Dagster Data Engineering Glossary:
Geospatial Analysis
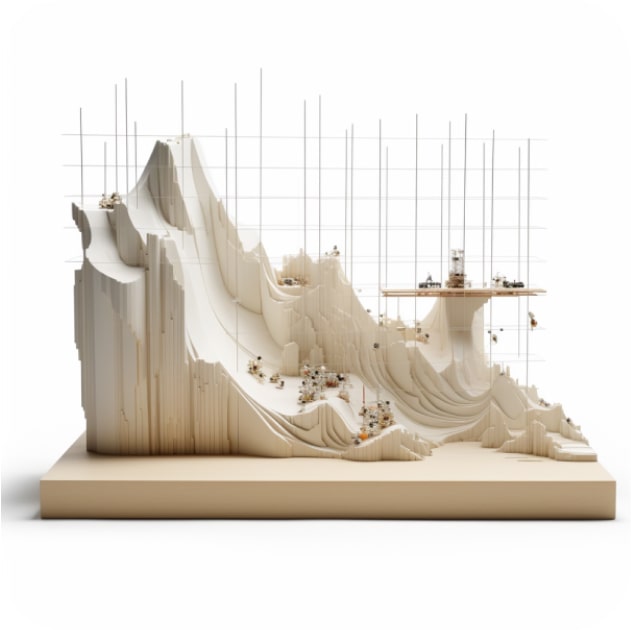
Geospatial analysis definition:
Geospatial analysis is the process of analyzing spatial data to extract meaningful insights and patterns. Geospatial data can be in the form of geographical coordinates, satellite images, and other related data. In the context of modern data pipelines, geospatial analysis can help in understanding the relationship between different geographic locations and other data points, such as demographics or climate data.
Geospatial data analysis is used to model and represent how people, objects, and phenomena interact within space, as well as to make predictions based on trends in the relationships between places.
Python has several libraries that can be used for geospatial analysis, including Geopandas, Shapely, Fiona, and PySAL. Here is a practical example using Geopandas and Matplotlib:
Geospatial data analysis in Python
- Matplotlib installation instructions are found here but just involve the command
python -m pip install -U matplotlib
.
import matplotlib.pyplot as plt
import geopandas as gpd
# Load the data
world = gpd.read_file(gpd.datasets.get_path('naturalearth_lowres'))
cities = gpd.read_file(gpd.datasets.get_path('naturalearth_cities'))
# Plot the world map
fig, ax = plt.subplots(figsize=(10, 6))
world.plot(ax=ax, color='white', edgecolor='black')
# Plot the cities
cities.plot(ax=ax, markersize=5, color='red')
# Set the title and axis labels
ax.set_title('Cities of the world')
ax.set_xlabel('Longitude')
ax.set_ylabel('Latitude')
# Show the plot
plt.show()
This code loads two datasets from the Geopandas library: world and cities. The world dataset contains the shapes of all countries in the world, while the cities dataset contains the locations of major cities worldwide.
The code then creates a plot of the world map using matplotlib. It sets the color of the countries to white and the color of the borders to black. It then plots the cities on top of the map as red dots.
Finally, the code sets the title and axis labels of the plot and displays it using plt.show()
.
This is just a simple example, but Geopandas and matplotlib offer a wide range of geospatial analysis tools that can be used for more advanced applications.
Geospatial analysis in Python using Xarray-spatial
Here's a basic example of using the Xarray-spatial package for geospatial analysis in Python. This example uses the hillshade
function to analyze topographical data from a digital elevation model (DEM). To keep this example self-contained, I am creating a simple synthetic DEM data.
If you want to work with real-world data, you could substitute this synthetic data with an Xarray DataArray that contains your actual geospatial data. Also, this is a simplified example and does not take into account various geospatial complexities you may encounter in real-world datasets.
# Required Libraries
import numpy as np
import xarray as xr
from xrspatial import hillshade
# Create synthetic Digital Elevation Model (DEM) data
dem_data = np.random.rand(5,5) * 100
dem_data = xr.DataArray(dem_data, dims=["x", "y"])
# Calculate hillshade
hillshade_data = hillshade(dem_data)
# Print the hillshade data
print(hillshade_data)
This script will generate hillshade data, a grayscale 3D representation of the surface, with the sun's relative position taken into account for shading the image. Hillshade is used to visualize terrain in a 2D map and is commonly used in geographical and environmental studies.
Please note that xrspatial.hillshade
function computes the hillshade for a DEM (which is an input 2D DataArray). It uses the sun's azimuth and altitude and the vertical exaggeration of the terrain to calculate the illumination value for each cell in the DEM. It does not require any projection system.
