Graph Theory
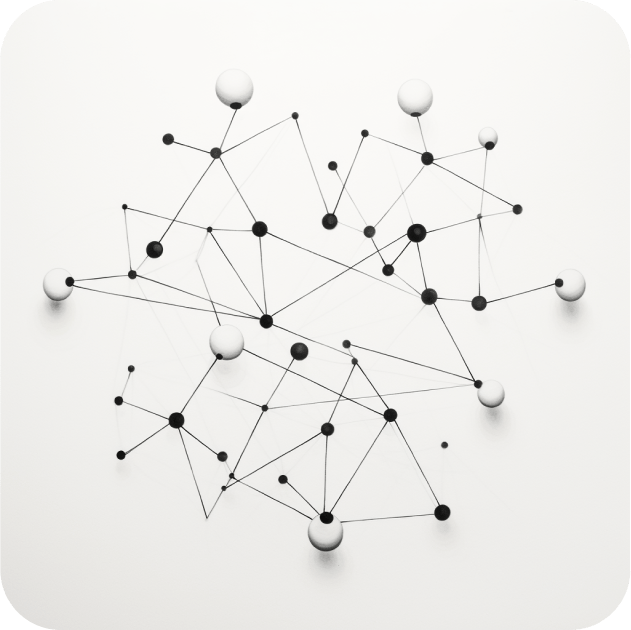
Graph Theory - a Definition:
Graph theory is the study of graphs—mathematical structures used to model pairwise relations between objects1.
The value of Graph Theory in Data Engineering
The objects mentioned in our definition above can be people in a social network, web pages connected by hyperlinks, or, in our case, data processing tasks or data assets. Graph Theory provides a way to represent and study the structure and behavior of data as graphs, which consist of nodes (vertices) and edges (connections) between them.
As data engineers, we're well aware of the complexity that underlies the world of data processing. The pipelines we build are intricate, often resembling complex networks of systems and compute environments, with interconnected tasks or interdependent assets. Graph Theory provides us with a powerful tool to model and understand intricate relationships within our data systems. Think of it as a visual representation that helps us make sense of complex dependencies, optimize workflows, and identify bottlenecks.
In the context of data engineering, graph theory has several important applications:
Data Modeling: Graphs can be used to model complex data relationships where entities and their connections are crucial. For example, nodes might represent tables for products, warehouses, and factories, while edges represent the movement of goods.
Database Design: Graph databases, such as Neo4j, are designed to store and query graph-structured data efficiently. They are particularly well-suited for scenarios where relationships between data points are as important as the data itself. Graph databases enable efficient traversal of relationships, making them valuable in recommendation engines, fraud detection, and social network analysis.
Data Integration: When dealing with data from multiple sources, graph theory can help model the relationships and dependencies between different datasets. This can be useful in building data pipelines and ETL (Extract, Transform, Load) processes, ensuring that data from various sources is integrated and transformed correctly.
Network Analysis: Graph theory is widely used in analyzing and optimizing network structures. This can be applied to data engineering in scenarios like optimizing data flows in a distributed system, identifying bottlenecks, or modeling dependencies between data processing tasks in a workflow.
Semantic Data Modeling: In semantic data modeling, graphs can be used to represent ontologies, taxonomies, or knowledge graphs. These are crucial for applications like natural language processing, recommendation systems, and data-driven decision-making.
Dependency Resolution: When dealing with data pipelines, dependencies between tasks or stages can be complex. Graph theory can help model and visualize these dependencies, making it easier to schedule, monitor, and troubleshoot data processing workflows.
Data Quality and Lineage: Graphs can be used to track the lineage of data, showing how data flows from its source to its consumption. This lineage tracking is valuable for data governance, auditing, and ensuring data quality.
Data Exploration and Visualization: In data analysis and exploration, graph theory can help visualize complex relationships within the data, making it easier to discover patterns, anomalies, and insights.
Directed Acyclic Graphs (DAGs)
One of the most significant applications of Graph Theory in data engineering is the use of Directed Acyclic Graphs, or DAGs. DAGs are a specific type of graph where edges have a direction, and there are no cycles (i.e., no way to start at a node and return to it by following the edges).
Why are DAGs so important to data engineers? Because they are a natural fit for modeling data processing workflows. Each node in a DAG represents a task or step in the workflow, and directed edges between nodes indicate task dependencies.
The absence of cycles ensures that the workflow can be executed without causing infinite loops or circular dependencies. In the diagram below, the relationships defined in examples 1 and 2 are valid non-cyclical flows, whereas example 3 breaks this rule.
Designing our data pipelines with DAGs supports some fundamental capabilities, including workflow optimization, dependency management, data lineage, fault tolerance/retries, and parallelism.
How Dagster Does Graphs
Dagster takes a unique approach to graphs thanks to its asset-centric framework.
Dagster helps you define, in a declarative way, the key data assets that your organization requires. It then builds a graph of dependencies and manages the relationships, lineage, prioritization, and observability for you.
Dagster manifests this graph of assets directly within the product. The asset graph is the center of the programming model, the subject of the development lifecycle, the seat of metadata, and the operational single pane of glass.
An example of Graph Theory using Python
Let's illustrate the concept of Graph Theory using Python and matplotlib
.
Imagine you are managing a complex data processing pipeline with multiple tasks, each with its dependencies. You want to optimize the execution of these tasks to minimize the overall processing time.
We can model the data processing pipeline as a DAG, where nodes represent tasks, and directed edges represent dependencies between tasks. We can then use topological sorting to find the optimal order in which to execute these tasks, considering their dependencies.
import networkx as nx
import matplotlib.pyplot as plt
# Create a Directed Acyclic Graph (DAG) representing the data processing pipeline
data_pipeline = nx.DiGraph()
# Define tasks and their dependencies
tasks_and_dependencies = {
"Task A": [],
"Task B": ["Task A"],
"Task C": ["Task A"],
"Task D": ["Task B", "Task C"],
"Task E": ["Task D"],
"Task F": ["Task D"],
"Task G": ["Task E", "Task F"],
}
# Add nodes and edges to the DAG
for task, dependencies in tasks_and_dependencies.items():
data_pipeline.add_node(task)
for dependency in dependencies:
data_pipeline.add_edge(dependency, task)
# Visualize the DAG (optional)
pos = nx.spring_layout(data_pipeline, seed=42)
nx.draw(data_pipeline, pos, with_labels=True, node_size=800, node_color="skyblue", font_size=10)
plt.title("Data Processing Workflow DAG")
plt.show()
# Find the optimal execution order using topological sorting
optimal_execution_order = list(nx.topological_sort(data_pipeline))
# Print the optimal execution order
print("Optimal Execution Order of Tasks:")
for task in optimal_execution_order:
print(task)
In this example:
- We create a DAG data_pipeline to represent the data processing pipeline, where tasks are nodes and dependencies are directed edges.
