Data wrangling definition:
Data wrangling, also known as data munging or data preprocessing, in the context of modern data engineering, refers to the process of manually converting or mapping data from one raw form into another format that allows for more convenient consumption of the data with the help of semi-automated tools. This involves cleaning, normalizing, and transforming data to eliminate any corruption or inaccuracies, ensure consistency, and make it suitable for analysis or use in machine learning models. The ultimate goal of data munging is to improve the quality and reliability of data insights and decisions derived from the data.
Data wrangling is the process of cleaning, structuring, and enriching raw data into a desired format for better decision-making in less time. This process can involve a wide range of operations, including parsing text data, dealing with missing or inconsistent data, converting data types, or even more complex tasks such as integrating multiple data sources.
Additionally, the transformation of unstructured data (like text, images, videos) into a structured format (like a database or spreadsheet) is often a key part of an ETL (Extract, Transform, Load) process in data engineering.
In the context of specific types of unstructured data like text, this process may also be referred to as text mining or text analytics, which involves extracting valuable information from unstructured text data and transforming it into a structured format.
An example of data wrangling using Python and Pandas
In this example, we'll demonstrate how to clean and transform a CSV file using the pandas library.
Given the following input file:
date,category,value
2023-01-01,A,10
2023-01-02,B,15
2023-01-03,A,8
2023-01-04,C,12
2023-01-05,B,20
2023-01-06,C,16
2023-01-07,A,11
2023-01-08,B,18
2023-01-09,C,14
2023-01-10,A,9
2023-01-11,B,16
2023-01-12,C,13
We can use the following code to clean and transform our data:
import pandas as pd
# Load the CSV file into a DataFrame
data = pd.read_csv('data.csv')
# Display the initial state of the data
print('Initial data:')
print(data.head())
# Drop any rows with missing values
data = data.dropna()
# Convert a column to datetime format
data['date'] = pd.to_datetime(data['date'])
# Filter the data for a specific date range
start_date = pd.to_datetime('2023-01-01')
end_date = pd.to_datetime('2023-05-01')
filtered_data = data[(data['date'] >= start_date) & (data['date'] <= end_date)]
# Group the data by a categorical column and calculate the average of a numeric column
grouped_data = filtered_data.groupby('category')['value'].mean().reset_index()
# Sort the grouped data by the average value in descending order
sorted_data = grouped_data.sort_values(by='value', ascending=False)
# Reset the index of the sorted data
sorted_data.reset_index(drop=True, inplace=True)
# Display the final state of the data
print('Final data:')
print(sorted_data.head())
In this example, we assume that you have a CSV file named data.csv
containing columns such as 'date', 'category', and 'value'. Here's a breakdown of the steps involved:
- We start by importing the
pandas
library, which provides powerful data manipulation tools. - The CSV file is loaded into a DataFrame using the
read_csv
function frompandas
. - We print the initial state of the data using the
head
function to display the first few rows. - The
dropna
method is used to remove any rows with missing values from the DataFrame. - The 'date' column is converted to datetime format using the
to_datetime
function. - We filter the data for a specific date range using boolean indexing.
- The data is grouped by the 'category' column, and the mean value of the 'value' column is calculated using the
groupby
andmean
functions. - The grouped data is sorted in descending order based on the average value using the
sort_values
method. - We reset the index of the sorted data using the
reset_index
function. - Finally, we print the final state of the data using the
head
function to display the sorted and transformed DataFrame.
This example demonstrates some common data wrangling operations such as dropping missing values, converting data types, filtering data based on conditions, grouping data, and sorting. These operations are commonly performed in modern data engineering pipelines to clean and prepare data for further analysis or processing.
