Data Tokenizing
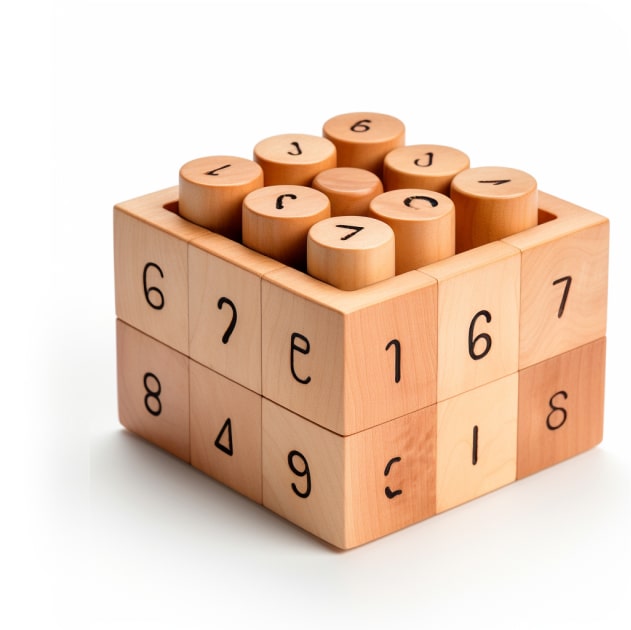
Tokenization definition:
Tokenization is the process of breaking down a piece of text into individual words or tokens. This is a common technique used in data engineering to prepare text data for analysis.
Tokenizing example using Python:
Here are some practical examples of tokenization in data engineering using Python-specific functions. Please note that you need to have the necessary Python libraries installed in your Python environment to run this code:
Using the split()
function: The split() function can be used to split a string into a list of words based on a delimiter, such as a space or a comma.
For example:
text = "This is a sample sentence."
tokens = text.split()
print(tokens)
This would output:
['This', 'is', 'a', 'sample', 'sentence.']
Using the word_tokenize() function from the NLTK library: The Natural Language Toolkit (NLTK) is a popular Python library for natural language processing. The word_tokenize() function from the NLTK library can be used to tokenize text data into individual words. For example:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
text = "This is a sample sentence."
tokens = word_tokenize(text)
print(tokens)
Will output:
['This', 'is', 'a', 'sample', 'sentence', '.']
Using regular expressions: Regular expressions can be used to define patterns for tokenizing text data. For example, the following code uses regular expressions to split a string into words based on whitespace and punctuation:
import re
text = "This is a sample sentence."
tokens = re.findall(r'\b\w+\b', text)
print(tokens)
This code would produce the following output in the terminal:
['This', 'is', 'a', 'sample', 'sentence']
Using the split()
function with a regular expression pattern: The split()
function can also be used with regular expression patterns to tokenize text data. For example:
import re
text = "This is a sample sentence."
tokens = re.split('\W+', text)
print(tokens)
This will yield:
['This', 'is', 'a', 'sample', 'sentence', '']
These are just a few examples of how tokenization can be used to prepare data for analysis and extract insights from text.
