Data modeling definition:
Data modeling is the process of creating a conceptual representation of data objects, their relationships, and the rules that govern them. In the context of modern data pipelines, data modeling is crucial to organizing and structuring data in a way that enables efficient data processing, analysis, and visualization.
There are various data modeling techniques such as Entity-Relationship (ER) modeling, UML modeling, and Data Flow Diagrams (DFDs). In addition, modern data pipelines rely heavily on the use of machine learning algorithms for predictive modeling and pattern recognition.
Data modeling example using Python:
Please note that you need to have the necessary Python libraries installed in your Python environment to run the following code examples.
In Python, there are several libraries and frameworks for data modeling, including:
- Scikit-learn: a popular machine-learning library that includes a wide range of tools for classification, regression, and clustering.
- TensorFlow: an open-source machine learning framework developed by Google that is widely used for building and training deep neural networks.
- PyTorch: another popular open-source machine learning framework that is known for its ease of use and flexibility.
- Pandas: a data manipulation library that provides data structures and functions for working with structured data.
Here is an example of using scikit-learn for data modeling:
Given an input file data.csv
as follows:
feature_1,feature_2,target
1,2,10
2,4,20
3,6,30
4,8,40
5,10,50
The code:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
import pandas as pd
# load data
data = pd.read_csv('data.csv')
