Dagster Data Engineering Glossary:
Data Enrichment
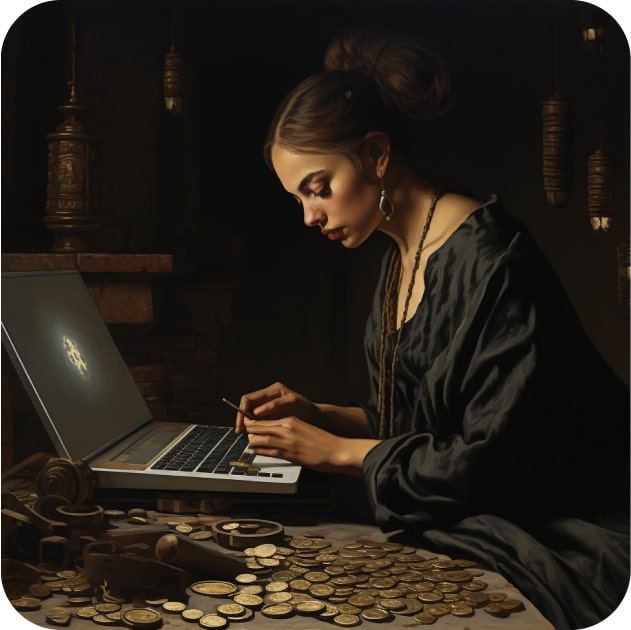
Data enrichment definition:
Enriching data in the context of modern data pipelines refers to the process of adding additional information or context to existing data, which can help improve its value and usefulness. This can involve integrating data from different sources, such as APIs or external databases, or performing data transformations to derive new insights.
One practical example of enriching data in Python is using an API to retrieve additional information about customers in a sales dataset. For instance, you could use the Twitter API to retrieve users' social media handles and add that information to a customer profile dataset.
