Data Deduplication
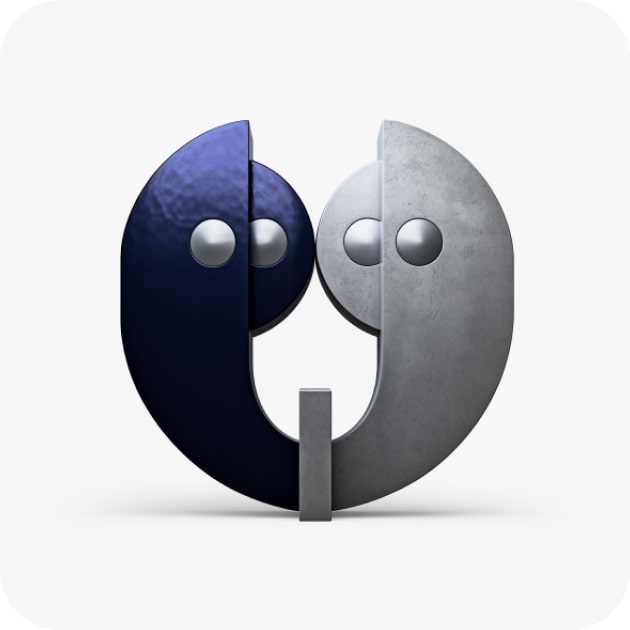
Data deduplication definition:
The presence of duplicates in your data can lead to incorrect analysis results and cause storage and processing inefficiencies, leading to increased costs.
There are several Python functions and techniques for de-duplicating data in regular programming, such as using hash functions, using set data structure, and comparing columns to identify duplicates. While traditional Python object lists and sets can be used for deduplicating data, they may not be the most efficient choice for large-scale data processing. This is because lists and sets require storing all data in memory, which can quickly become a bottleneck and slow down the processing.
For deduplicating large datasets, it's recommended to use specialized libraries and data structures designed for efficient processing, such as the pandas library in Python. Pandas has built-in functions like drop_duplicates()
that can easily handle large datasets. Additionally, distributed processing frameworks like Apache Spark and Dask can be used to process large datasets in a distributed and parallelized manner, which can further improve the performance of data deduplication.
Deduplicating data in Python
Please note that you must have the necessary Python libraries installed in your Python environment to run the code examples below.
Python provides several libraries and functions for de-duplicating data, such as:
Pandas: Pandas provides a drop_duplicates()
function that can be used to remove duplicate rows from a Pandas DataFrame. Given an input file data.csv
with 56 rows of which 10 are duplicates, the following code…
import pandas as pd
df = pd.read_csv('data.csv')
print(f"This dataframe has {len(df)} rows.")
df = df.drop_duplicates()
print(f"This dataframe now has {len(df)} rows.")
… might yield this output:
This dataframe has 56 rows.
This dataframe now has 44 rows.
Dask: Dask is a parallel computing library in Python that can be used for big data processing. Dask provides a drop_duplicates()
function that can be used to remove duplicates from a Dask DataFrame.
In the following example, the first line imports the dask.dataframe module as dd.
The second line reads in CSV files using the dd.read_csv() function, which returns a Dask DataFrame. The in the filename parameter data.csv is a wildcard character that matches any file with a name starting with "data" and ending with ".csv". If there are multiple files that match this pattern, Dask will concatenate them into a single dataframe.
import dask.dataframe as dd
df = dd.read_csv('data*.csv')
df = df.drop_duplicates()
The resulting dataframe will be distributed across multiple workers, which can perform computations in parallel.
PySpark: PySpark is the Python API for Apache Spark, a big data processing framework. PySpark provides a dropDuplicates()
function that can be used to remove duplicates from a PySpark DataFrame.
Given the input data.csv
file:
name,zip,amount
James,12345,99
Bob,19876,23
Claire,212565,124
Bob,19876,23
Claire,212565,123
James,12345,99
We can use PySpark to deduplicate the data:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("De-duplication").getOrCreate()
df = spark.read.csv("data.csv", header=True, inferSchema=True)
df = df.dropDuplicates()
df.show()
The df.show()
command will provide us with the output:
+------+------+------+
| name| zip|amount|
+------+------+------+
| James| 12345| 99|
| Bob| 19876| 23|
|Claire|212565| 124|
|Claire|212565| 123|
+------+------+------+
Note that the lines have to be identical across all columns to be considered a duplicate, which is why the last two lines remain in the dataframe.
