Dagster Data Engineering Glossary:
Data Denoising
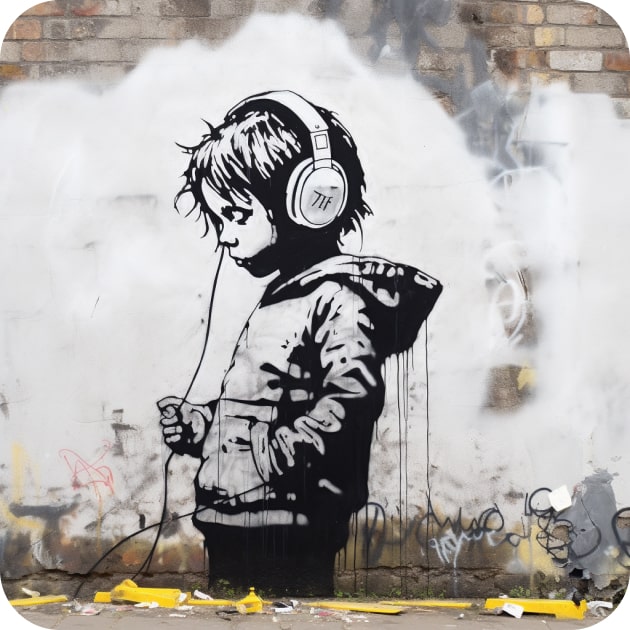
Data denoising - a definition:
In the context of data pipelines, denoising refers to the process of removing noise from the data, which could be caused by various factors such as measurement errors, faulty sensors, or even human error during data entry. The goal is to extract useful information from the data and remove any unwanted signals that could interfere with the analysis.
Data denoising in Python:
Here are some practical examples of denoising techniques in Python:
Please note that you need to have the necessary Python libraries installed in your Python environment to run this code.
Moving Average Filter: This is a simple denoising technique that involves taking the average of a sliding window of data points to smooth out the noise. It can be implemented using the rolling function in pandas or convolve function in numpy.
import numpy as np
def moving_average_filter(data, window_size):
window = np.ones(window_size)/float(window_size)
convolved_data = np.convolve(data, window, 'same')
print("Original data: {}".format(data))
print("Moving average filtered data with window size {}: {}".format(window_size, convolved_data))
return convolved_data
# example usage
data = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
window_size = 4
filtered_data = moving_average_filter(data, window_size)
This example will put yield the following output:
Original data: [ 1 2 3 4 5 6 7 8 9 10]
Moving average filtered data with window size 4: [0.75 1.5 2.5 3.5 4.5 5.5 6.5 7.5 8.5 6.75]
Median Filter: This technique involves replacing each data point with the median of its neighboring points. It can be implemented using the signal.medfilt function in scipy.
from scipy import signal
def median_filter(data, window_size):
filtered_data = signal.medfilt(data, kernel_size=window_size)
print("Original data: {}".format(data))
print("Median filtered data with window size {}: {}".format(window_size, filtered_data))
return filtered_data
# example usage
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
window_size = 5
filtered_data = median_filter(data, window_size)
Which will output:
Original data: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Median filtered data with window size 5: [1 2 3 4 5 6 7 8 8 8]
Try changing the window_size
value to 4 for an interesting result.
Since we are applying a median filter to an input signal using the medfilt()
function from the scipy.signal
module. The medfilt()
function applies a median filter to the input signal using a sliding window with a specified size, and returns the filtered signal.
When the window size is even, such as when window_size
= 4, the function raises an error because the medfilt()
function requires an odd number for the window size. This is because the median filter operates by taking the median value of the data points within the window, and when the window size is even, the median value is not well-defined.
