Data Preprocessing
Data preprocessing definition:
Data Preprocessing is a fundamental step in any data science or data engineering project.
Data preprocessing is a data mining technique that transforms raw data into an understandable and efficient format. This step typically takes place before the data analysis or machine learning modeling stages. Data preprocessing may involve several processes like:
Data Cleaning: Removing noise and handling missing or inconsistent data. This step ensures that the quality of data is good enough for the subsequent stages.
Data Transformation: Normalization, aggregation, or general scaling of data to prepare it for the machine learning algorithms.
Data Reduction: Reducing the volume but producing the same or similar analytical results.
Data Discretization: The process of converting continuous data to categorical data.
Feature Extraction or Feature Selection: The process of creating new features or modifying existing features to improve the performance of machine learning models.
A simple example of data preprocessing using Python:
Please note that you need to have the necessary Python libraries installed in your Python environment to run this code.
Here is a simple example of text preprocessing. This script uses NLTK and re (regular expressions) to preprocess a simple text string. The preprocessing tasks include:
- Text lowercase
- Remove numbers
- Remove punctuation
- Remove whitespaces
- Remove stopwords
- Stem (reduce words to their root form.)
import re
import nltk
from nltk.corpus import stopwords
from nltk.stem.porter import PorterStemmer
nltk.download('punkt')
nltk.download('stopwords')
# Define the text string
text_string = "Rein in the chaos and maintain control over your data as the complexity scales. Centralize your metadata in one tool with built-in observability, diagnostics, cataloging, and lineage. Spot any of 100 issues and identify performance improvement opportunities."
# Step 1: Convert text to lowercase
text_string = text_string.lower()
# Step 2: Remove numbers
text_string = re.sub(r'\d+', '', text_string)
# Step 3: Remove punctuation
text_string = re.sub(r'[^\w\s]', '', text_string)
# Step 4: Remove whitespaces
text_string = text_string.strip()
