Data Integration
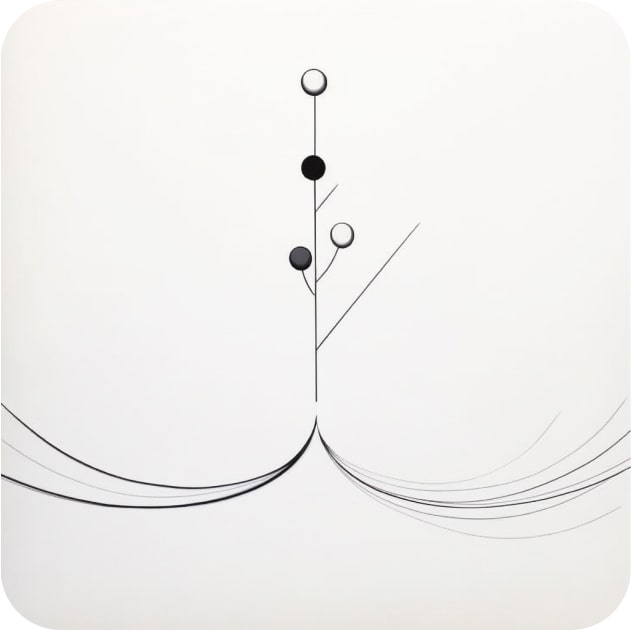
Data integration definition:
Data integration refers to the process of combining data from multiple sources into a single, unified dataset. This is an important step in many data analysis and machine learning projects, as data is often stored in different formats and locations.
Integration involves several steps, including data cleaning, transformation, and normalization to ensure that the data from different sources can be combined. This can involve resolving naming conflicts, converting data types, and removing duplicate data.
Data integration example using Python and PySpark:
