Schema mapping definition:
Schema mapping is the process of mapping the schema of one dataset to the schema of another dataset in order to integrate or merge them. It involves mapping the fields or attributes of one dataset to the fields or attributes of another dataset, ensuring that the data types, formats, and other properties match between the two datasets. Schema mapping is a crucial step in data integration, as it enables datasets with different schemas to be combined and analyzed together.
Schema mapping example using Python:
Please note that you need to have the necessary Python libraries installed in your Python environment to run this code.
In Python, one way to perform schema mapping is by using the PySpark library. Here's an example:
# Importing the required libraries
from pyspark.sql import SparkSession
from pyspark.sql.functions import col
# Creating a SparkSession
spark = SparkSession.builder.appName("Schema Mapping Example").getOrCreate()
# Creating a sample dataframe with multiple columns
data = [("John", 25, "25000.50"), ("Smith", 30, "35000.75"), ("Jane", 28, 32000.25)]
df = spark.createDataFrame(data, ["name", "age", "salary"])
# Defining the mapping schema
mapping_schema = {
"name": "string",
"age": "integer",
"salary": "double"
}
# Creating a new dataframe with the mapped schema
mapped_df = df.select([col(c).cast(mapping_schema[c]).alias(c) for c in mapping_schema.keys()])
# Displaying the original and mapped dataframes
print(f"Original dataframe: {df}")
print(f"Mapped dataframe: {mapped_df}")
# Applying some transformations on the mapped dataframe
filtered_df = mapped_df.filter(mapped_df.age > 25)
grouped_df = filtered_df.groupBy("name").agg({"salary": "sum"})
# Stopping the SparkSession
spark.stop()
This will print out the schema of each dataframe showing the new mapping:
Original dataframe: DataFrame[name: string, age: bigint, salary: string]