Wavelet Transform: This is a more advanced denoising technique that involves decomposing the signal into different frequency bands using wavelets and then thresholding the coefficients to remove the noise. It can be implemented using the pywt library (which may require installation using pip install PyWavelets
.
import numpy as np
import pywt
def wavelet_denoising(data, wavelet='db4', level=1):
coeffs = pywt.wavedec(data, wavelet, level=level)
threshold = np.std(coeffs[-level])
coeffs = [pywt.threshold(c, threshold) for c in coeffs]
denoised_data = pywt.waverec(coeffs, wavelet)
print("Original data: {}".format(data))
print("Denoised data using wavelet {}: {}".format(wavelet, denoised_data))
return denoised_data
# example usage
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
wavelet = 'db4'
level = 1
denoised_data = wavelet_denoising(data, wavelet, level)
Which will yield the following output:
Original data: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Denoised data using wavelet db4: [0.95707075 2.00990332 2.94189799 3.95561577 5.00005885 5.96165328
6.97197144 7.97478225 8.97266171 9.96551716]
These are just a few examples of denoising techniques that can be used in data pipelines. The choice of technique depends on the nature of the noise and the requirements of the analysis.
Align
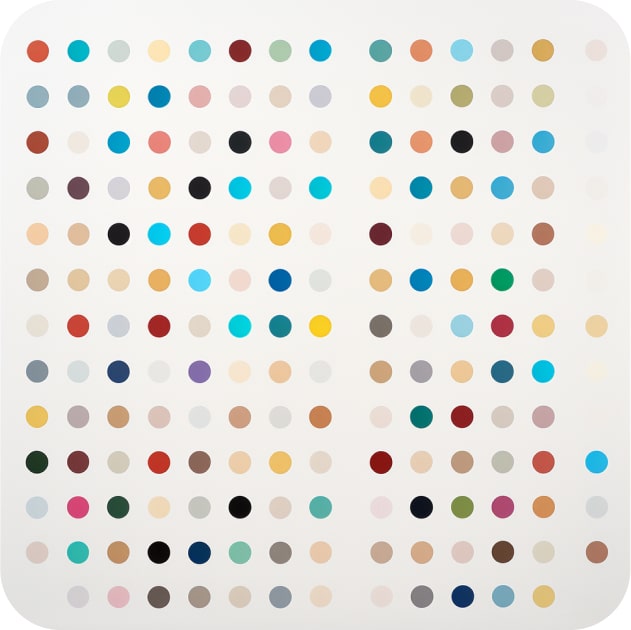
Clean or Cleanse
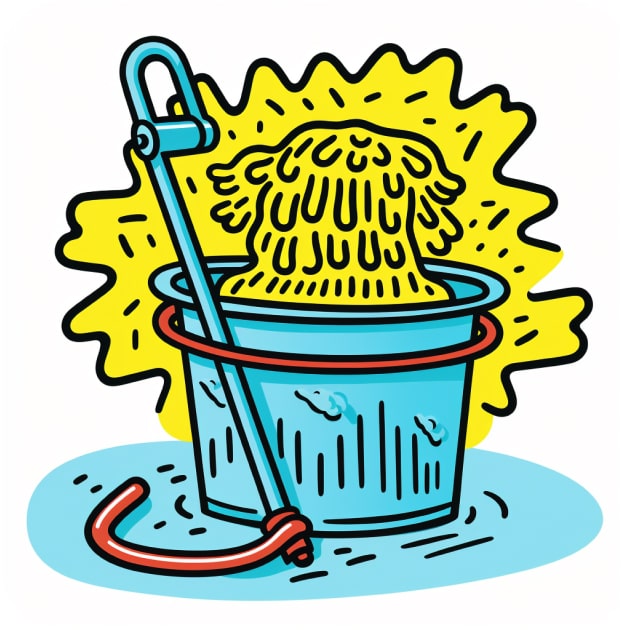
Cluster
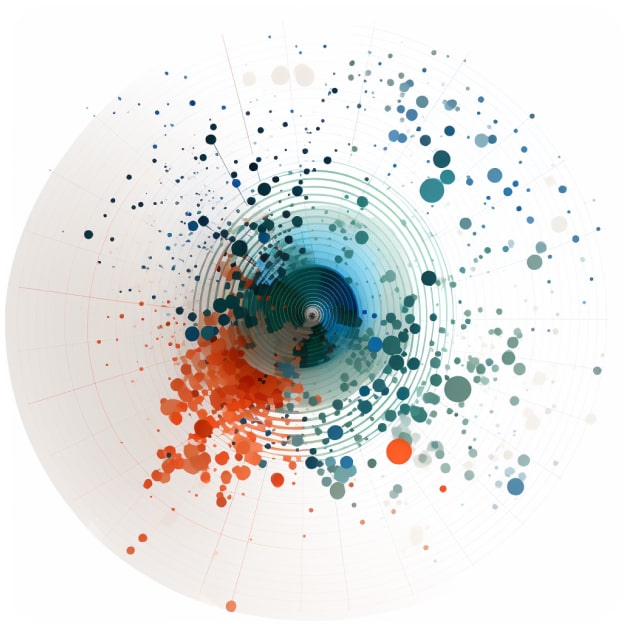
Curate
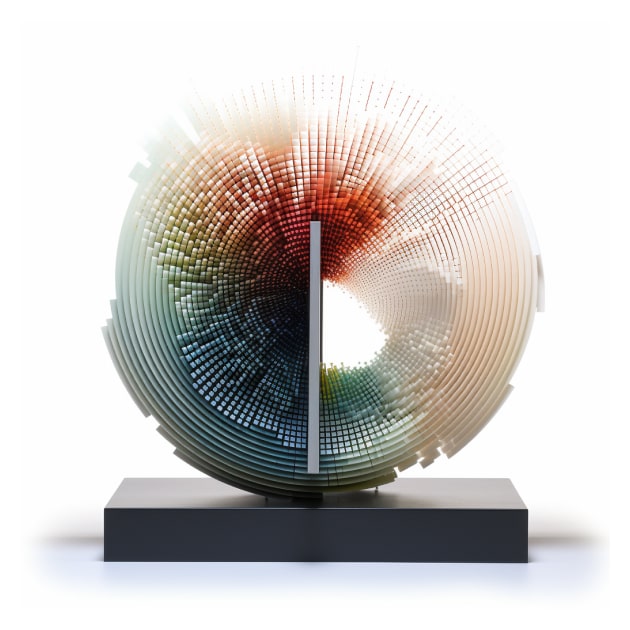
Denormalize
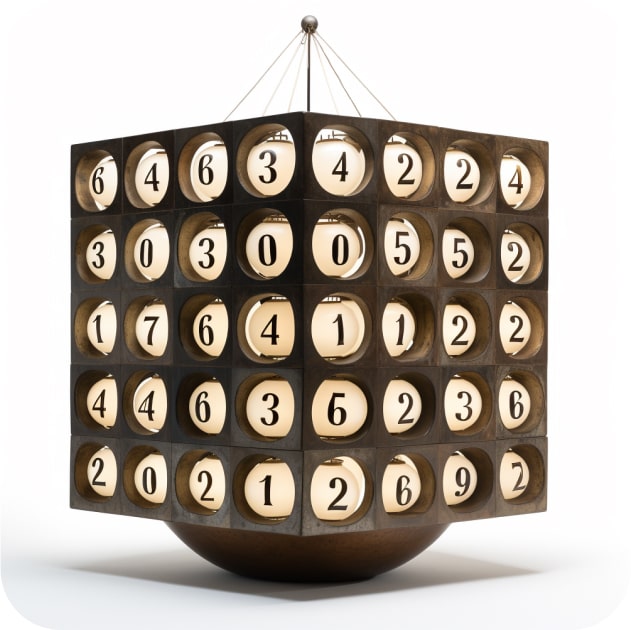
Derive
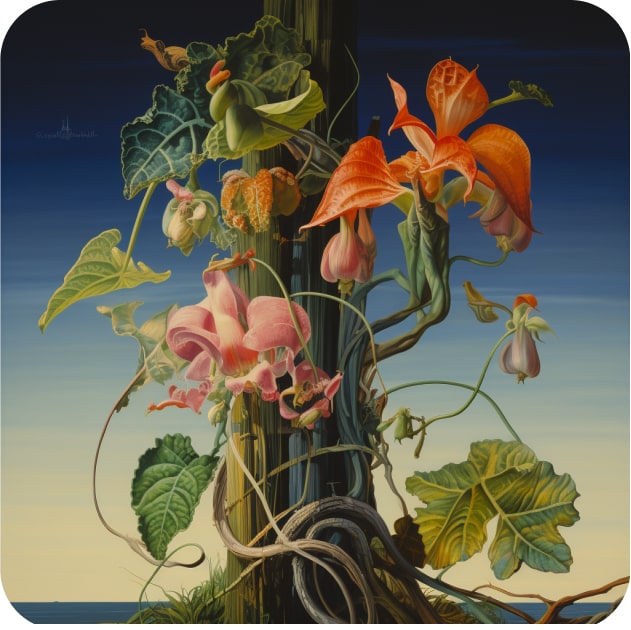
Discretize
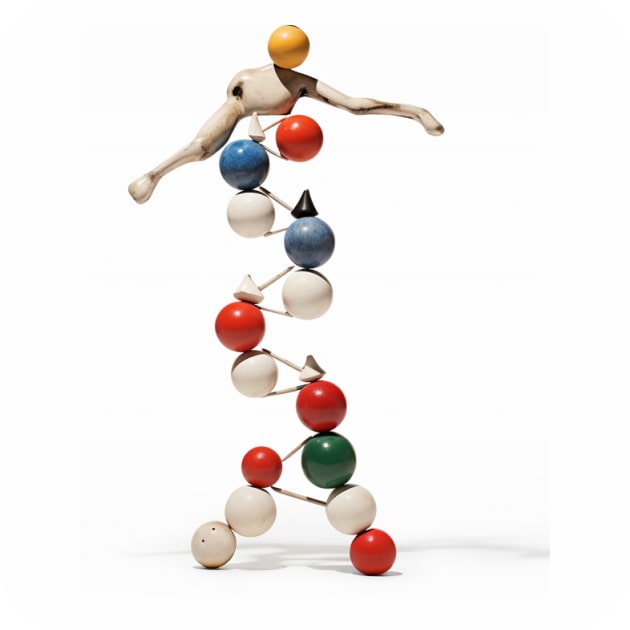
