Dagster Data Engineering Glossary:
Batch Processing
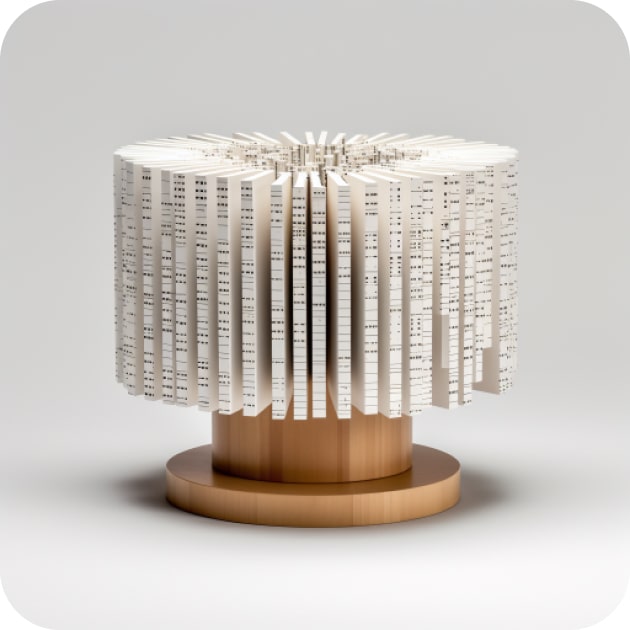
Definition of batch processing:
Batch processing in data engineering refers to the practice of processing large volumes of data all at once in a single operation or 'batch'. This method is often utilized when the data doesn't need to be processed in real-time and can be processed without the need for user interaction. Batch processing is most effective when dealing with large amounts of data where it's more efficient to load and process the data in chunks, rather than one record at a time.
Batch processing steps
Batch processing usually involves four main steps:
- Gathering data: Data is accumulated over a period of time.
- Input: The collected data is loaded into the system.
- Processing: The system executes the pre-defined process.
- Output: The final result is delivered, which could be a report, updates to a database, or other types of output.
Example of batch processing in Python
For example, consider a bank that needs to update its customers' balances overnight. Rather than updating each balance as a separate task, the bank could gather all transactions made during the day and process them together in a single batch during off-peak hours.
Here's a simple Python script that illustrates the concept of batch processing by reading a large CSV file in chunks, performing a calculation, and then saving the results to a new CSV file.
Please note that you need to have the necessary Python libraries installed in your Python environment to run this code.
Say we have a file large_input.csv
as follows:
column1,column2
10,5
20,4
30,3
40,2
50,1
15,6
25,7
35,8
45,9
55,10
...
Our batch processing script might look like this:
import pandas as pd
chunksize = 10 ** 6 # Number of lines to process at each iteration
i = 0
for chunk in pd.read_csv('large_input.csv', chunksize=chunksize):
# Perform some kind of processing, for example, adding a new column which is a calculation of two existing columns
chunk['new_column'] = chunk['column1'] * chunk['column2']
if i == 0:
# Save the first chunk to the output file, creating it
chunk.to_csv('output.csv', index=False)
else:
# Append subsequent chunks to the output file
chunk.to_csv('output.csv', mode='a', header=False, index=False)
i += 1
This script reads the large_input.csv
file in chunks, processes each chunk by performing a calculation and adding a new column, then writes the processed chunk to output.csv
. This is a form of batch processing as it allows the script to process a large file that might not fit in memory by breaking it into manageable chunks.
Best practices in implementing batch processing:
Batch processing can be a highly efficient way to handle large data sets, but like any method, it can be further optimized to improve performance and properly manage costs. Here are some general guidelines to consider when optimizing batch processing in data engineering:
Parallel Processing: If the batch processing tasks are independent and can be performed in parallel, distributing the work across multiple machines can significantly speed up processing time.
Optimize Chunk Size: If your system allows you to process data in chunks, finding the right chunk size can improve efficiency. Too small, and the overhead of initiating the process can slow things down. Too large, and you may run into memory issues. The optimal chunk size depends on the specific computations you're performing and your chosen infrastructure.
Schedule During Off-Peak Times: If possible, schedule batch processing tasks to run during off-peak hours when there's less competition for resources. This is where an orchestrator like Dagster can greatly improve your efficiency.
Preprocessing: Reduce the volume of data processed in the batch by performing filtering, aggregation, or downsampling during a preprocessing step.
Optimize Code: As with any programming task, ensure your code is as efficient as possible. This might include using built-in functions, optimizing your use of data structures, or minimizing expensive operations like disk I/O.
Monitor and Adjust: Continually monitor the performance of your batch processing tasks. Identify any bottlenecks or areas of inefficiency and adjust as necessary. This might involve upgrading hardware, modifying your algorithms, or changing your data structures.
Error Handling: Make sure your batch processes can handle errors gracefully. If a single operation fails, it shouldn't cause the entire batch to fail. A well-designed error handling system can also make it easier to identify and fix problems.
Data Partitioning: Partitioning the data can make the data processing and querying more efficient. This can be based on certain criteria like date, region, etc., which largely depends on how the data is accessed.
Resource Provisioning: Provision resources according to the batch job needs. Too much or too less can affect the efficiency of the job. Utilizing cloud resources can be advantageous here, as you can scale up resources when required and scale them down when not in use.
Data Caching: In case the batch job needs to reprocess some data, caching that data can save significant time and resources.
Remember that these are general guidelines, and their effectiveness can vary depending on the specific use case, available resources, and other factors. Always measure and monitor the impact of any changes you make to ensure they're having the desired effect.
Pros and Cons of batch processing in data engineering
Batch processing, as with all data processing methods, has its own set of pros and cons. These are equally dependent on the specific use case, available resources, and operational requirements. Here are some rule-of-thumb main advantages and disadvantages:
Pros of Batch Processing:
Efficiency: Batch processing can be more efficient for large volumes of data because you can often perform the same operation once for many pieces of data, rather than repeating the operation for each individual piece of data.
Resource Management: It allows for better resource utilization as heavy operations can be scheduled for off-peak times. By partitioning your data and using a data-aware orchestrator like Dagster, you can achieve highly granular control over the execution of your batch processes.
Simplicity: Batches make error handling and recovery easier because you can often simply rerun the entire batch instead of trying to find and fix individual failed operations.
Cost-effective: It can be less expensive than real-time processing because it doesn't require the system to be constantly running and waiting for new data to arrive.
Cons of Batch Processing:
Latency: The most significant drawback is the delay in data processing and availability of results, as batch processing is not real-time. This can make it unsuitable for use cases where real-time data analysis is needed.
