Data Append
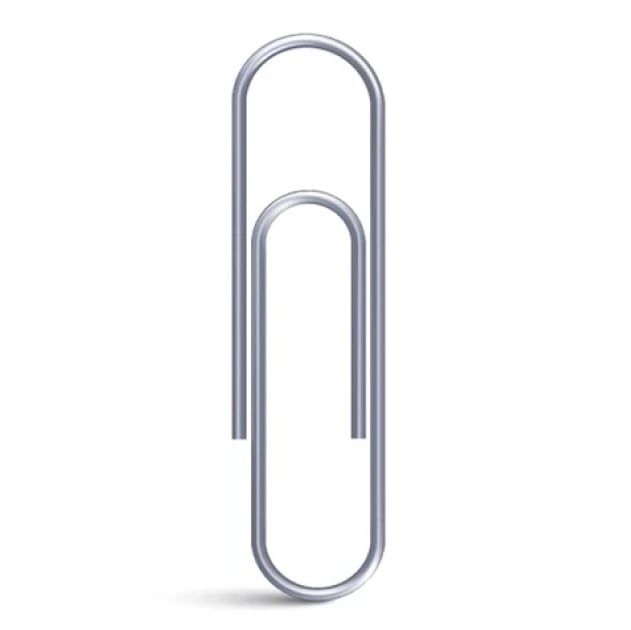
A definition of data appending
In the context of data engineering, "append" typically refers to the operation of adding or attaching new records or data items to the end of an existing dataset, database table, file, or list.
In distributed data processing systems like Apache Hadoop or Spark, appending data is a common operation when aggregating or processing large volumes of data. The append operation ensures that new information is added in sequence, maintaining the integrity and order of the dataset. For example, appending can be used to add new rows to a table in a database or new entries to a log file without modifying or deleting the existing content.
For data engineers working on complex data pipelines, data appending is a crucial and delicate operation, especially when dealing with streaming data or batch-processing workflows in both real-time and batch processing systems.
Data appending is not just about adding records to the end of a dataset. It involves considerations around consistency, efficiency, scalability, and reliability, especially when dealing with complex data pipelines in distributed and high-volume environments. Balancing these factors is critical for building robust and efficient data pipelines that can seamlessly handle the continuous influx of new data while maintaining the integrity and utility of the existing datasets.
Appending vs. merging
Appending and merging are related but separate operations. In appending, we bring together two datasets with similar structures, whereas merging requires aligning the data and creating the rules for deduplicating and clensing data during the merge.
Data appending use cases:
There are many use cases for data appending in data engineering including:
Log Aggregation: Appending log entries from various services to a centralized log store for analysis, monitoring, and troubleshooting.
Time-series Databases: Appending new time-stamped records in time-series databases for monitoring and analyzing trends over time.
Stream Processing: Continuously appending data points from streaming sources to datasets or tables for real-time analysis and processing.
Batch Processing: Accumulating and appending processed records in batch-oriented workflows to build up comprehensive datasets for analytics and reporting.
ETL (Extract, Transform, Load): Data appending is often used in the context of ETL processes where data from various sources is ingested, transformed, and loaded into a data warehouse, data lake, or other storage solutions.
Key considerations in data appending:
When appending data, engineers often deal with structured or semi-structured data formats such as JSON, CSV, Avro, or Parquet. As you append new records to the end of an existing dataset or file, you must ensure that the schema is consistent and that any transformations or enrichments applied to the new data are in line with existing records.
Data Integrity and Consistency: It is essential to maintain data integrity and schema consistency when appending new records, avoiding data corruption or discrepancies which can impact downstream analytics or applications.
Concurrency and Locking: In environments where multiple processes or threads may be trying to append data simultaneously, managing concurrent writes and implementing appropriate locking mechanisms are crucial to prevent data loss or corruption.
Indexing and Partitioning: Proper indexing and partitioning strategies must be considered to efficiently append and query data, especially in large-scale distributed storage systems.
Error Handling: Robust error handling mechanisms need to be in place to deal with failures during the append operation, such as network issues, disk failures, or schema mismatches.
Performance: The append operation should be optimized to ensure high throughput and low latency, particularly in real-time data processing pipelines where the timely addition of data is critical.
Data Retention and Storage Management: As appending accumulates more data, managing storage resources, implementing data retention policies, and optimizing storage costs become essential.
Idempotency and Deduplication: Ensuring that the append operation is idempotent and implementing deduplication strategies are crucial in situations where data might be replayed or where duplicate records need to be avoided.
"Big Data" Technologies:
When dealing with big data, numerous technologies are available that enable efficient and scalable data appending. The diverse range of technologies for data appending in big data ecosystems facilitates the development of scalable, efficient, and robust data pipelines, capable of handling varying workloads and data types. Selecting the appropriate stack of technologies is paramount and should align with the specific requirements and constraints of the given big data project.
- Data engineers often use distributed computing frameworks like Apache Spark or Apache Flink for appending data in scalable and fault-tolerant manners.
- Distributed file systems like HDFS or cloud-based storage solutions like Amazon S3 are often used as the underlying storage where data is appended.
- Databases like Apache Cassandra and Apache HBase are used when a combination of write and read efficiency is needed for appending operations.
An example of data appending in Python
To demonstrate data appending using Apache Spark in Python, let's consider a scenario where we have an existing Parquet file (existing_data.parquet) in a distributed file system, and we have a new batch of data in a CSV file (new_data.csv) that we need to append to the existing Parquet file.
For this illustration we will first run a short script that will generate both a local Parquet data and our new .csv file:
from pyspark.sql import SparkSession
from pyspark.sql import Row
import csv
# Define the path for the new CSV file
file_path = 'radiohead_data.csv'
# Define the data to be written to the CSV file
data = [
['id', 'name', 'created_date', 'role'], # Header row
[1, 'Thom Yorke', '1968-10-07', 'vocals'],
[2, 'Jonny Greenwood', '1971-10-05', 'guitar'],
[3, 'Ed OBrien', '1968-04-15', 'guitar'],
]
# Write to the CSV file
with open(file_path, mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
print(f'{file_path} has been created successfully.')
