Data Encapsulation
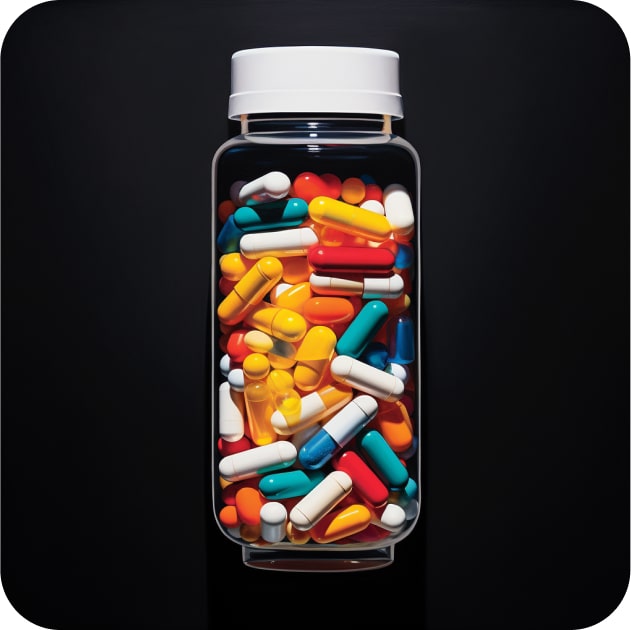
Definition of Data Encapsulation:
Data encapsulation is the practice of bundling data with the methods that operate on that data, while restricting direct access to some of an object's components.
Why Data Encapsulation is a fundamental concept in data engineering:
This fundamental concept is crucial in data engineering for several reasons:
Modularity: Encapsulation promotes the design of modular systems. By keeping data and its associated operations together, systems become more organized, making them easier to understand, develop, and maintain.
Information Hiding: One of the key aspects of encapsulation is information hiding. This means that the internal state of an object is hidden from the outside. Only the object's own methods can access and modify this state, which protects the integrity of the data and prevents external entities from causing inconsistencies or errors.
Abstraction: Encapsulation allows for a high level of abstraction. Users or other parts of the system interact with an object through a well-defined interface (methods or functions), without needing to understand the complexities of its internal workings.
Reusability and Maintenance: Encapsulated code is often more reusable and easier to maintain. Since changes to the internal workings of an object do not affect other parts of the system, updates and bug fixes are more straightforward.
Security: In data engineering, security is paramount, and encapsulation can play a role in securing data. By controlling how data is accessed and modified, and who has the authority to do so, encapsulation helps in maintaining data integrity and security.
Uses of data encapsulation:
In modern data engineering, encapsulation is used in various forms, such as:
Object-Oriented Programming (OOP): This is the most direct implementation of encapsulation. Classes in OOP languages like Python, Java, or C++ encapsulate data and methods.
Data APIs: Encapsulation is also seen in how data is accessed and manipulated through APIs. APIs provide a controlled interface to data sources, ensuring that data is accessed in a structured and secure manner.
Data Storage and Management: Encapsulation is crucial in data management systems, like databases, where the internal structure of the database is hidden. Users interact with the data through a set of predefined queries and operations, without needing to know how the data is stored or maintained internally.
Microservices Architecture: In a microservices architecture, each microservice encapsulates a specific functionality or data set. This encapsulation ensures that services are loosely coupled and can be developed and scaled independently.
Example of data encapsulation in Python
Let's design a Python class that simulates a more complex data processing pipeline. This pipeline will include data ingestion, cleaning, transformation, and storage. The example will showcase encapsulation, abstraction, and modular design.
We will create a DataPipeline
class that encapsulates all the steps in a data processing workflow:
- Data Ingestion: Load data from a source (in this case a .csv file).
- Data Cleaning: Clean the data to ensure quality.
- Data Transformation: Transform the data into a format suitable for analysis, including some values derived from the source data.
- Data Storage: Store the processed data in a .csv file system.
Here's an implementation:
import pandas as pd
class DataPipeline:
def __init__(self, source_config):
self.__source_config = source_config
self.__data = None
def __load_data(self):
# Private method to load data based on the source configuration
if self.__source_config['type'] == 'csv':
self.__data = pd.read_csv(self.__source_config['path'])
def __clean_data(self):
# Convert 'Date' from string to datetime
self.__data['Date'] = pd.to_datetime(self.__data['Date'])
# Handling missing values - Example: fill with mean or drop
self.__data.fillna(self.__data.mean(numeric_only=True), inplace=True)
# Handling outliers - Example: using a simple Z-score method for demonstration
# This is a basic approach and can be replaced with more sophisticated methods as needed.
for col in ['Temperature', 'Humidity', 'WindSpeed']:
z_scores = (self.__data[col] - self.__data[col].mean()) / self.__data[col].std()
self.__data = self.__data[abs(z_scores) < 3]
# Ensuring correct data types and number of decimals
self.__data['Temperature'] = self.__data['Temperature'].astype(float).round(4)
self.__data['Humidity'] = self.__data['Humidity'].astype(float).round(2)
self.__data['WindSpeed'] = self.__data['WindSpeed'].astype(int)
def __transform_data(self):
# Example: Aggregate data by 'Date' if it's a time series with multiple entries per date.
# This example assumes daily aggregation, taking the mean of the values.
self.__data = self.__data.groupby('Date').agg({
'Temperature': 'mean',
'Humidity': 'mean',
'WindSpeed': 'mean'
}).reset_index()
# Creating new features - Example: 'FeelsLike' temperature using a simple formula
# This is a hypothetical formula just for demonstration.
self.__data['FeelsLike'] = self.__data['Temperature'] - 0.1 * self.__data['WindSpeed']
# Time Series Features - Adding day of the week, month as new columns
self.__data['DayOfWeek'] = self.__data['Date'].dt.day_name()
self.__data['Month'] = self.__data['Date'].dt.month
def __store_data(self, target_config):
# Private method to store data
if target_config['type'] == 'csv':
self.__data.to_csv(target_config['path'])
def execute_pipeline(self, target_config):
# Public method to execute the entire pipeline
self.__load_data() # Step 1: Load the data
self.__clean_data() # Step 2: Clean the data
self.__transform_data() # Step 3: Transform the data
self.__store_data(target_config) # Step 4: Store the data
# Example usage
source_config = {'type': 'csv', 'path': 'https://dagster.io/glossary/data-encapsulation.csv'}
target_config = {'type': 'csv', 'path': 'data-encapsulation-out.csv'}
pipeline = DataPipeline(source_config)
pipeline.execute_pipeline(target_config)
In this example:
Modularity: Each step of the data processing workflow is encapsulated into a private method. This modular approach makes the code more maintainable and scalable.