Error Propagation: If there's a critical error in the process, the whole batch can fail, and identifying and fixing the problem might require rerunning the entire batch.
Resource Peaks: Batch processing can cause peak loads on the system when the batches are processed, especially for larger datasets or more complex operations, which could impact other processes.
Data Duplication: If your data is continually updated and the source data changes before the batch is processed, this can lead to duplicated data or the processing of outdated information.
In modern data pipelines, a combination of batch and real-time (stream) processing is often used to balance the benefits and drawbacks of each. This approach, called Lambda Architecture, provides the latency of real-time processing and the simplicity and robustness of batch processing.
Append
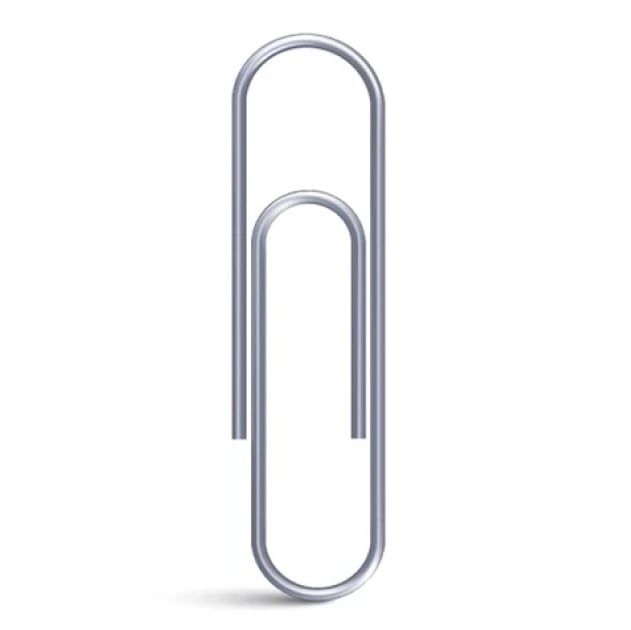
Archive
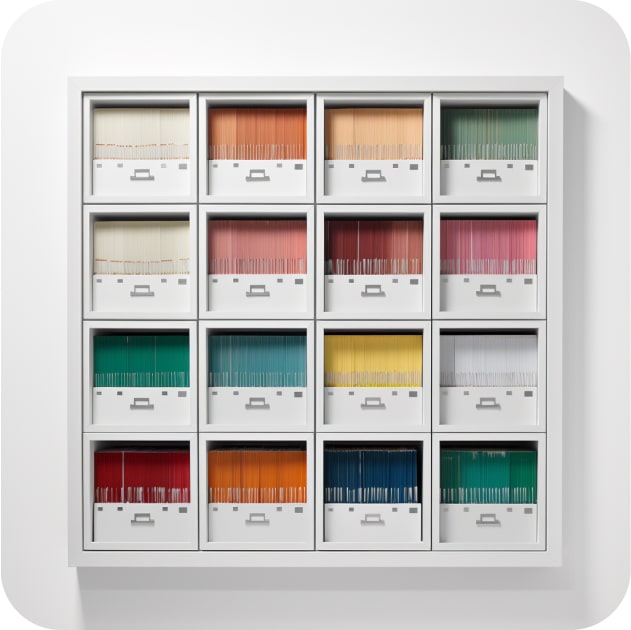
Augment
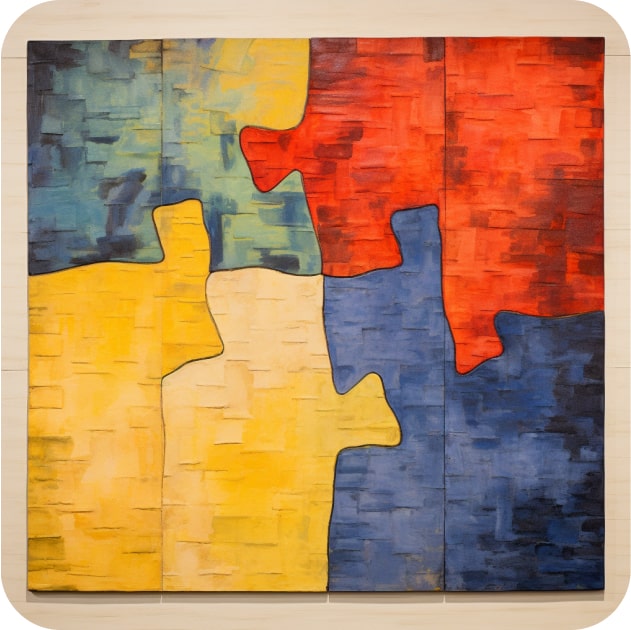
Auto-materialize
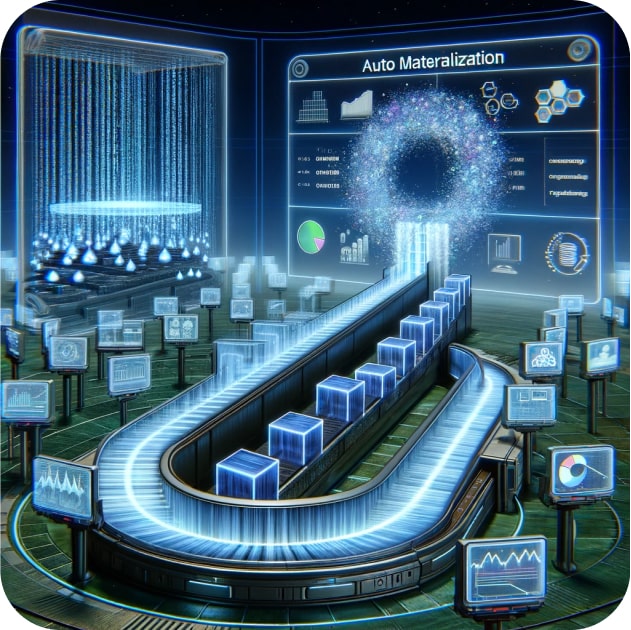
Backup
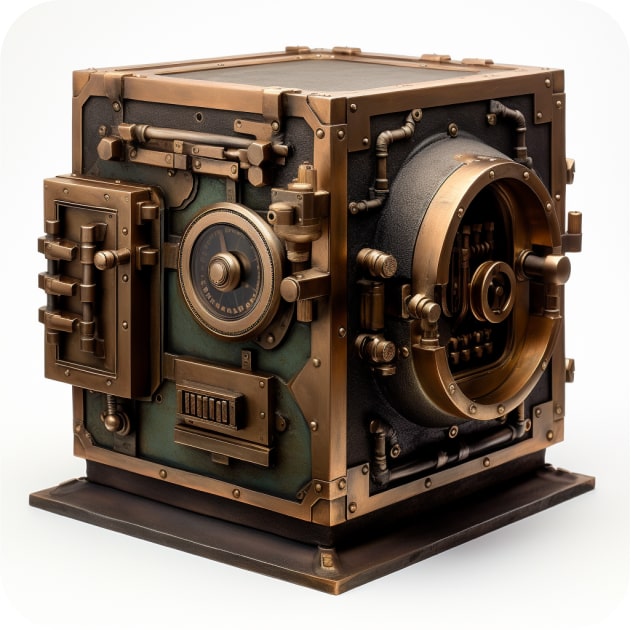
Cache
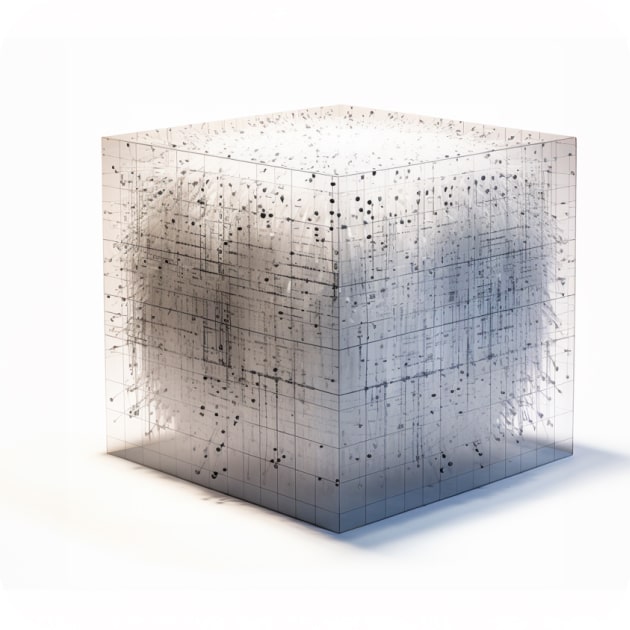