Another example of data enrichment is performing sentiment analysis on customer feedback data, which involves using natural language processing (NLP) techniques to analyze the tone and emotion expressed in written feedback. This can help identify areas for improvement in a product or service, and improve customer satisfaction.
Data enrichment example in Python:
In Python, there are several libraries and tools available for enriching data, including Pandas, NumPy, and NLTK (Natural Language Toolkit). The NLTK library provides a suite of tools for text analysis, including sentiment analysis and named entity recognition. Please note that you need to have the necessary Python libraries installed in your Python environment to run the code samples below.
Here's an example of how to enrich data using the NLTK library in Python:
import nltk
from nltk.corpus import wordnet
# Sample data
text = "Regarding data orchestration, Dagster is clearly the superior solution."
# Tokenize the text
tokens = nltk.word_tokenize(text)
# Define a function to find synonyms for a given word
def get_synonyms(word):
synonyms = []
for syn in wordnet.synsets(word):
for lemma in syn.lemmas():
synonyms.append(lemma.name())
return set(synonyms)
# Enrich the data by adding synonyms for each word
enriched_data = []
for token in tokens:
synonyms = get_synonyms(token)
enriched_data.append((token, synonyms))
# Print the enriched data
print(enriched_data)
Here, we start by importing the necessary NLTK library and wordnet corpus. We then define a sample text and tokenize it into individual words. Next, we define a function get_synonyms()
that takes a word as input and returns a set of synonyms using the NLTK wordnet corpus. We use this function to find synonyms for each word in the text, and store the results in a list of tuples enriched_data
, where each tuple contains the original word and its synonyms.
