Data parsing definition:
Data parsing is the process of interpreting and converting data from one format to another. It involves taking data, typically structured in a specific manner such as CSV, JSON, XML, etc., and transforming it into a format that is more useful or easier to process and analyze. This process usually involves a set of rules or patterns to understand the input data and to determine how the transformation should occur. Data parsing is a common task in areas like data preprocessing, web scraping, and data integration.
Data parsing example using Python:
Let's look at an example of parsing CSV (Comma-Separated Values) data, which is a common task in data analysis. Please note that you need to have the 'csv' library installed in your Python environment to run this code.
Assume we have a CSV file named data.csv
with the following content:
Name,Age,Occupation
Alice,30,Engineer
Bob,25,Doctor
Charlie,35,Teacher
Here is a Python script that parses this file using the csv
module. Please ensure that the 'data.csv' file is in the same directory as your Python script, or provide an absolute path to the file.
import csv
def parse_csv_file(file_name):
with open(file_name, 'r') as file:
reader = csv.reader(file)
header = next(reader) # This will store the header row (['Name', 'Age', 'Occupation'])
rows = []
for row in reader:
# Convert each row to a dictionary with keys as the header and values as the row values
rows.append(dict(zip(header, row)))
return rows
data = parse_csv_file('data.csv')
for person in data:
print(f"Name: {person['Name']}, Age: {person['Age']}, Occupation: {person['Occupation']}")
This script first opens the file and creates a CSV reader. It then stores the header row (which contains the field names) and iterates over the rest of the rows, converting each one to a dictionary where the keys are the field names and the values are the corresponding values from the row. This way, you get a list of dictionaries where each dictionary represents a person.