Align
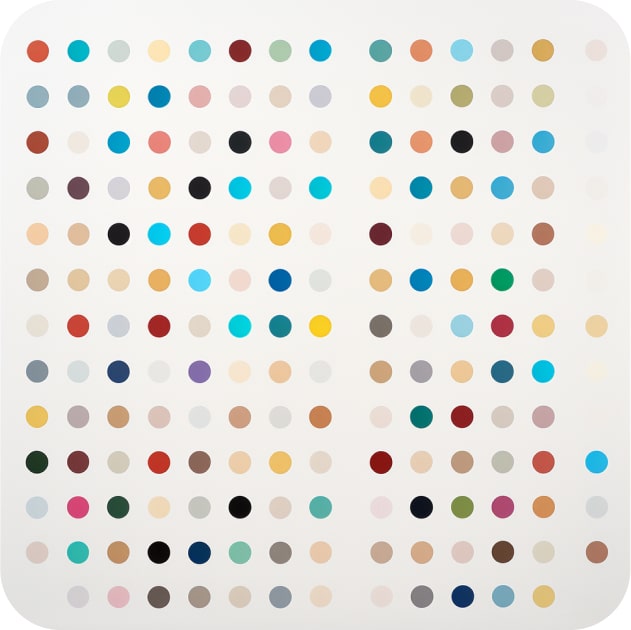
Clean or Cleanse
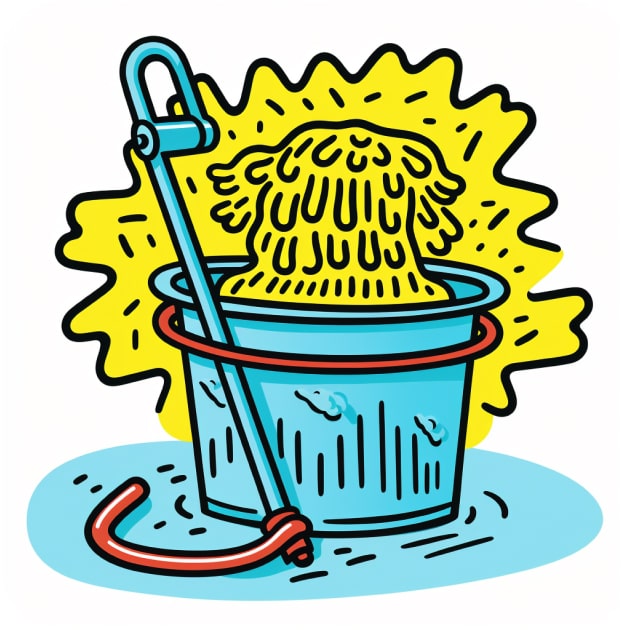
Cluster
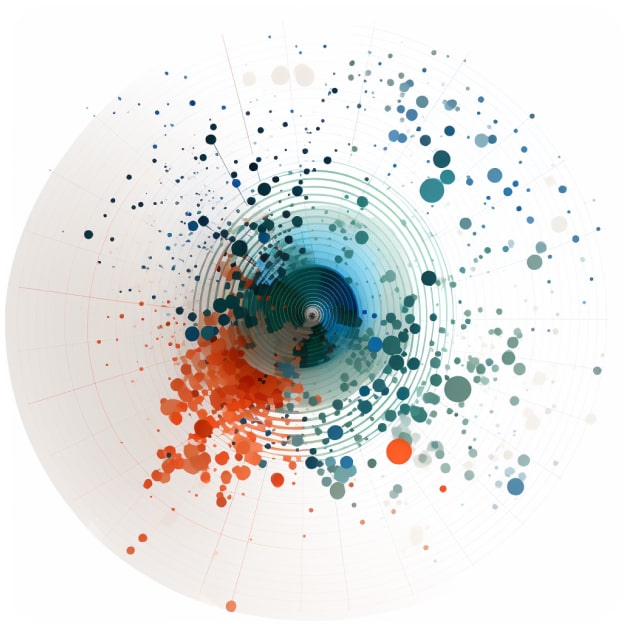
Curate
