Dagster Data Engineering Glossary:
Data Interpolation
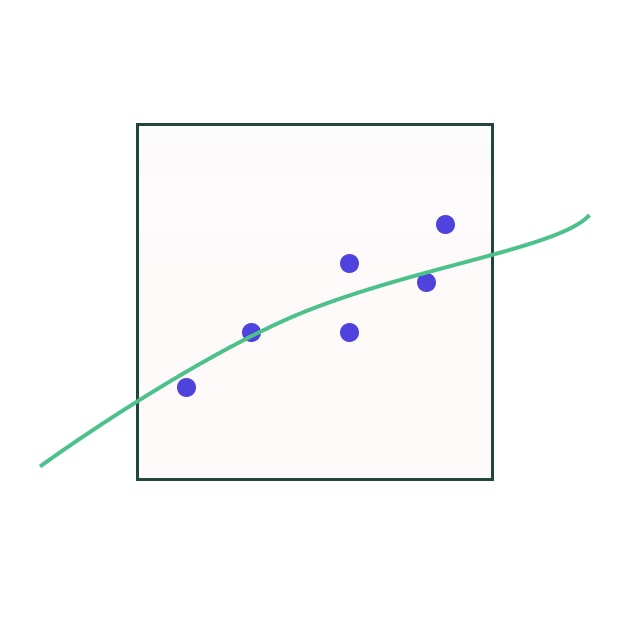
Interpolation definition
Data Interpolation is a statistical technique used to estimate or predict unknown values within a known range of data points, based on existing data. Interpolation assumes that there is a consistent trend or pattern between the known data points and uses this to fill gaps in the data. Its accuracy relies on the assumption that the trend between existing points extends to unknown points.
An example of data interpolation in Python
Interpolation is often used in data engineering to fill missing values or to make predictions within the range of existing data. A common method for doing this is using polynomial interpolation.
Let's consider an example where we use numpy's polyfit
function to fit a polynomial to some data, then use this polynomial to interpolate some values.
import numpy as np
import matplotlib.pyplot as plt
# Set a random seed for reproducibility
np.random.seed(42)
# Define the true function
def f(x):
return np.sin(2 * np.pi * x)
# Generate some synthetic data
X = np.sort(np.random.rand(10))
y = f(X) + np.random.normal(scale=0.3, size=10)
# Fit a polynomial of degree 3 to the data
coefficients = np.polyfit(X, y, 3)
polynomial = np.poly1d(coefficients)
# Generate points for interpolation
X_interp = np.linspace(0, 1, 100)
y_interp = polynomial(X_interp)
# Plot the original data
plt.scatter(X, y, label='Original data', color='blue')
# Plot the interpolated data
plt.plot(X_interp, y_interp, label='Interpolation', color='red')
# Set title and legend
plt.title('Polynomial Interpolation')
plt.legend()
# Show the plot
plt.show()
Here we first define a function f(x)
which is the true underlying function that we are trying to learn. We then generate some data by sampling x
values randomly, calculating the corresponding y
values using the function, and adding some random noise.
Next, we fit a 3rd degree polynomial to the data using np.polyfit
, and use this polynomial to interpolate y
values at 100 points evenly spaced between 0 and 1.
The resulting plot shows the original data as blue points and the interpolated values as a red line. As you can see, the interpolated values fit the original data well, suggesting that the polynomial is a good model of the underlying function.
