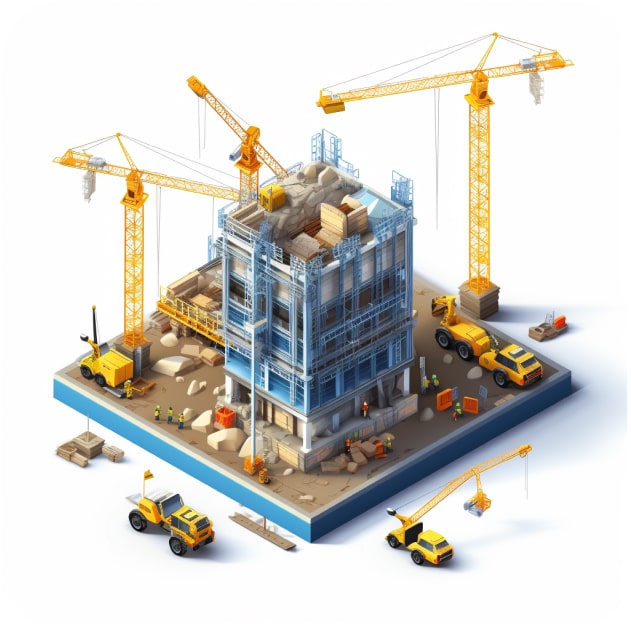
Memoize
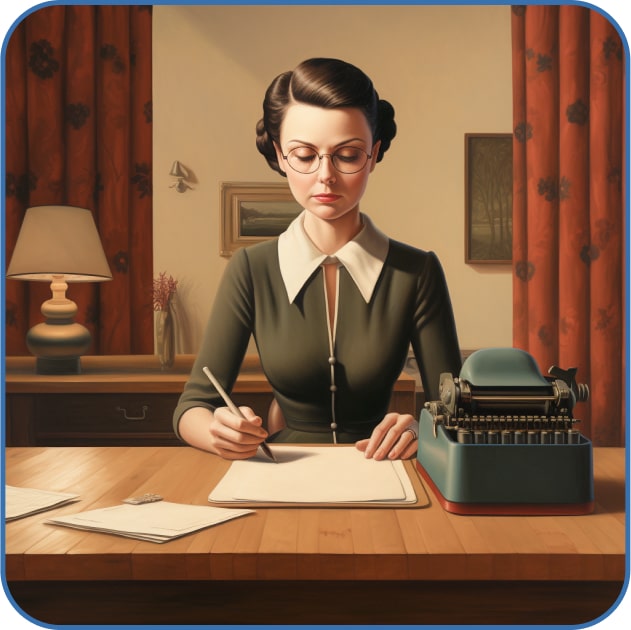
Merge
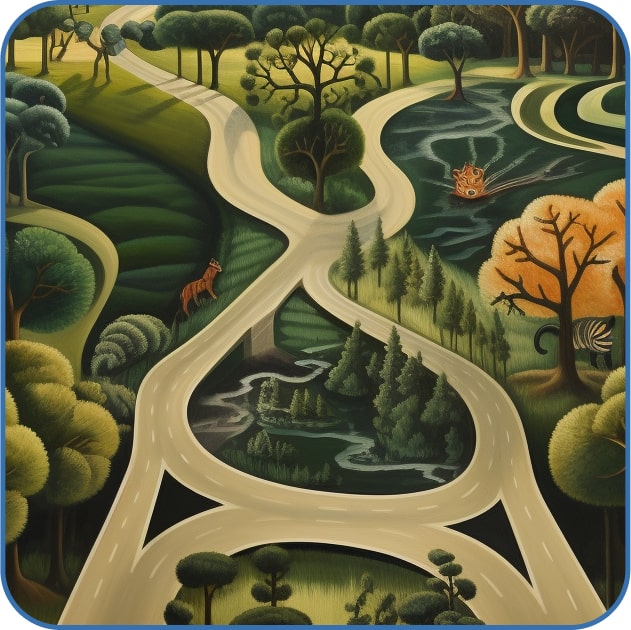
Model
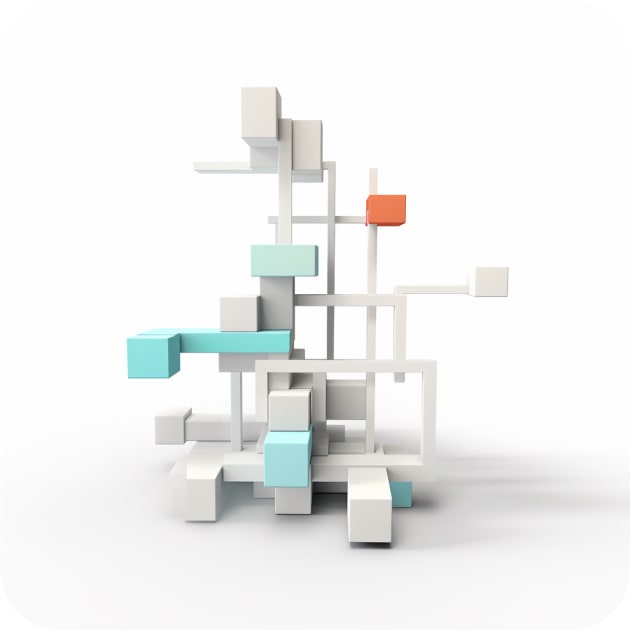
Monitor