Mapped dataframe: DataFrame[name: string, age: int, salary: double]
Append
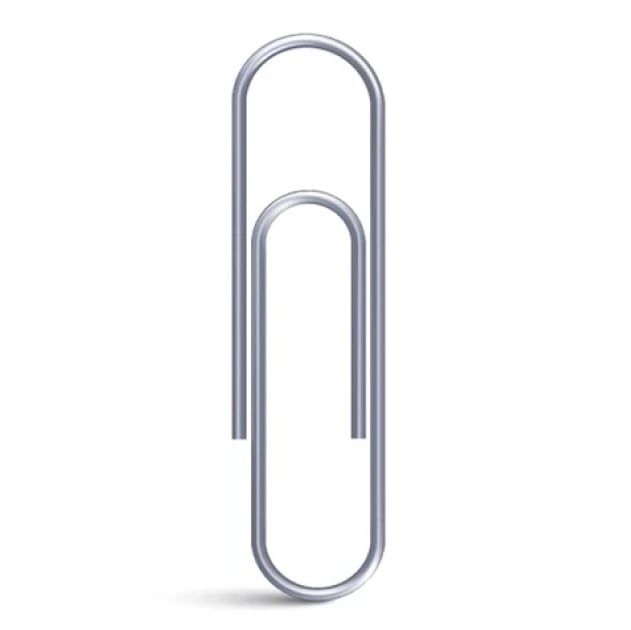
Archive
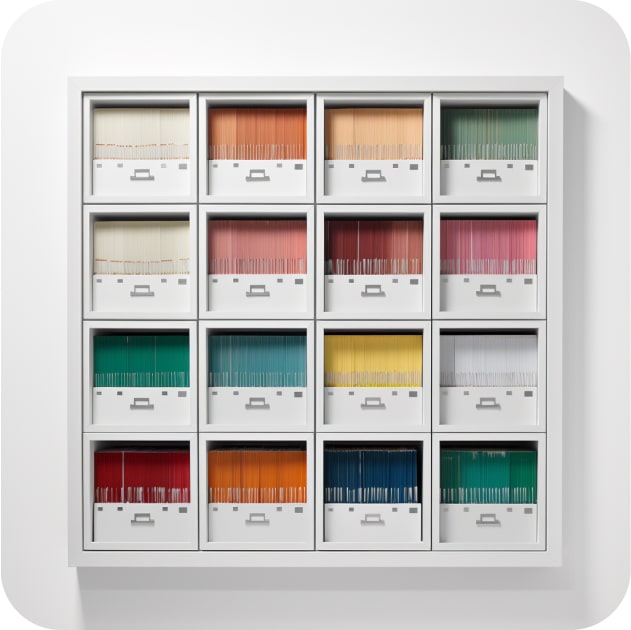
Augment
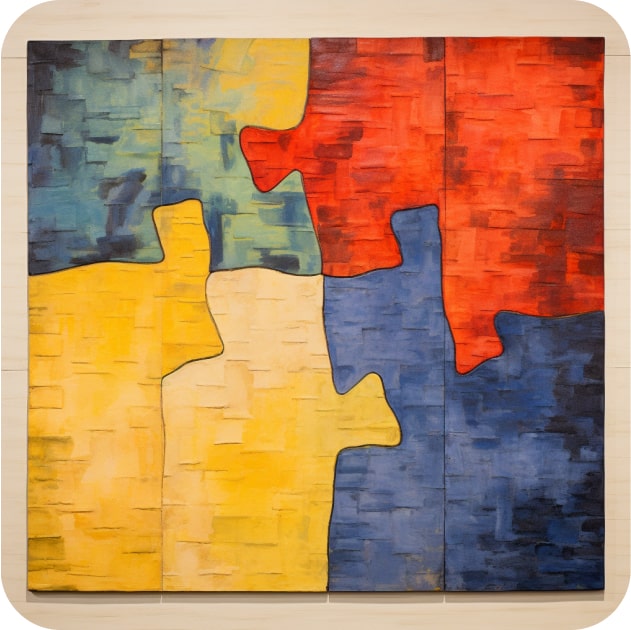
Auto-materialize
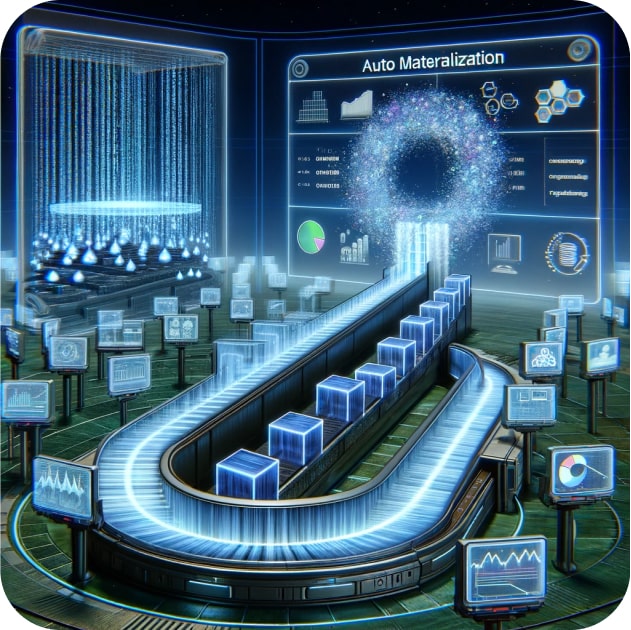
Backup
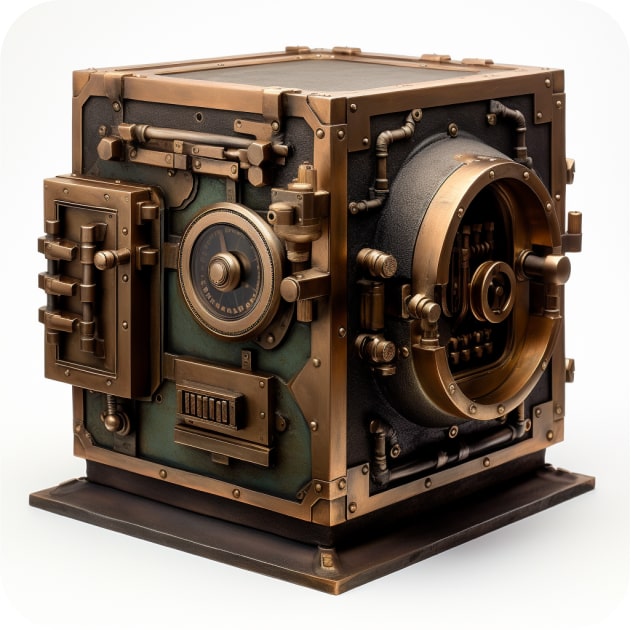
Batch Processing
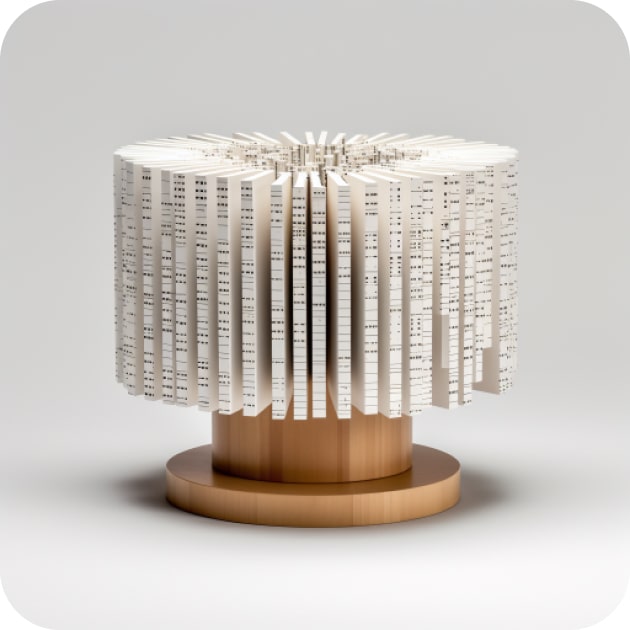
Cache
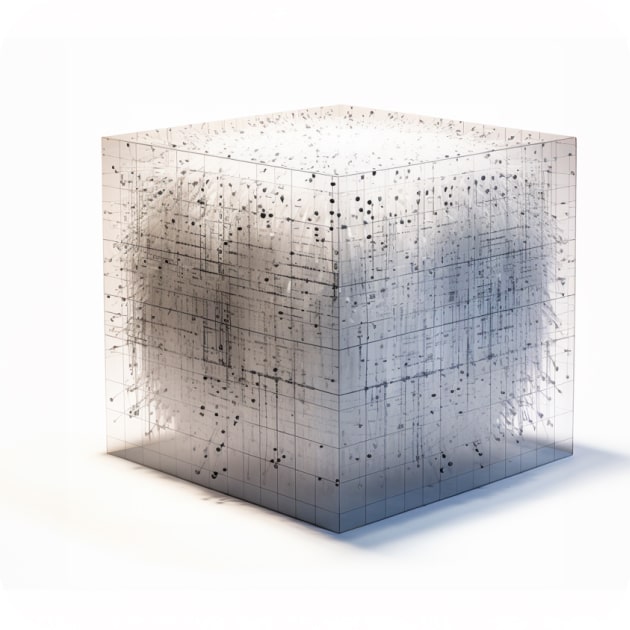
Categorize
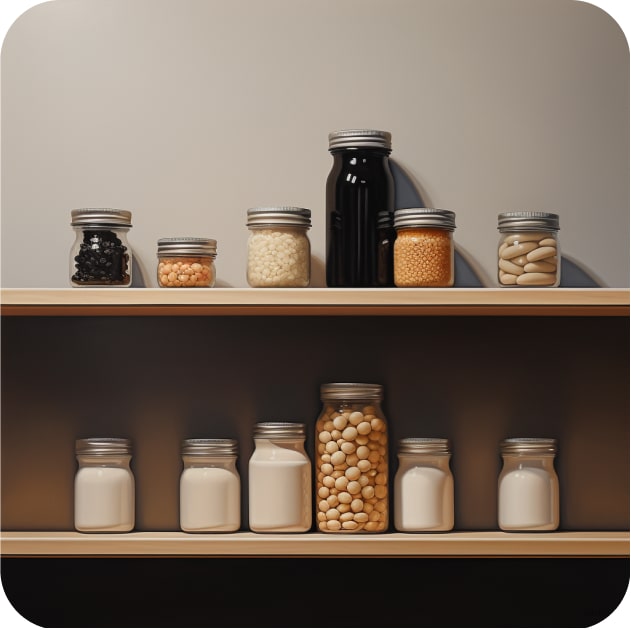
Checkpointing
