Sentiment Analysis
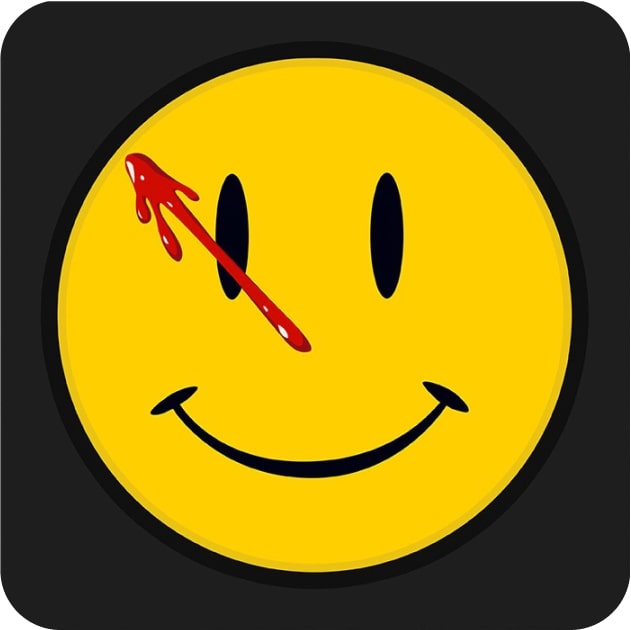
Sentiment analysis - a definition:
Sentiment analysis is a process of analyzing and classifying subjective data such as customer feedback, reviews, or social media posts into categories of positive, negative, or neutral sentiment. Sentiment analysis can be used to extract insights from large volumes of textual data.
Sentiment analysis techniques
There are different techniques for performing sentiment analysis, including rule-based methods, machine learning-based methods, and hybrid approaches that combine both. In general, the process involves the following steps:
Data collection: Collect the data that you want to analyze, such as customer feedback, reviews, or social media posts.
Text preprocessing: Clean and preprocess the text data by removing irrelevant information such as stop words, punctuations, and numbers. You can also perform techniques such as stemming or lemmatization to normalize the text.
Feature extraction: Convert the preprocessed text into numerical features that can be used for sentiment analysis. This can be done using techniques such as bag-of-words, word embeddings, or topic modeling.
Sentiment classification: Train a classification model, such as logistic regression or Naive Bayes, on a labeled dataset to classify the text into positive, negative, or neutral sentiment.
Evaluation: Evaluate the performance of the model using metrics such as accuracy, precision, recall, and F1 score.
Deployment: Deploy the sentiment analysis model into the data pipeline to classify new incoming data in real-time.
Sentiment analysis example using Python:
Here's an example of how to perform sentiment analysis using Python's Natural Language Toolkit (NLTK) library. Please note that you need to have the nltk libraries installed in your Python environment to run this code:
import nltk
from nltk.sentiment import SentimentIntensityAnalyzer
nltk.download('vader_lexicon')
# Load the sentiment analyzer
sia = SentimentIntensityAnalyzer()
# Example text
input_text = {
"Product A":"I love Product A! It's amazing and works really well. Good value for money.",
"Product B":"Wow, Product B is really limited and not fit for purpose. Do not buy this rubbish.",
"Product C":"I guess product C is OK but I have seen better. It should be cheaper, IMHO."
}
# Perform sentiment analysis
for text in input_text:
sentiment_scores = sia.polarity_scores(input_text[text])
print(f"{text} scores: {sentiment_scores}")
This will output a dictionary containing the sentiment scores of the text:
{'neg': 0.0, 'neu': 0.326, 'pos': 0.674, 'compound': 0.9479}
{'neg': 0.214, 'neu': 0.597, 'pos': 0.189, 'compound': 0.1273}
{'neg': 0.0, 'neu': 0.654, 'pos': 0.346, 'compound': 0.7019}
The pos
score indicates a high positive sentiment, while the neg score is 0, indicating no negative sentiment. The compound
score is a normalized score between -1 and 1 that represents the overall sentiment polarity. In this case, Product A gets 0.9479, indicating a highly positive sentiment.