Here is the output this example will produce:
Initial data:
date category value
0 2023-01-01 A 10
1 2023-01-02 B 15
2 2023-01-03 A 8
3 2023-01-04 C 12
4 2023-01-05 B 20
Final data:
category value
0 B 17.25
1 C 13.75
2 A 9.50
Align
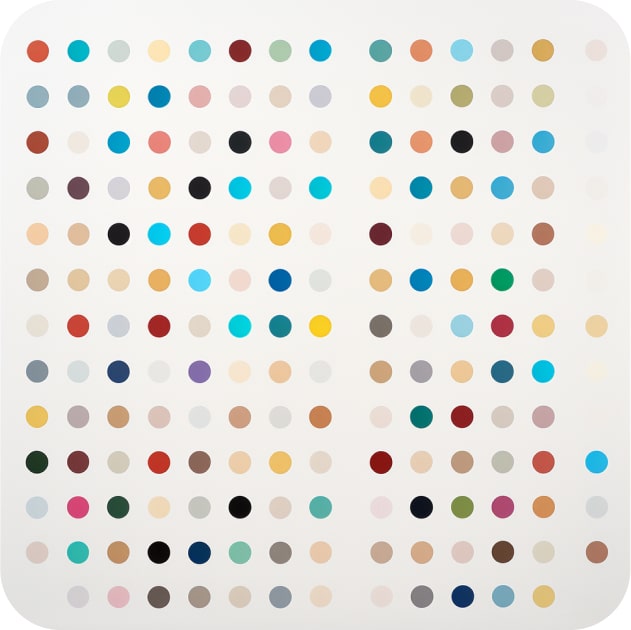
Clean or Cleanse
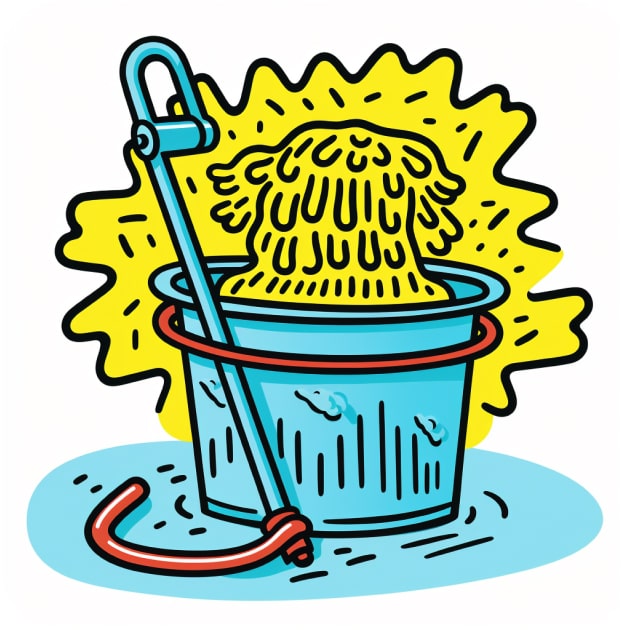
Cluster