Please note that you need to have the necessary Python libraries installed in your Python environment to run the following code examples.
Python provides several libraries and tools for integrating data, such as pandas, NumPy, and Apache Spark. These tools can help with data cleaning, transformation, and normalization, as well as providing a range of functions and algorithms for analyzing and visualizing the integrated dataset.
For example, let's say you are working on a project to analyze customer behavior for an e-commerce site. You might need to integrate data from several sources, including website logs, customer transaction data, and social media activity. Using Python, you can use tools such as pandas and NumPy to clean and transform the data, and then integrate it into a single dataset for analysis.
Here's an example of data integration using Apache Spark:
Let's say we have two data sources, customers and orders, and we want to integrate them based on the customer_id
field. We can load both data sources into Spark DataFrames, and then join them on the customer_id
field:
from pyspark.sql import SparkSession
# Create a SparkSession
spark = SparkSession.builder.appName("DataIntegration").getOrCreate()
# Load the customers data into a DataFrame
customers_df = spark.read.csv("customers.csv", header=True, inferSchema=True)
# Load the orders data into a DataFrame
orders_df = spark.read.csv("orders.csv", header=True, inferSchema=True)
# Join the two DataFrames on the customer_id field
joined_df = customers_df.join(orders_df, "customer_id")
# Show the resulting integrated data
joined_df.show()
In this example, customers.csv might look like this:
customer_id,name,email
1,Alice,alice@example.com
2,Bob,bob@example.com
3,Charlie,charlie@example.com
And orders.csv might look like this:
order_id,customer_id,product,quantity