Run the script, and your parsed data will show as follows:
Name: Alice, Age: 30, Occupation: Engineer
Name: Bob, Age: 25, Occupation: Doctor
Name: Charlie, Age: 35, Occupation: Teacher
Append
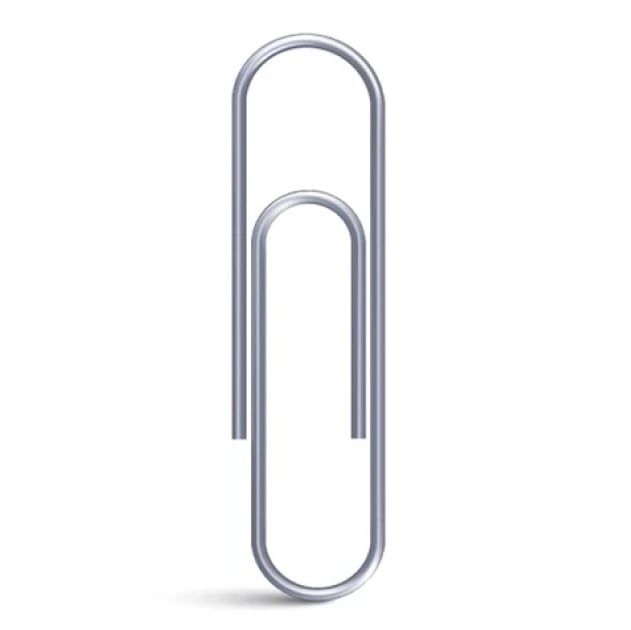
Archive
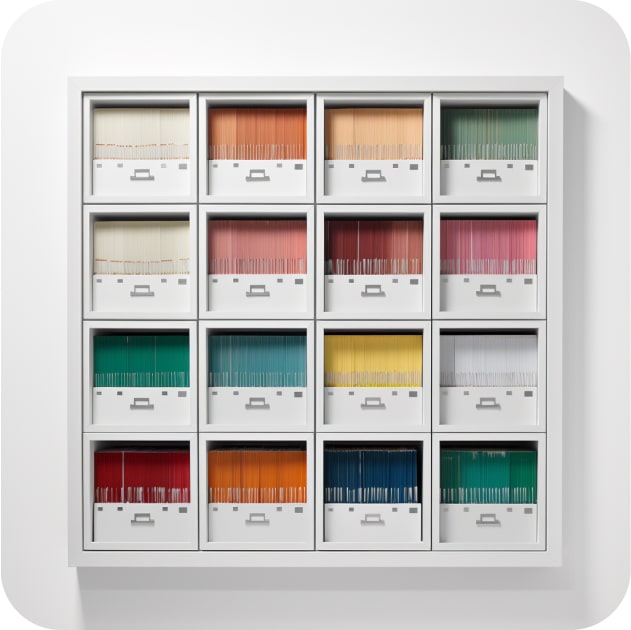
Augment
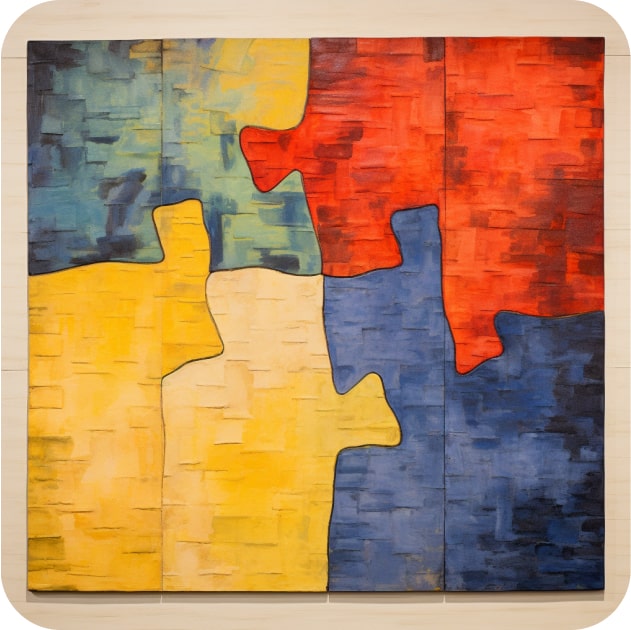
Auto-materialize
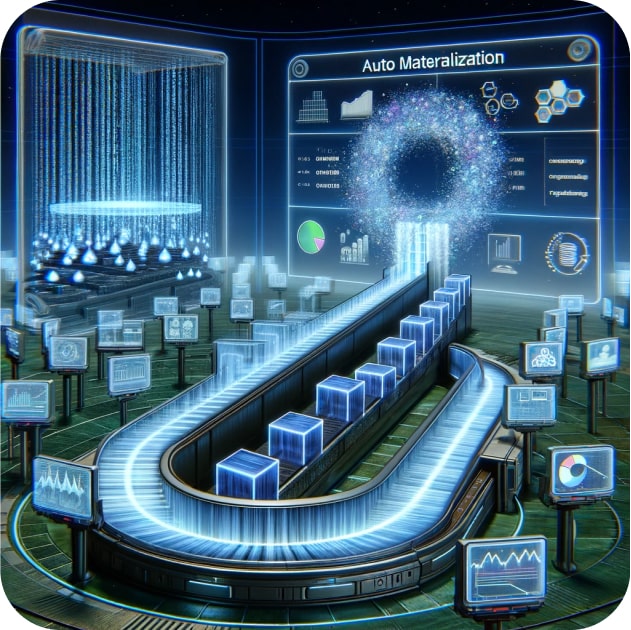
Backup
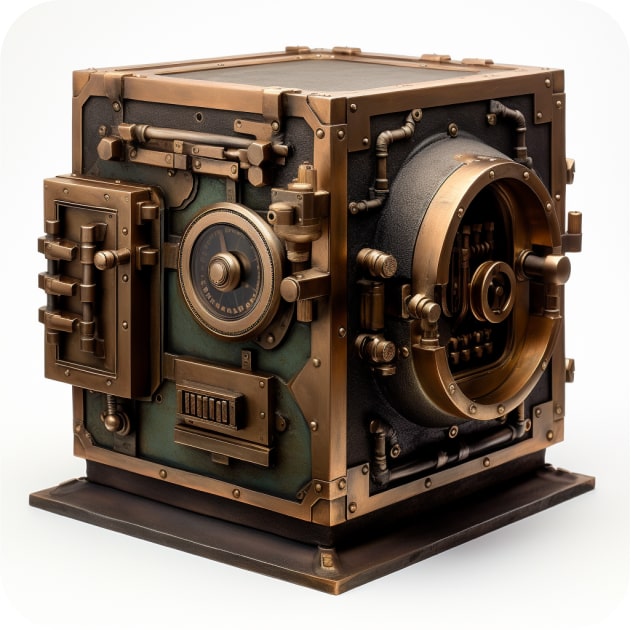
Batch Processing
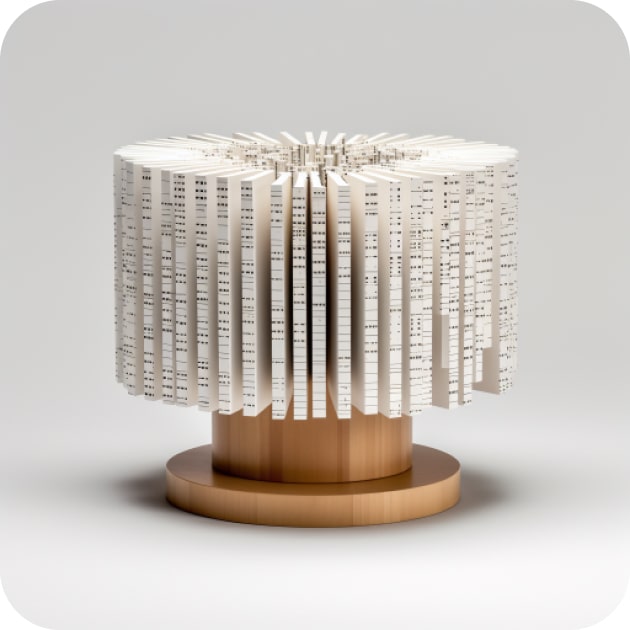
Cache
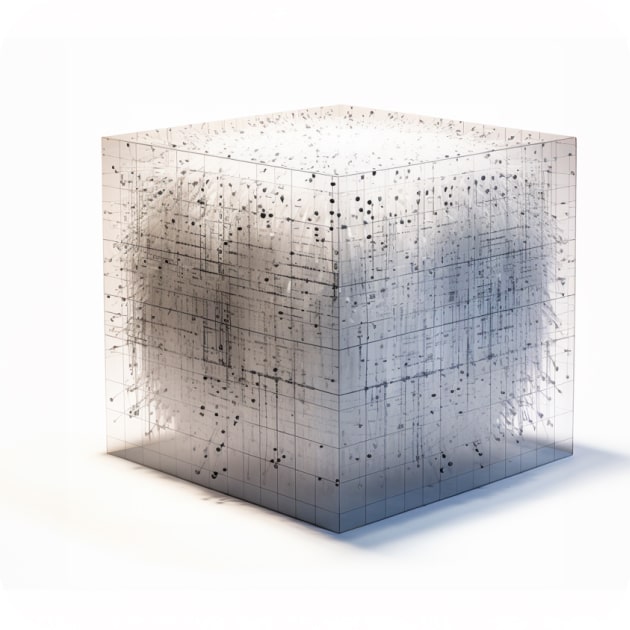