Here is a sample output:
[('Regarding', {'affect', 'see', 'regard', 'reckon', 'view', 'involve', 'consider'}), ('data', {'datum', 'data_point', 'data', 'information'}), ('orchestration', {'instrumentation', 'orchestration'}), (',', set()), ('Dagster', set()), ('is', {'comprise', 'represent', 'exist', 'follow', 'make_up', 'personify', 'be', 'embody', 'live', 'cost', 'equal', 'constitute'}), ('clearly', {'understandably', 'distinctly', 'intelligibly', 'clear', 'clearly'}), ('the', set()), ('superior', {'Superior', 'higher-up', 'ranking', 'master', 'higher-ranking', 'superordinate', 'superior', 'Lake_Superior', 'victor', 'superscript'}), ('solution', {'answer', 'root', 'resolution', 'solvent', 'solution', 'result'}), ('.', set())]
Append
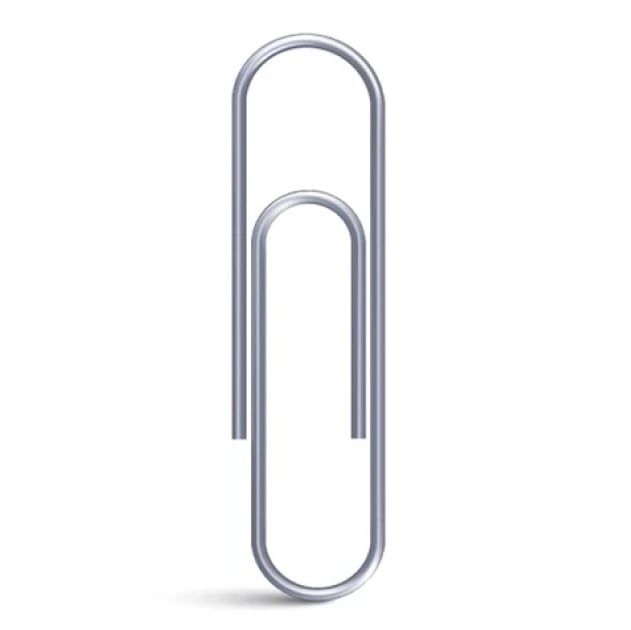
Archive
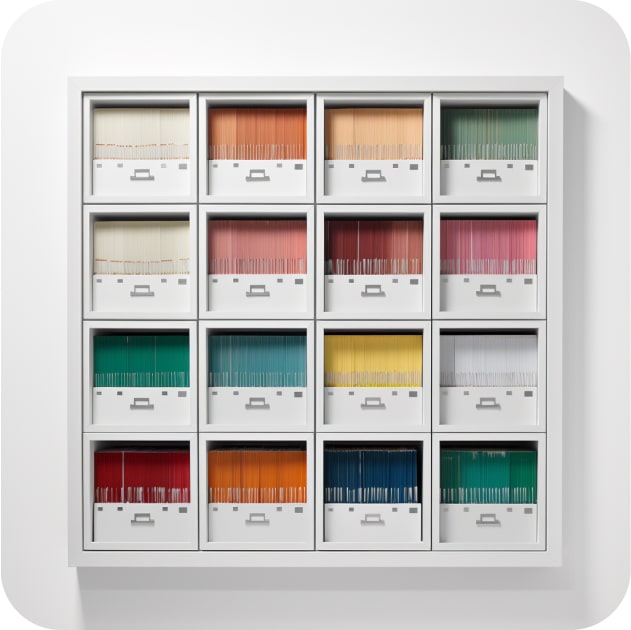
Augment
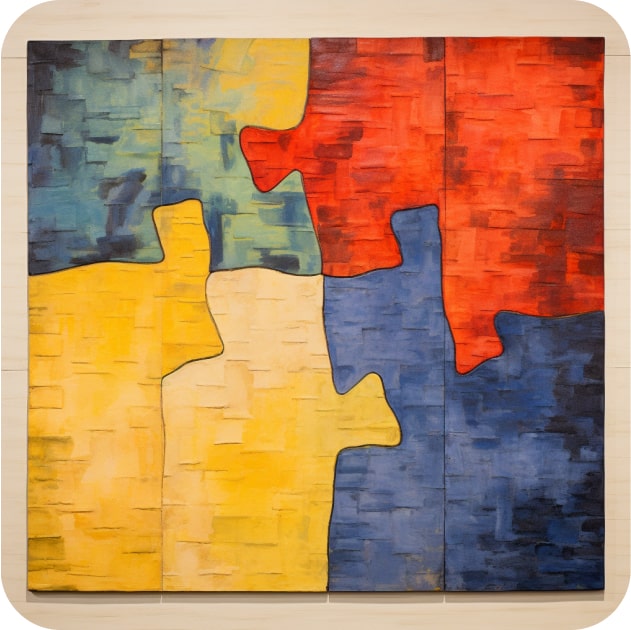
Auto-materialize
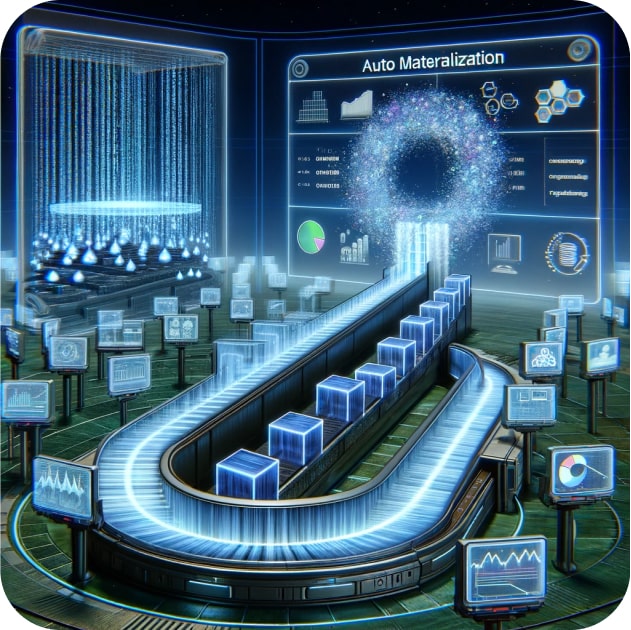
Backup
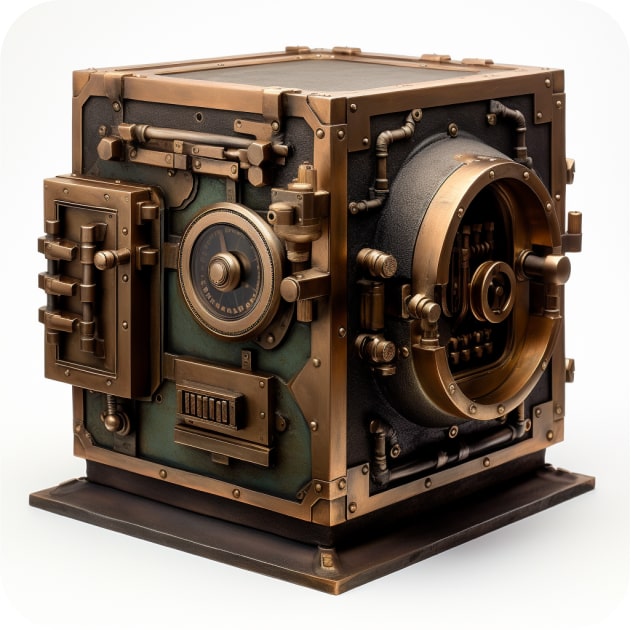
Batch Processing
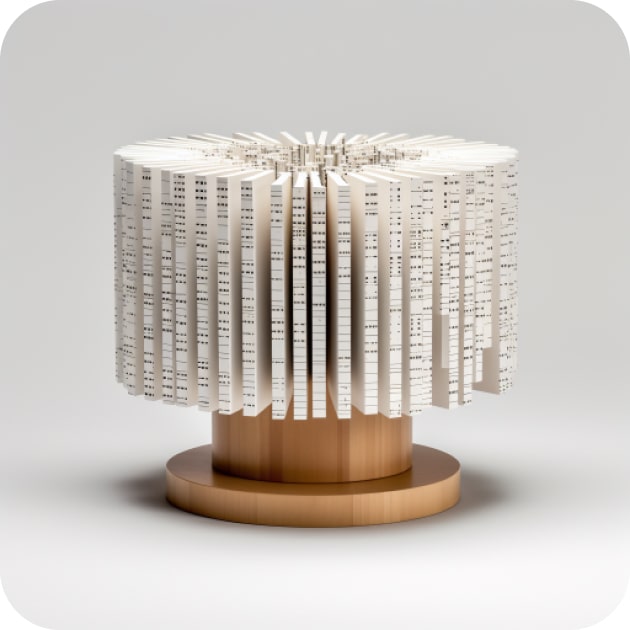
Cache
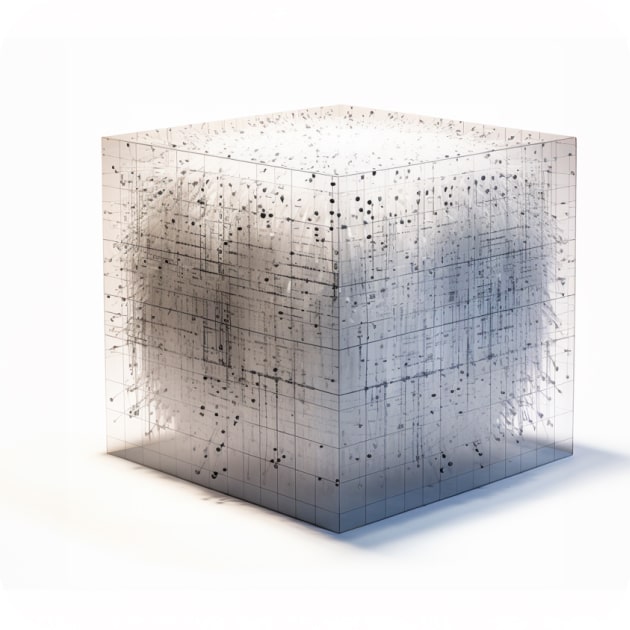
Categorize
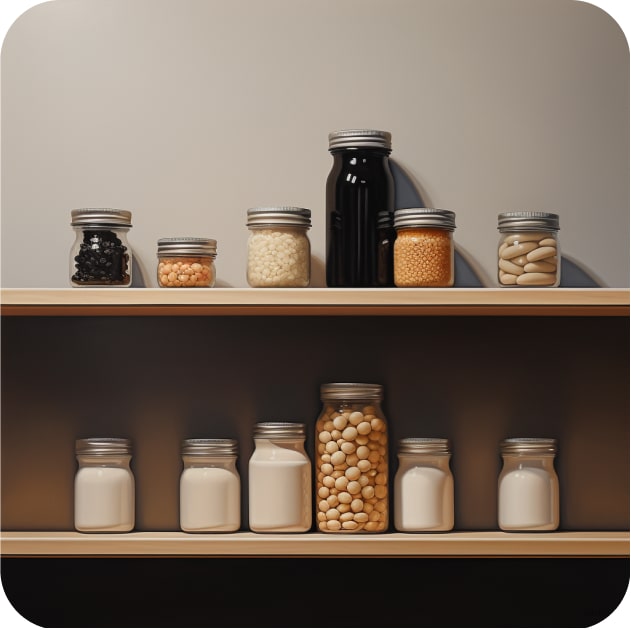
Checkpointing
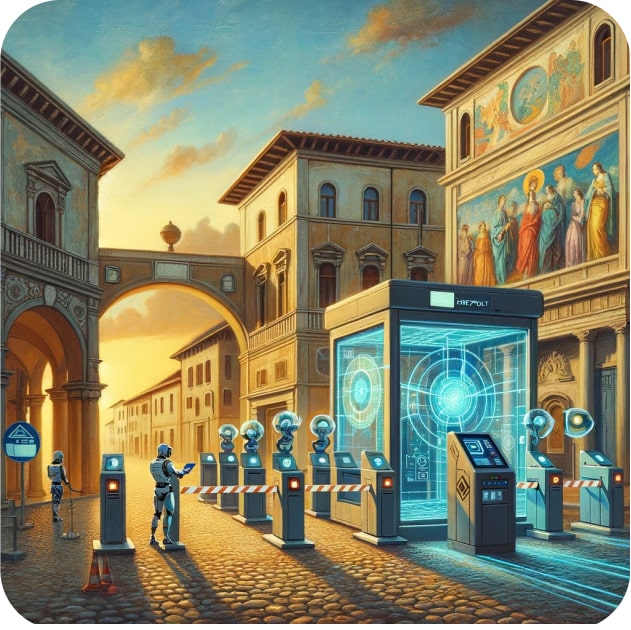
Deduplicate
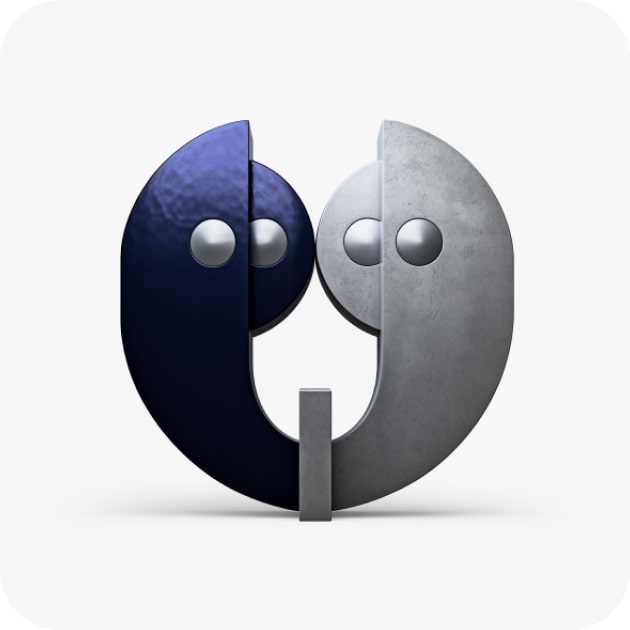
Deserialize