1001,1,Widget,3
1002,1,Gadget,1
1003,2,Widget,5
1004,3,Thingy,2
The resulting joined_df
DataFrame would look like this:
+-----------+------+-----------------+-------+---------+
|customer_id| name| email|order_id| product|
+-----------+------+-----------------+-------+---------+
| 1| Alice|alice@example.com| 1001| Widget|
| 1| Alice|alice@example.com| 1002| Gadget|
| 2| Bob| bob@example.com| 1003| Widget|
| 3|Charlie|charlie@example.com| 1004|Thingy|
+-----------+------+-----------------+-------+---------+
This integrated DataFrame combines the data from both sources into a single dataframe based on the customer_id
field. We are now set up to perform further analysis or transformations on this integrated data using Apache Spark.
Append
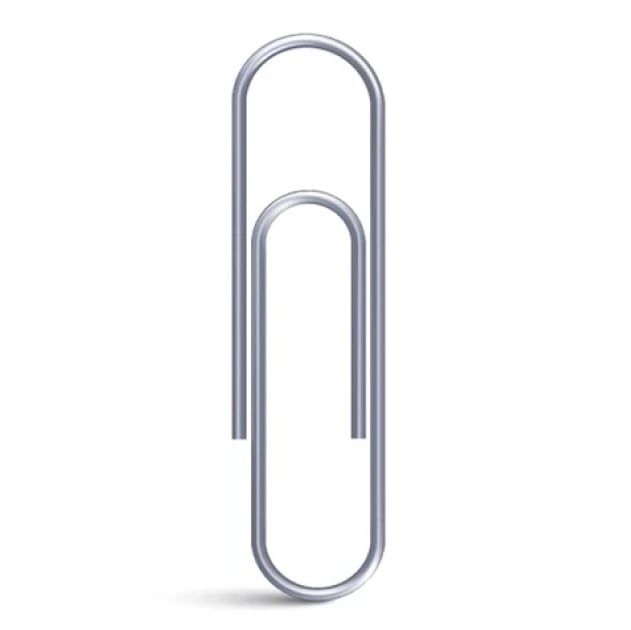
Archive
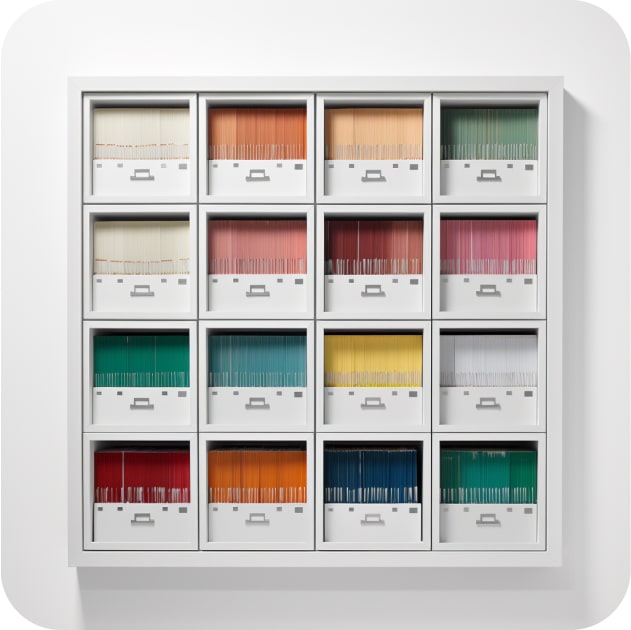
Augment
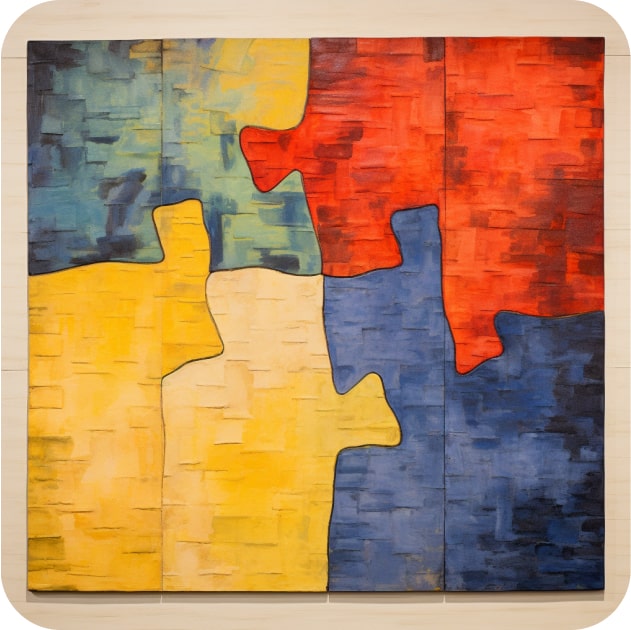
Auto-materialize
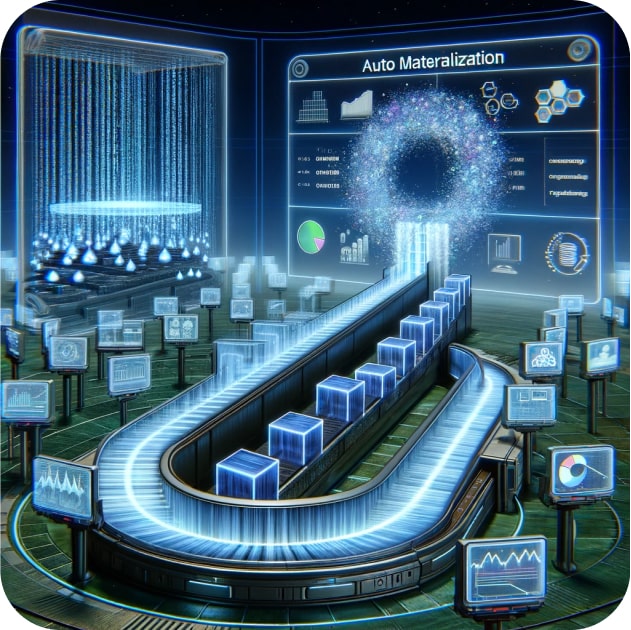
Backup
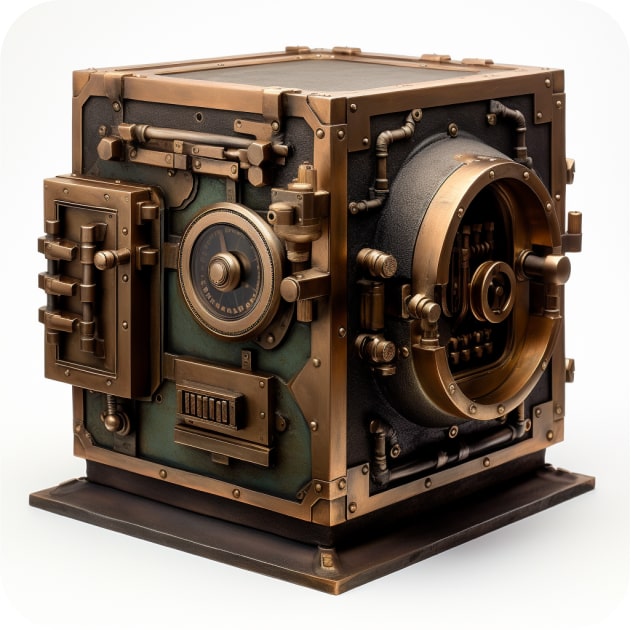
Batch Processing
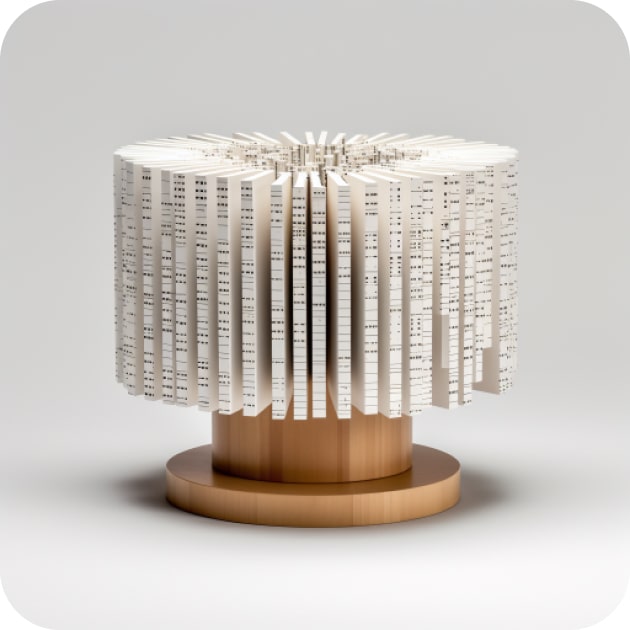
Cache
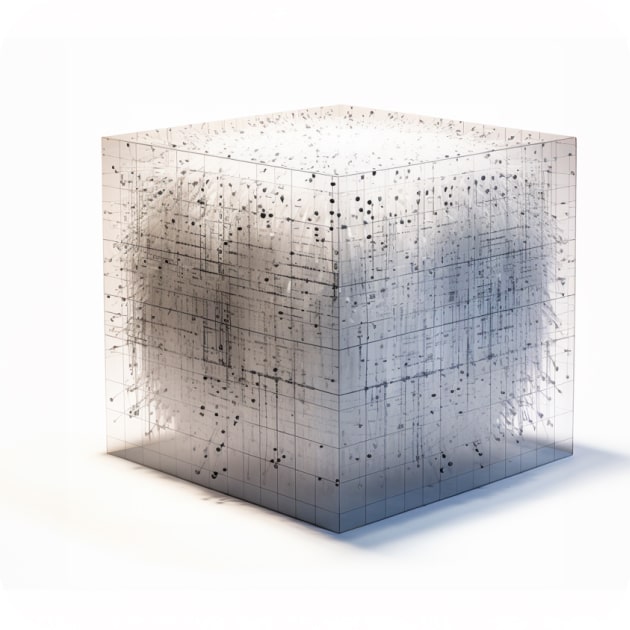
Categorize
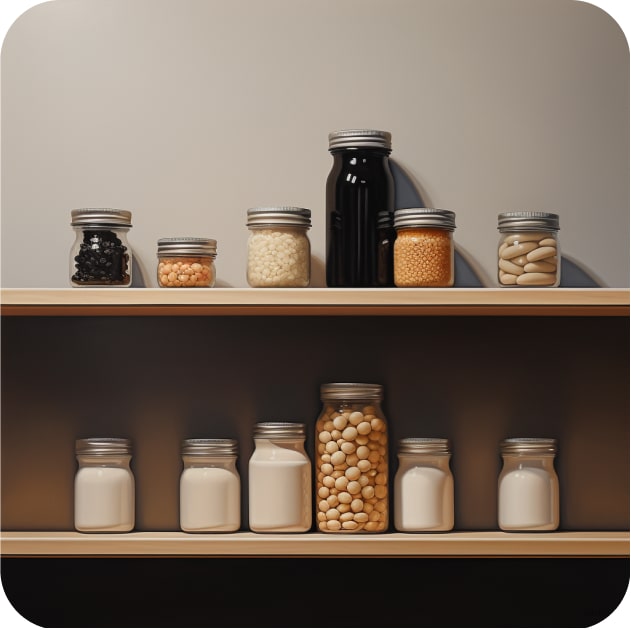
Deduplicate
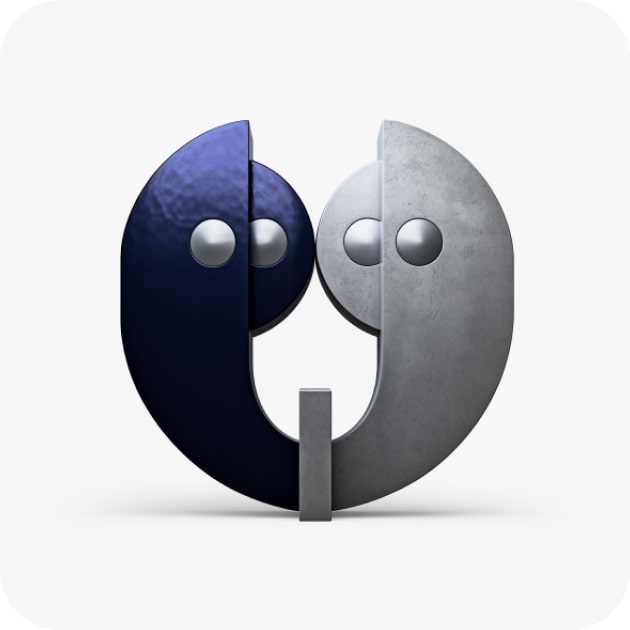
Deserialize
