Dagster Data Engineering Glossary:
Named Entity Recognition
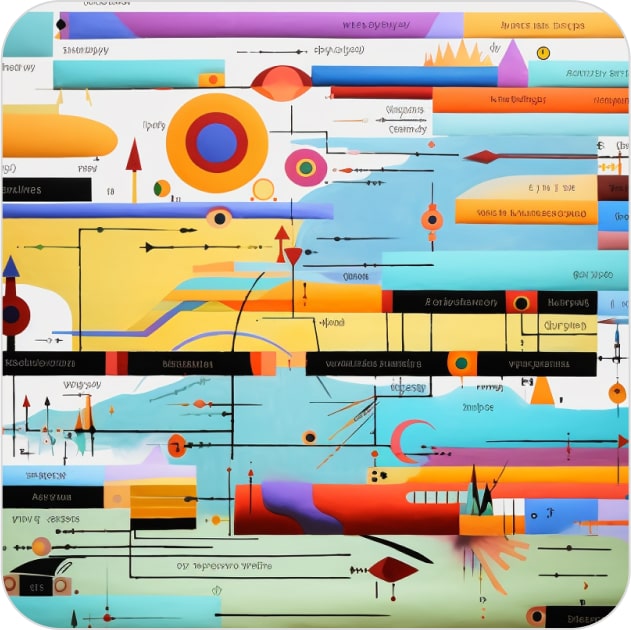
Named entity recognition definition:
Named Entity Recognition (NER) is a subtask of information extraction that seeks to locate and classify named entities in text into pre-defined categories such as person names, organizations, locations, medical codes, time expressions, quantities, monetary values, percentages, etc. Here is a simple Python program for NER using Spacy, a popular library for Natural Language Processing (NLP).
An example of named entity recognition in Python:
Please note that you need to have the necessary Python libraries installed in your Python environment to run the following code examples.
To run this example, you'll need to install the spacy
library and download a language model. You can do this using pip:
pip install spacy
python -m spacy download en_core_web_sm
Now run the Python code:
In this example, we first import the spacy
library and load the English language model. We then process a text string with the nlp
function, which returns a Doc
object that holds all information about the tokens, their linguistic features and their relationships.
We print out all noun phrases and verbs in the text, and then iterate over the ents
property of the Doc
object, which gives us the named entities. For each entity, we print its text and its label.
Please note that the model may not be perfect and might not catch all named entities correctly, especially with informal language, slang, or typos.
import spacy
# Load English tokenizer, tagger, parser, NER and word vectors
nlp = spacy.load("en_core_web_sm")
# Process whole documents
text = ("Dagster is a new data orchestration solution for modern data engineering."
"Unlike other solutions, Dagster provides a declarative approach to building data pipelines."
"With Dagster, your data engineering team can speed up development cycles, "
"observe your critical data assets, "
"and confidently test your code so that you can push to production in full confidence."
)
doc = nlp(text)
# Analyze syntax
print("Noun phrases:", [chunk.text for chunk in doc.noun_chunks])
print("Verbs:", [token.lemma_ for token in doc if token.pos_ == "VERB"])
# Find named entities, phrases and concepts
for entity in doc.ents:
print(entity.text, entity.label_)
This should yield the following output:
Noun phrases: ['Dagster', 'a new data orchestration solution', 'modern data engineering', 'other solutions', 'Dagster', 'a declarative approach', 'data pipelines', 'Dagster', 'your data engineering team', 'development cycles', 'your critical data assets', 'your code', 'you', 'production', 'full confidence']
Verbs: ['provide', 'build', 'speed', 'observe', 'test', 'push']
Dagster PERSON
Dagster PERSON
Dagster PERSON
Append
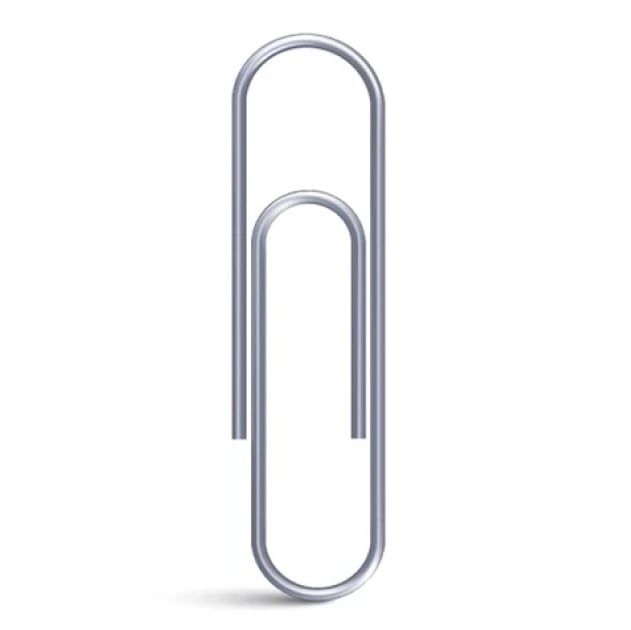
Archive
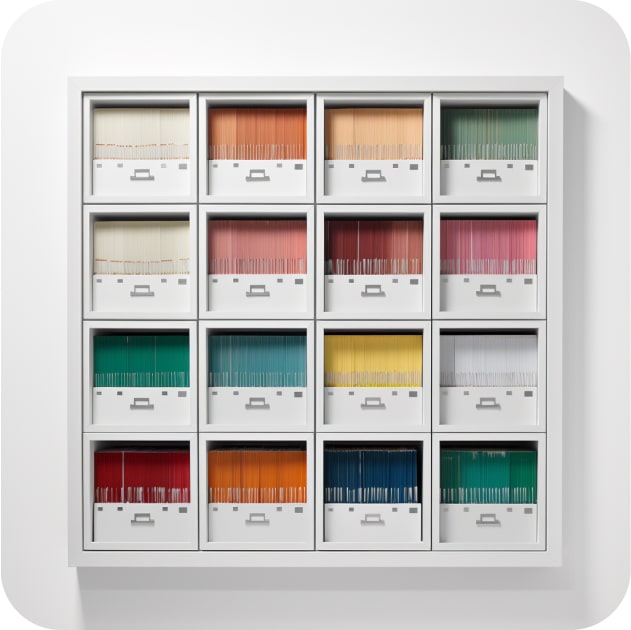