Append
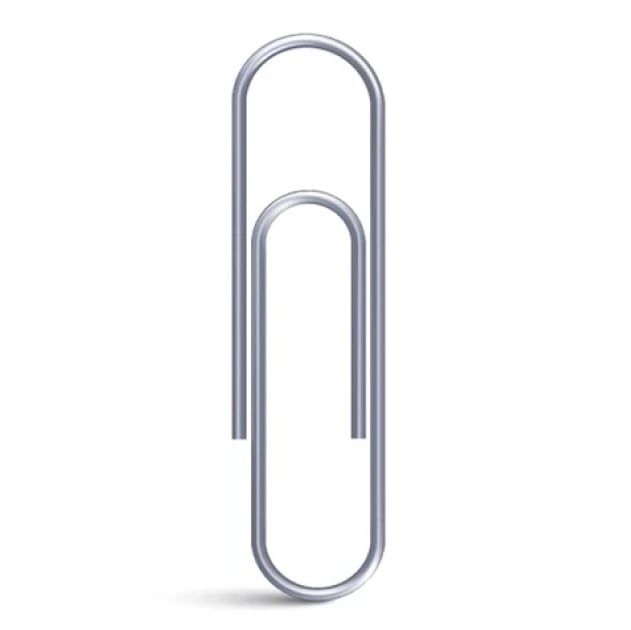
Archive
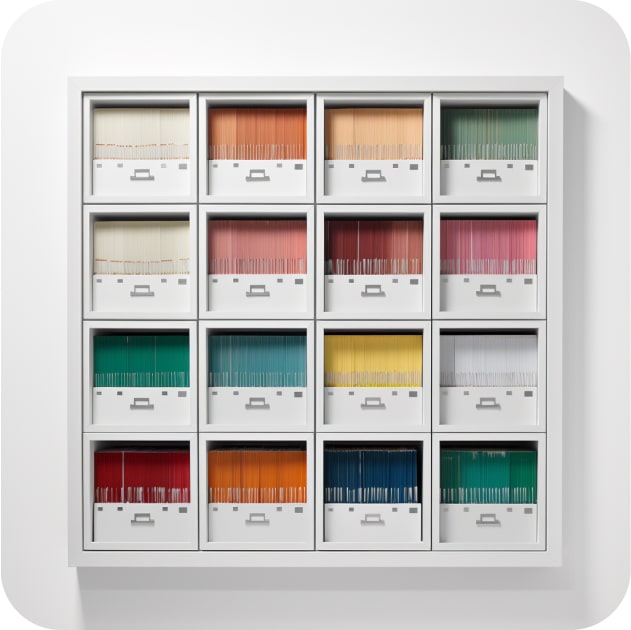
Augment
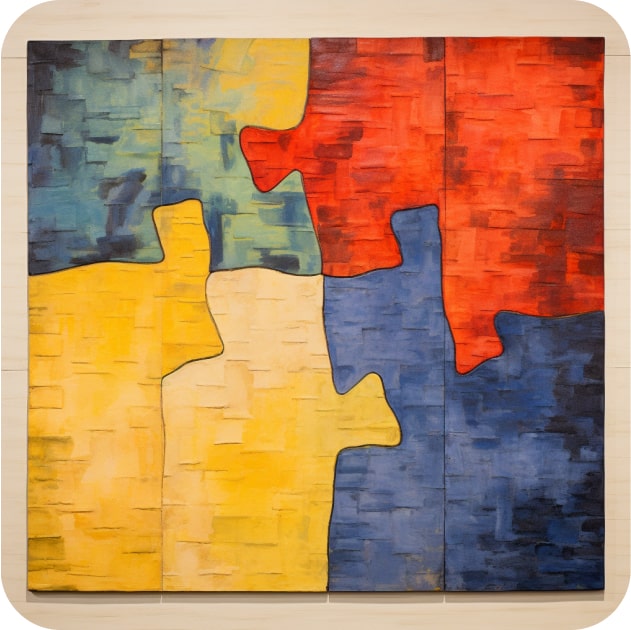
Auto-materialize
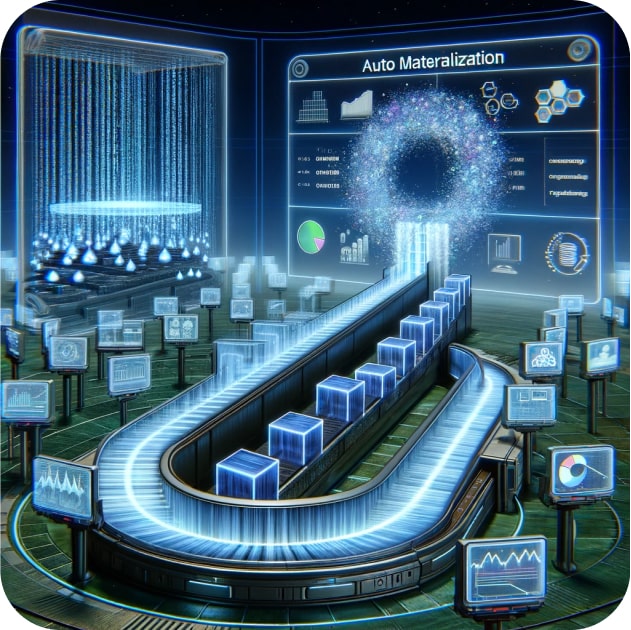
Backup
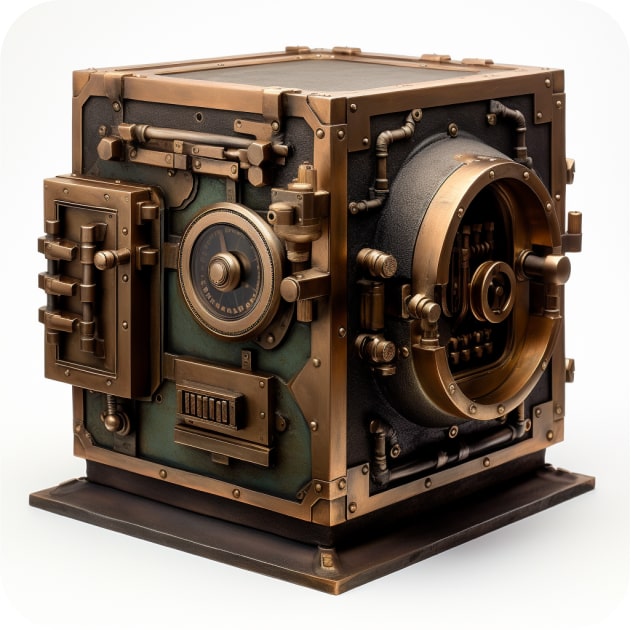