# split data into training and testing sets
train_data, test_data = train_test_split(data, test_size=0.2)
# prepare training data
X_train = train_data.drop('target', axis=1)
y_train = train_data['target']
# create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# prepare testing data
X_test = test_data.drop('target', axis=1)
y_test = test_data['target']
# make predictions on test data
predictions = model.predict(X_test)
# calculate mean squared error
mse = mean_squared_error(y_test, predictions)
print('Mean squared error:', mse)
Will yield an output of:
Mean squared error: 1.262177448353619e-29
In this example, we are using the scikit-learn library to create a simple linear regression model. We load our data from a CSV file, split it into training and testing sets, and prepare the training and testing data. We then create and train the linear regression model on the training data and use it to make predictions on the testing data. Finally, we calculate the mean squared error to evaluate the performance of the model.
Append
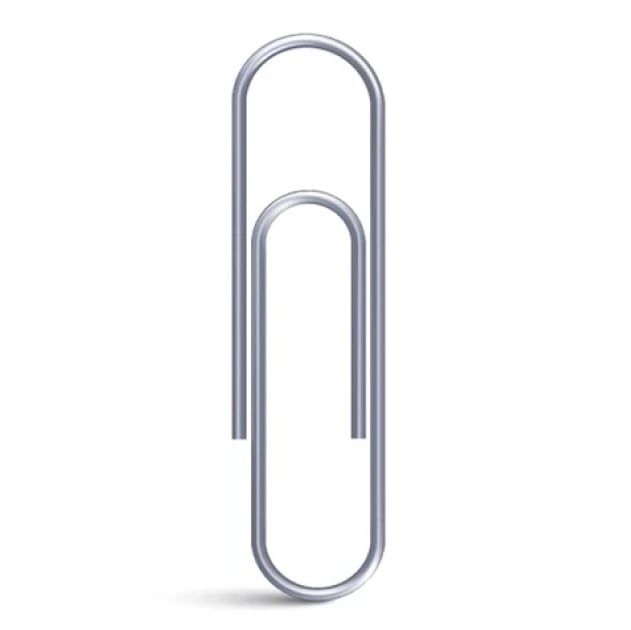
Archive