Categorize
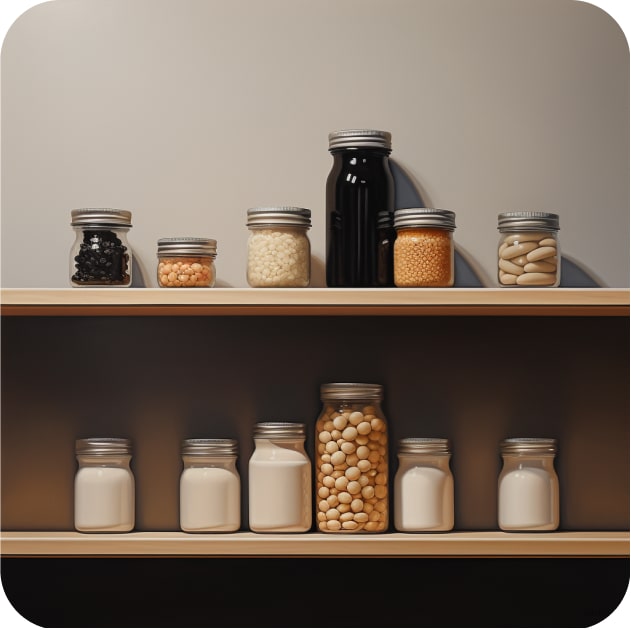
Checkpointing
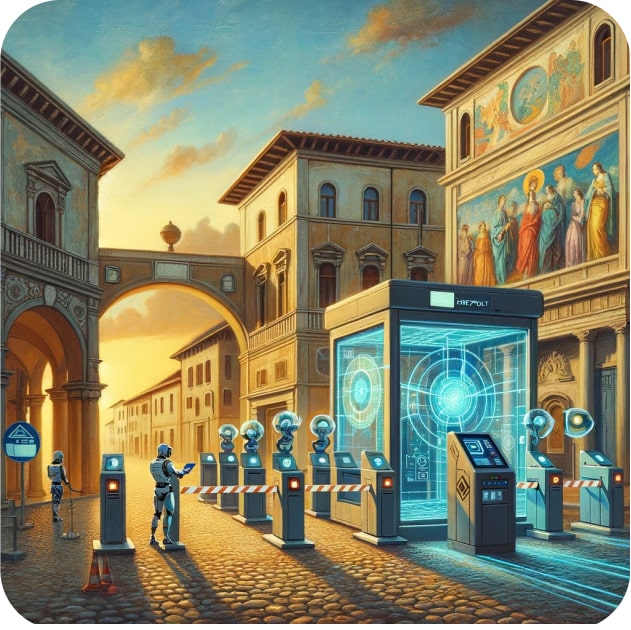
Deduplicate
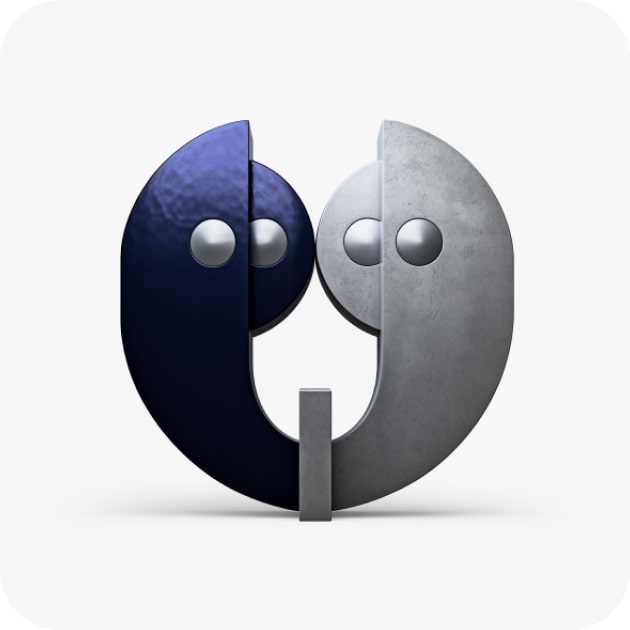
Deserialize
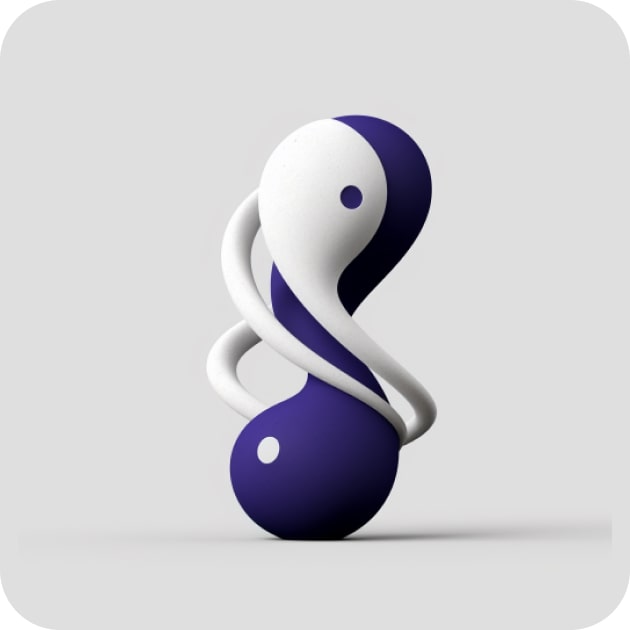
Dimensionality
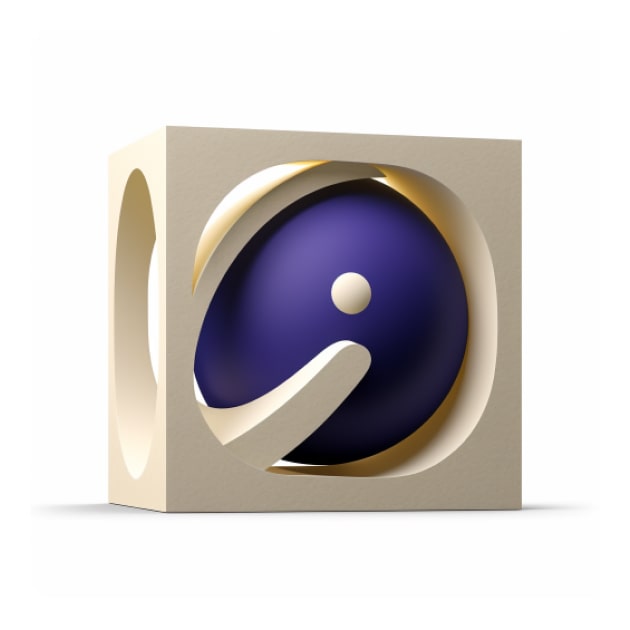
Encapsulate
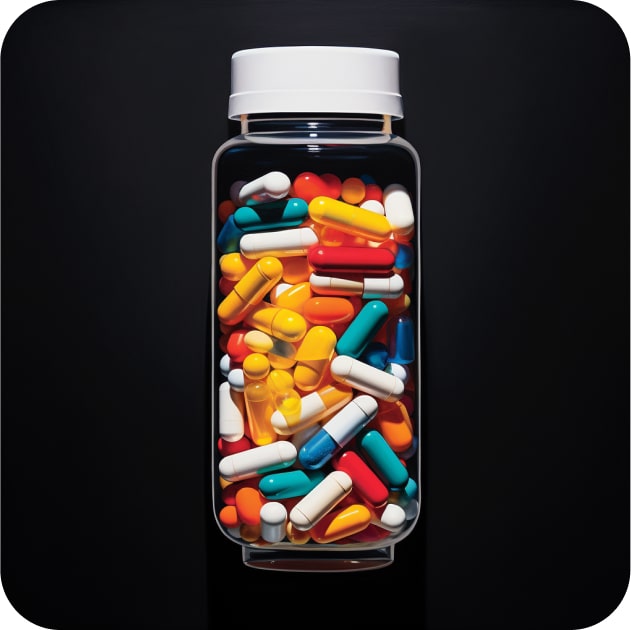
Enrich
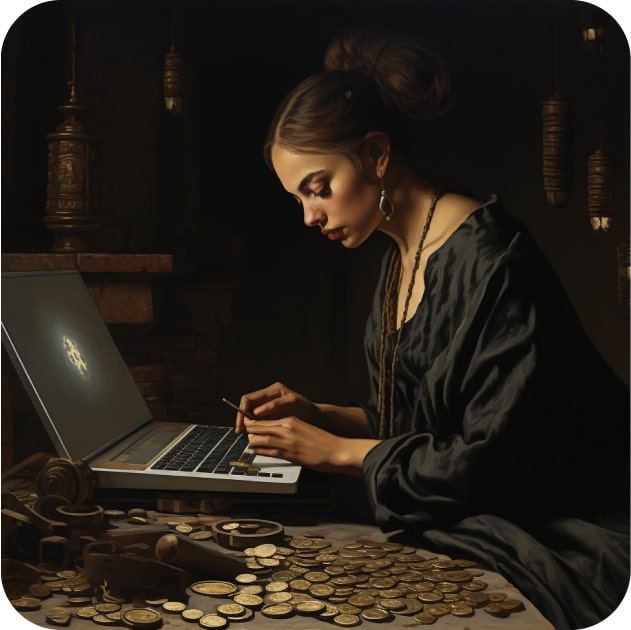
Export