# Initialize a Spark session
spark = SparkSession.builder.appName("CreateParquetExample").getOrCreate()
# Sample Data
data = [
Row(id=4, name='Philip Selway', created_date='1967-05-23', role='drums'),
Row(id=5, name='Colin Greenwood', created_date='1969-06-26', role='bass'),
Row(id=2, name='Jonny Greenwood', created_date='1971-10-05', role='guitar'),
Row(id=3, name='Ed OBrien', created_date='', role='vocals'),
]
# Creating a DataFrame from the sample data
df = spark.createDataFrame(data)
# Writing the DataFrame to a Parquet file
df.write.mode('overwrite').parquet('sample_data.parquet')
# Stop the Spark session
spark.stop()
Now that we have our two files we can append the data form the .csv file to the Parquet file.
from pyspark.sql import SparkSession
from pyspark.sql.types import StructType, StructField, StringType, IntegerType, DateType
# Initialize a Spark Session
spark = SparkSession.builder.appName("DataAppendingExample").getOrCreate()
# Define a schema for the CSV file
schema = StructType([
StructField("id", IntegerType(), True),
StructField("name", StringType(), True),
StructField("created_date", DateType(), True),
StructField("role", StringType(), True)
])
# Load the existing Parquet file
existing_data = spark.read.parquet("sample_data.parquet")
existing_data.createOrReplaceTempView("radioheadData")
print(spark.catalog.listTables())
# Load the new data from the CSV file
new_data = spark.read.csv("radiohead_data.csv", schema=schema, header=True)