Information Hiding and Abstraction: The internal implementation of data loading, cleaning, transforming, and storing is hidden from the user. The user interacts with the
DataPipeline
class through theexecute_pipeline
method.Flexibility and Scalability: The design allows for easy extension to support additional data sources and storage options. You can add more complex logic to each method to handle a wide range of data processing tasks.
This example provides a foundational structure that can be expanded and customized for specific needs in large-scale data engineering projects. The encapsulation of data and methods within the DataPipeline
class facilitates easy maintenance and scalability, key considerations in complex data engineering environments.
Append
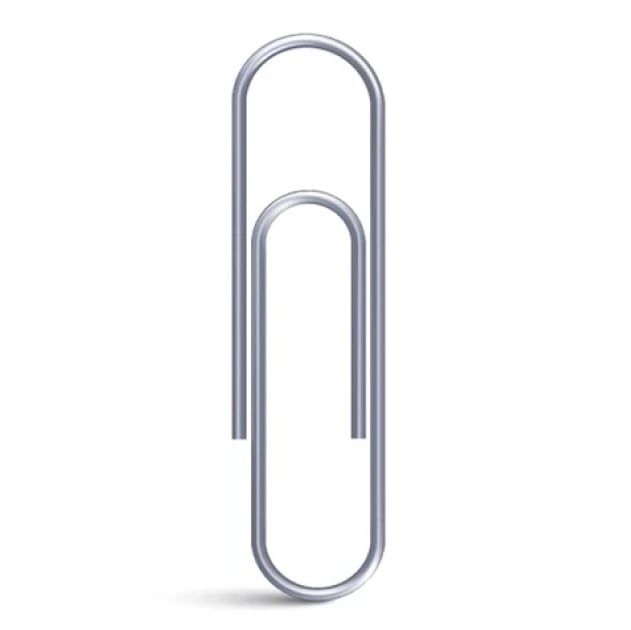
Archive
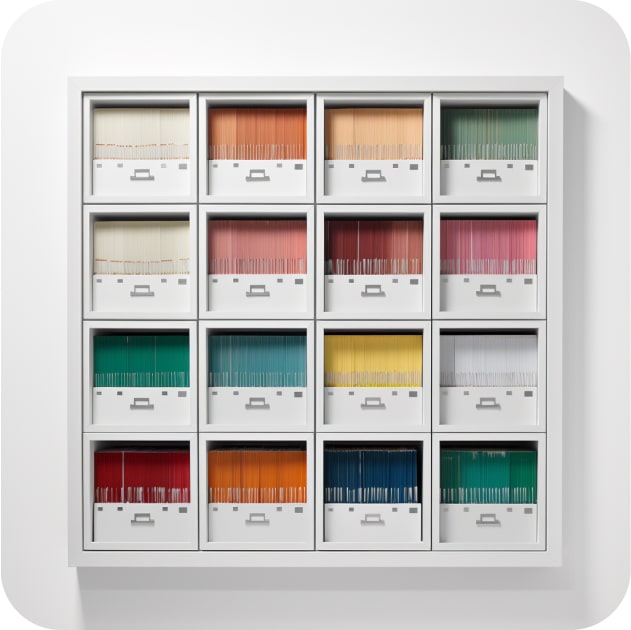
Augment
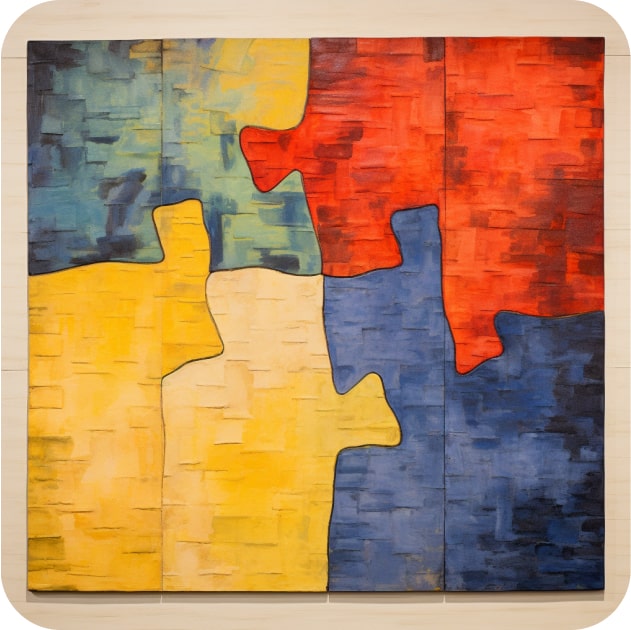
Auto-materialize
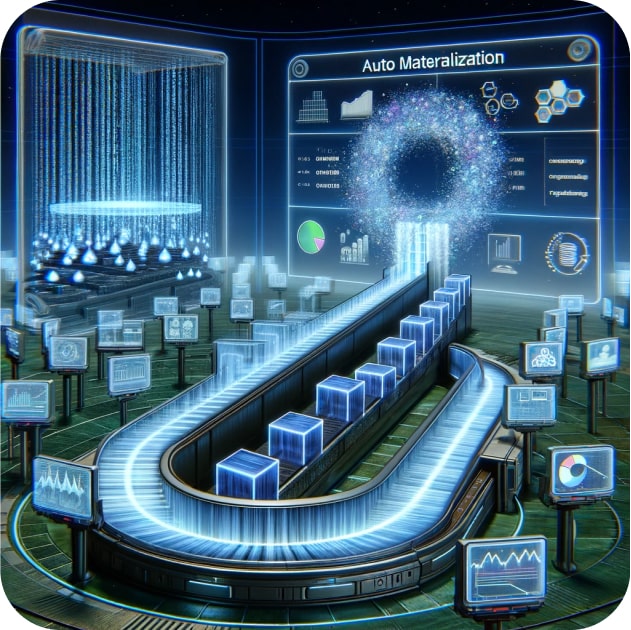
Backup
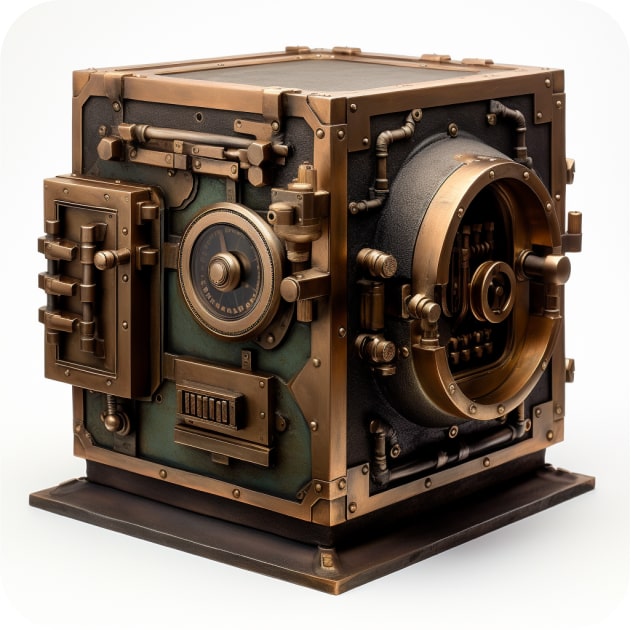
Batch Processing
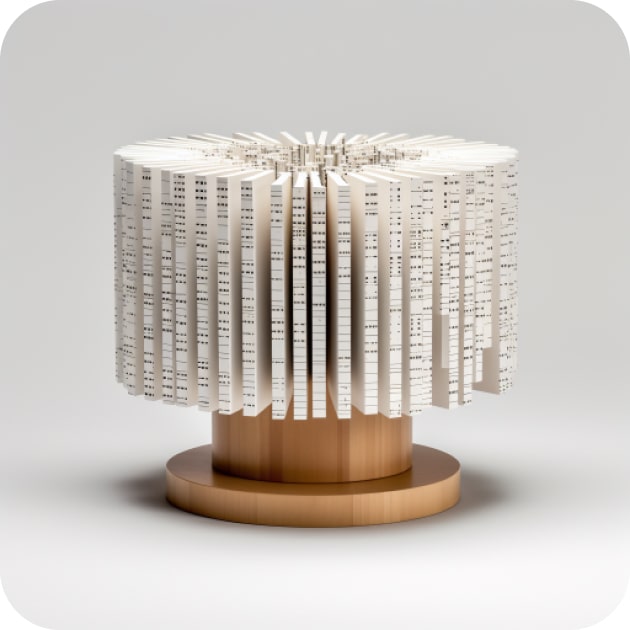