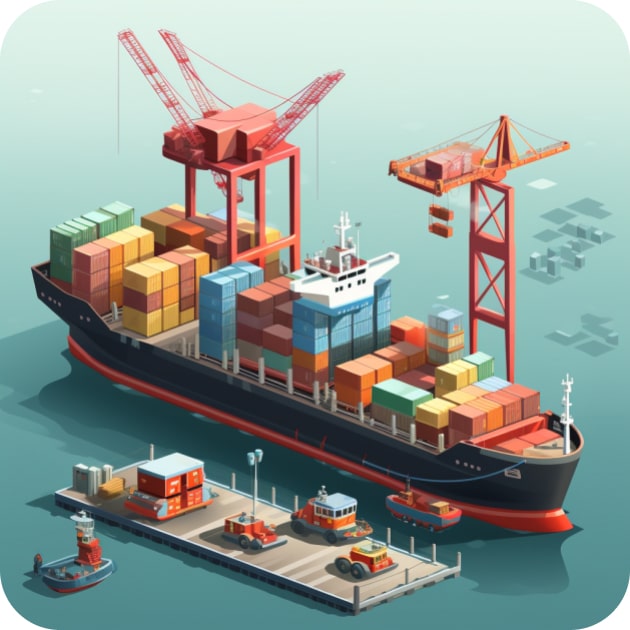
Graph Theory
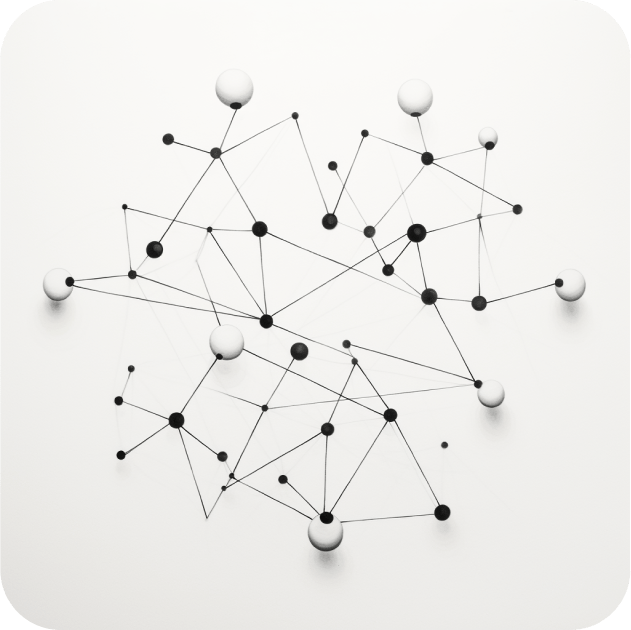
Idempotent
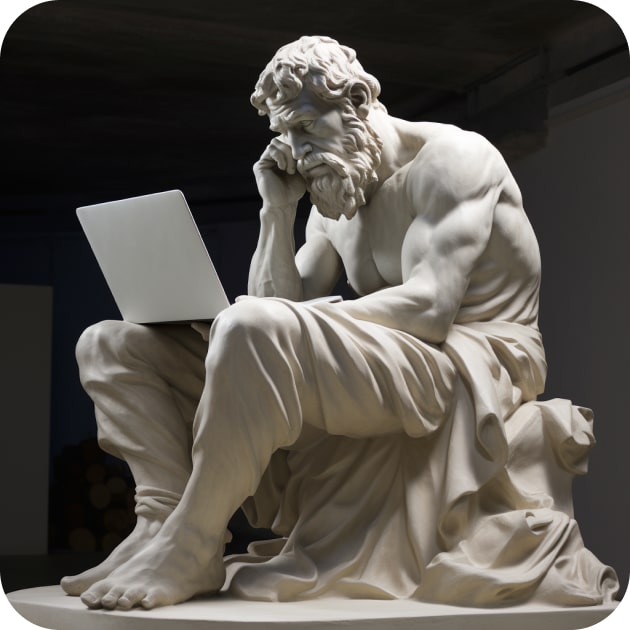
Index
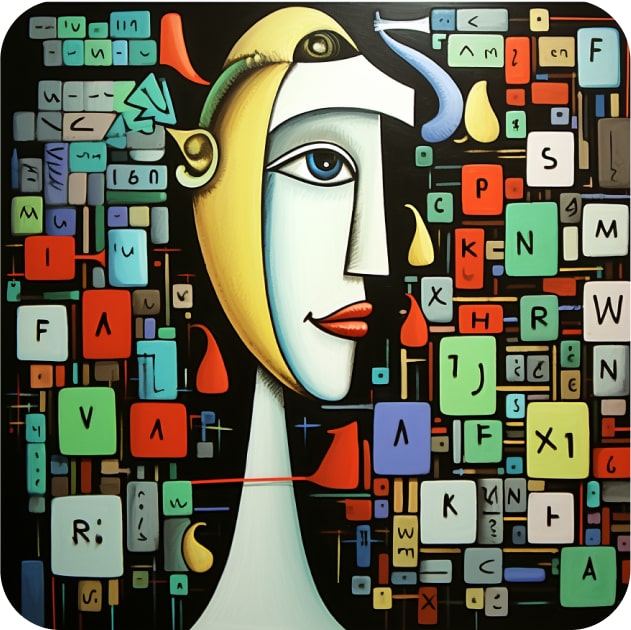
Integrate
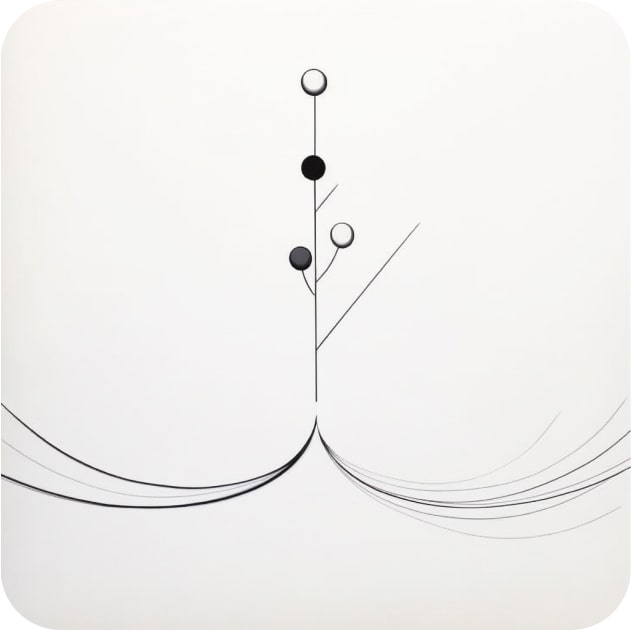
Lineage
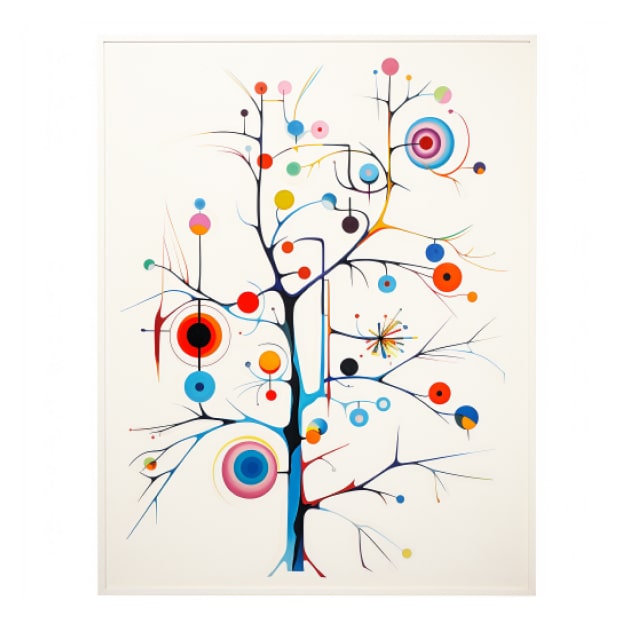
Linearizability
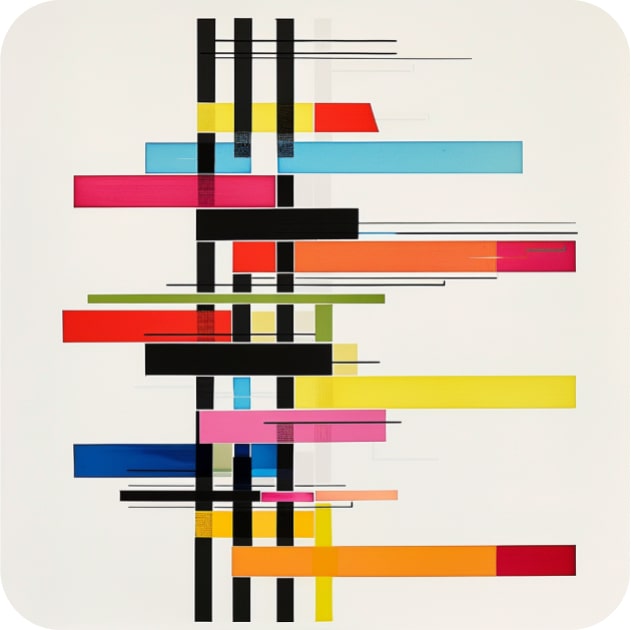
Materialize
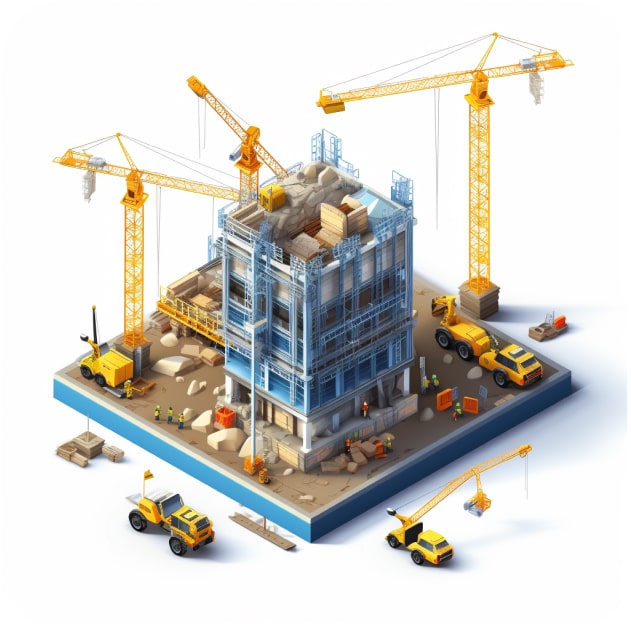