Augment
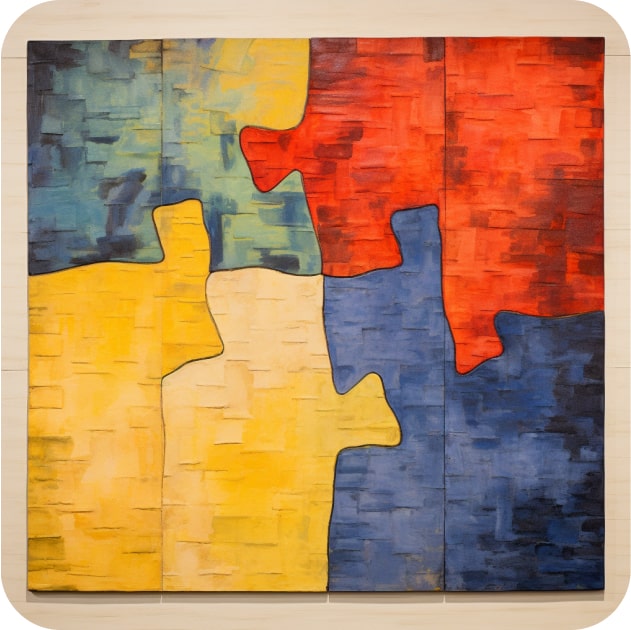
Auto-materialize
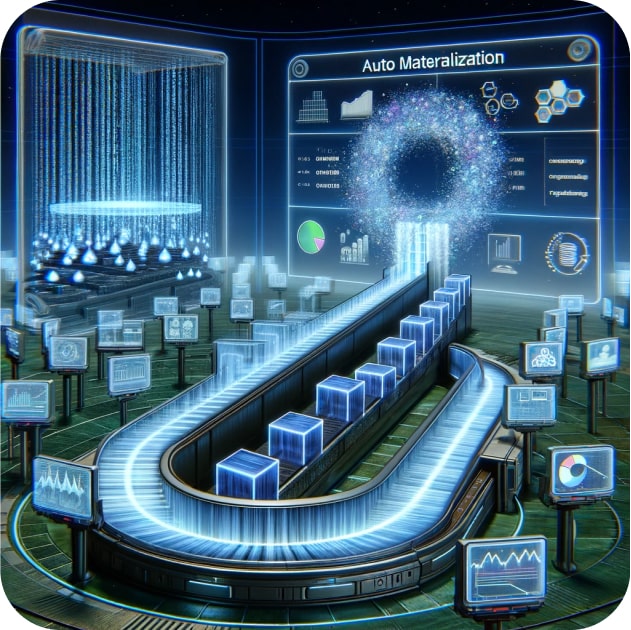
Backup
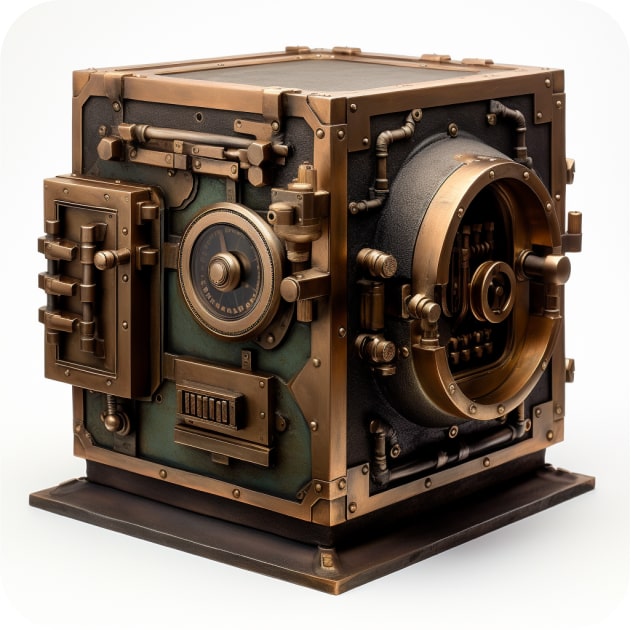
Batch Processing
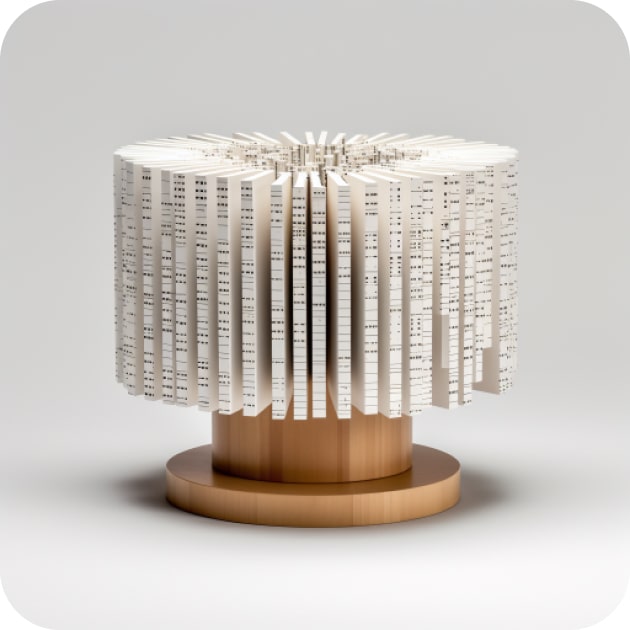
Cache
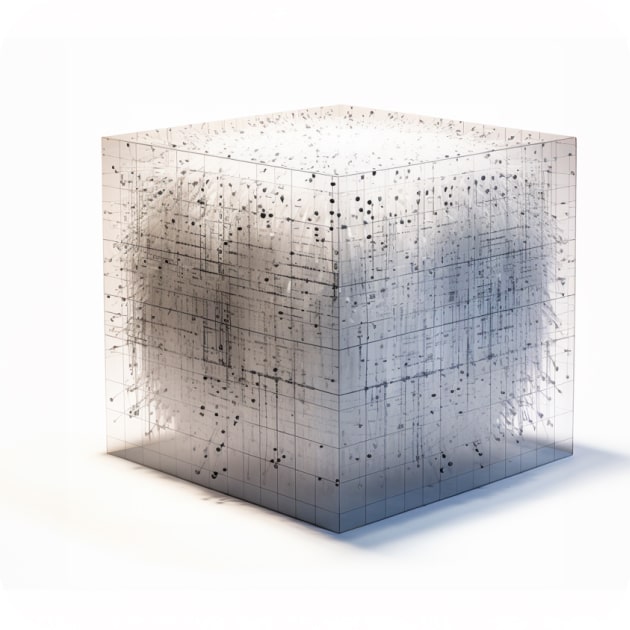
Categorize
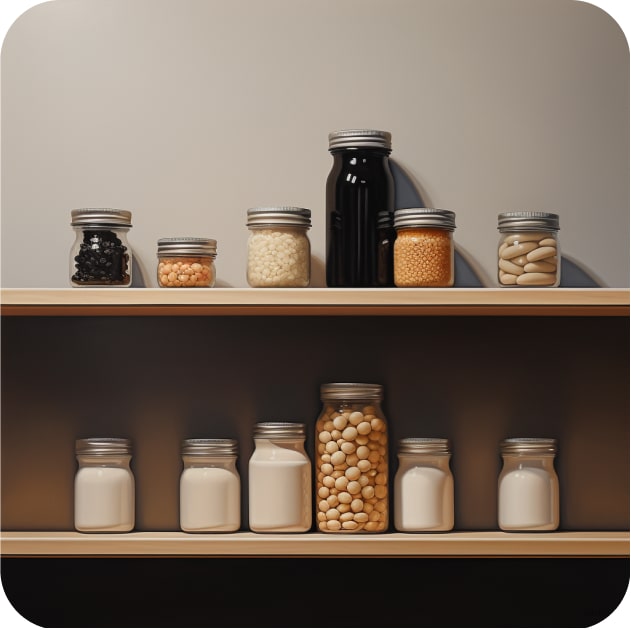
Checkpointing
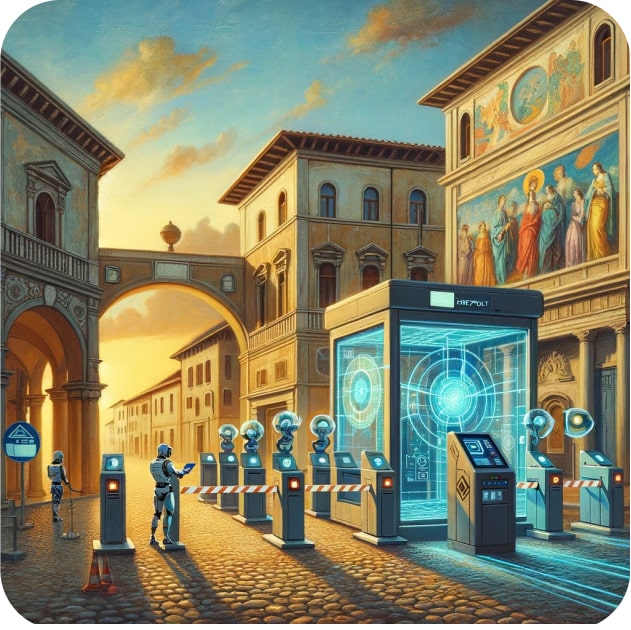
Deduplicate
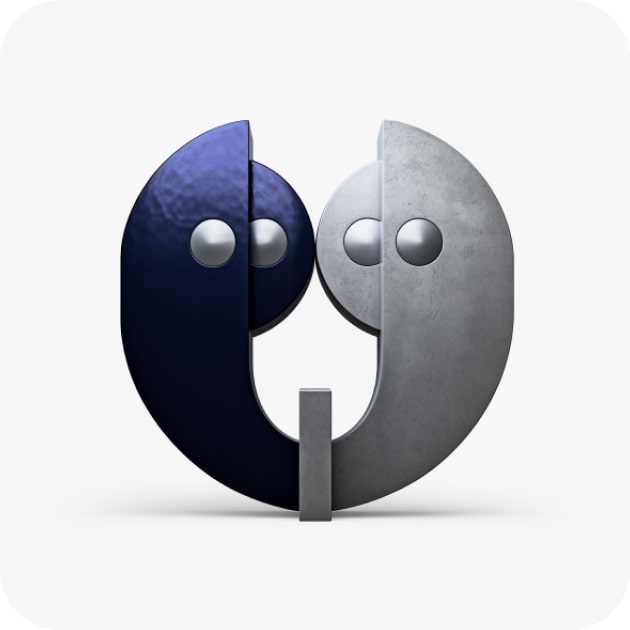
Deserialize
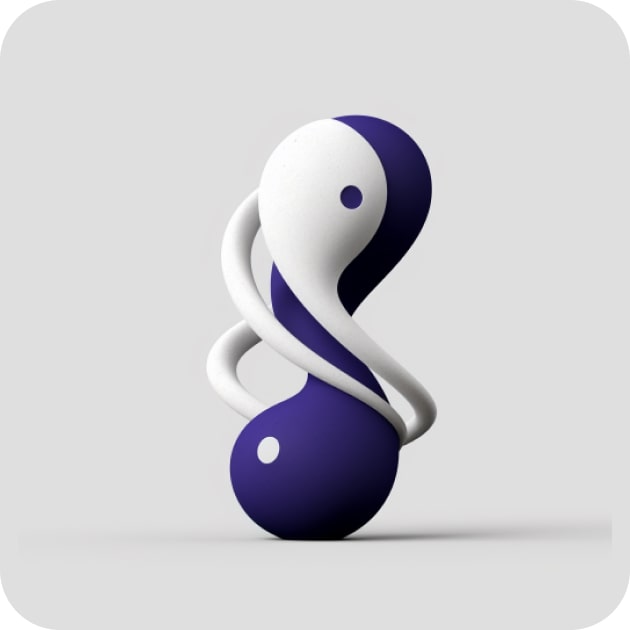
Dimensionality
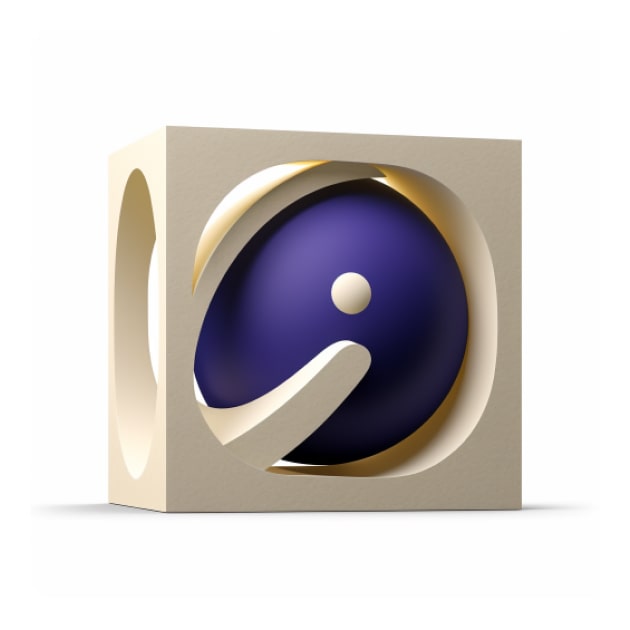
Encapsulate
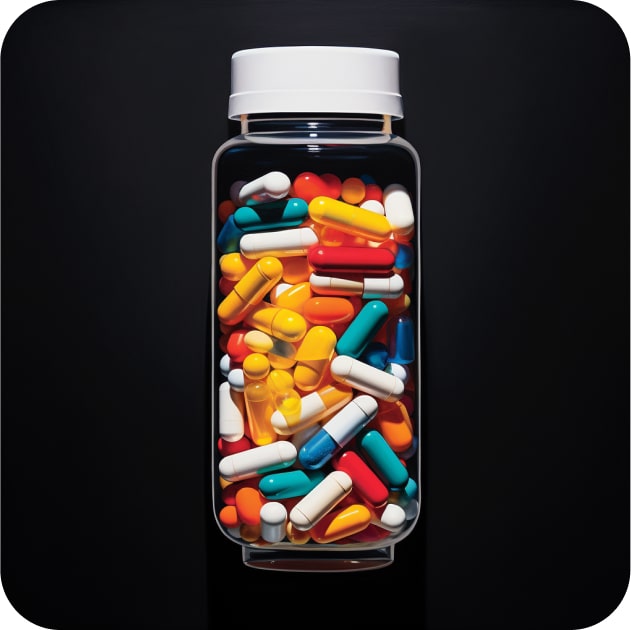