# Step 5: Remove stopwords
stop_words = set(stopwords.words('english'))
tokens = nltk.word_tokenize(text_string)
tokens = [token for token in tokens if token not in stop_words]
# Step 6: Stemming
porter = PorterStemmer()
stemmed = [porter.stem(word) for word in tokens]
print(f"Original Text: {text_string}")
print("Preprocessed Text: ", ' '.join(stemmed))
If you execute this code you will see the following output:
Original Text: rein in the chaos and maintain control over your data as the complexity scales centralize your metadata in one tool with builtin observability diagnostics cataloging and lineage spot any of issues and identify performance improvement opportunities
Preprocessed Text: rein chao maintain control data complex scale central metadata one tool builtin observ diagnost catalog lineag spot issu identifi perform improv opportun
Data preprocessing vs. Data Preparation:
Data preparation is a broader term than data preprocessing that includes all activities to construct and consolidate a dataset for analysis or operational use. This can involve:
Data Acquisition: Collecting or integrating data from various sources.
Data Integration: Combining data from different sources and providing users with a unified view of the data.
Data Preprocessing: As described above, which includes cleaning, transformation, reduction, etc.
Data Partitioning: Splitting the data into training, validation, and test sets for machine learning models.
In essence, data preprocessing can be seen as a subset of data preparation. While data preparation encompasses the entire journey from raw data to the ready-for-analysis or operational-use data, data preprocessing is specifically concerned with refining and transforming the data after it's been acquired and integrated, and before it's analyzed or fed into a machine learning model. Both steps are critical to ensuring the accuracy, reliability, and efficiency of the downstream processes and outcomes.
Append
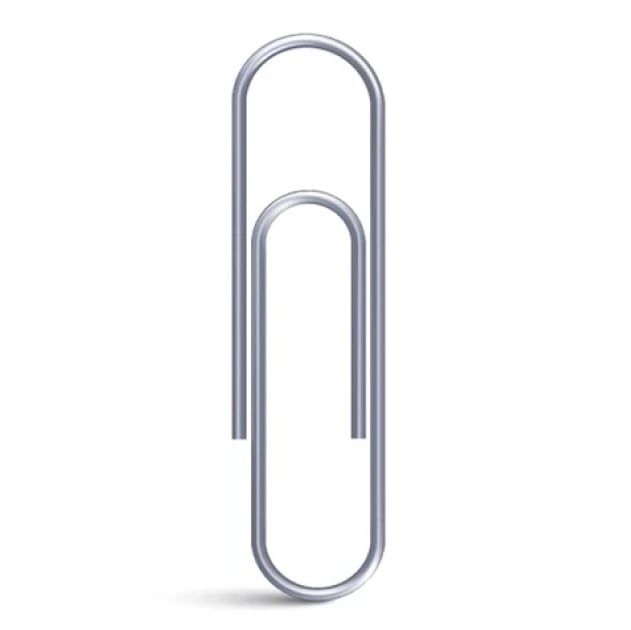
Archive
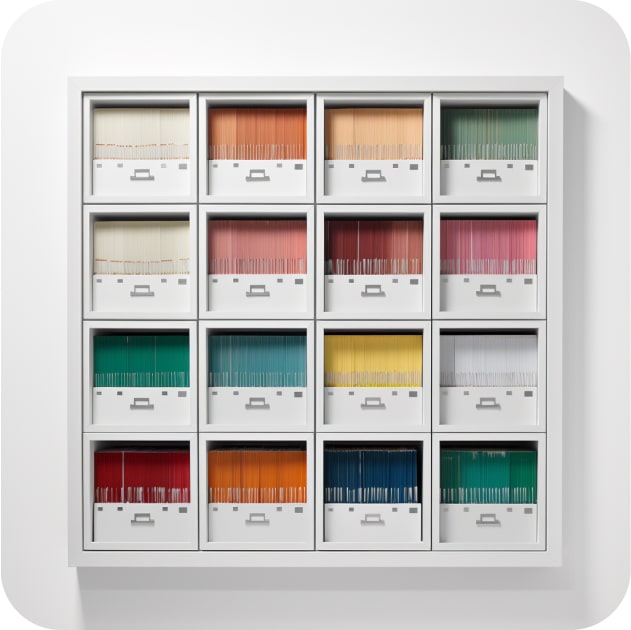
Augment
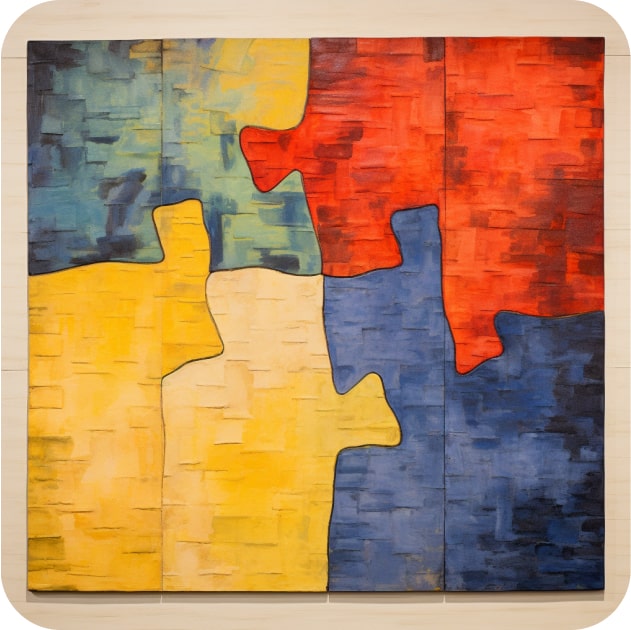
Auto-materialize
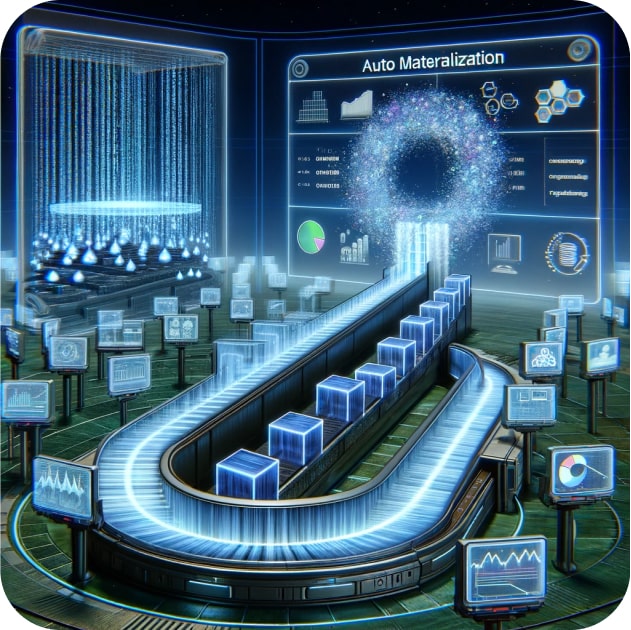
Backup
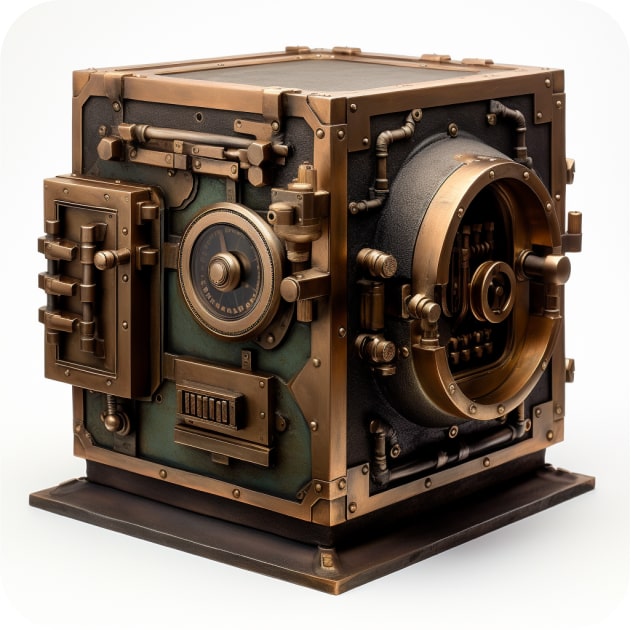
Batch Processing
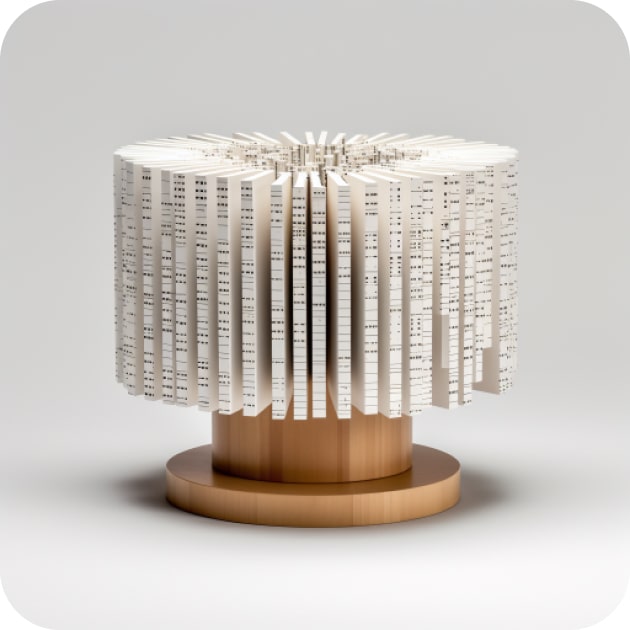
Cache