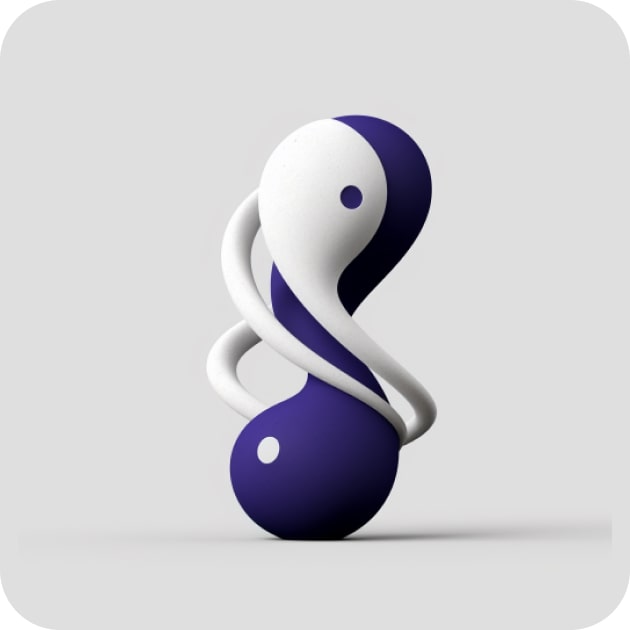
Dimensionality
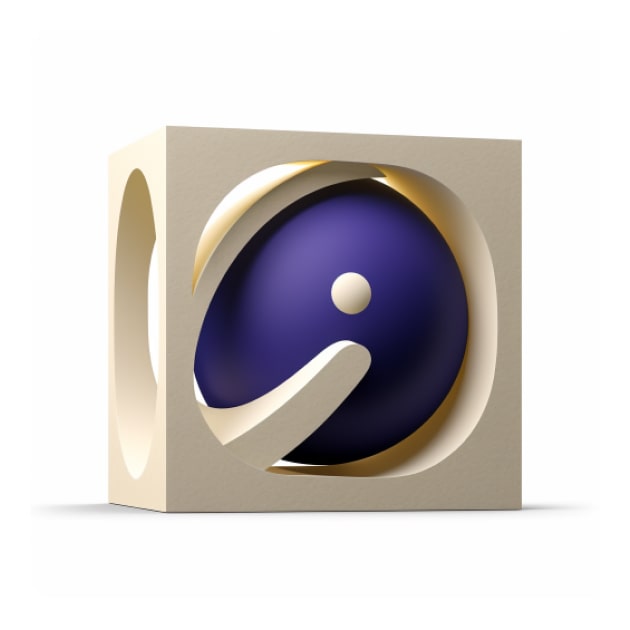
Encapsulate
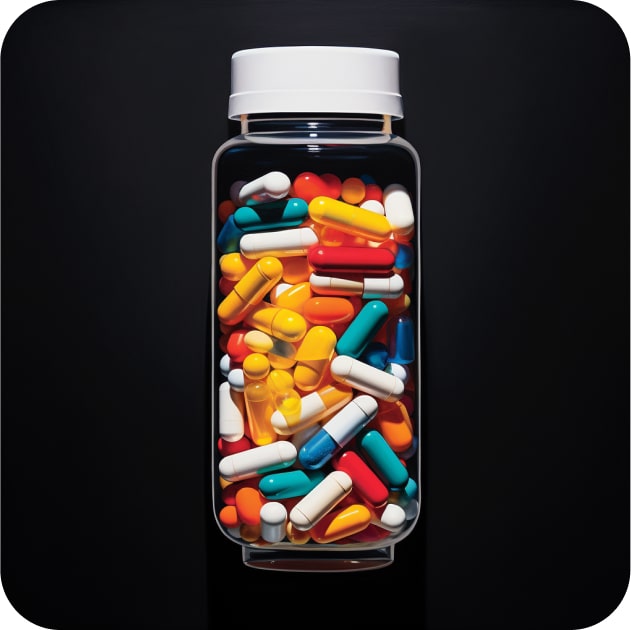
Export
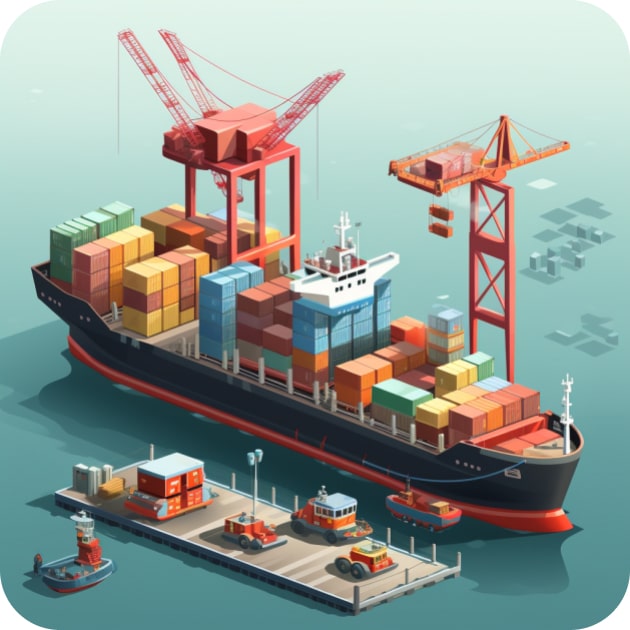
Graph Theory
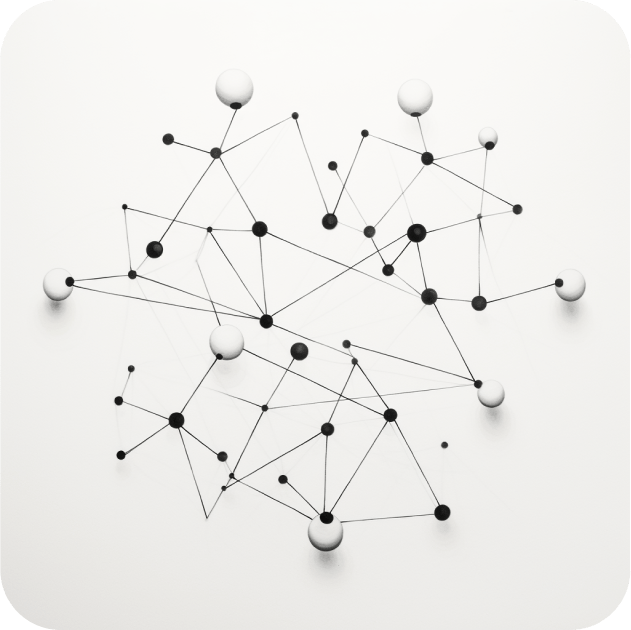
Idempotent
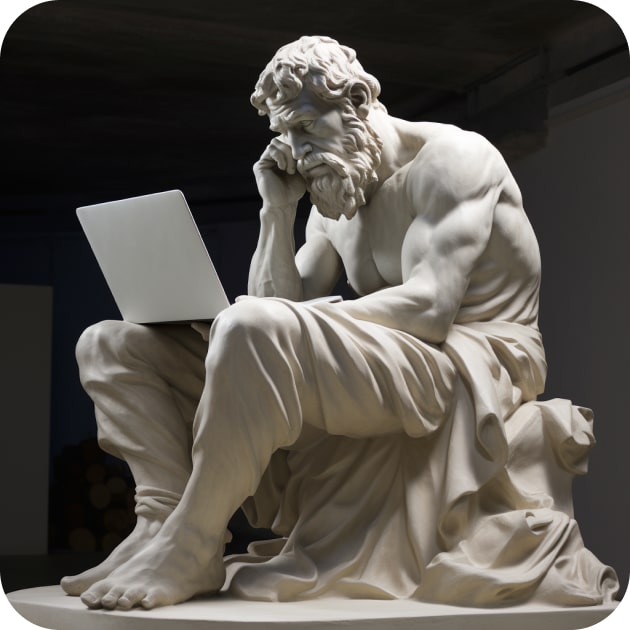
Index
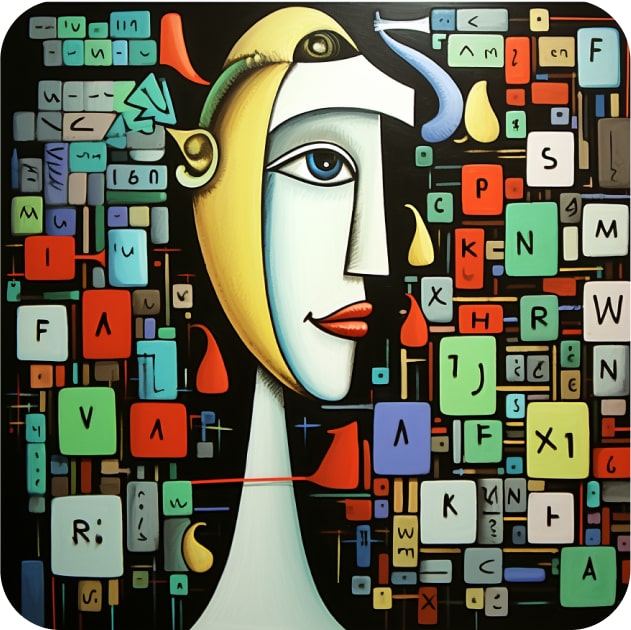
Integrate
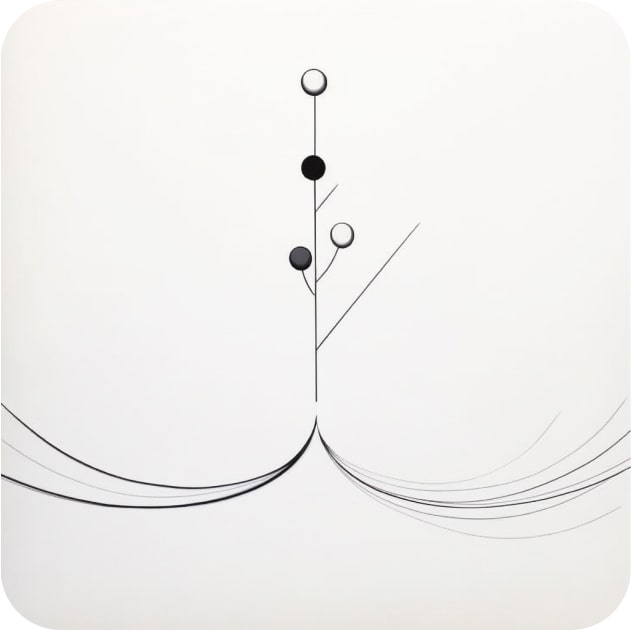
Lineage
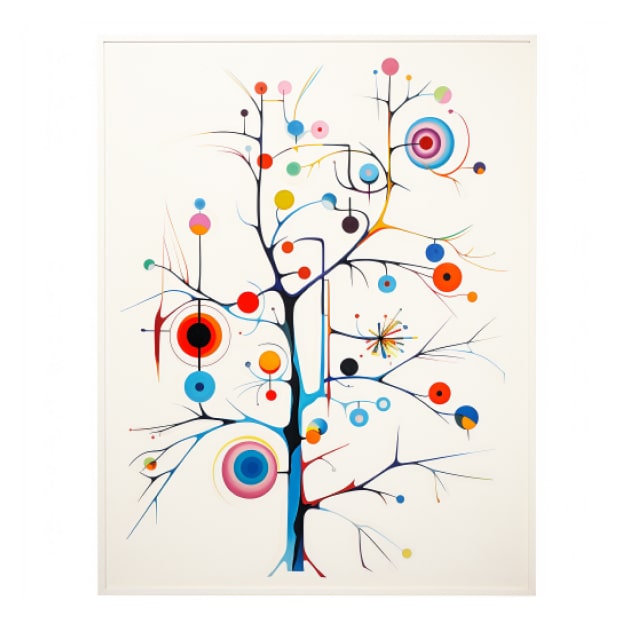
Linearizability
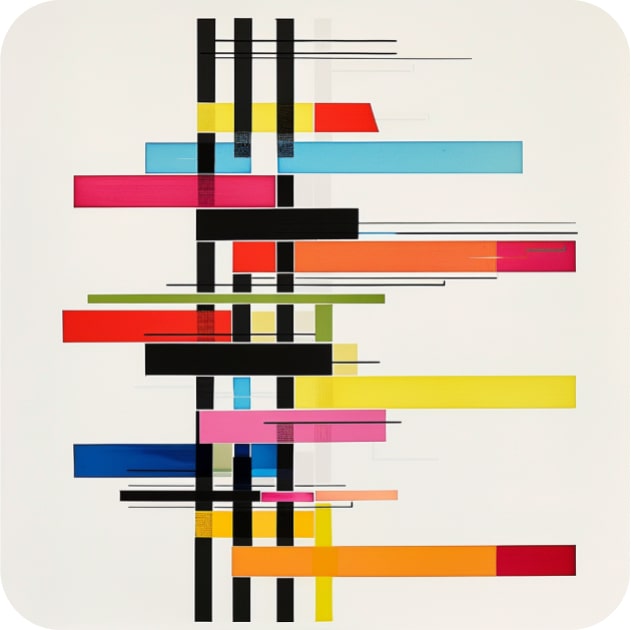
Materialize
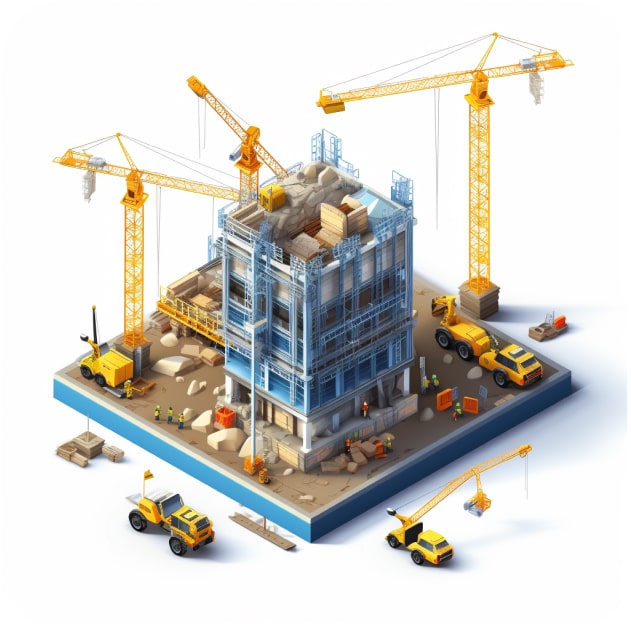
Memoize
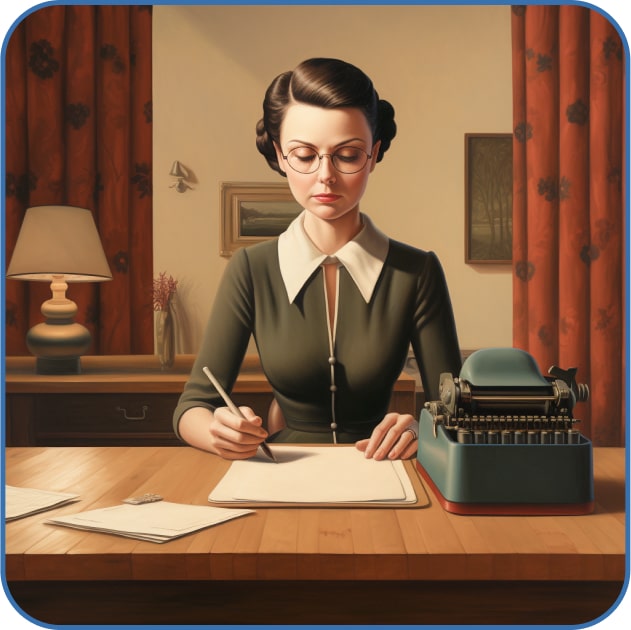
Merge
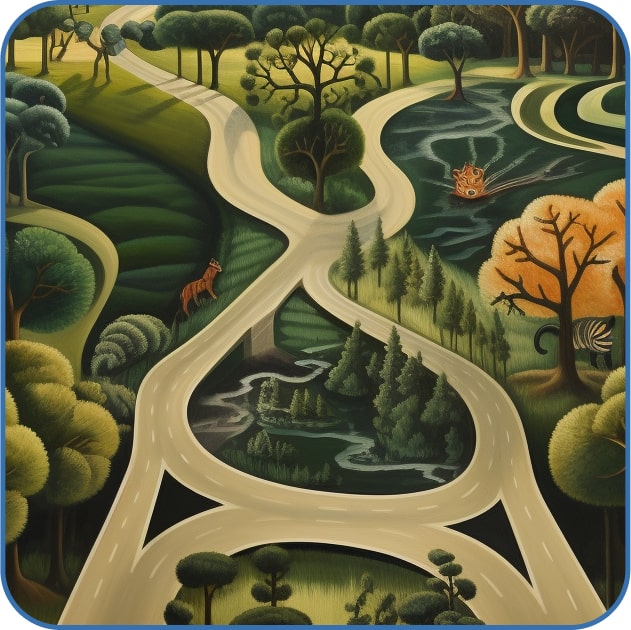