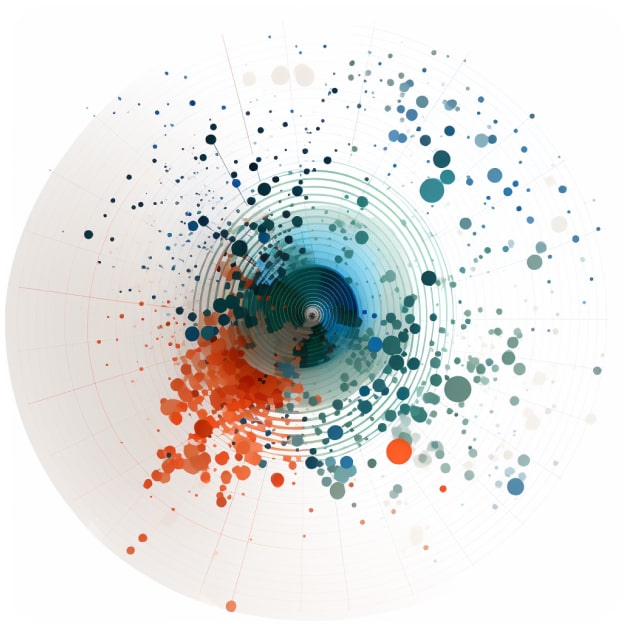
Curate
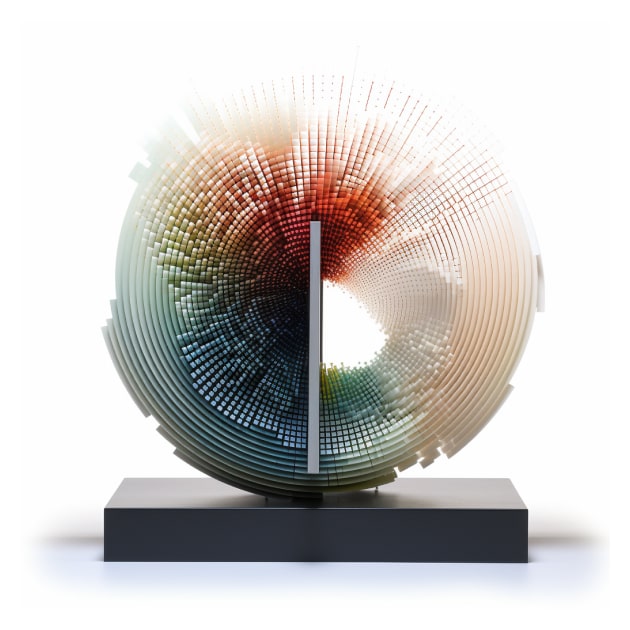
Denoise
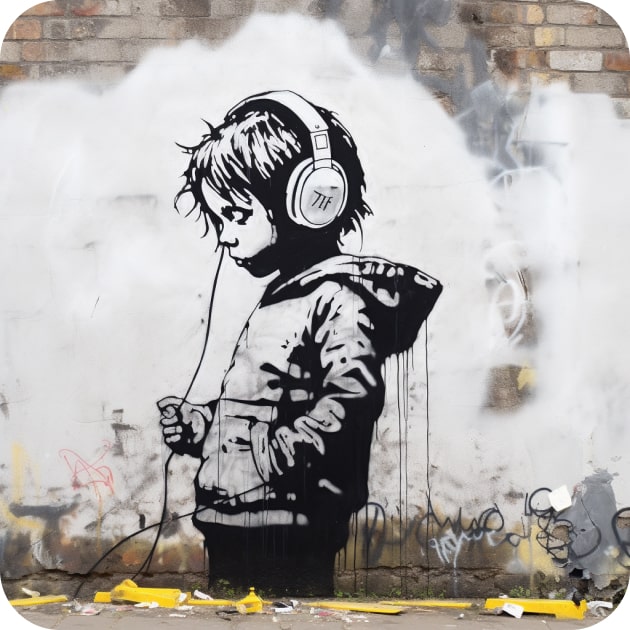
Denormalize
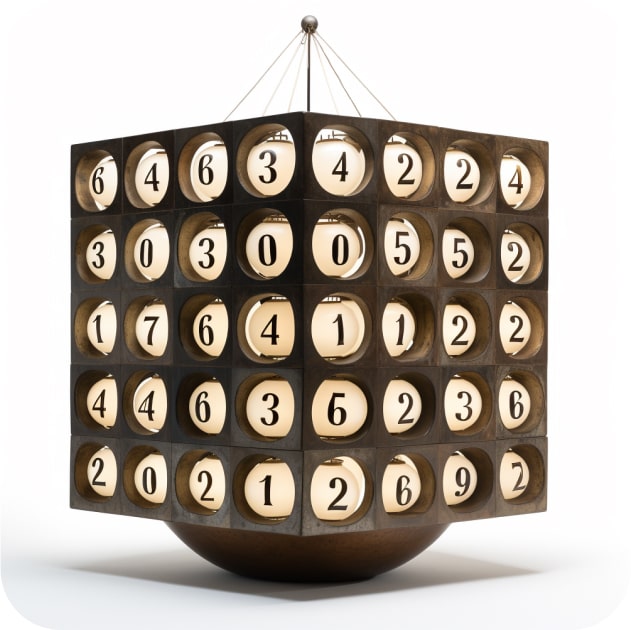
Derive
