Dagster Data Engineering Glossary:
Data Idempotency
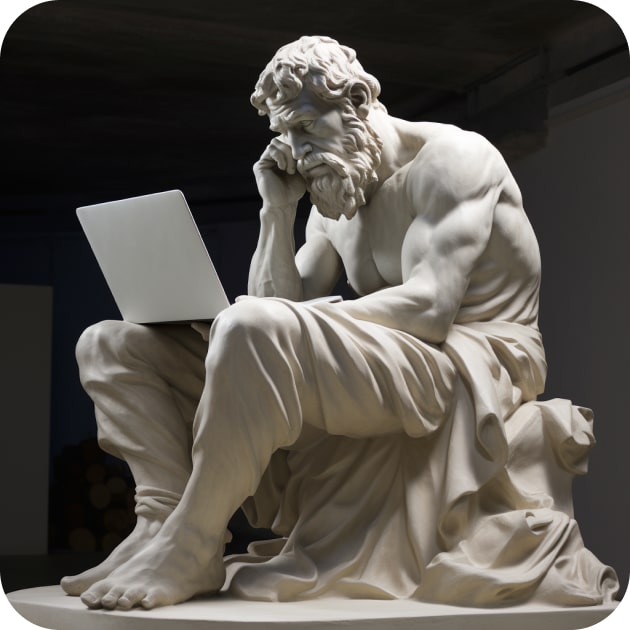
The concept of idempotency is fundamental in computer science and is especially crucial in data engineering due to the need for reliable and repeatable data operations.
Definition of idempotent:
An operation is said to be idempotent if performing it multiple times produces the same result as performing it once.
Importance in Data Engineering:
Data Recovery and Redundancy: In the world of big data processing, there can be failures - a node in a cluster might crash, or a network hiccup might disrupt data transmission. In such scenarios, if an operation is re-executed (due to retry logic or some failure recovery mechanism), idempotency ensures that the data remains consistent and is not duplicated or corrupted.
Atomicity and Consistency: Idempotent operations help maintain atomicity and consistency in data operations. When you're updating a dataset, you want to be sure that either all the changes are made or none are. Idempotent operations can be re-run safely without unintended side effects.
Batch Processing: In batch data processing, if a batch job fails, being able to rerun that job knowing that the operations are idempotent is crucial. It ensures that data is not double counted or wrongly aggregated.
APIs and Data Integration: In data integration tasks, where data might be ingested from APIs, ensuring idempotency means that if you re-fetch the data (maybe due to a failure or a scheduled update), you won't end up with duplicate or inconsistent records.
Examples in Data Engineering:
Appending to a Log: Consider a distributed log like Apache Kafka. If a producer sends a message to a topic and doesn't receive an acknowledgment due to a transient network failure, it might retry sending that message. The system should be designed such that this retry does not result in duplicate records in the log.
Database Writes: When writing data to a database, especially in distributed systems, ensuring that a write operation can be repeated without side effects can be vital. For instance, using unique constraints or upsert operations where an insert becomes an update if a record with a given key already exists.
Data Transformation: When applying transformations in systems like Apache Spark, the operations should be such that re-running a failed transformation job on the same data results in the same output.
The opposite of idempotency:
The opposite of "idempotent" is "non-idempotent." In computing and data operations, if an action or operation is idempotent, then applying it multiple times yields the same result as applying it once. Conversely, if an action is non-idempotent, applying it multiple times may yield different results than applying it once.
In the context of HTTP methods, for instance:
GET
is generally considered idempotent. Retrieving a resource multiple times should provide the same result every time, assuming the resource hasn't changed.POST
is typically considered non-idempotent. Making aPOST
request multiple times (e.g., to create a new entry in a database) may lead to multiple new entries.
To be clear, non-idempotent doesn't mean that the result will always differ upon repeated applications, but rather that it can differ, depending on the operation and circumstances.
Implementation:
There are multiple ways to achieve idempotency:
Use of Unique Identifiers: This often involves using some unique identifier for each operation or record. If an operation with a particular ID has been executed, subsequent operations with the same ID will not be re-executed.
State Tracking: By keeping track of the state of each operation, the system can determine if it needs to execute a particular operation or if it has already been executed.
De-duplication: In post-processing steps, systems can use de-duplication logic to remove any duplicates that might have arisen due to non-idempotent operations.
In summary, idempotency is a crucial concept in data engineering that ensures consistency and reliability in data processing systems. Achieving idempotency often requires careful design and considerations in the systems' architecture and operations.
Example of idempotency in Python
Let's use the example of adding a user to a system. If we attempt to add the same user multiple times, an idempotent operation would ensure that after any number of attempts, the user is only added once. This often represents itself in real-world applications such as database upserts, where a record is updated if it exists and inserted if it does not.
Here's a simple example in Python:
class UserManager:
def __init__(self):
# Let's use a dictionary to store users by their username
self.users = {}
def add_user(self, username, details):
"""Idempotent method to add a user."""
if username not in self.users:
self.users[username] = details
return f"User {username} added."
else:
return f"User {username} already exists. No action taken."
# Testing the idempotent method:
manager = UserManager()
print(manager.add_user("Alice", {"age": 30, "email": "alice@example.com"})) # User Alice added.
print(manager.add_user("Alice", {"age": 30, "email": "alice@example.com"})) # User Alice already exists. No action taken.
In this code:
- When you first try to add "Alice", she's added to the system.
- When you try to add "Alice" again, the system recognizes that she's already in there, and it takes no action, illustrating the idempotency concept. Even after multiple attempts, Alice still exists as a single user in the system.
This simple example will output:
User Alice added.
User Alice already exists. No action taken.