Cosine Similarity
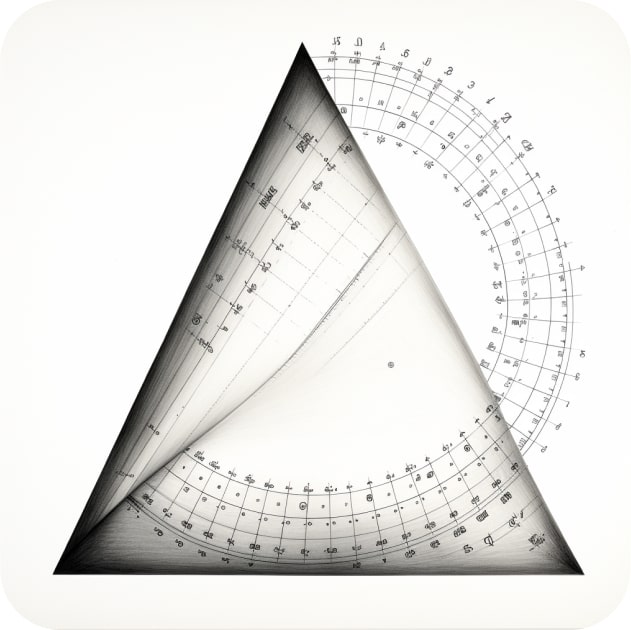
Explore
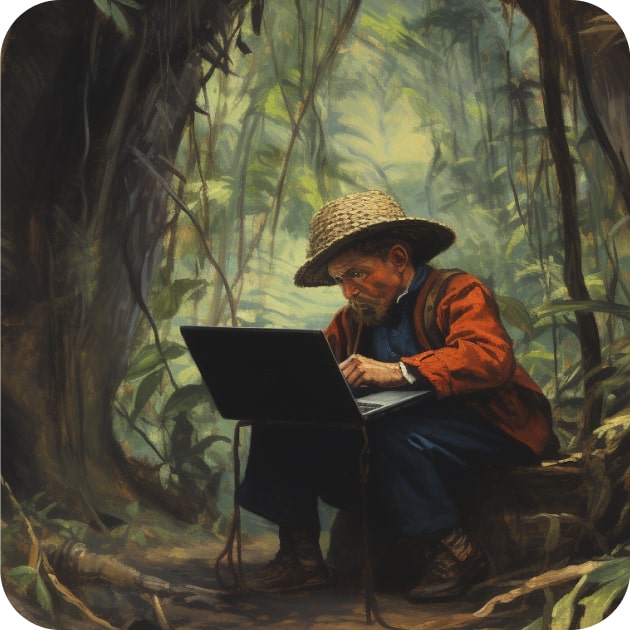
Extrapolate
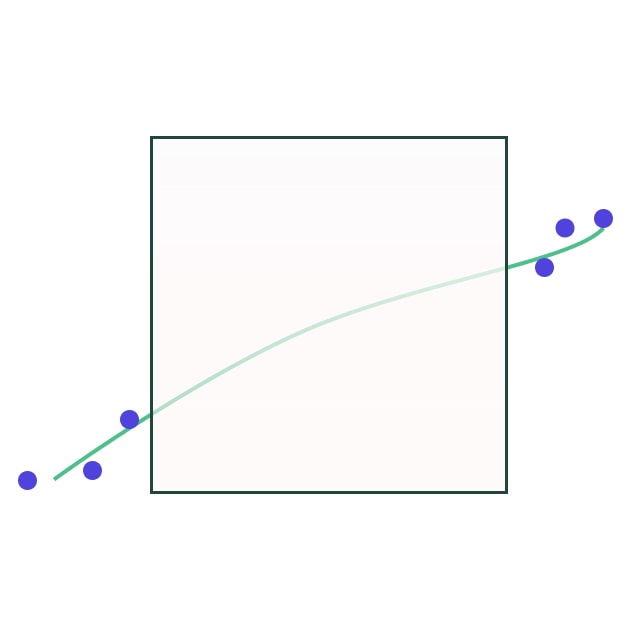
Geospatial Analysis