Batch Processing
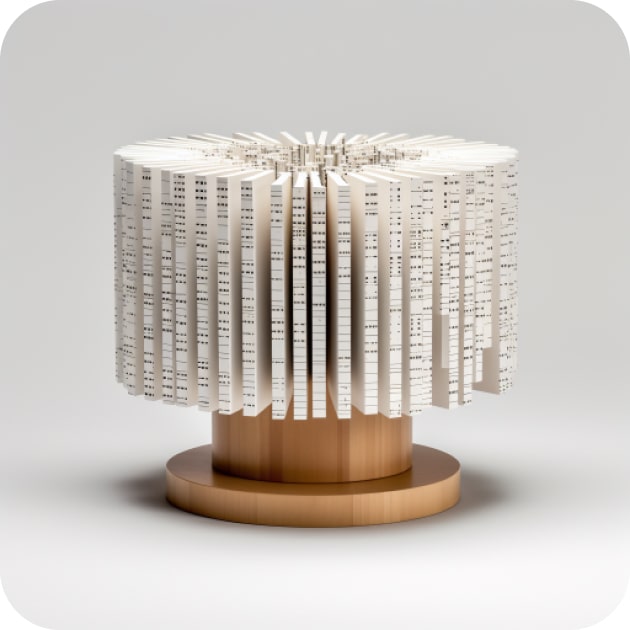
Cache
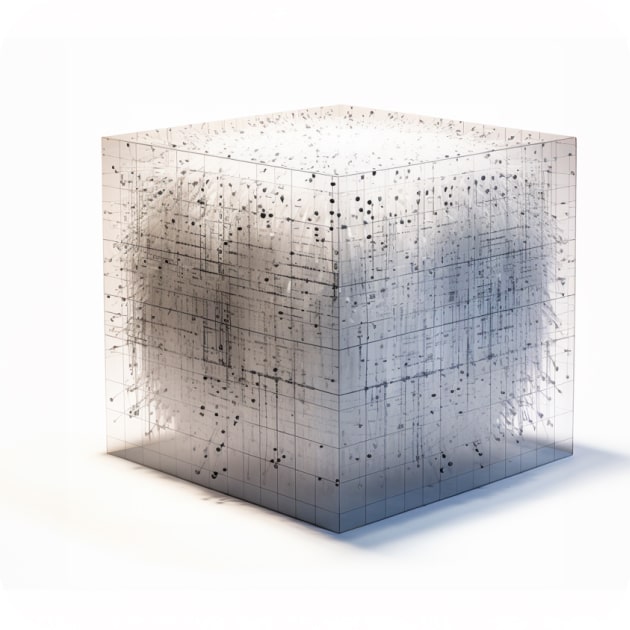
Categorize
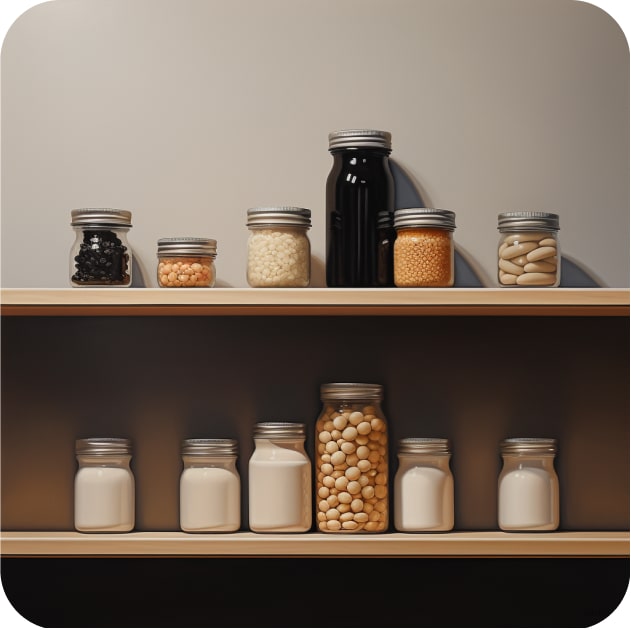
Deserialize
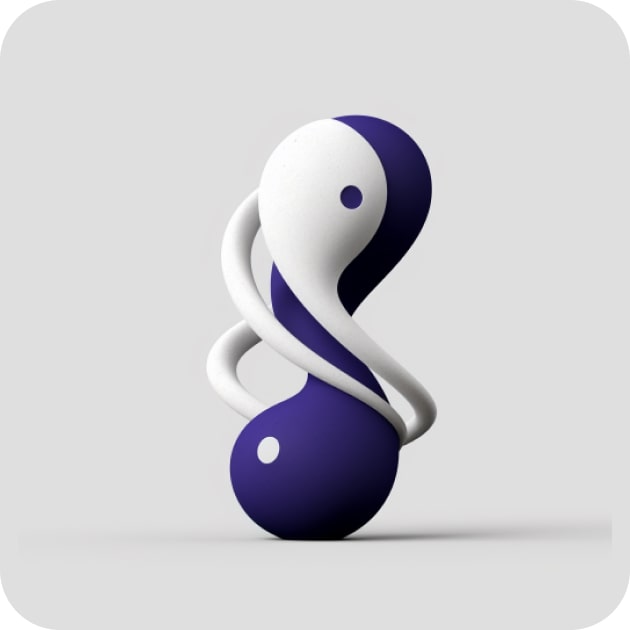
Dimensionality