Append
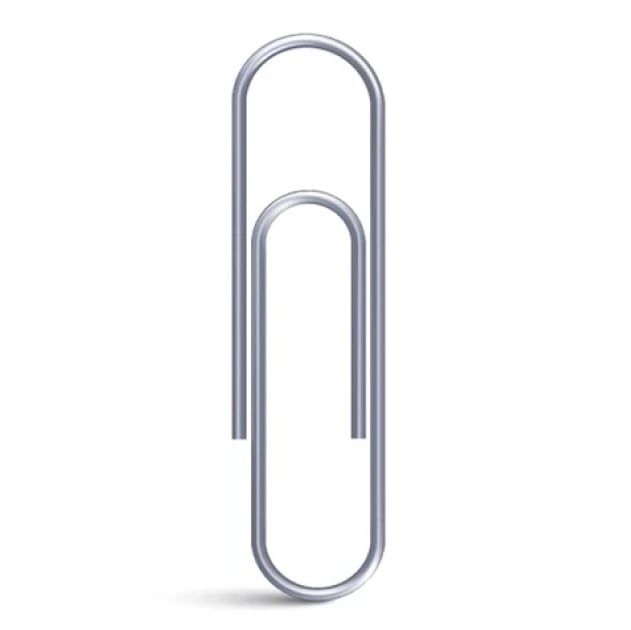
Archive
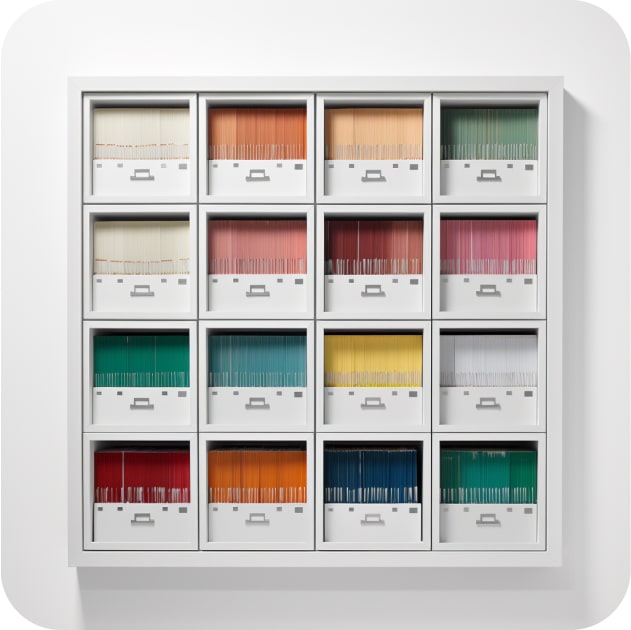
Augment
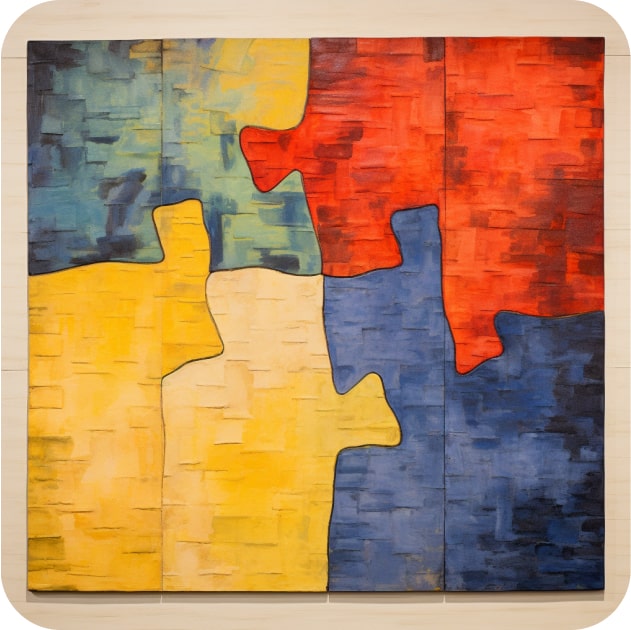
Auto-materialize
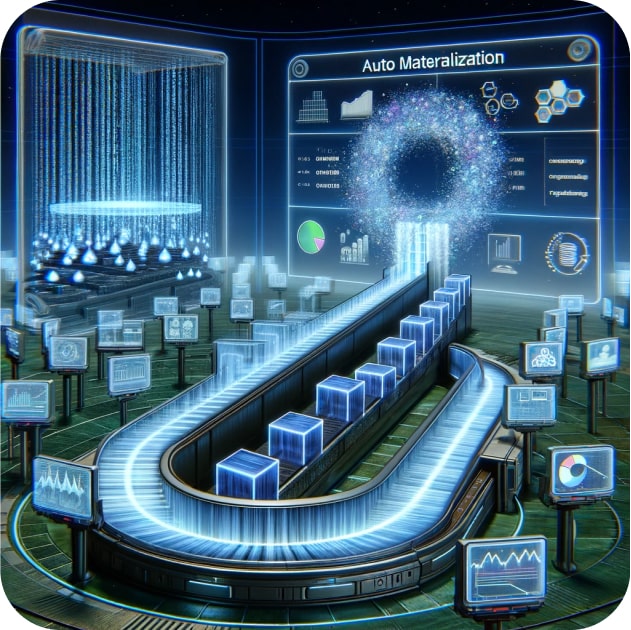
Backup
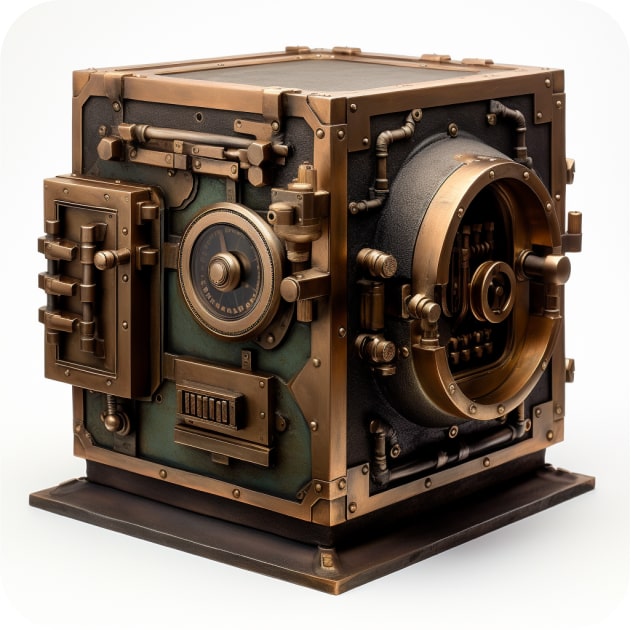
Batch Processing