# Append the new data to the existing data
appended_data = existing_data.union(new_data)
# Perform some transformations if needed, e.g., deduplication
final_data = appended_data.dropDuplicates()
# Write the appended data back to the Parquet file
final_data.write.mode('overwrite').parquet("sample_data.parquet")
# Because the data has changed, we must refresh the table before printing out the data
spark.sql('REFRESH TABLE radioheadData')
# Optionally, you can perform some validations or exploratory data analysis
final_data.show()
# Stop the Spark Session
spark.stop()
The output from this exercise should be as follows:
Setting default log level to "WARN".
To adjust logging level use sc.setLogLevel(newLevel). For SparkR, use setLogLevel(newLevel).
23/09/24 14:21:44 WARN NativeCodeLoader: Unable to load native-hadoop library for your platform... using builtin-java classes where applicable
[Table(name='radioheadData', catalog=None, namespace=[], description=None, tableType='TEMPORARY', isTemporary=True)]
+---+---------------+------------+------+
| id| name|created_date| role|
+---+---------------+------------+------+
| 5|Colin Greenwood| 1969-06-26| bass|
| 4| Philip Selway| 1967-05-23| drums|
| 3| Ed OBrien| |vocals|
| 2|Jonny Greenwood| 1971-10-05|guitar|
| 3| Ed OBrien| 1968-04-15|guitar|
| 1| Thom Yorke| 1968-10-07|vocals|
+---+---------------+------------+------+
Note that we are using dropDuplicates()