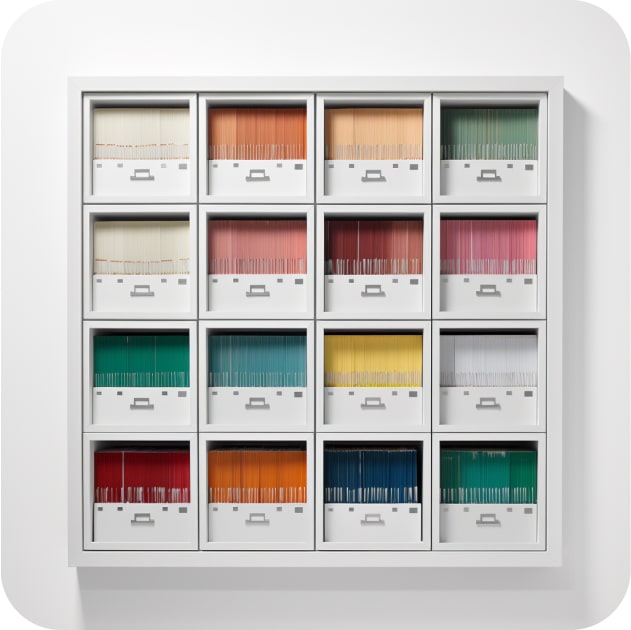
Augment
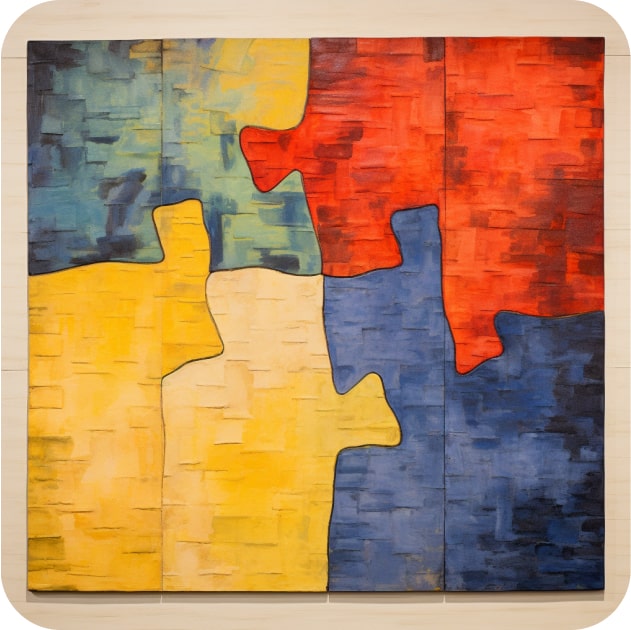
Auto-materialize
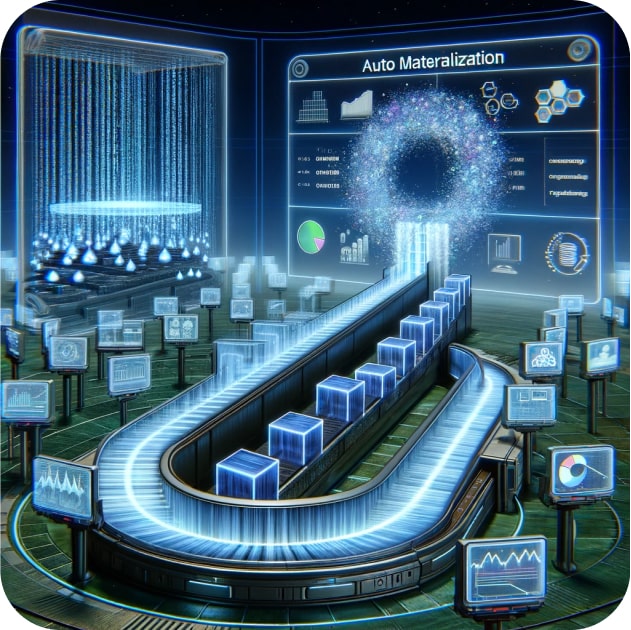
Backup
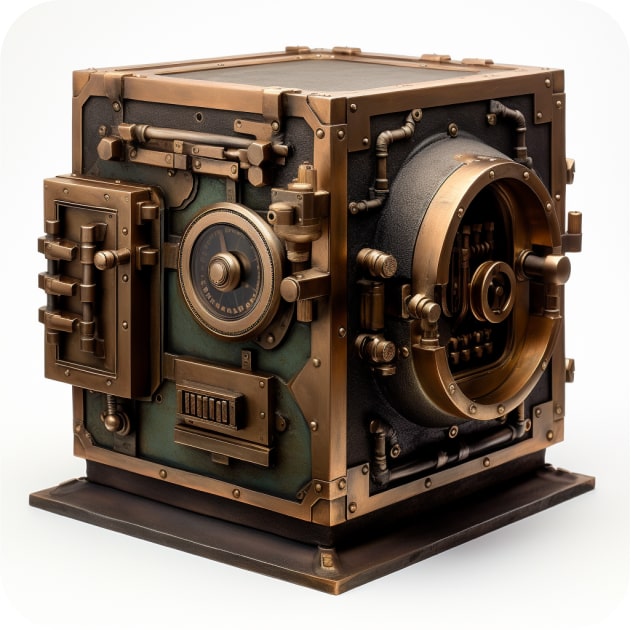
Batch Processing
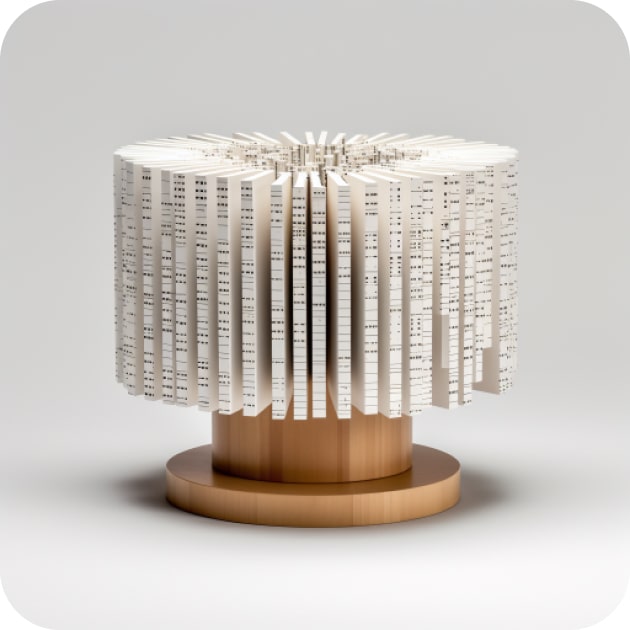
Cache
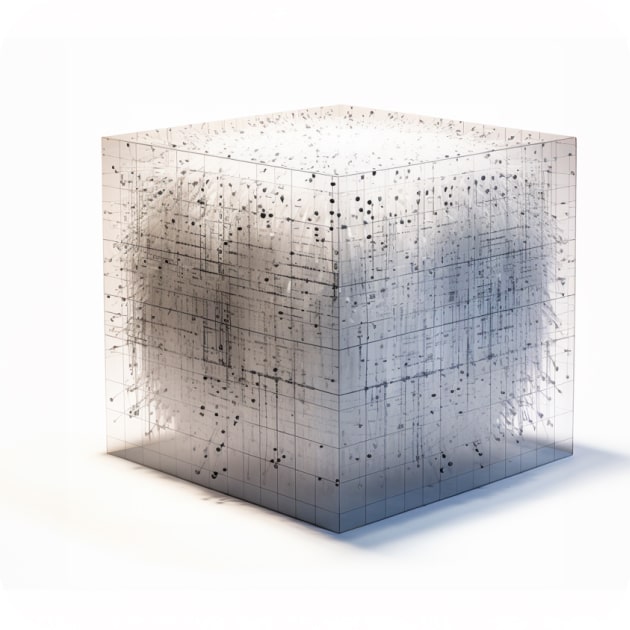
Categorize
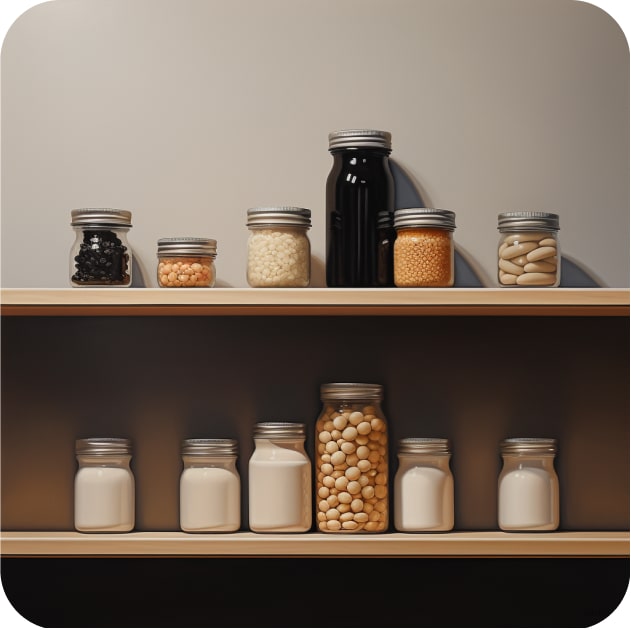
Deduplicate
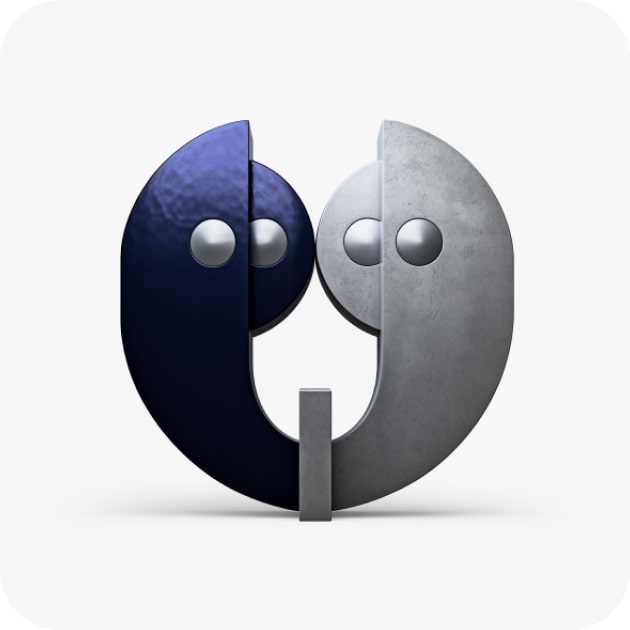
Deserialize
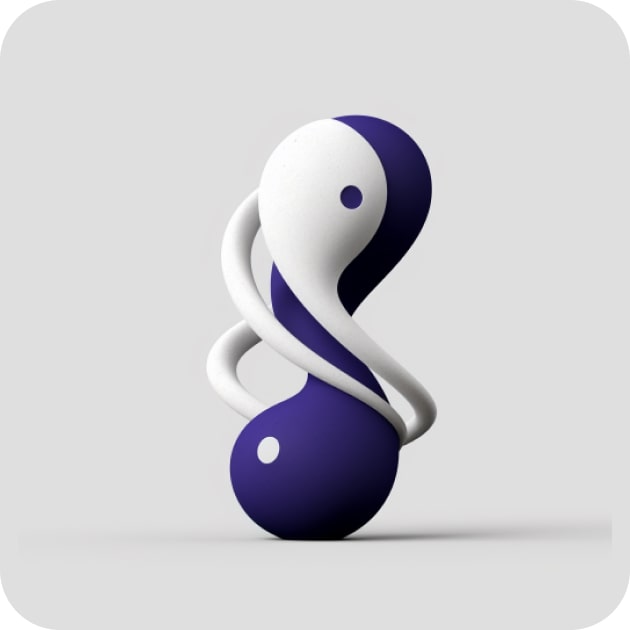
Dimensionality
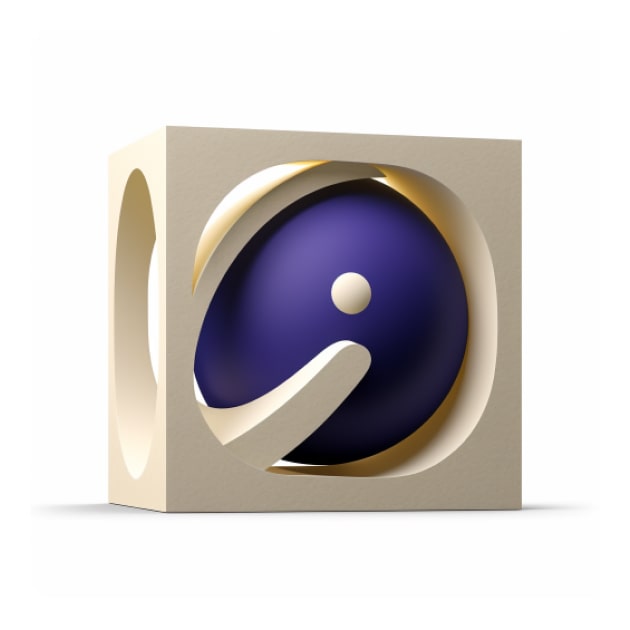
Encapsulate
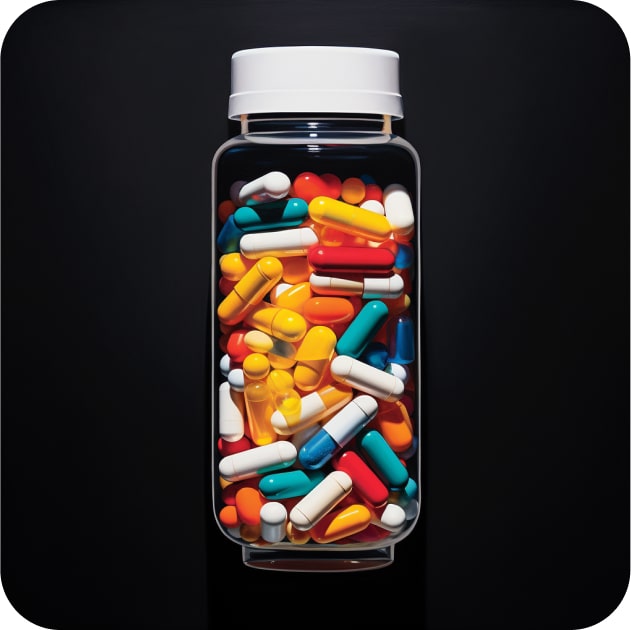