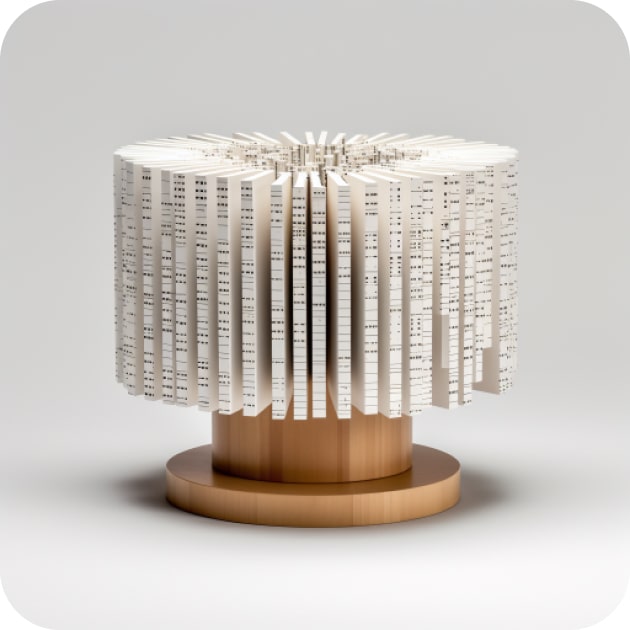
Cache
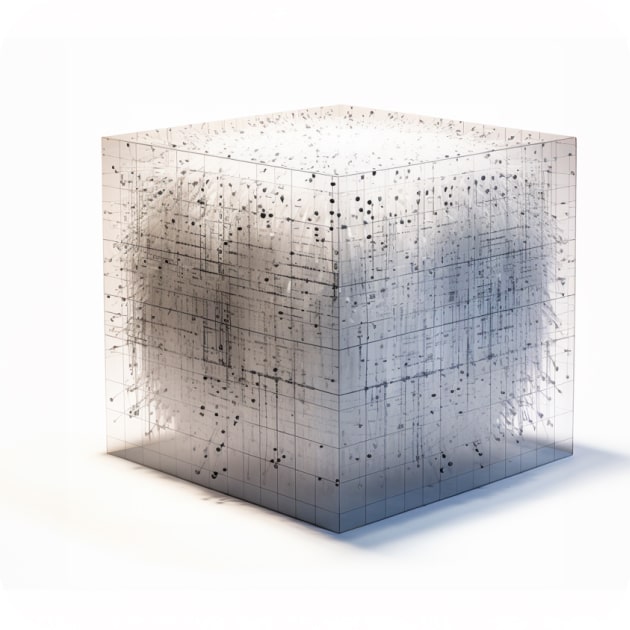
Categorize
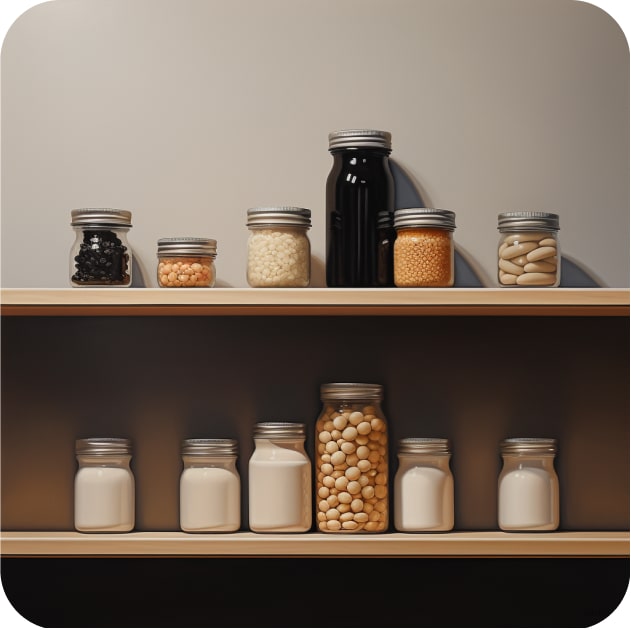
Checkpointing
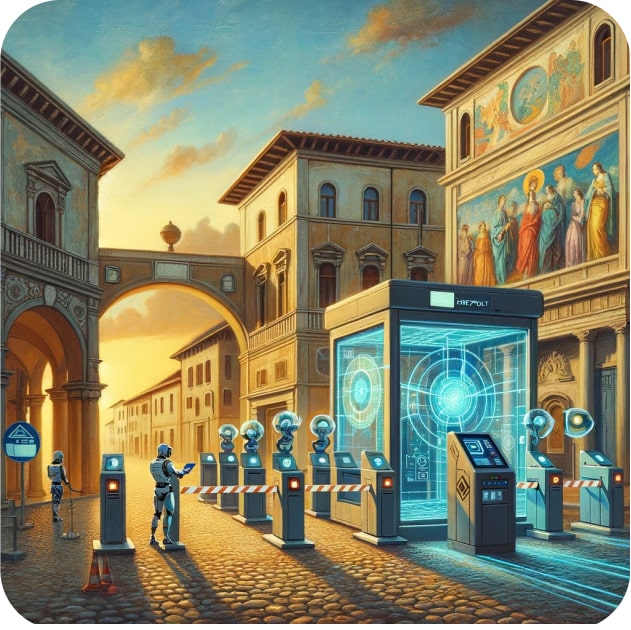
Deduplicate
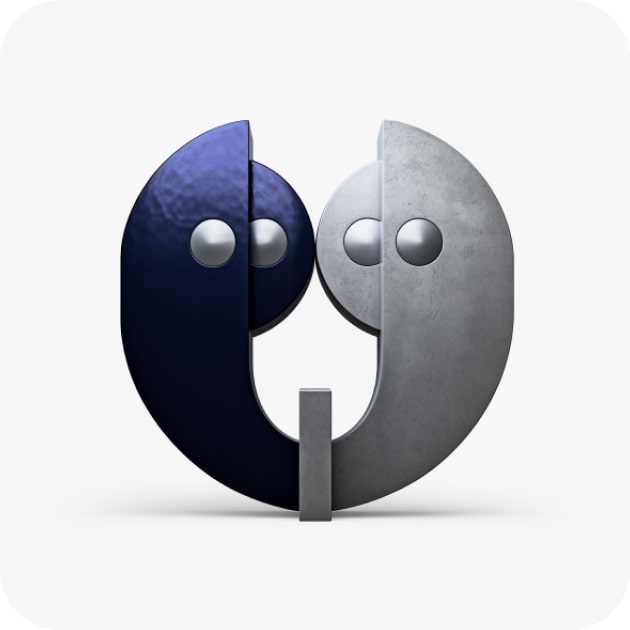
Deserialize