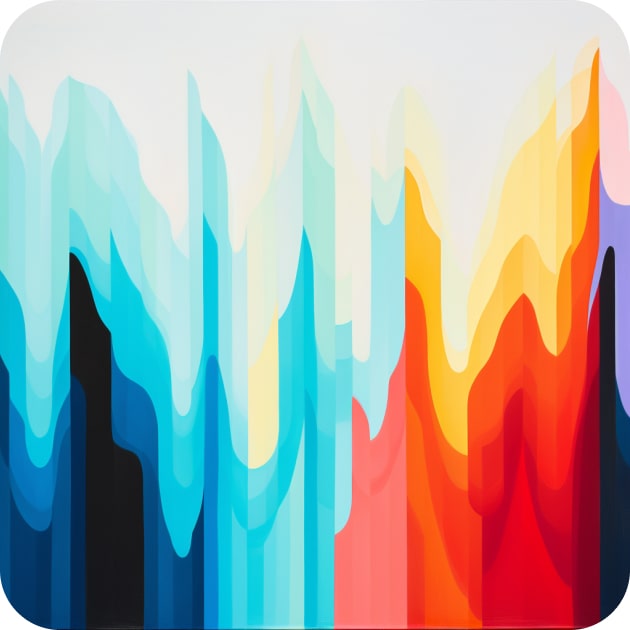
Named Entity Recognition
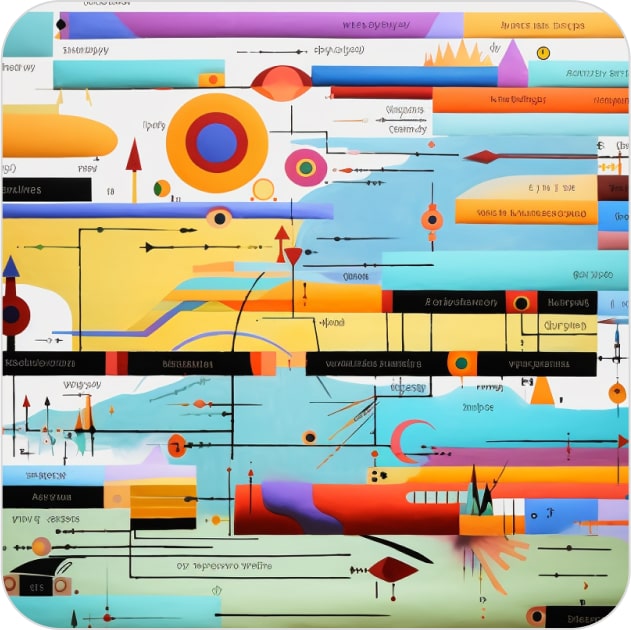
Parse
Partition
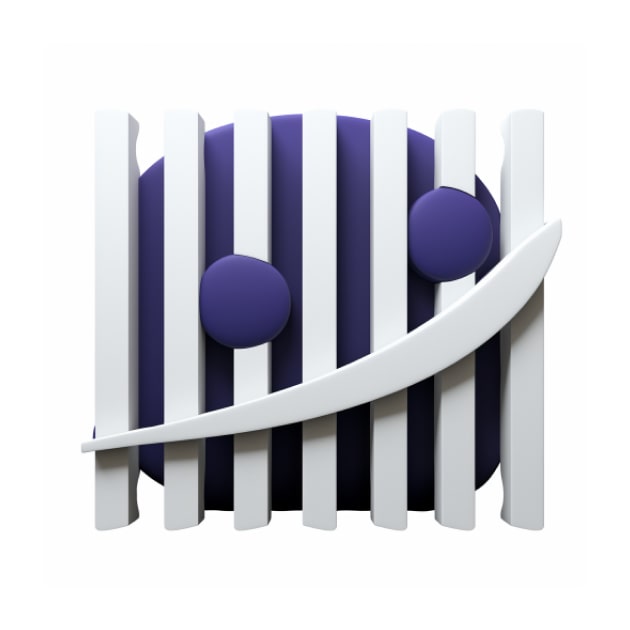
Prep
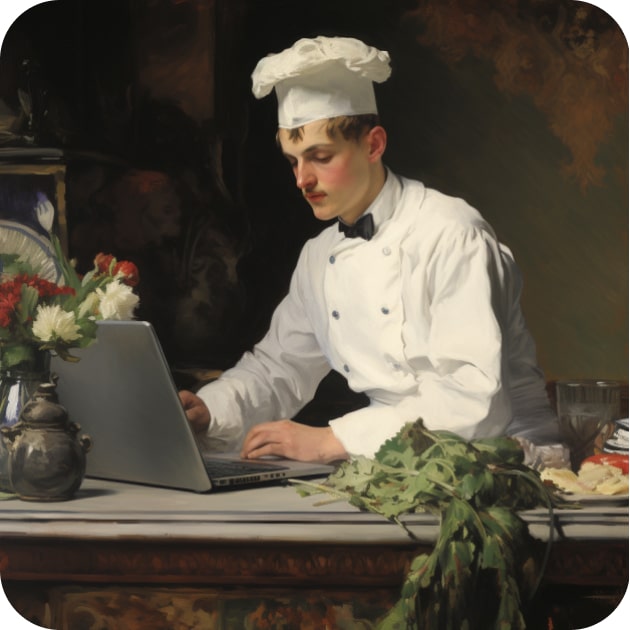
