Data merging definition:
Data merging is the process of combining two or more datasets into a single dataset. It is a critical step in modern data pipelines when working with data from multiple sources or with different formats that need to be merged for analysis.
Data merging example using Python:
Please note that you need to have the Pandas library installed in your Python environment to run the following code examples.
In Python, one of the most popular libraries for merging data are Pandas and Polars. The Pandas library provides several functions for merging data, including merge()
, concat()
, and join()
.
The merge() function
The merge()
function allows you to merge two data frames on one or more common columns. For example, let's say we have two data frames:
import pandas as pd
df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'], 'value': [1, 2, 3, 4]})
df2 = pd.DataFrame({'key': ['B', 'D', 'E', 'F'], 'value': [5, 6, 7, 8]})
We can merge these two data frames on the 'key' column like this:
merged_df = pd.merge(df1, df2, on='key', how='inner')
print(merged_df)
For an output of:
key value_x value_y
0 B 2 5
1 D 4 6
The concat() function
The concat()
function allows you to concatenate two or more data frames vertically or horizontally. For example, let's say we have two data frames:
df1 = pd.DataFrame({'col1': ['A', 'B', 'C', 'D'], 'col2': [1, 2, 3, 4]})
df2 = pd.DataFrame({'col1': ['E', 'F', 'G', 'H'], 'col2': [5, 6, 7, 8]})