Cache
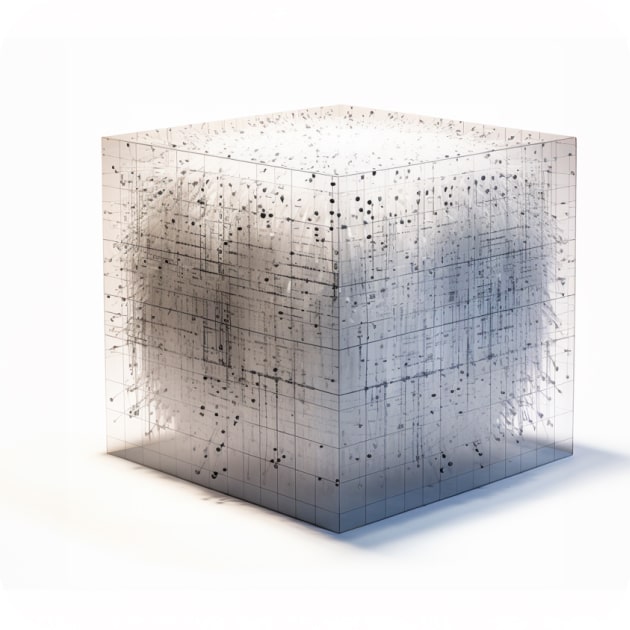
Categorize
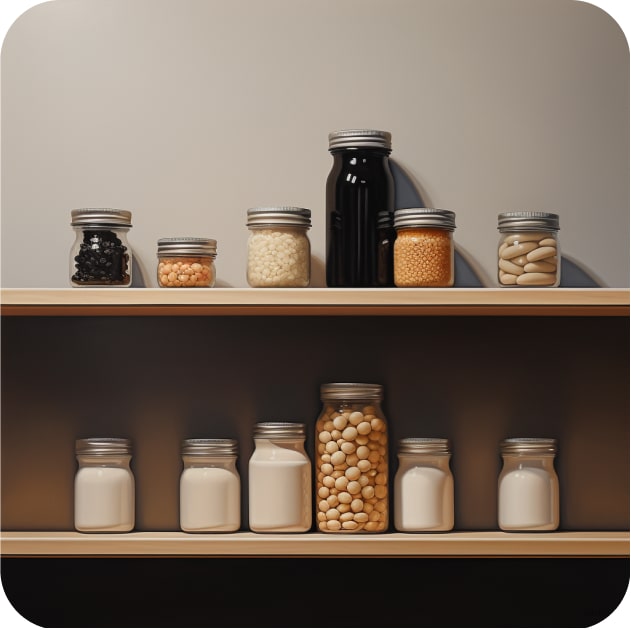
Deduplicate
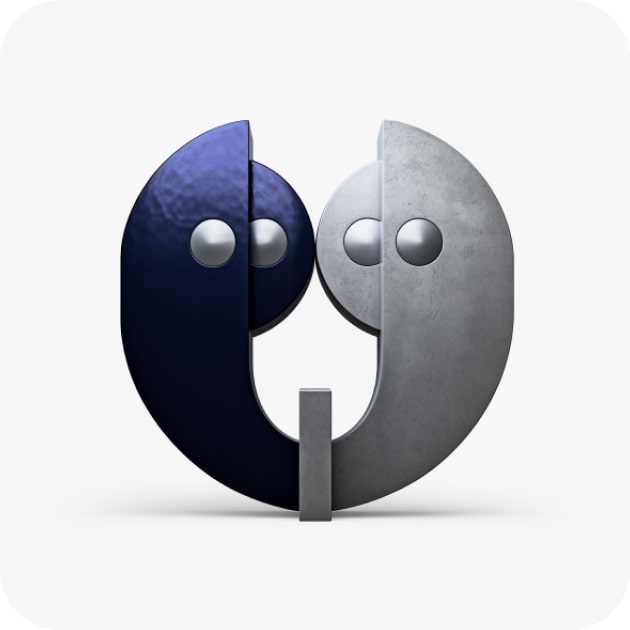
Deserialize
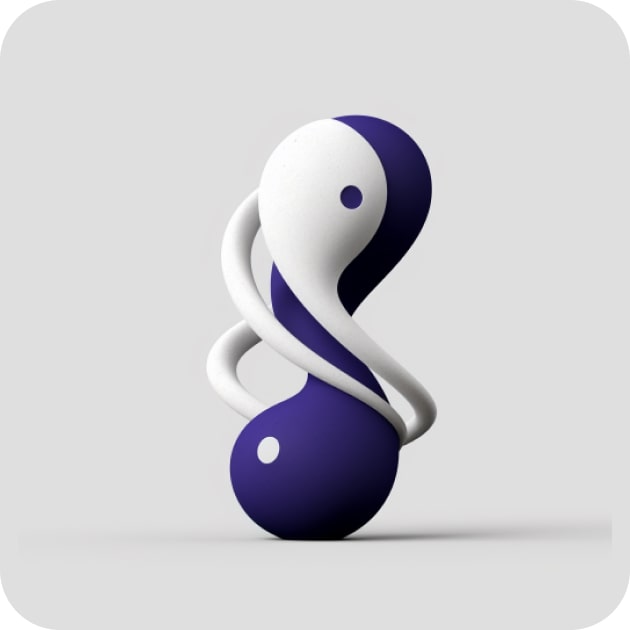
Dimensionality
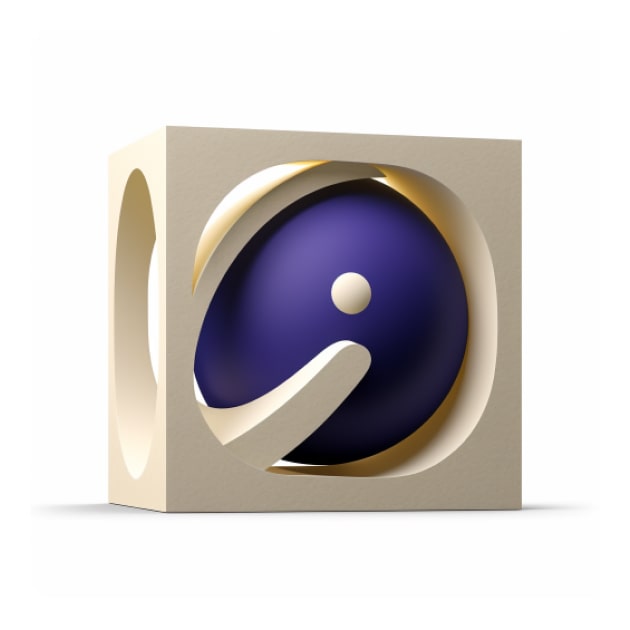
Enrich
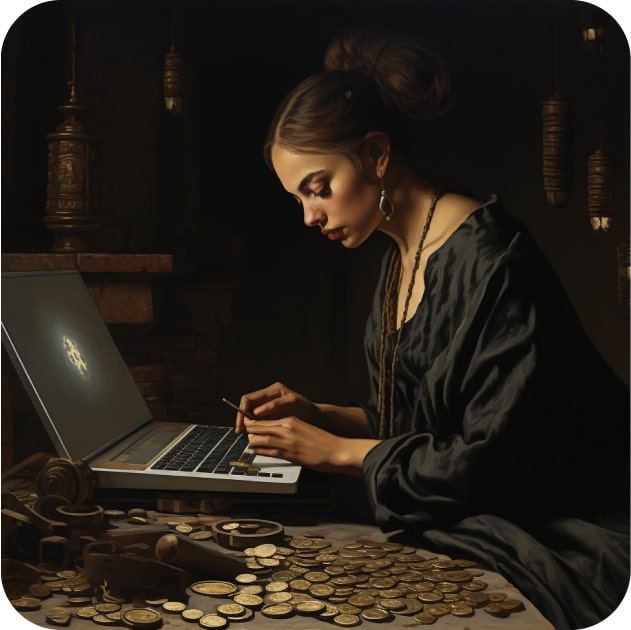
Export
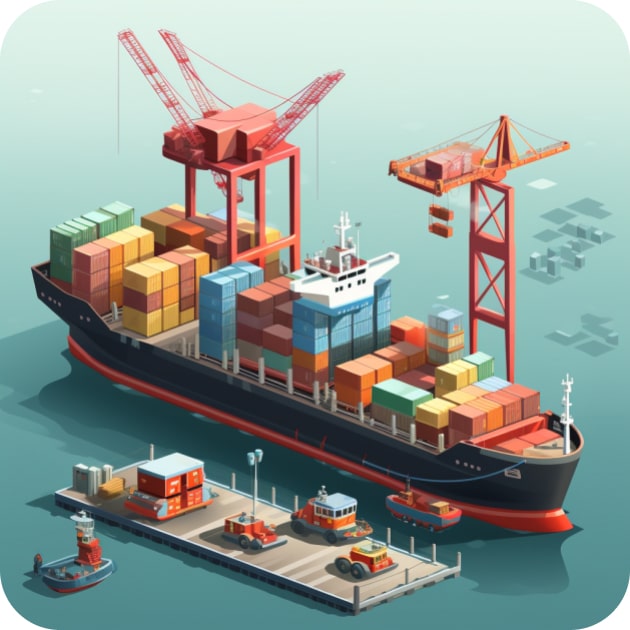
