Dagster Data Engineering Glossary:
Time Series Analysis
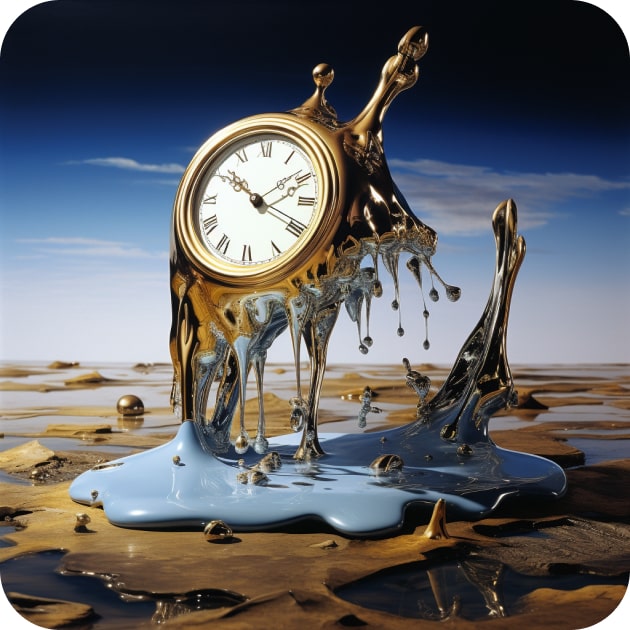
Time series analysis - a definition:
Time series analysis is the process of analyzing time series data, which is a sequence of data points indexed in time order. Time Series Analysis is important in many fields, including finance, economics, climate science, and engineering.
In the context of modern data pipelines, Time Series Analysis involves processing and analyzing large volumes of time series data in real-time or near-real-time to extract valuable insights and detect patterns.
Here are the steps involved in Time Series Analysis:
- Data collection: Collecting time series data from various sources such as sensors, social media, and websites.
- Data pre-processing: Cleaning, filtering, and normalizing the data to remove any inconsistencies, missing values, or outliers.
- Data exploration: Visualizing the data to gain insights, detect trends, and patterns.
- Feature engineering: Extracting meaningful features from the time series data that can be used for modeling.
- Model selection: Choosing an appropriate statistical or machine learning model that best fits the data and the problem at hand.
- Model training: Training the selected model using historical time series data.
- Model evaluation: Evaluating the model's performance using appropriate metrics and validation techniques.
- Model deployment: Deploying the model in a production environment to generate predictions and insights in real-time.
Python provides many powerful libraries for Time Series Analysis, including:
- pandas: A library for data manipulation and analysis that provides efficient data structures for handling time series data.
- NumPy: A library for numerical computing that provides tools for working with time series data, including indexing and date-time handling.
- Matplotlib: A library for data visualization that provides powerful tools for creating visualizations of time series data. Matplotlib installation instructions are found here but basically just involves the command
python -m pip install -U matplotlib
. - Statsmodels: A library for statistical modeling and analysis that provides tools for time series analysis, including autoregression and moving average models.
Example of Time Series Analysis in Python
Here's an example of using Python and pandas to perform Time Series Analysis. Please note that you need to have the necessary Python libraries installed in your Python environment to run this code:
Given an input time series file time_series_data.csv
of:
date,price
2021-04-23,4180.17
2021-04-22,4134.98
2021-04-21,4166.45
2021-04-20,4134.94
2021-04-19,4163.29
2021-04-16,4185.47
2021-04-15,4170.42
2021-04-14,4124.66
2021-04-13,4141.59
2021-04-12,4127.99
2021-04-09,4128.80
2021-04-08,4080.36
2021-04-07,4063.04
2021-04-06,4067.42
2021-04-05,4077.91
2021-04-01,4019.87
2021-03-31,3972.89
2021-03-30,3958.55
2021-03-29,3974.54
2021-03-26,3974.54
2021-03-25,3889.14
2021-03-24,3881.37
2021-03-23,3910.52
2021-03-22,3910.52
2021-03-19,3913.10
2021-03-18,3915.46
2021-03-17,3957.79
2021-03-16,3952.34
2021-03-15,3968.94
Our simple analysis:
import pandas as pd
import matplotlib.pyplot as plt
# load the time series data into a pandas DataFrame
data = pd.read_csv('time_series_data.csv')
# convert the 'date' column to a datetime object
data['date'] = pd.to_datetime(data['date'])
# set the 'date' column as the index of the DataFrame
data.set_index('date', inplace=True)
# visualize the time series data
plt.plot(data)
plt.title("Daily price")
plt.show()
# compute the rolling mean of the data
rolling_mean = data.rolling(window=7).mean()
# visualize the rolling mean
plt.plot(rolling_mean)
plt.title("Rolling mean")
plt.show()
Will generate two graphs:
In the above example, we load the time series data from a CSV file into a pandas DataFrame. We then convert the 'date' column to a datetime
object and set it as the index of the DataFrame.