Enrich
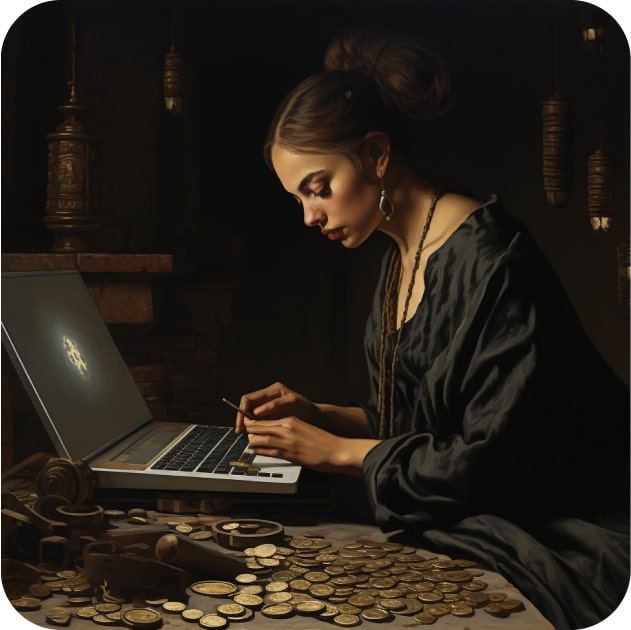
Export
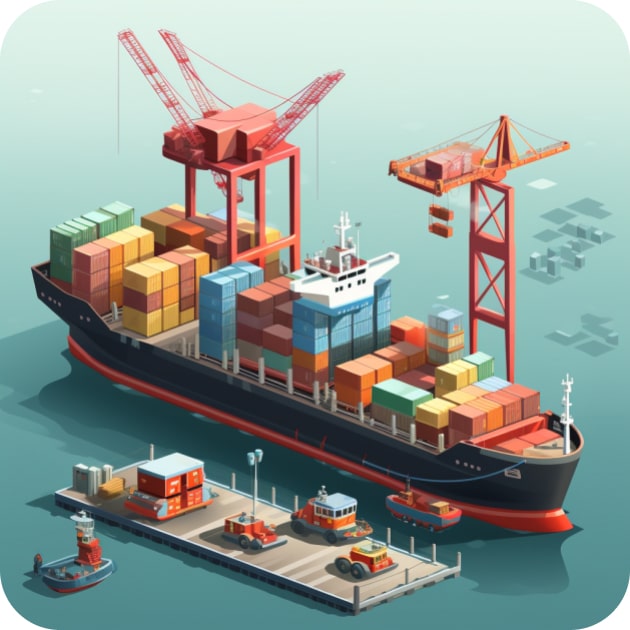
Graph Theory
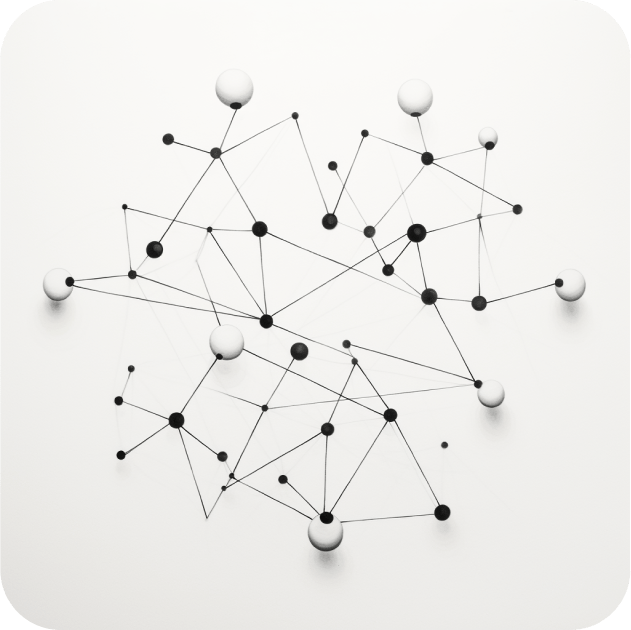
Idempotent
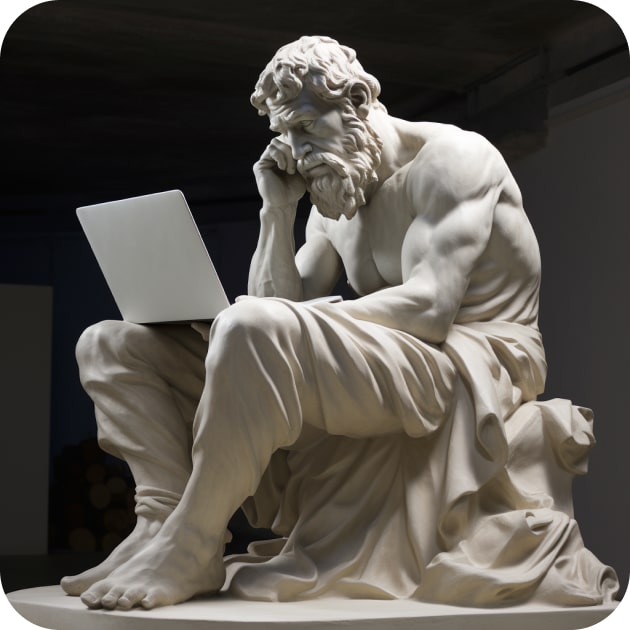
Index
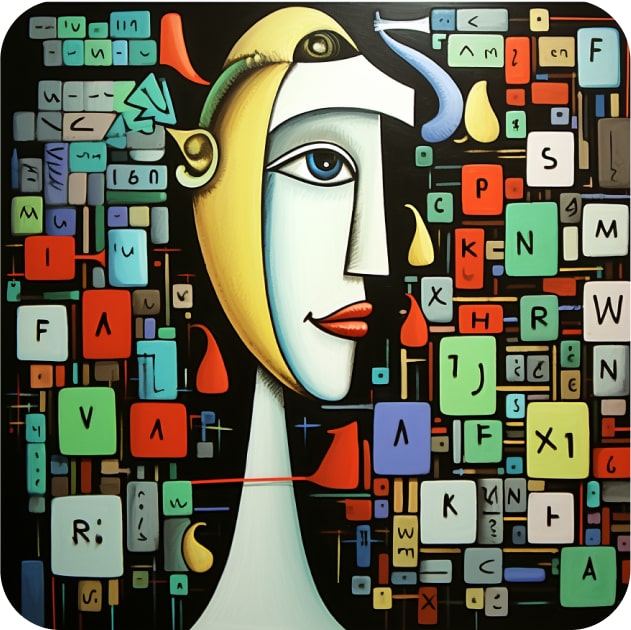
Integrate
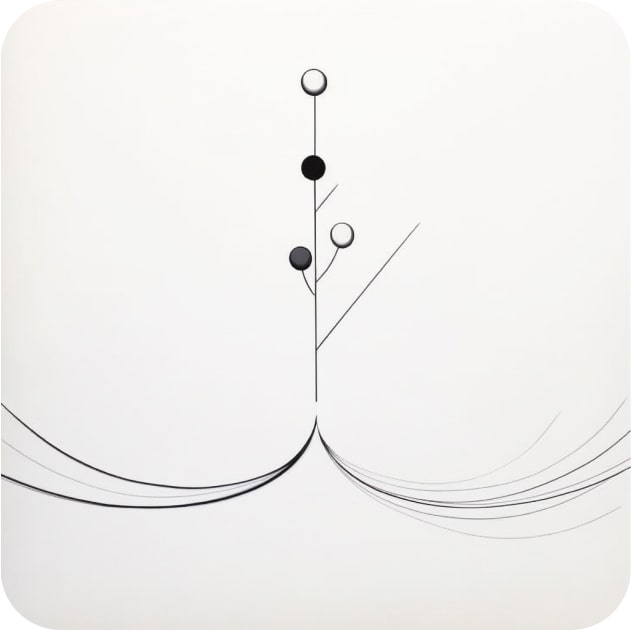
Lineage
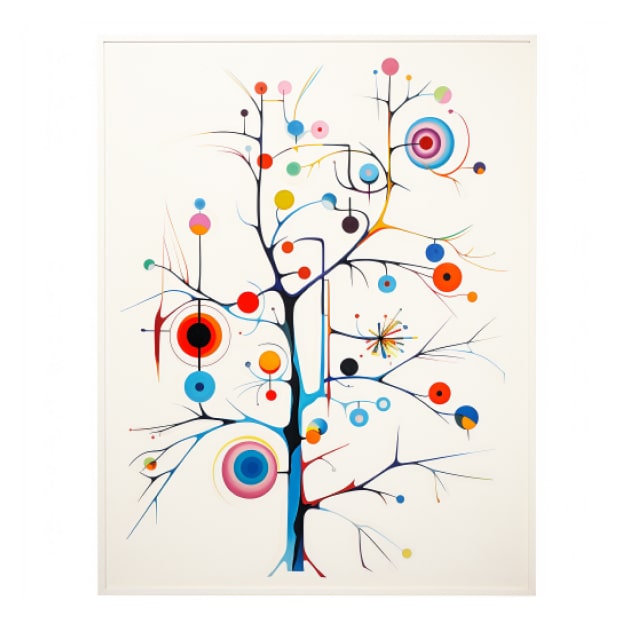
Linearizability
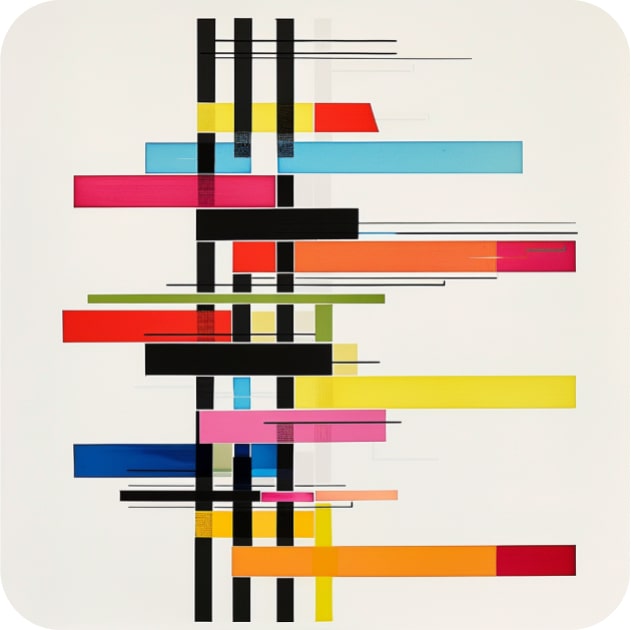
Materialize
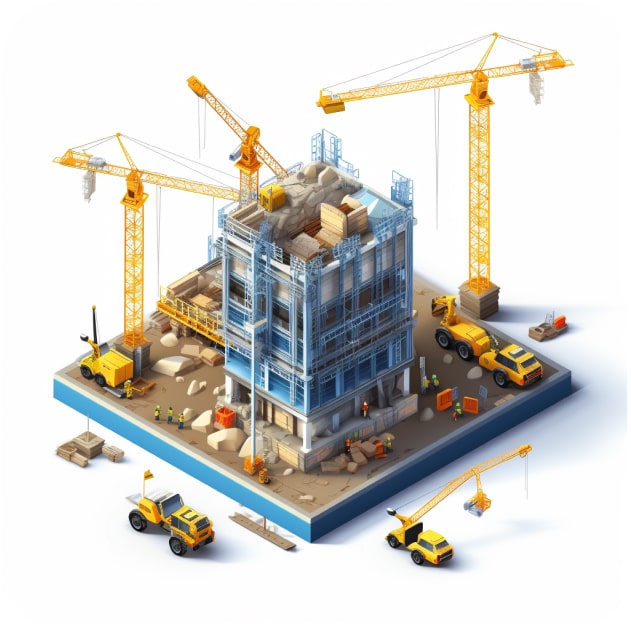
Memoize
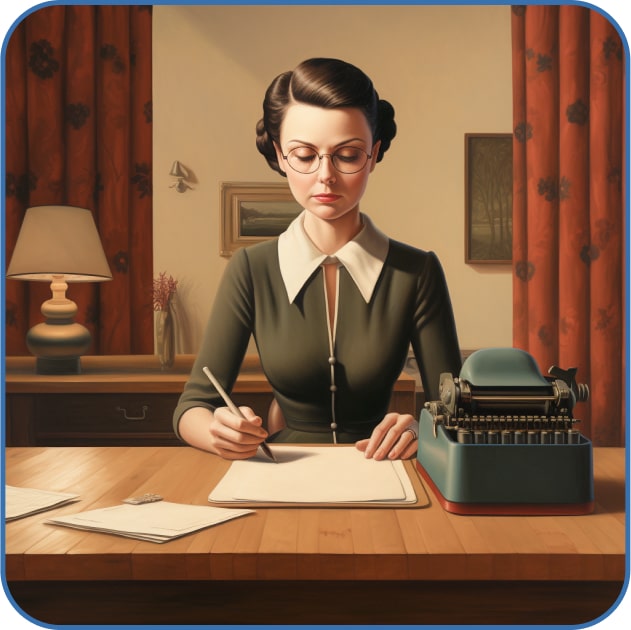
Merge
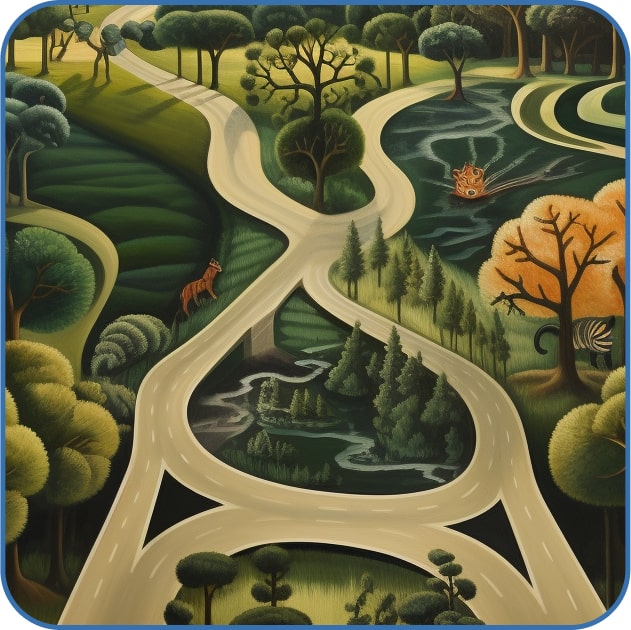