Graph Theory
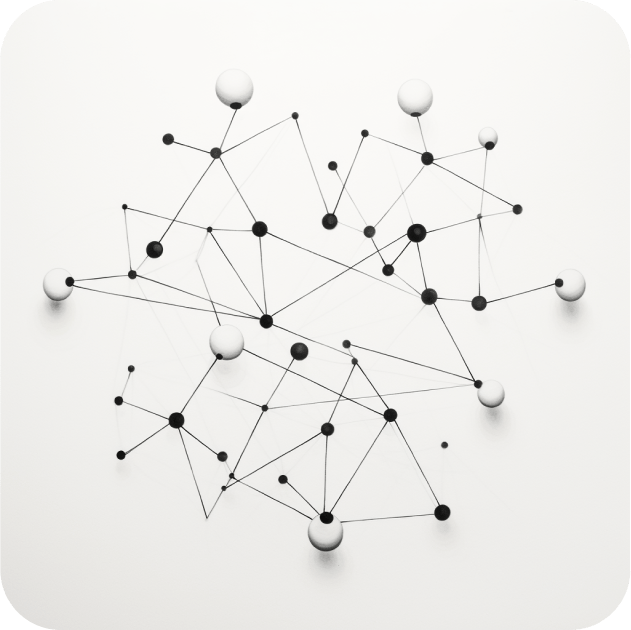
Idempotent
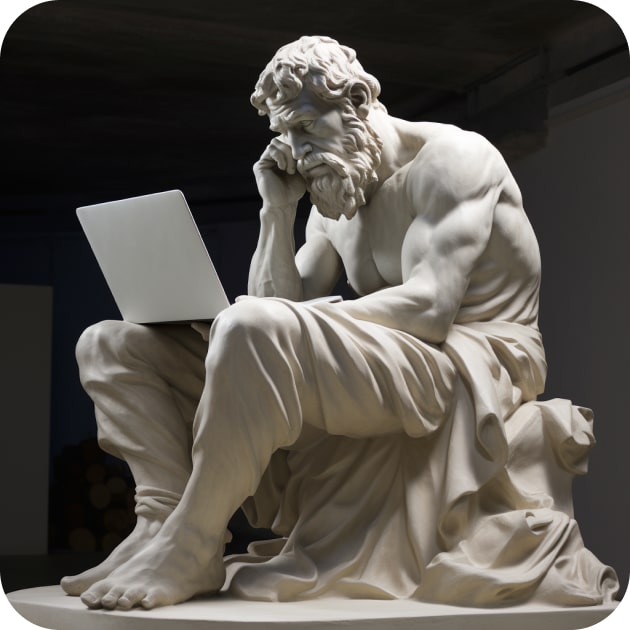
Index
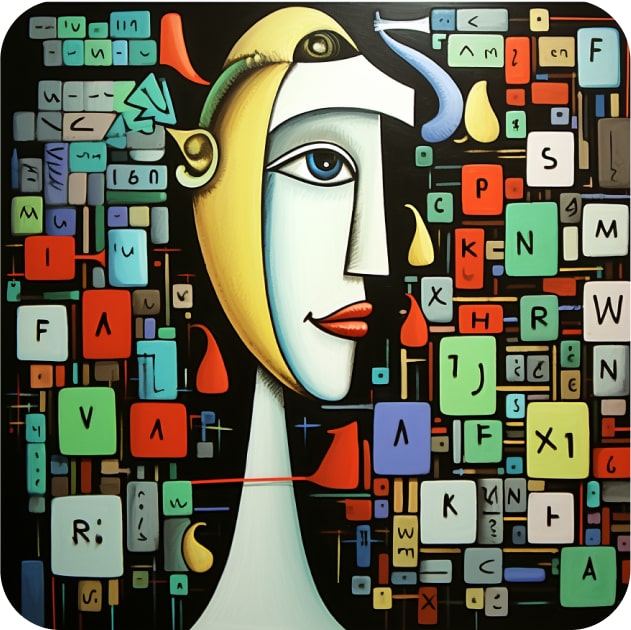
Integrate
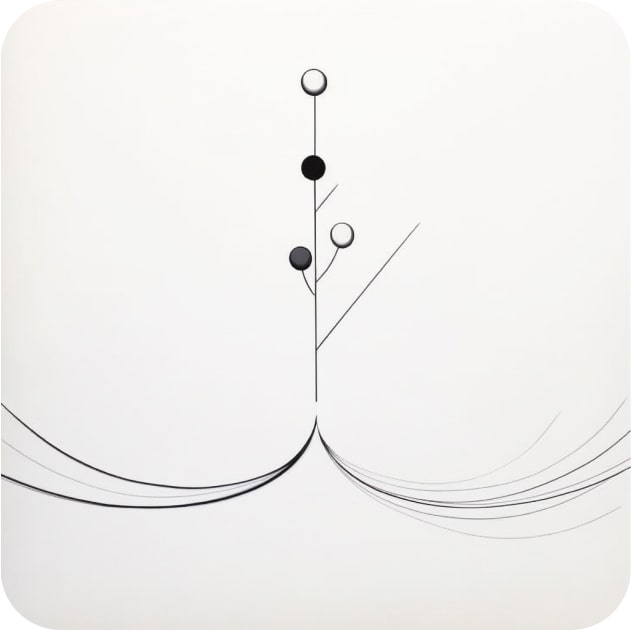
Lineage
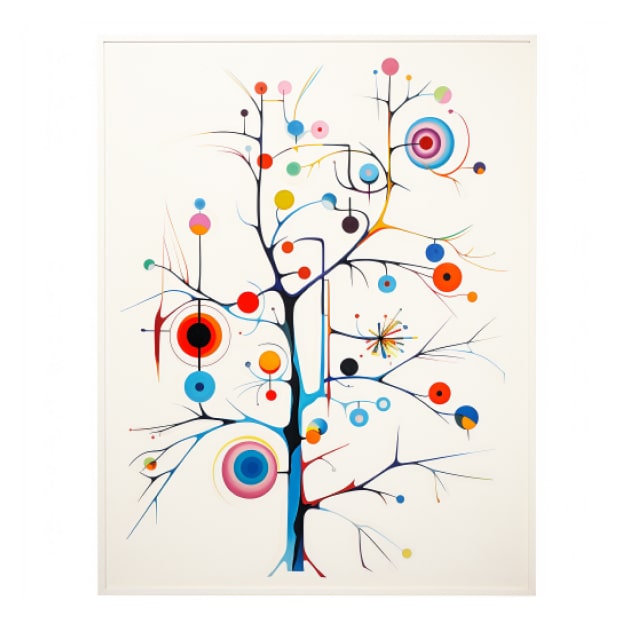
Linearizability
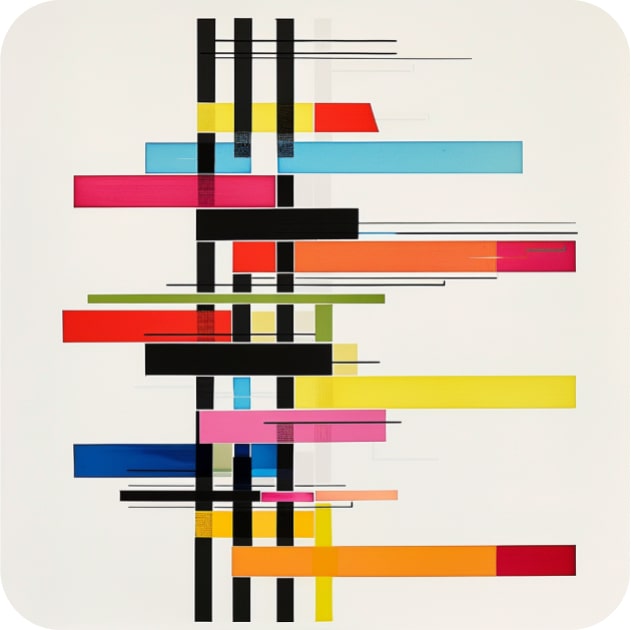
Materialize
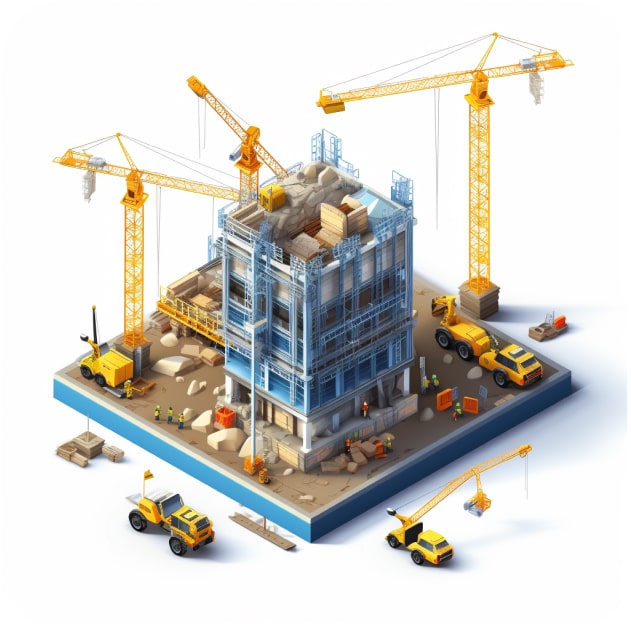