For more advanced and specific operations, the library allows operations like terrain, proximity, focal, zonal, global, and local statistics, generalization, classification, and pathfinding.
Output:
<xarray.DataArray 'hillshade' (x: 5, y: 5)>
array([[ nan, nan, nan, nan, nan],
[ nan, 0.27194697, 0.08499345, 0.90350395, nan],
[ nan, 0.88516366, 0.2557956 , 0.07063997, nan],
[ nan, 0.73102015, 0.8325217 , 0.8833356 , nan],
[ nan, nan, nan, nan, nan]],
dtype=float32)
Dimensions without coordinates: x, y
Cosine Similarity
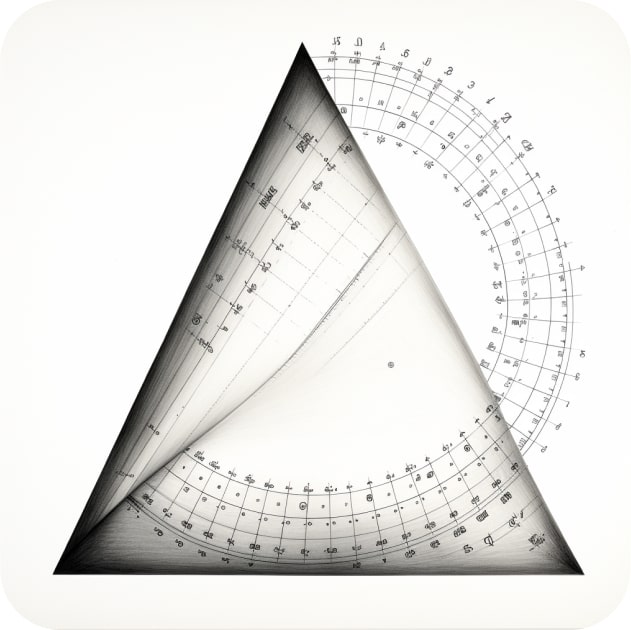
Explore
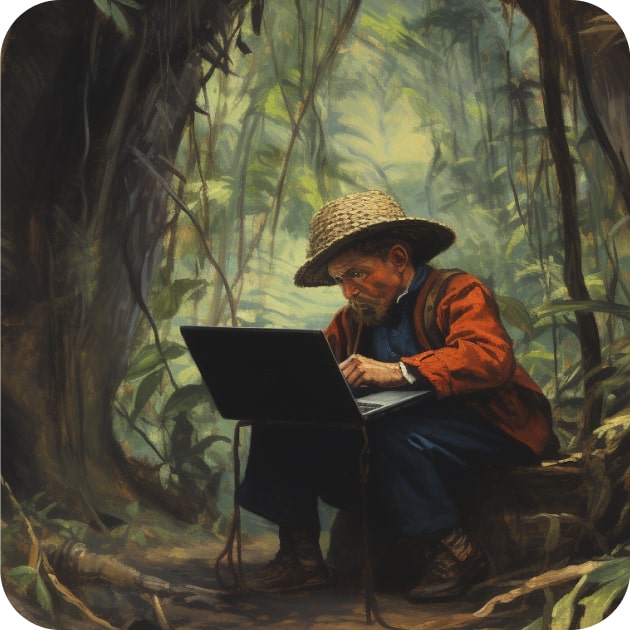
Extrapolate
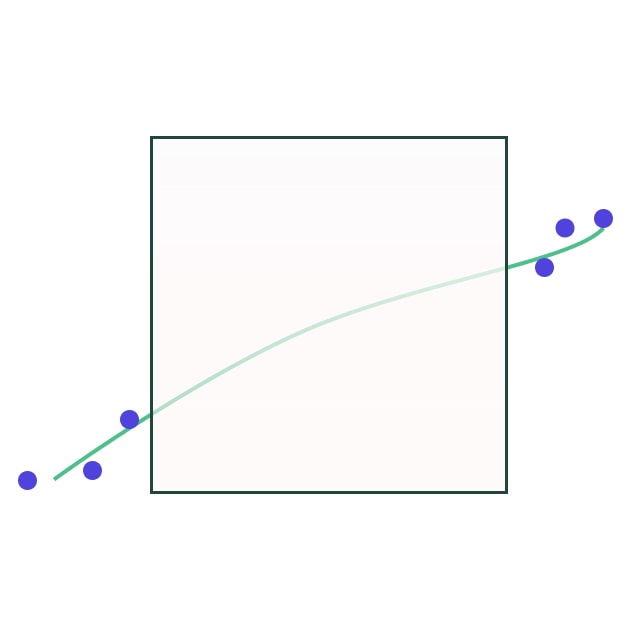
Interpolate
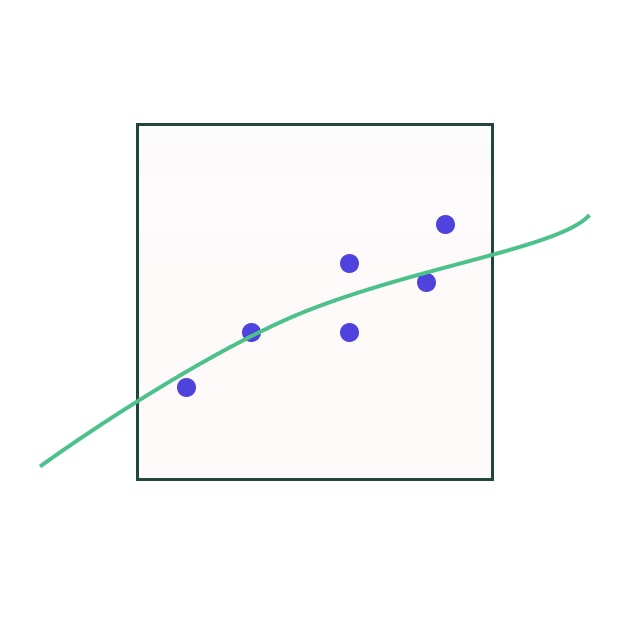