in this example, so the process will not blindly append the new data to the old, but will also remove exact duplicate records from the data. To illustrate this point you will notice that there are two duplicate rows in the datasets: one for "Jonny Greenwood" which is a perfect record match and one for "Ed OBrien" which is not a perfect match. The end result is that the exact match is removed fome the final file, but the patial match results in two entries for "Ed OBrien".
Archive
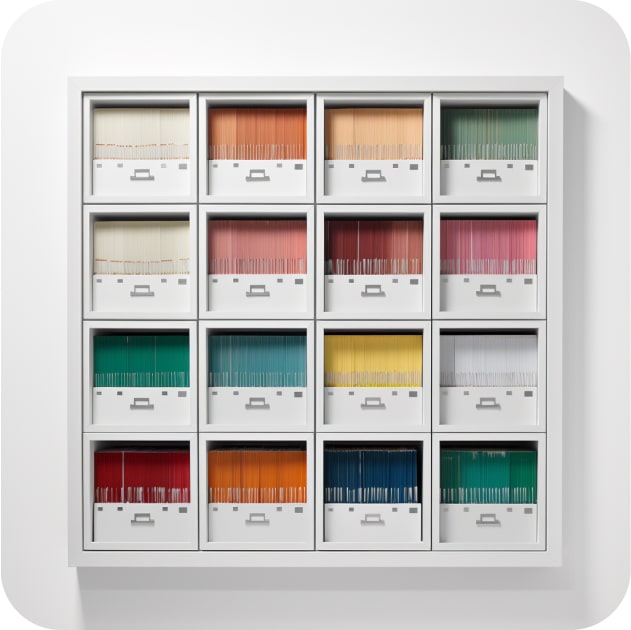
Augment
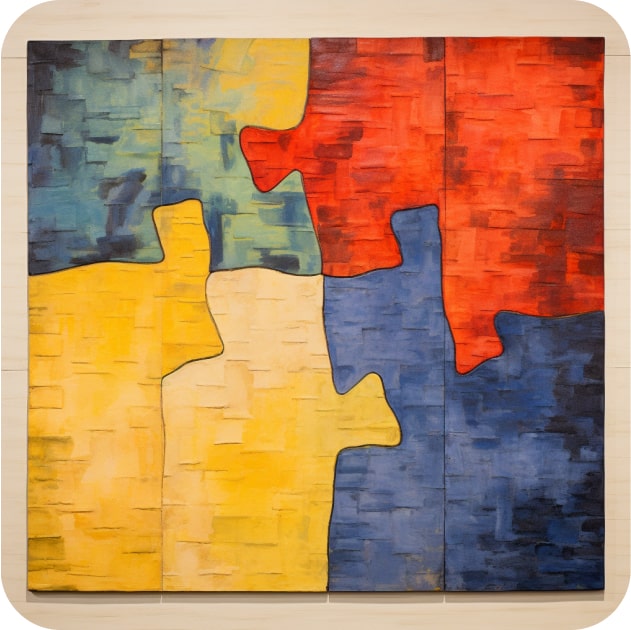
Auto-materialize
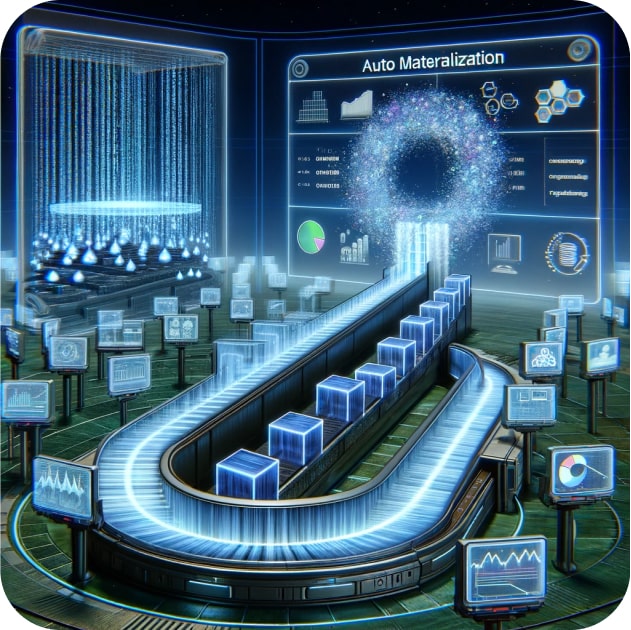
Backup
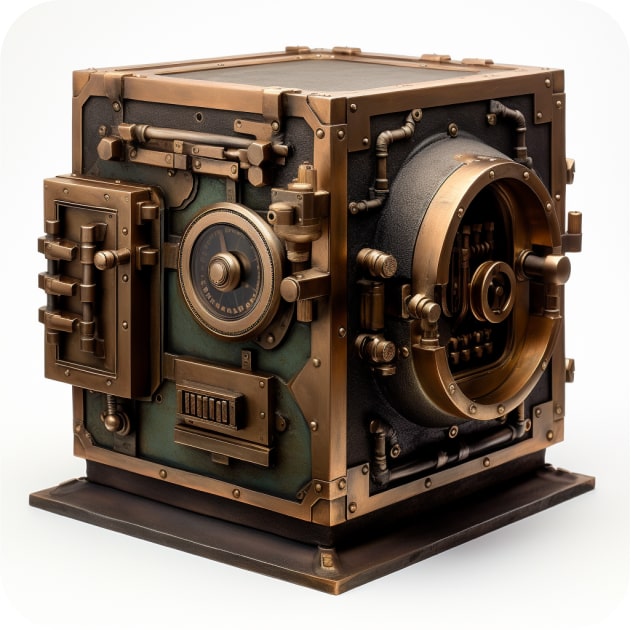
Batch Processing
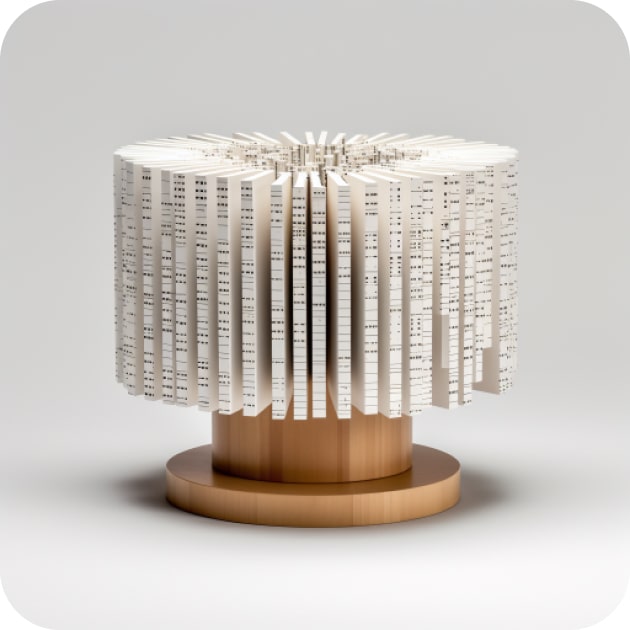
Cache