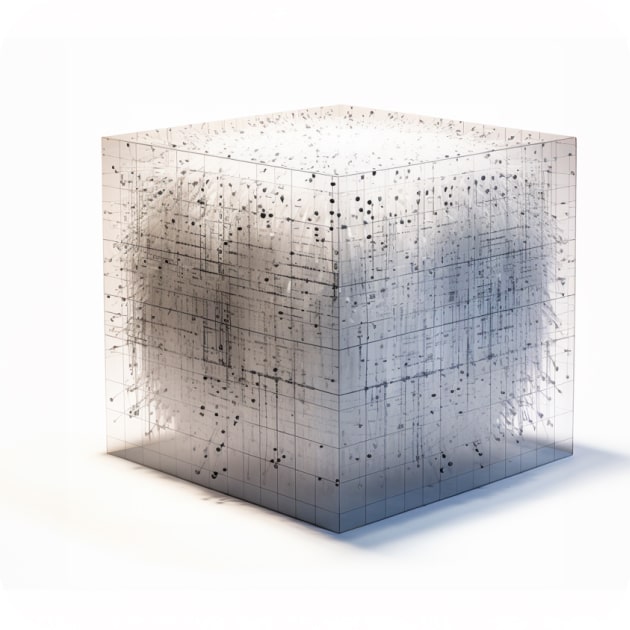
Categorize
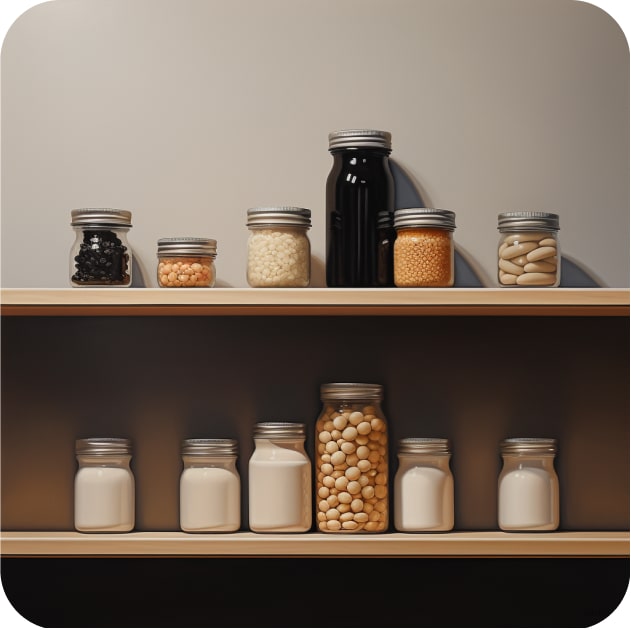
Checkpointing
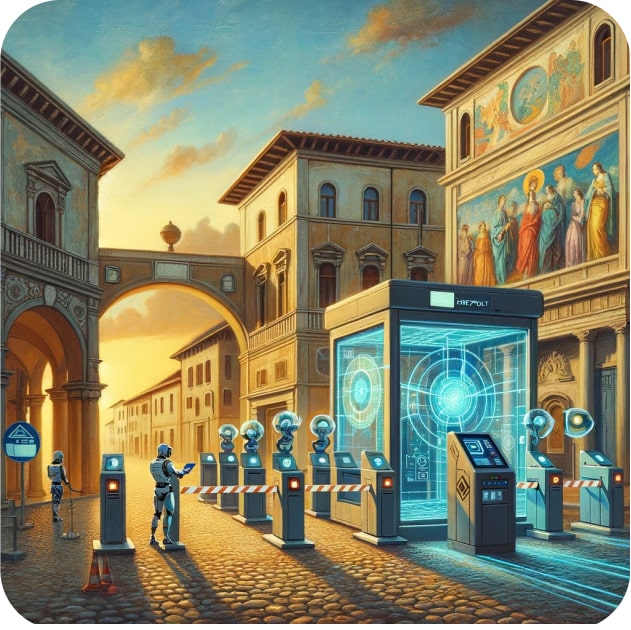
Deduplicate
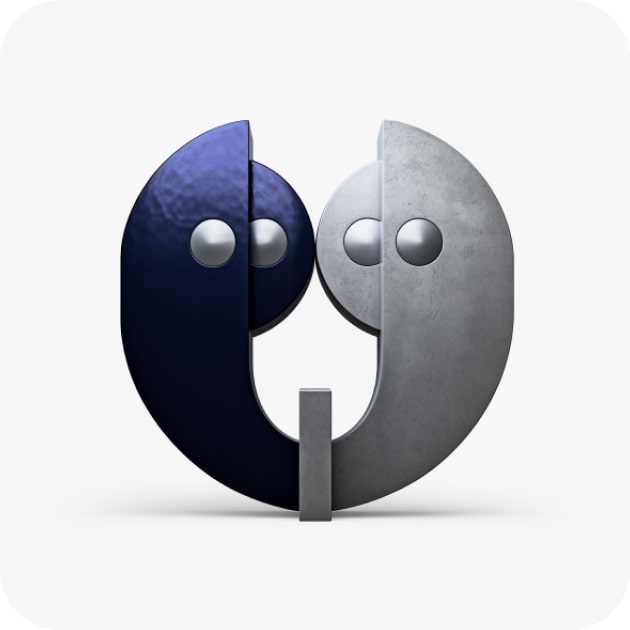
Deserialize
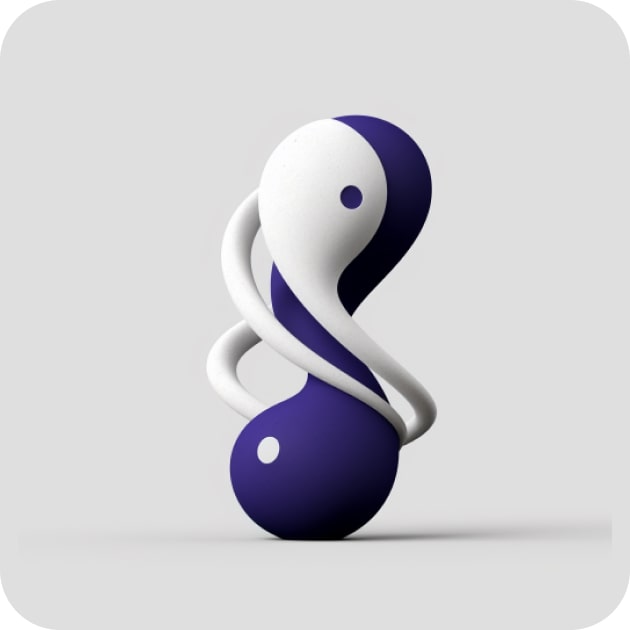
Dimensionality
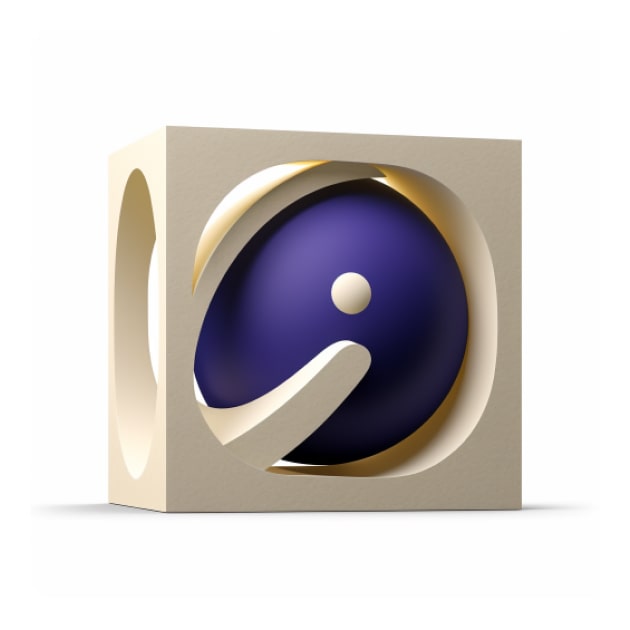
Encapsulate
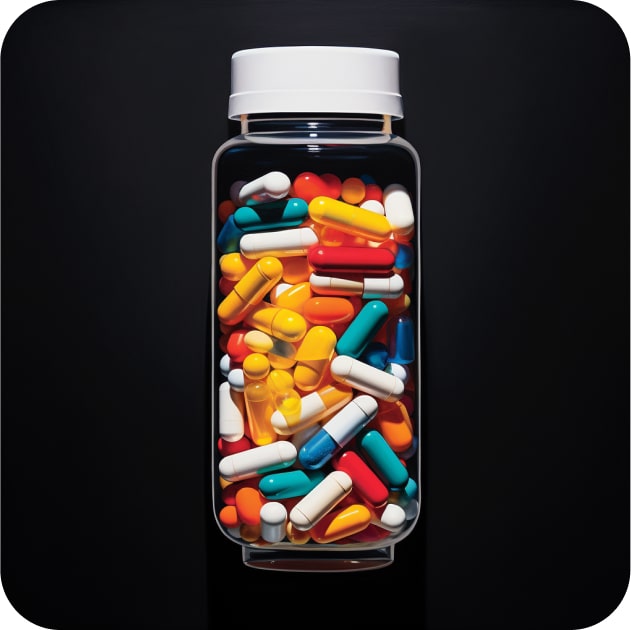
Enrich
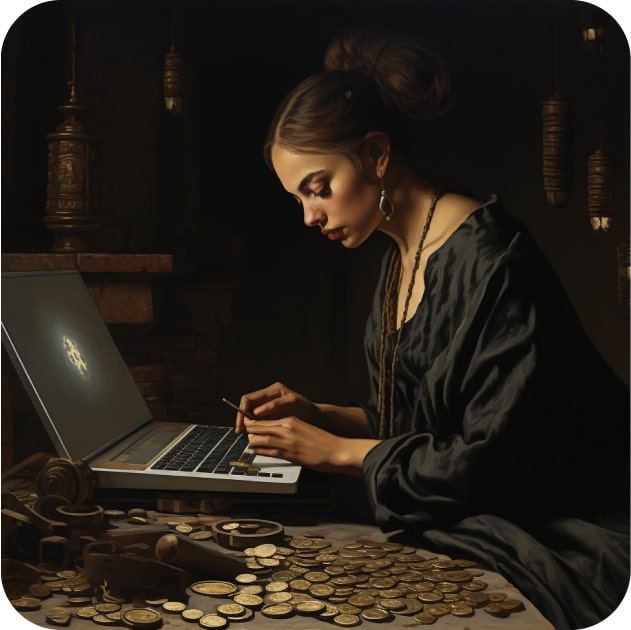
Export
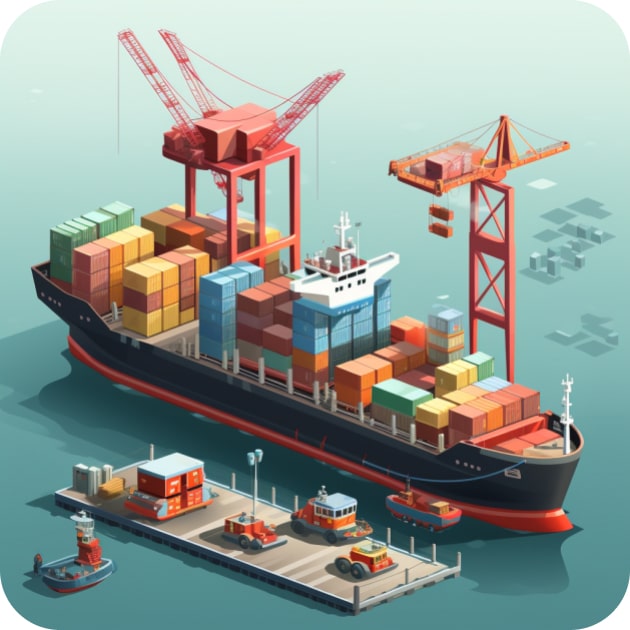
Graph Theory
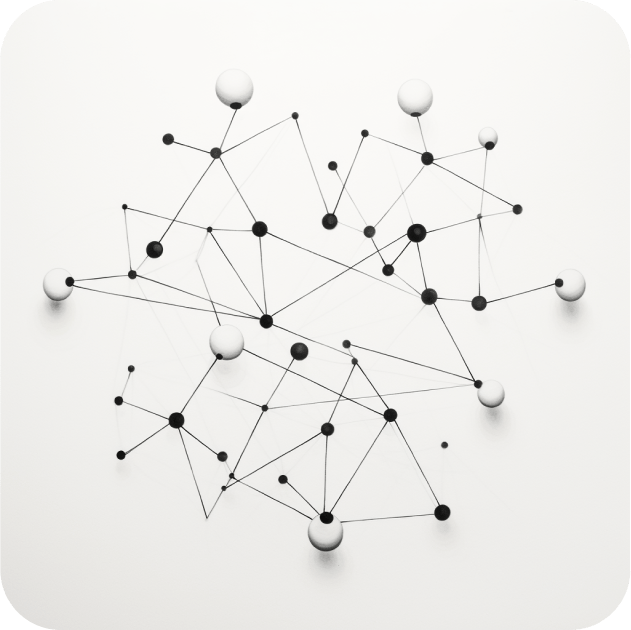
Idempotent
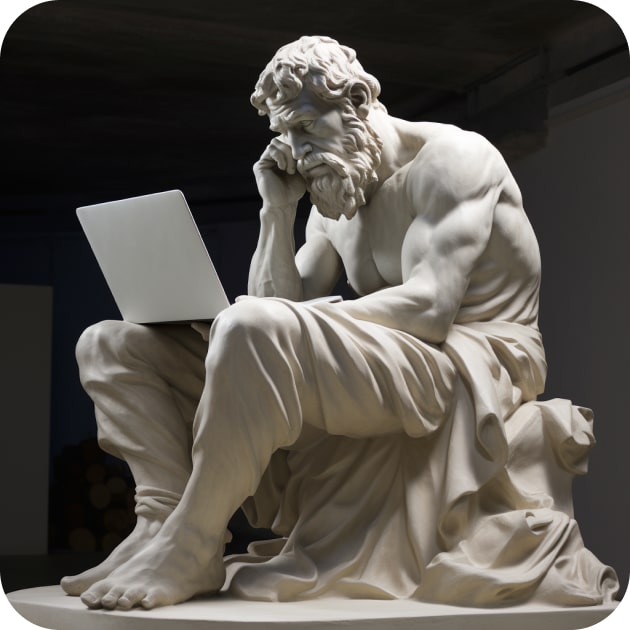
Index
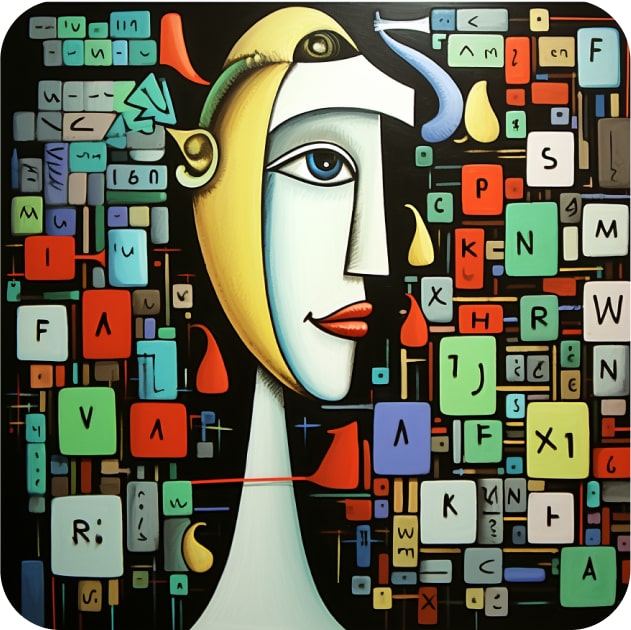
Integrate
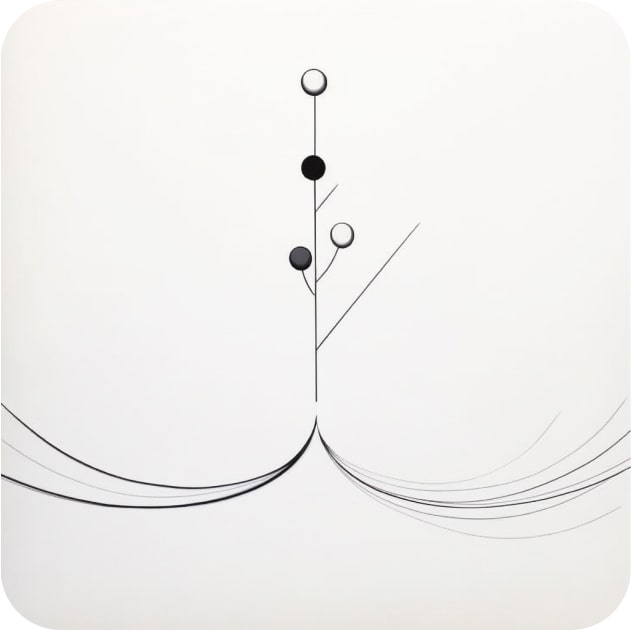
Lineage
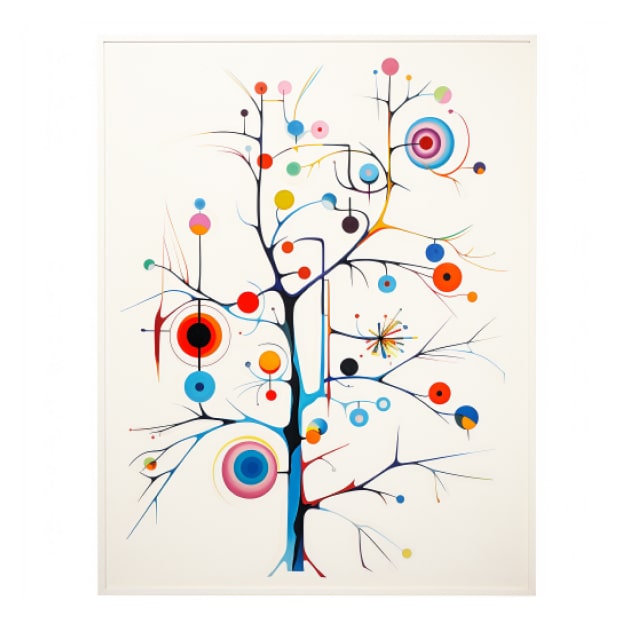
Linearizability
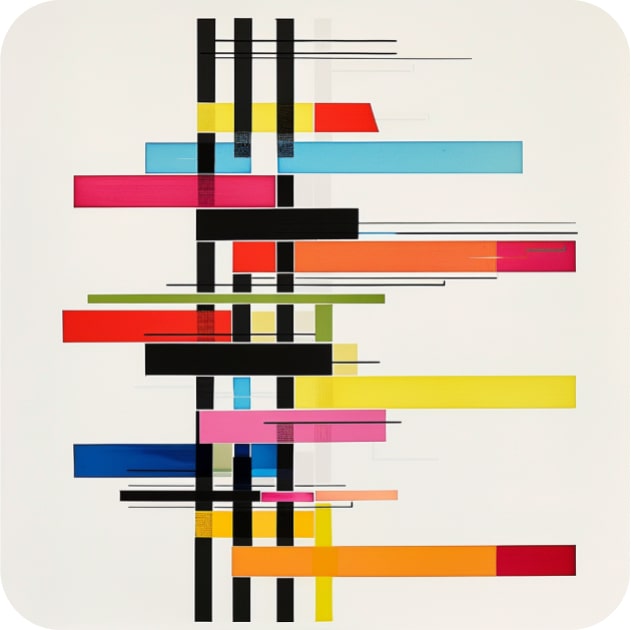