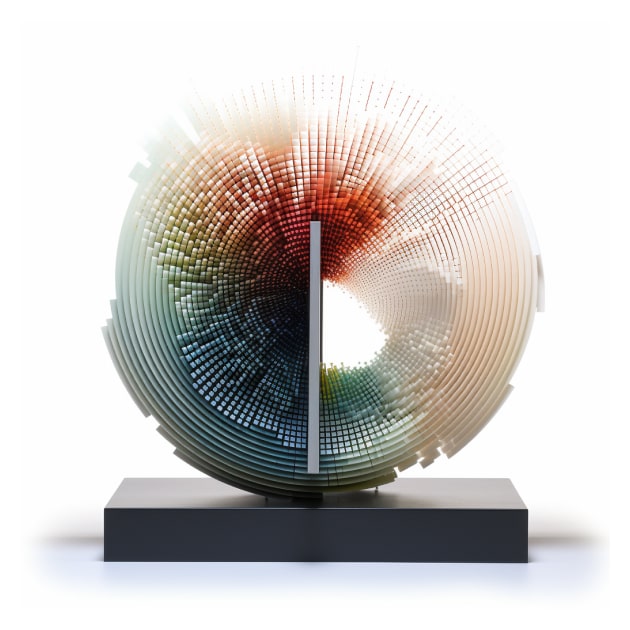
Denoise
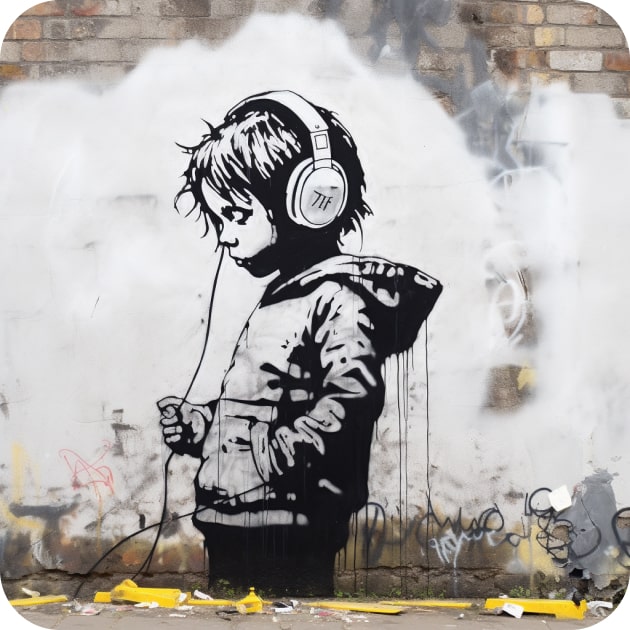
Denormalize
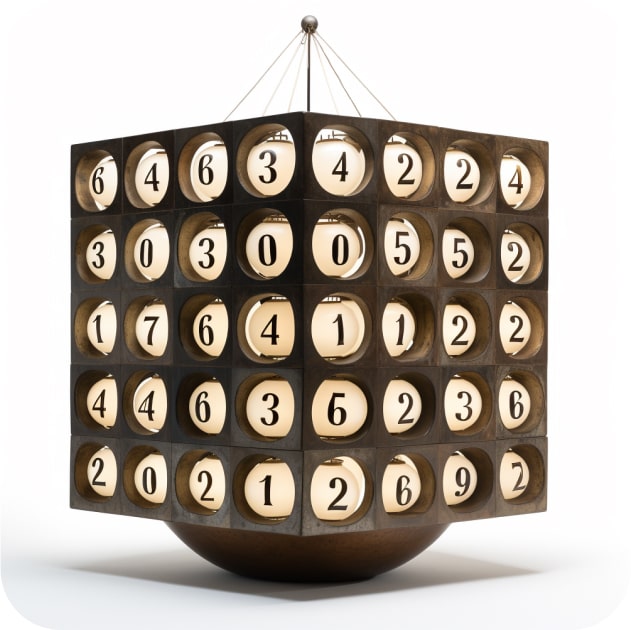
Derive
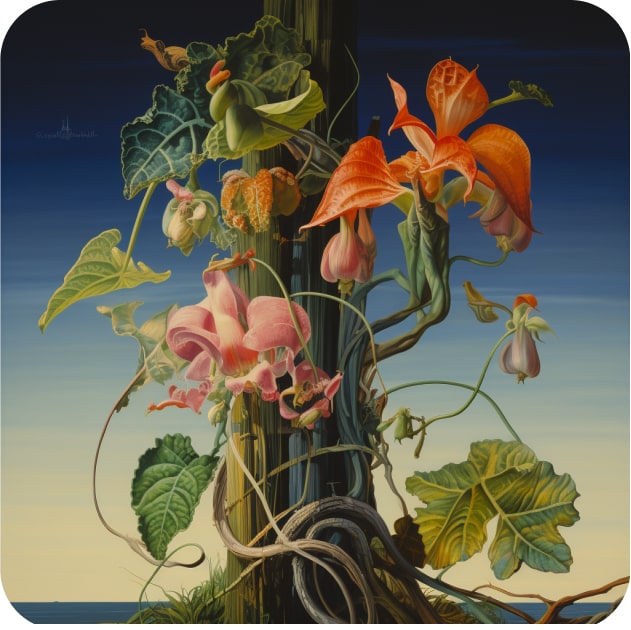