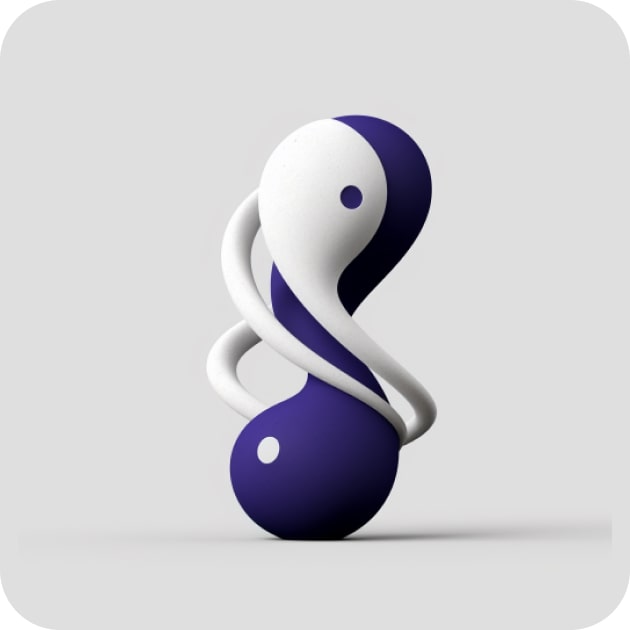
Dimensionality
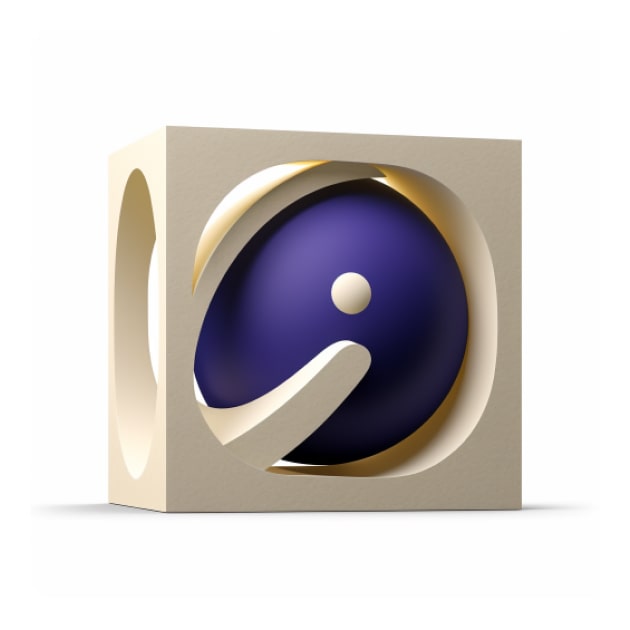
Encapsulate
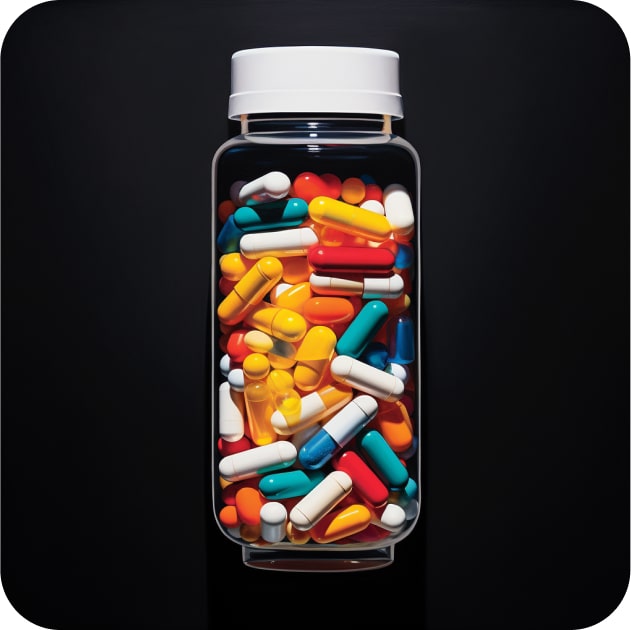
Enrich
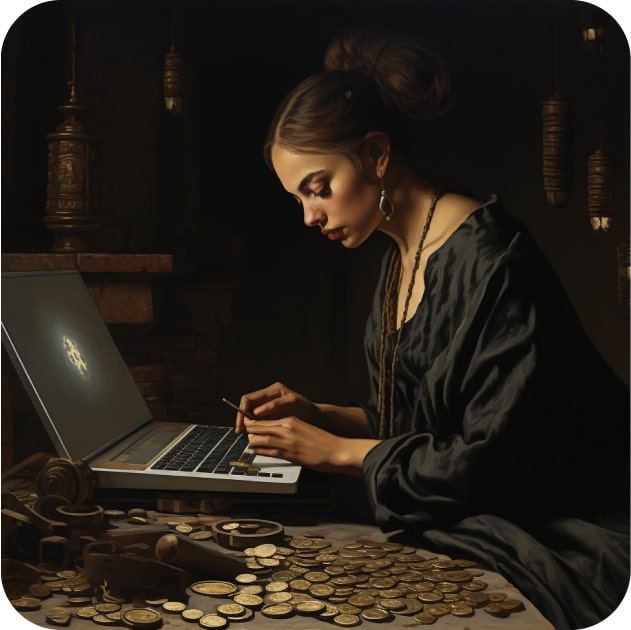
Export
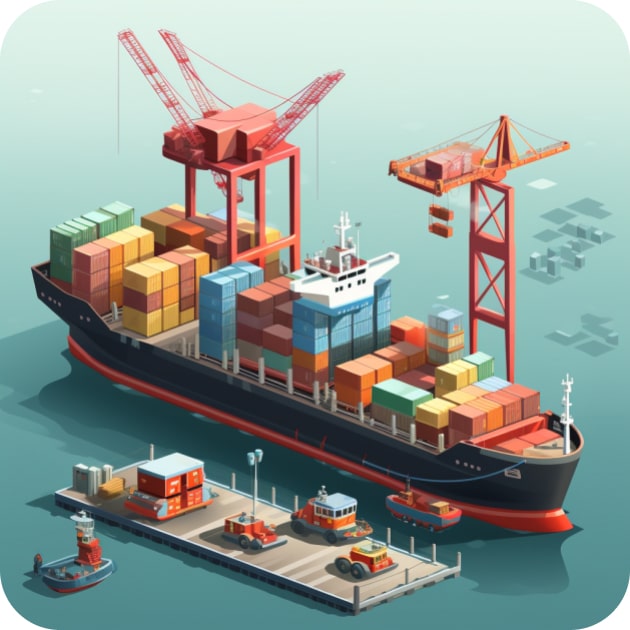
Graph Theory