Materialize
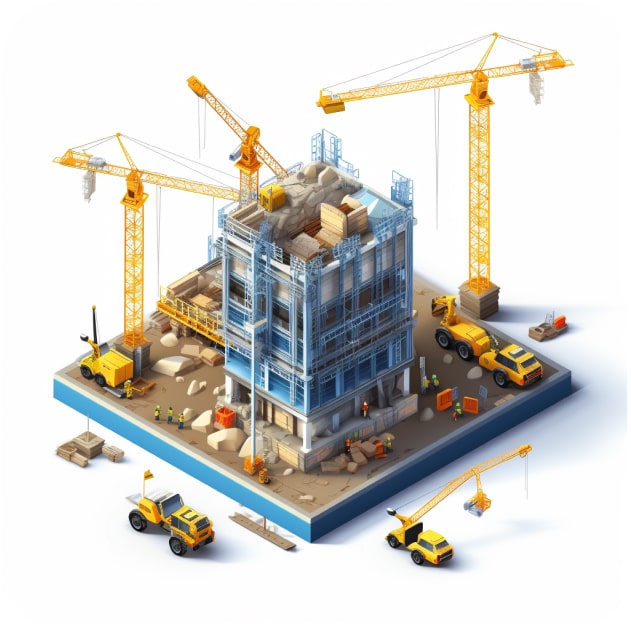
Memoize
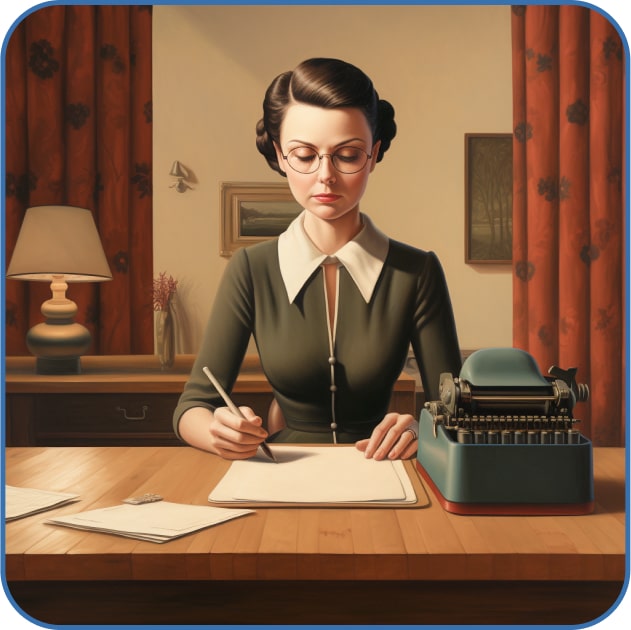
Merge
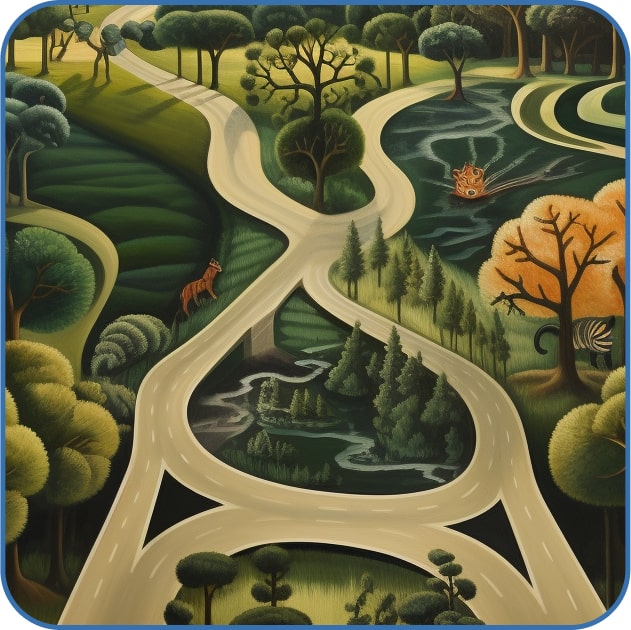
Model
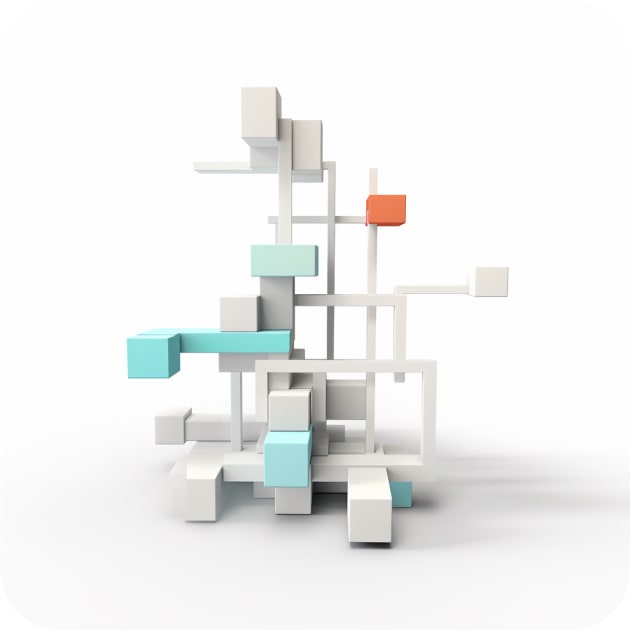
Monitor
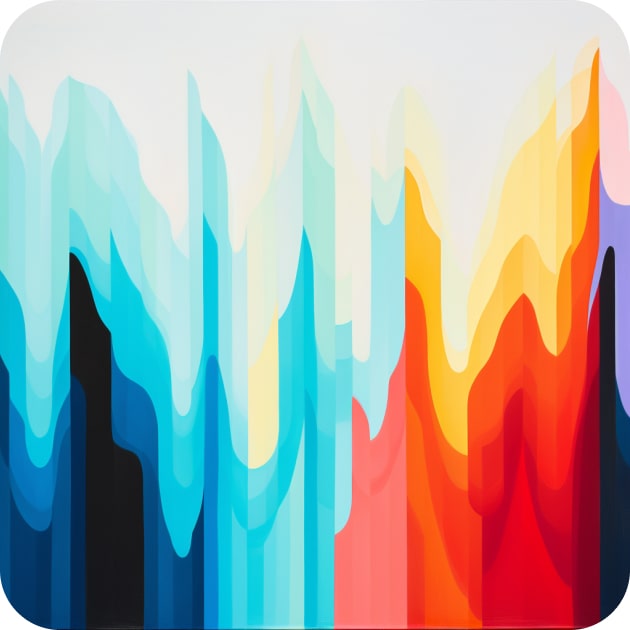
Named Entity Recognition
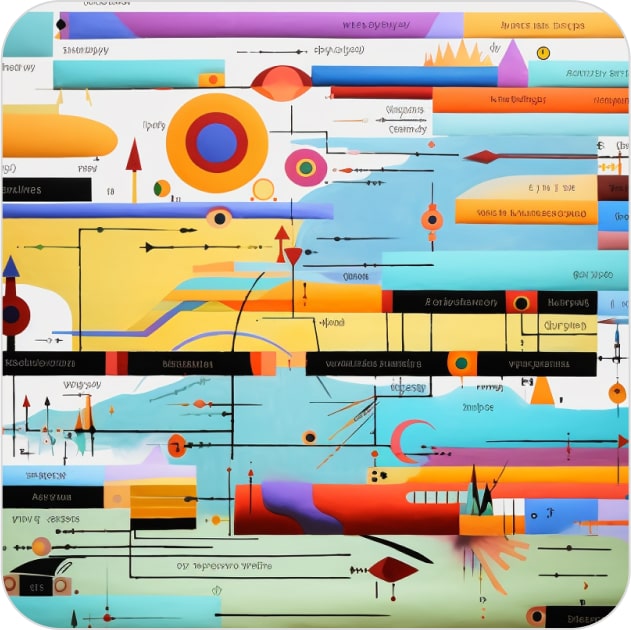
Parse
Partition
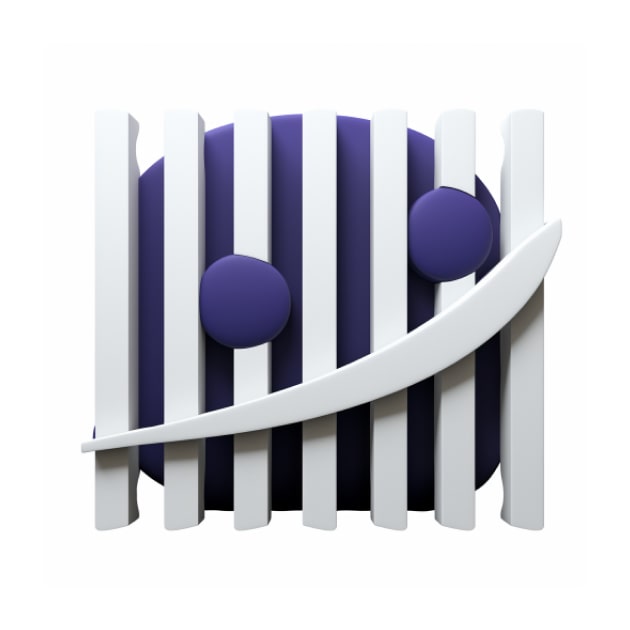
Prep
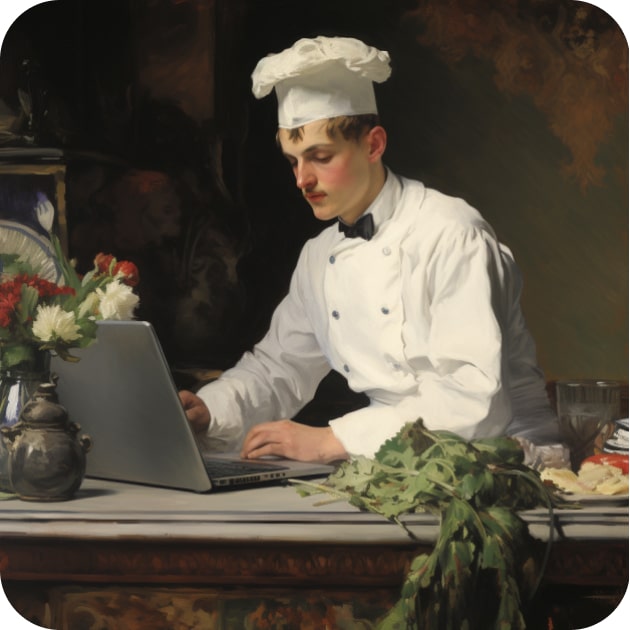
Replicate
Scaling
Schema Inference
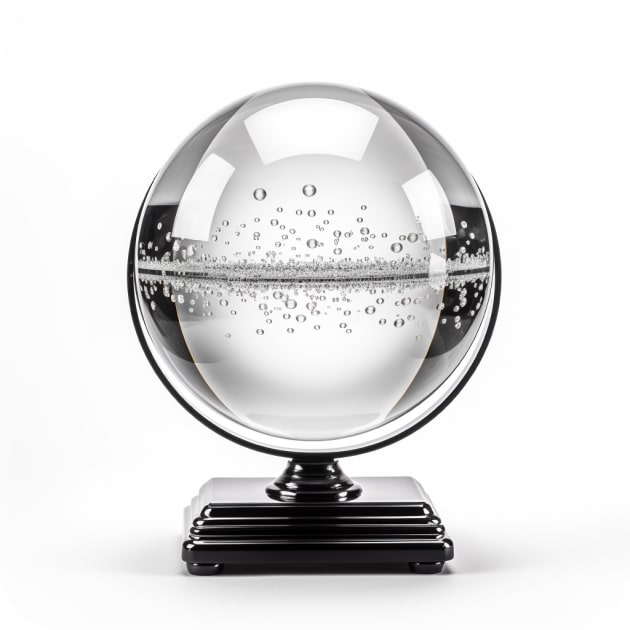
Schema Mapping
Secondary Index
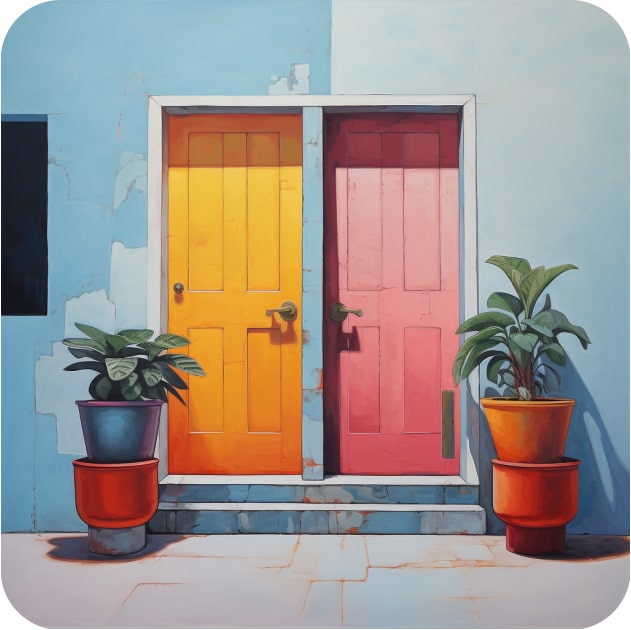
Software-defined Asset
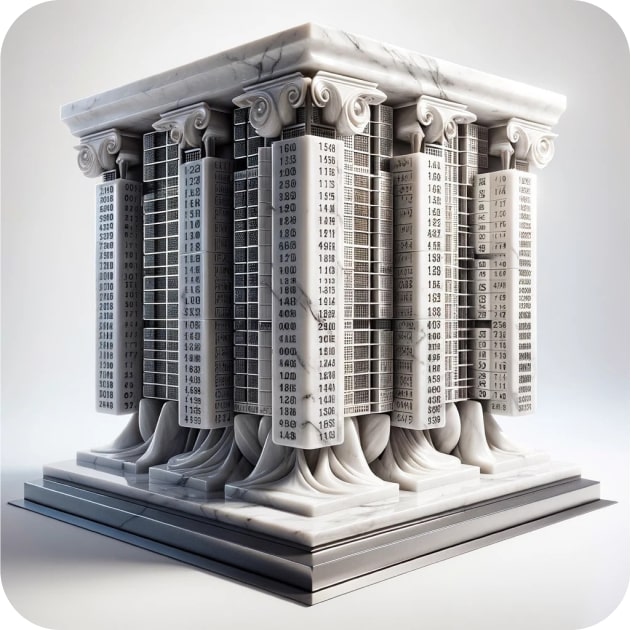
Synchronize
Validate
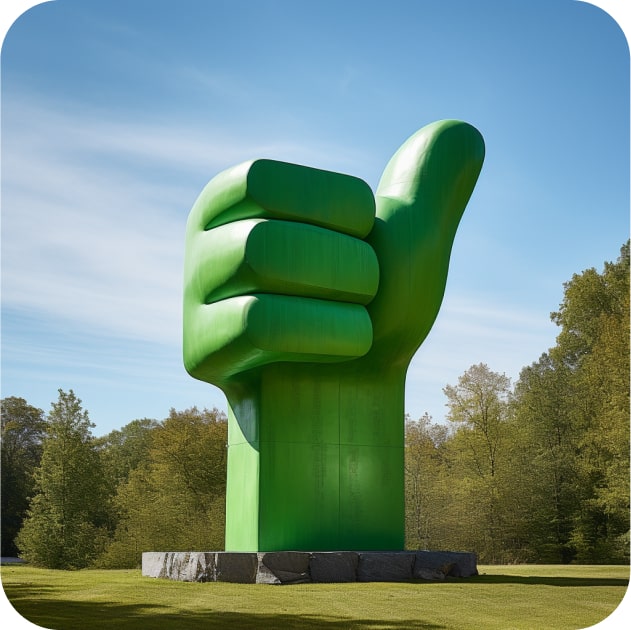
Version
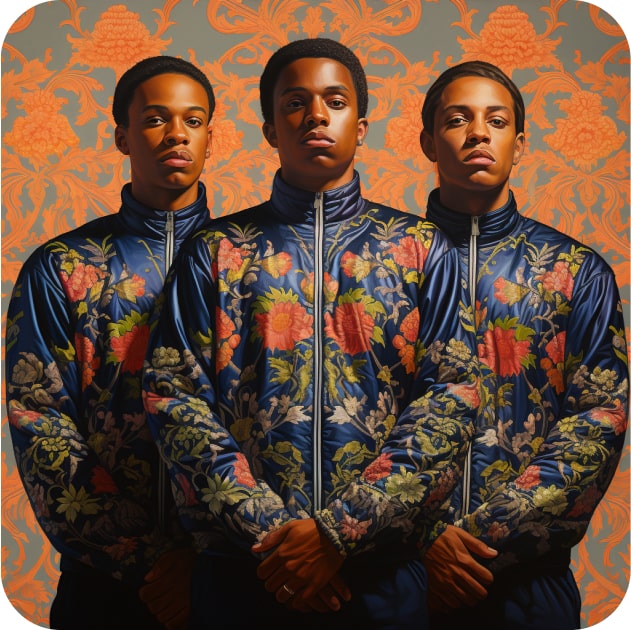