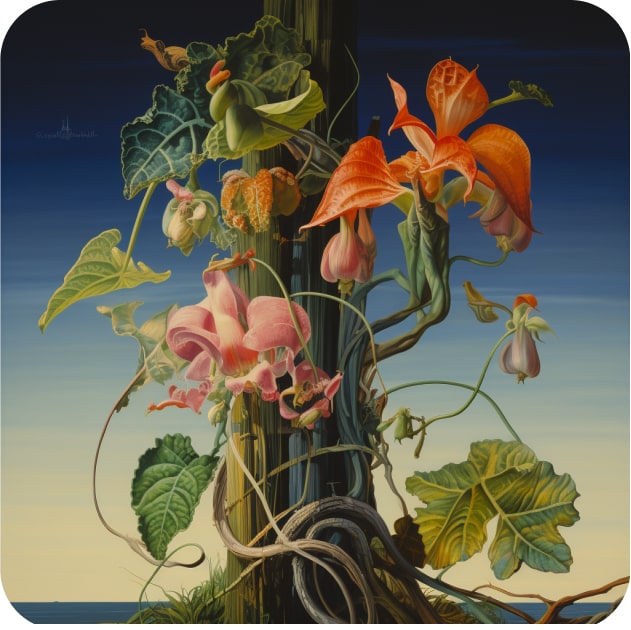
Discretize
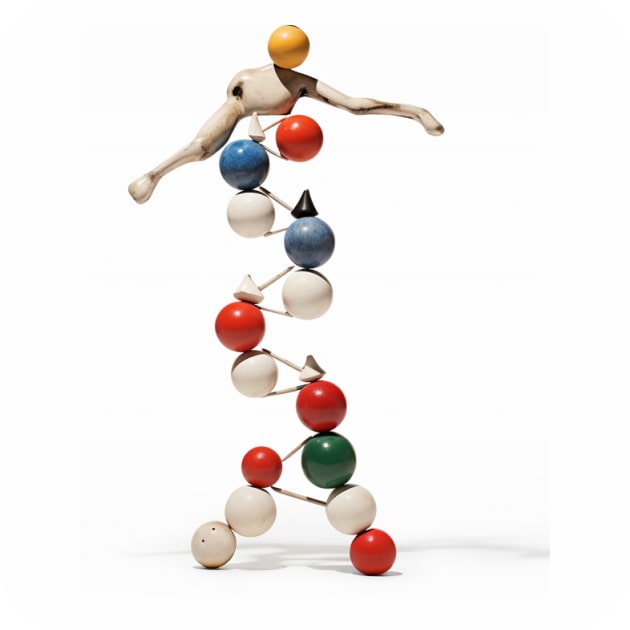
ETL
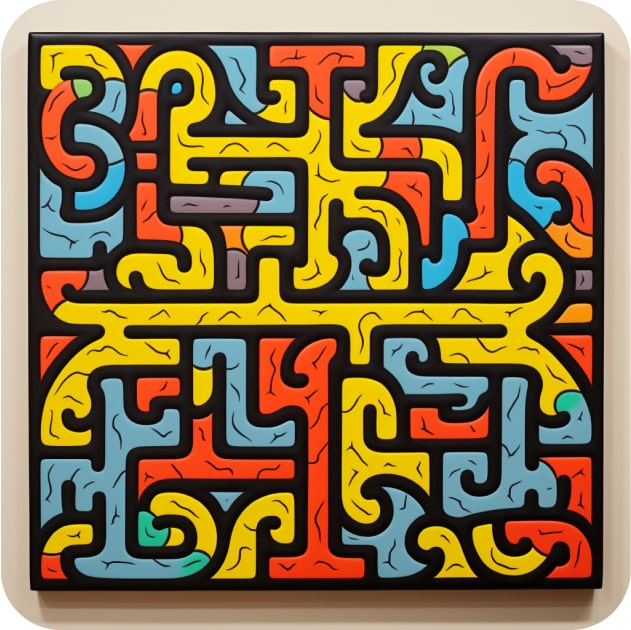
Encode
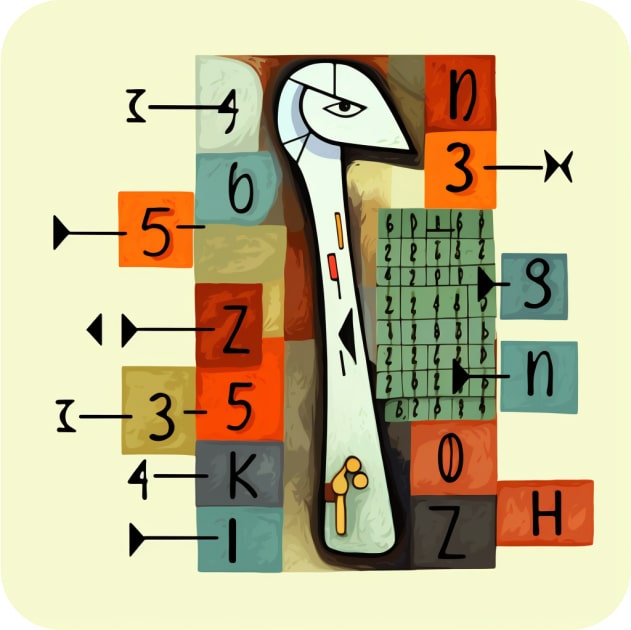