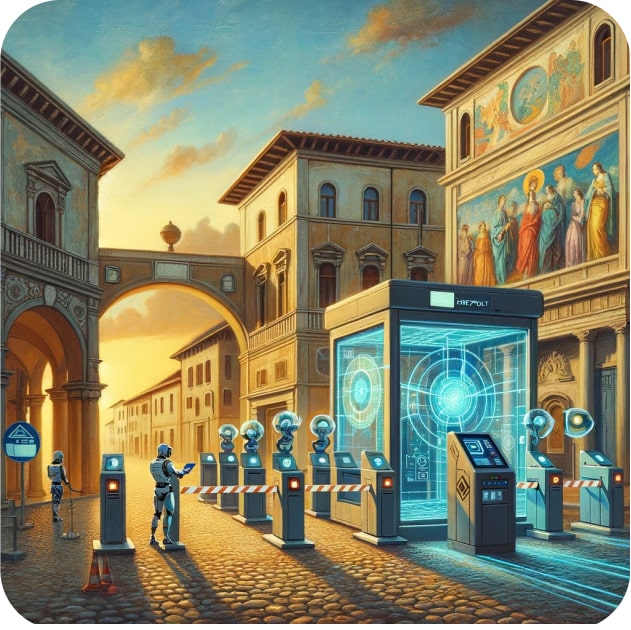
Deduplicate
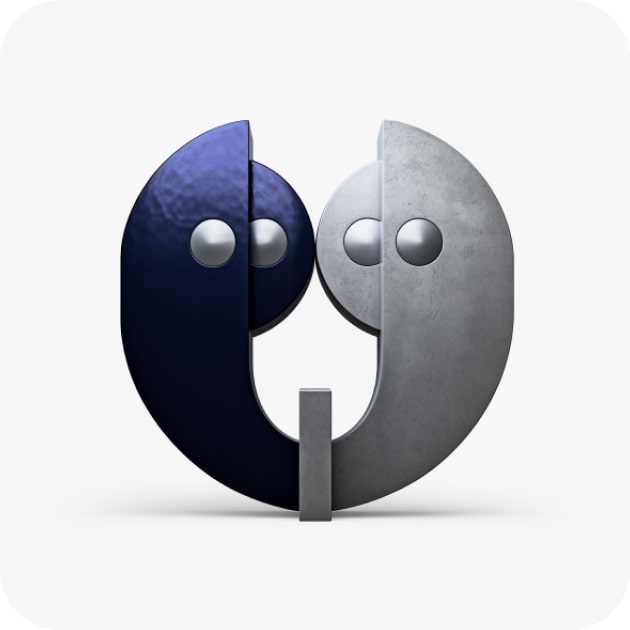
Deserialize
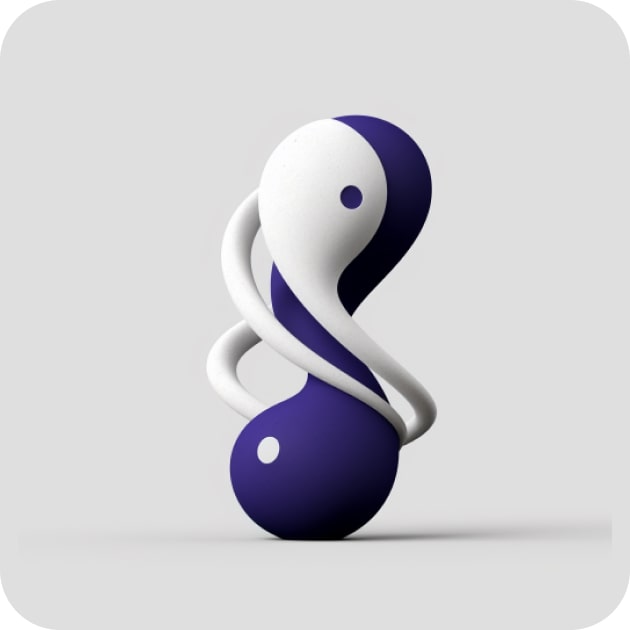
Dimensionality
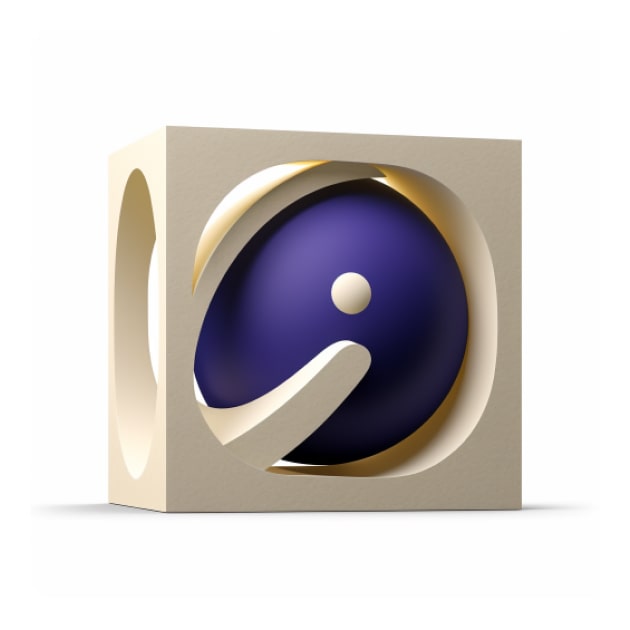
Encapsulate
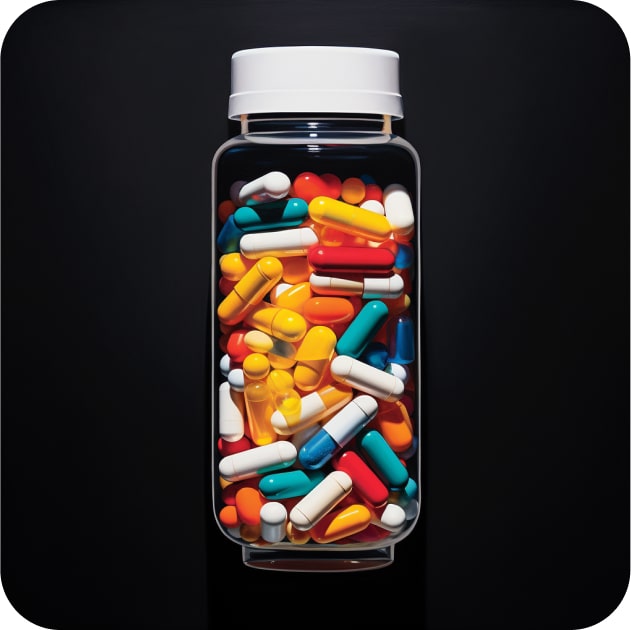
Enrich
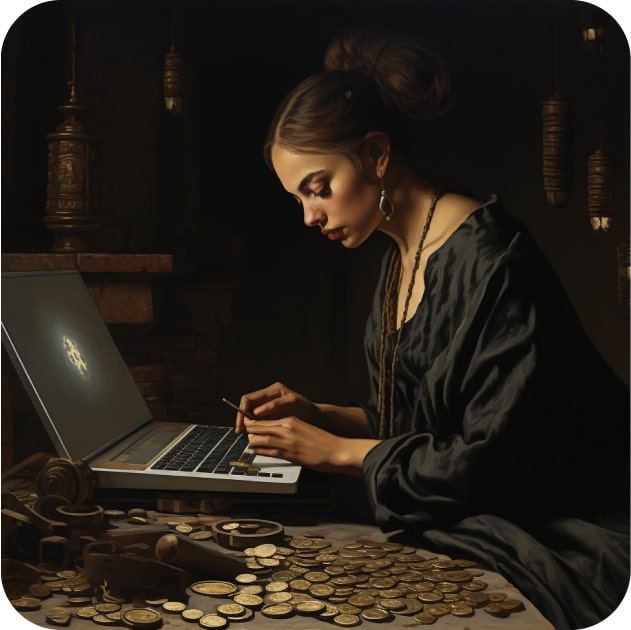
Export
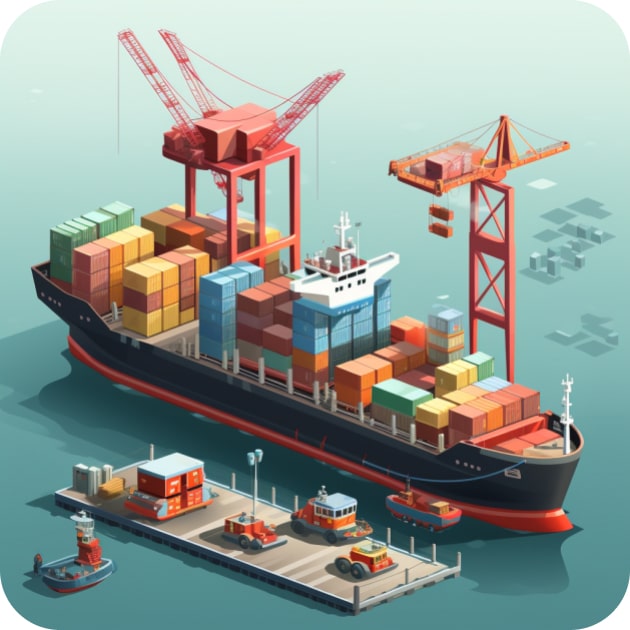
Graph Theory
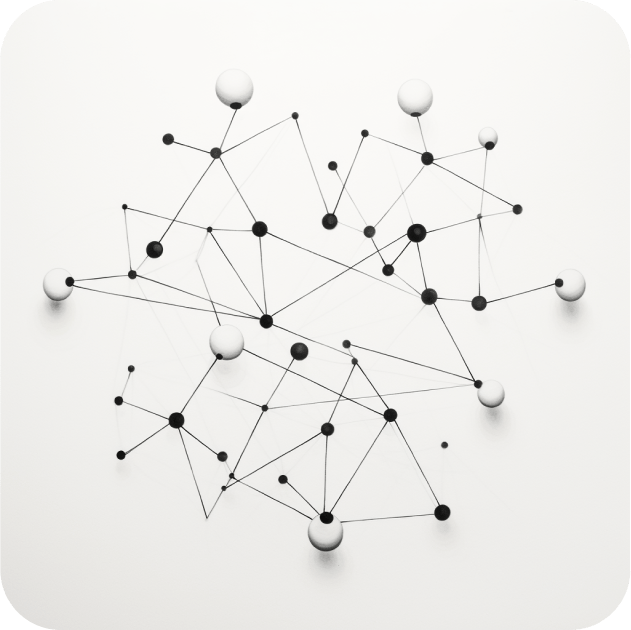
Idempotent
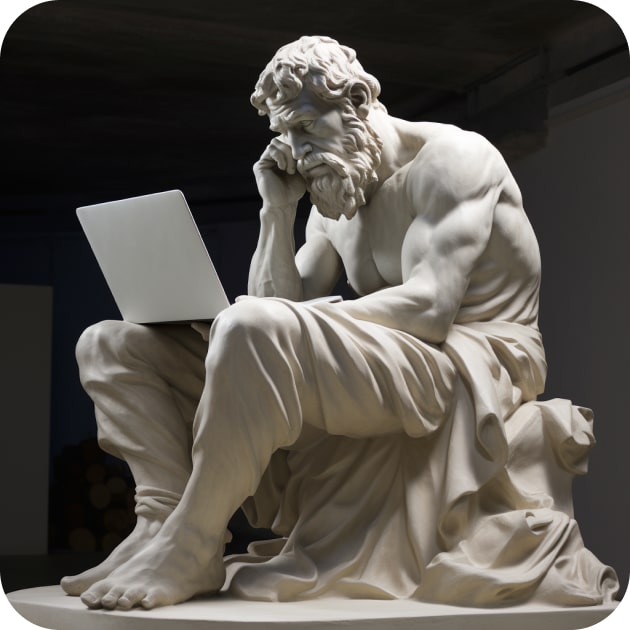
Index
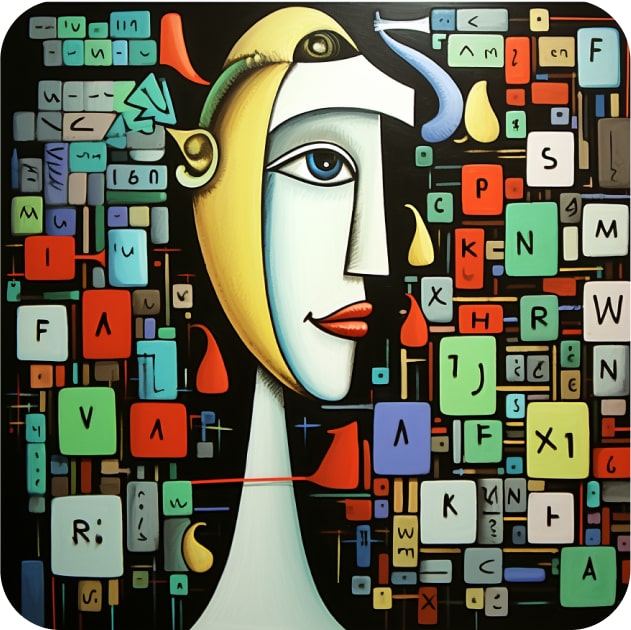