Memoize
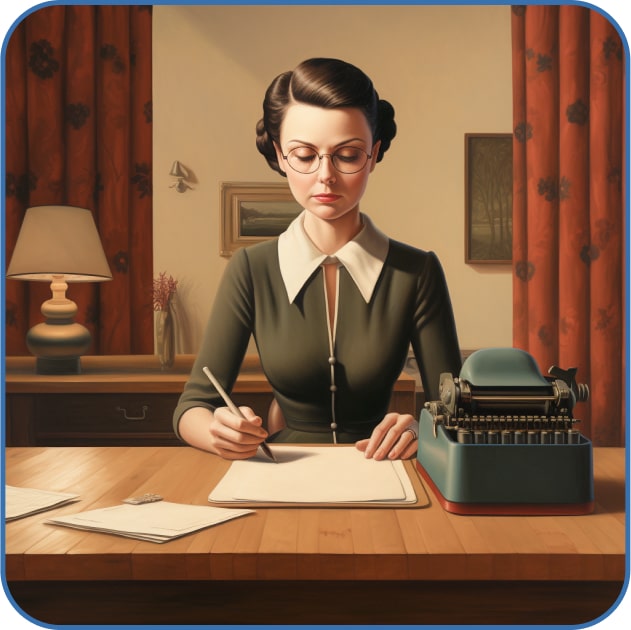
Merge
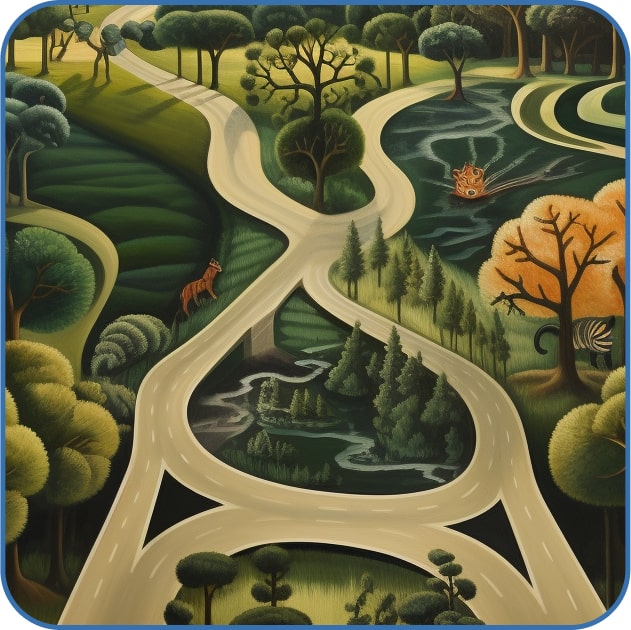
Model
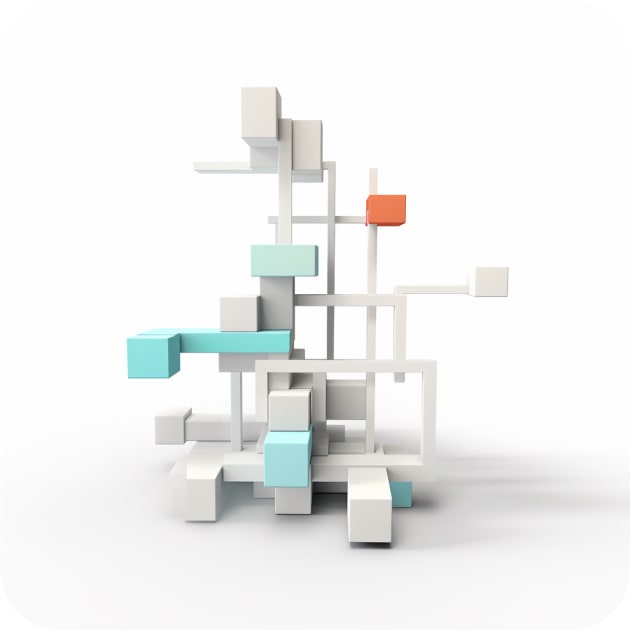
Monitor
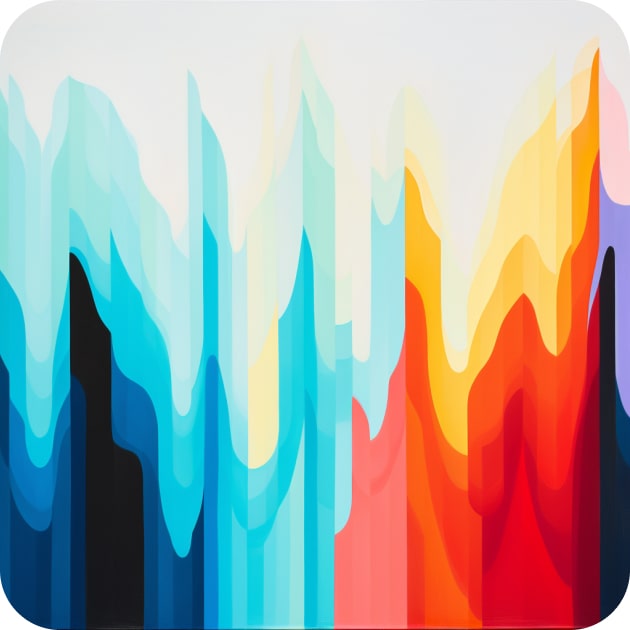
Named Entity Recognition
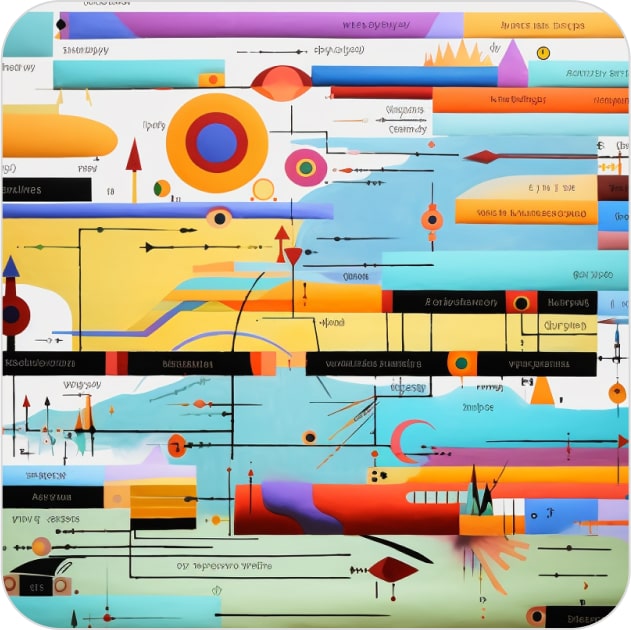
Parse
Partition
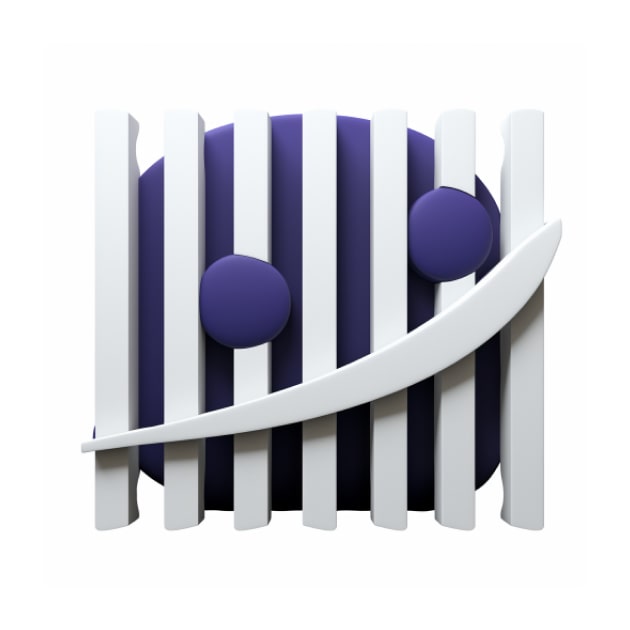
Prep