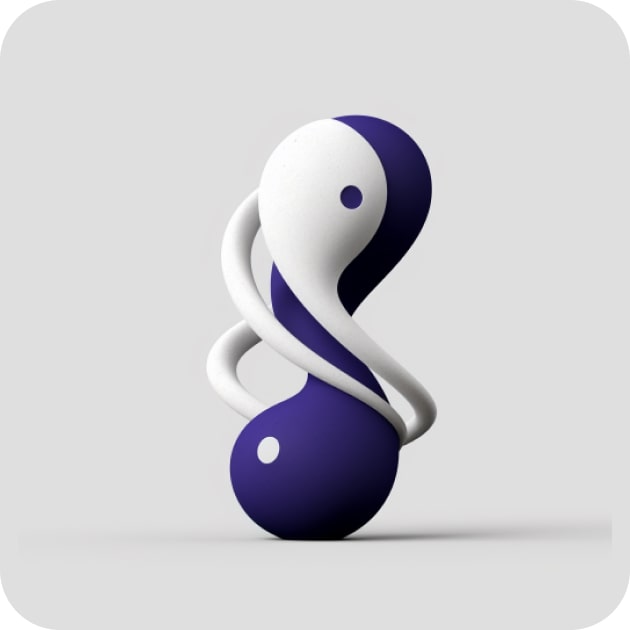
Dimensionality
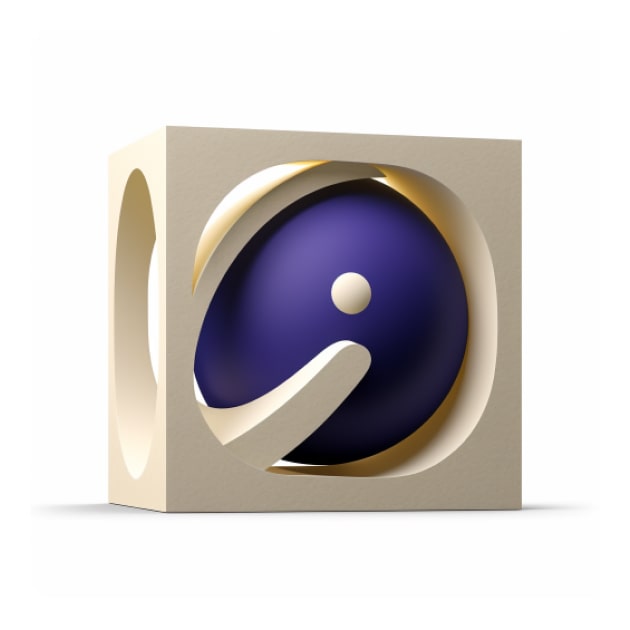
Encapsulate
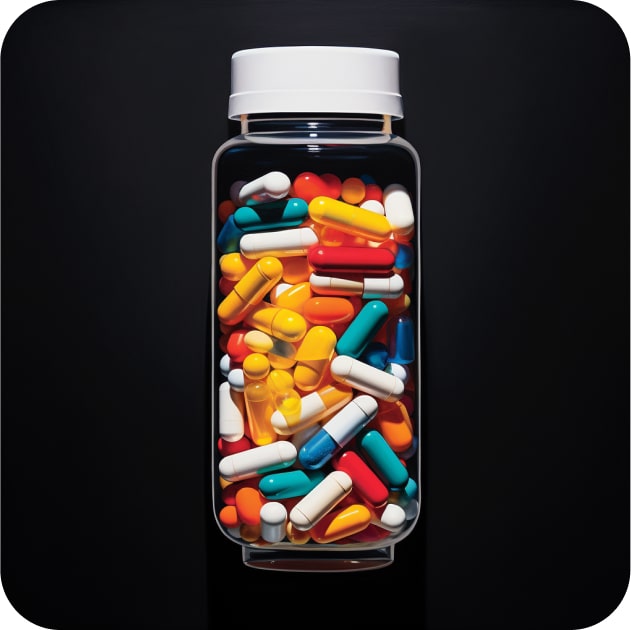
Enrich
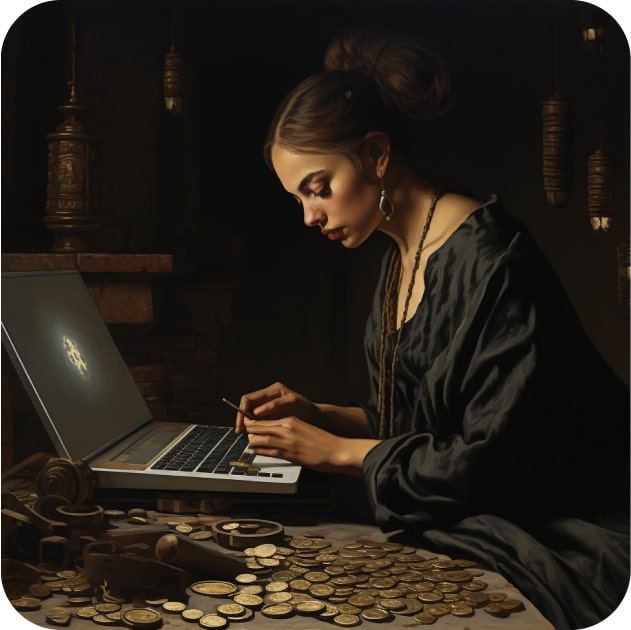
Export
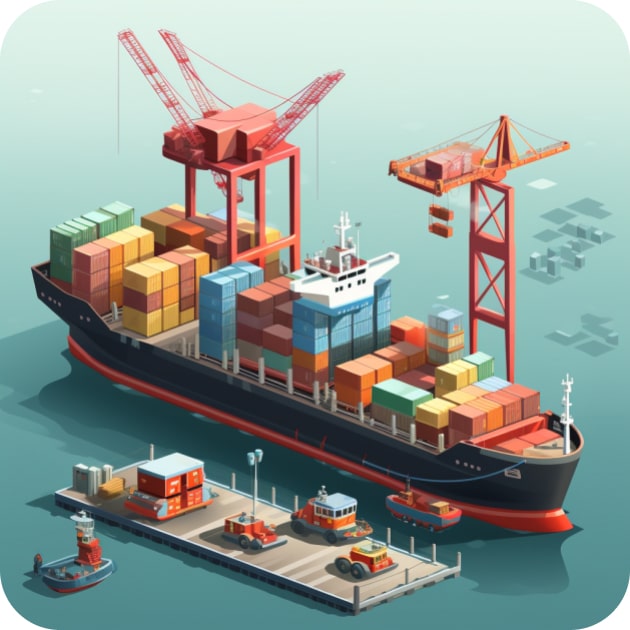
Graph Theory
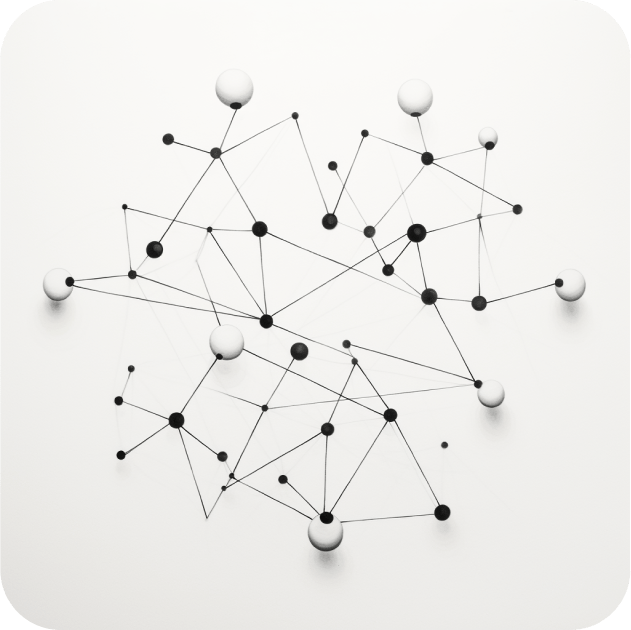
Idempotent
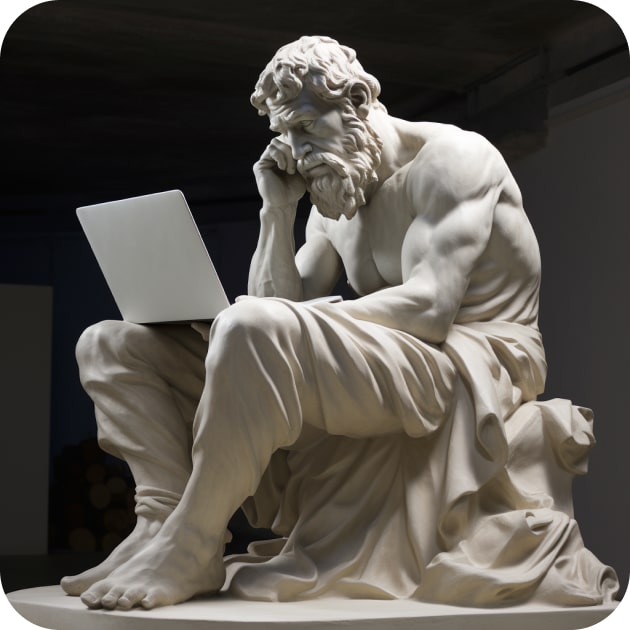
Index
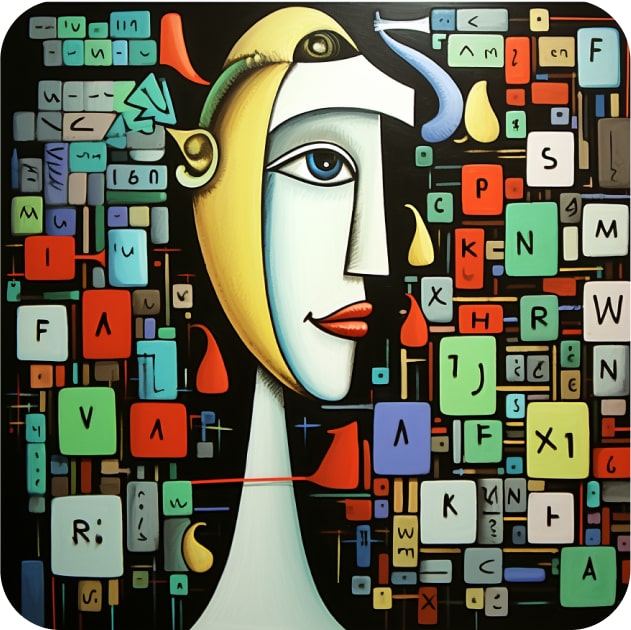
Lineage
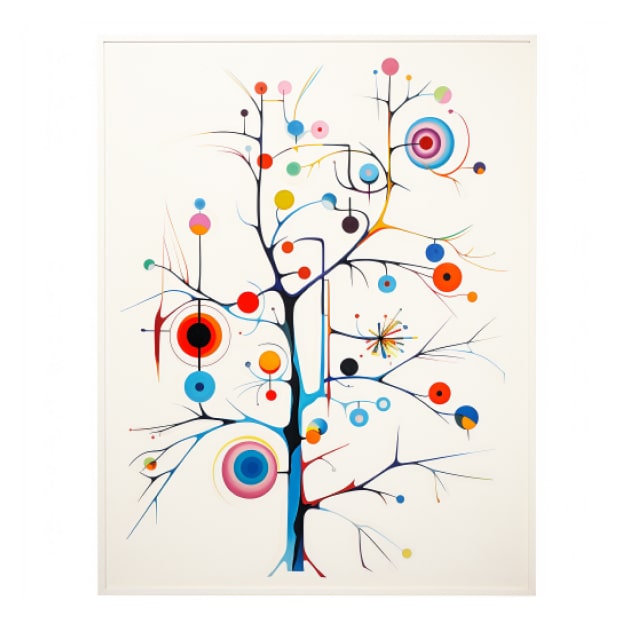
Linearizability
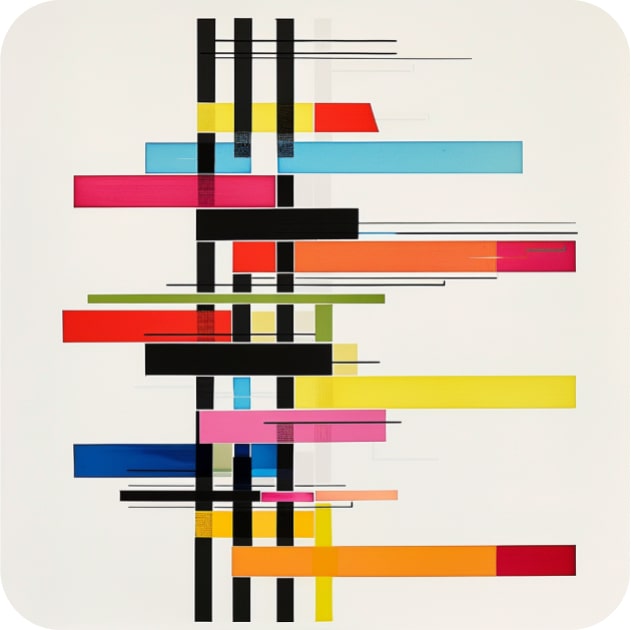
Materialize
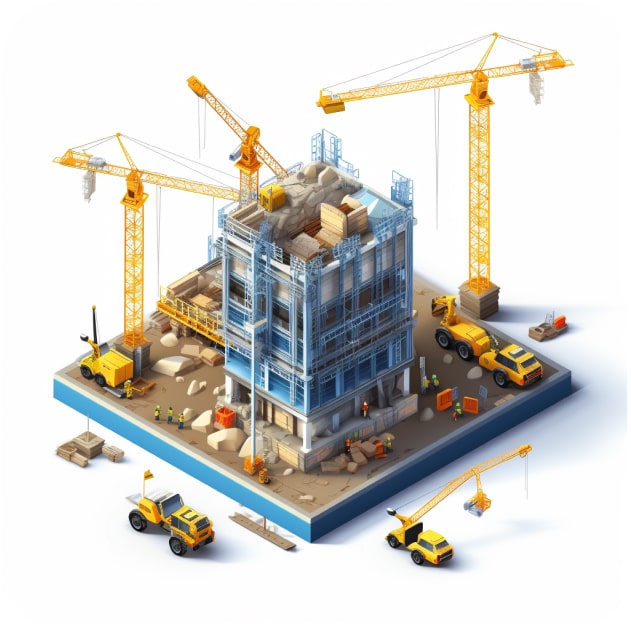
Memoize
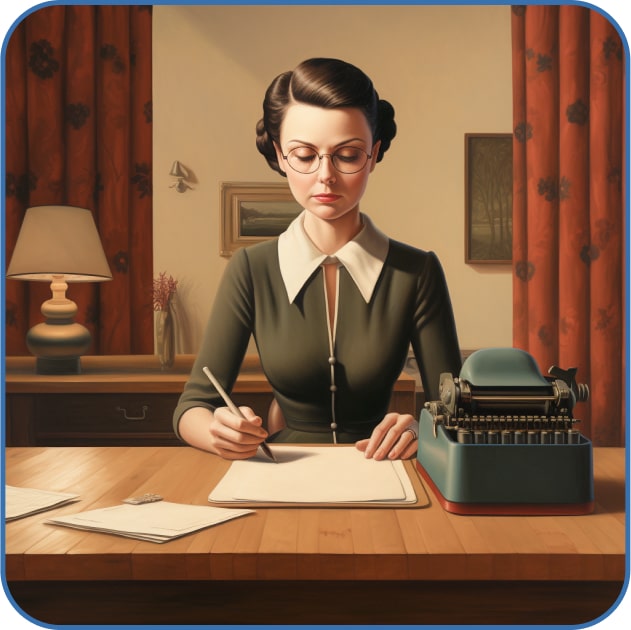
Merge