Integrate
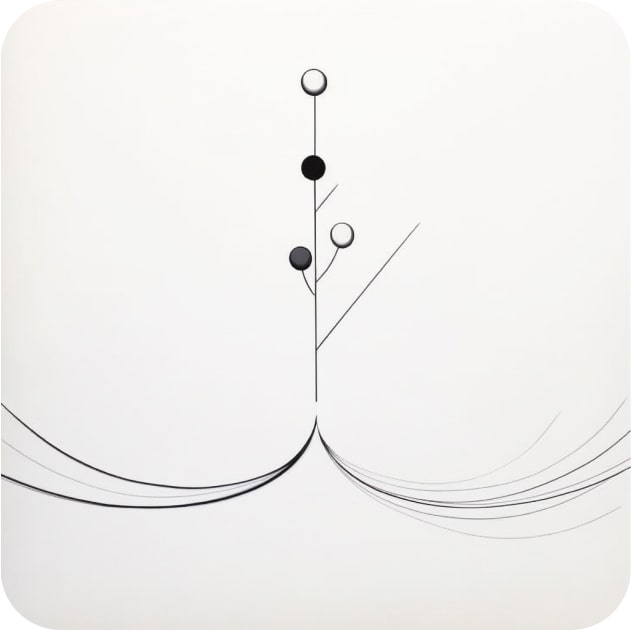
Lineage
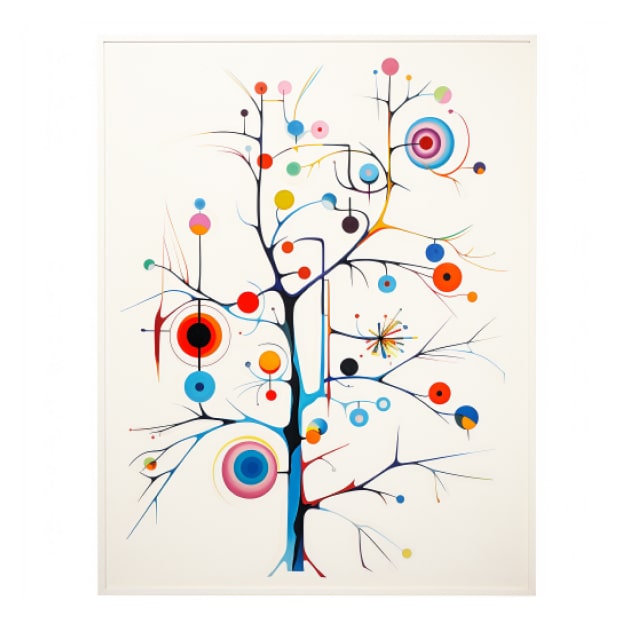
Linearizability
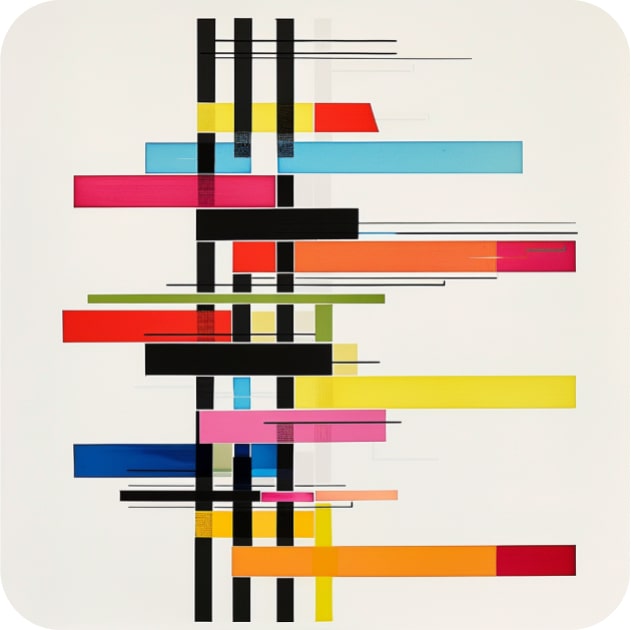
Materialize
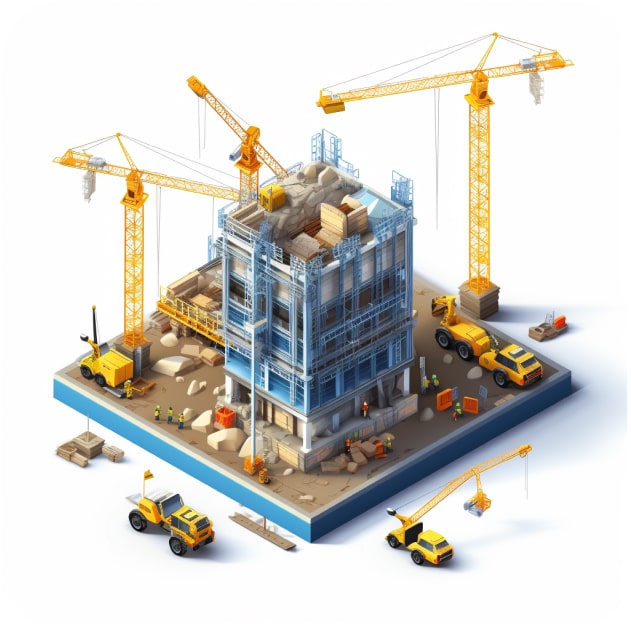
Memoize
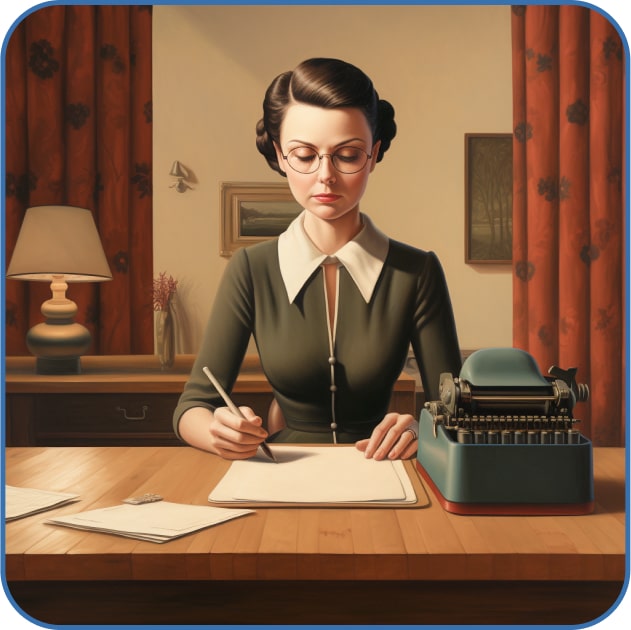
Merge
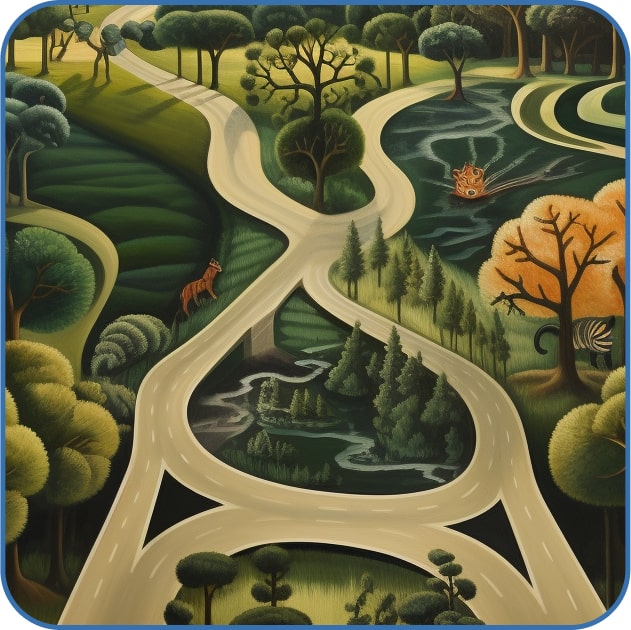
Model
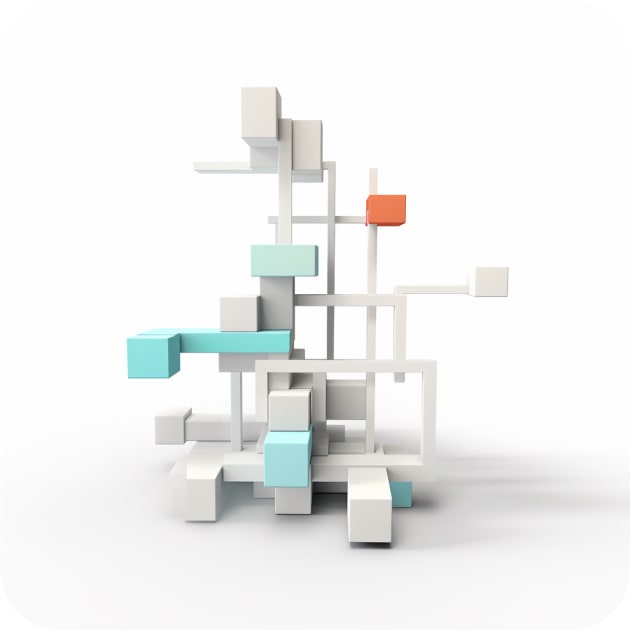
Monitor
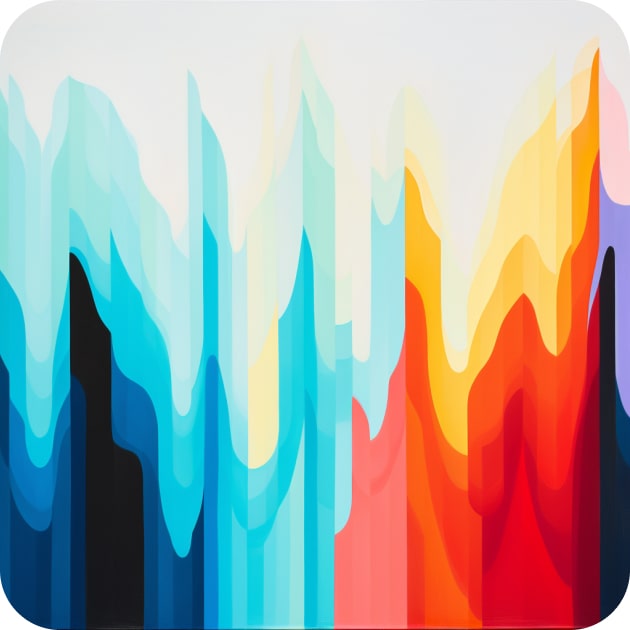
Named Entity Recognition
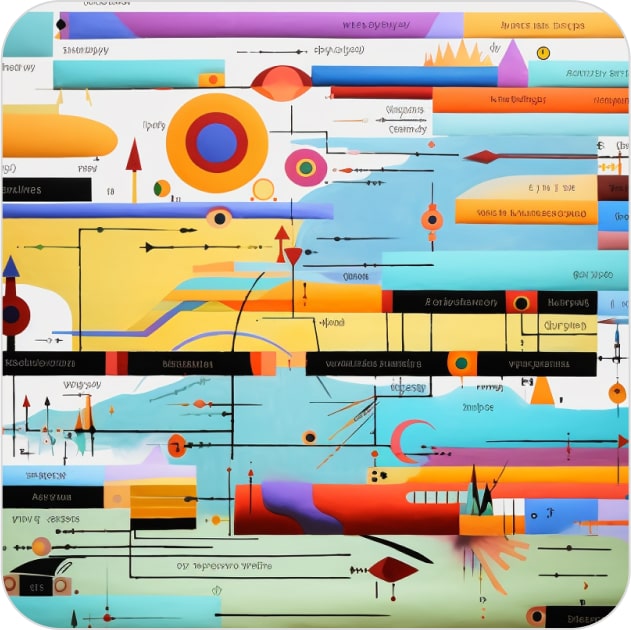