- We define the tasks and their dependencies in the tasks_and_dependencies dictionary.
- We add nodes and edges to the DAG based on the defined dependencies.
- Optionally, we visualize the DAG to gain a visual representation of the data processing workflow.
- We use nx.topological_sort from NetworkX to find the optimal execution order of tasks. Topological sorting ensures that tasks are executed in a way that respects their dependencies and minimizes the overall processing time.
- Finally, we print the optimal execution order of tasks, which is the order in which they should be executed to optimize the pipeline.
Here is what our result looks like:
This example demonstrates how graph theory, along with topological sorting, can be used to optimize complex data processing workflows in data engineering, ensuring efficient execution while respecting task dependencies.
Conclusion
As data engineers, our mission is to build robust, efficient, and scalable data processing pipelines. Graph Theory, especially through the lens of Directed Acyclic Graphs (DAGs), provides us with a framework to achieve these goals. By understanding the relationships and dependencies within our data systems, we can design and optimize workflows that meet the demands of the ever-growing data landscape. So, the next time you're architecting a data pipeline, remember that Graph Theory and DAGs are your trusted companions on the journey to data engineering excellence.
Learn more about Dagster's Graph-backed Assets in the Dagster docs.
[1] See Wikipedia entry for Graph Theory
Append
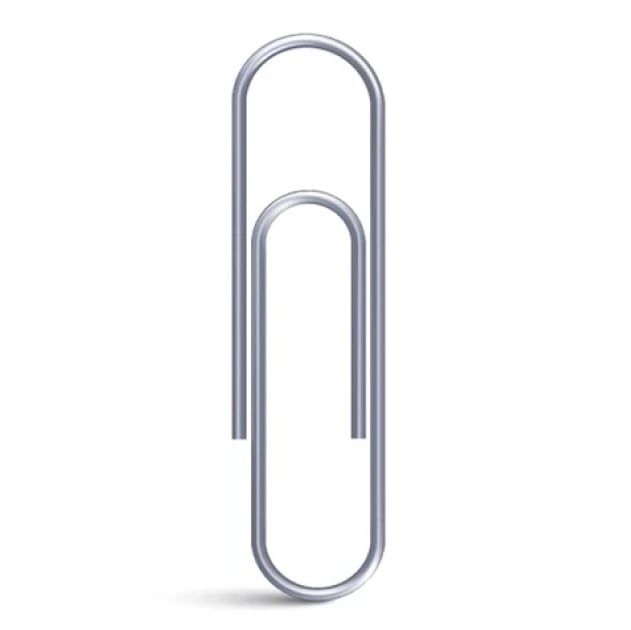
Archive
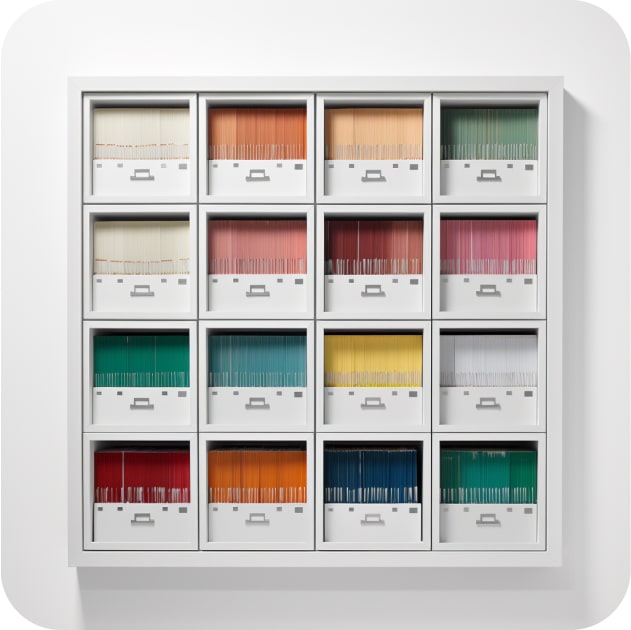
Augment
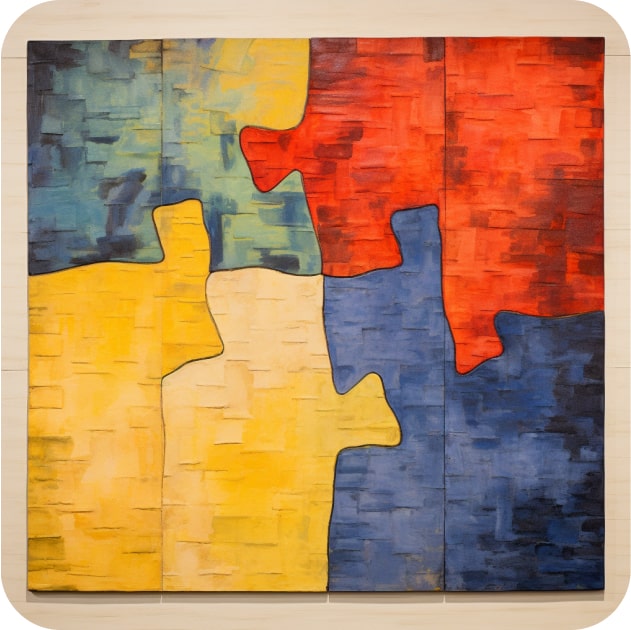
Auto-materialize
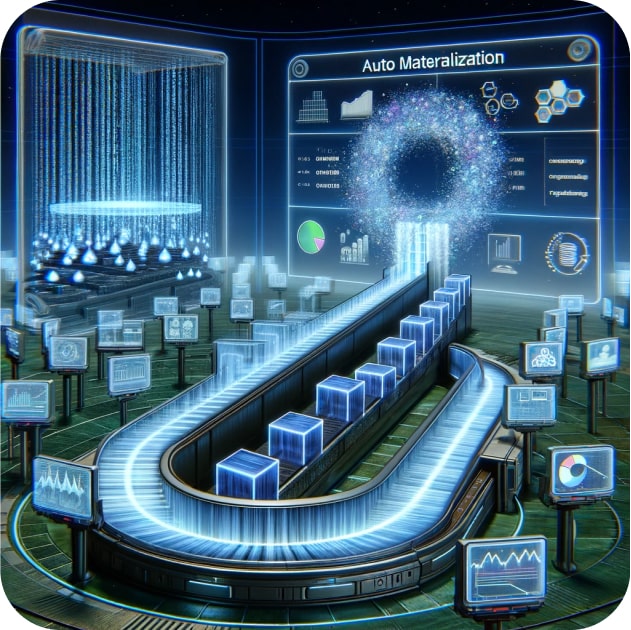
Backup
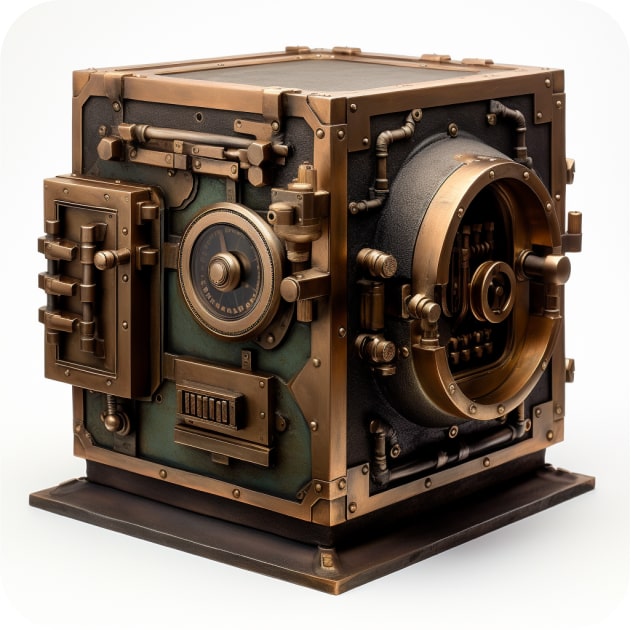
Batch Processing
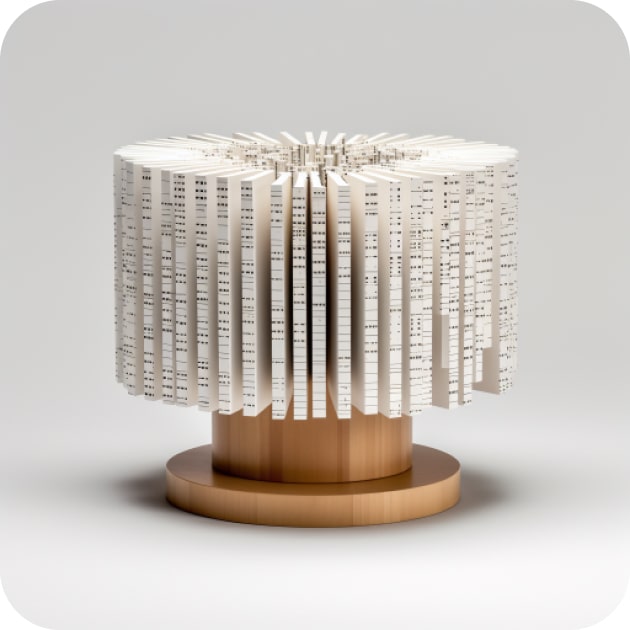
Cache