Categorize
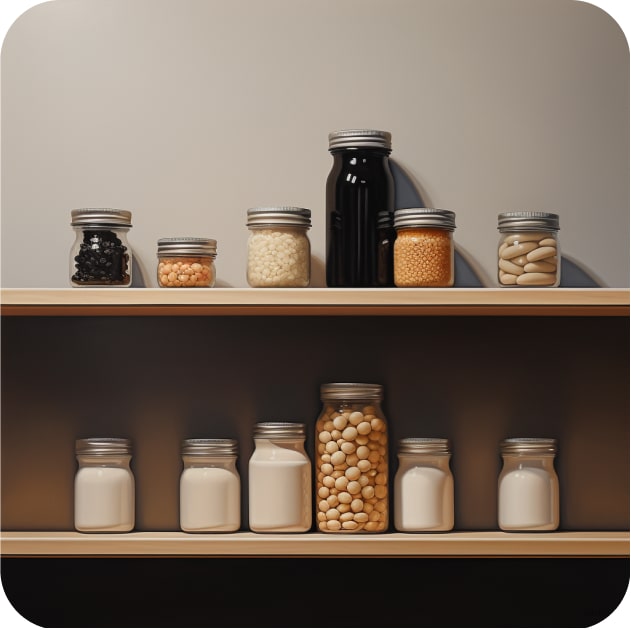
Checkpointing
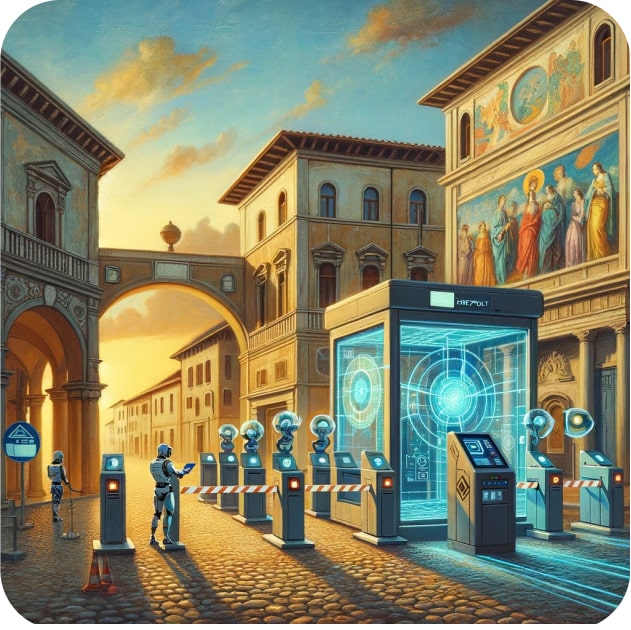
Deduplicate
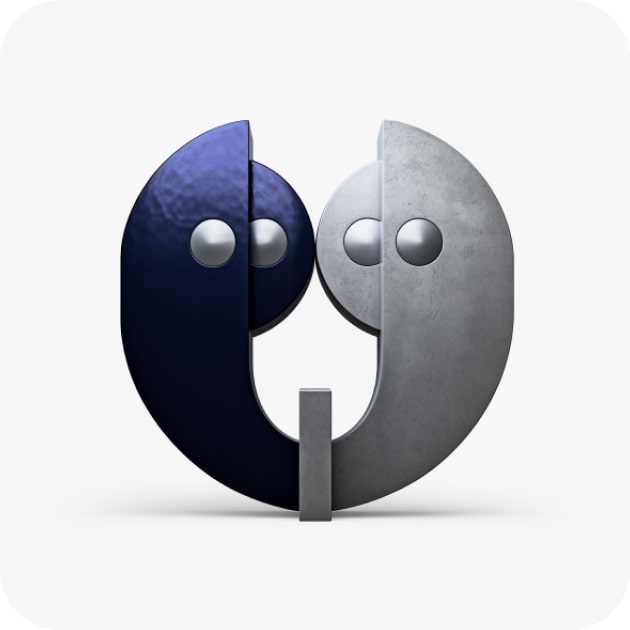
Deserialize
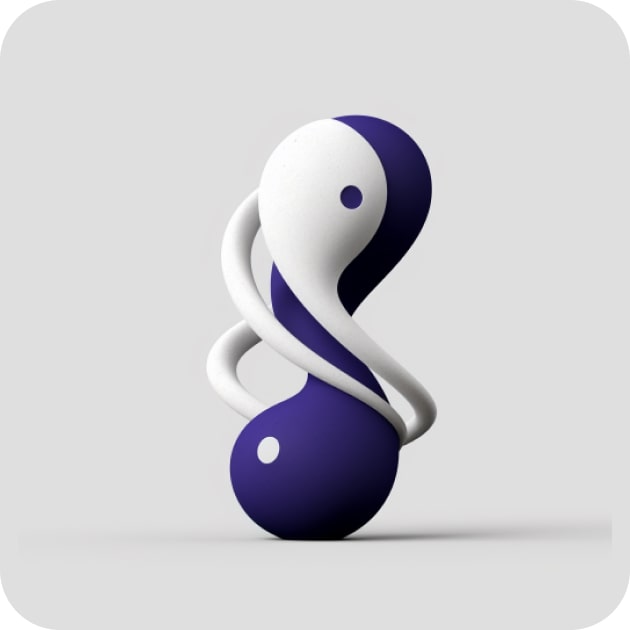
Dimensionality
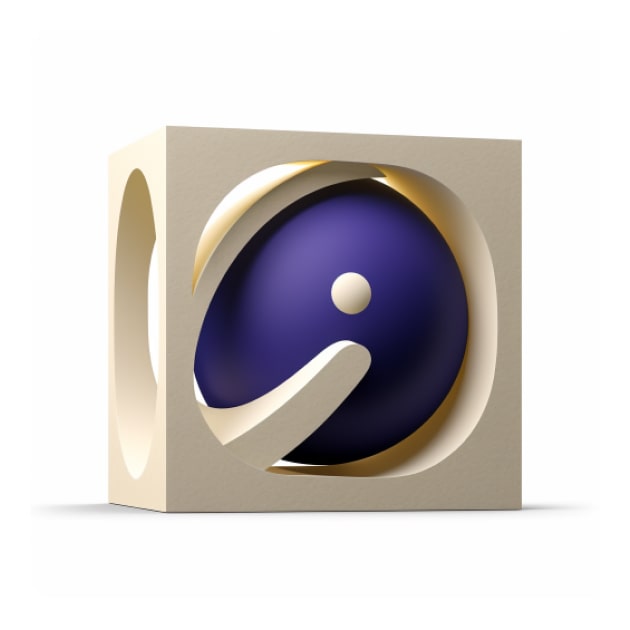
Encapsulate
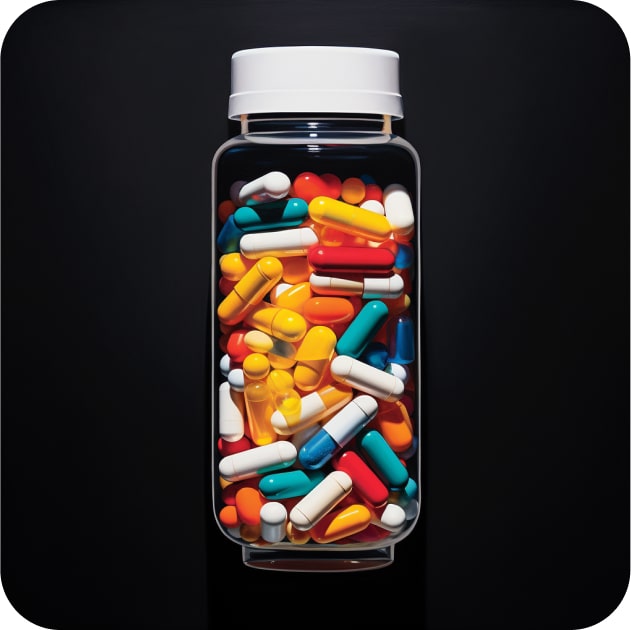
Enrich
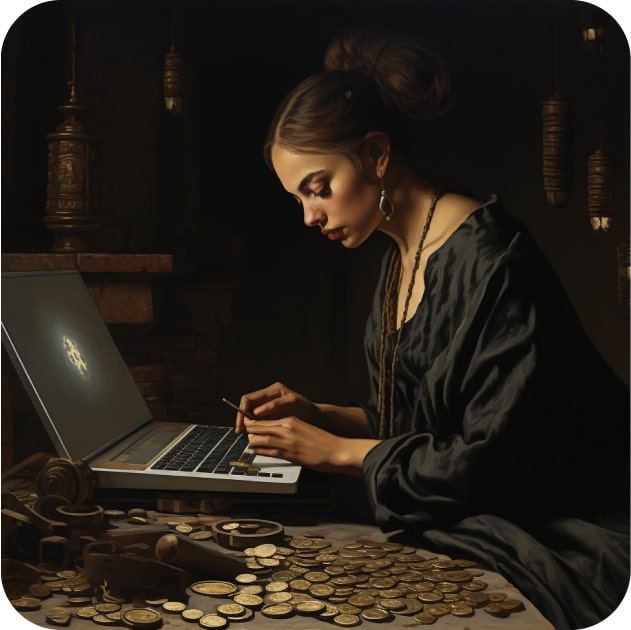
Export
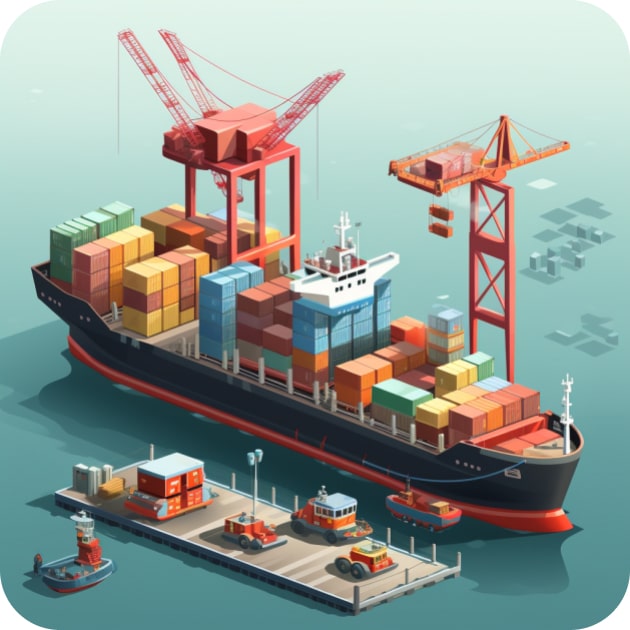
Graph Theory
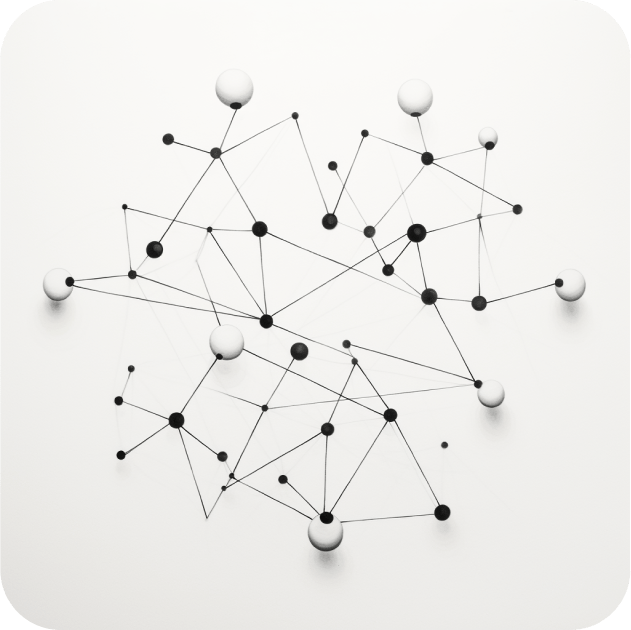
Idempotent
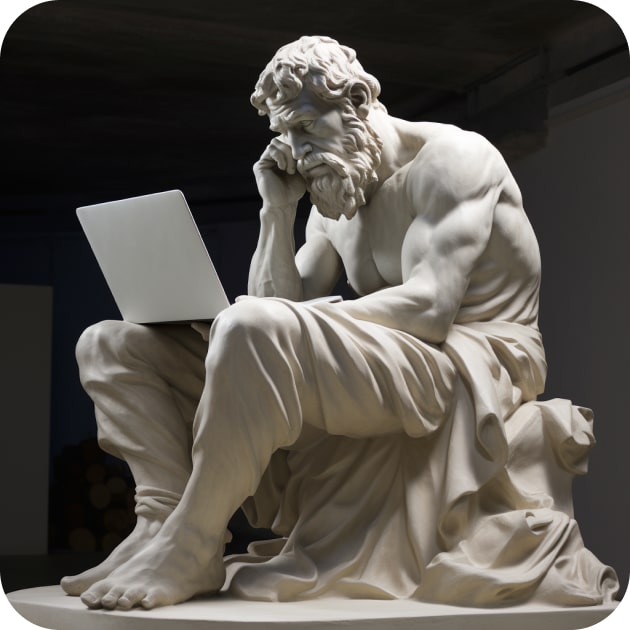