Filter
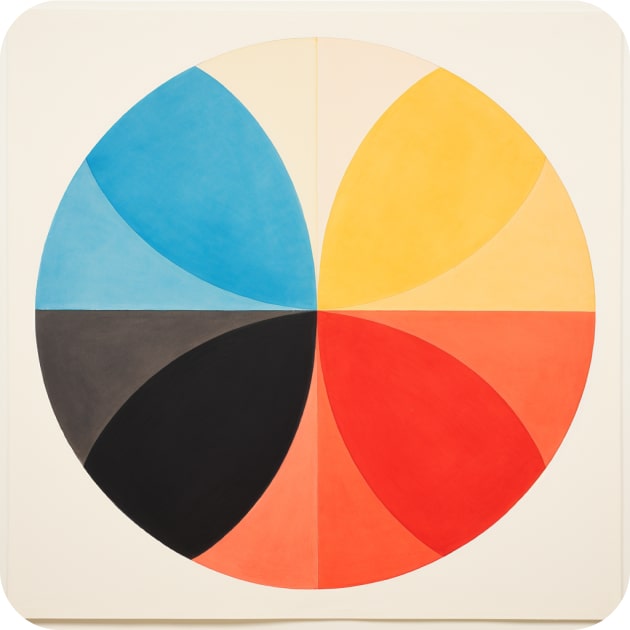
Fragment
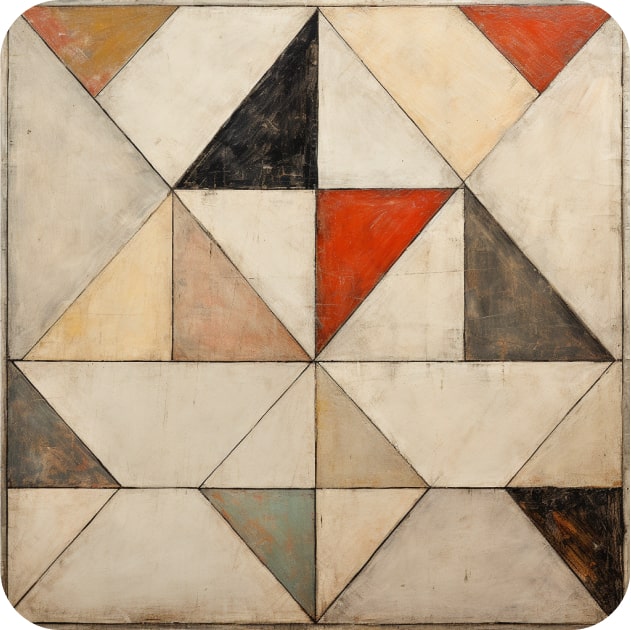
Homogenize
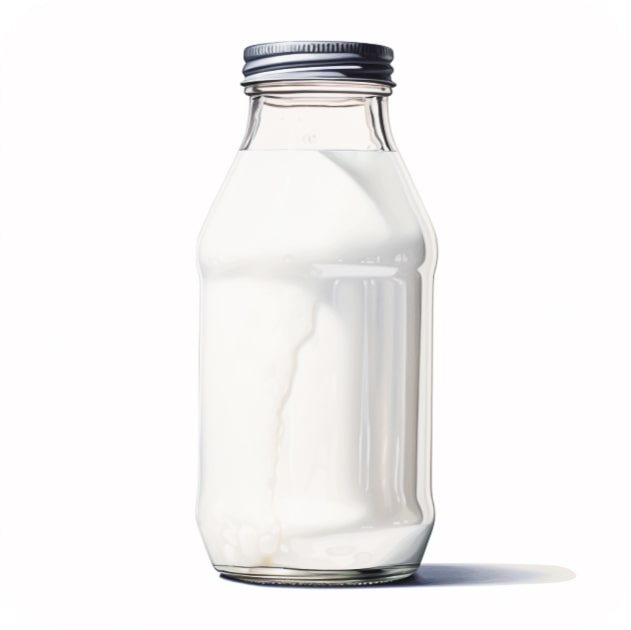
Impute