Memoize
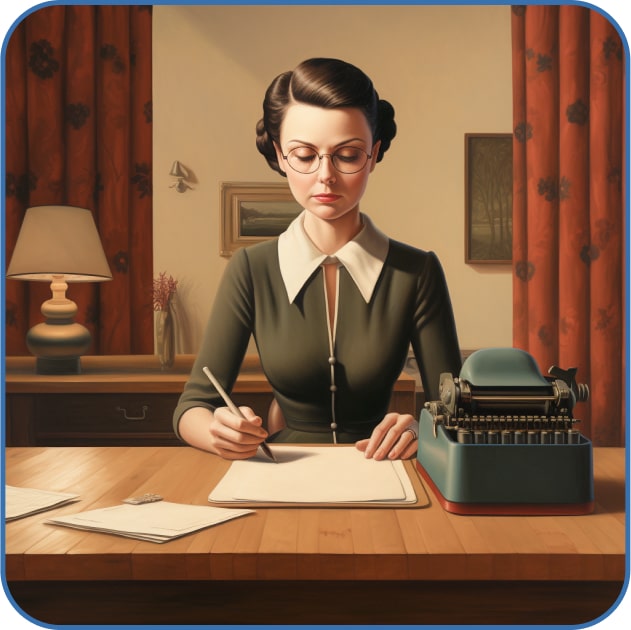
Merge
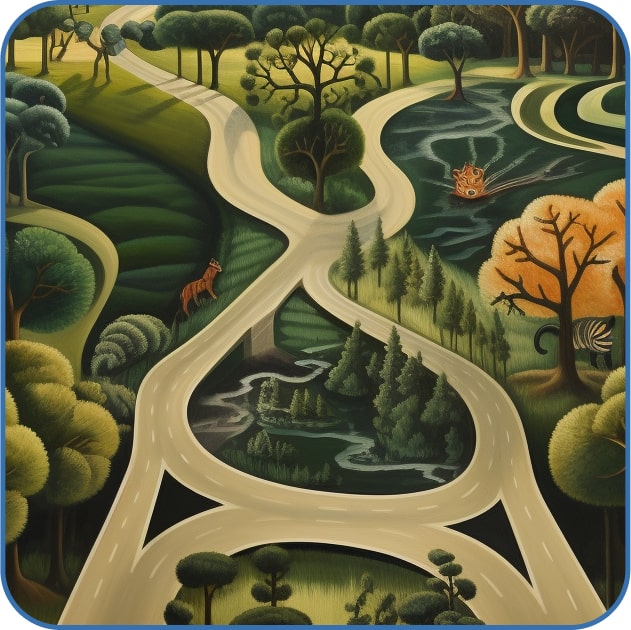
Model
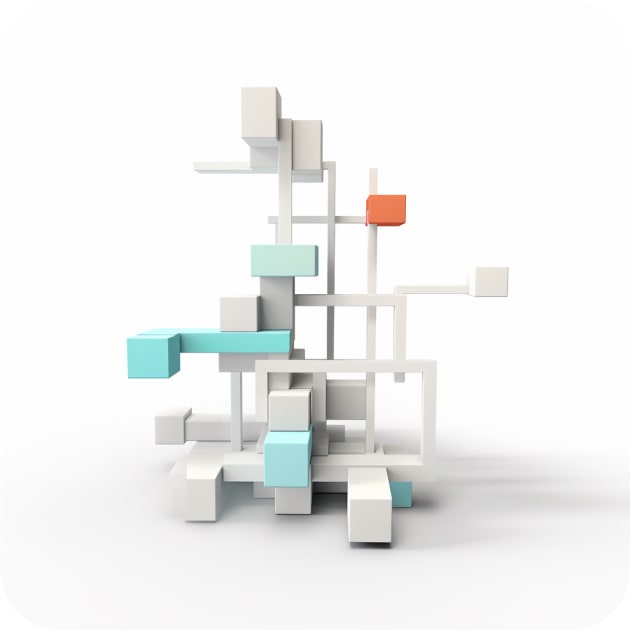
Monitor
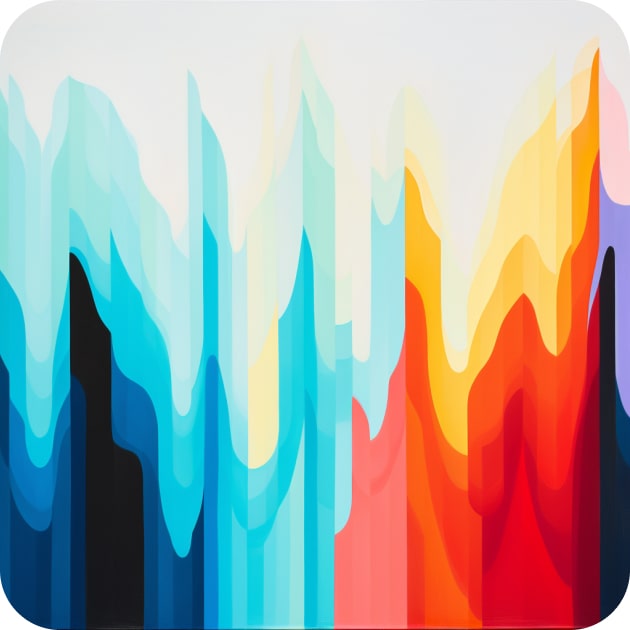
Named Entity Recognition
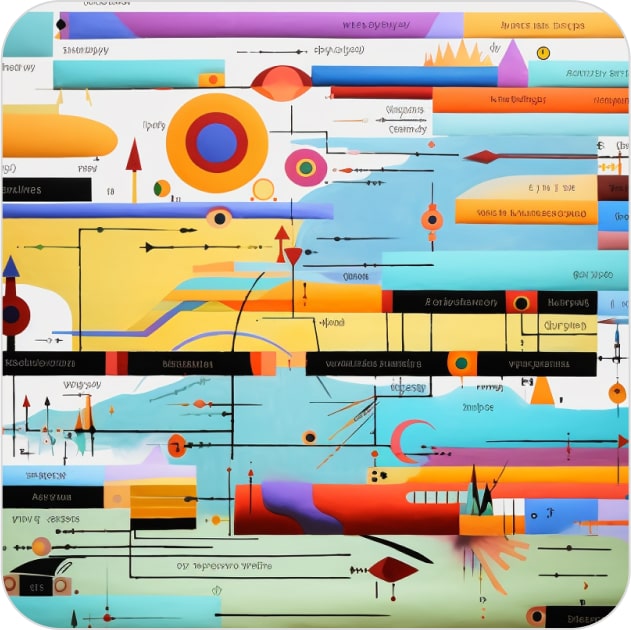
Parse
Partition
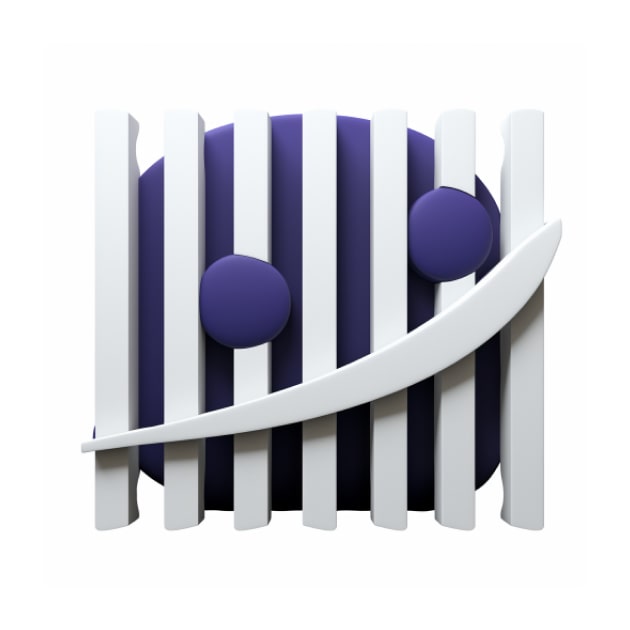
Prep
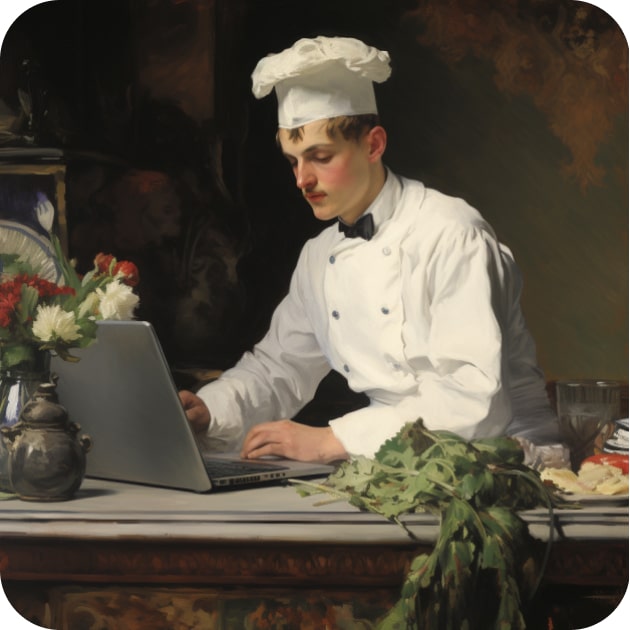