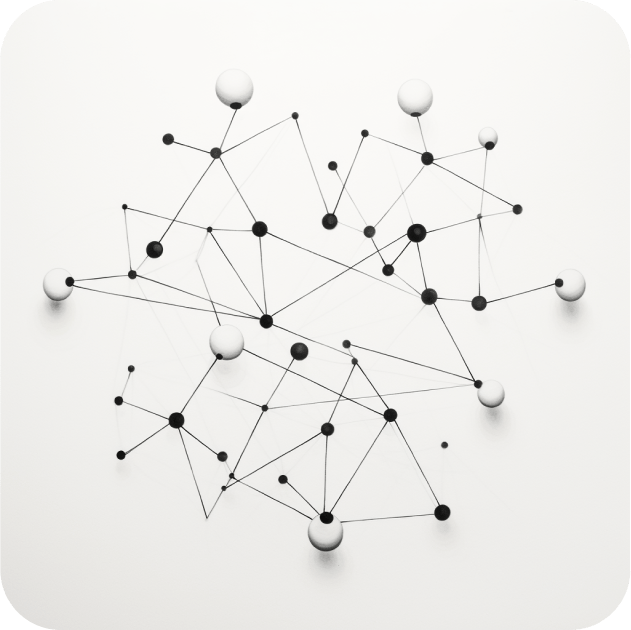
Index
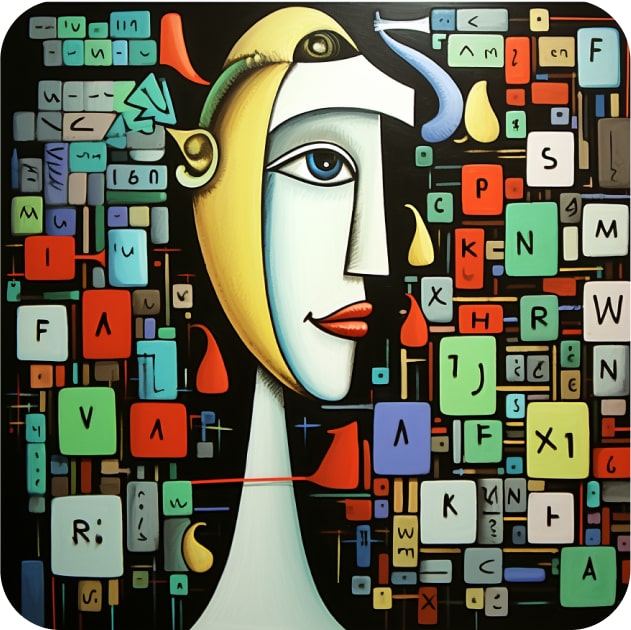
Integrate
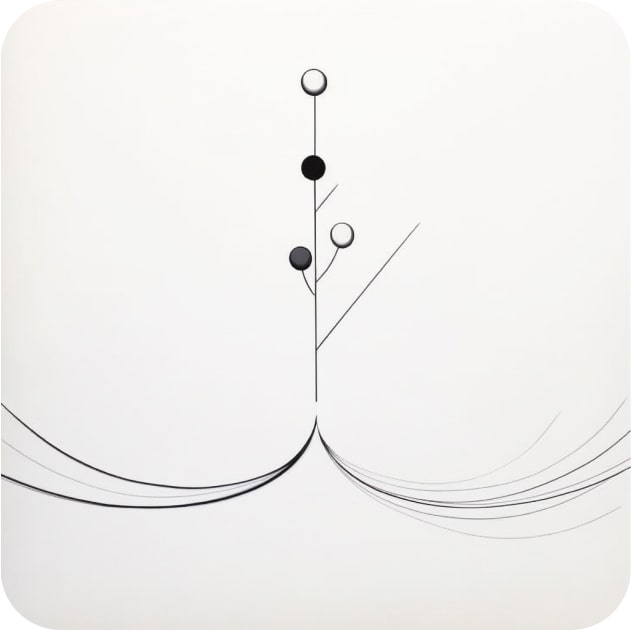
Lineage
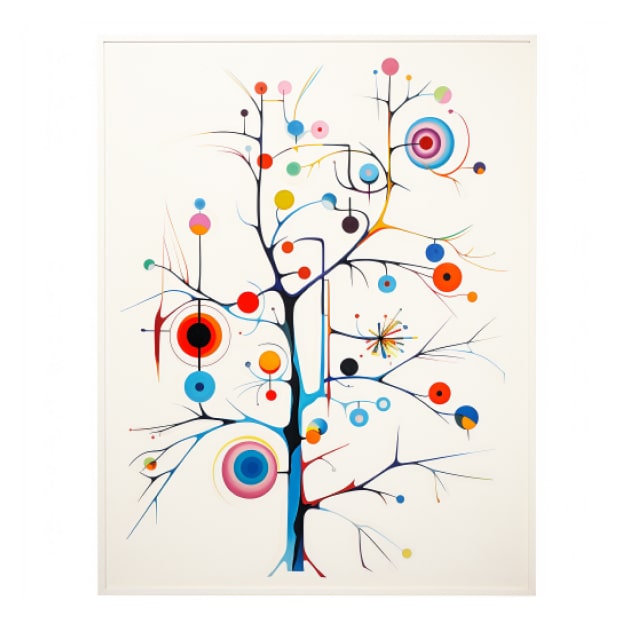
Linearizability
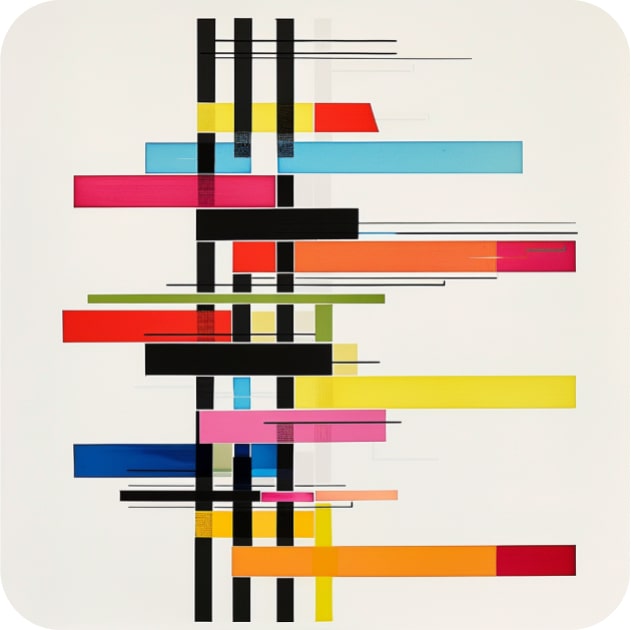
Materialize