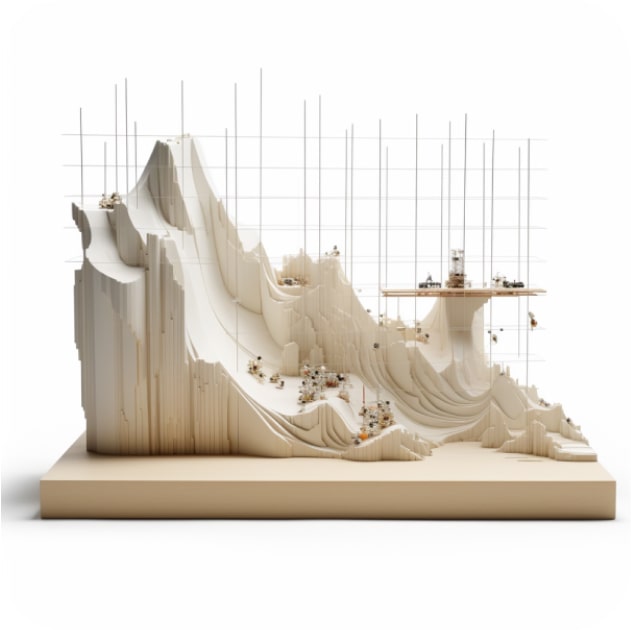
Mine
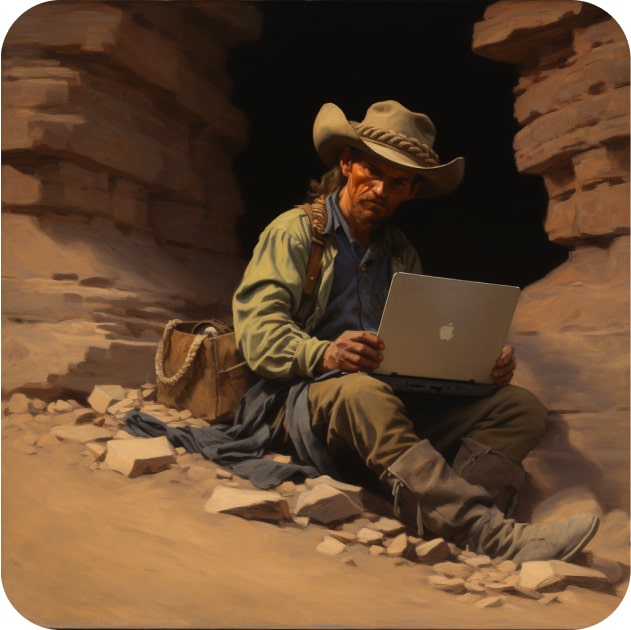
Normality Testing
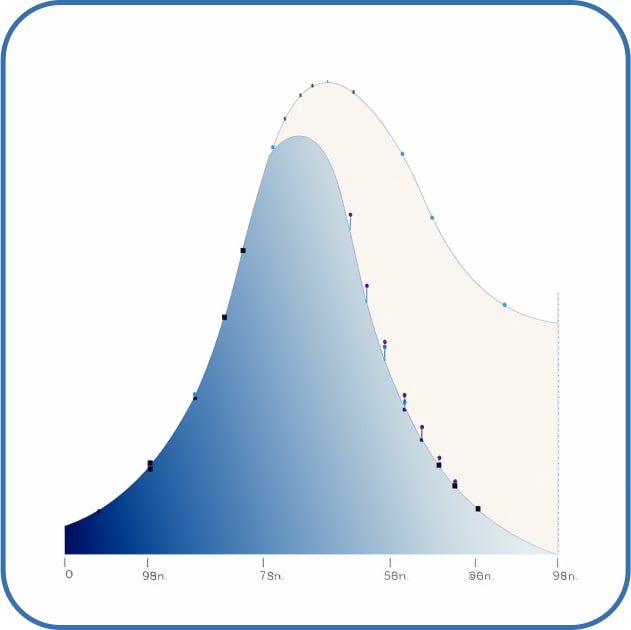
Profile
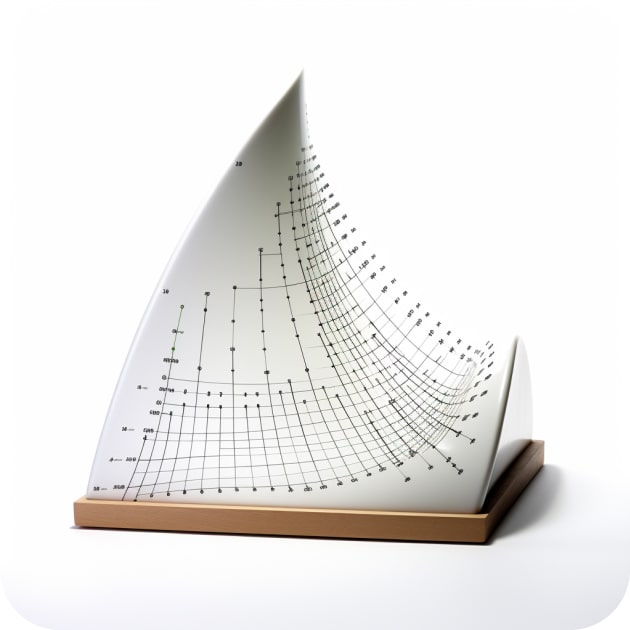