Monitor
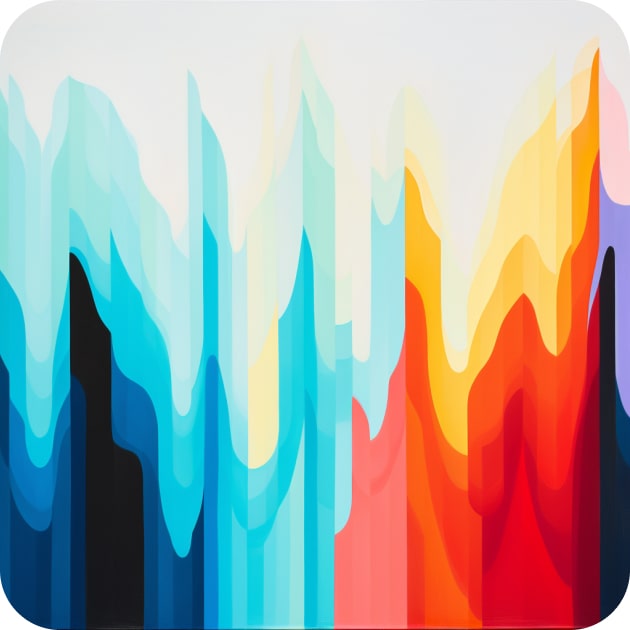
Named Entity Recognition
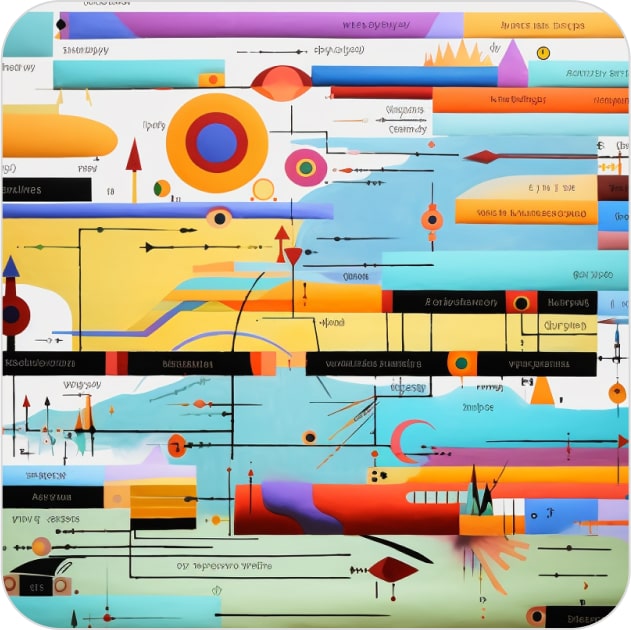
Parse
Partition
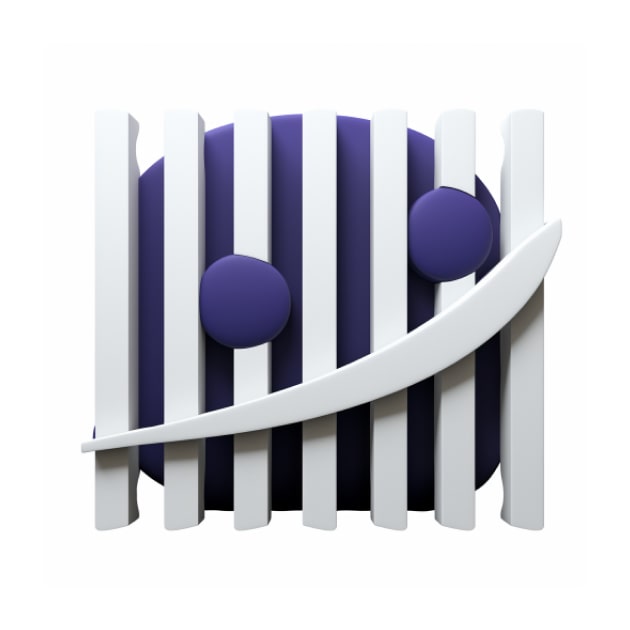
Prep
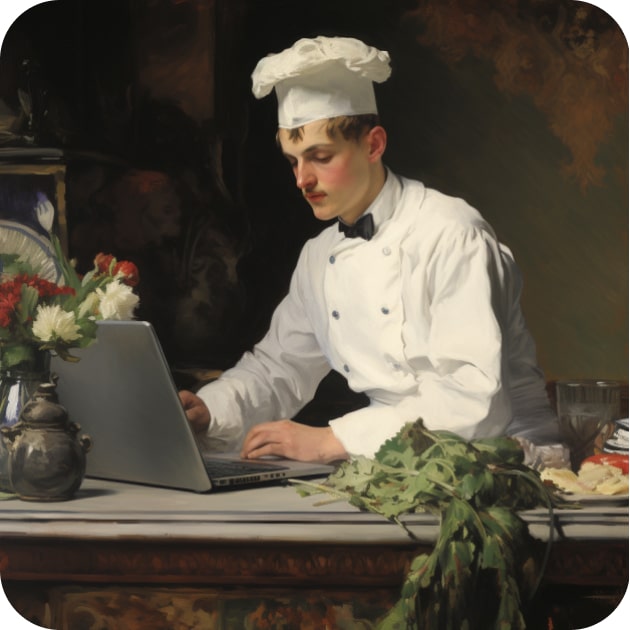
Preprocess
Replicate
Scaling
Schema Inference
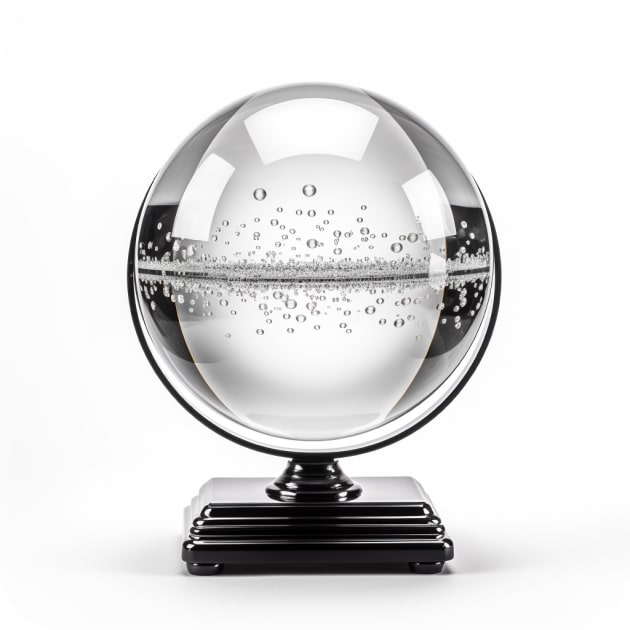
Schema Mapping
Secondary Index
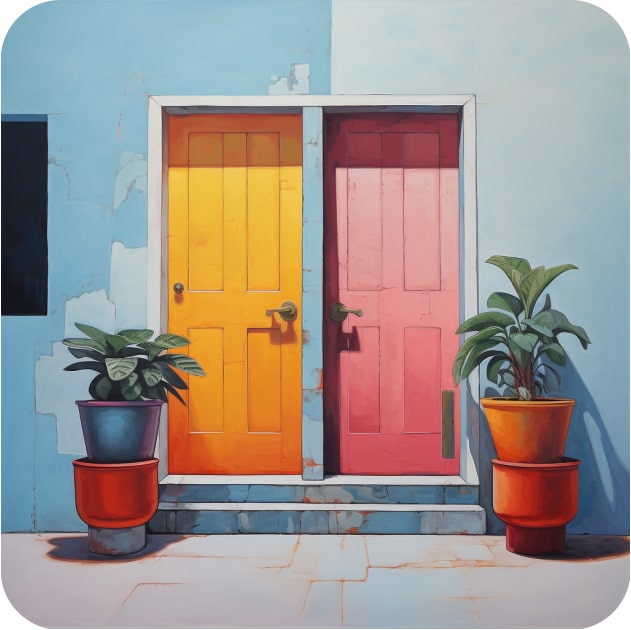
Software-defined Asset
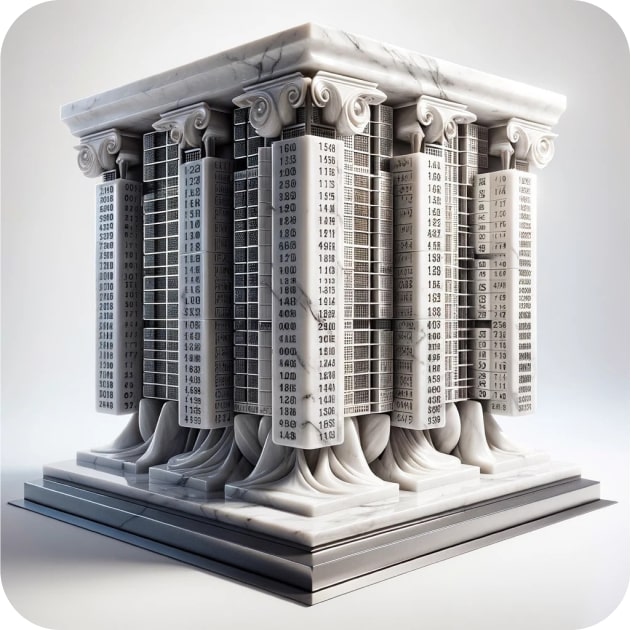
Synchronize
Validate
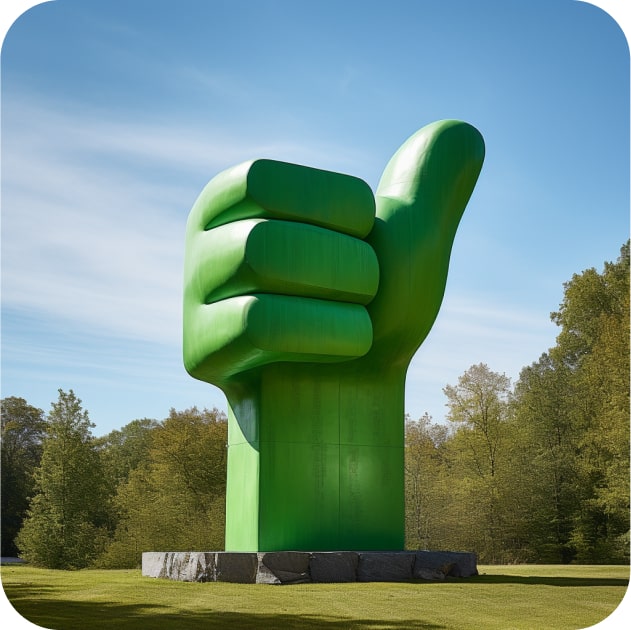
Version
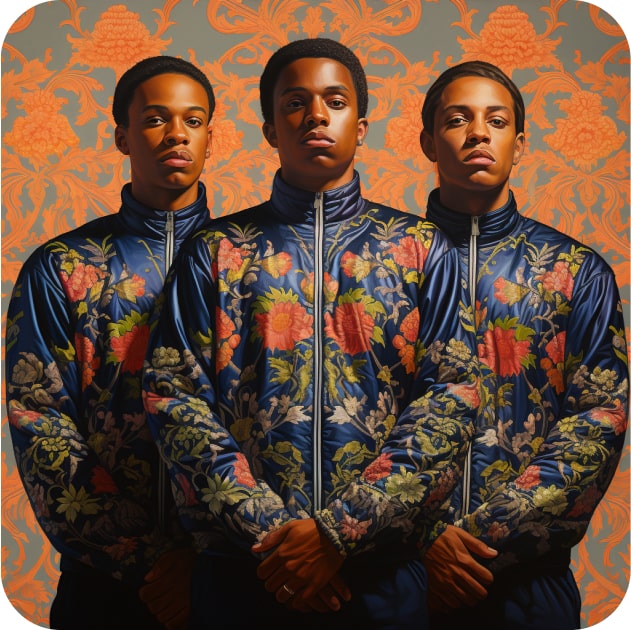