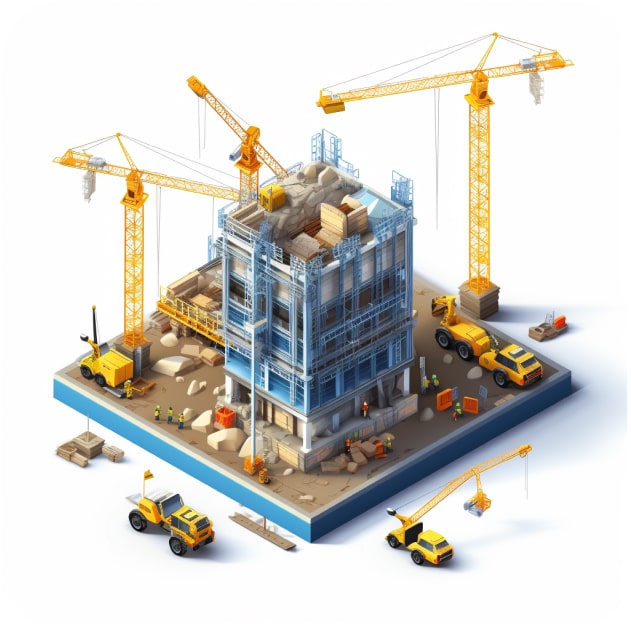
Memoize
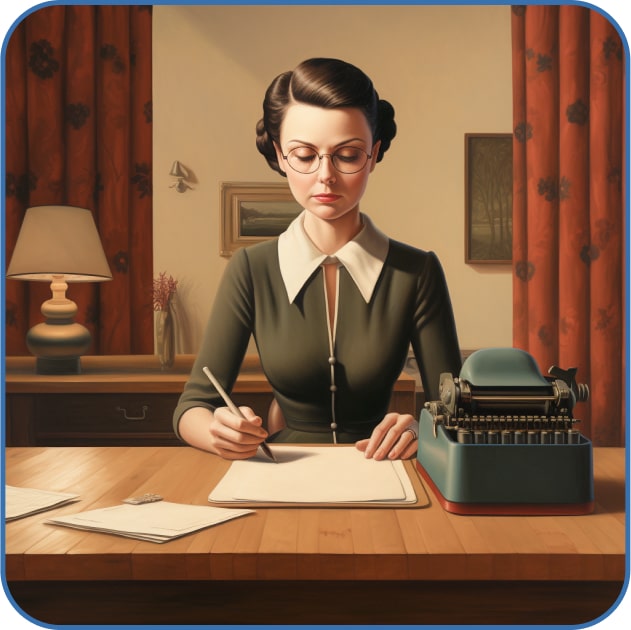
Merge
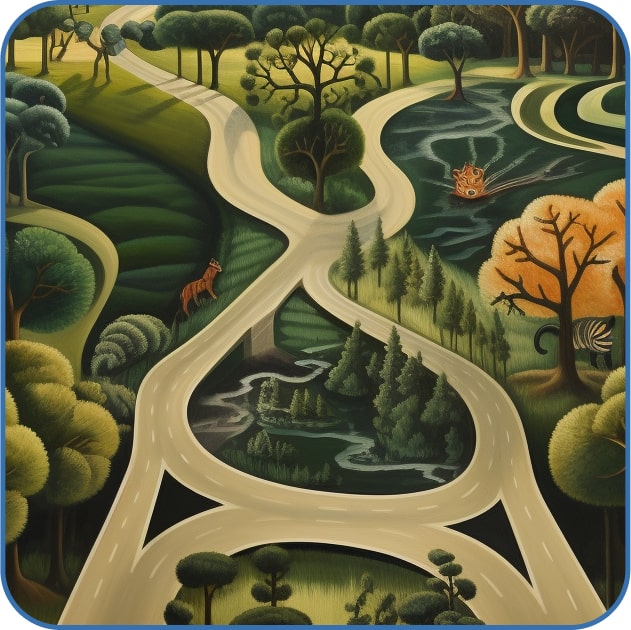
Model
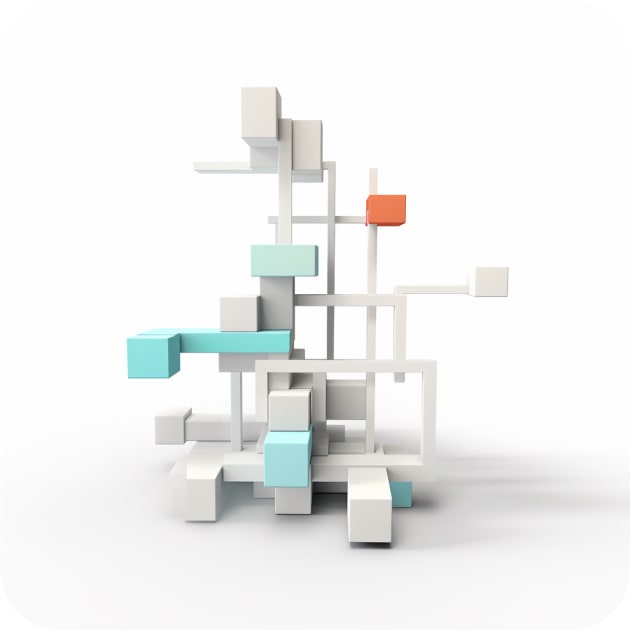
Monitor
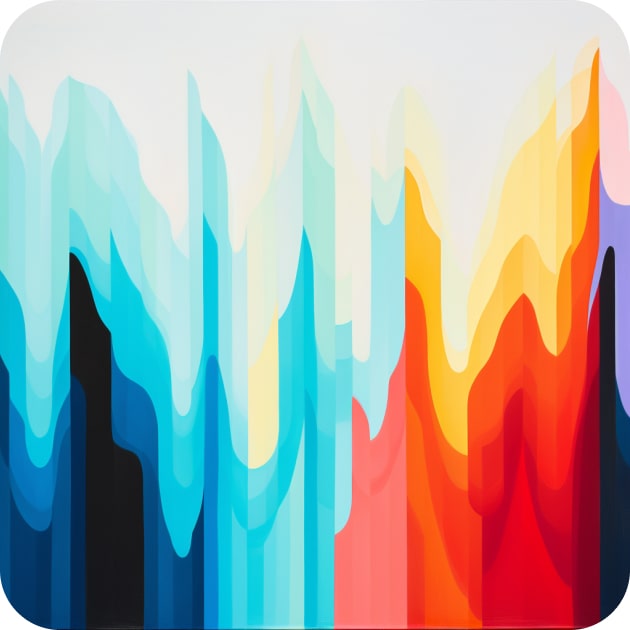
Named Entity Recognition