Cosine Similarity
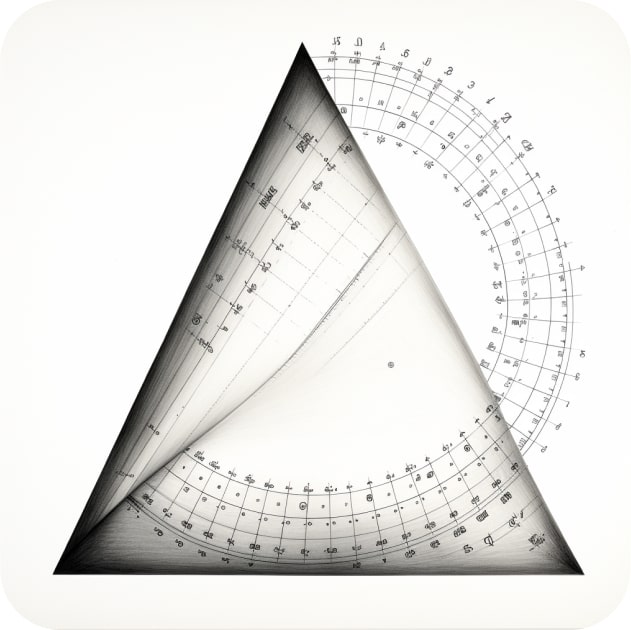
Explore
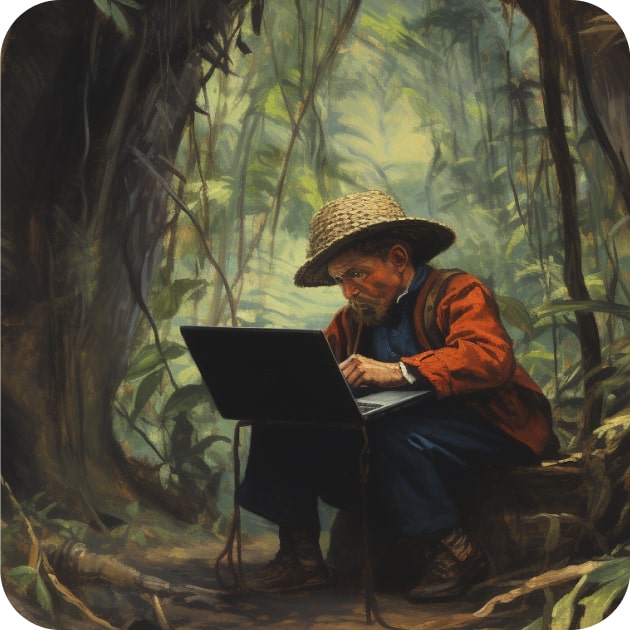
Extrapolate
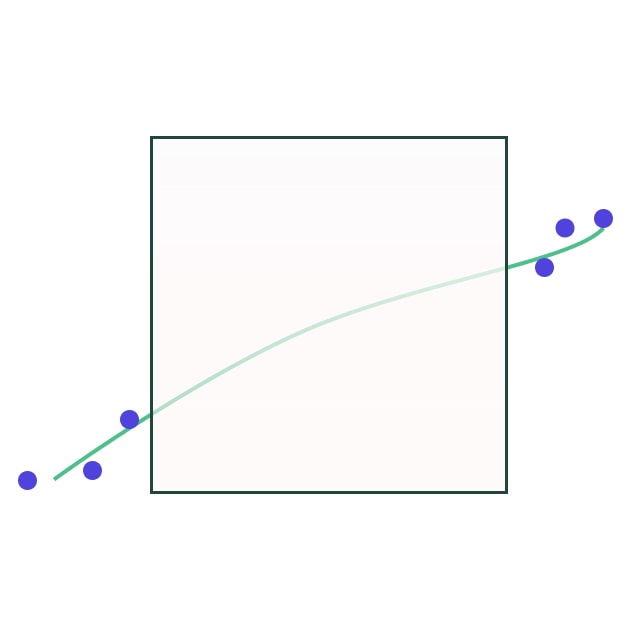
Geospatial Analysis