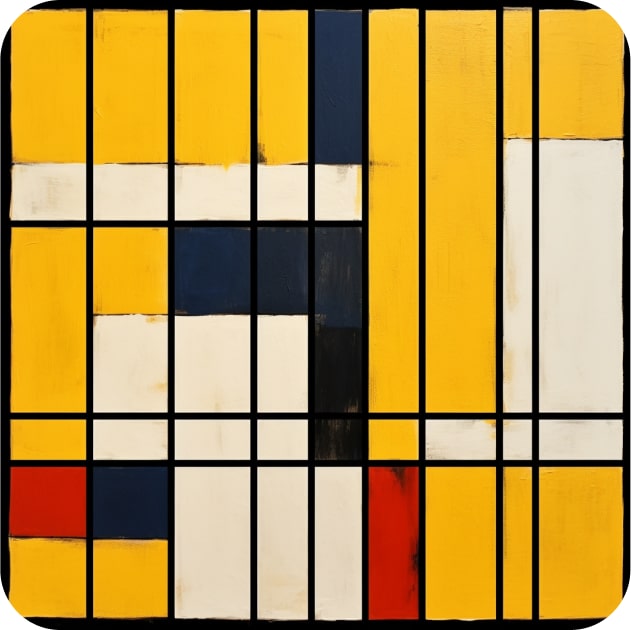
Linearize
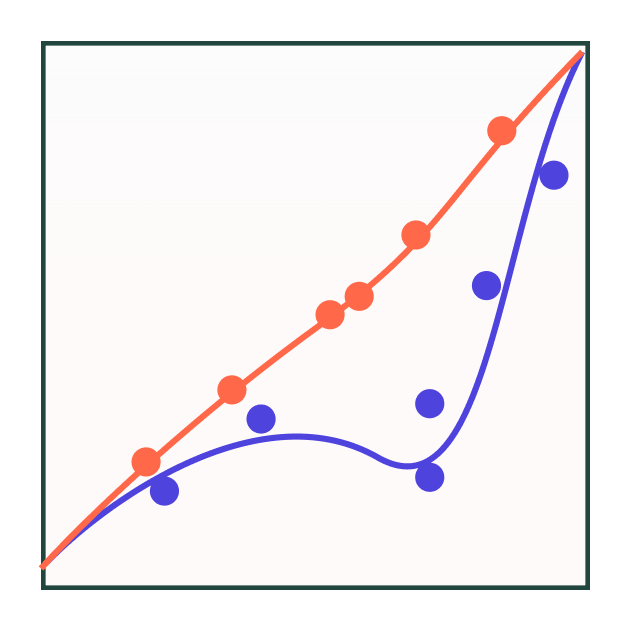
Munge
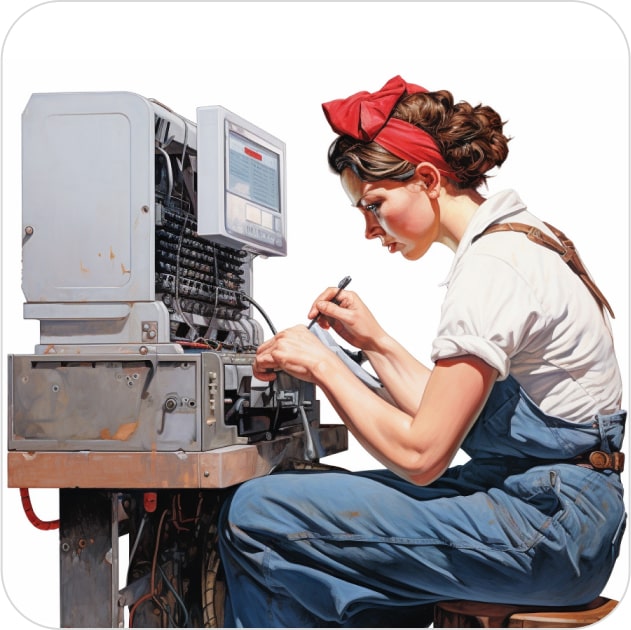
Normalize
Reduce
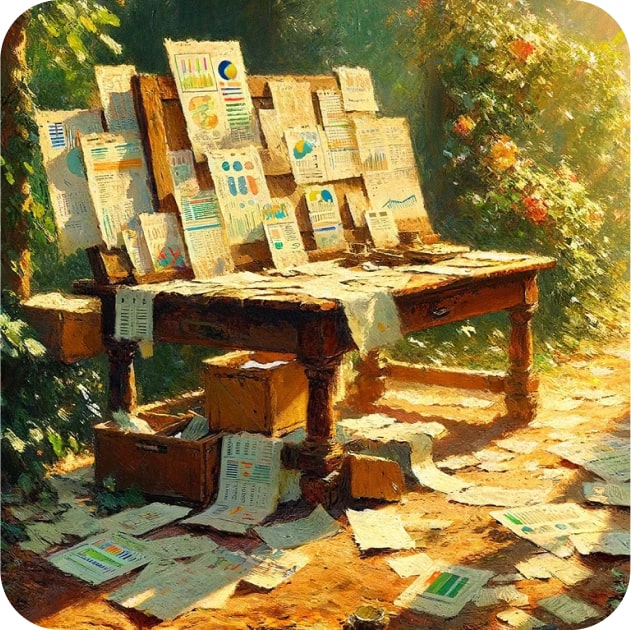