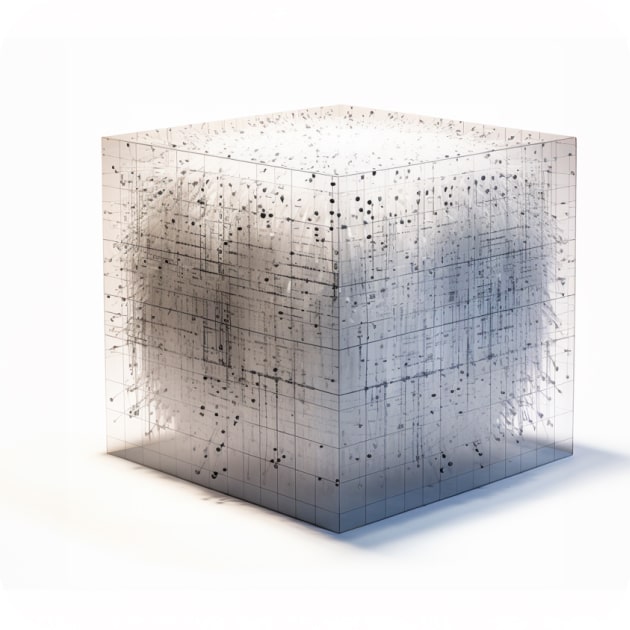
Categorize
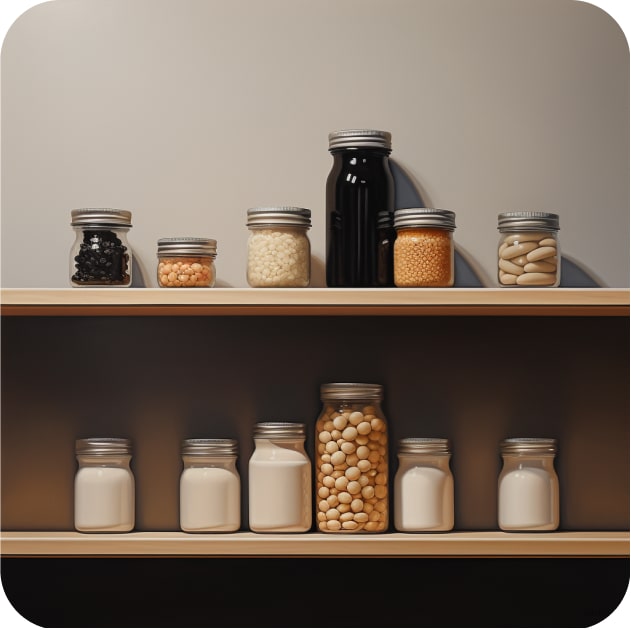
Deduplicate
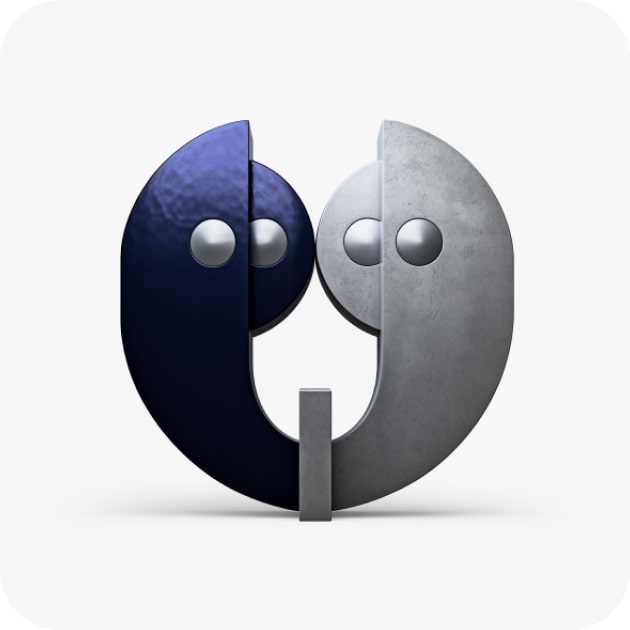
Deserialize
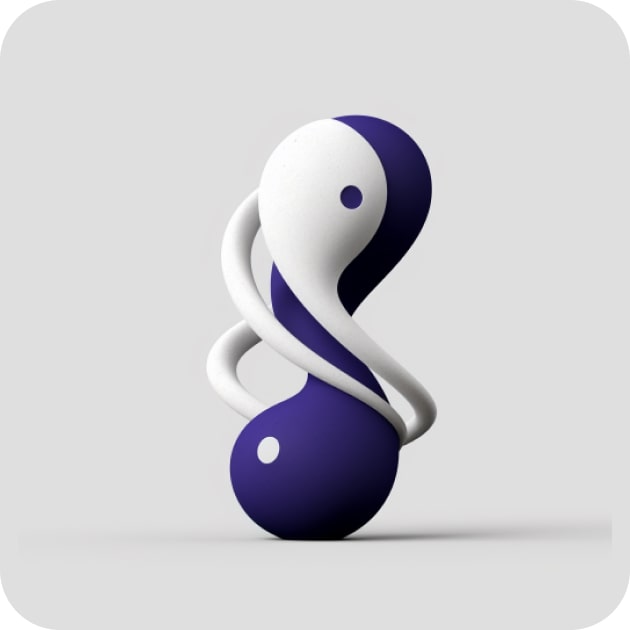
Dimensionality
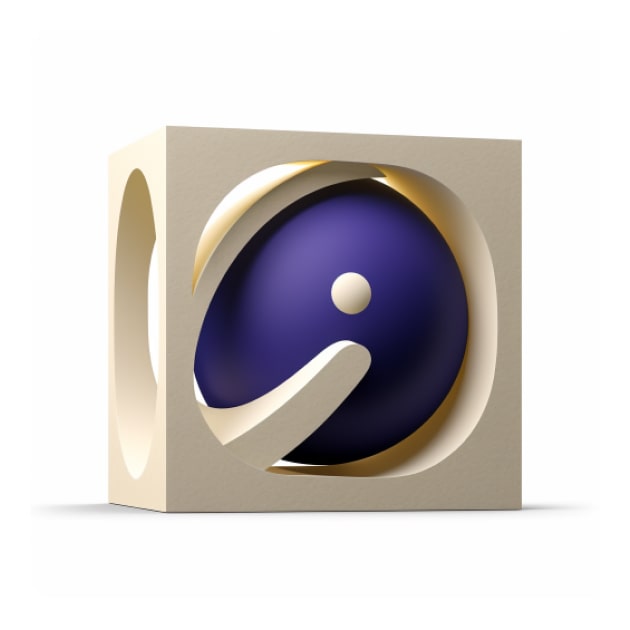
Encapsulate
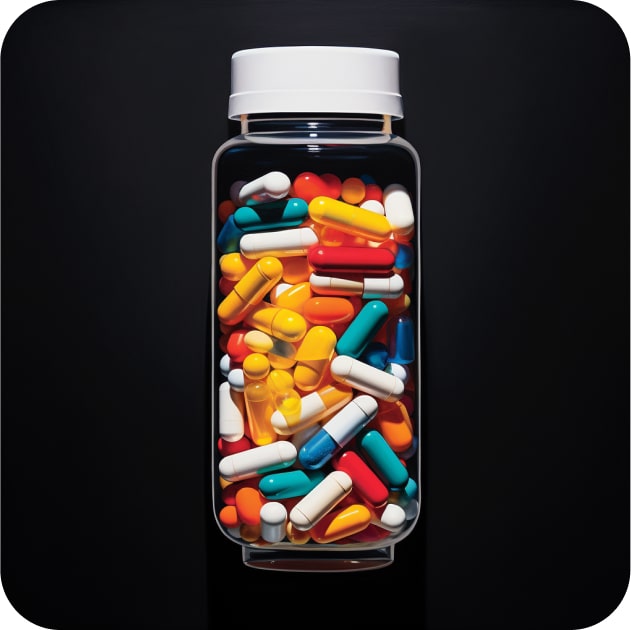
Enrich
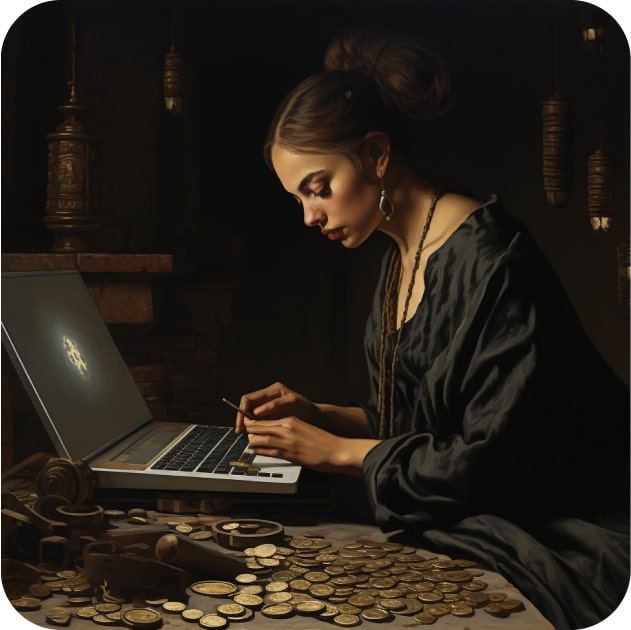
Export
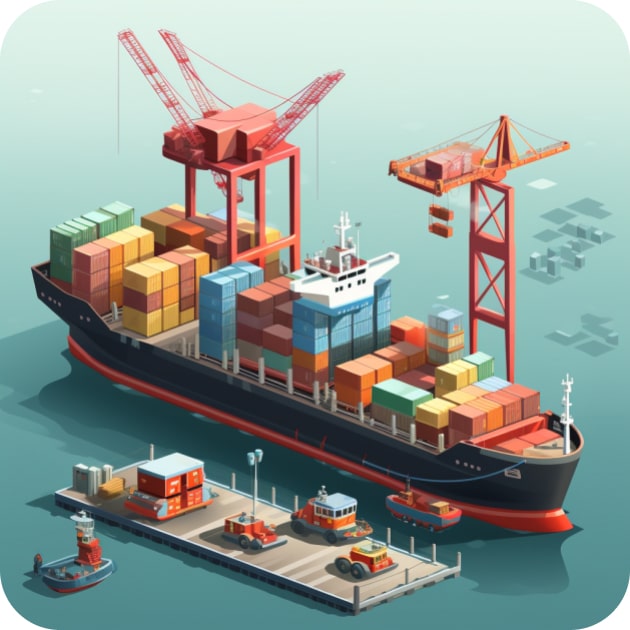
Graph Theory
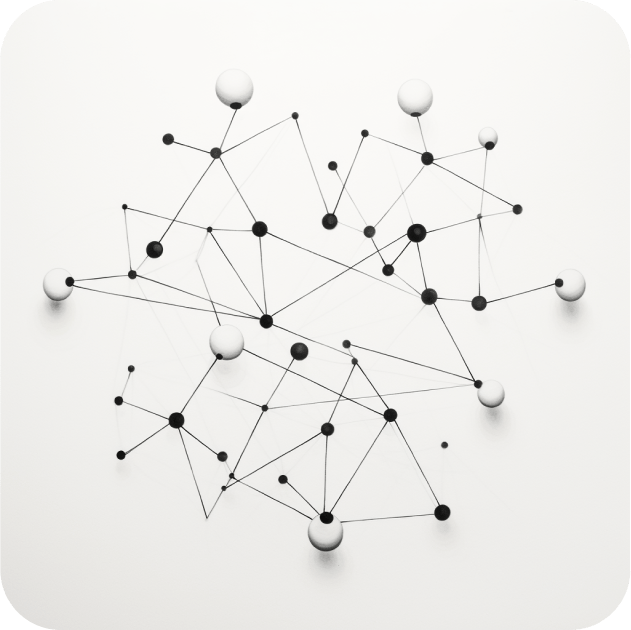
Idempotent
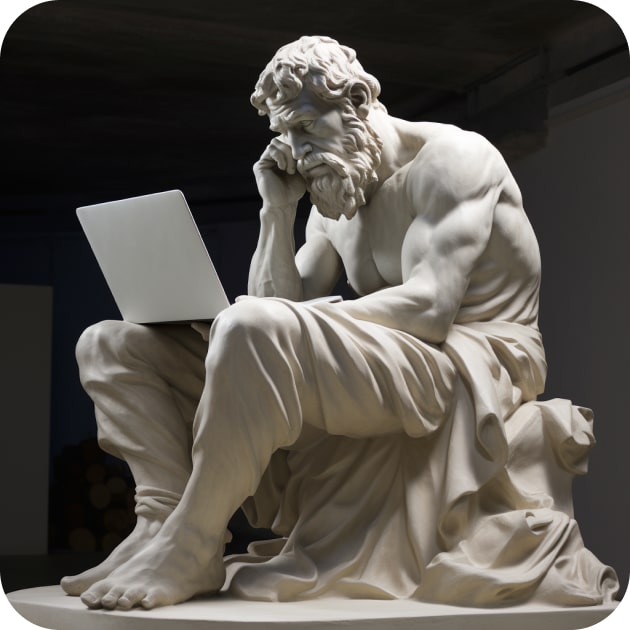
Index
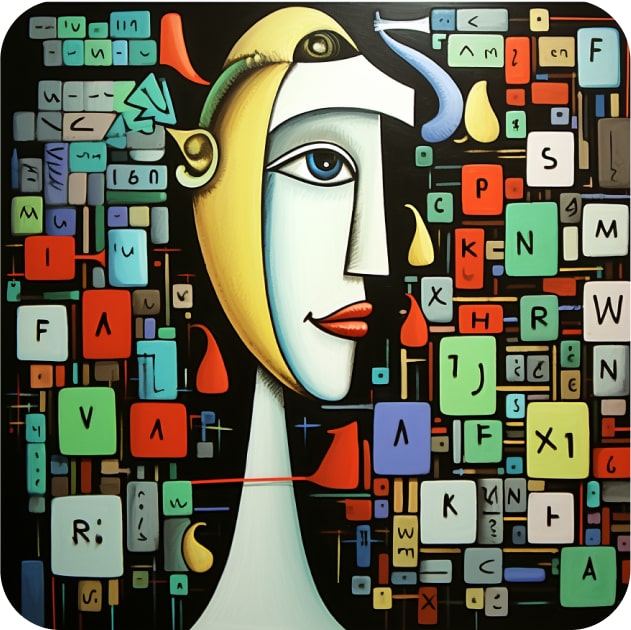
Integrate
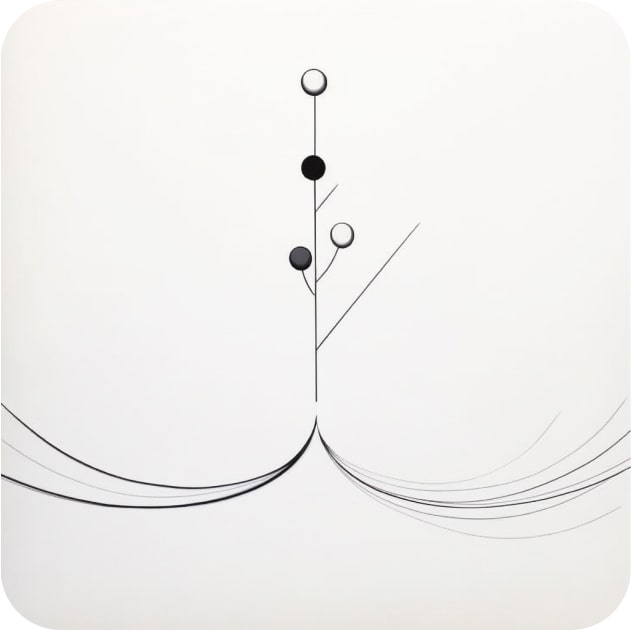
Lineage
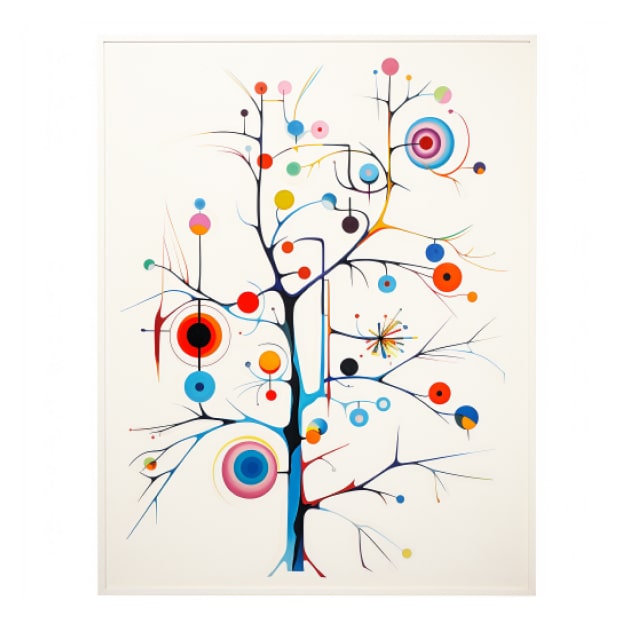
Linearizability
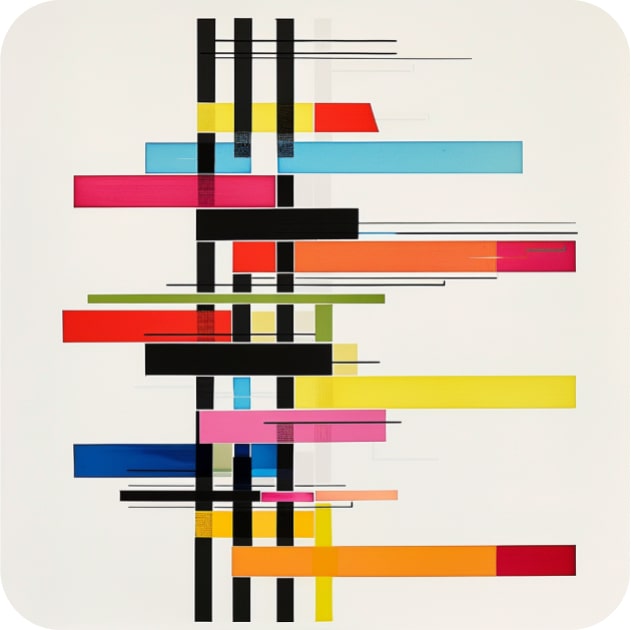
Materialize