Mine
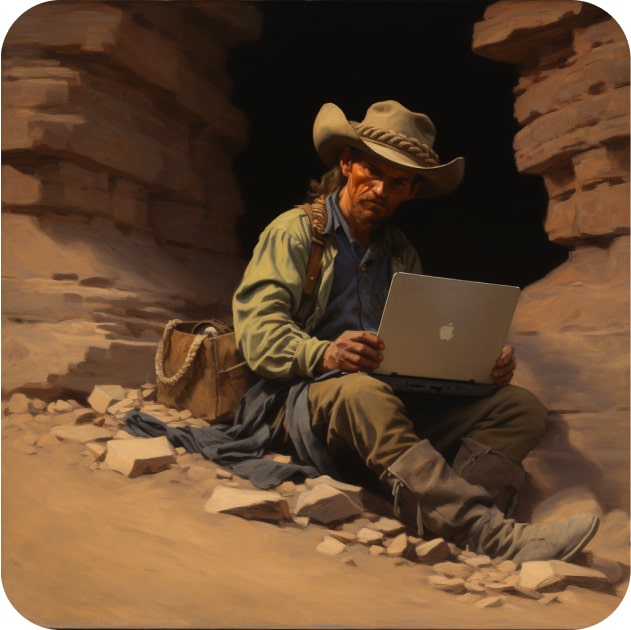
Normality Testing
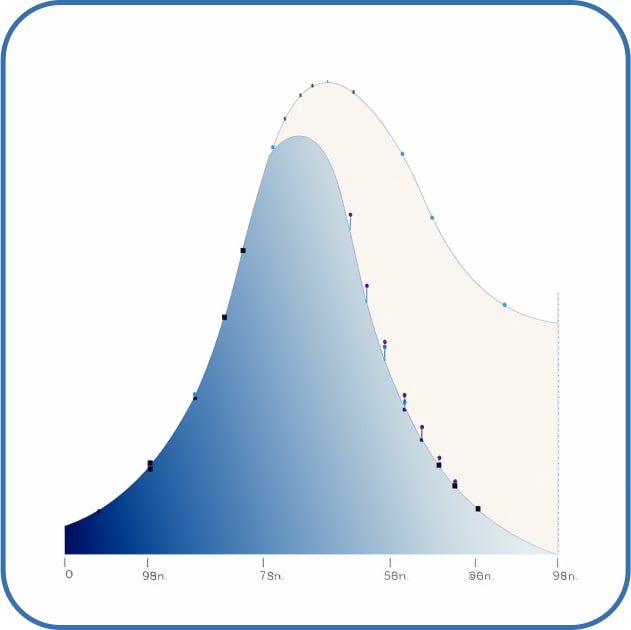
Profile
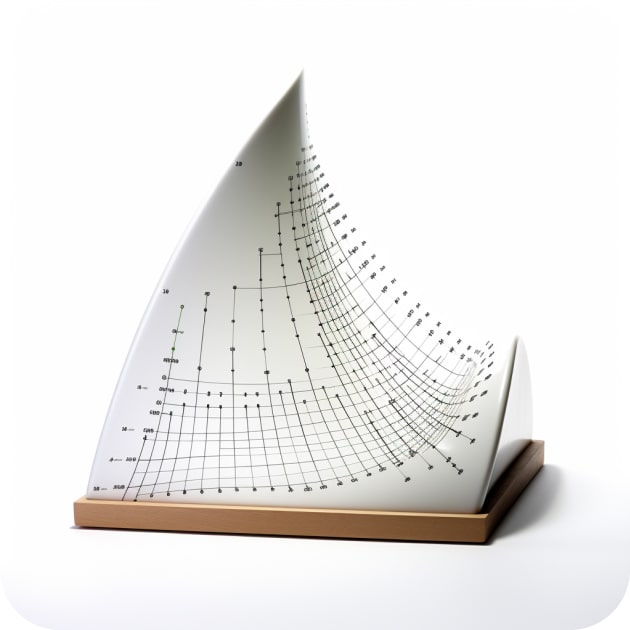
Sentiment Analysis
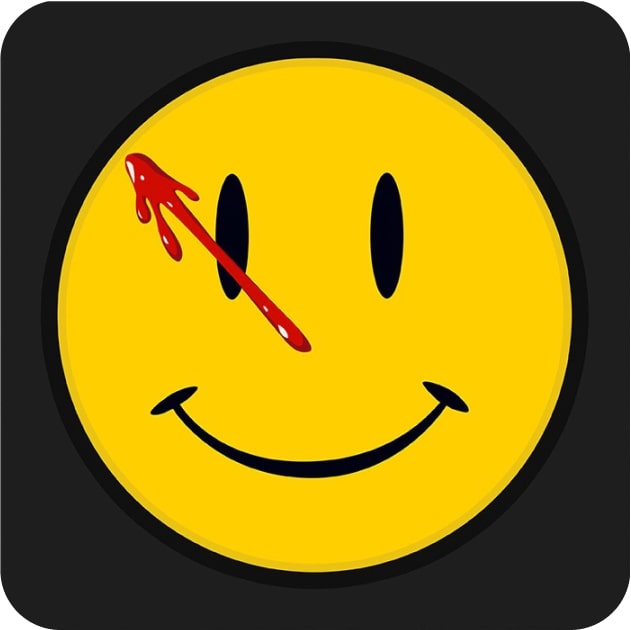
Time Series Analysis
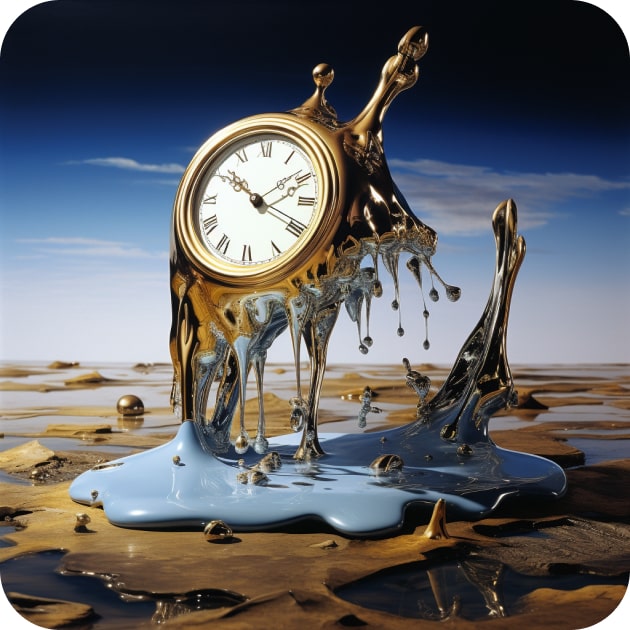
Unstructured Data Analysis
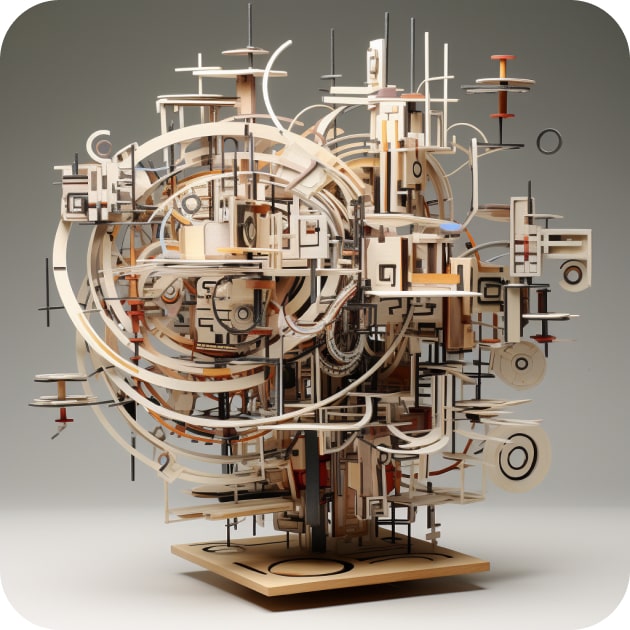