Data Pickling in Python
Data pickling definition:
In Python programming, 'pickling' refers to the process of converting a Python object into a byte stream, which can be stored in a file or transferred across a network. This process is also known as serialization or marshaling.
The byte stream created during pickling contains all the information about the object's state, such as its variables, methods, and attributes. When the byte stream is unpickled, the object is reconstructed with all its original attributes and methods.
Pickling is a useful feature in Python as it allows you to save and retrieve complex data structures easily. For example, you can use pickling to store the state of a machine learning model or save the state of a game so that it can be resumed later.
Data pickling example using Python:
Python provides the built-in pickle
module, which provides functions to serialize and deserialize Python objects. The dump
function is used to serialize the object to a file, and the load
function is used to deserialize the object from the file.
Here is a simple example of using Python’s pickle
library:
import pickle
# Define a sample dictionary
data = {'name': 'John', 'age': 30, 'city': 'New York'}
# Serialize the dictionary using Pickle
with open('data.pickle', 'wb') as f:
pickle.dump(data, f)
# Deserialize the dictionary from the Pickle file
with open('data.pickle', 'rb') as f:
new_data = pickle.load(f)
# Print the deserialized dictionary
print(new_data)
Backpressure
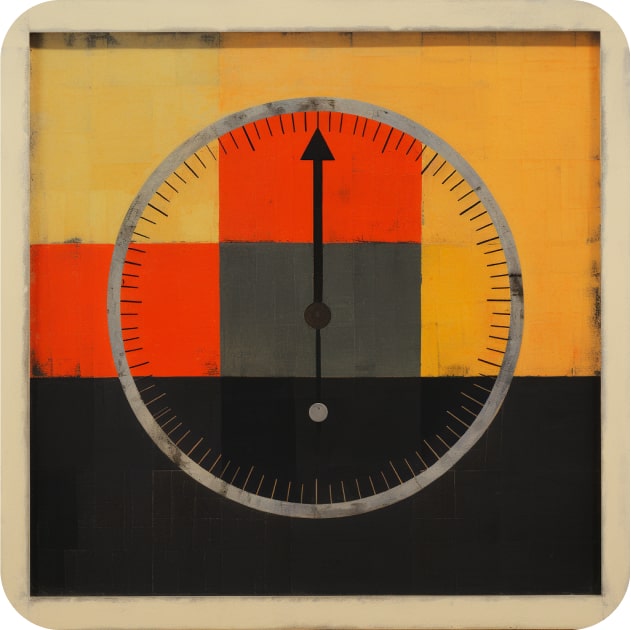