Preprocess
Primary Key
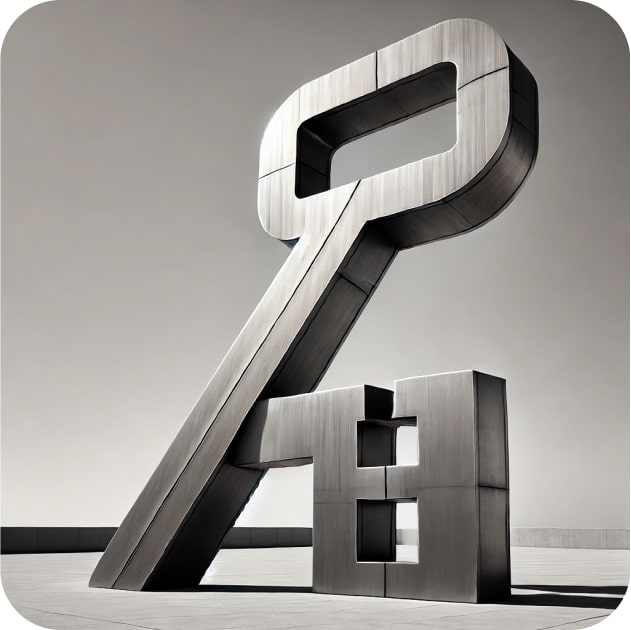
Scaling
Schema Inference
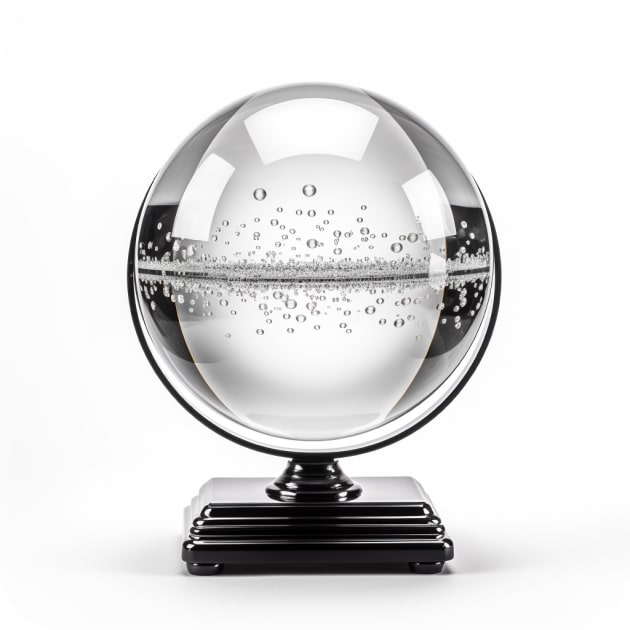
Schema Mapping
Secondary Index