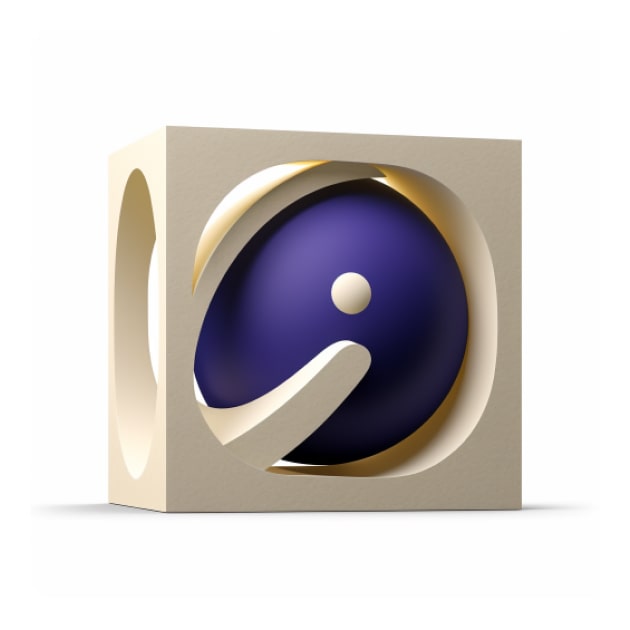
Encapsulate
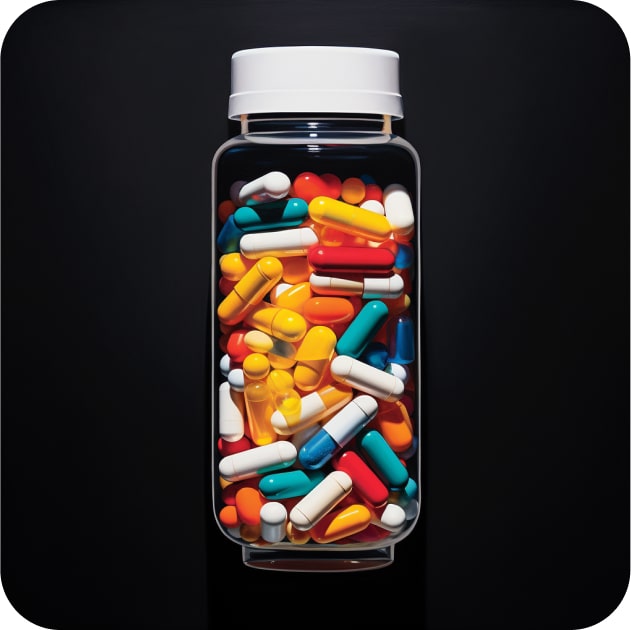
Enrich
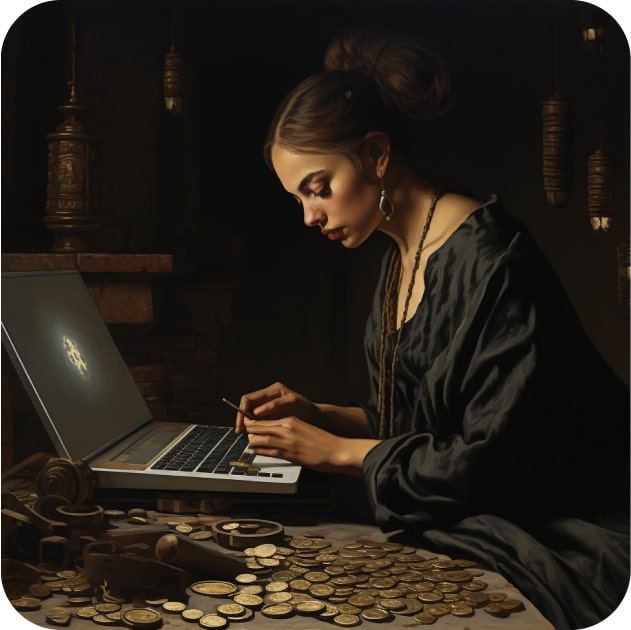
Export
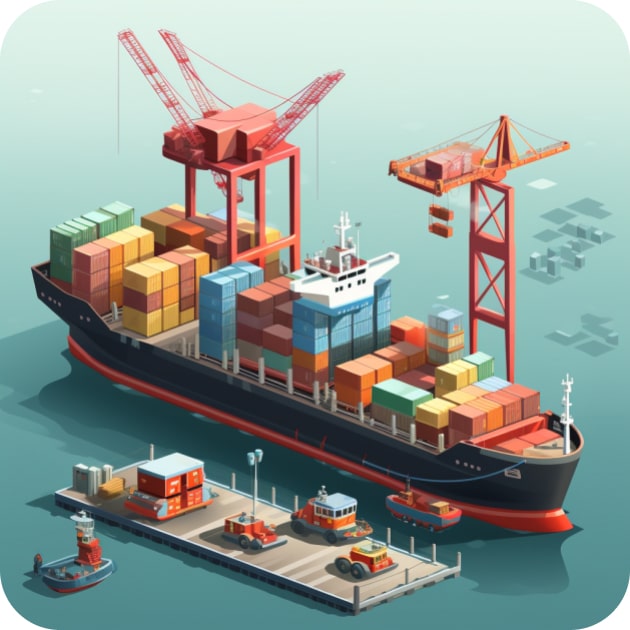
Graph Theory
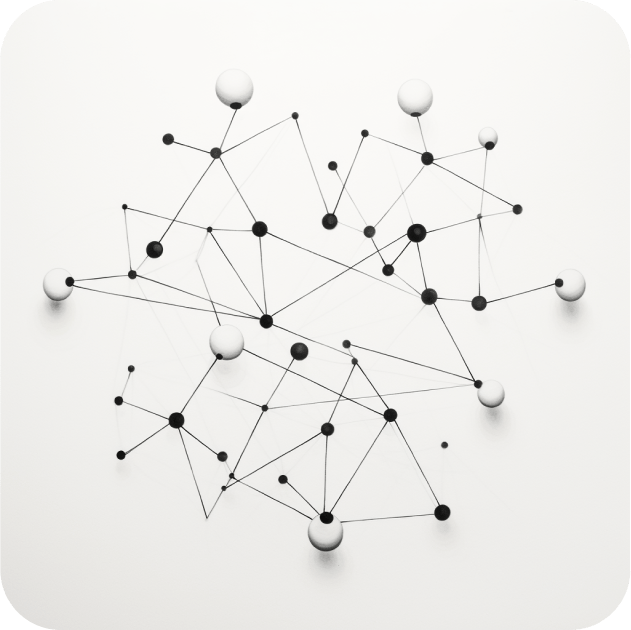
Idempotent