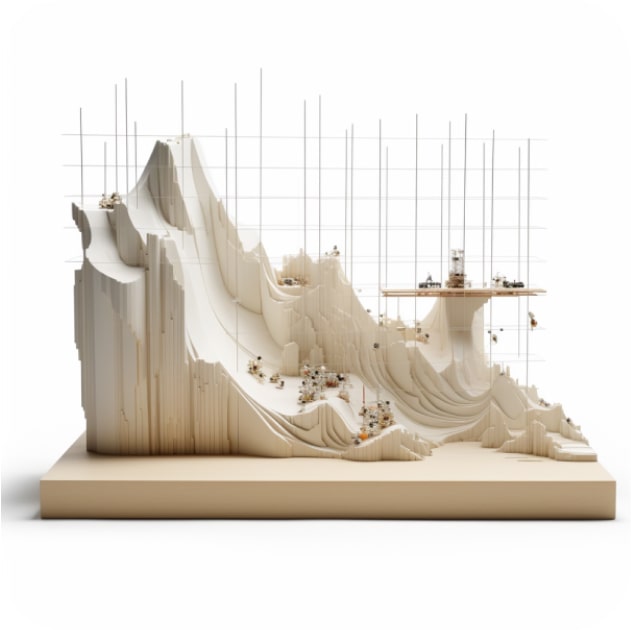
Interpolate
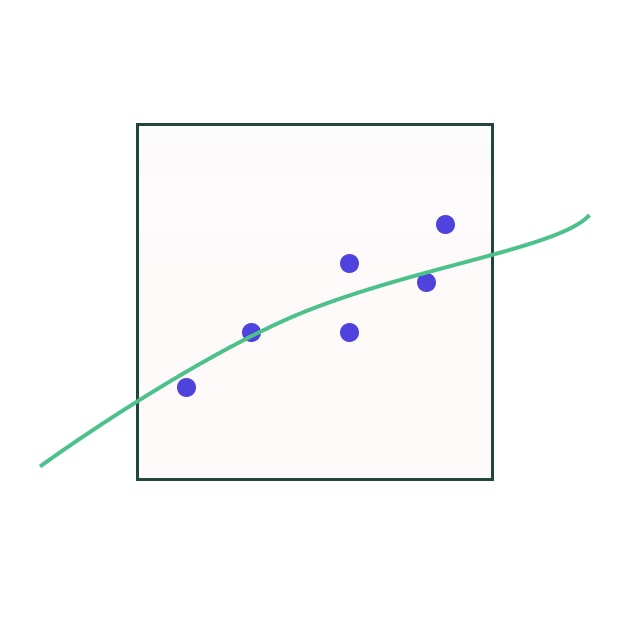
Mine
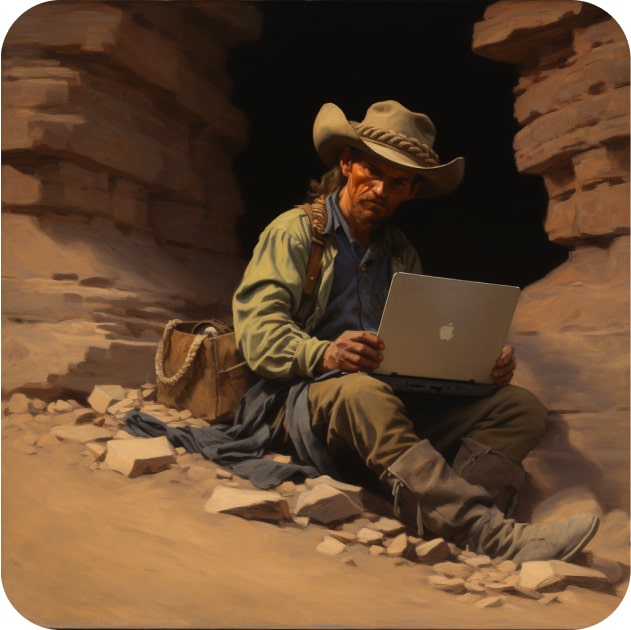
Normality Testing
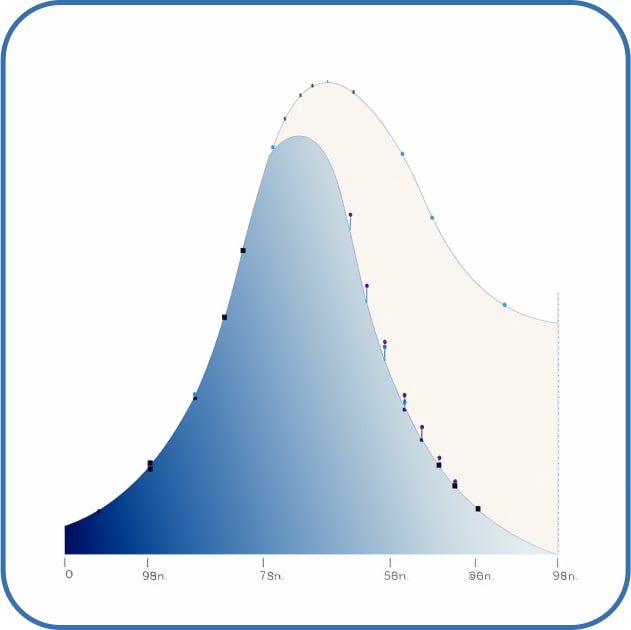
