Dagster Data Engineering Glossary:
Race Conditions in Data Engineering
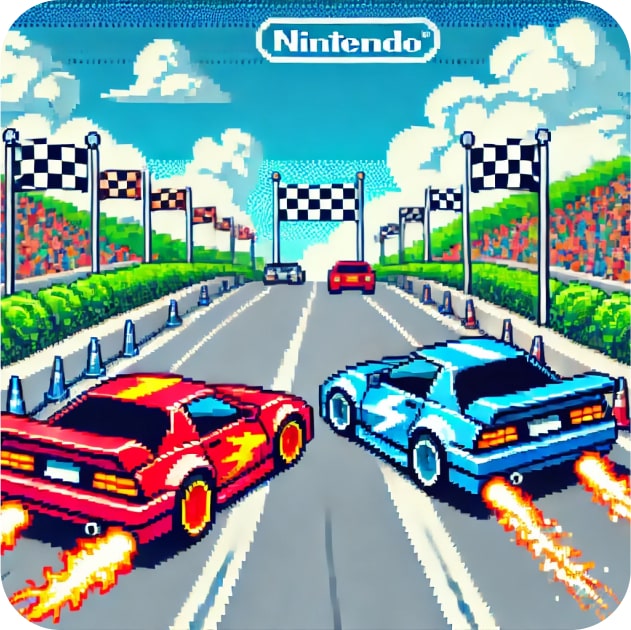
- Race Condition Definition
- How Dagster Helps Avoid Race Conditions
- Race Conditions: An Example in Python
Race Condition Definition:
In data engineering, a "race condition" refers to a situation where the behavior of a system or application depends on the sequence or timing of uncontrollable events such as the order in which threads or processes execute. This can lead to unpredictable and erroneous outcomes because the system does not properly handle the concurrency of operations.
Here are some key aspects of race conditions in data engineering:
Concurrency Issues: Race conditions often occur in concurrent systems where multiple processes or threads access shared resources (such as databases, files, or variables) simultaneously. If these accesses are not properly synchronized, inconsistent or incorrect data can result.
Example Scenario: Consider a database system where two users attempt to update the same record at the same time. If the system does not handle these concurrent updates correctly, one update might overwrite the other, leading to data loss or corruption.
Critical Sections: Race conditions typically involve critical sections of code where shared resources are accessed. Without proper locking mechanisms (like mutexes or semaphores), multiple threads might enter a critical section simultaneously, causing race conditions.
Impact: The impact of race conditions can range from minor bugs to significant data corruption, security vulnerabilities, or system crashes. In data engineering, this can mean incorrect data analytics, faulty data processing pipelines, and unreliable data systems.
Prevention: Preventing race conditions involves proper synchronization techniques, such as:
- Locks: Using locks to ensure that only one thread can access a critical section at a time.
- Atomic Operations: Utilizing atomic operations that are completed in a single step, preventing interruptions.
- Transaction Management: Implementing transaction management in databases to ensure consistency and isolation of concurrent operations.
- Thread-safe Data Structures: Using data structures designed to handle concurrent access safely.
By understanding and mitigating race conditions, data engineers can ensure the reliability, consistency, and accuracy of their data systems and applications.
How Dagster Helps Avoid Race Conditions
As the leading data orchestration solution, Dagster helps avoid race conditions through several key features and design principles:
Resource Management:
Dagster allows you to define resources that your pipelines depend on, such as database connections, APIs, or compute resources. By managing these resources explicitly, you can control access and usage, preventing multiple processes from accessing the same resource simultaneously in an uncontrolled manner.Concurrency Control:
Dagster provides mechanisms to limit concurrency within your pipelines. For example, you can configure job concurrency to ensure that only a certain number of jobs run simultaneously, which helps prevent race conditions by reducing the chances of multiple jobs competing for the same resources.Partitioning:
Dagster supports partitions and partition sets, which allow you to slice your dataset by time or other dimensions. This can help in organizing and executing batch computations in a way that avoids race conditions, as each partition can be processed independently.Federated Architecture:
Dagster’s architecture enables teams to isolate their pipelines, which helps avoid issues like starvation, monolithic dependencies, and coupled failures.Observability and Monitoring:
Dagster offers detailed insights into pipeline runs, including logs and execution timing. This observability helps in identifying and diagnosing race conditions quickly, allowing you to take corrective actions before they cause significant issues.