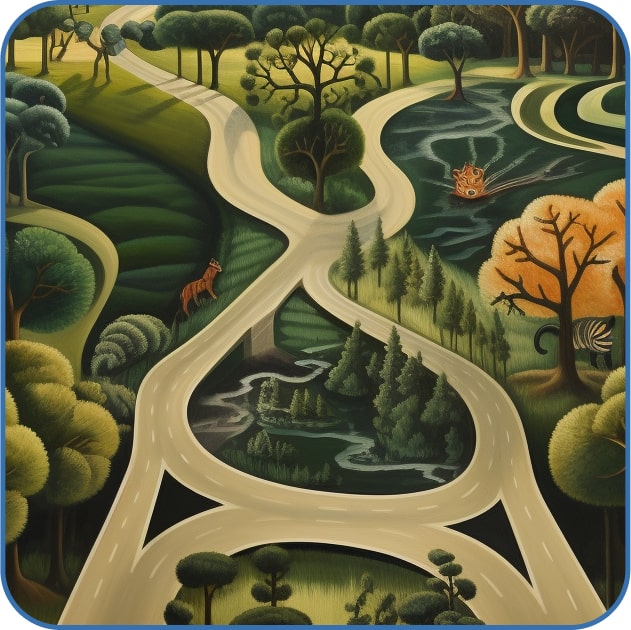
Model
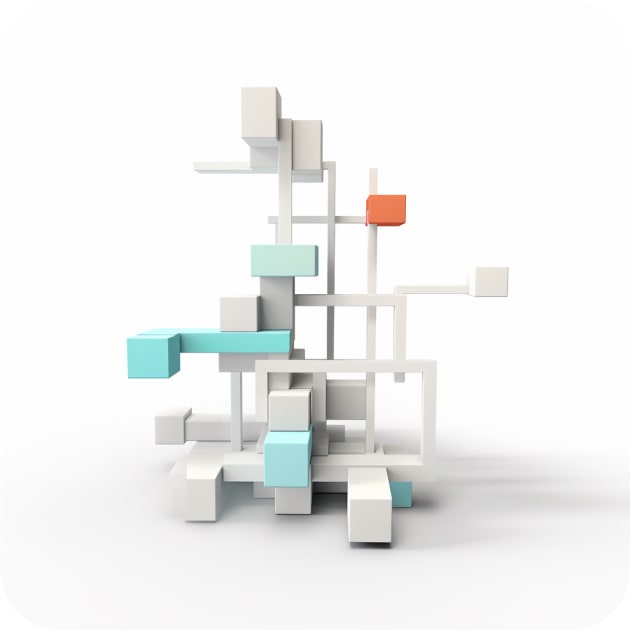
Monitor
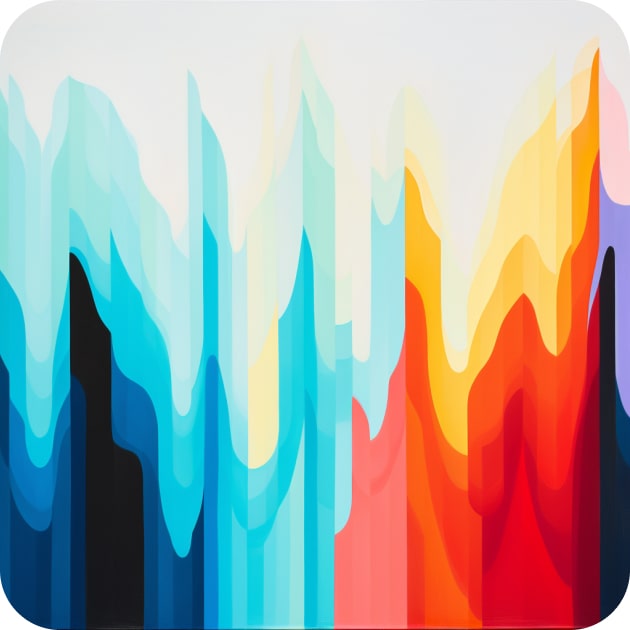
Named Entity Recognition
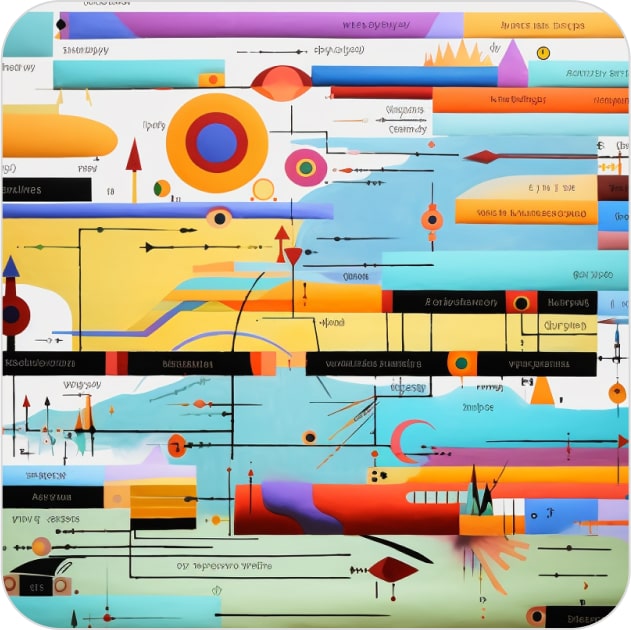
Parse
Partition
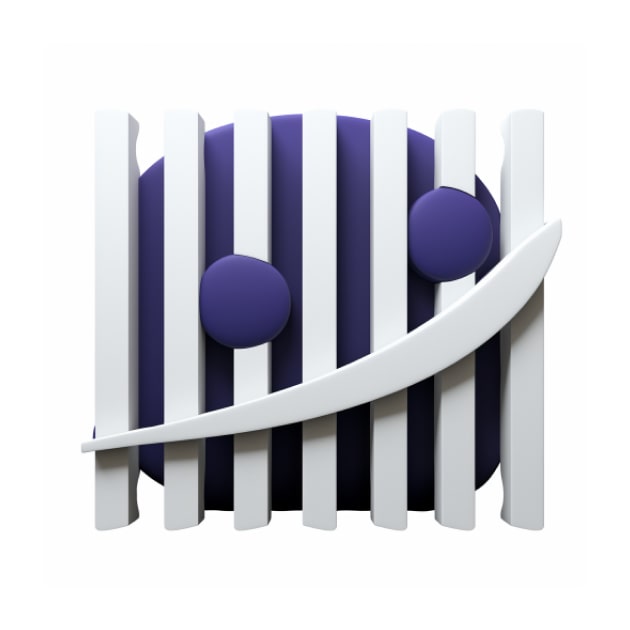
Prep
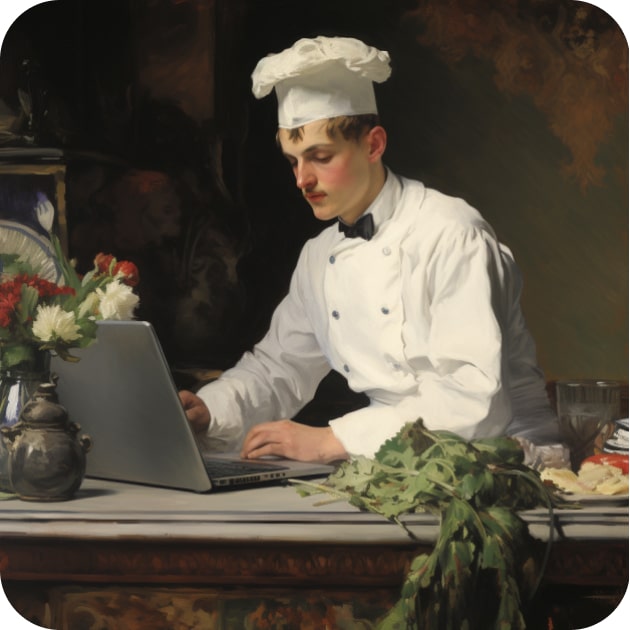
Preprocess
Replicate
Scaling
Schema Inference
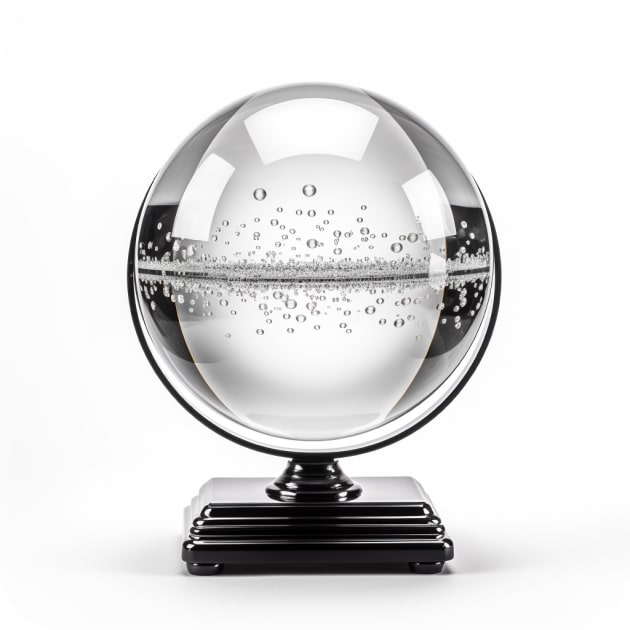
Schema Mapping
Secondary Index
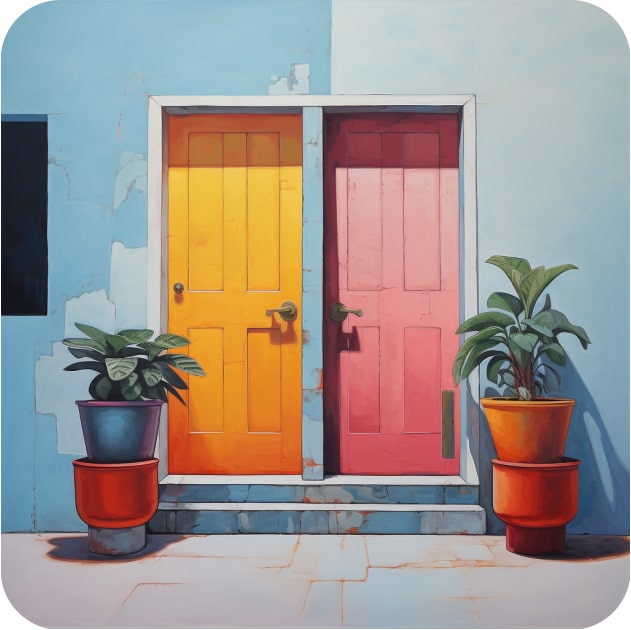
Software-defined Asset
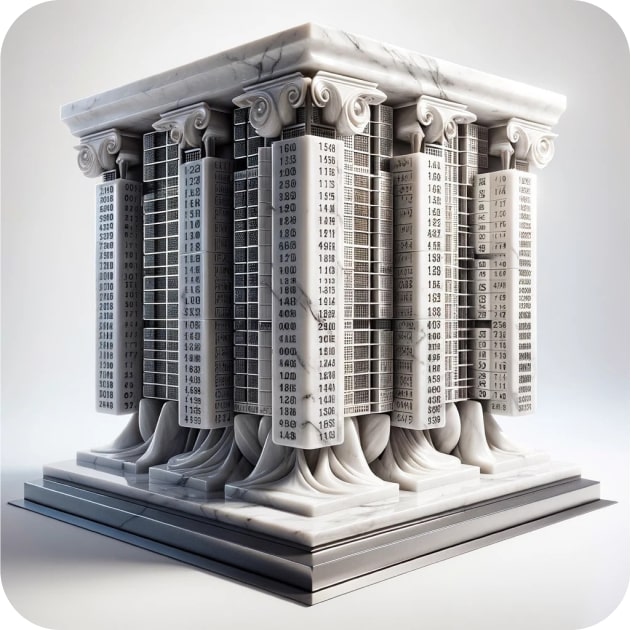
Synchronize
Validate
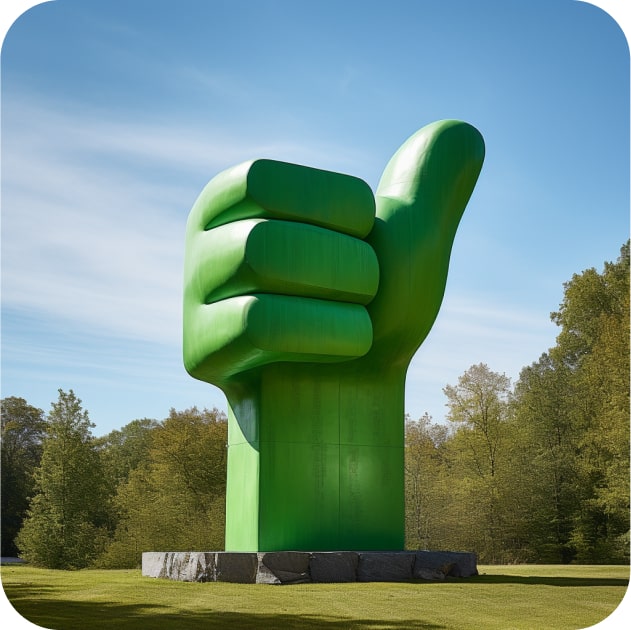
Version
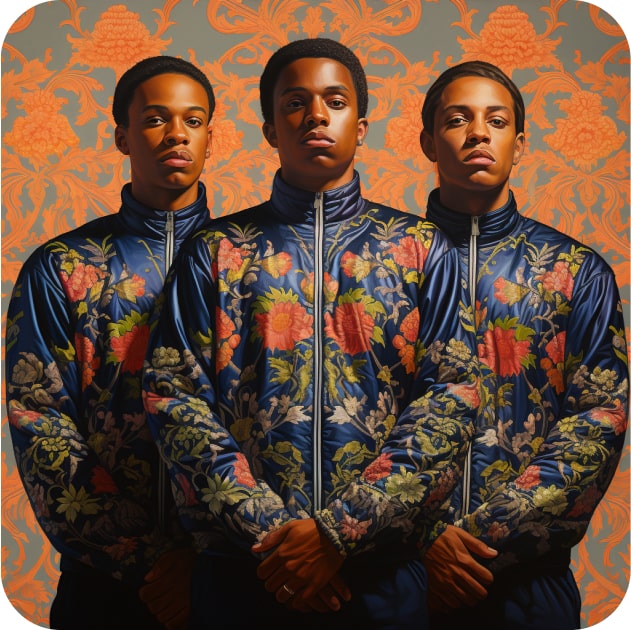