Sentiment Analysis
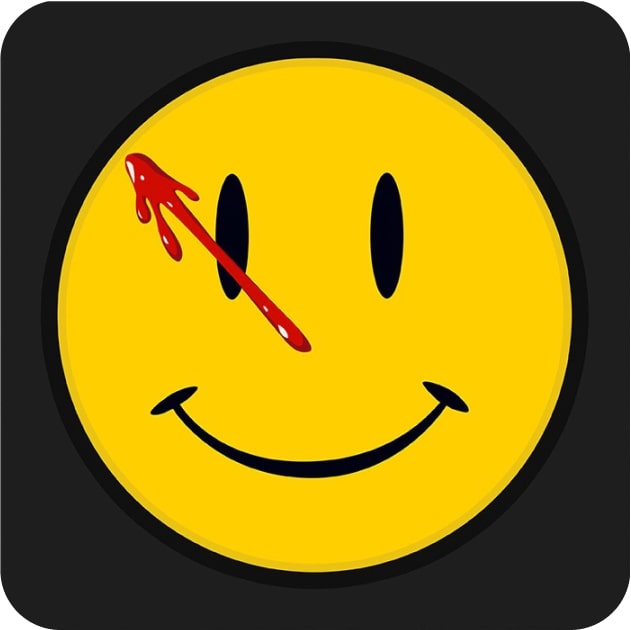
Time Series Analysis
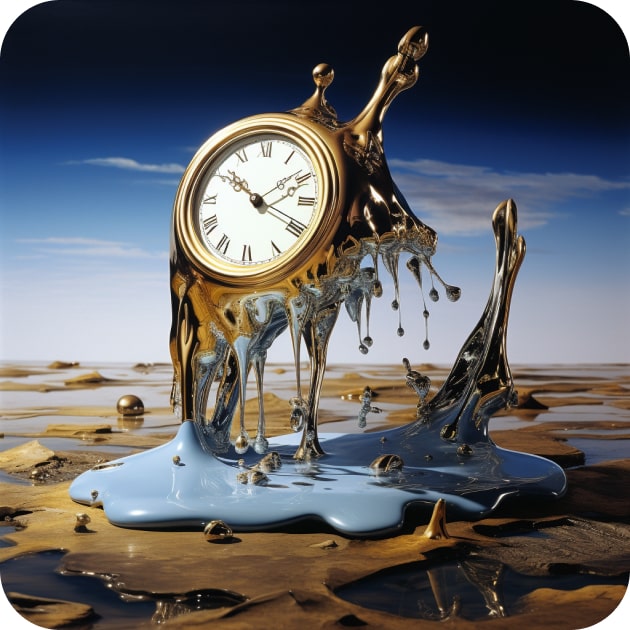
Unstructured Data Analysis
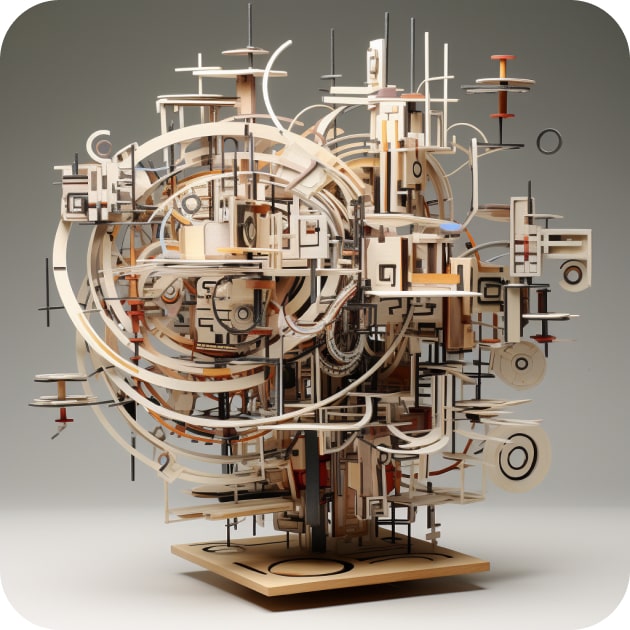