We can concatenate these two data frames horizontally like this:
concatenated_df = pd.concat([df1, df2], axis=1)
print(concatenated_df)
This will yield an output of:
key value key value
0 A 1 B 5
1 B 2 D 6
2 C 3 E 7
3 D 4 F 8
The join() function
The join()
function is similar to merge()
, but it joins two data frames on their index rather than on a column. For example, let's say we have two data frames:
df1 = pd.DataFrame({'value1': [1, 2, 3, 4]}, index=['A', 'B', 'C', 'D'])
df2 = pd.DataFrame({'value2': [5, 6, 7, 8]}, index=['B', 'D', 'E', 'F'])
We can join these two data frames like this:
joined_df = df1.join(df2, how='inner')
print(joined_df)
In this example, we join df1
and df2
on their index and keep only the rows that are present in both data frames (specified by how='inner'
).
This will yield an output of:
value1 value2
B 2 5
D 4 6
Data merge vs. data join vs. data matching
It's worth disambiguating a few terms here as these refer to different ways of combining datasets:
- Data merging combines datasets by simply appending columns from different datasets
- Data join combines datasets based on a common key or column.
- Data matching combines records or data elements from different datasets based on their similarity or matching criteria.
Data merge vs. data join
As opposed to data merging discussed above, data join is a specific type of data merging that involves combining two or more datasets based on a common column or key. In a data join, the resulting dataset will only include rows