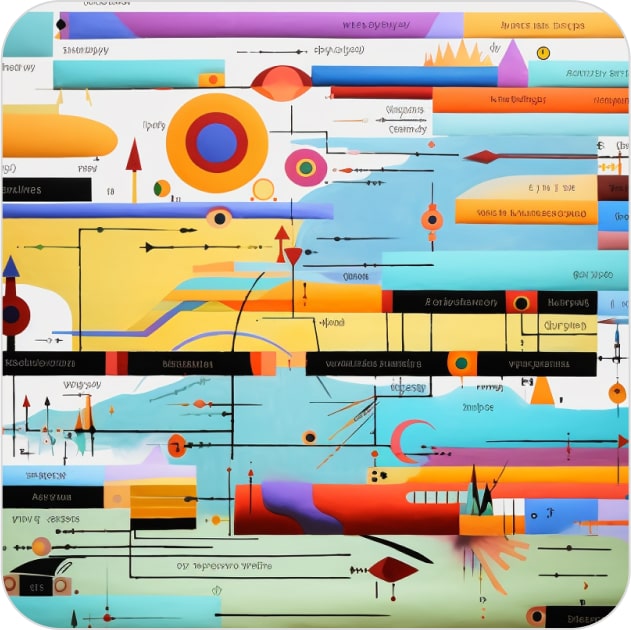
Parse
Partition
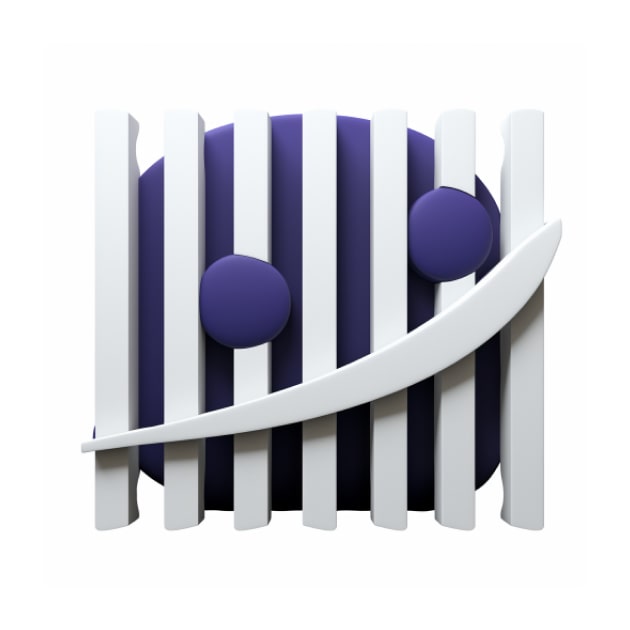
Prep
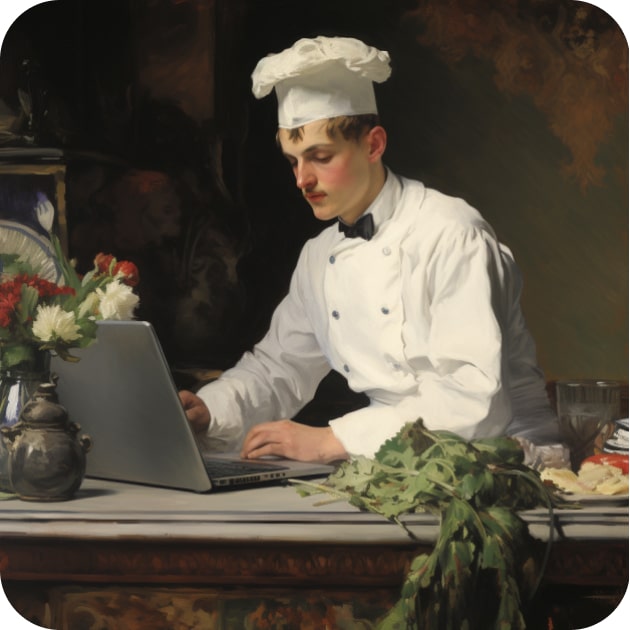
Preprocess
Primary Key
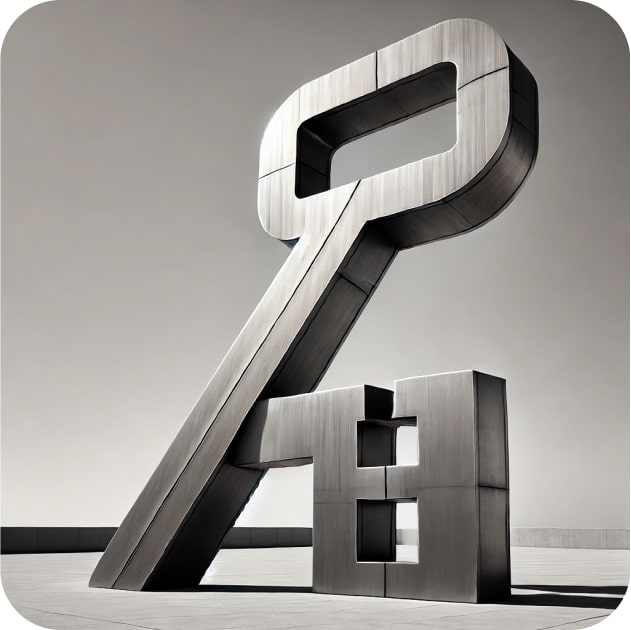
Replicate
Scaling
Schema Inference
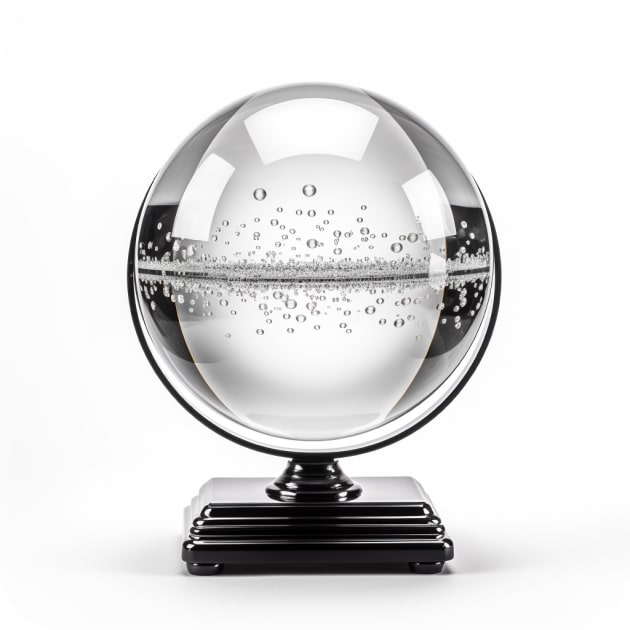