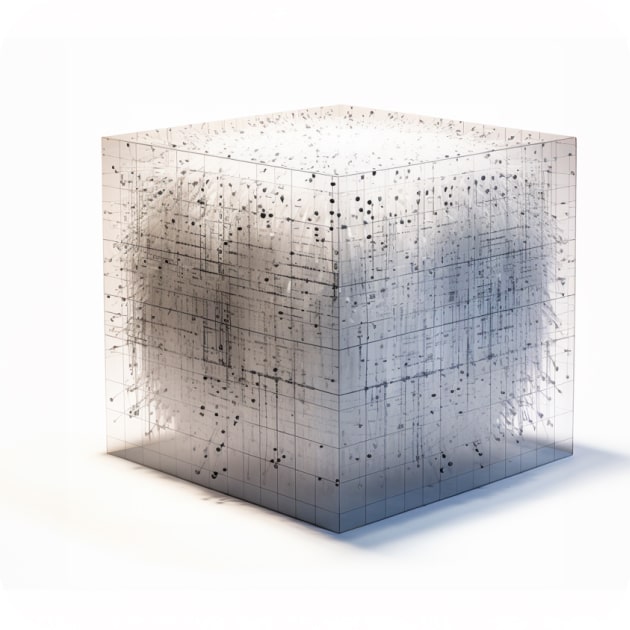
Categorize
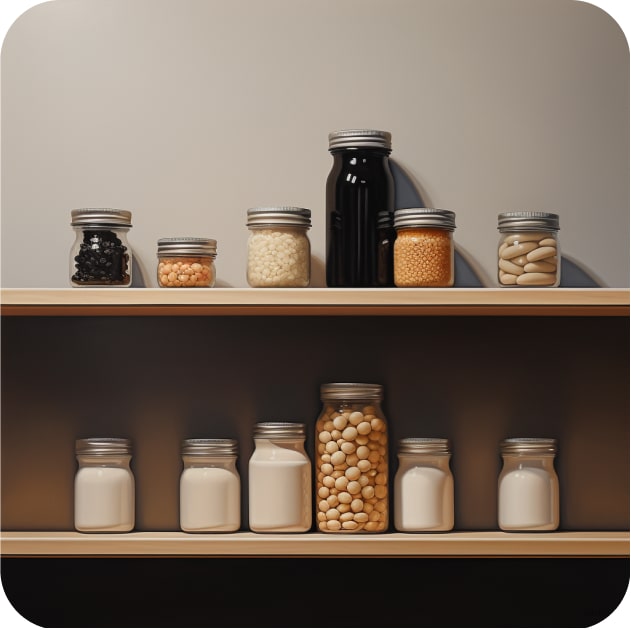
Deduplicate
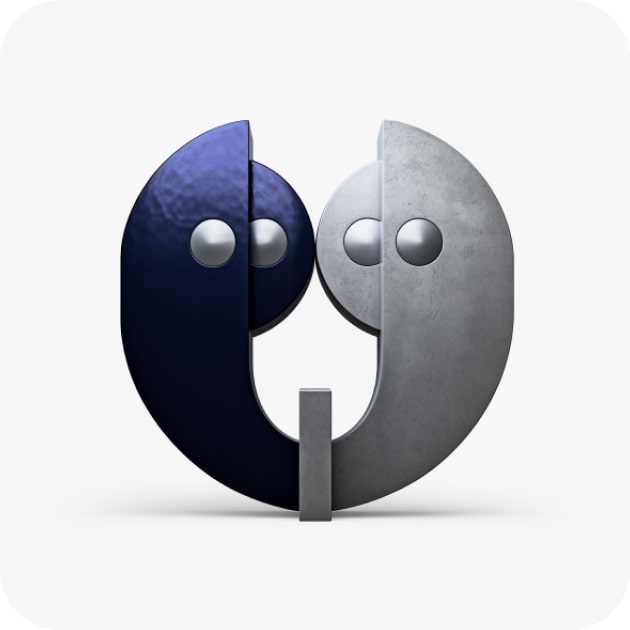
Deserialize
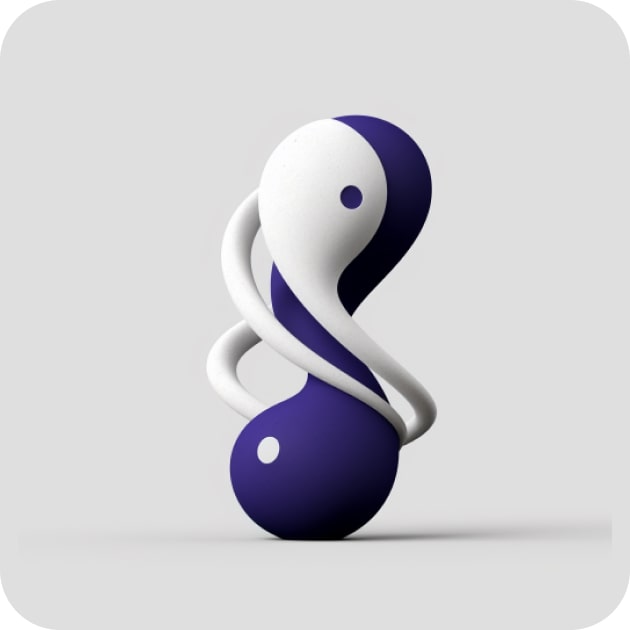
Dimensionality
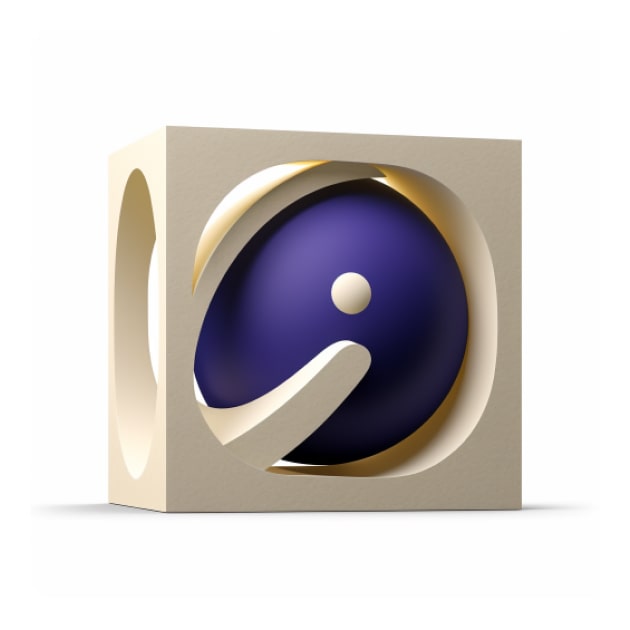
Encapsulate
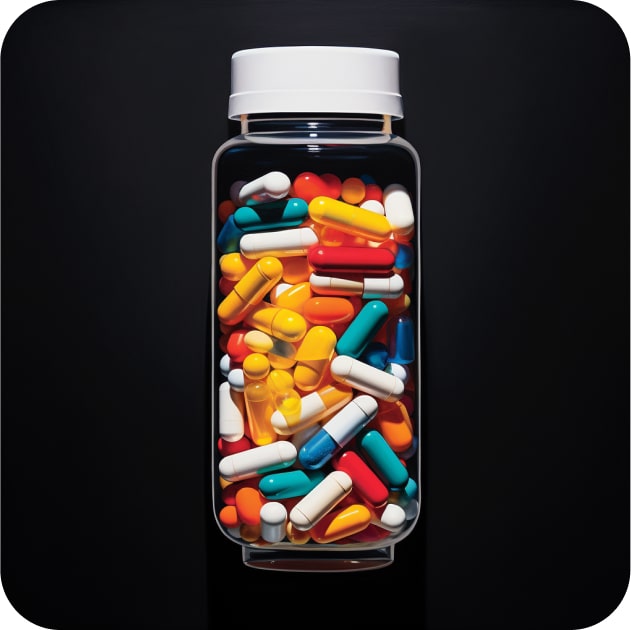
Enrich
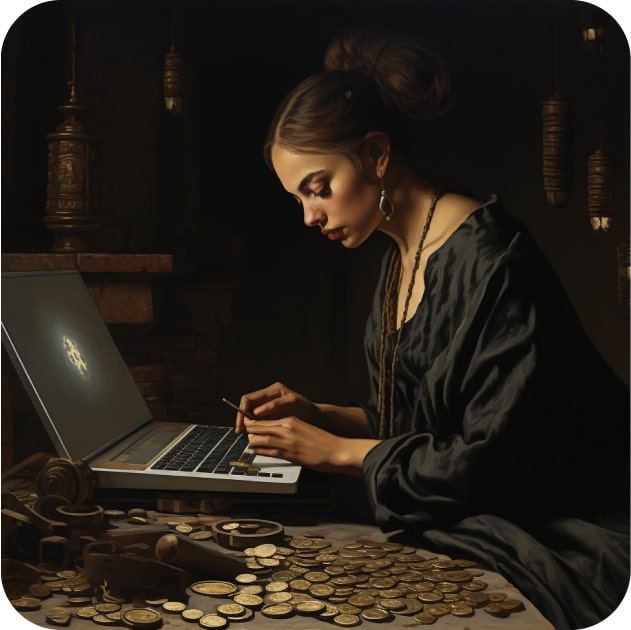
Export
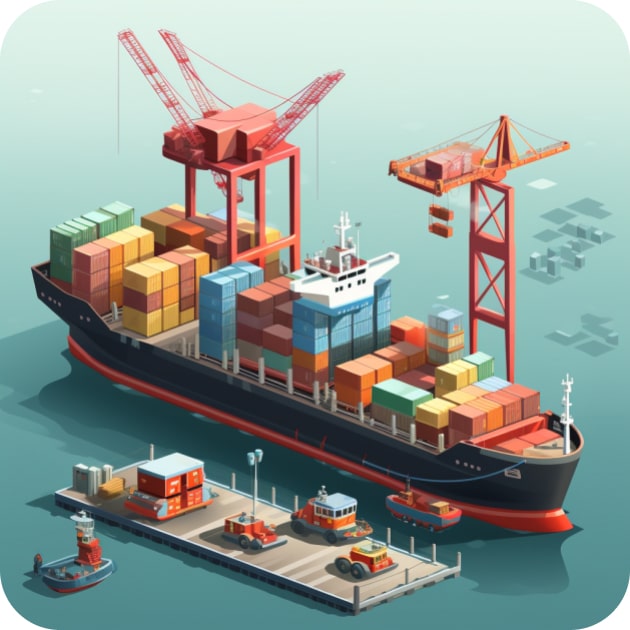
Idempotent
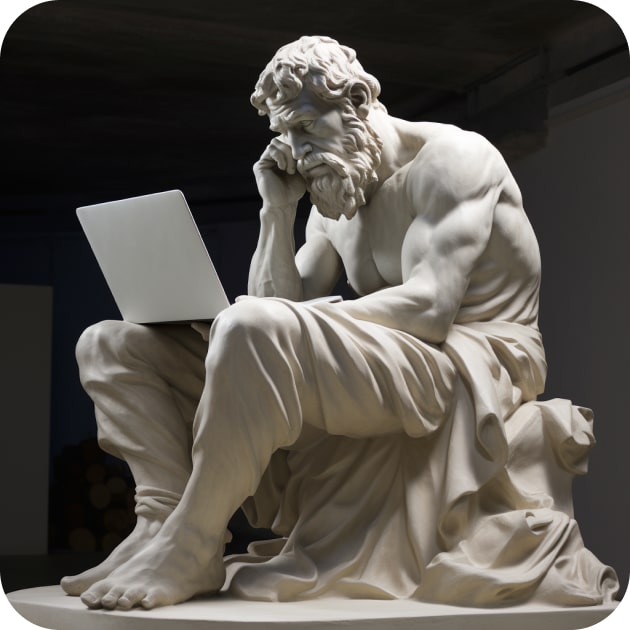
Index