where the key matches in both datasets. This means that any rows that do not have a matching key in both datasets will not be included in the final output. Data join is often used in relational databases, where tables can be joined based on a common key.
Data join is more selective in its output than data merging. Data join only includes rows where the key matches in both datasets, while data merging includes all rows from both datasets.
Data merge vs. data match
Data matching involves comparing two or more datasets to identify and extract common records or data elements. The goal of data matching is to find matching records or data elements in different datasets and combine them into a single, unified record or dataset. Data matching can be done using a variety of methods, including fuzzy matching, exact matching, and probabilistic matching.
While data merging is a relatively straightforward process that involves identifying a common variable or column and combining datasets based on that variable, data matching can be a more complex process that involves comparing multiple datasets and identifying common records or data elements based on complex algorithms and rules.
Append
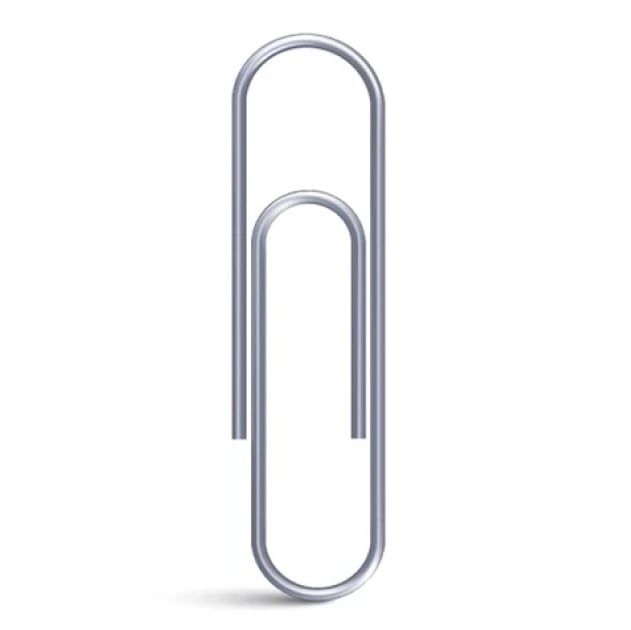
Archive
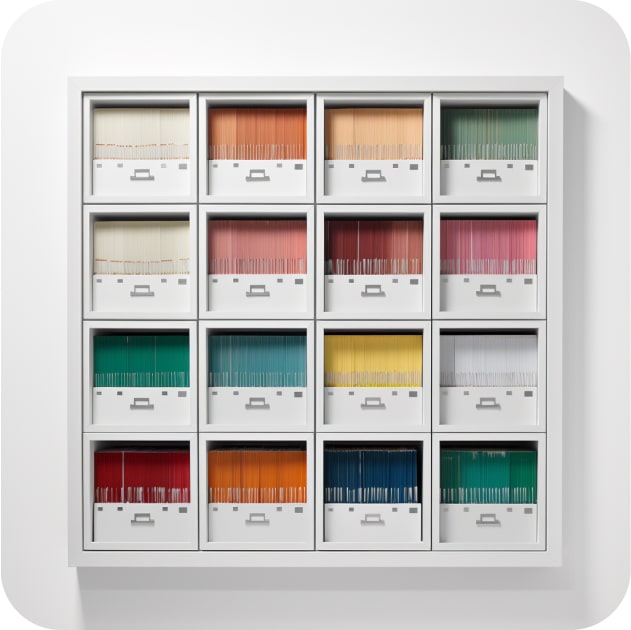
Augment
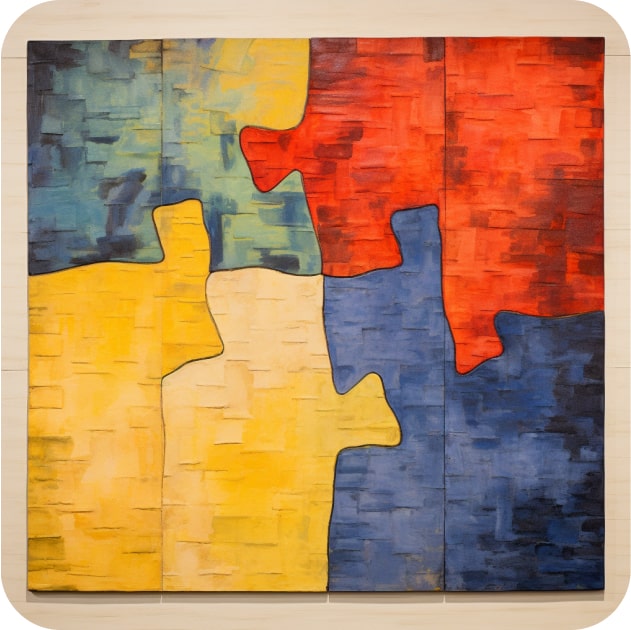
Auto-materialize
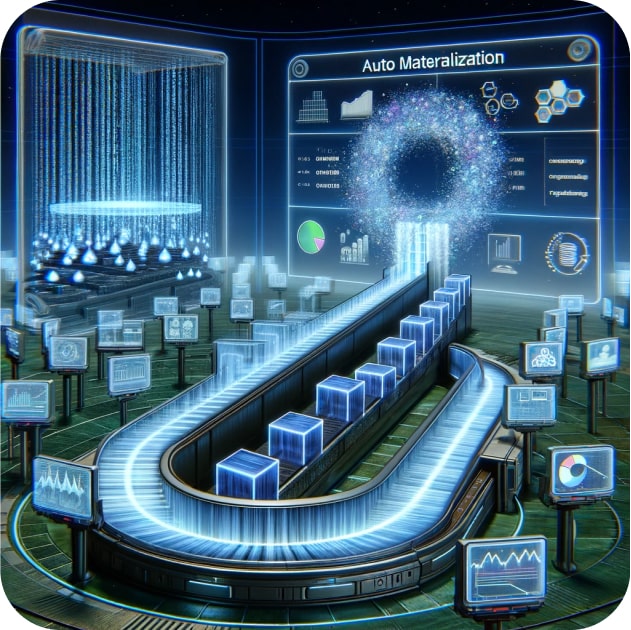
Backup
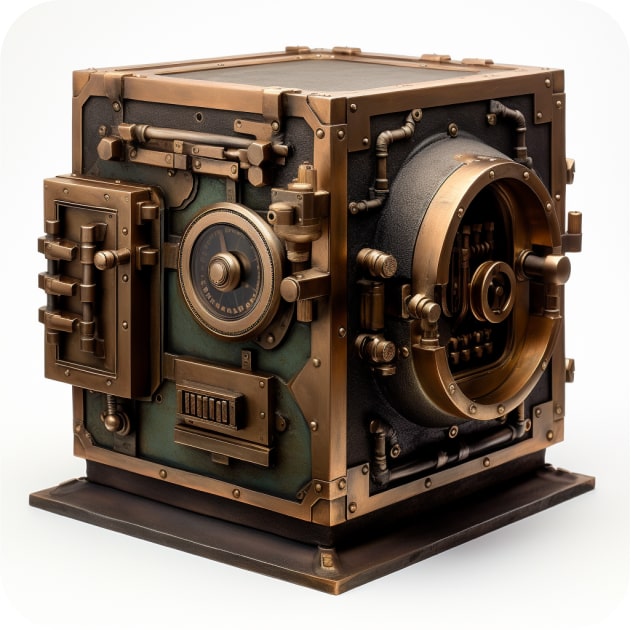
Batch Processing
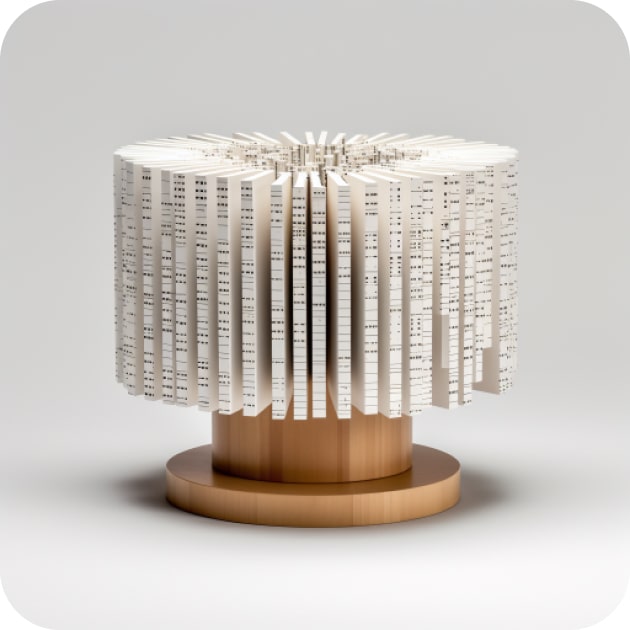