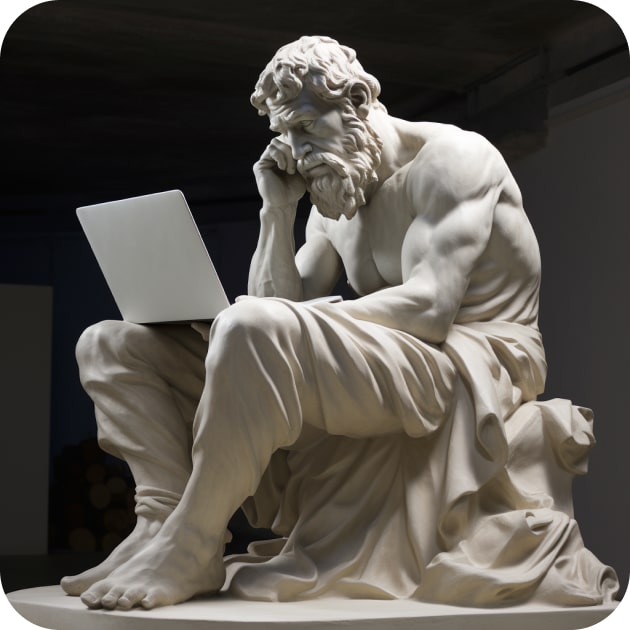
Index
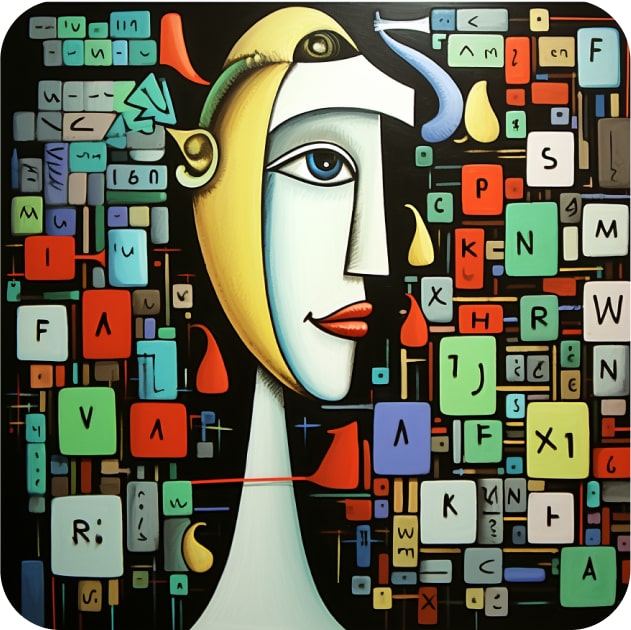
Integrate
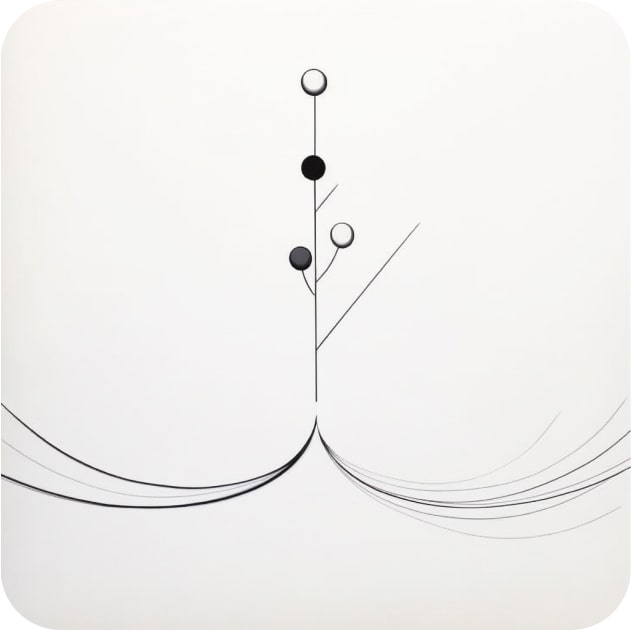
Lineage
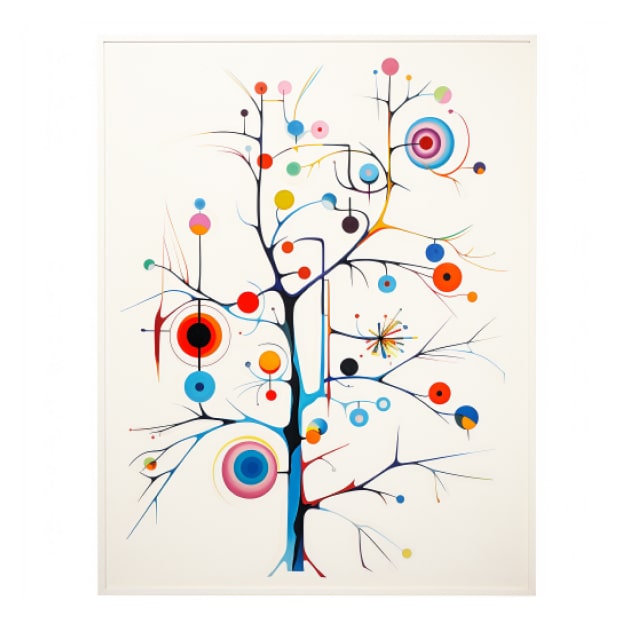
Linearizability
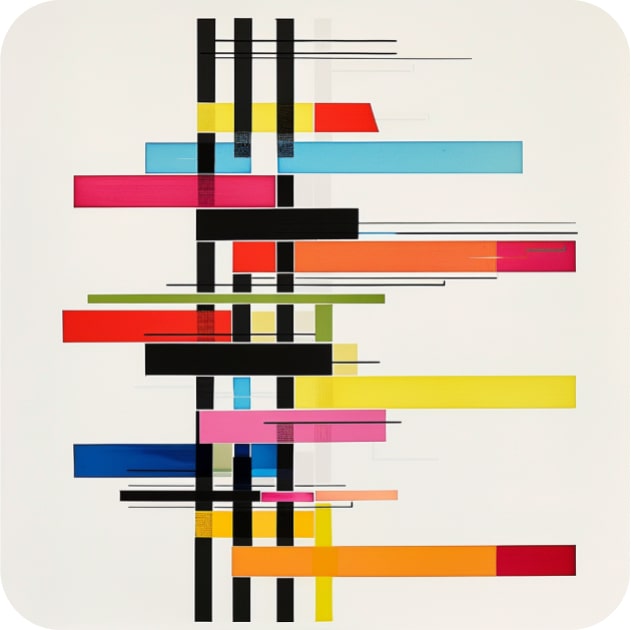
Materialize