Discretize
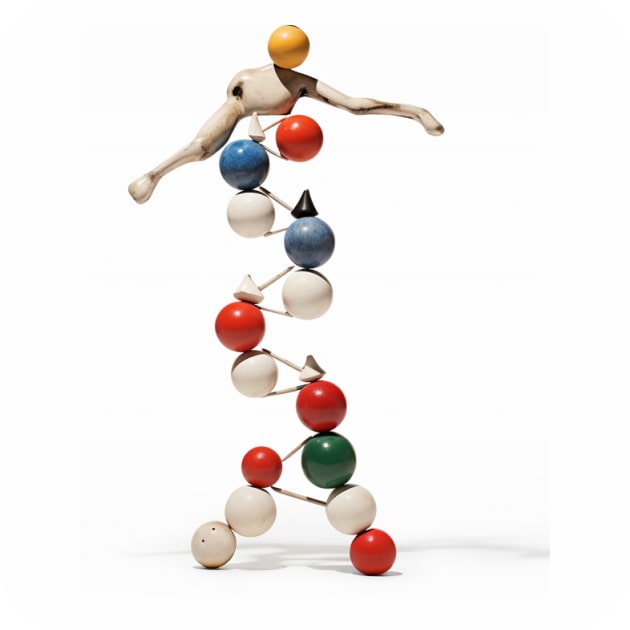
ETL
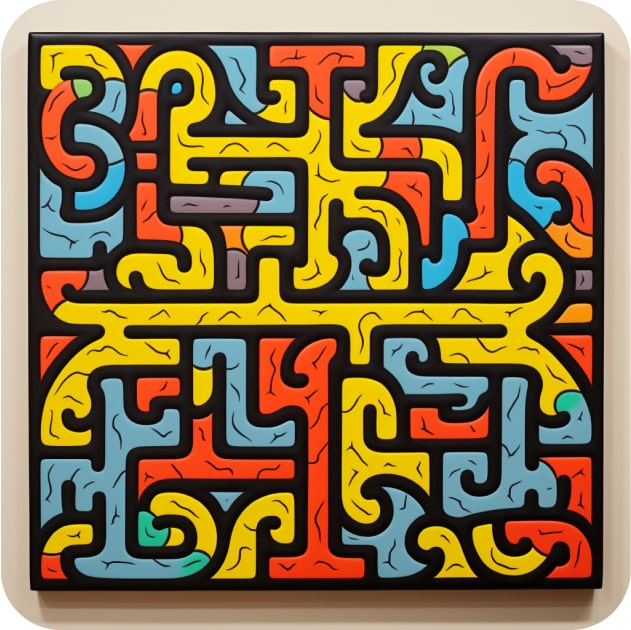
Encode
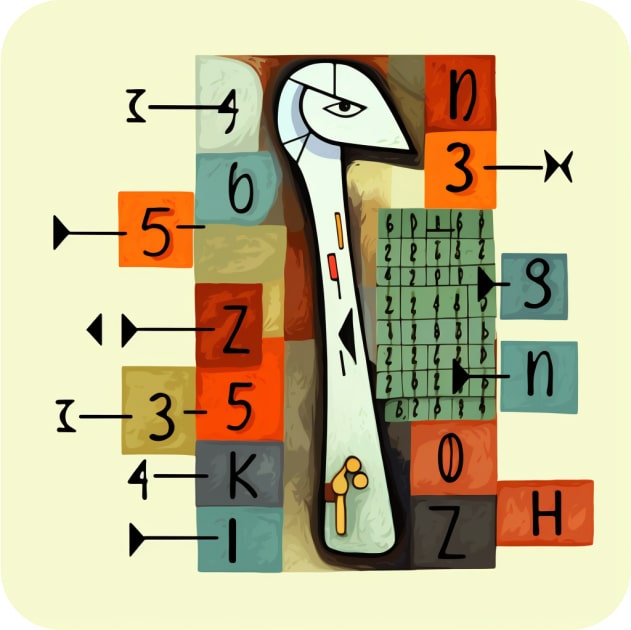
Filter