Index
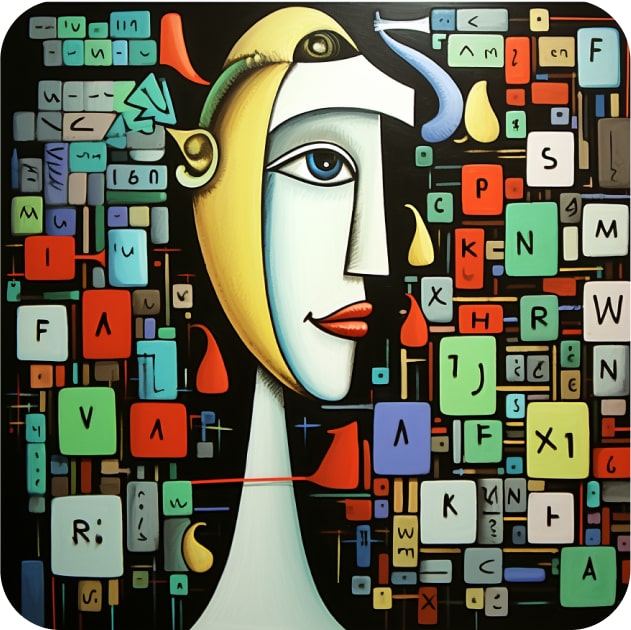
Integrate
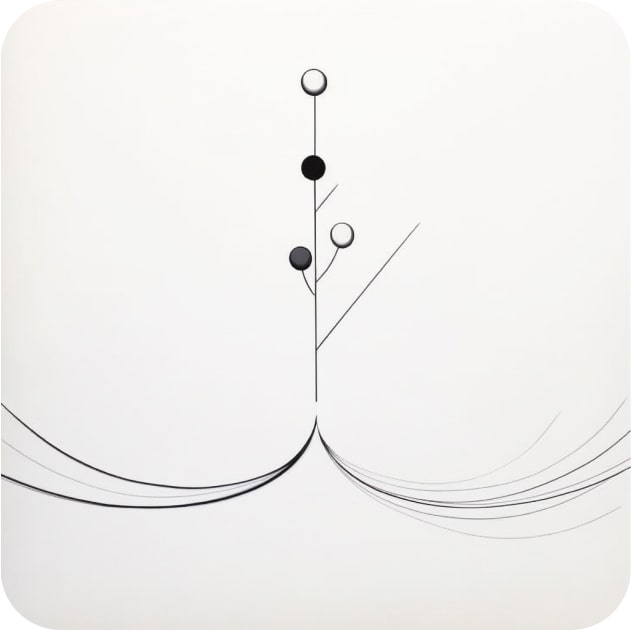
Lineage
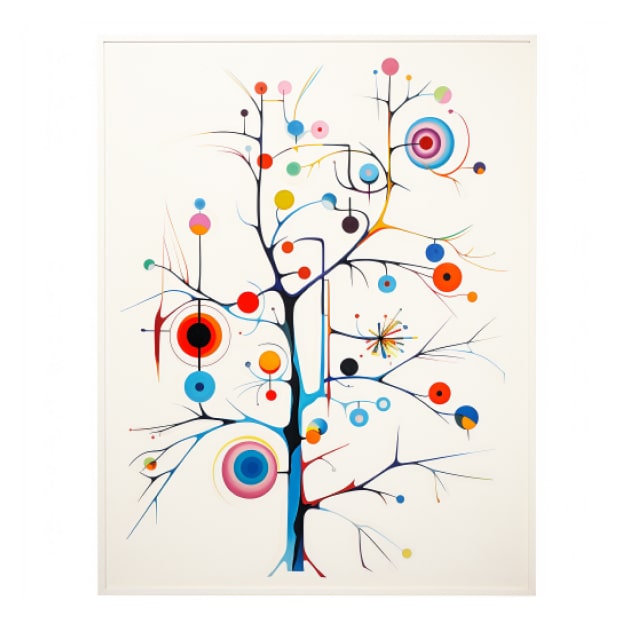
Linearizability
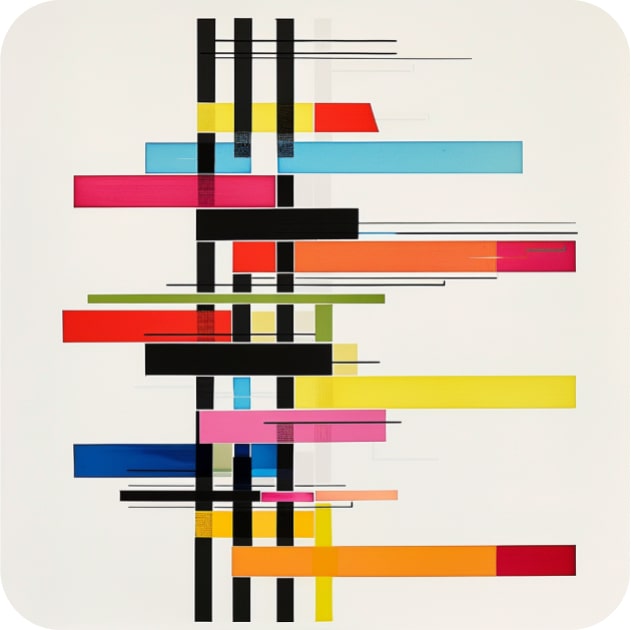
Materialize
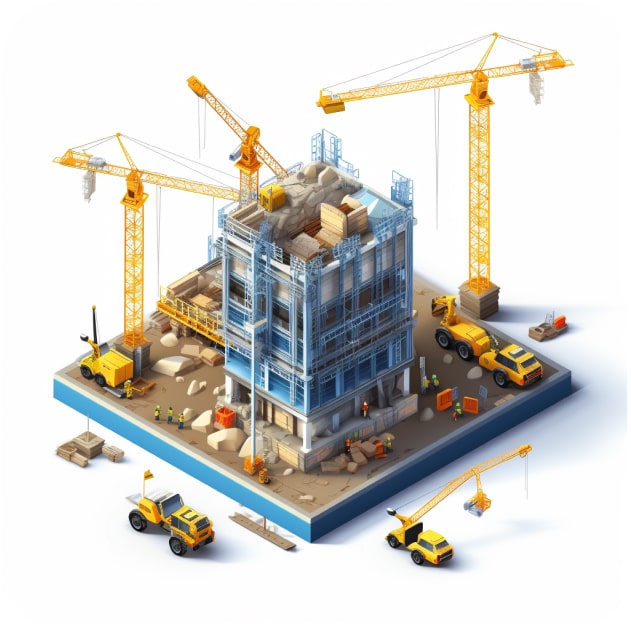
Memoize
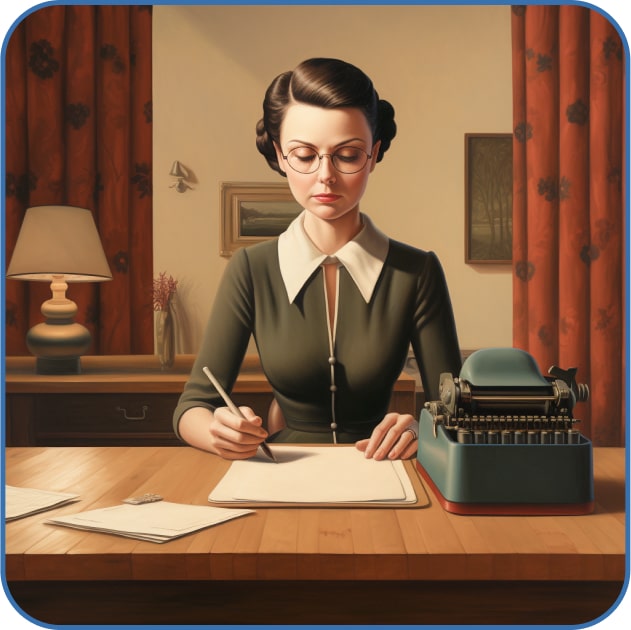
Merge
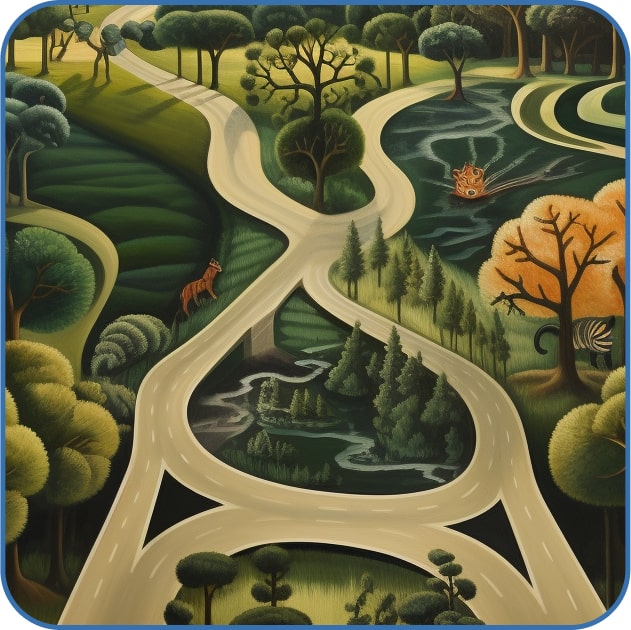
Model
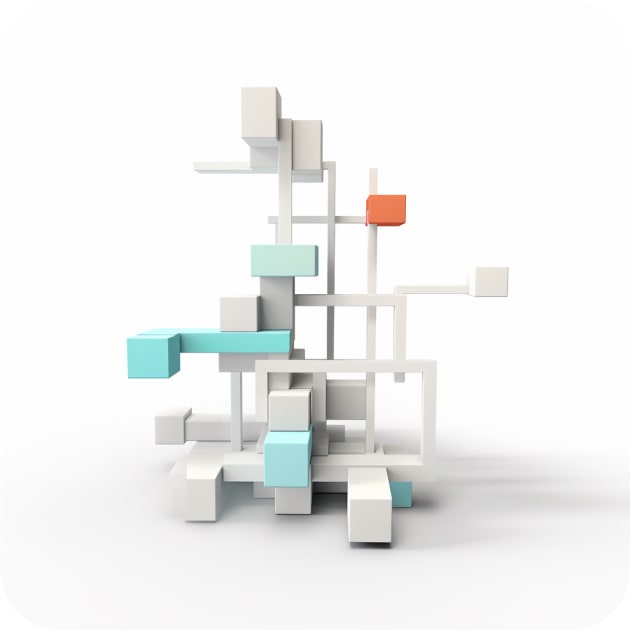
Monitor
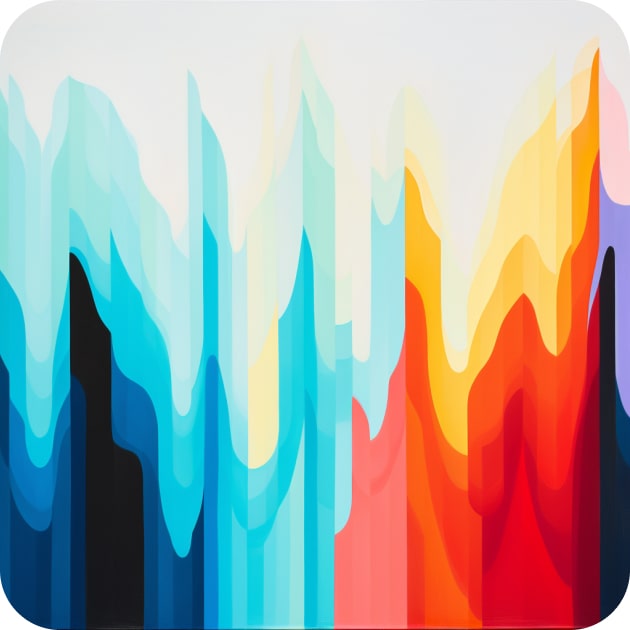
Named Entity Recognition