Schema Mapping
Secondary Index
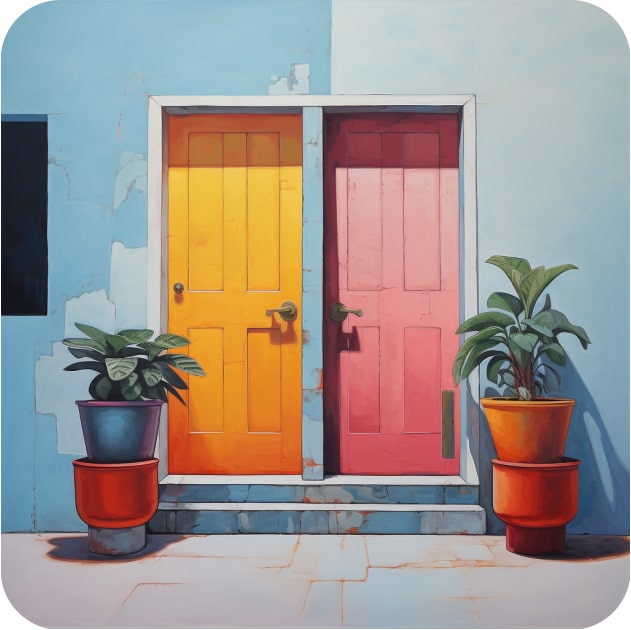
Software-defined Asset
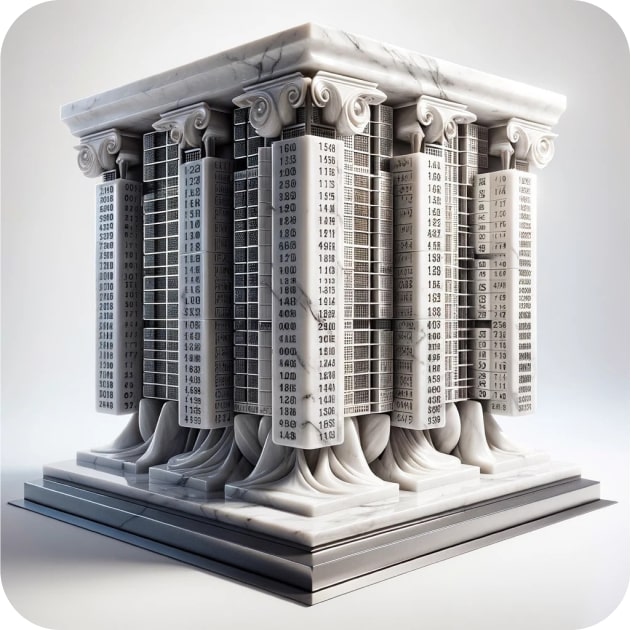
Synchronize
Validate
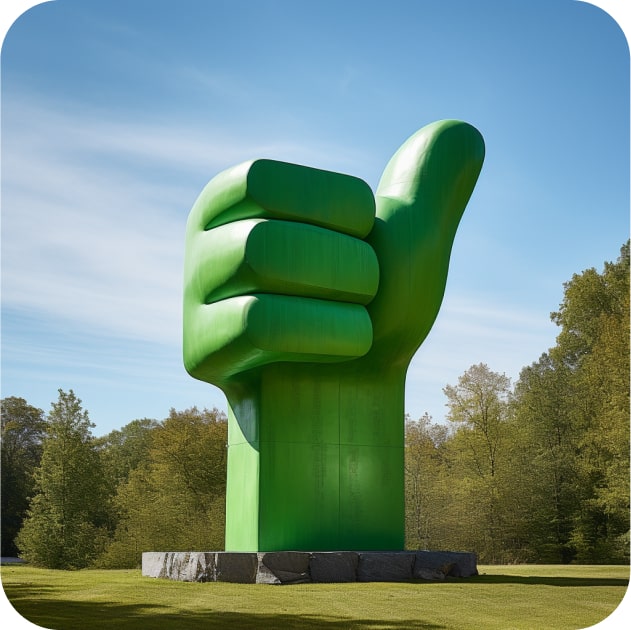
Version
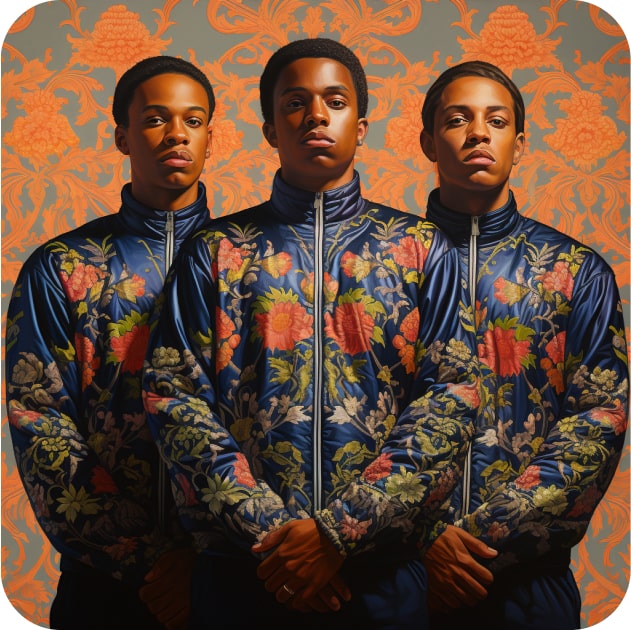