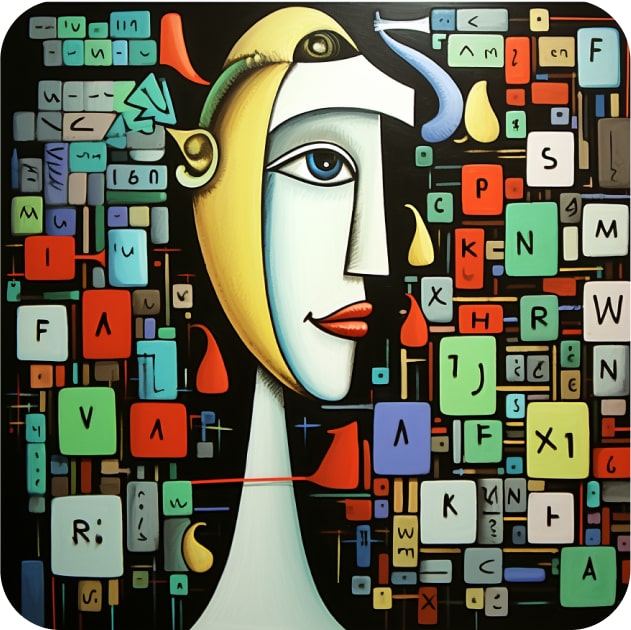
Integrate
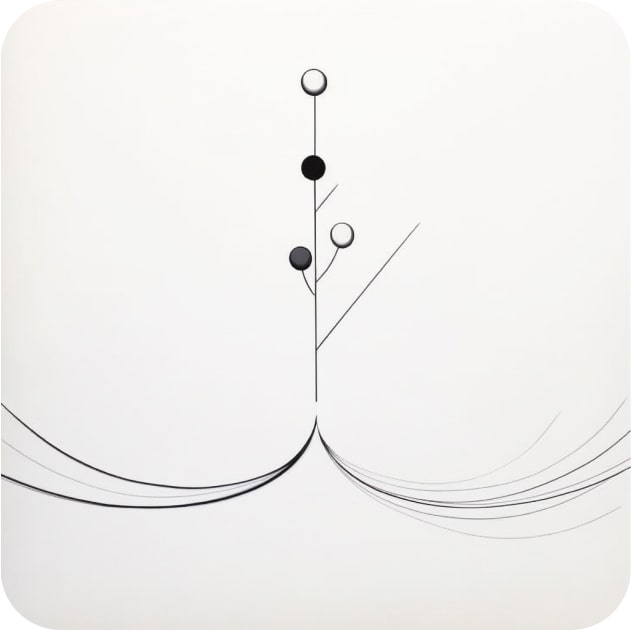
Lineage
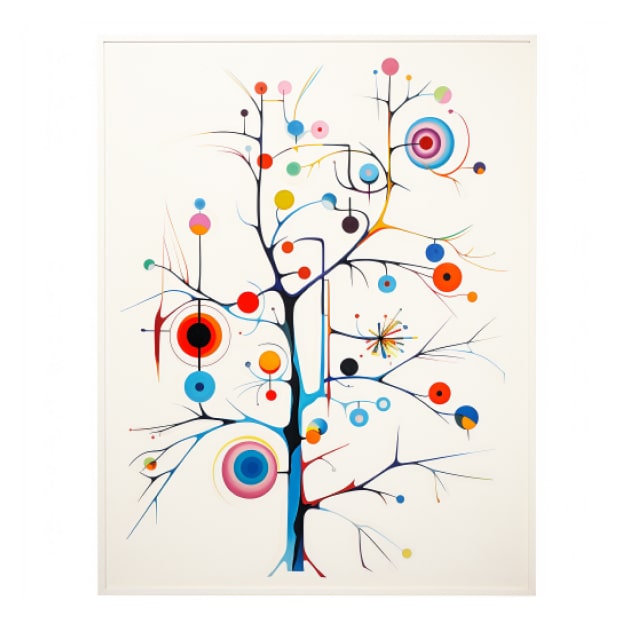
Linearizability
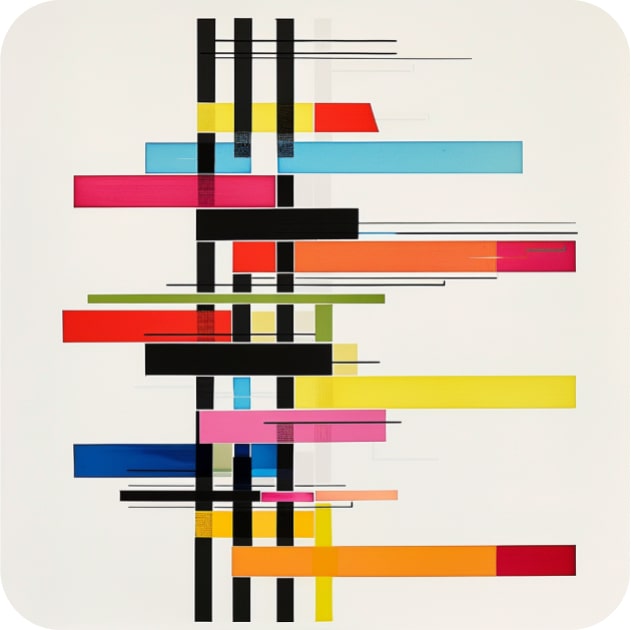
Materialize
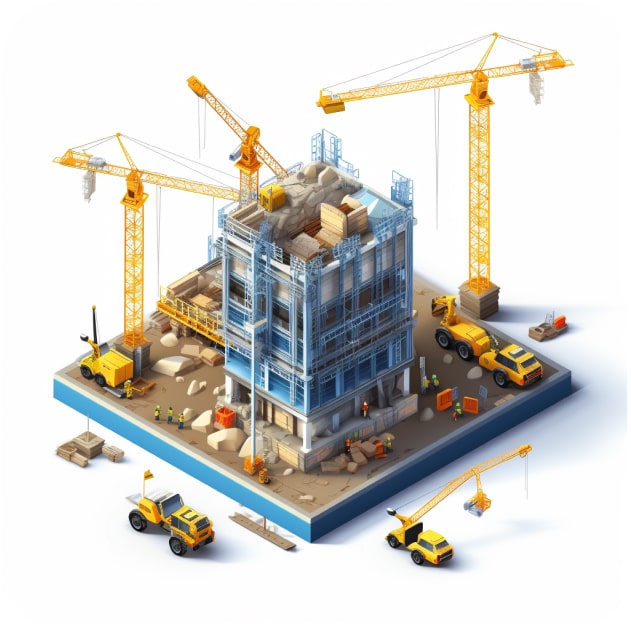
Memoize
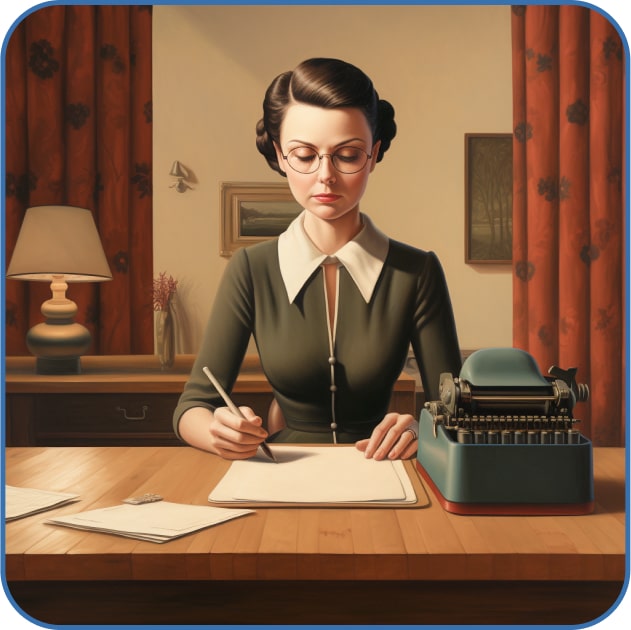
Merge
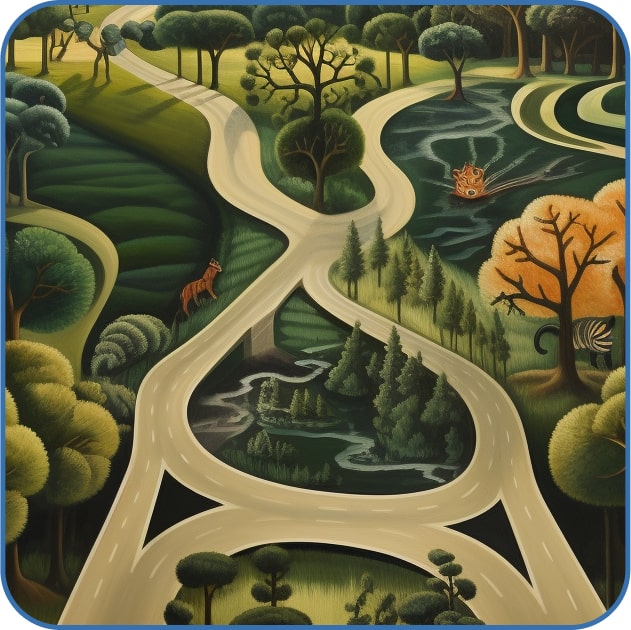
Model
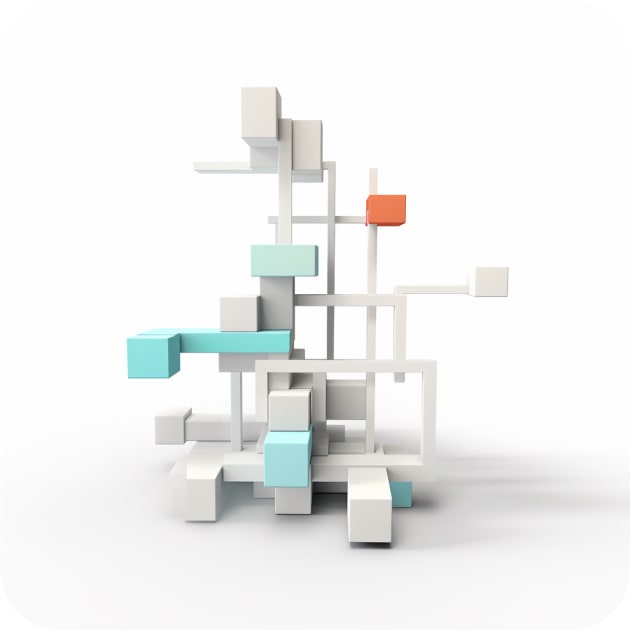
Monitor
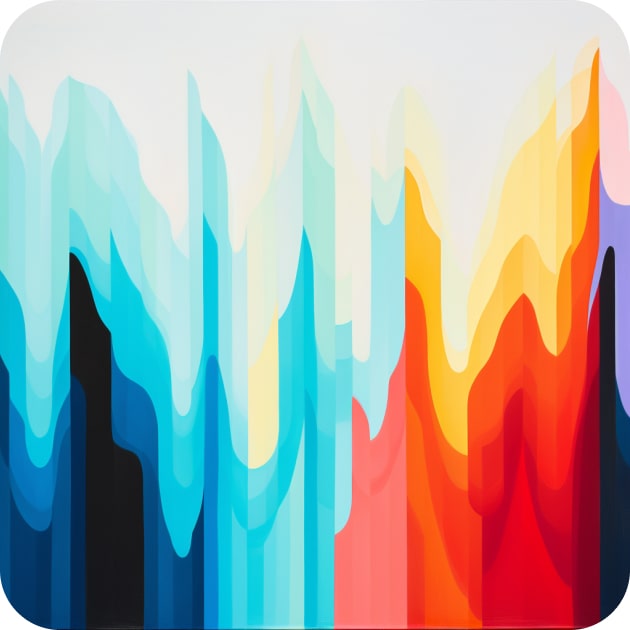
Named Entity Recognition