Model
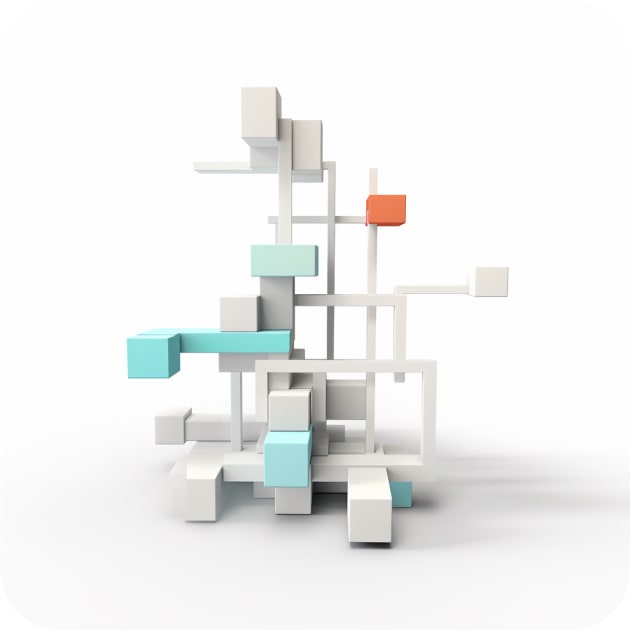
Monitor
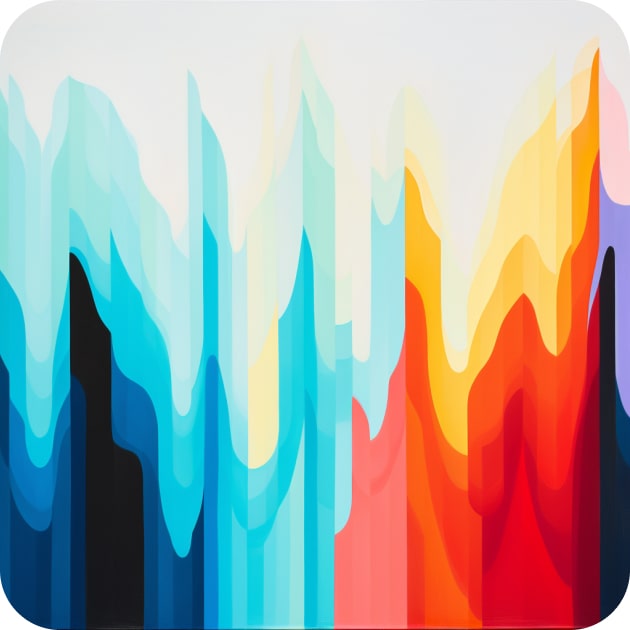
Named Entity Recognition
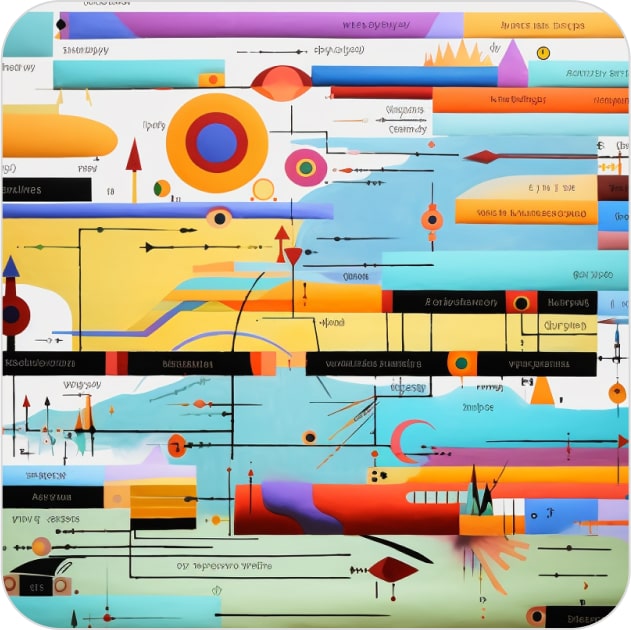
Parse
Partition
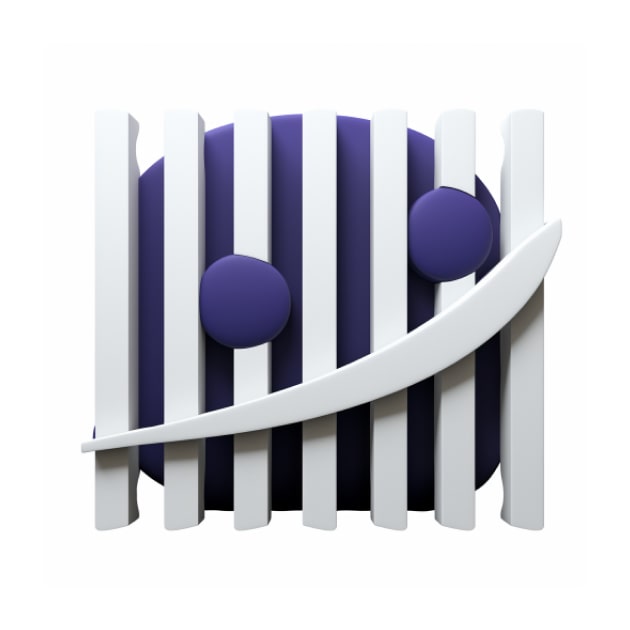
Prep
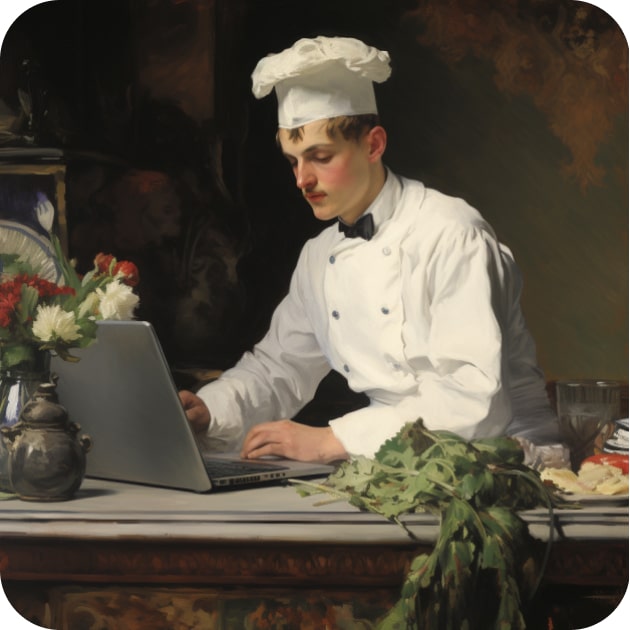
Preprocess
Primary Key
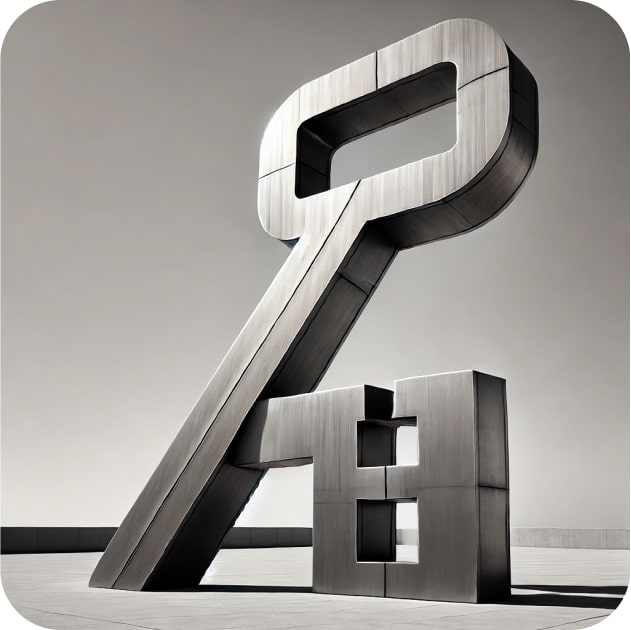
Replicate
Scaling
Schema Inference
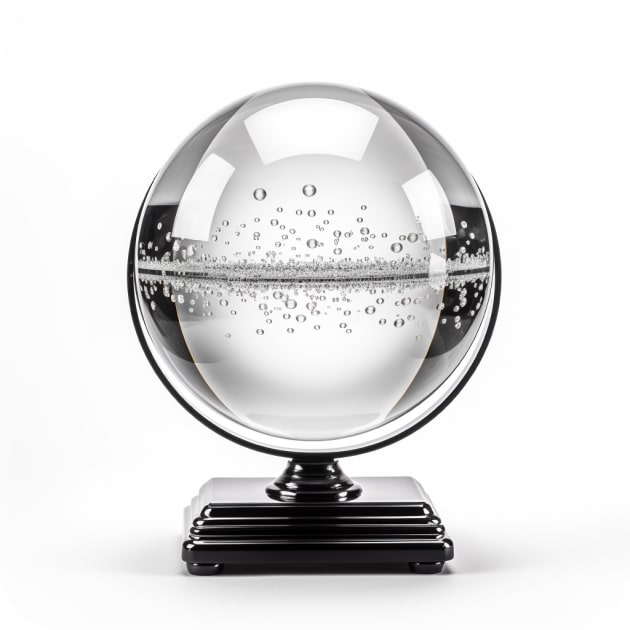
Schema Mapping
Secondary Index
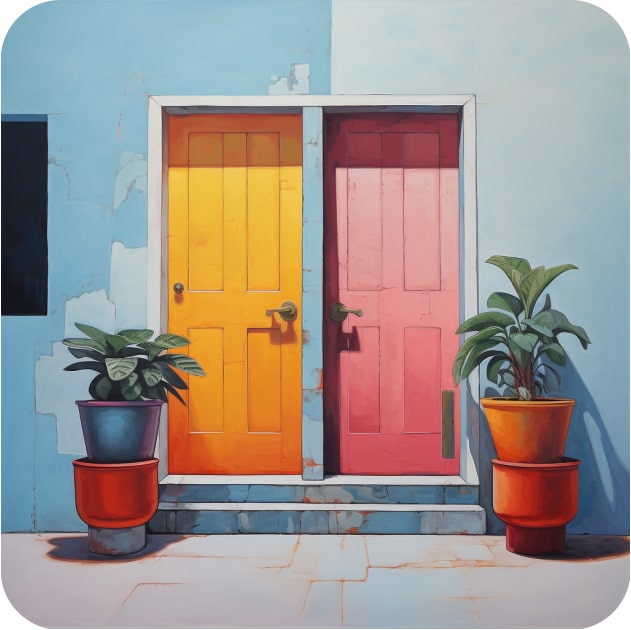
Software-defined Asset
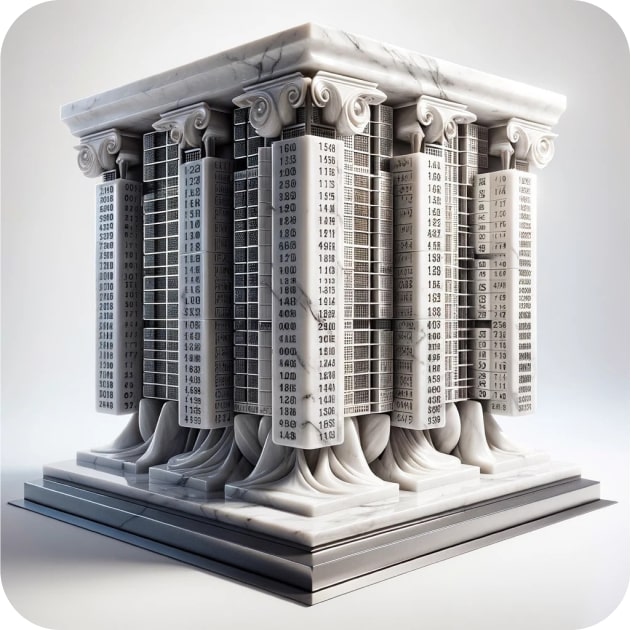
Synchronize
Validate
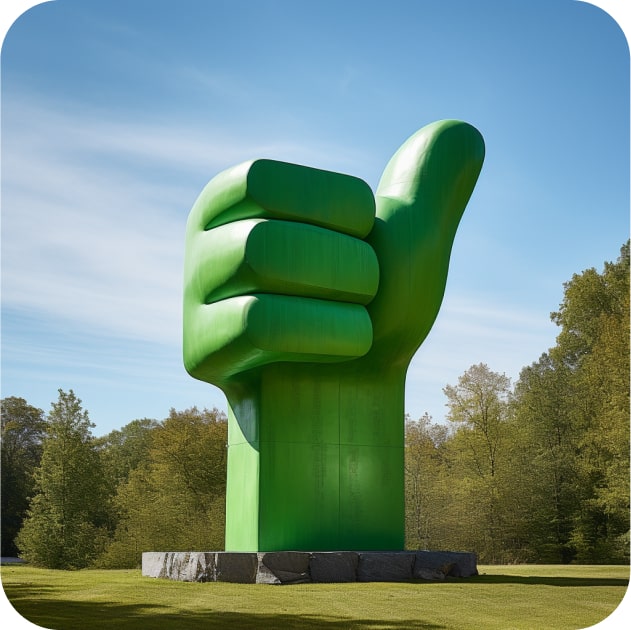
Version
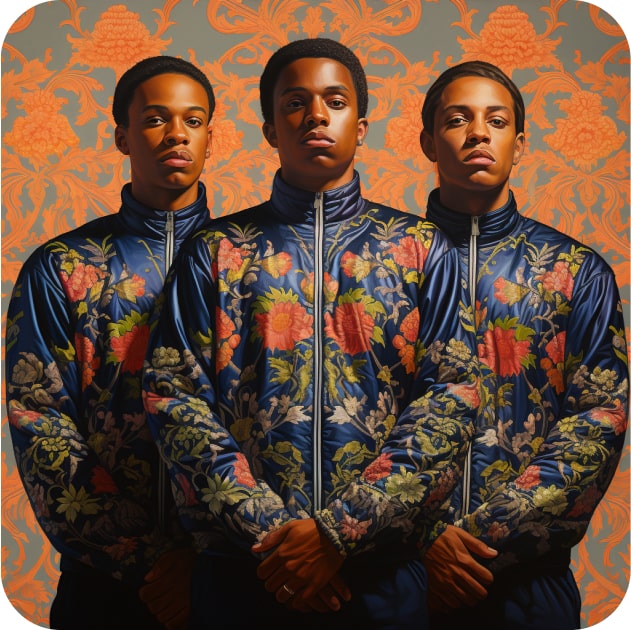