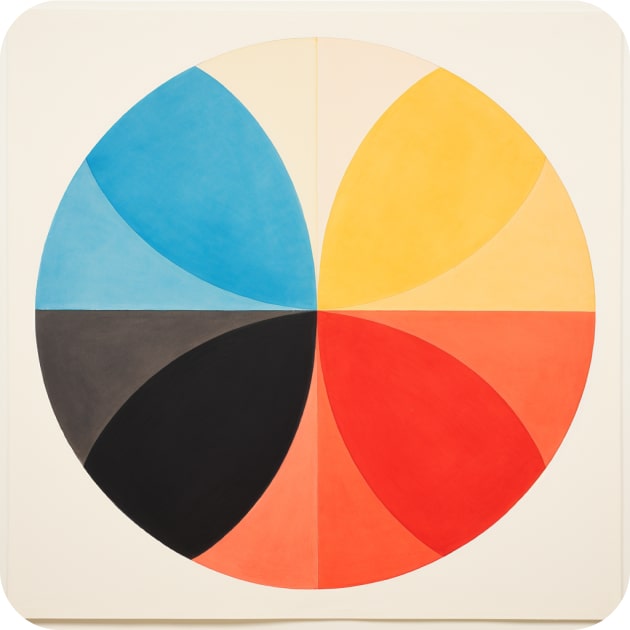
Fragment
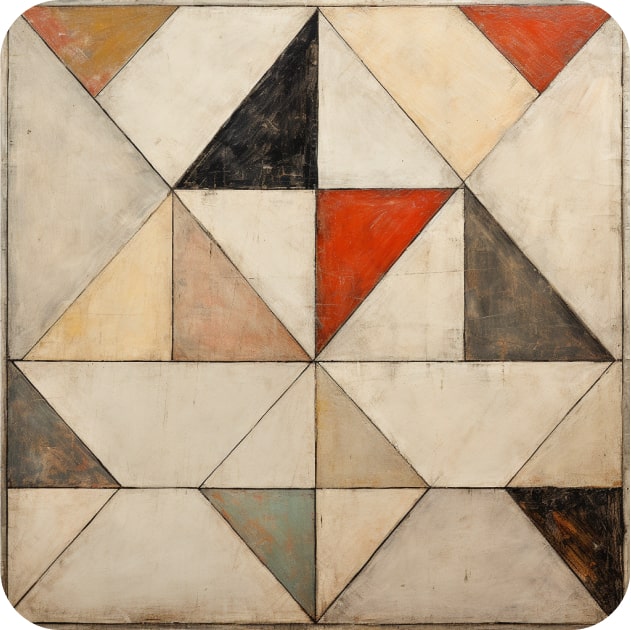
Homogenize
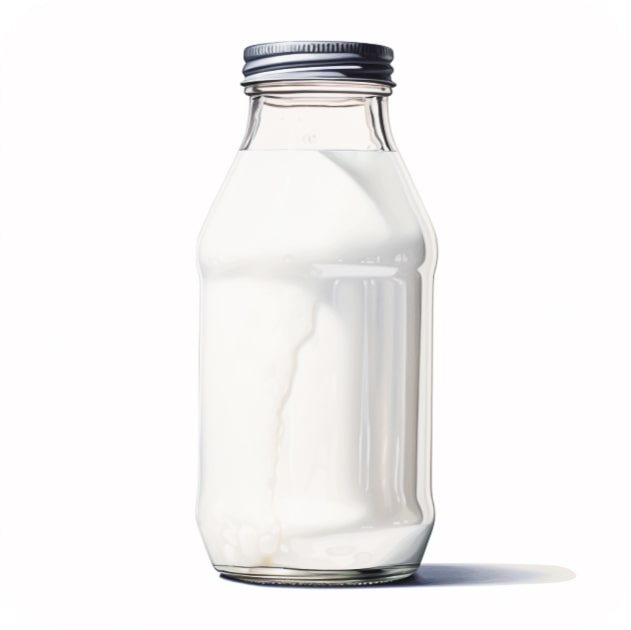
Impute
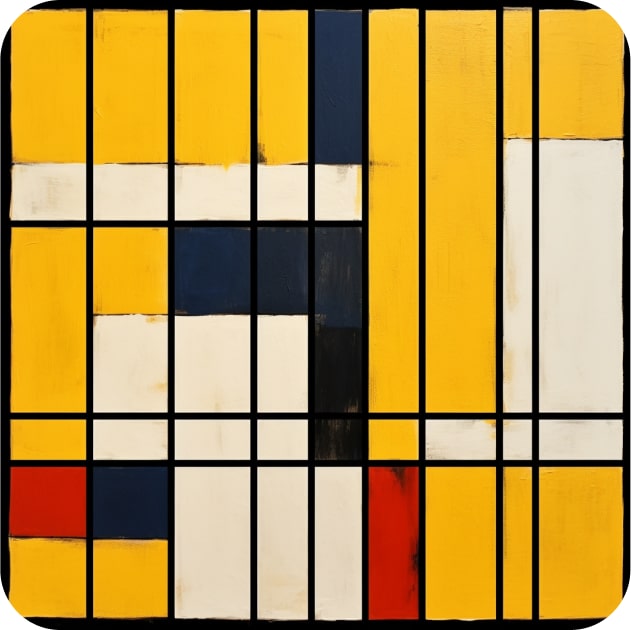