Reshape
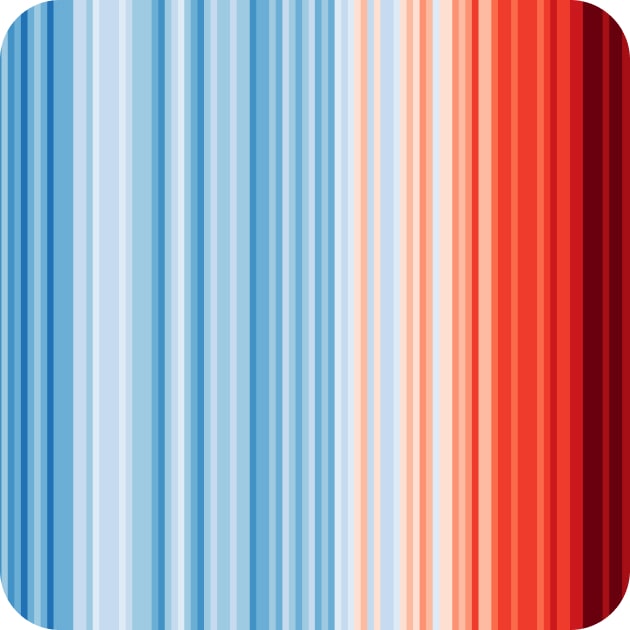
Serialize
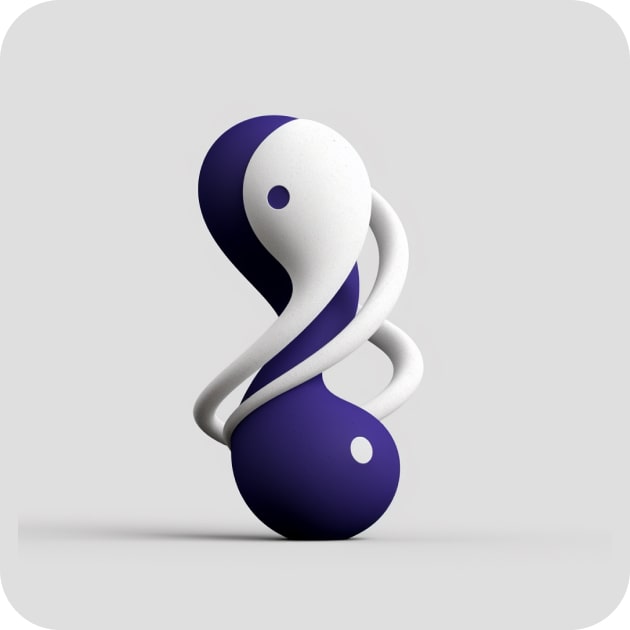
Shred
Skew
Split
Standardize
Tokenize
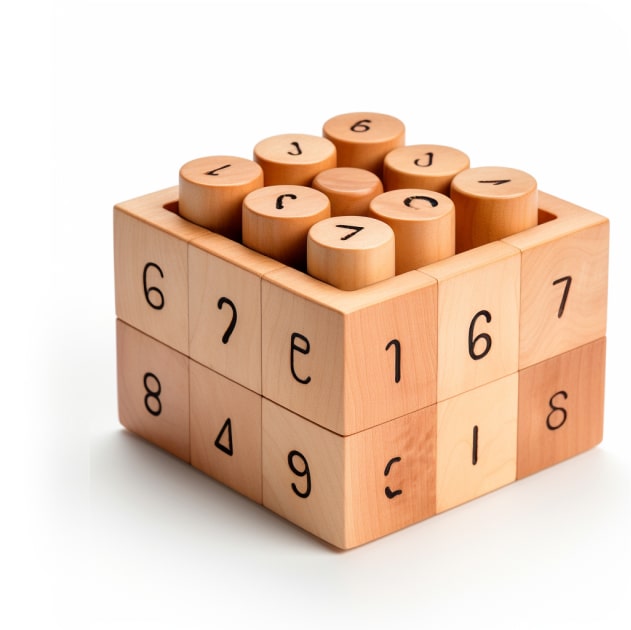