Cache
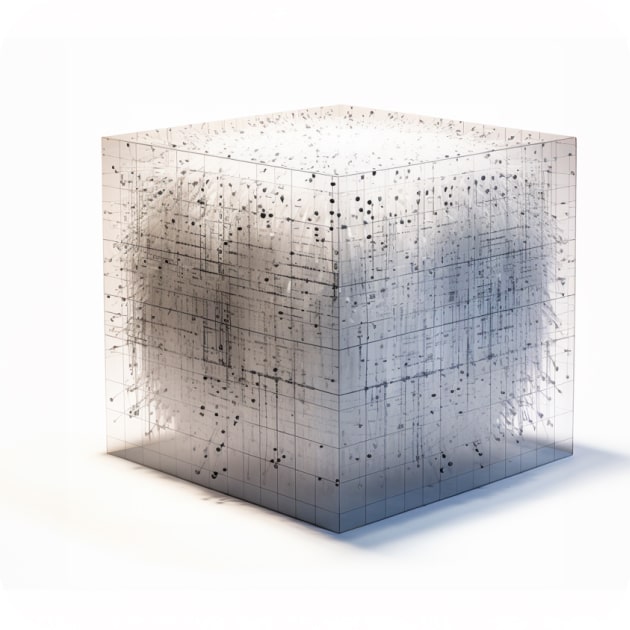
Categorize
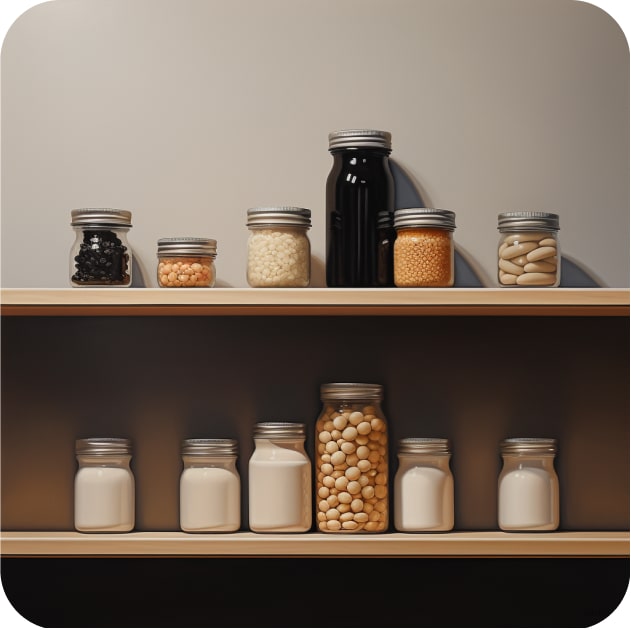
Checkpointing
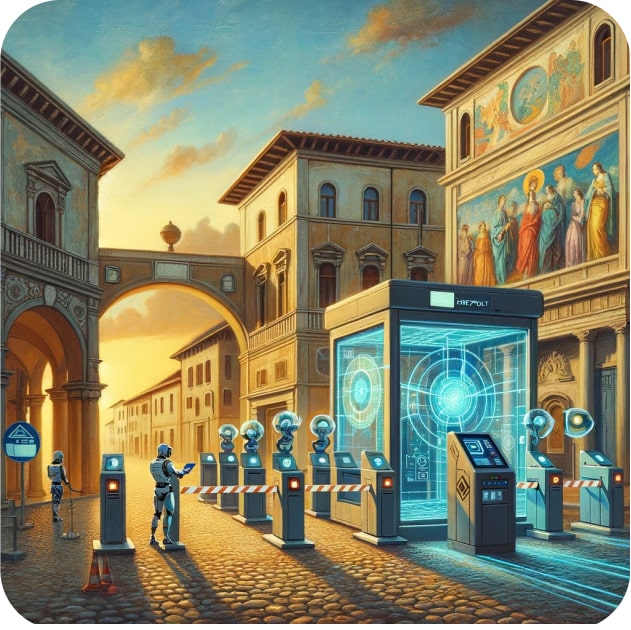
Deduplicate
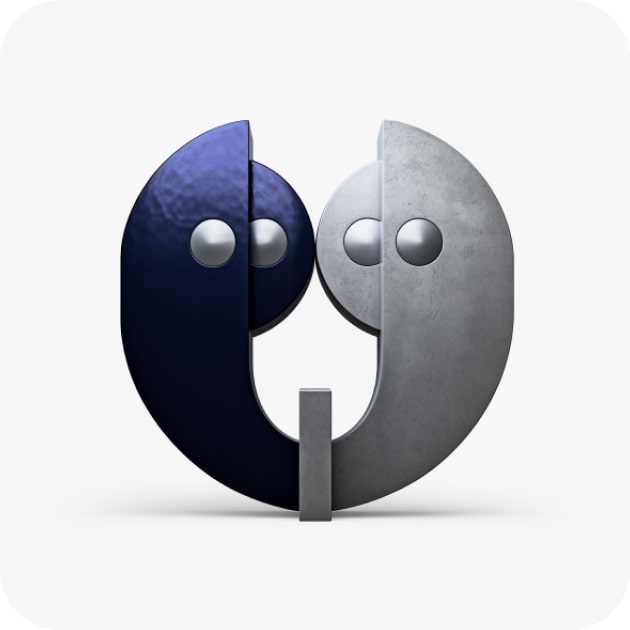
Deserialize
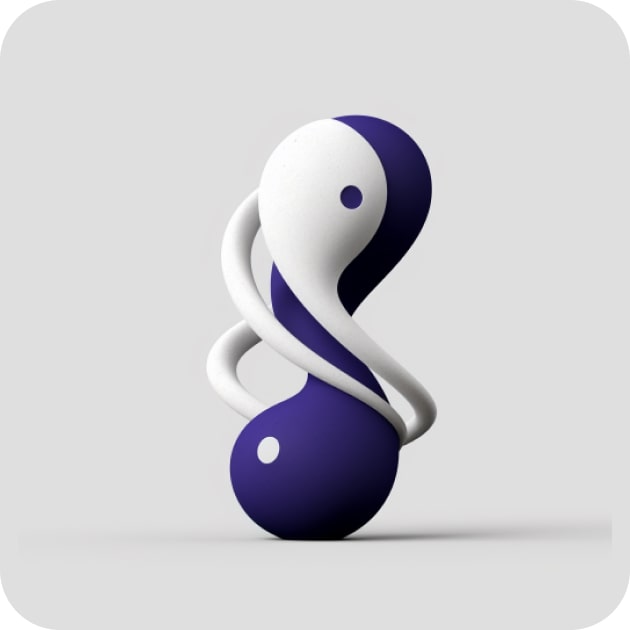
Dimensionality
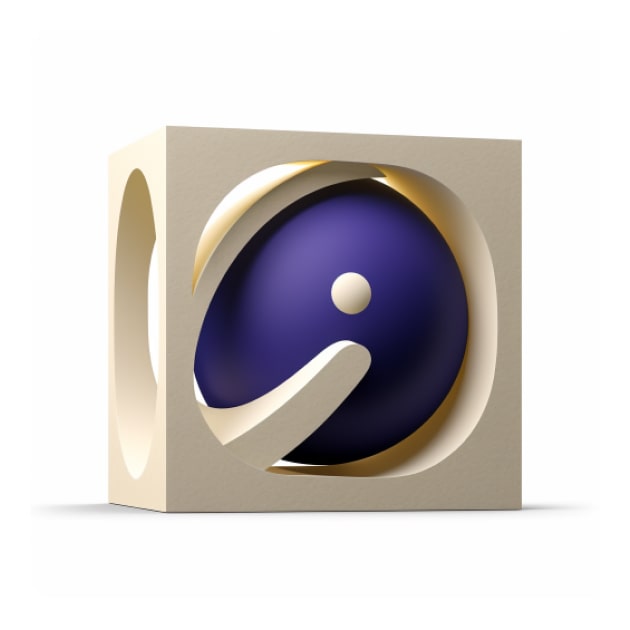
Encapsulate
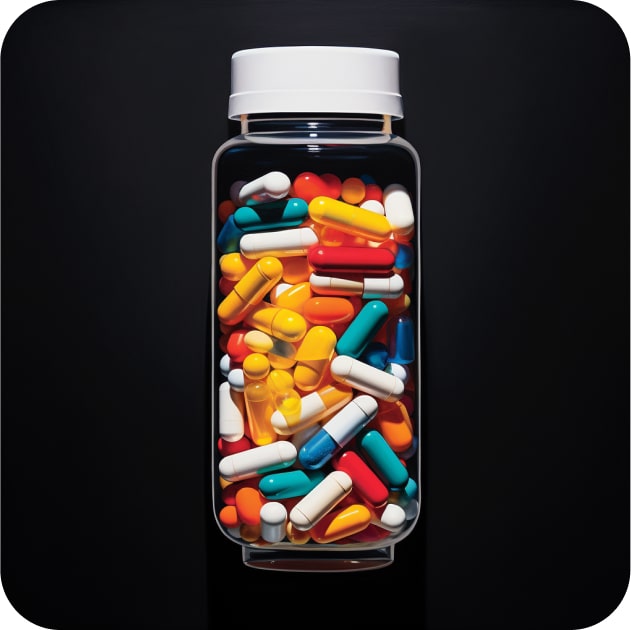
Enrich
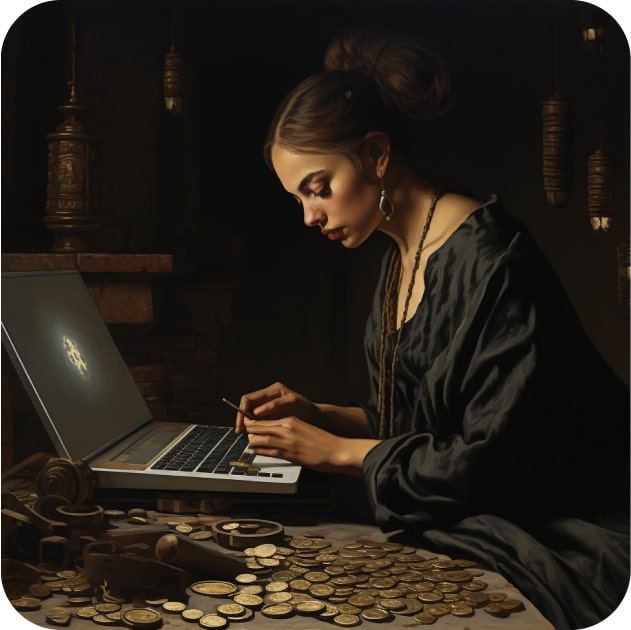
Export
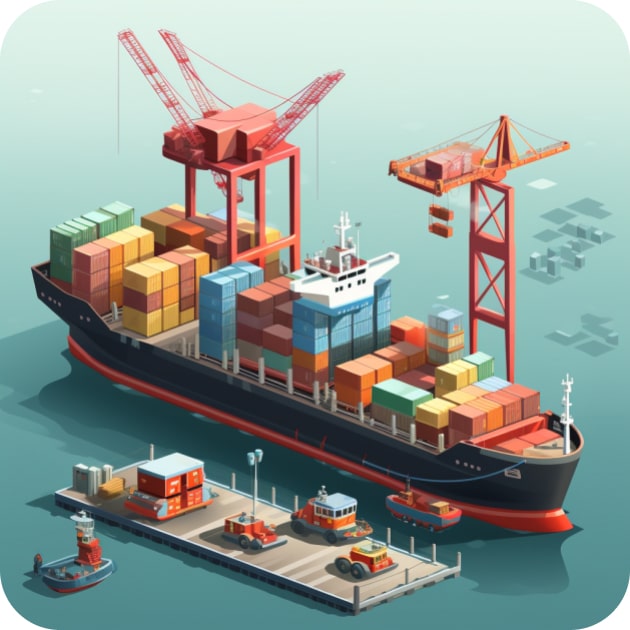
Graph Theory
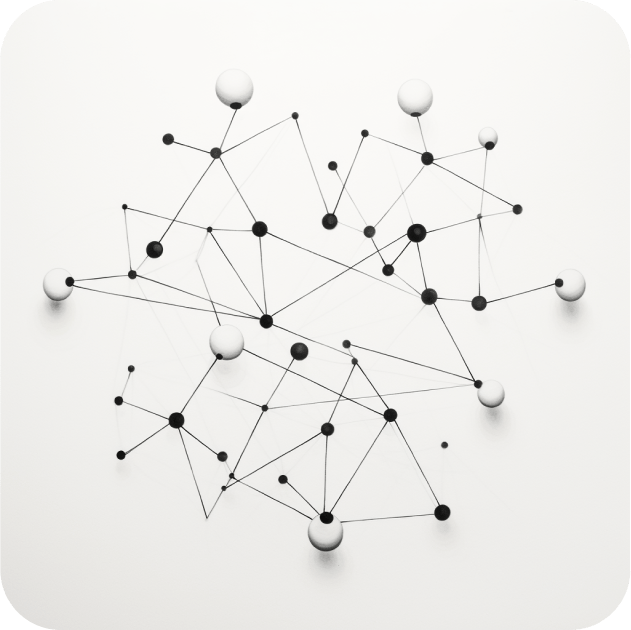
Idempotent
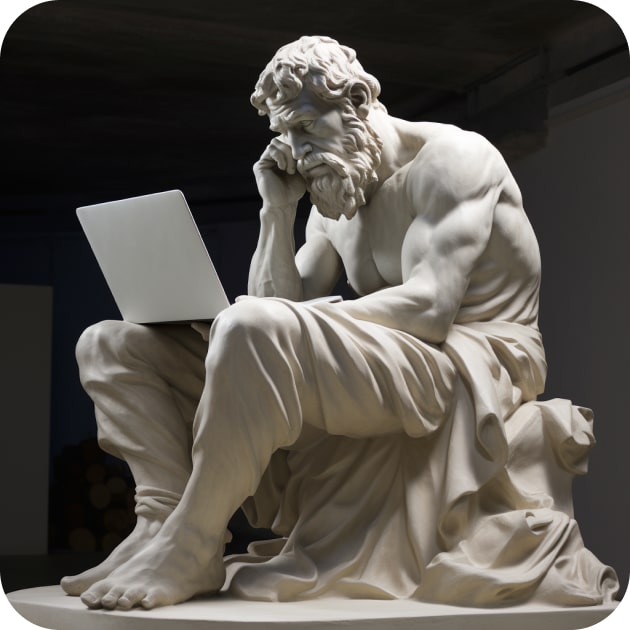
Index
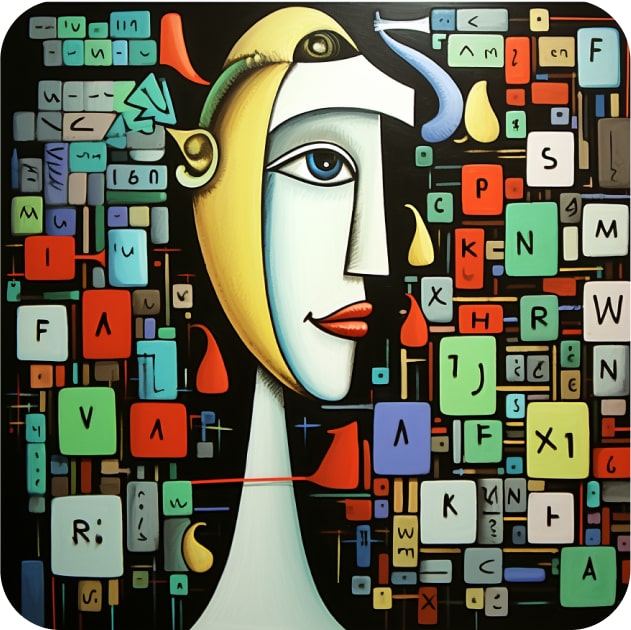
Integrate
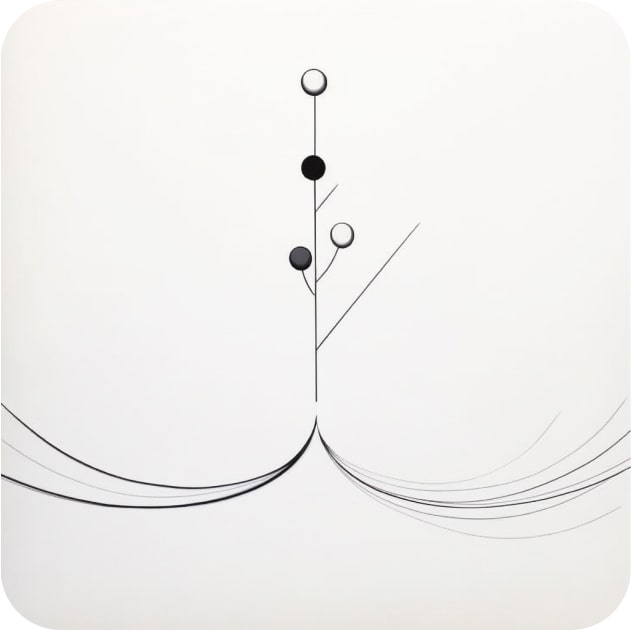
Lineage
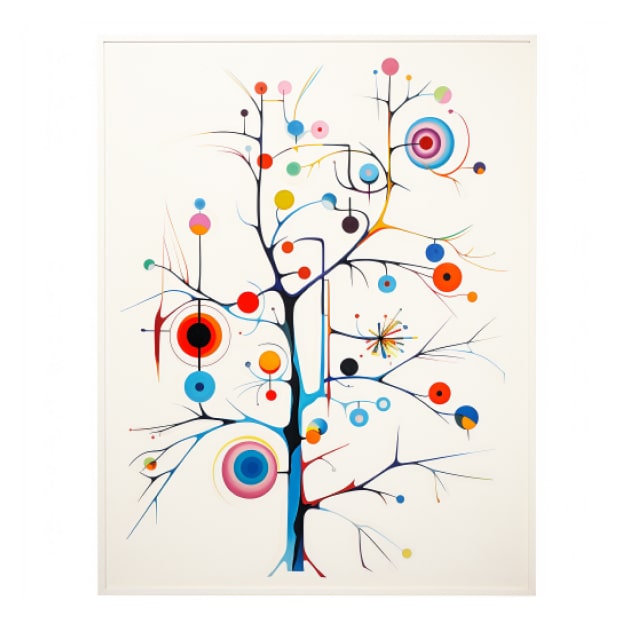
Linearizability