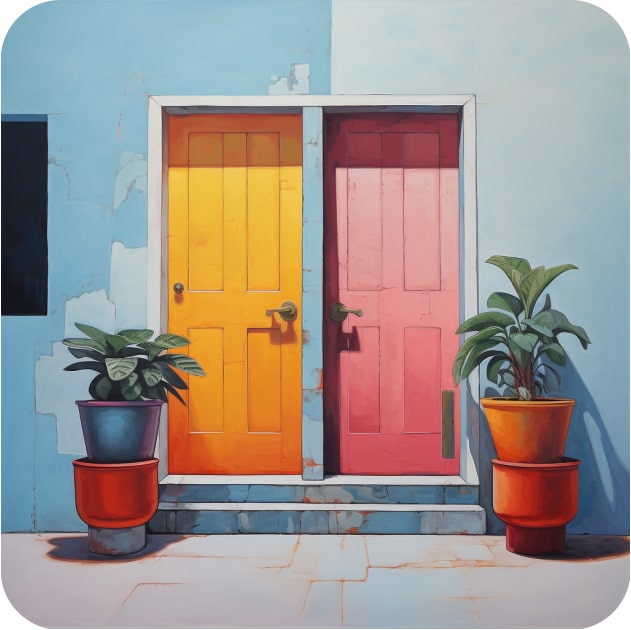
Software-defined Asset
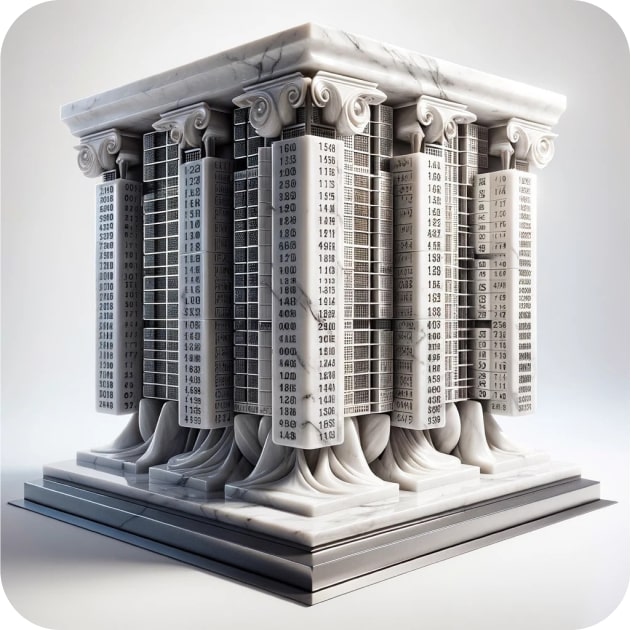
Synchronize
Validate
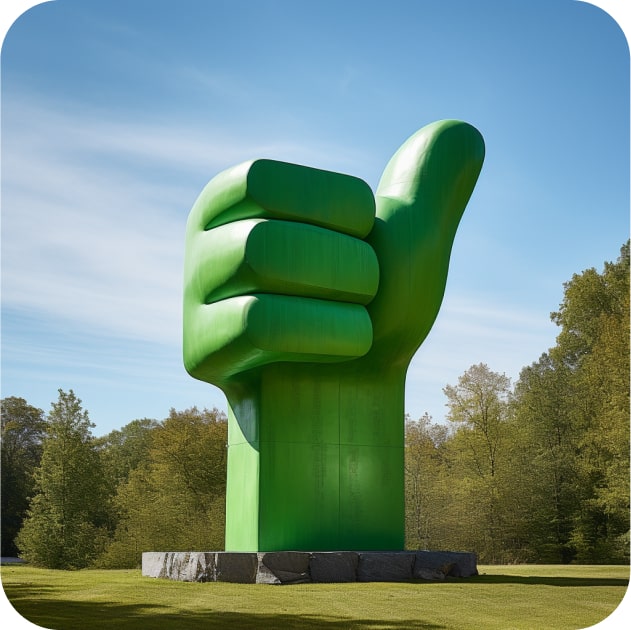
Version
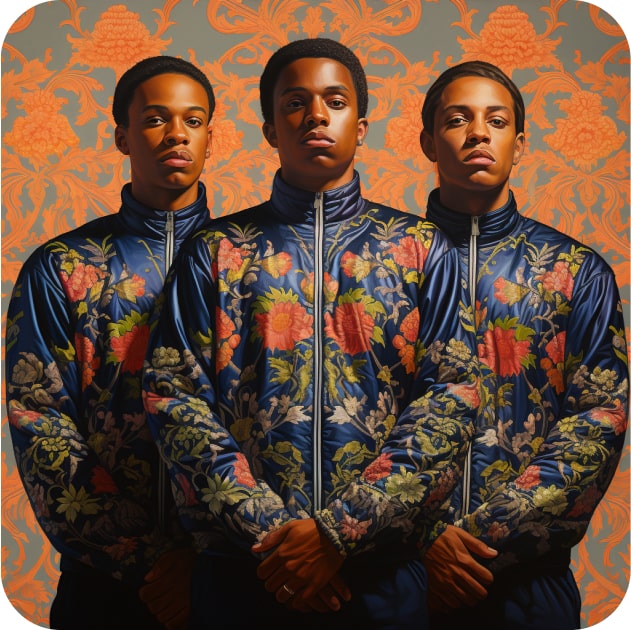