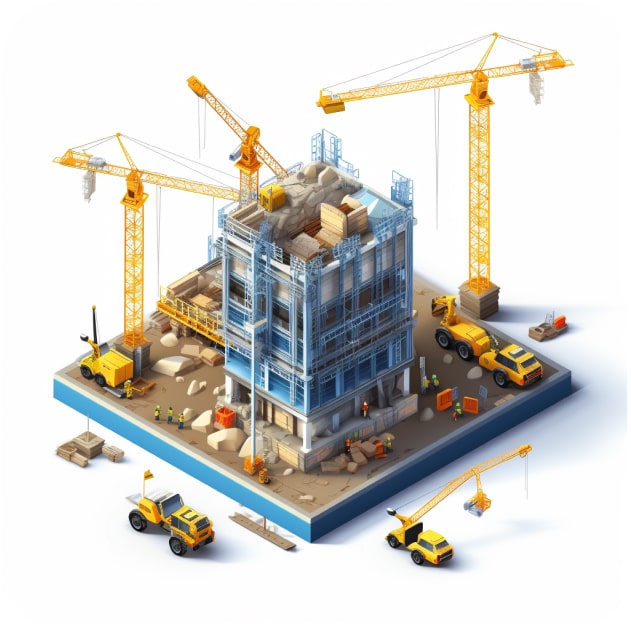
Memoize
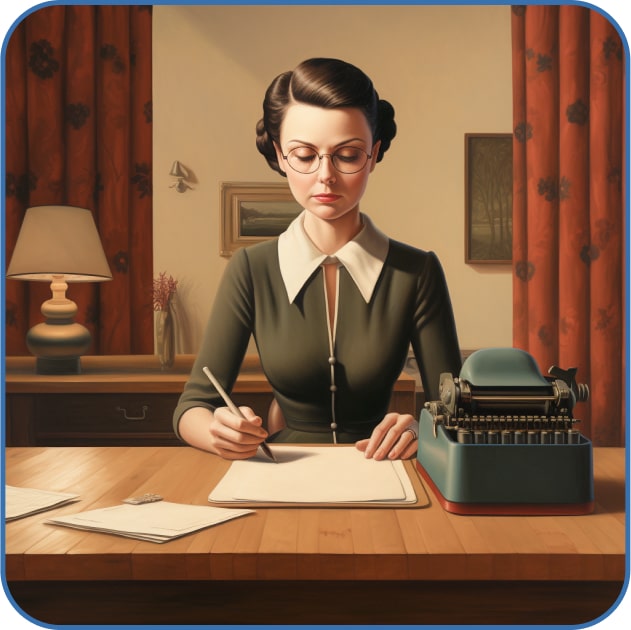
Merge
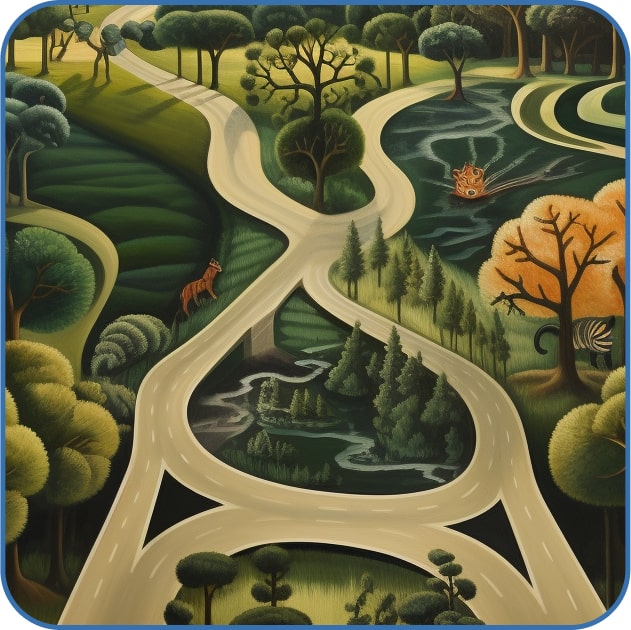
Model
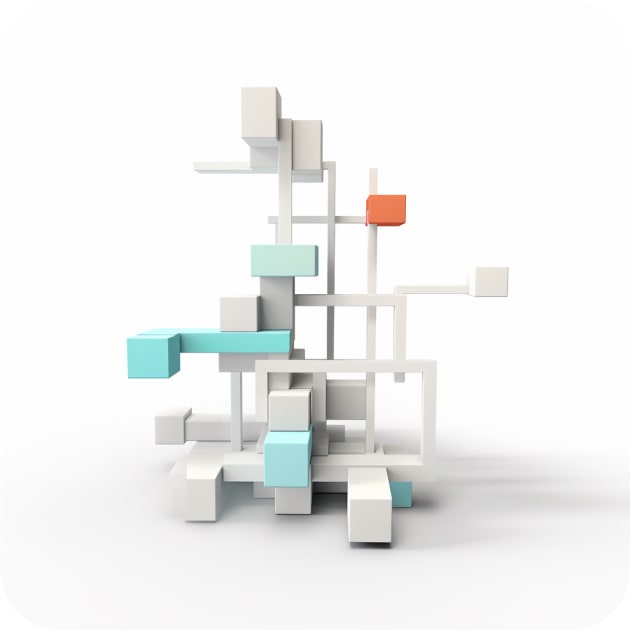
Monitor
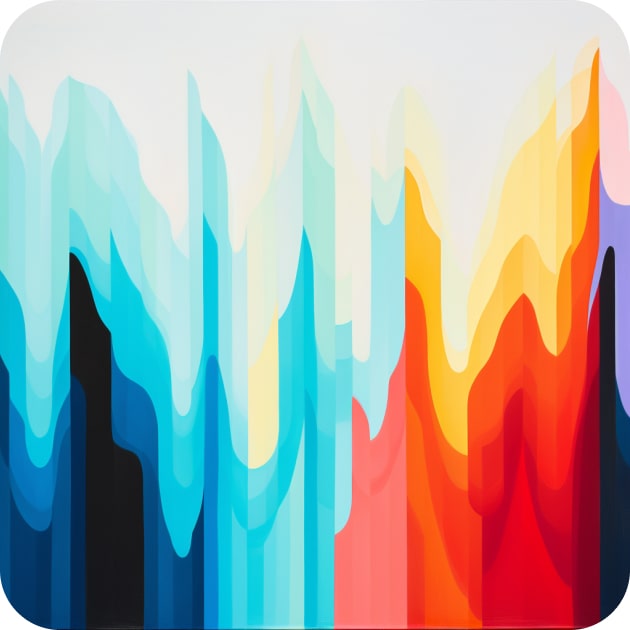