Profile
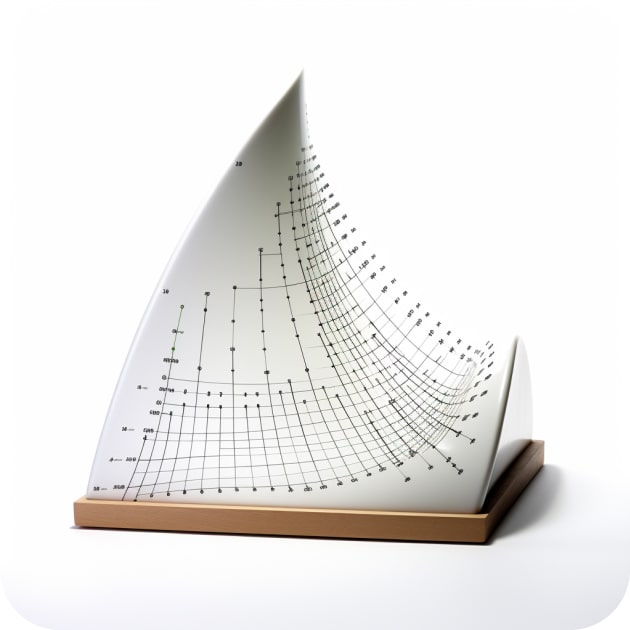
Time Series Analysis
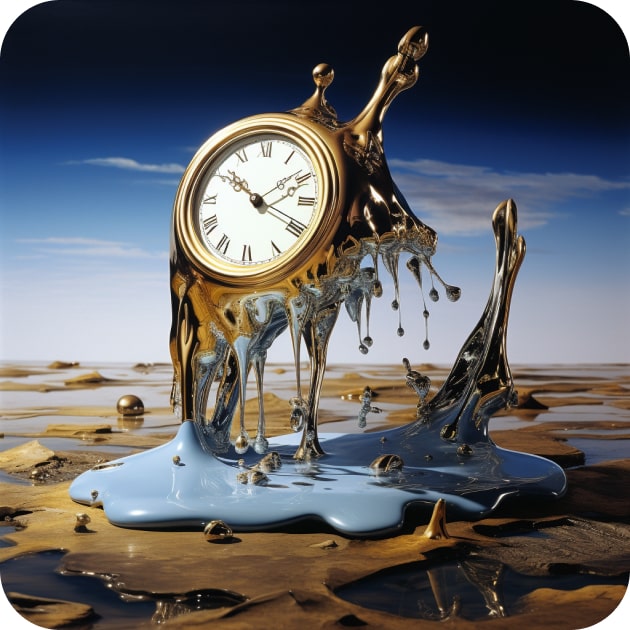
Unstructured Data Analysis
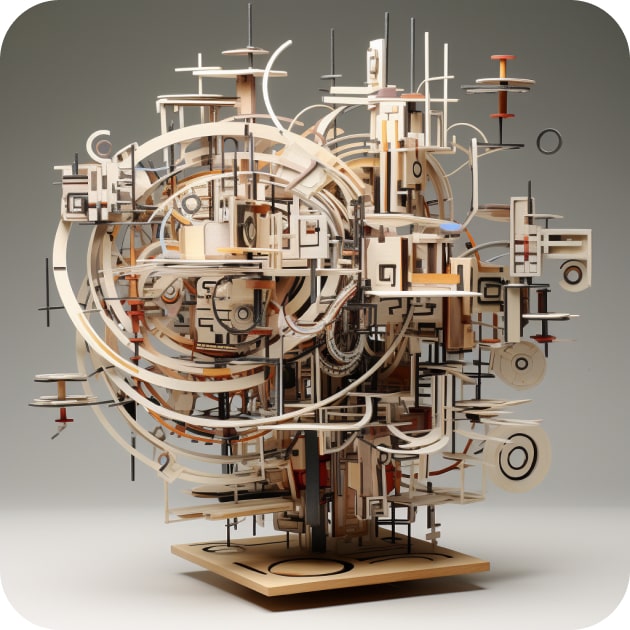