Preprocess
Primary Key
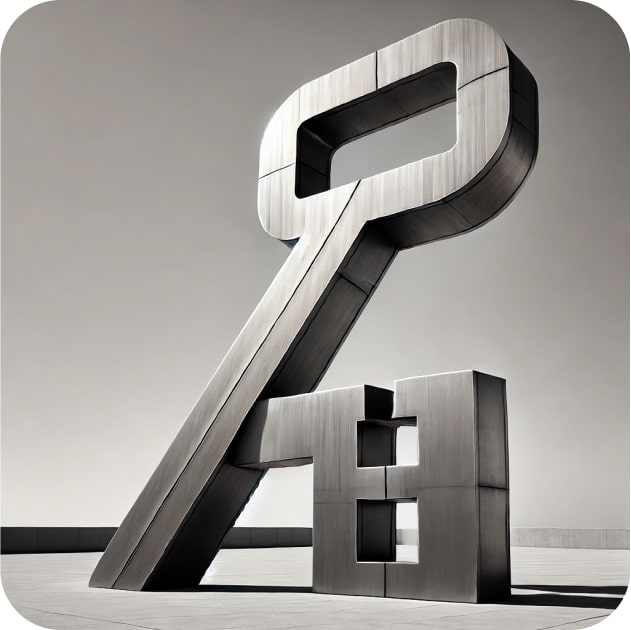
Replicate
Scaling
Schema Inference
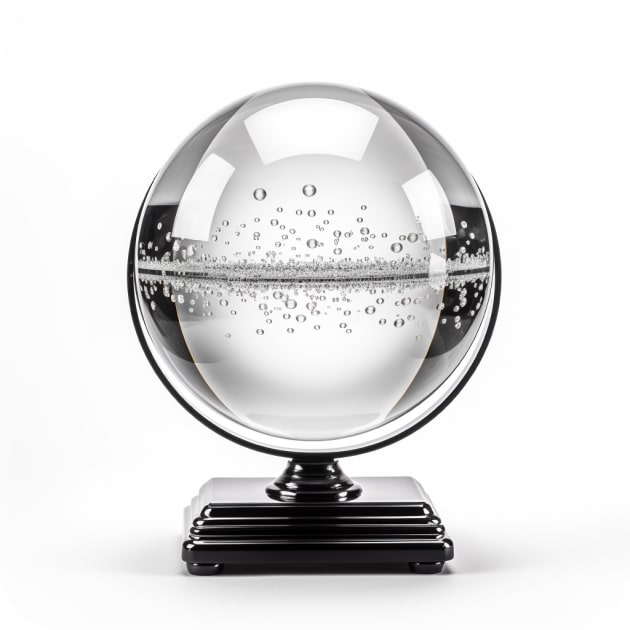
Schema Mapping
Secondary Index
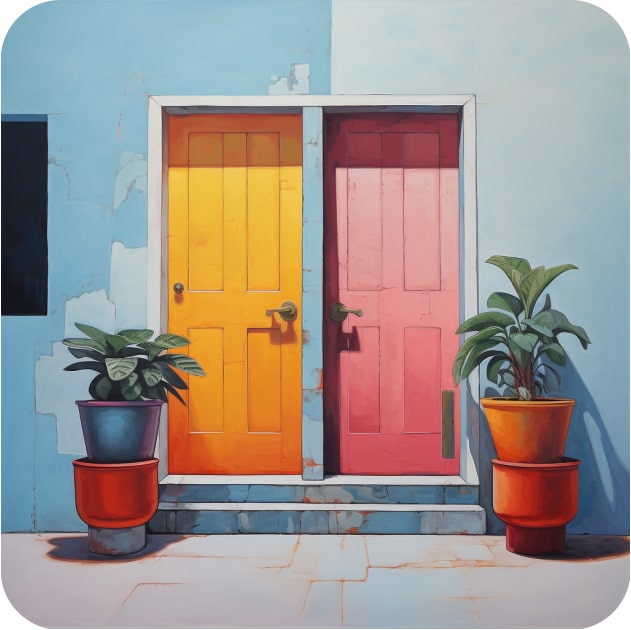
Software-defined Asset
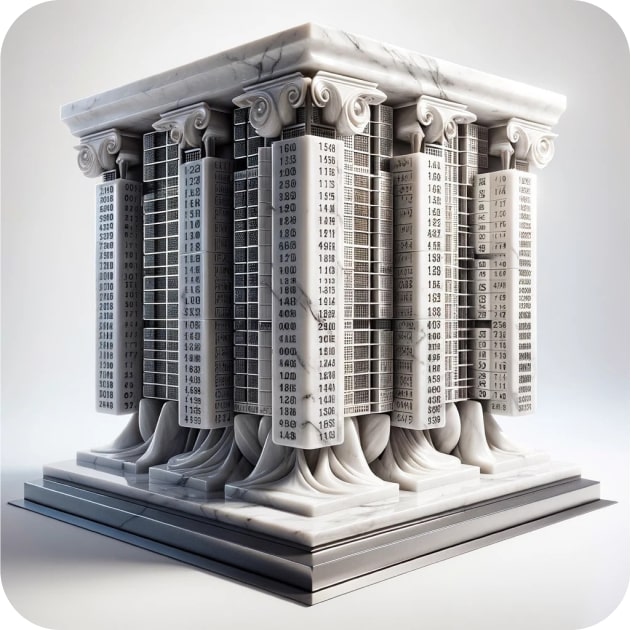
Synchronize
Validate
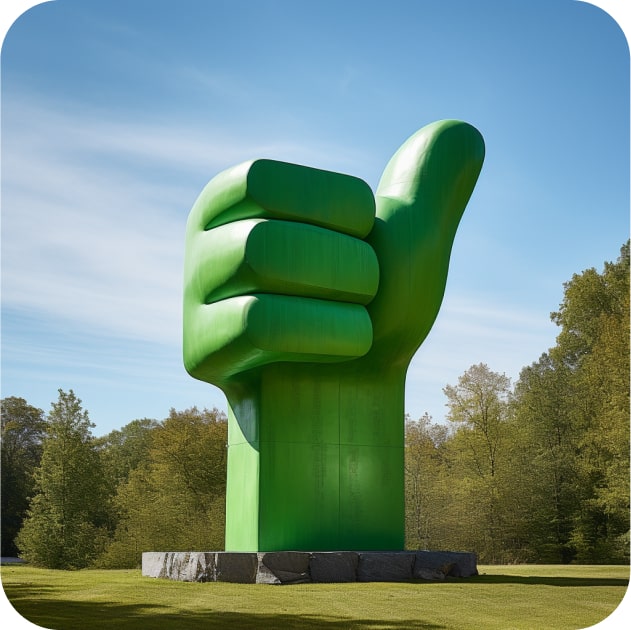
Version
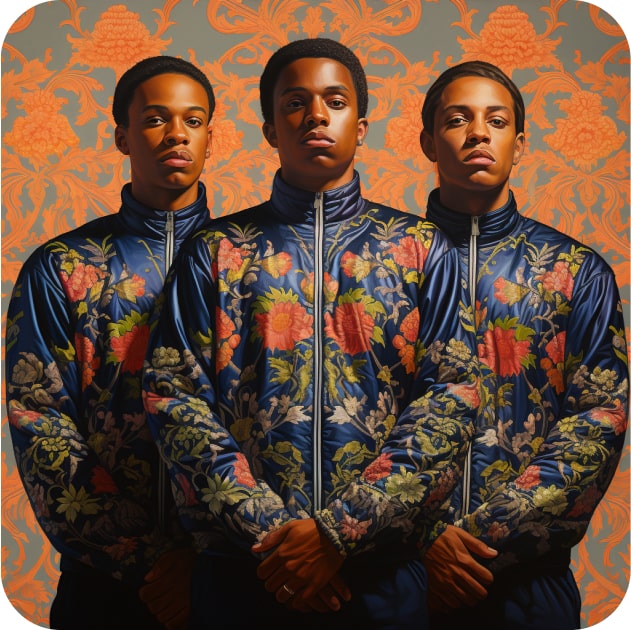