Named Entity Recognition
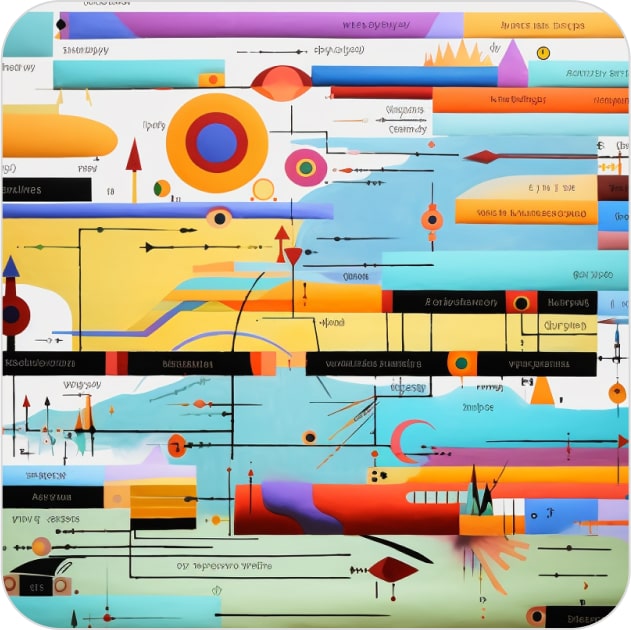
Parse
Partition
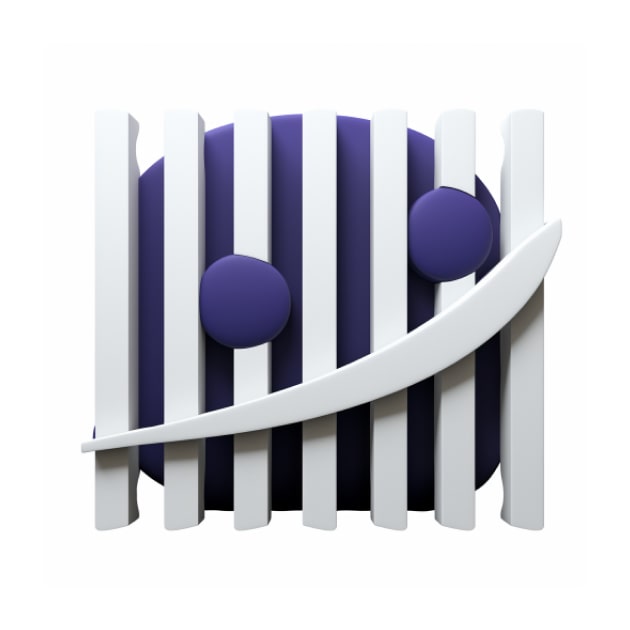
Prep
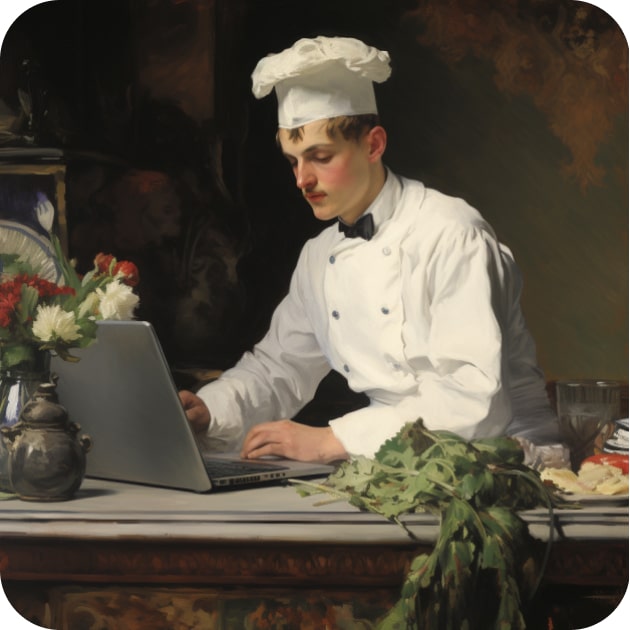
Preprocess
Replicate
Scaling
Schema Inference
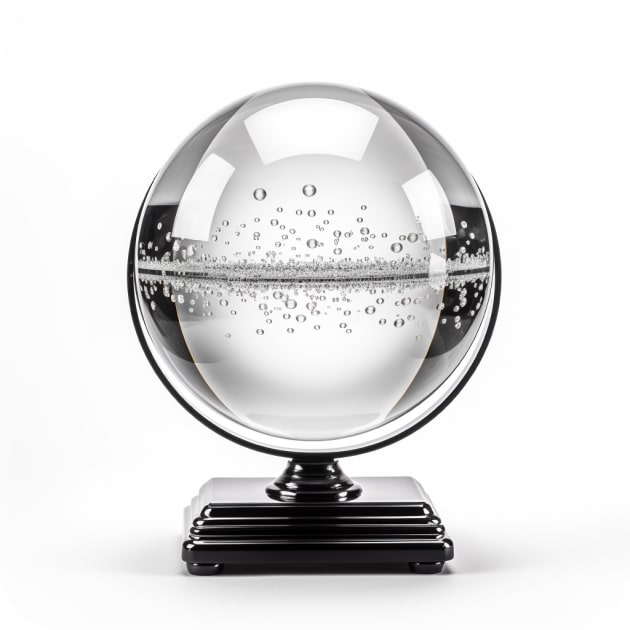