Linearize
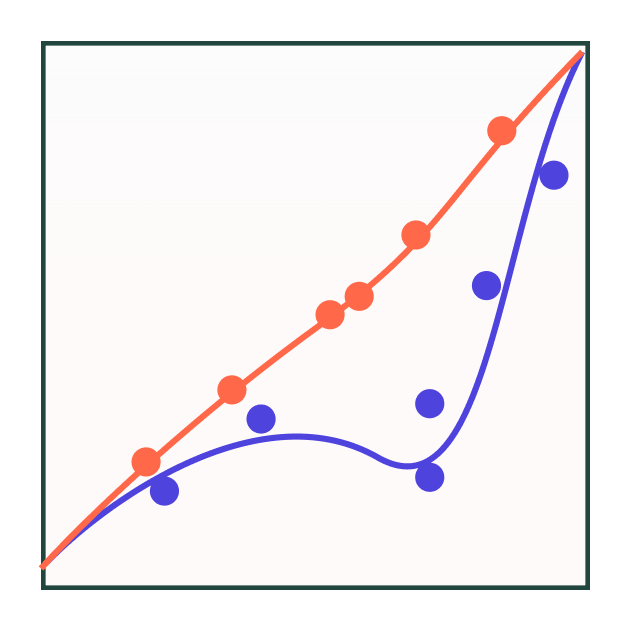
Munge
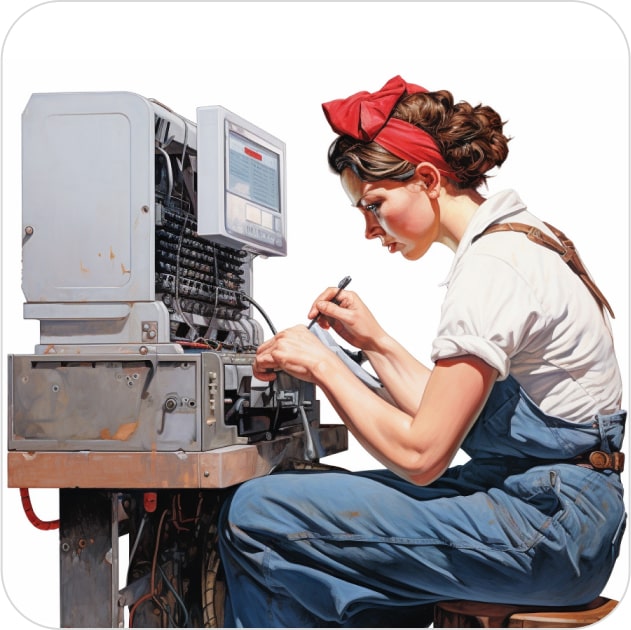
Normalize
Reduce
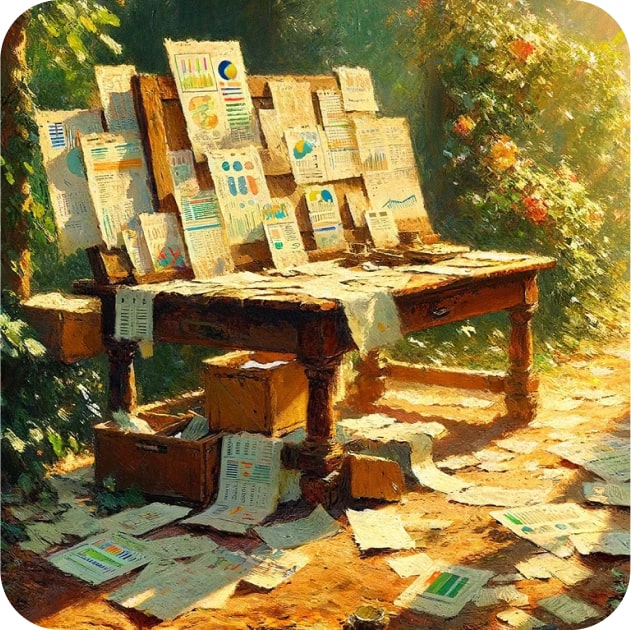
Reshape