Enrich
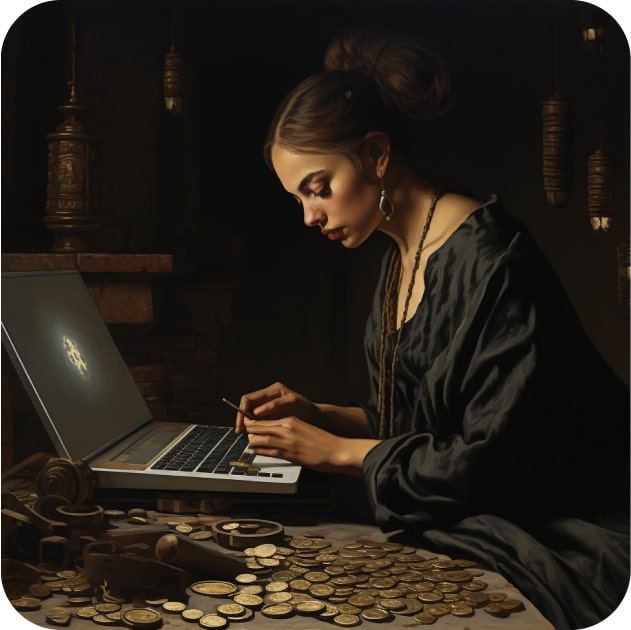
Export
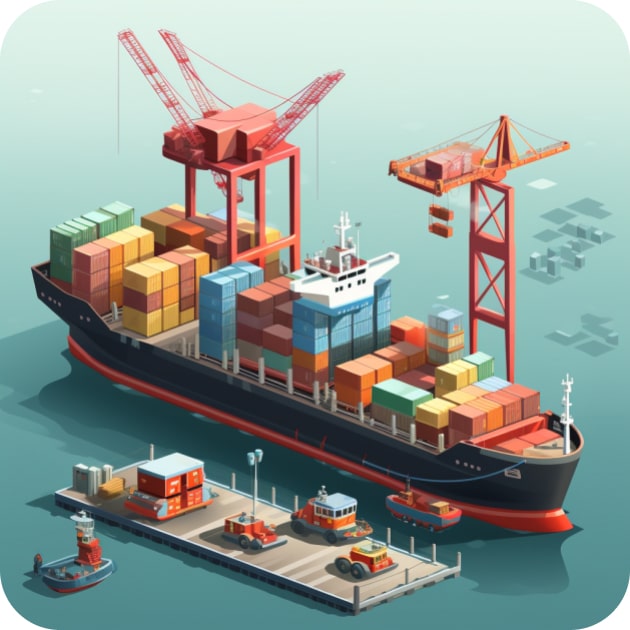
Graph Theory
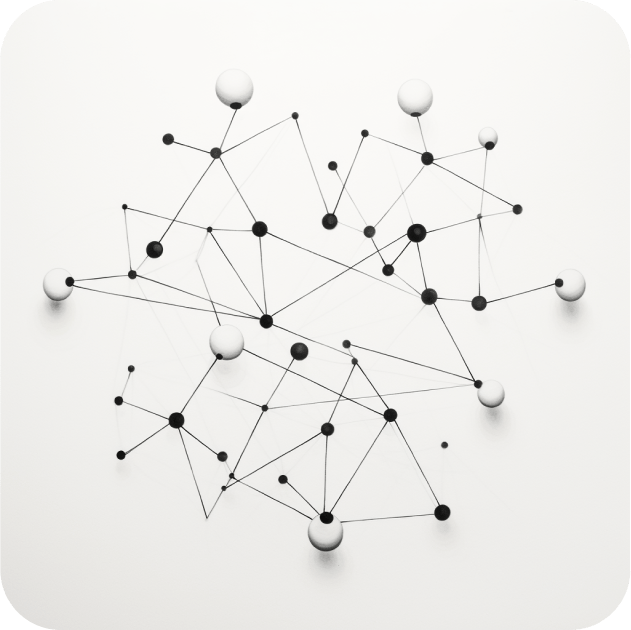
Idempotent
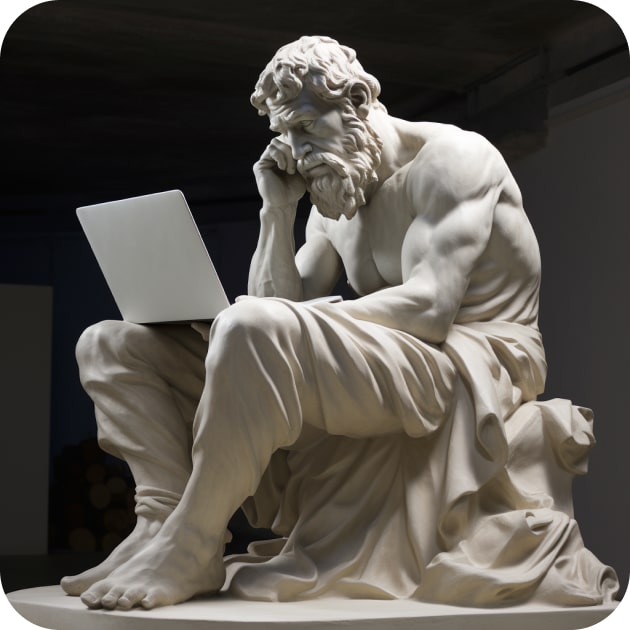
Index
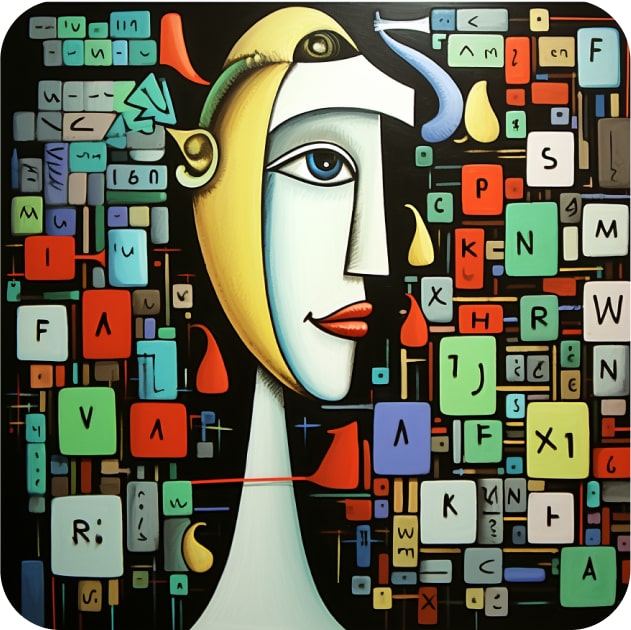
Integrate
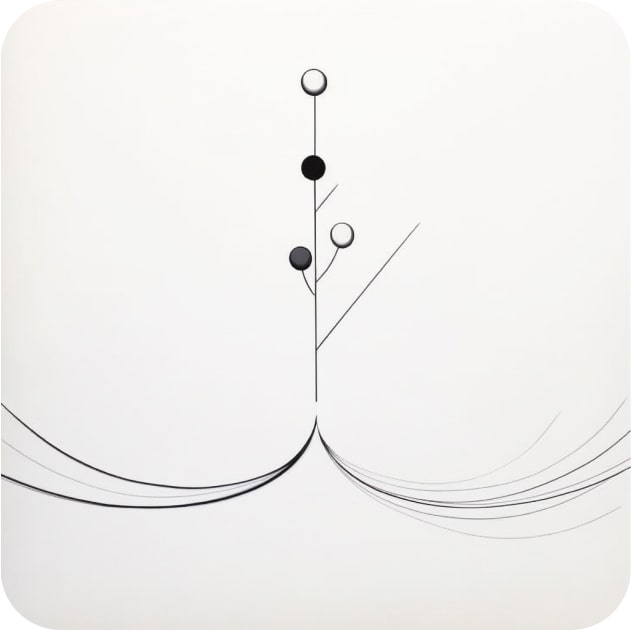
Lineage
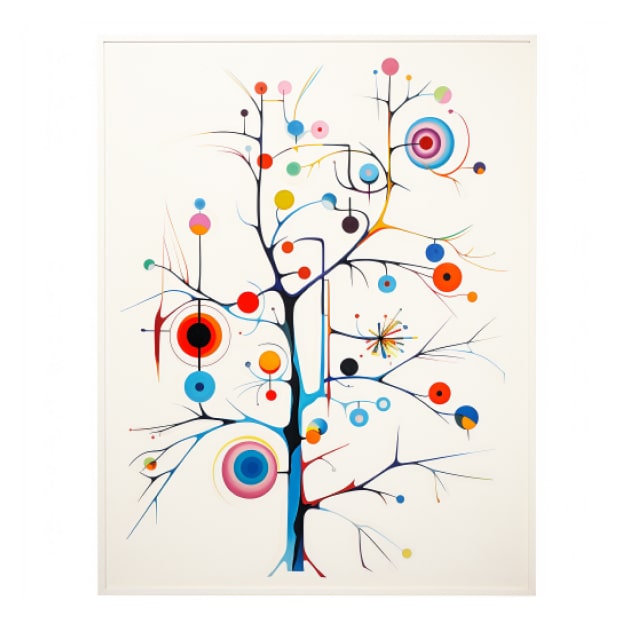
Linearizability
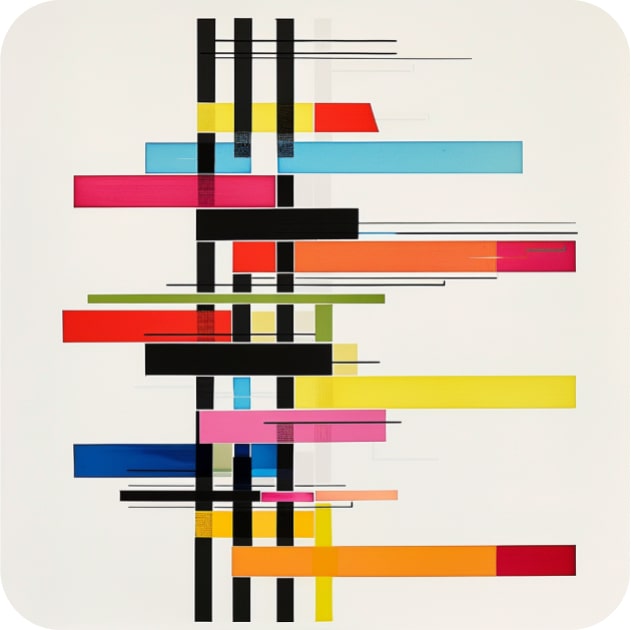
Materialize
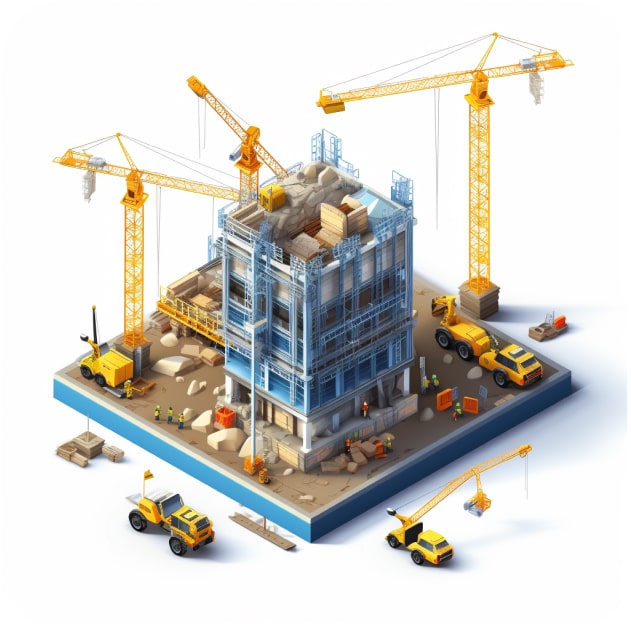
Memoize
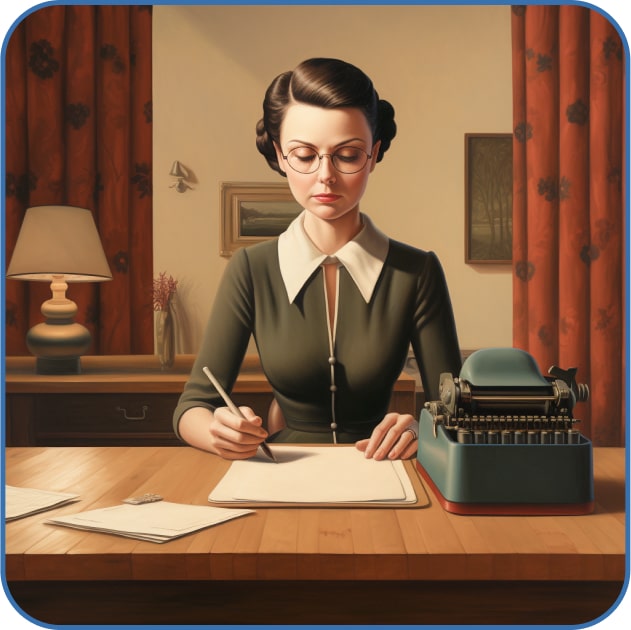
Merge
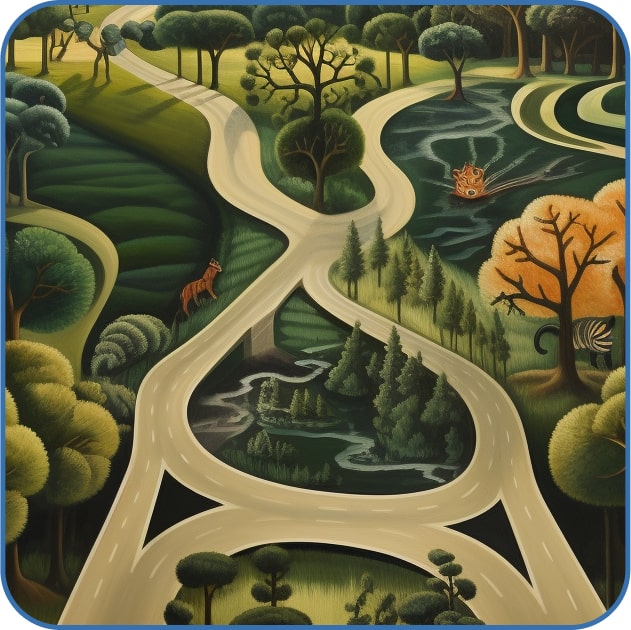
Model