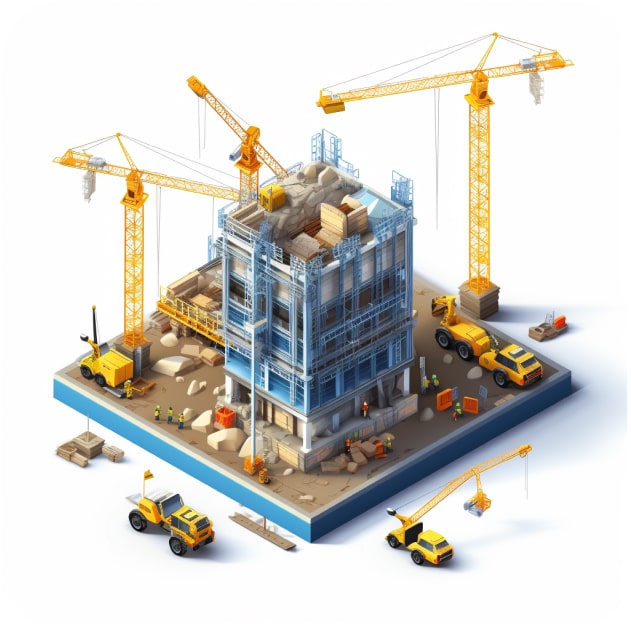
Memoize
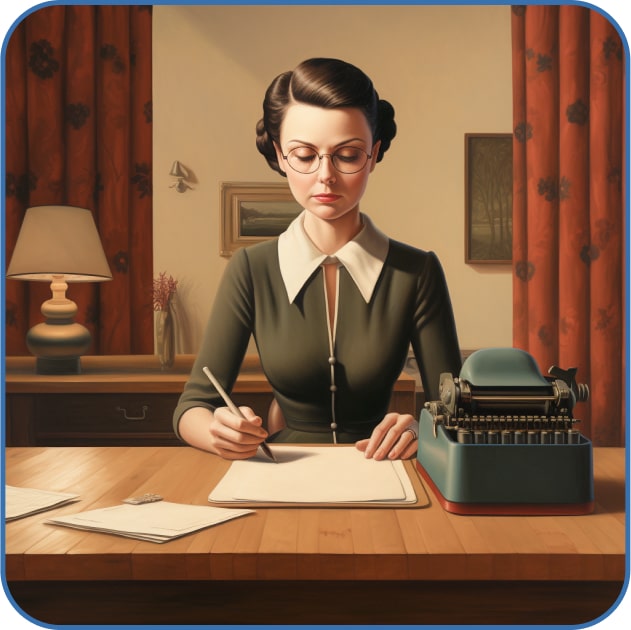
Merge
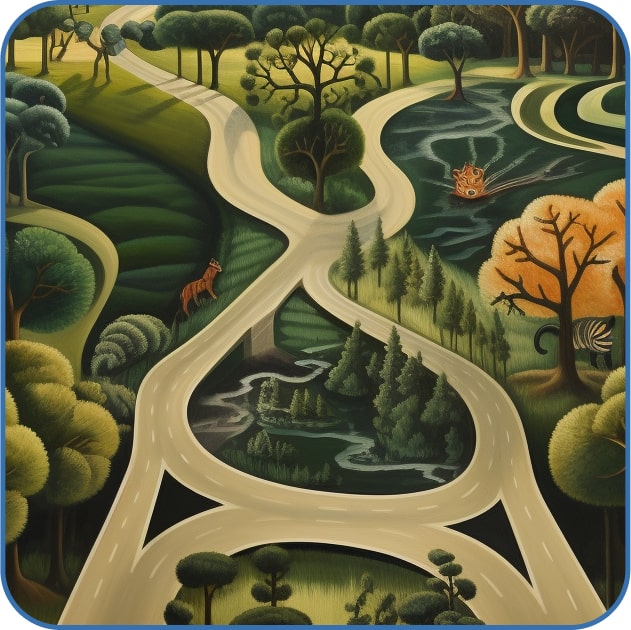
Model
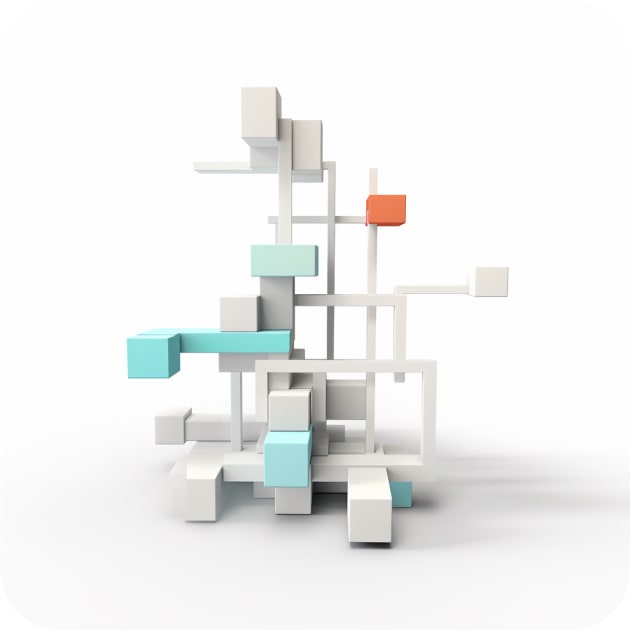
Monitor
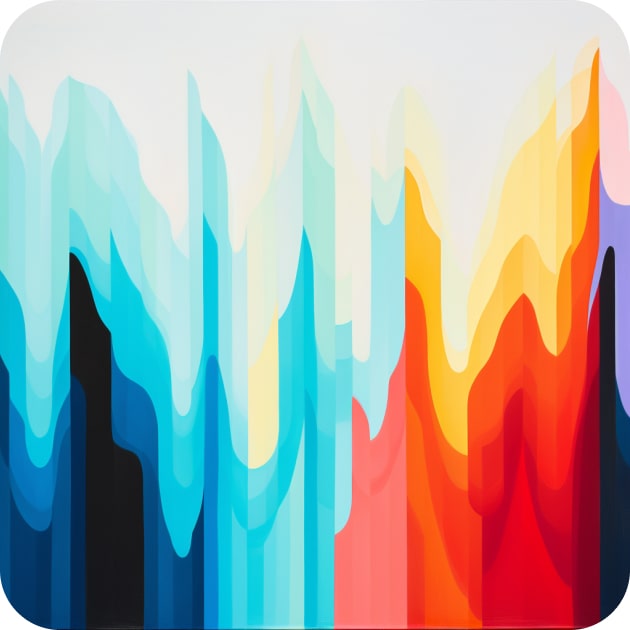
Named Entity Recognition
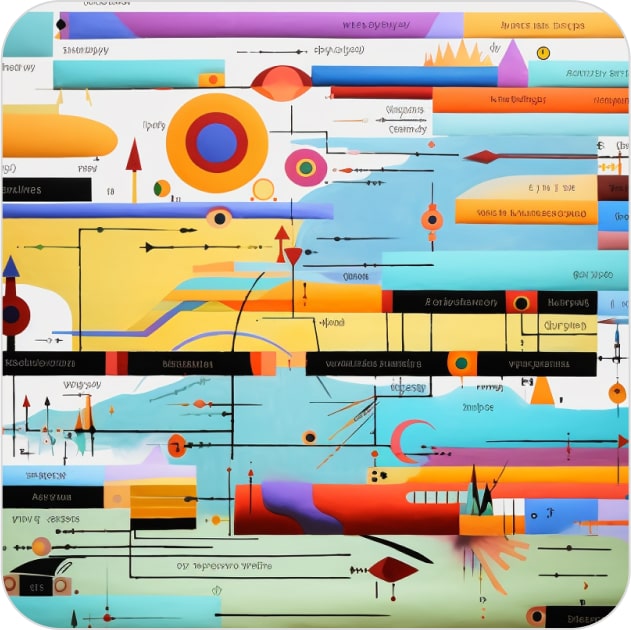
Parse
Partition
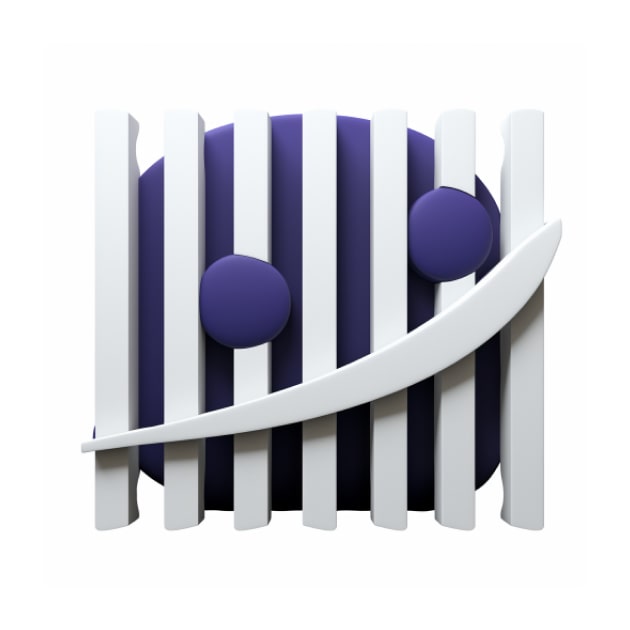
Prep
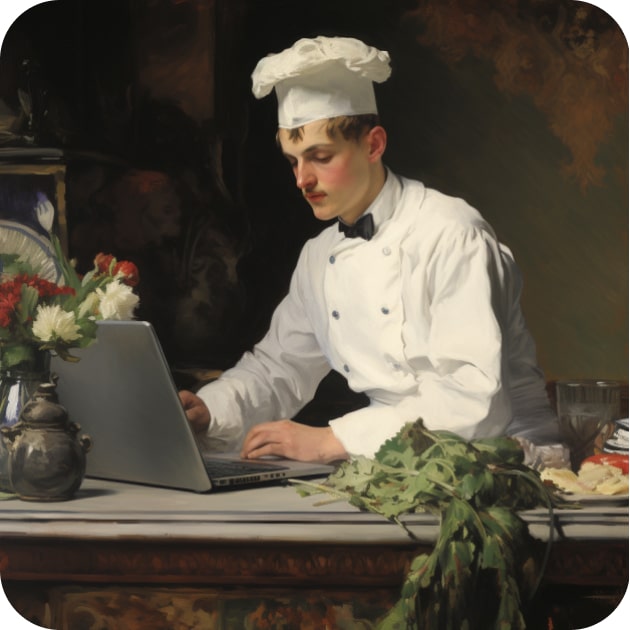
Preprocess
Replicate
Scaling
Schema Inference
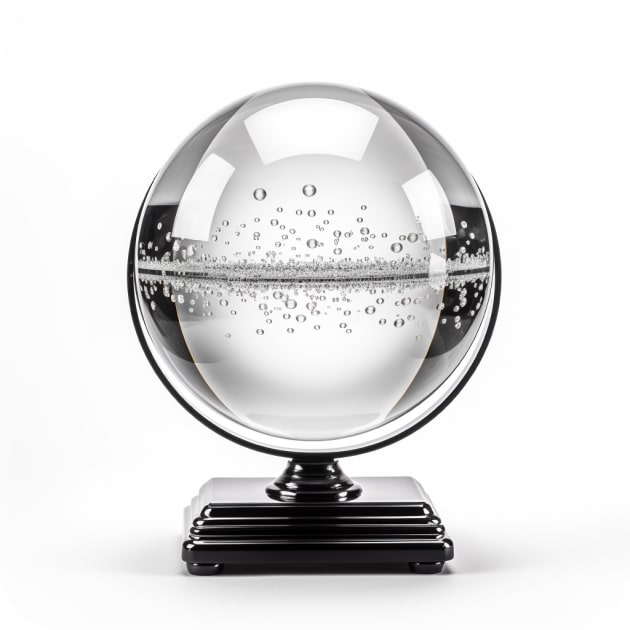
Schema Mapping
Secondary Index
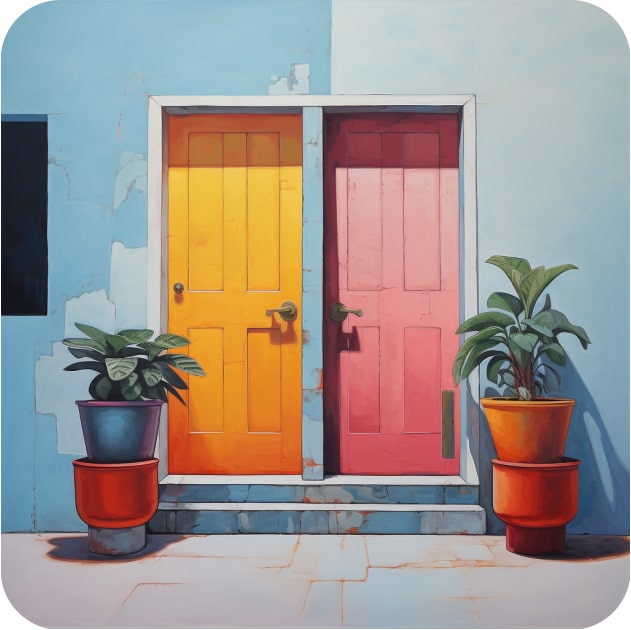
Software-defined Asset
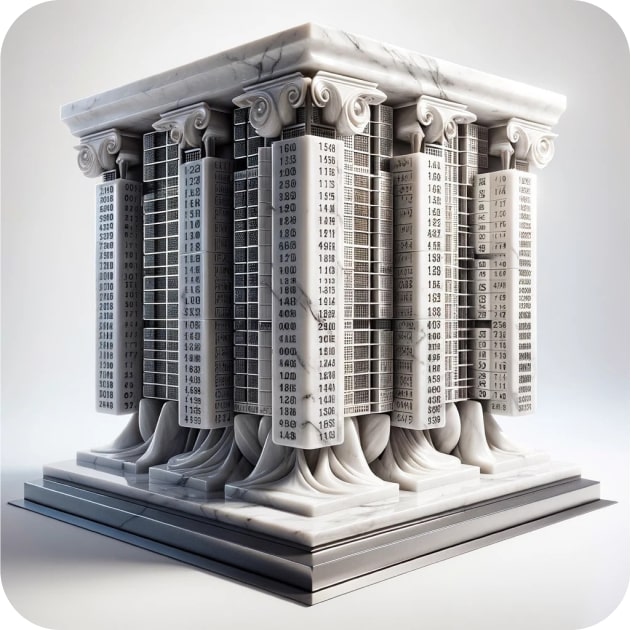
Synchronize
Validate
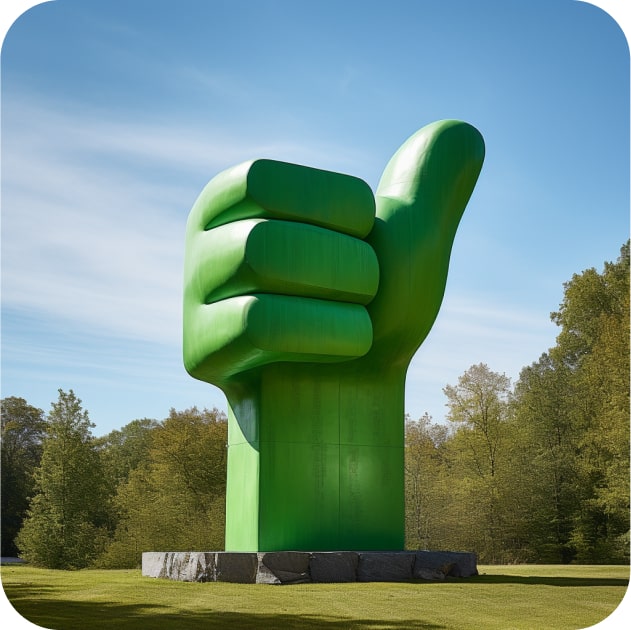
Version
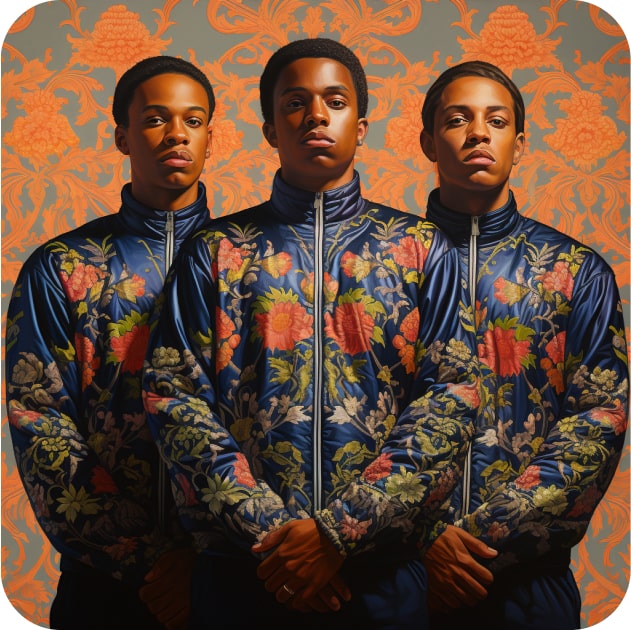