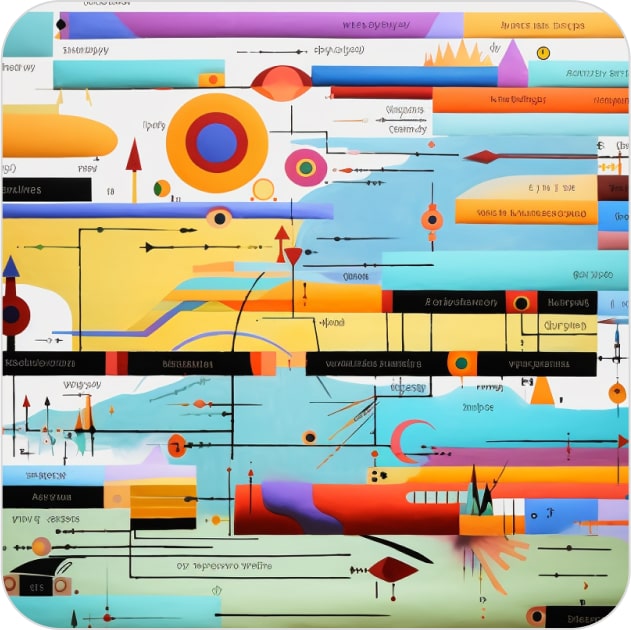
Partition
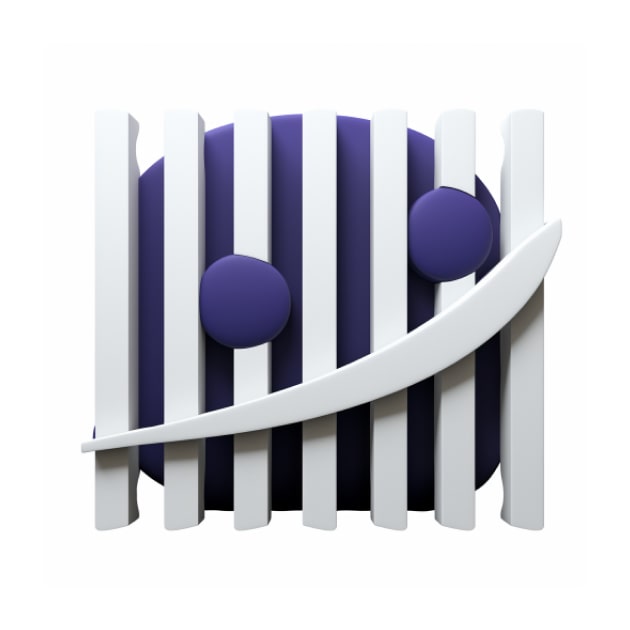
Prep
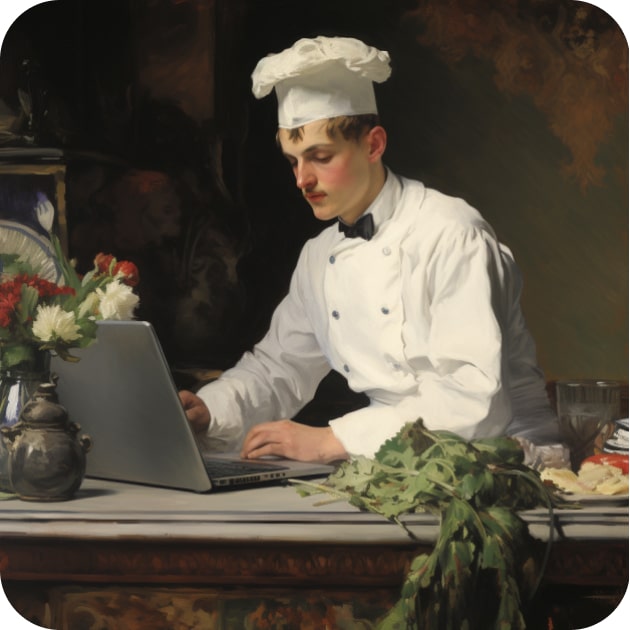
Preprocess
Primary Key
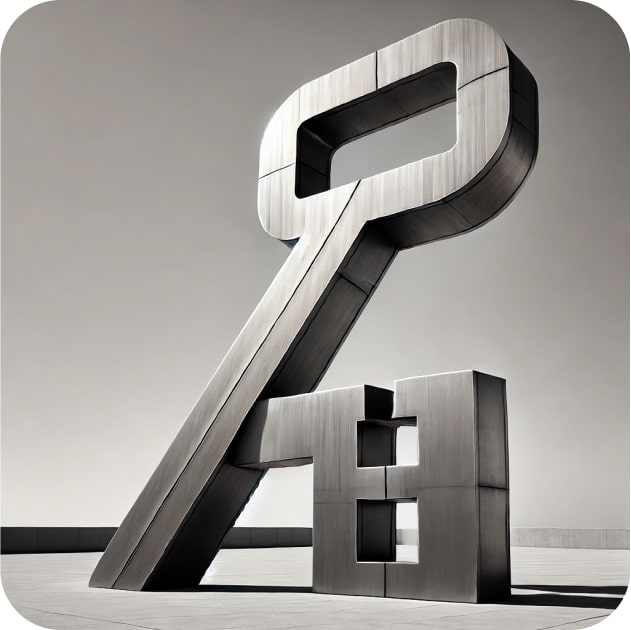
Replicate
Scaling
Schema Inference
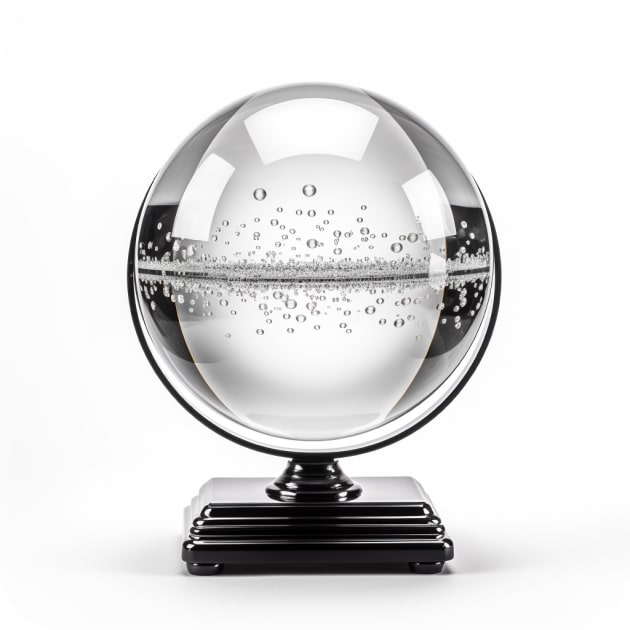
Schema Mapping
Secondary Index
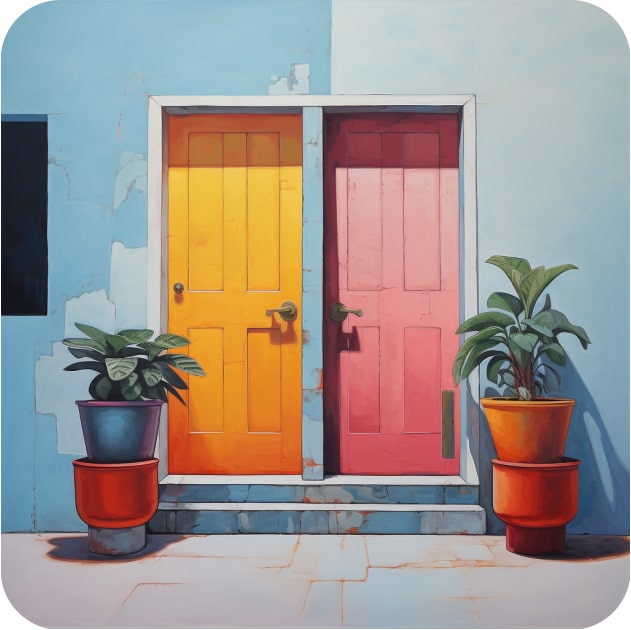
Software-defined Asset
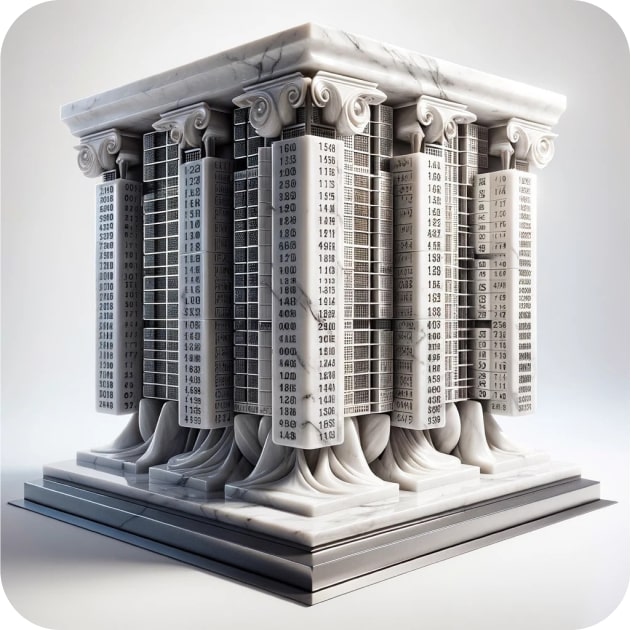
Synchronize
Validate
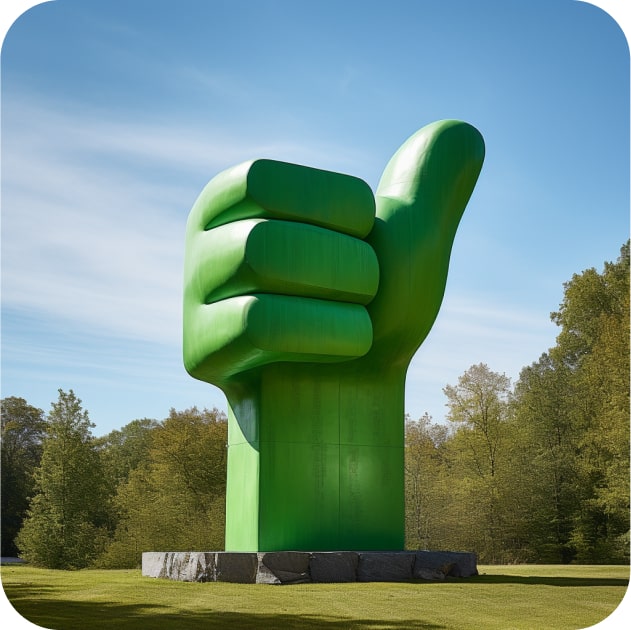
Version
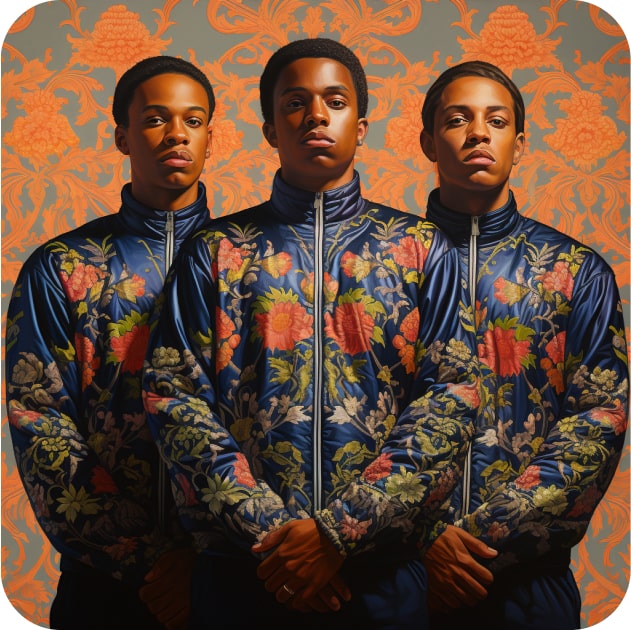