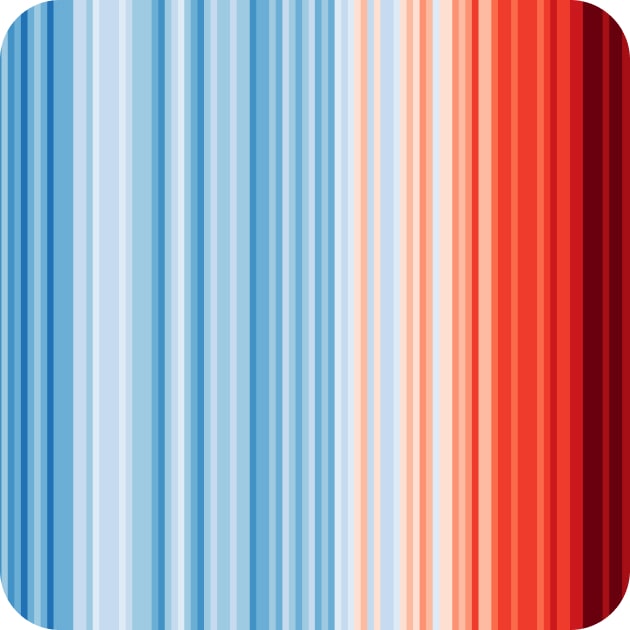
Serialize
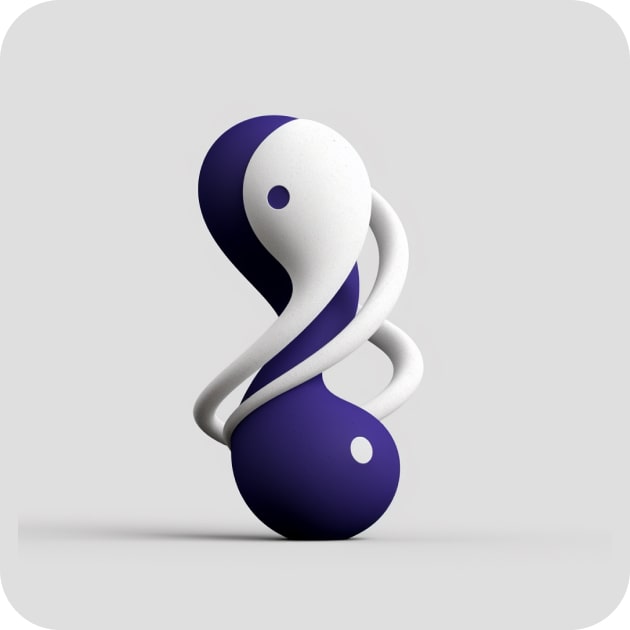
Shred
Skew
Split
Standardize
Transform
Wrangle
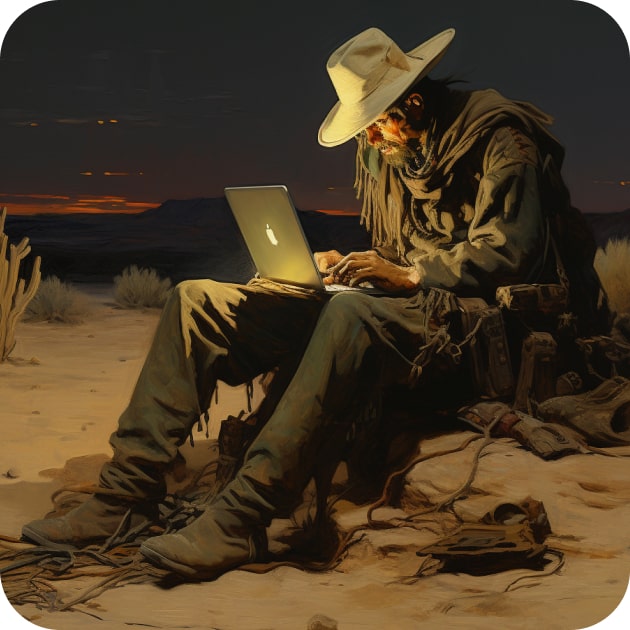