By leveraging these features, Dagster provides a robust framework for managing data pipelines in a way that minimizes the risk of race conditions, ensuring reliable and consistent execution of your data workflows.
Race Conditions: An Example in Python
A race condition occurs when two or more threads access shared data and try to change it at the same time. In the context of data engineering, this often happens when multiple processes attempt to read from and write to the same file simultaneously, leading to unpredictable results and potential data corruption.
Below is a Python example demonstrating a race condition using threading. The example involves two threads attempting to read from and write to the same file.
Python Example of Race Condition with Threads
- Setup: First, let's create a sample file to work with.
- Threads: We will create two threads: one for reading from the file and one for writing to the file.
- Race Condition: Due to the lack of synchronization, the two threads will likely interfere with each other.
Setup
Create a sample file named data.txt
with some initial content.
## Create a sample file with initial content
with open('data.txt', 'w') as f:
f.write("Initial Content\n")
Threads with Race Condition
Here is the code to demonstrate the race condition:
import threading
import time
## Function to write to the file
def write_to_file():
for i in range(5):
with open('data.txt', 'a') as f:
f.write(f"Writing line {i}\n")
time.sleep(0.1)
## Function to read from the file
def read_from_file():
for i in range(5):
with open('data.txt', 'r') as f:
print(f.read())
time.sleep(0.1)
## Create threads for writing and reading
writer_thread = threading.Thread(target=write_to_file)
reader_thread = threading.Thread(target=read_from_file)
## Start threads
writer_thread.start()
reader_thread.start()
## Wait for threads to complete
writer_thread.join()
reader_thread.join()
Explanation
- write_to_file: This function opens the file in append mode (
'a'
) and writes a line to it. It then sleeps for 0.1 seconds to simulate processing time. - read_from_file: This function opens the file in read mode (
'r'
) and prints its contents. It also sleeps for 0.1 seconds.
When the two threads run simultaneously, you might see inconsistent output due to the race condition. For example, the read operation might happen while the file is being written to, leading to partial reads or other unexpected behaviors.
Expected Output
The output may vary with each run, but an example output might look like this:
Initial Content
Writing line 0
Writing line 0
Writing line 0
Initial Content
Writing line 0
Initial Content
Writing line 0
Writing line 1
...
The actual output will vary, illustrating the unpredictable nature of race conditions. You might see partial reads or mixed content because the threads are not synchronized.
Resolving the Race Condition
To resolve this race condition, you can use thread synchronization mechanisms such as locks.
Here is the modified code with a lock:
import threading
import time
## Create a lock object
lock = threading.Lock()
## Function to write to the file with a lock
def write_to_file():
for i in range(5):
with lock:
with open('data.txt', 'a') as f:
f.write(f"Writing line {i}\n")
time.sleep(0.1)
## Function to read from the file with a lock
def read_from_file():
for i in range(5):
with lock:
with open('data.txt', 'r') as f:
print(f.read())
time.sleep(0.1)
## Create threads for writing and reading
writer_thread = threading.Thread(target=write_to_file)
reader_thread = threading.Thread(target=read_from_file)
## Start threads
writer_thread.start()
reader_thread.start()
## Wait for threads to complete
writer_thread.join()
reader_thread.join()
In this version, the lock
ensures that only one thread can access the file at a time, thus avoiding the race condition. This will result in consistent and predictable output as follows:
Writing line 0
Writing line 0
Writing line 1
Writing line 0
Writing line 1
Writing line 2
Writing line 0
Writing line 1
Writing line 2
Writing line 3
Writing line 0
Writing line 1
Writing line 2
Writing line 3
Writing line 4
AsyncIO
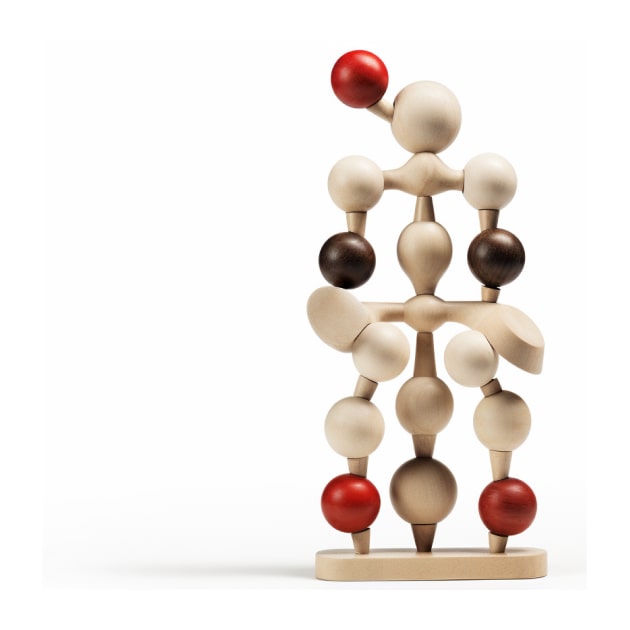
Big Data Processing
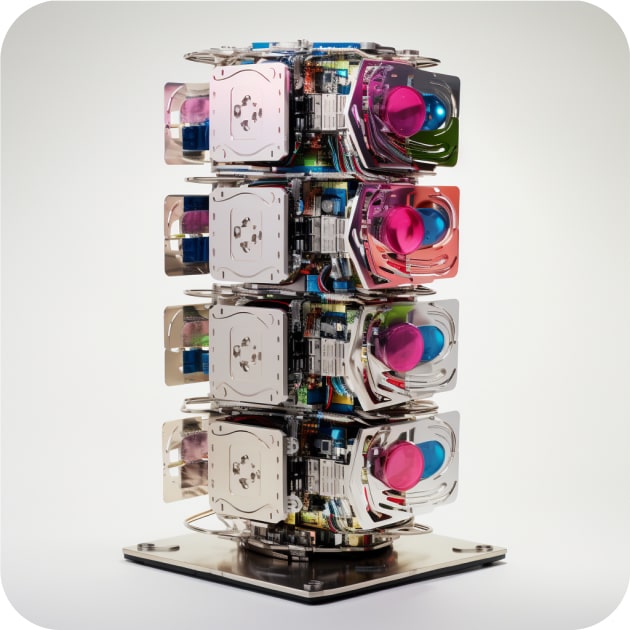
Fan-Out
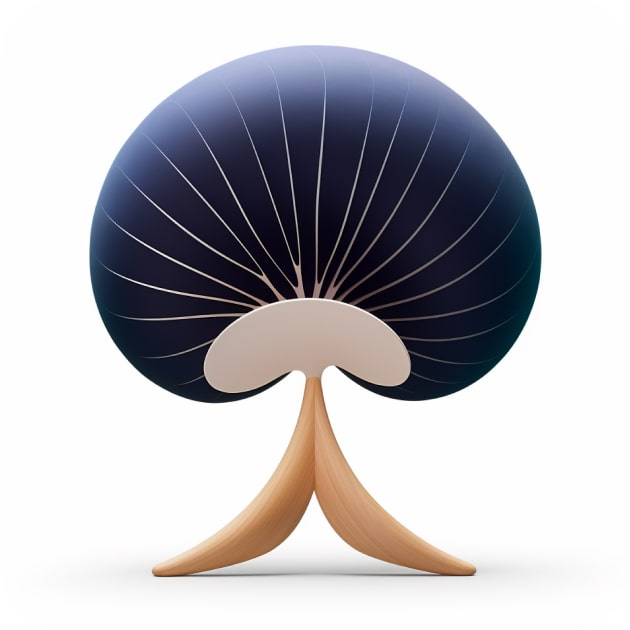
Multiprocessing
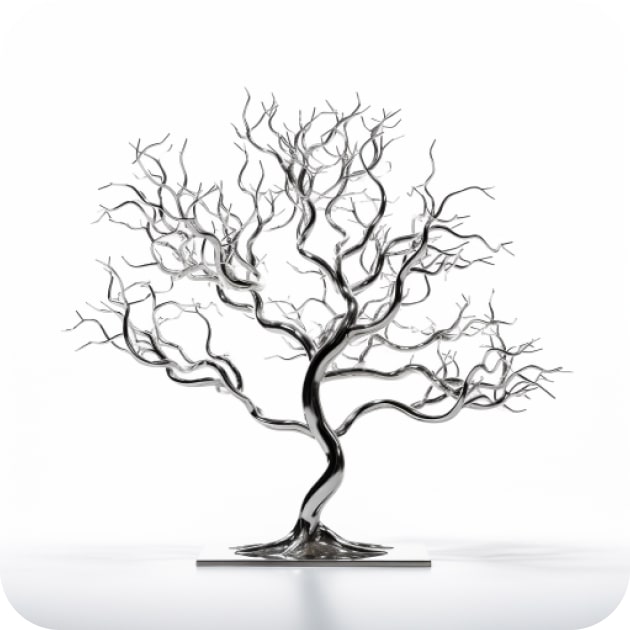
Parallelize
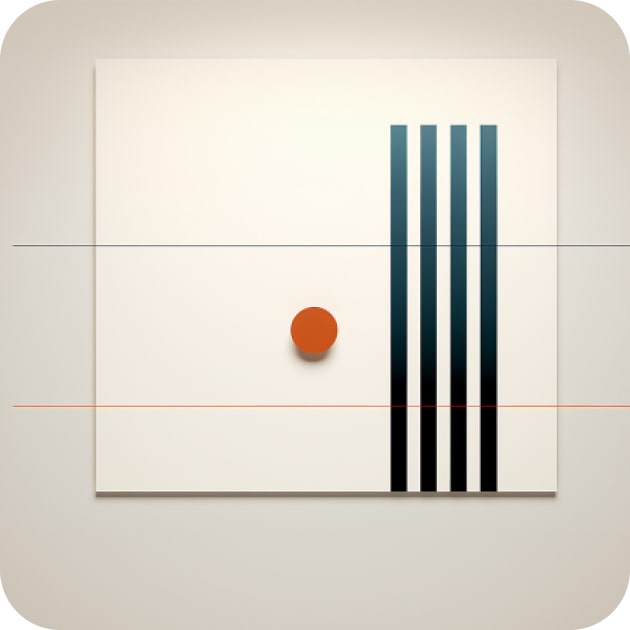
Thread
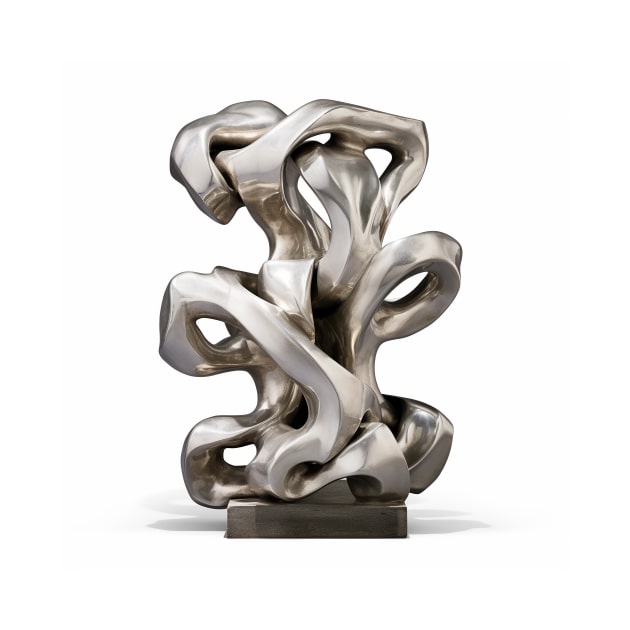
Vectorize
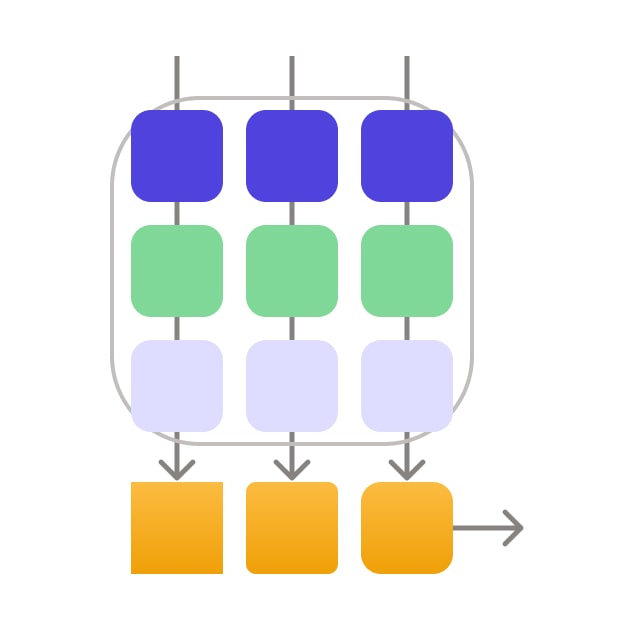