We visualize the time series data using Matplotlib and compute the rolling mean of the data using the rolling()
function provided by pandas.
Finally, we visualize the rolling mean using Matplotlib. This is just a basic example, but Time Series Analysis can involve much more complex and sophisticated techniques, depending on the nature of the data and the problem at hand.
Here is an example of time series analysis using numpy and Matplotlib:
import random
import datetime
import matplotlib.pyplot as plt
# Generate random time series data
x = list(range(1, 101))
y = [10 + i + random.gauss(0, 1) for i in x]
# Convert x values to datetime format
start_date = datetime.date.today() - datetime.timedelta(days=100)
x_dates = [start_date + datetime.timedelta(days=i) for i in x]
# Plot the time series using Matplotlib
fig, ax = plt.subplots()
ax.plot(x_dates, y)
# Format the x-axis to show dates
date_format = '%m/%d/%Y'
ax.xaxis.set_major_formatter(plt.FixedFormatter([i.strftime(date_format) for i in x_dates]))
fig.autofmt_xdate(rotation=45)
# Add labels and title
ax.set_xlabel('Date')
ax.set_ylabel('Value')
ax.set_title('Random Time Series with Noise')
plt.show()
This code generates a random time series data with some noise, and then converts the x values to datetime format using the datetime module. It then plots the time series using Matplotlib, with the x-axis showing dates and the y-axis showing values. The resulting plot should look like a wavy line with some random variation.
Time-Series Compaction:
In time-series databases, compaction techniques are often applied to efficiently store historical data. Older data points may be downsampled or aggregated to reduce storage requirements while still preserving important trends. See the Data Compaction entry for more details.