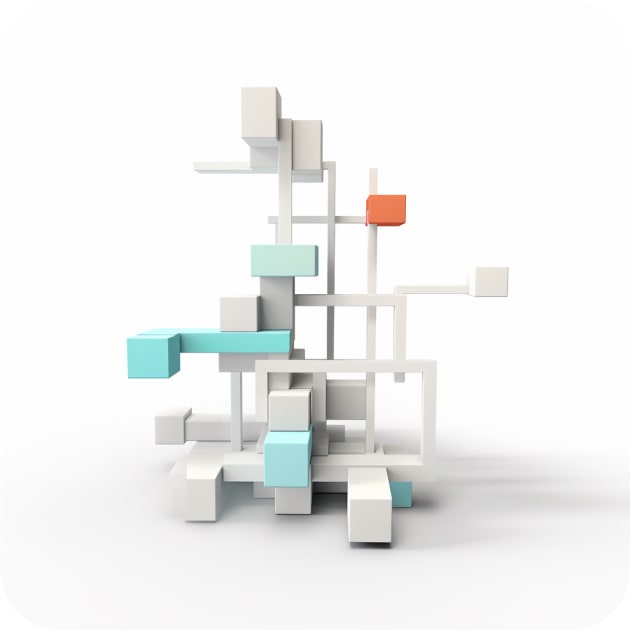
Monitor
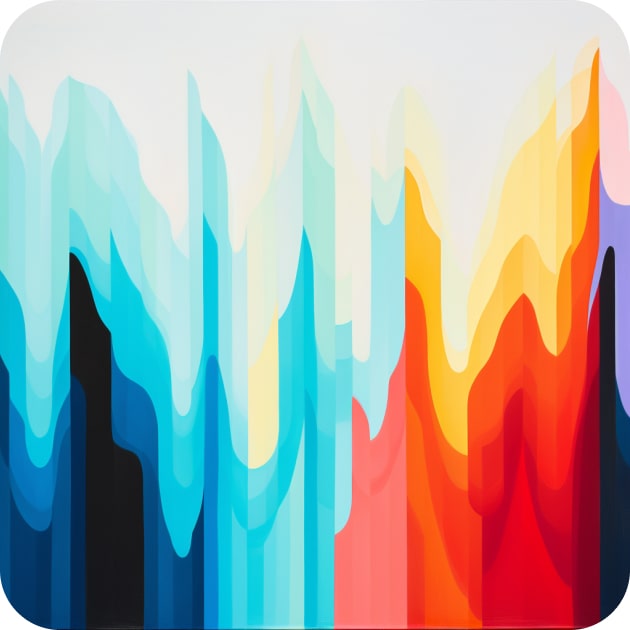
Parse
Partition
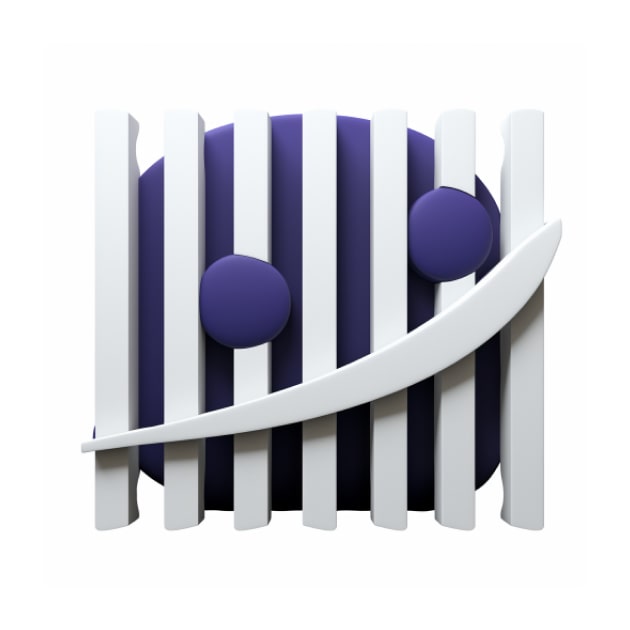
Prep
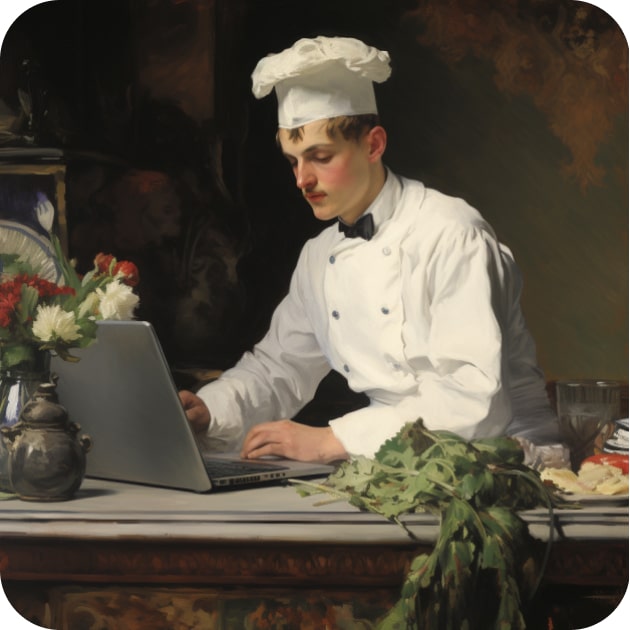
Preprocess
Primary Key
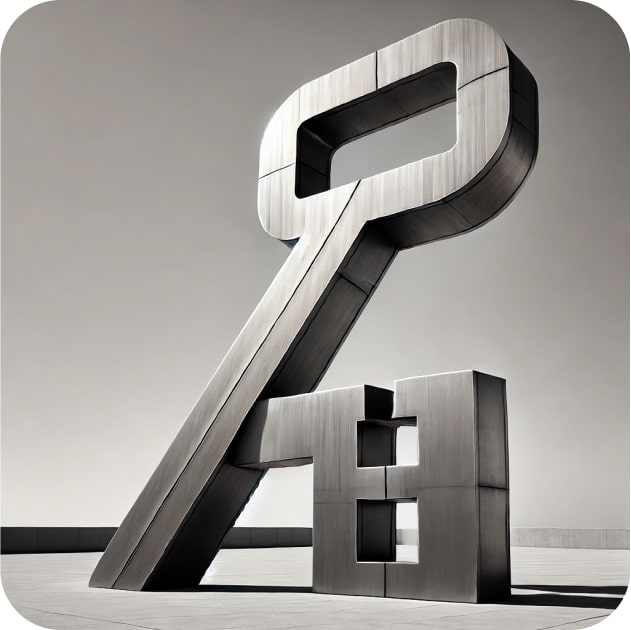
Replicate
Scaling
Schema Inference
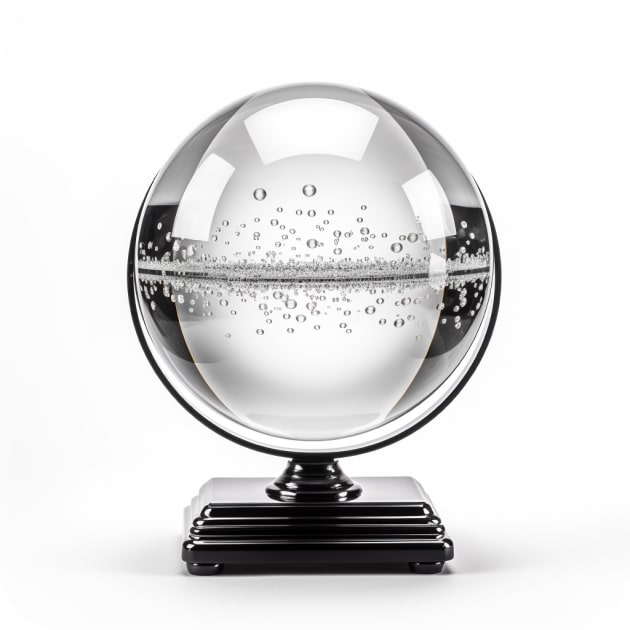
Schema Mapping
Secondary Index
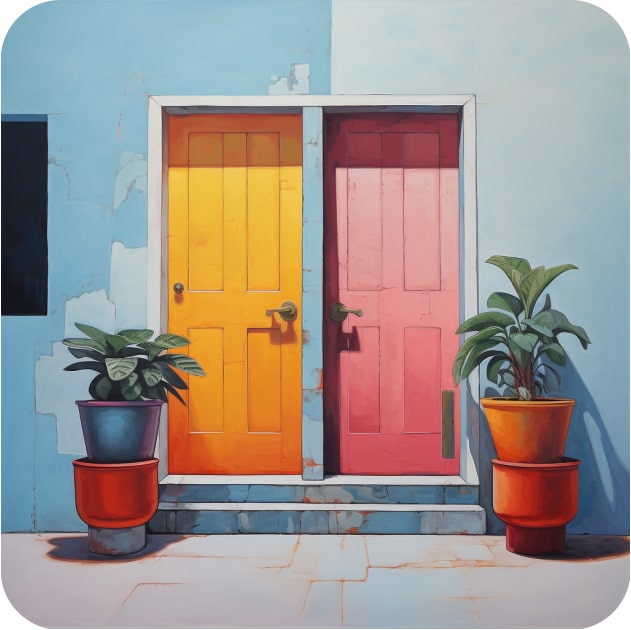
Software-defined Asset
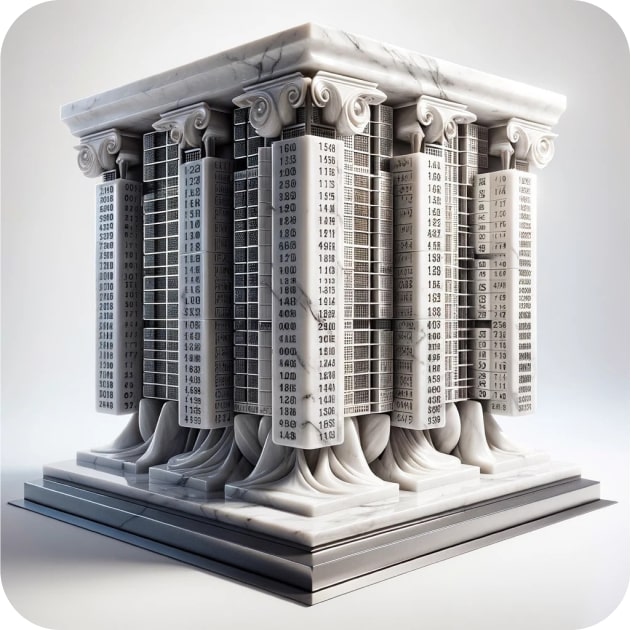
Synchronize
Validate
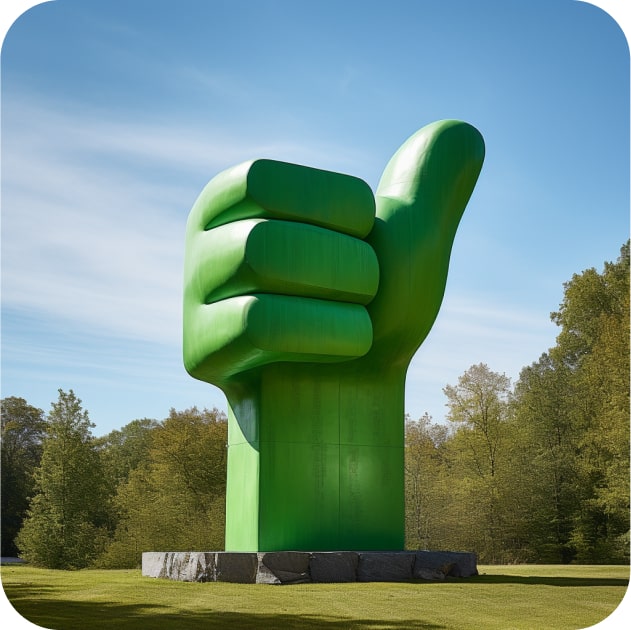
Version
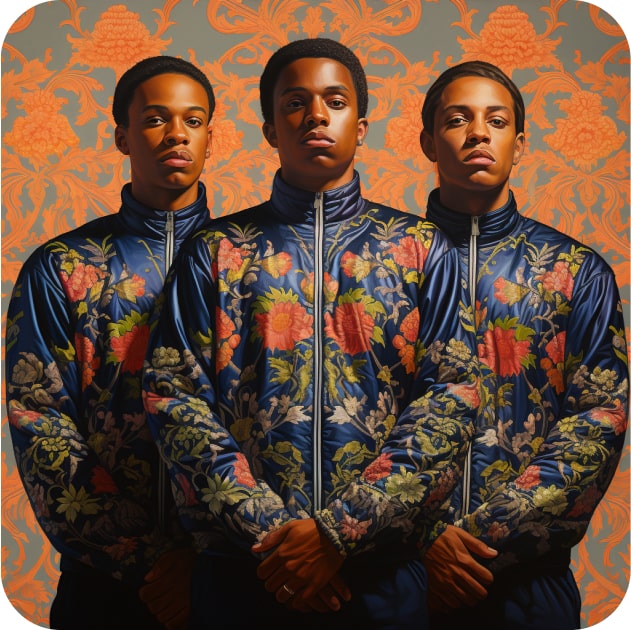