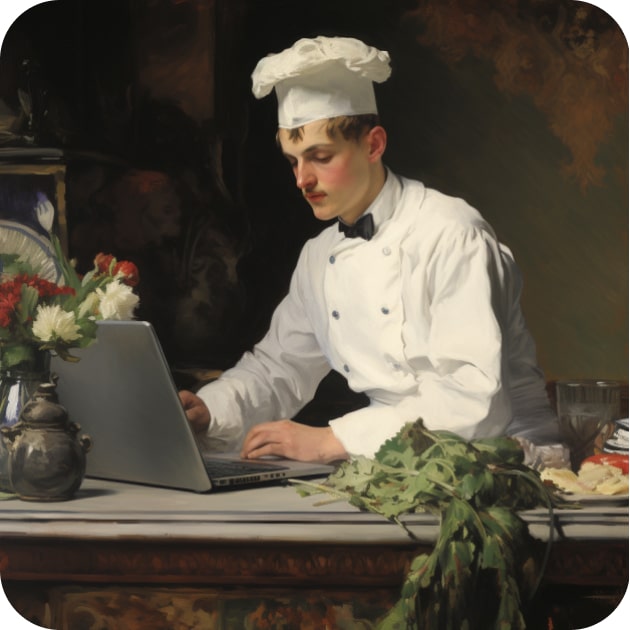
Preprocess
Replicate
Scaling
Schema Inference
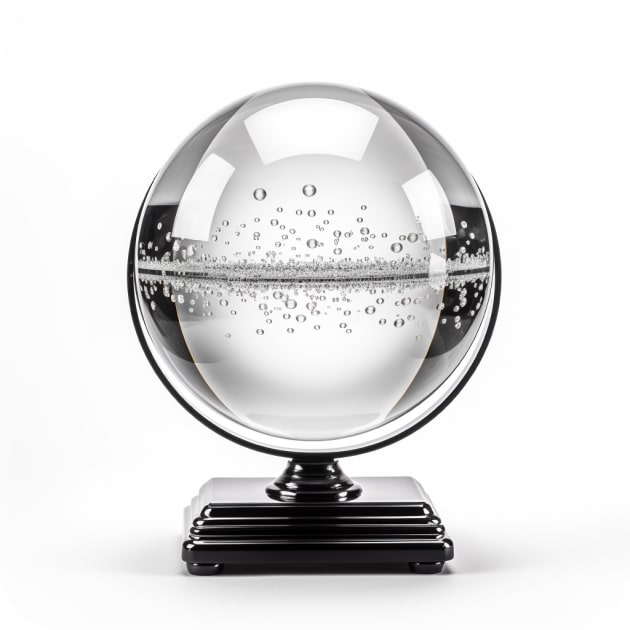
Schema Mapping
Secondary Index
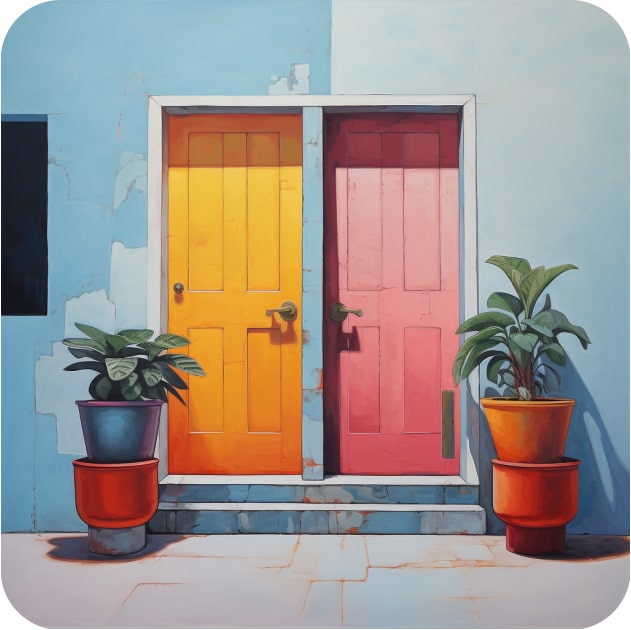
Software-defined Asset
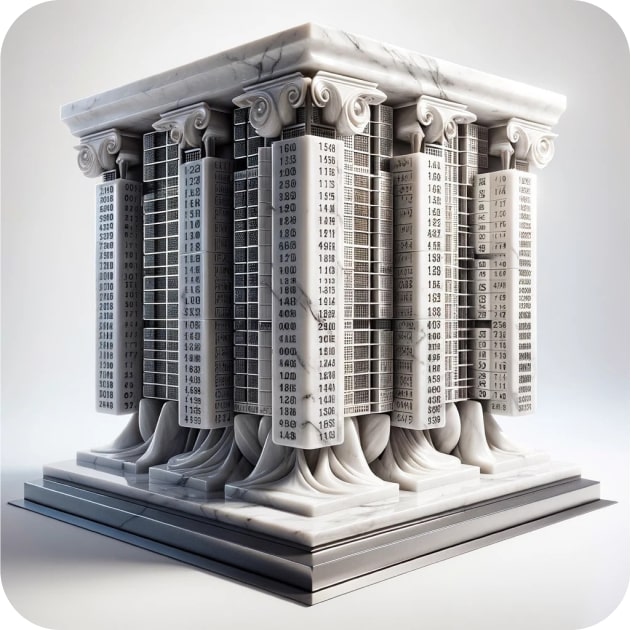
Synchronize
Validate
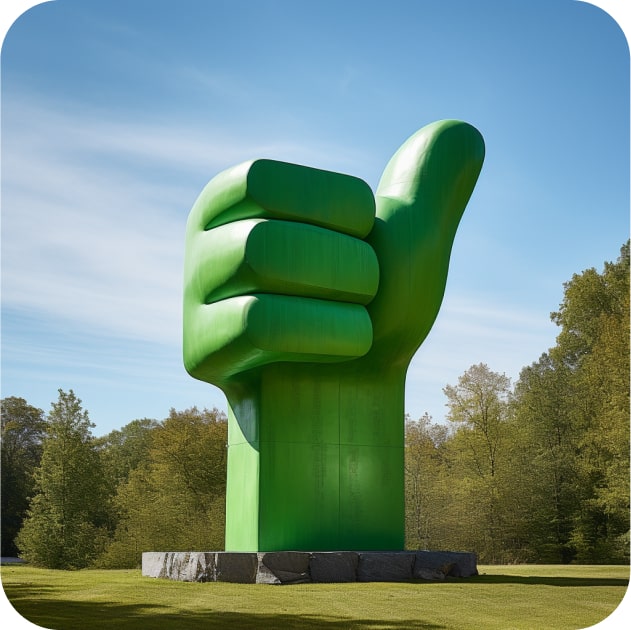
Version
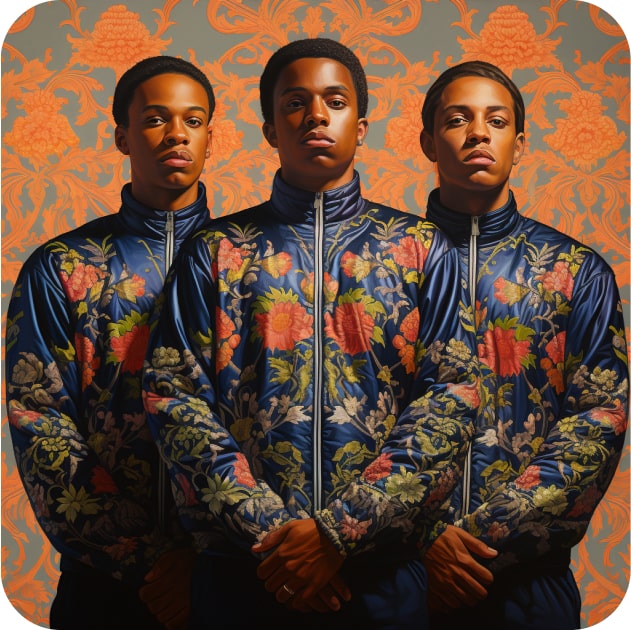