Cosine Similarity
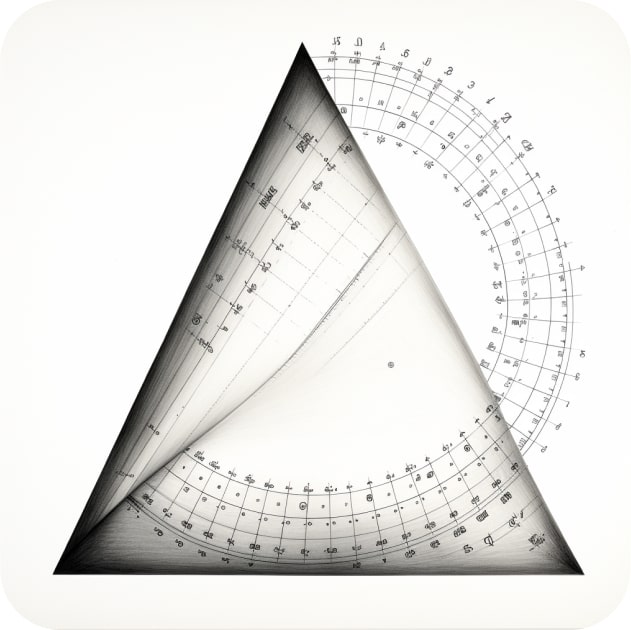
Explore
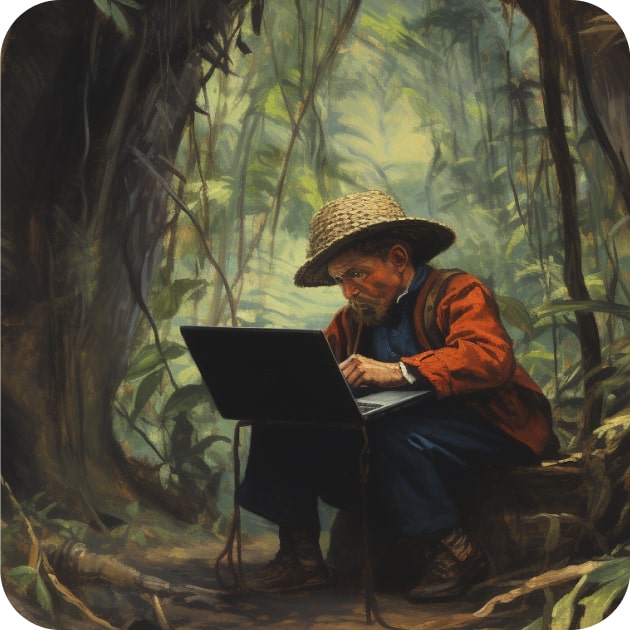
Extrapolate
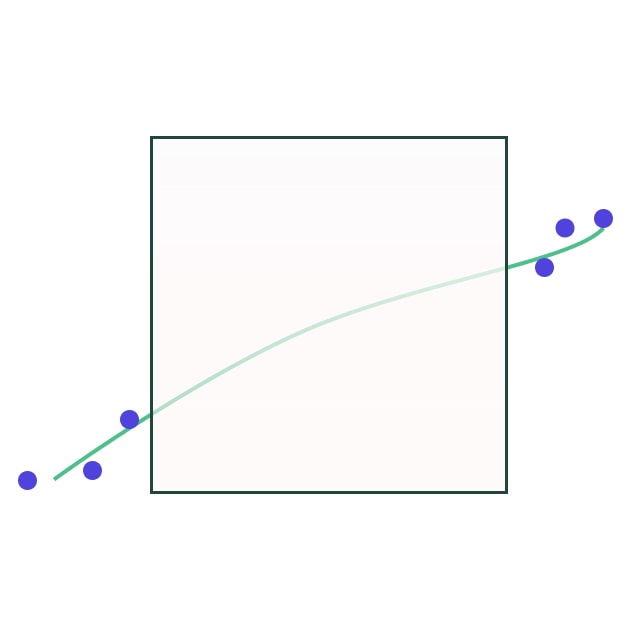
Geospatial Analysis
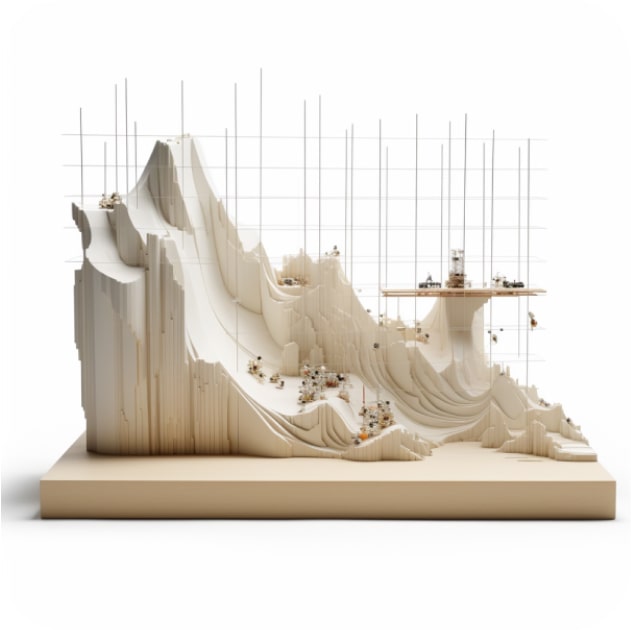
Interpolate
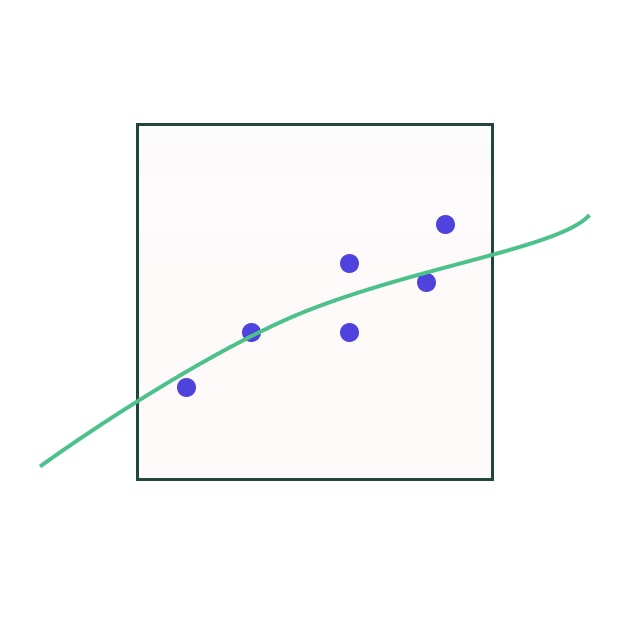
Mine
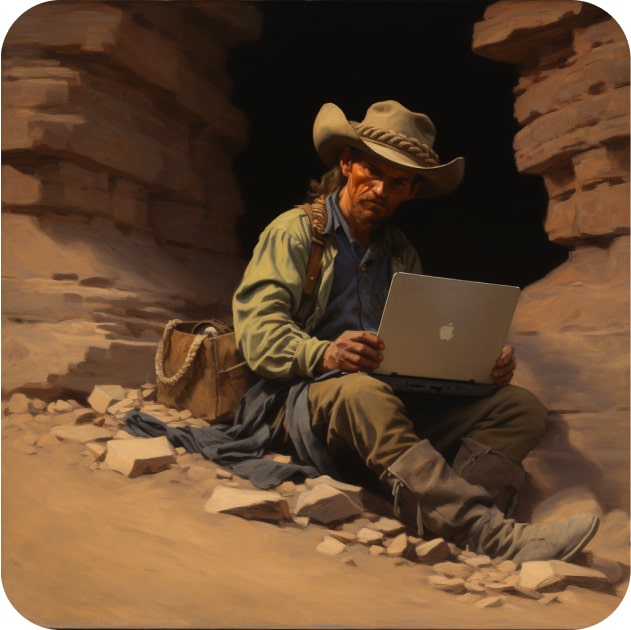
Normality Testing
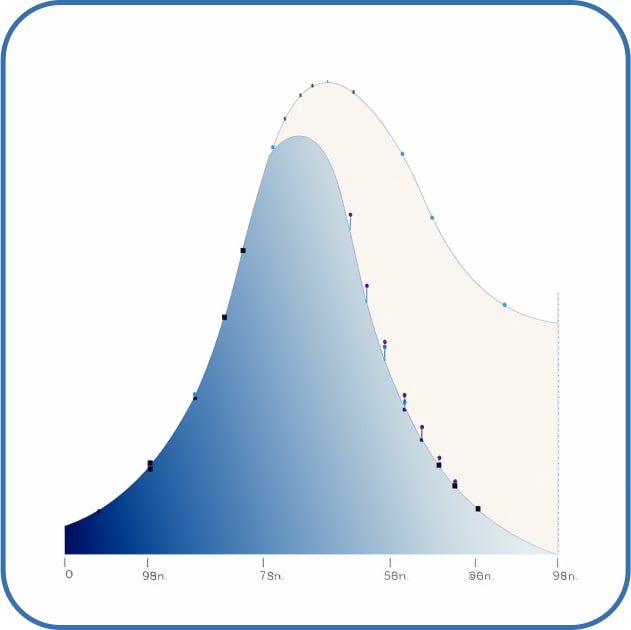
Profile
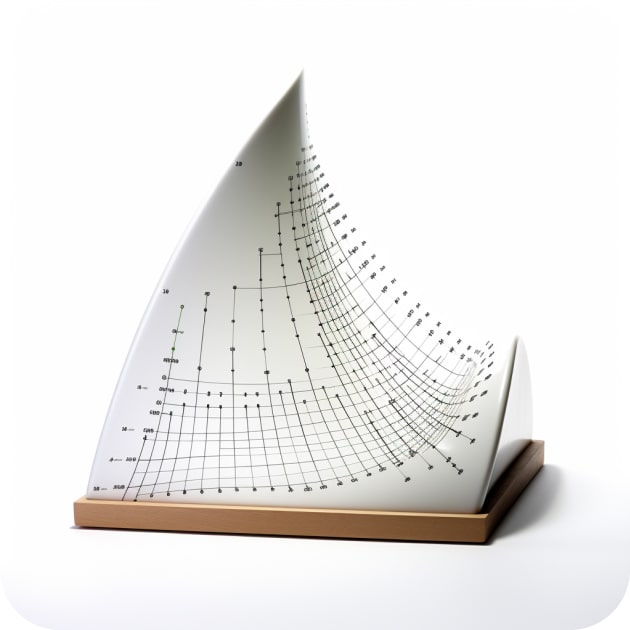
Sentiment Analysis
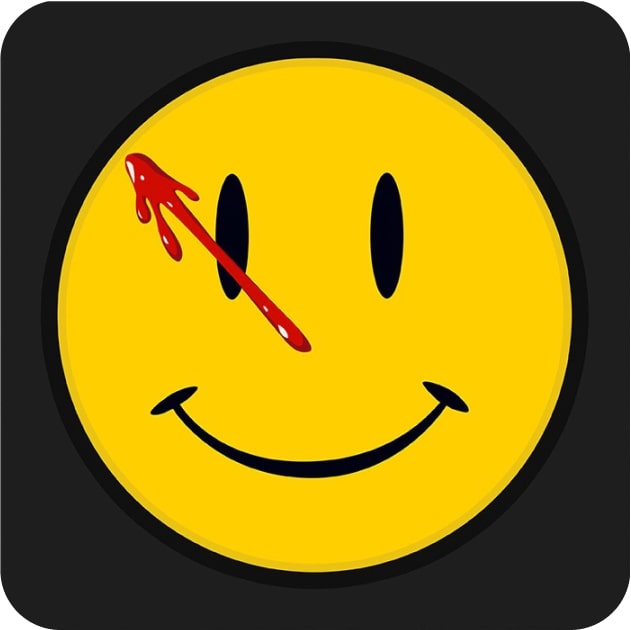
Unstructured Data Analysis
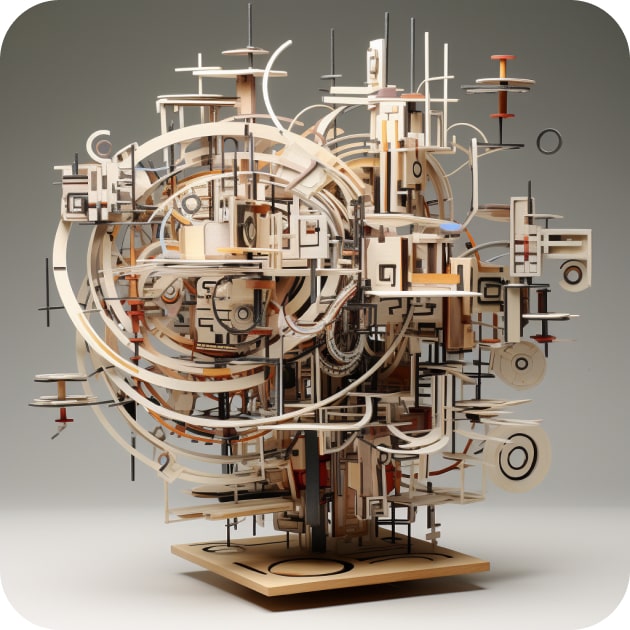