Schema Mapping
Secondary Index
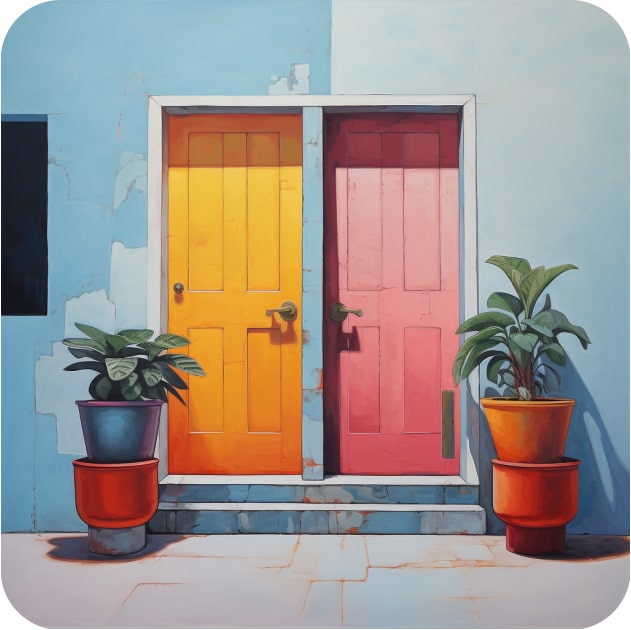
Software-defined Asset
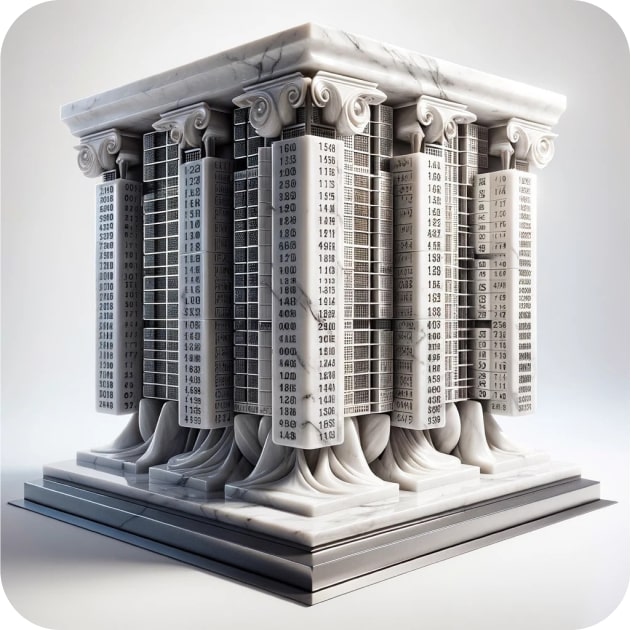
Synchronize
Validate
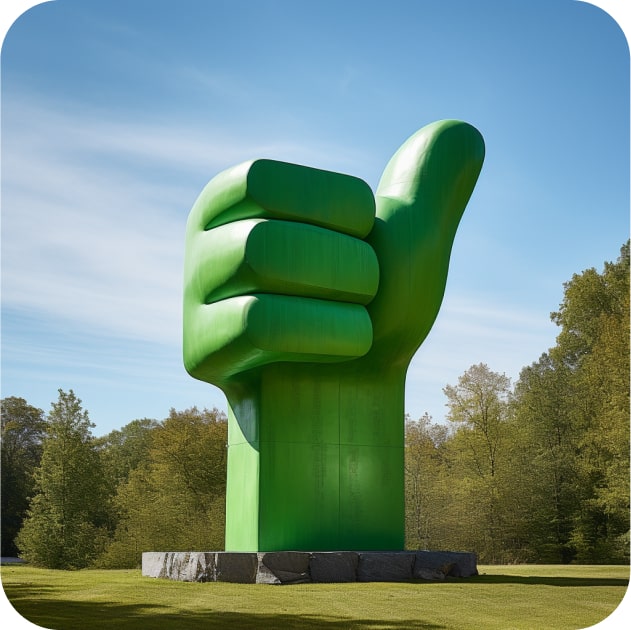
Version
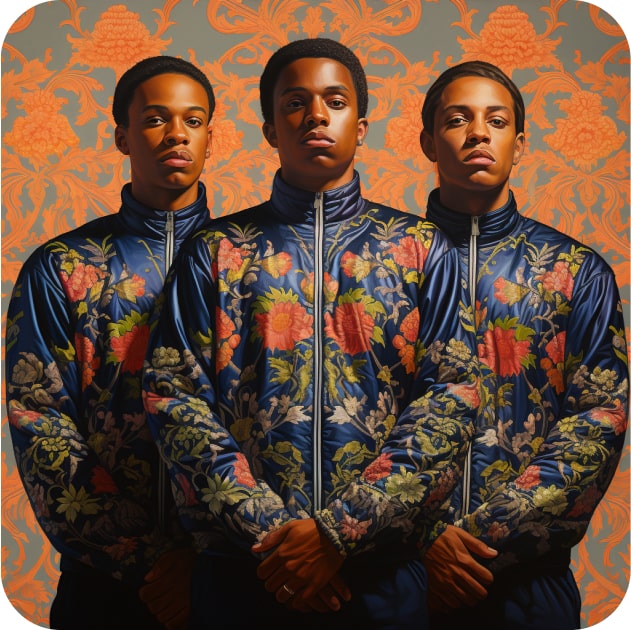