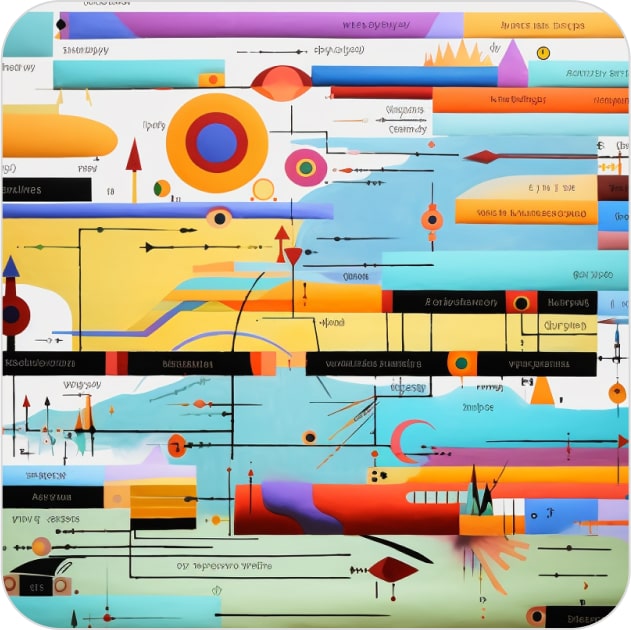
Parse
Partition
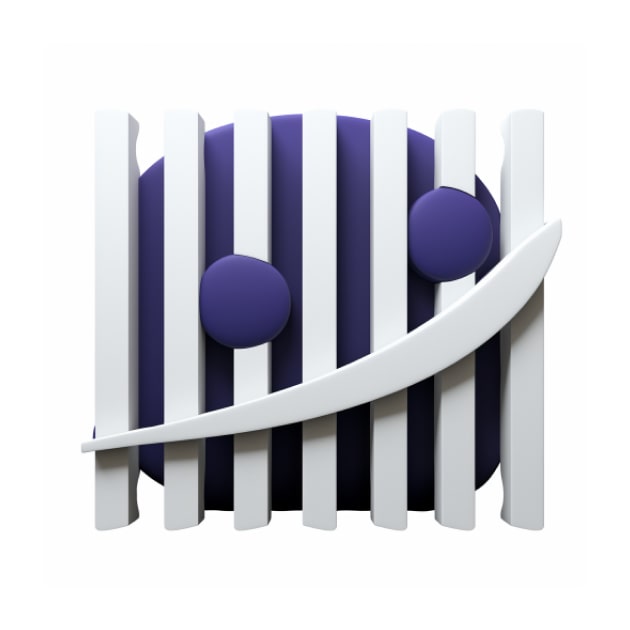
Prep
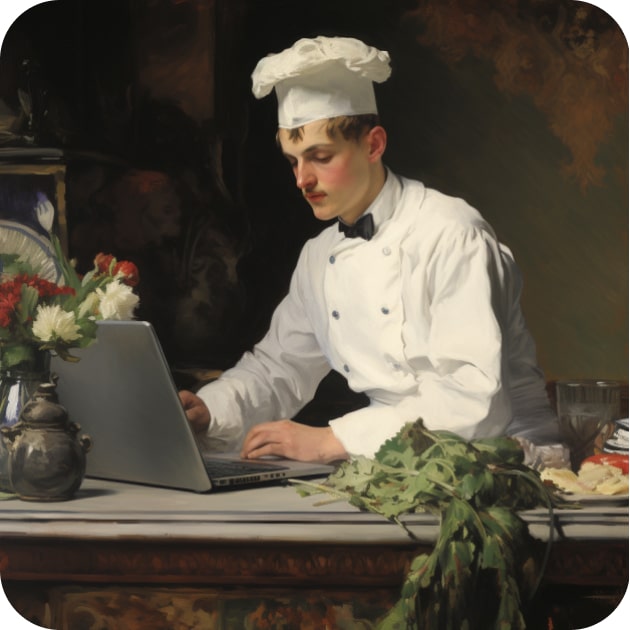
Preprocess
Replicate
Scaling
Schema Inference
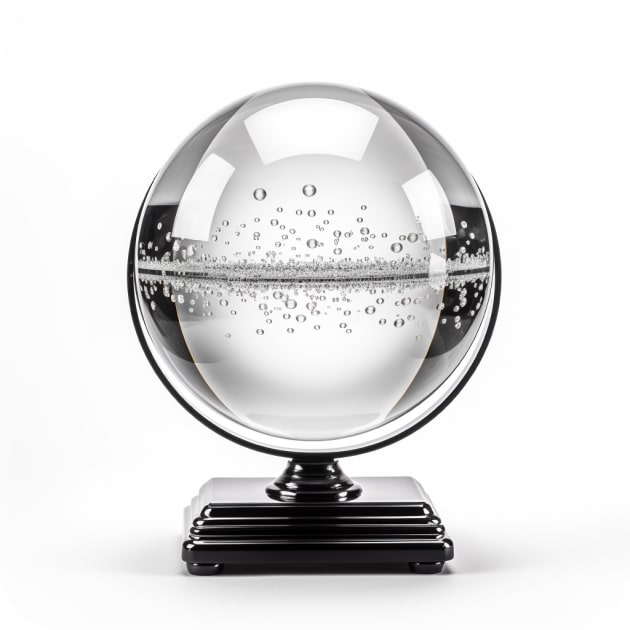
Schema Mapping
Secondary Index
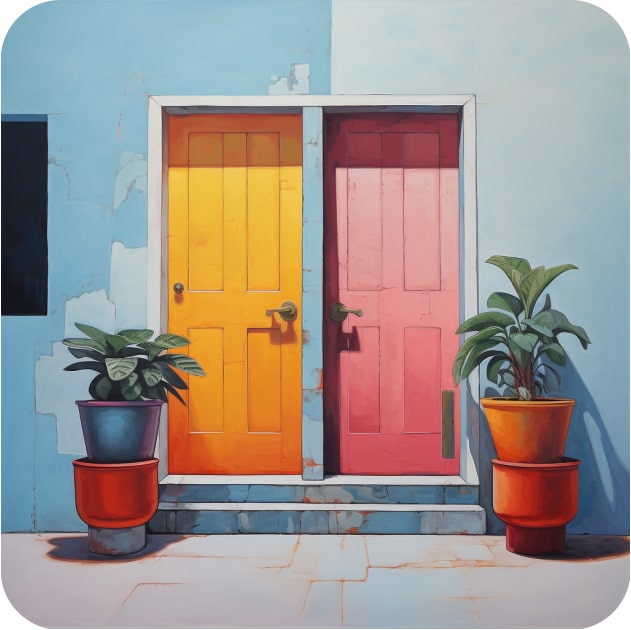
Software-defined Asset
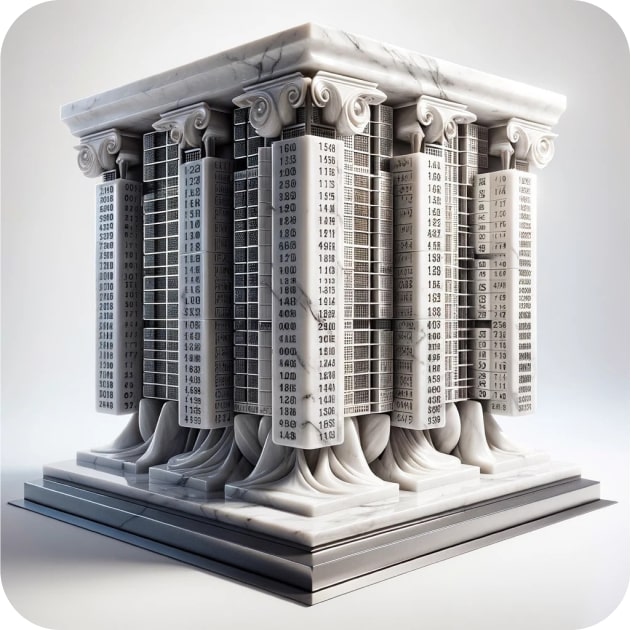
Synchronize
Validate
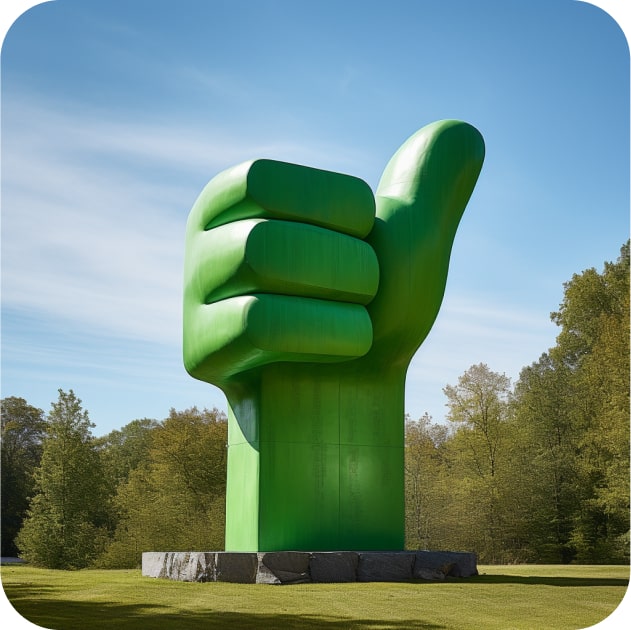
Version
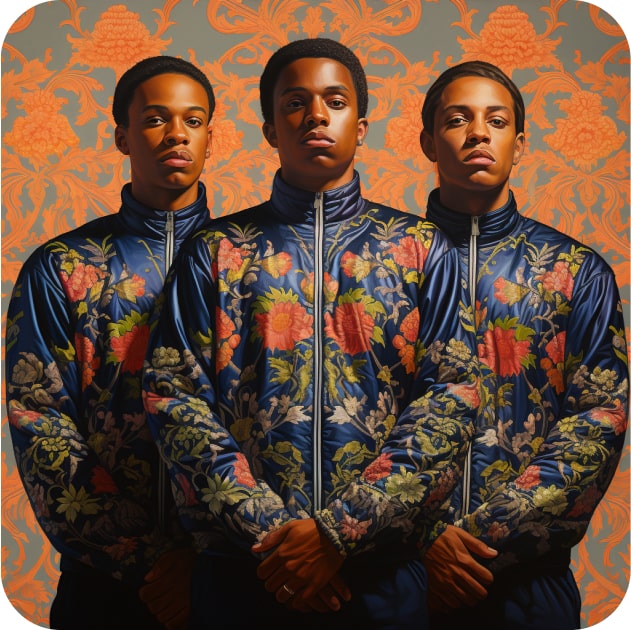