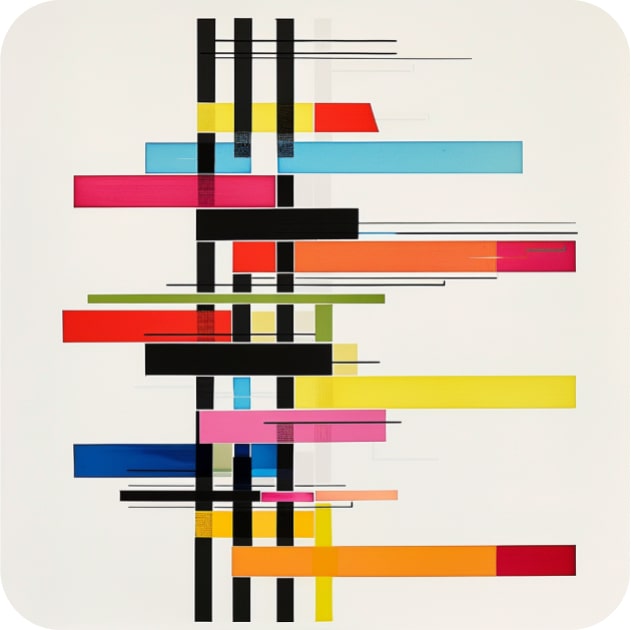
Materialize
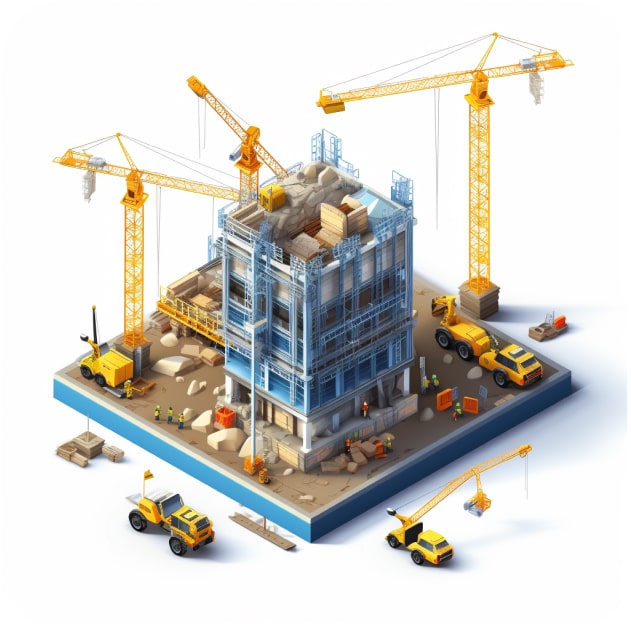
Memoize
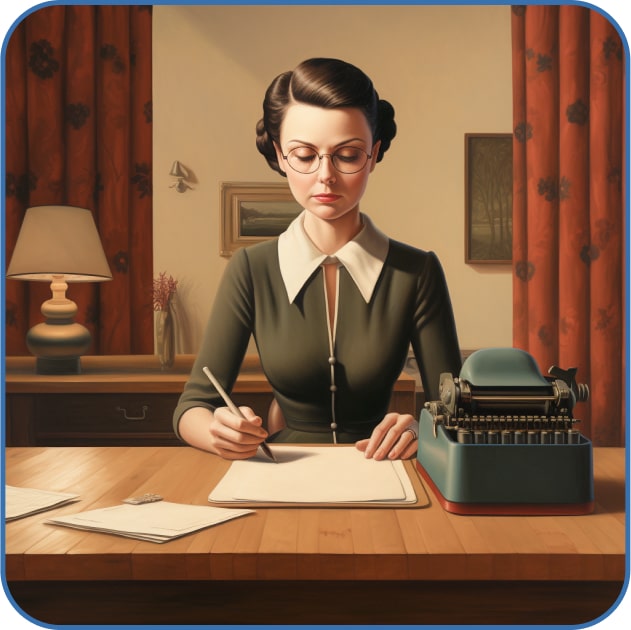
Model
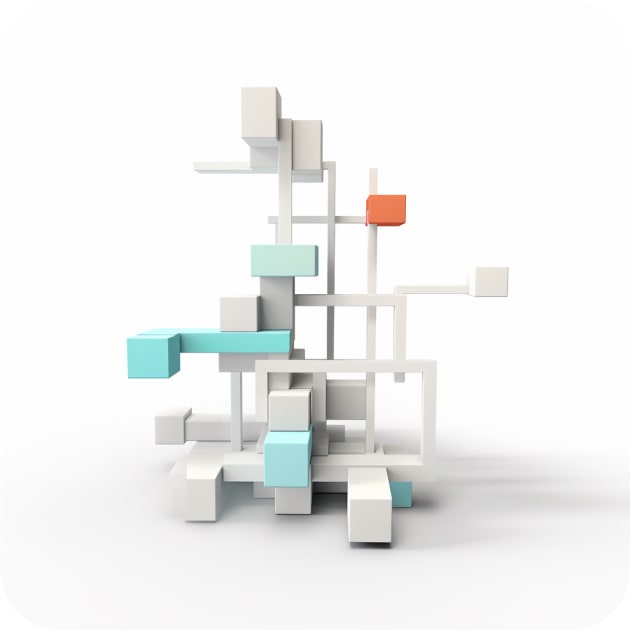
Monitor
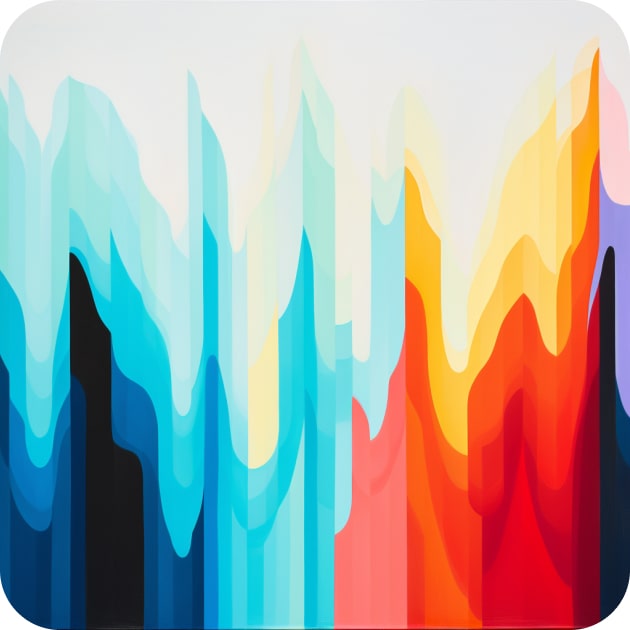
Named Entity Recognition
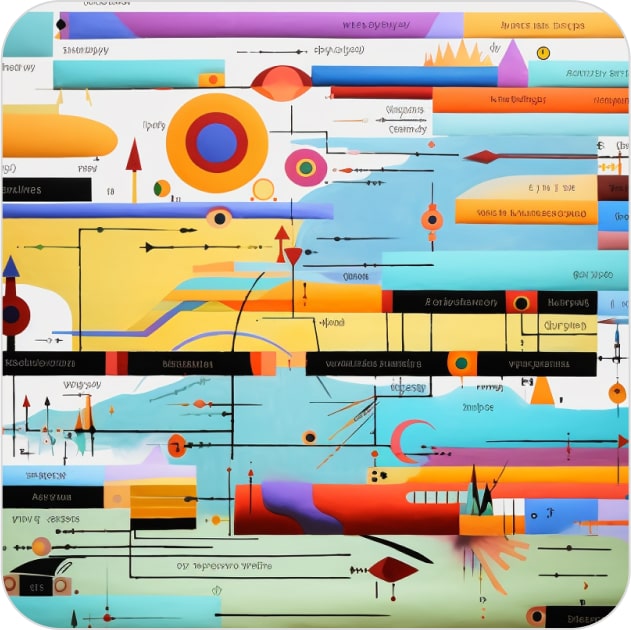
Parse
Partition
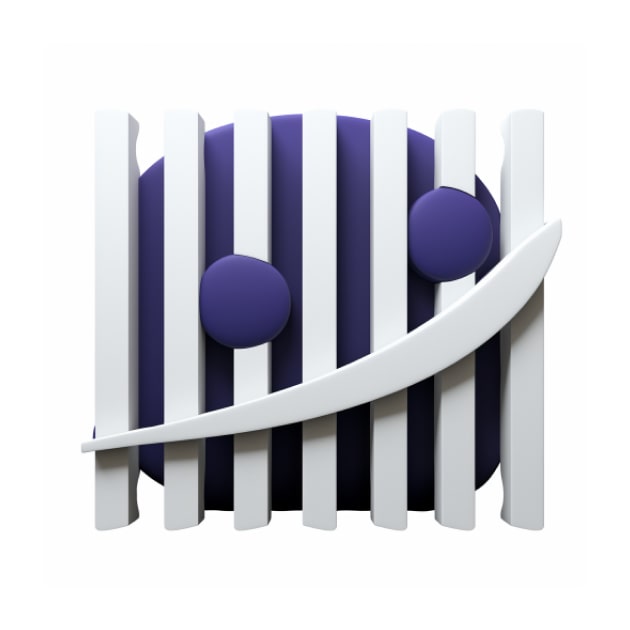
Prep
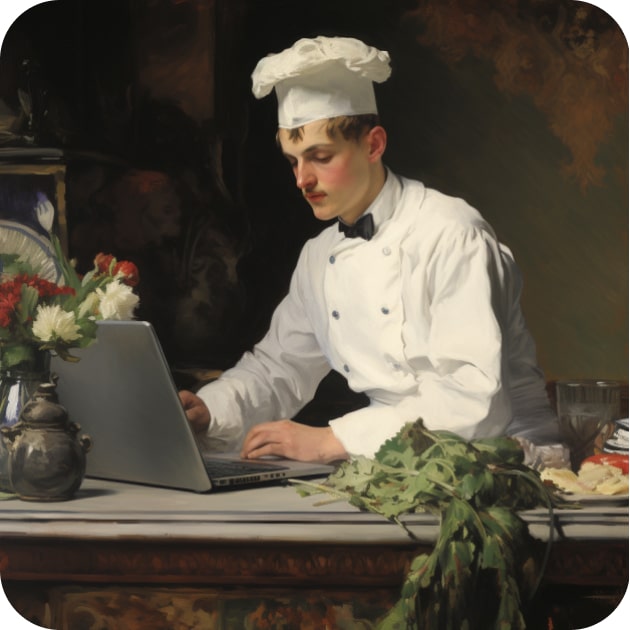
Preprocess
Replicate
Scaling
Schema Inference
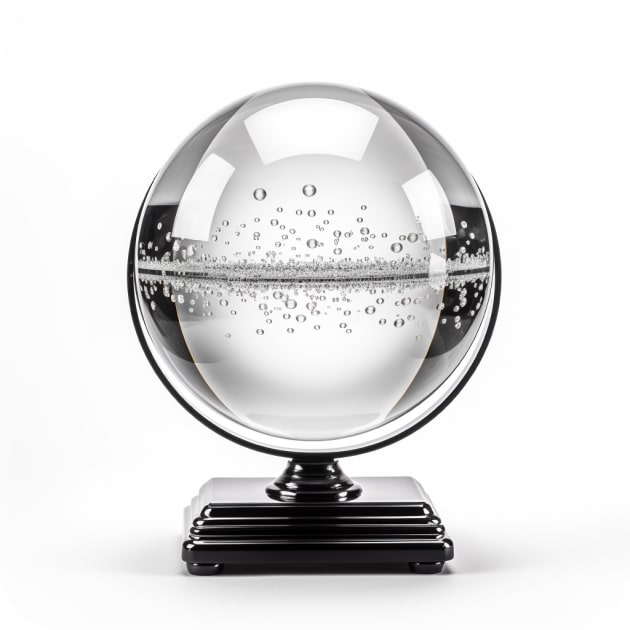
Schema Mapping
Secondary Index
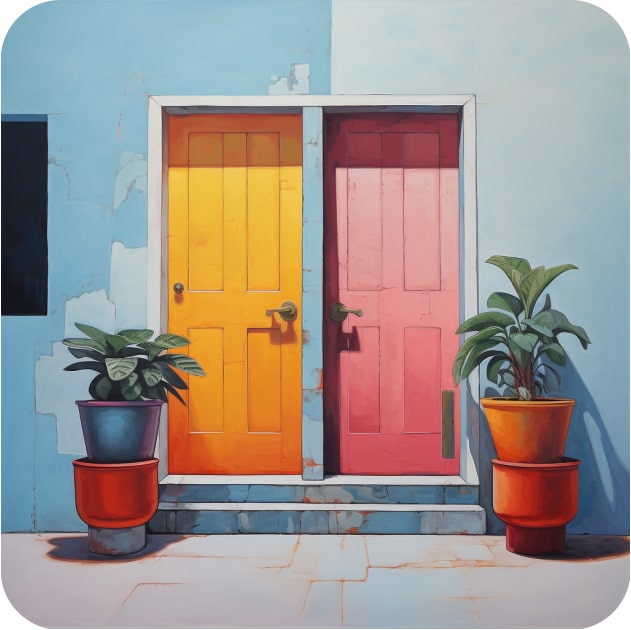
Software-defined Asset
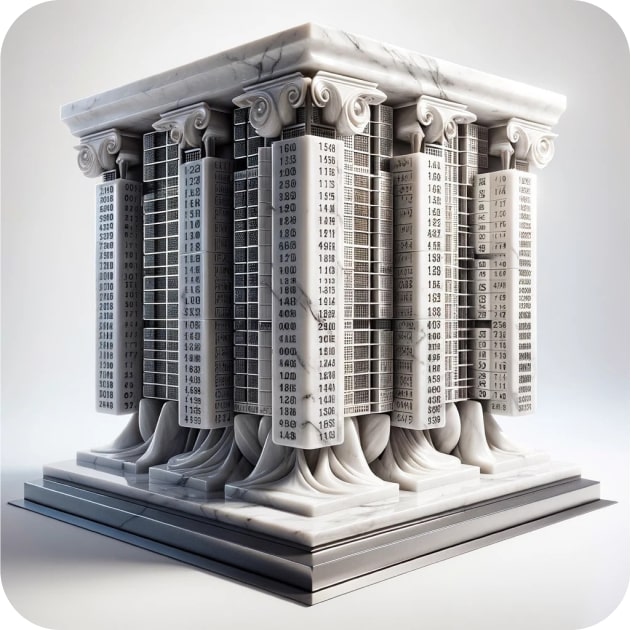
Synchronize
Validate
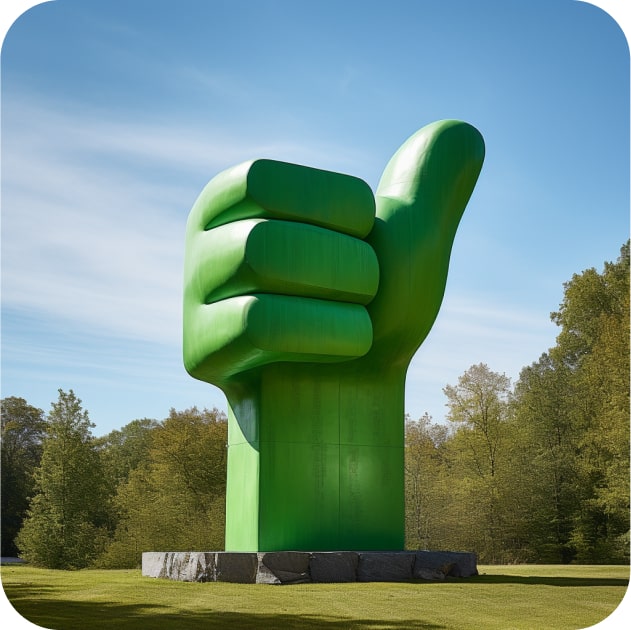
Version
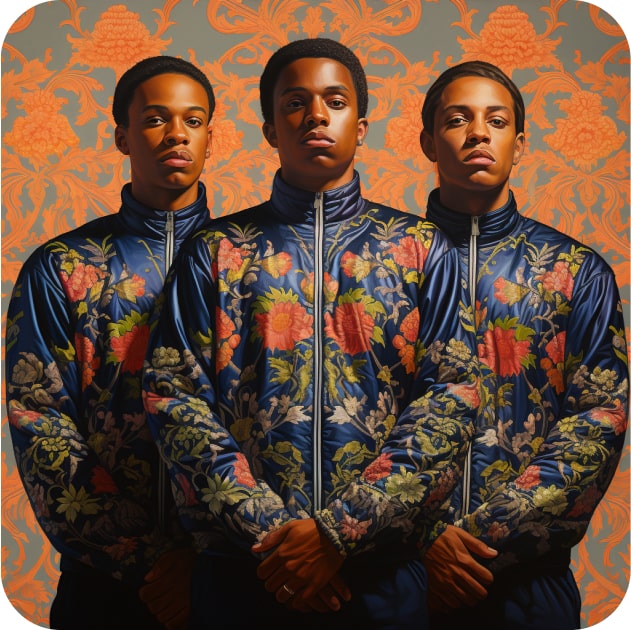