Parse
Partition
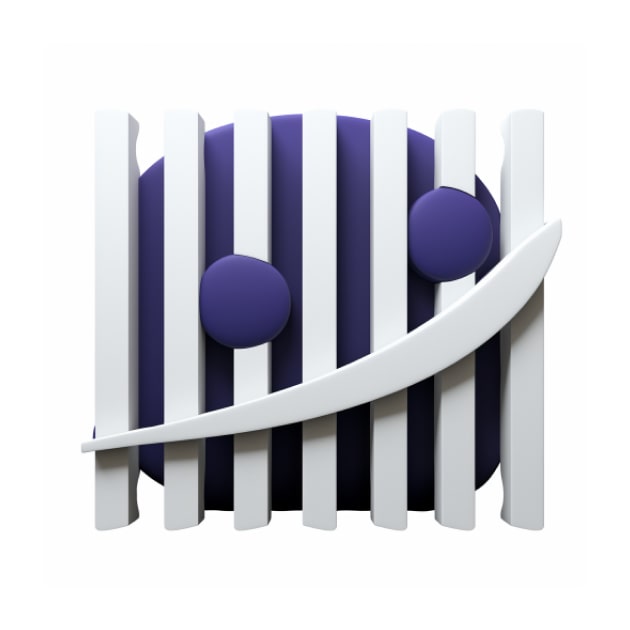
Prep
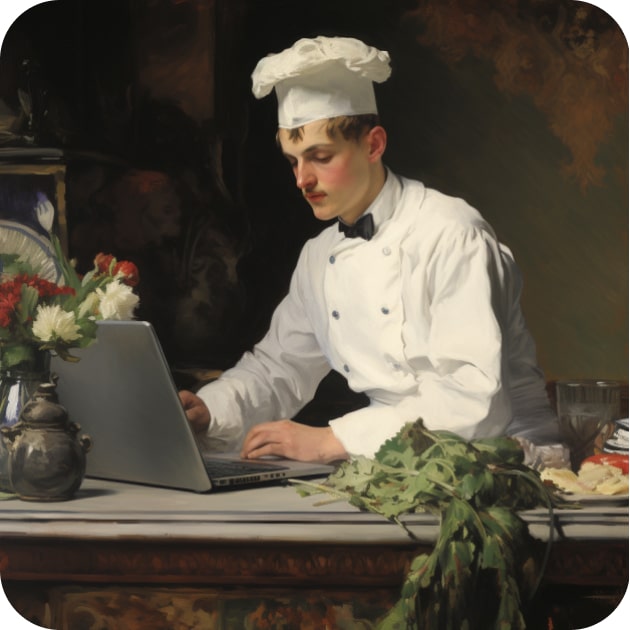
Preprocess
Primary Key
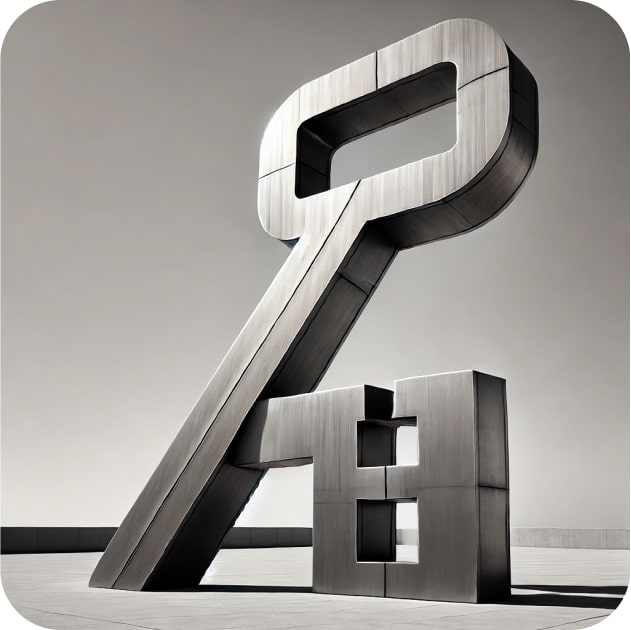
Replicate
Scaling
Schema Inference
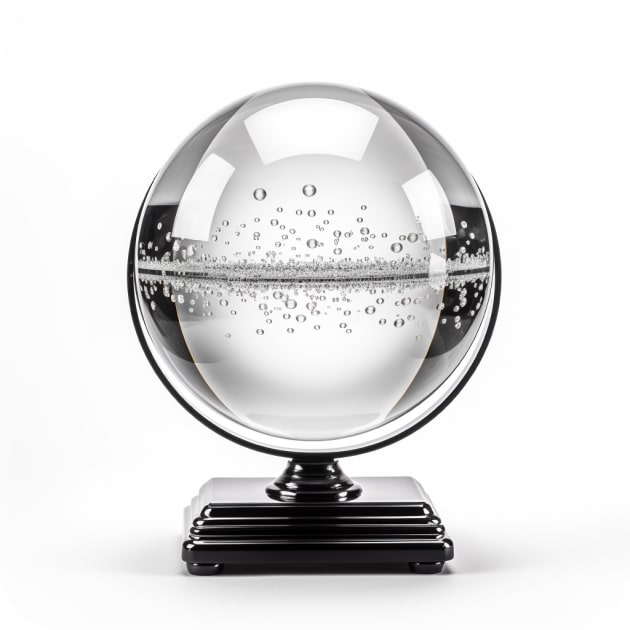
Secondary Index
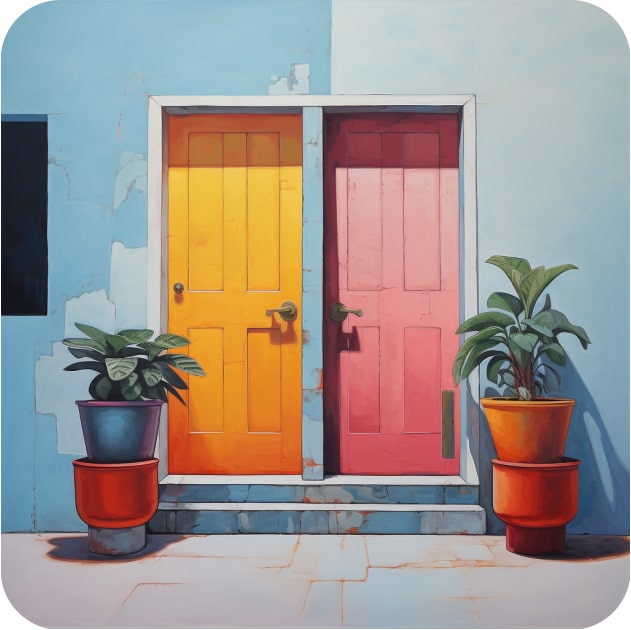
Software-defined Asset
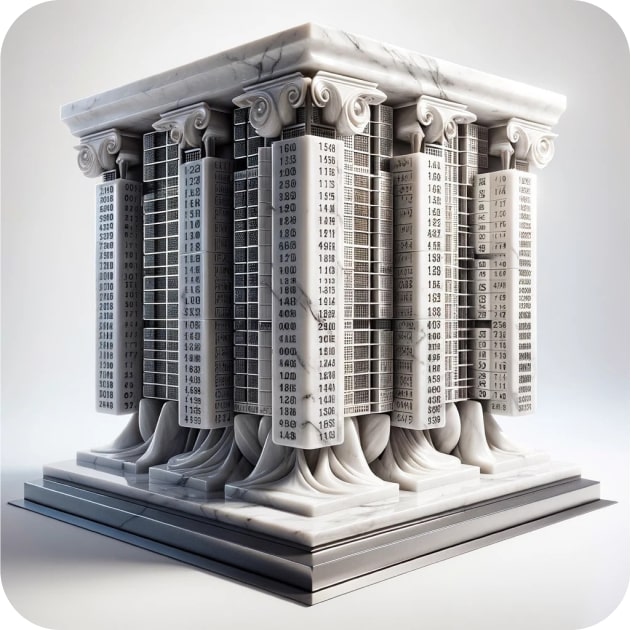
Synchronize
Validate
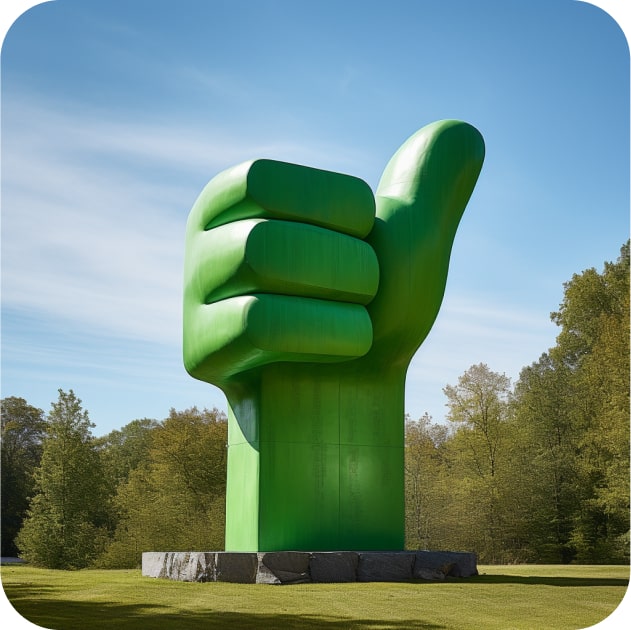
Version
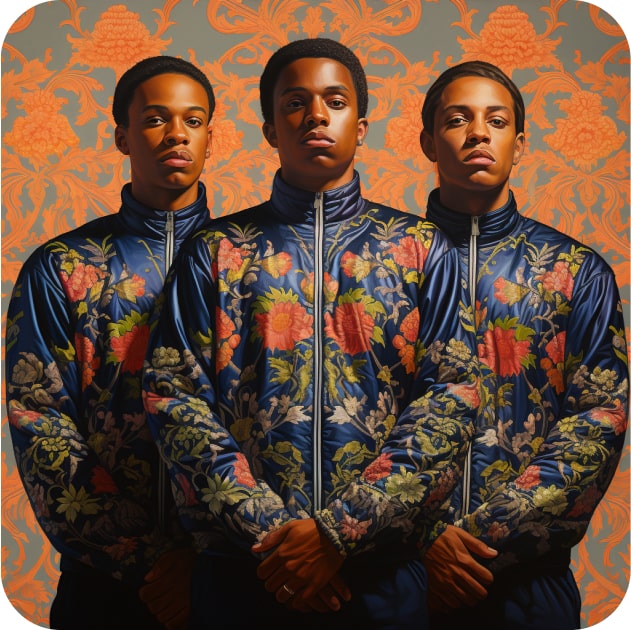