Compact
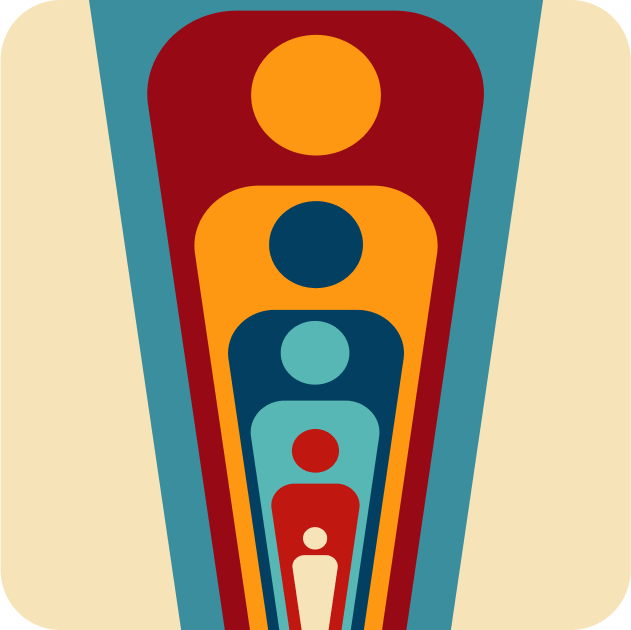
Compress
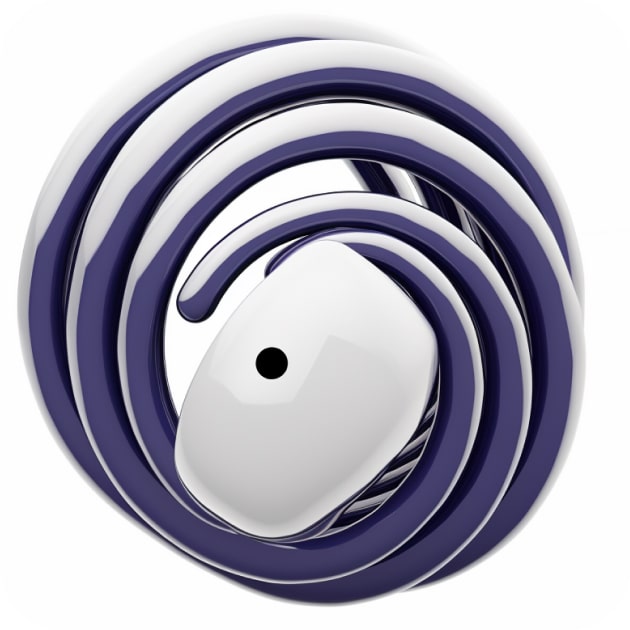
Ingest
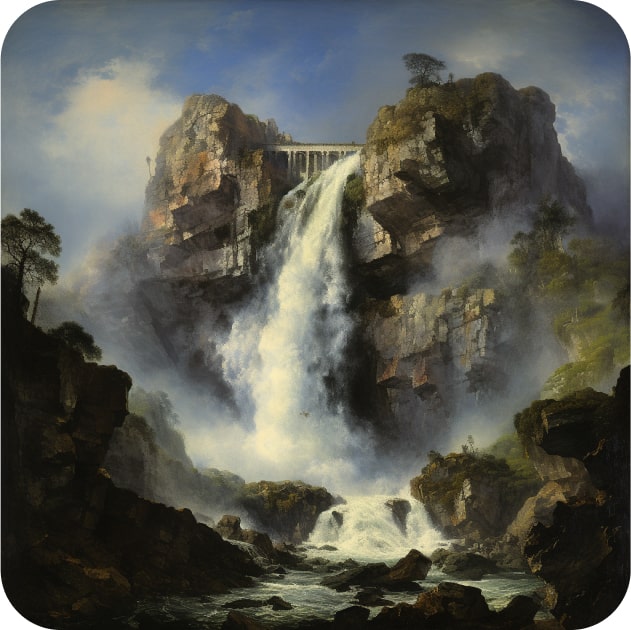
Load
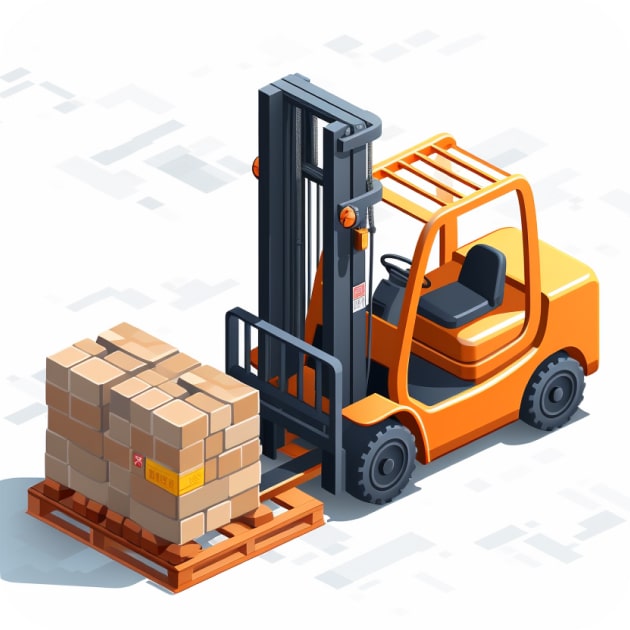
NoSQL
Rebalance
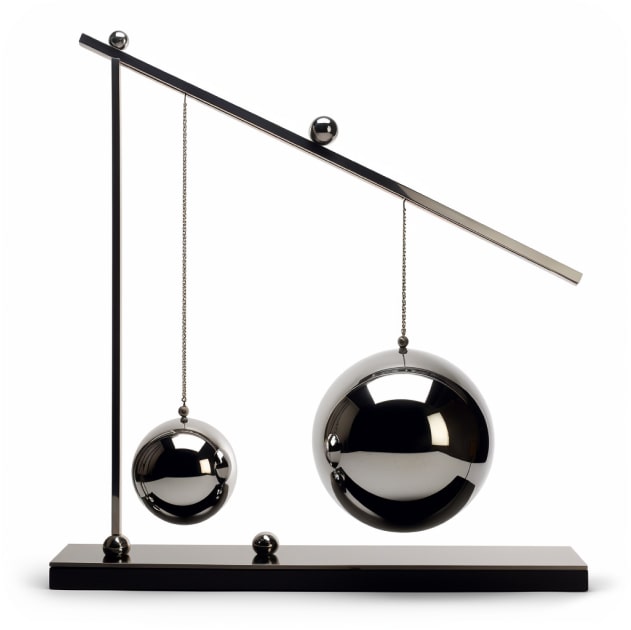
Repartition
Scrape
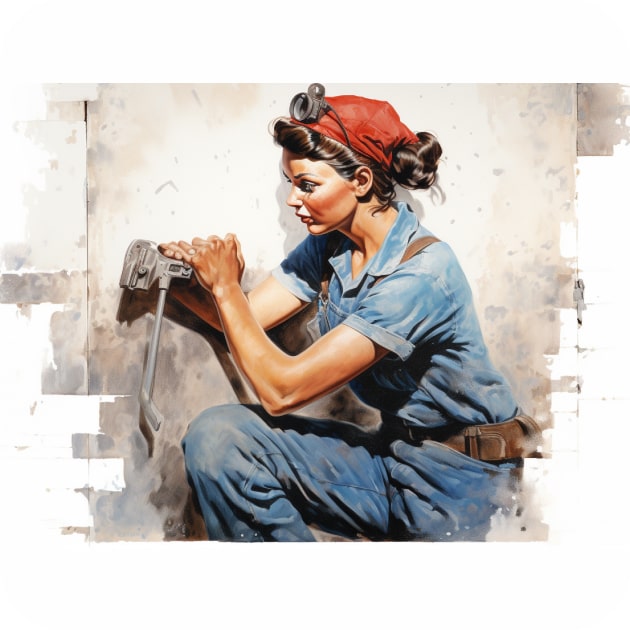
Shard
Shuffle
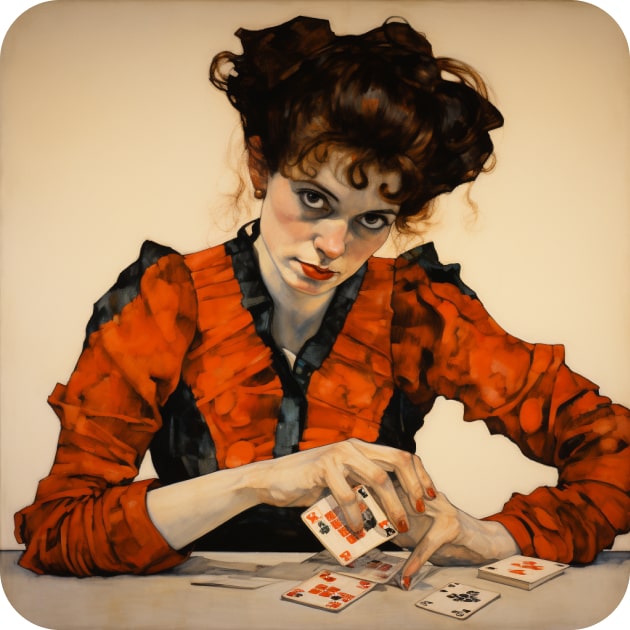
Spill
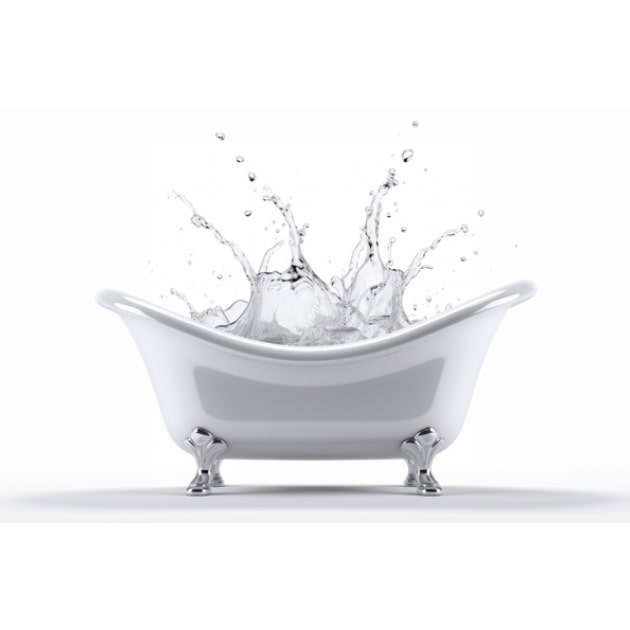
Stored Procedure
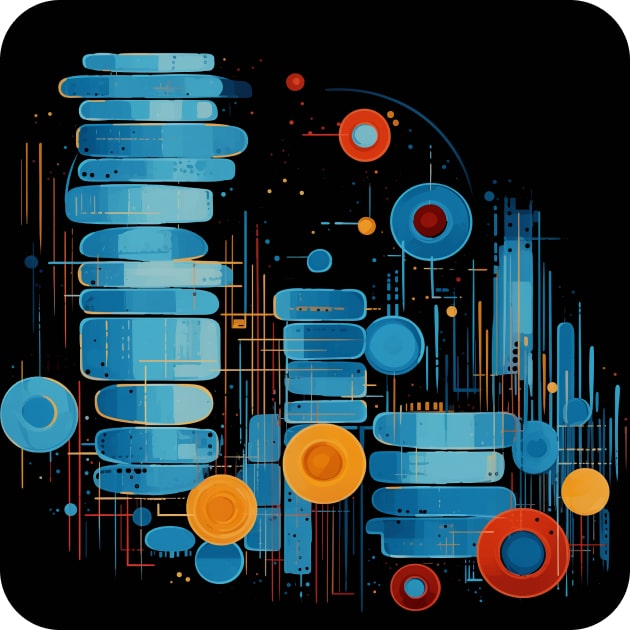
Upsert
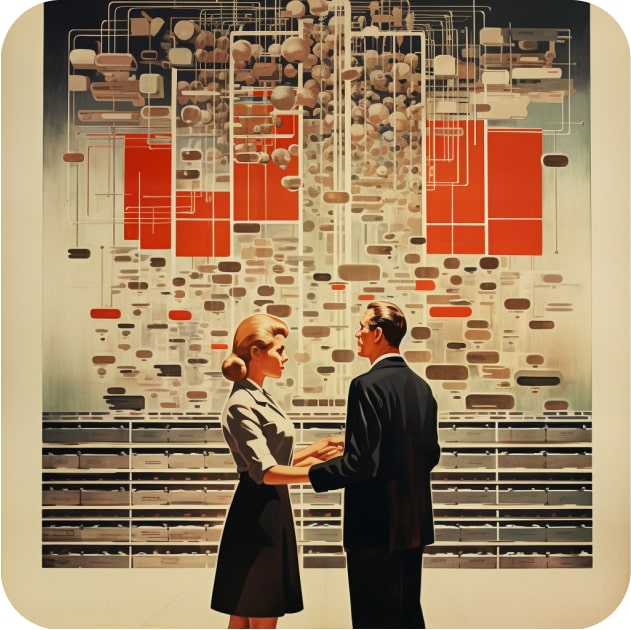