ETL
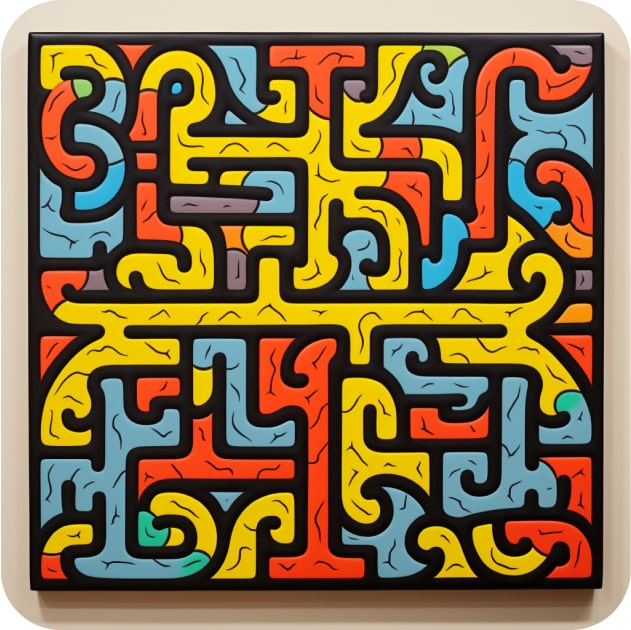
Encode
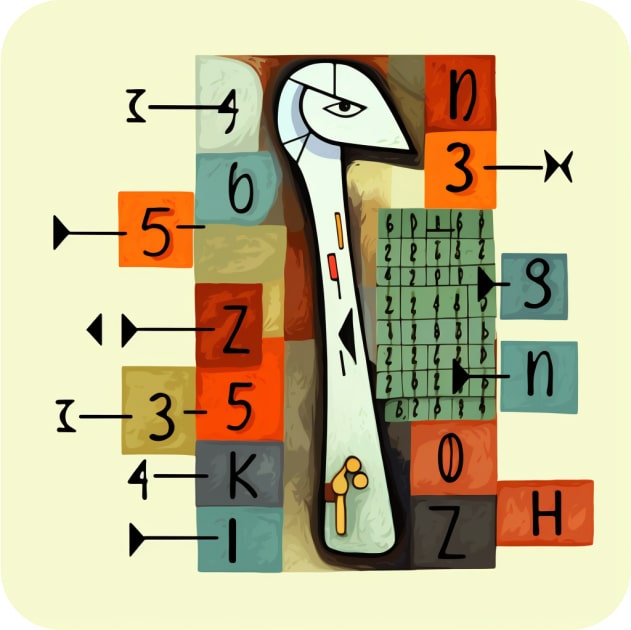
Filter
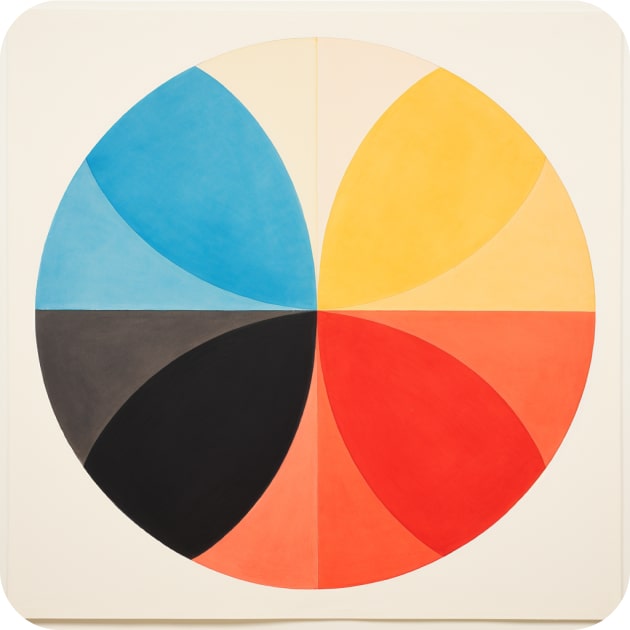
Fragment
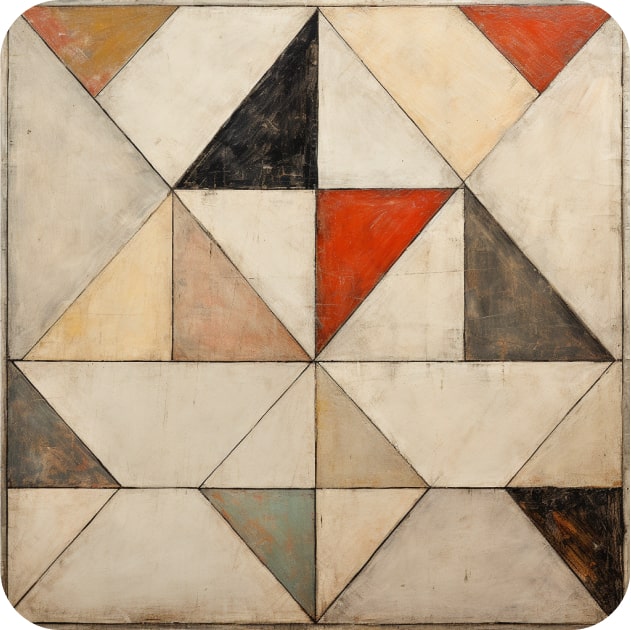
Homogenize
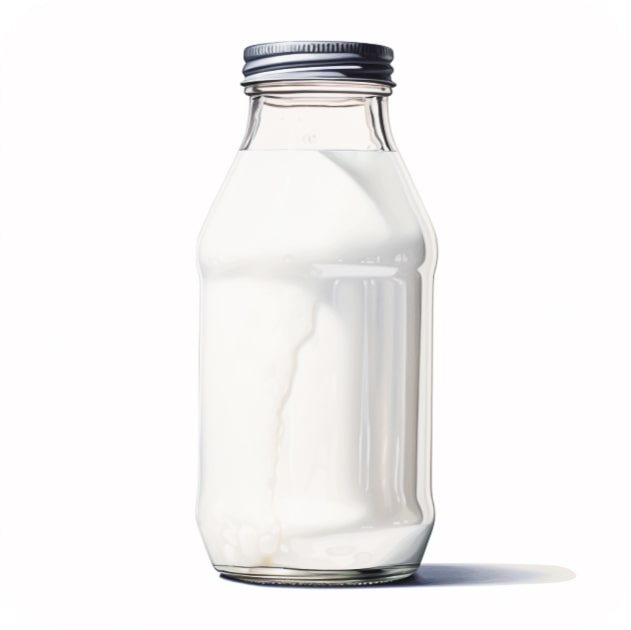
Impute
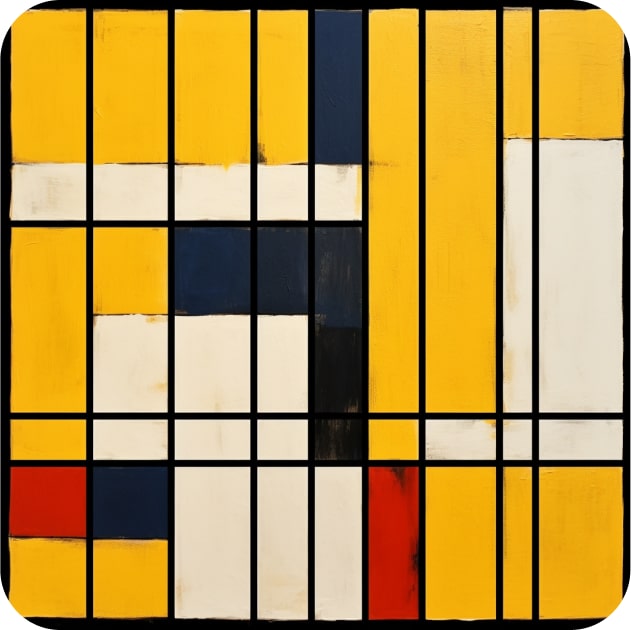
Linearize
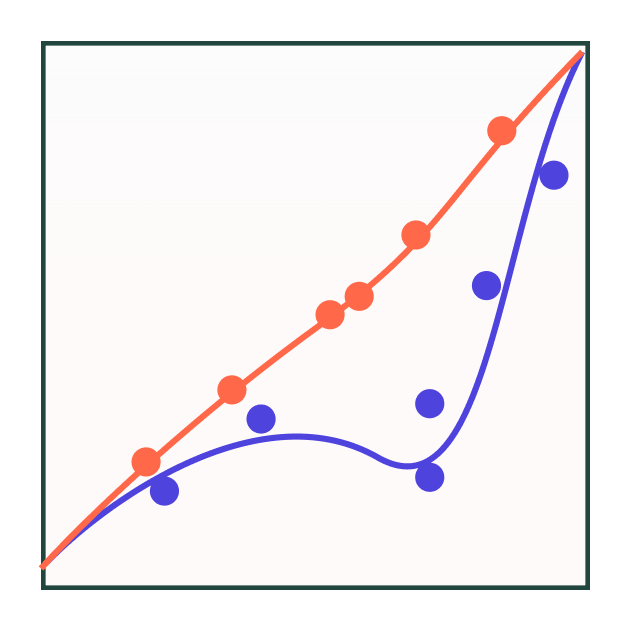
Munge
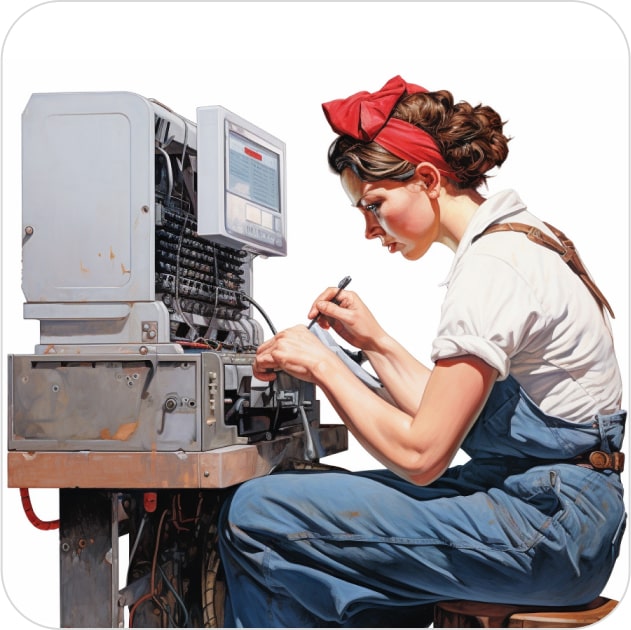
Normalize
Reduce
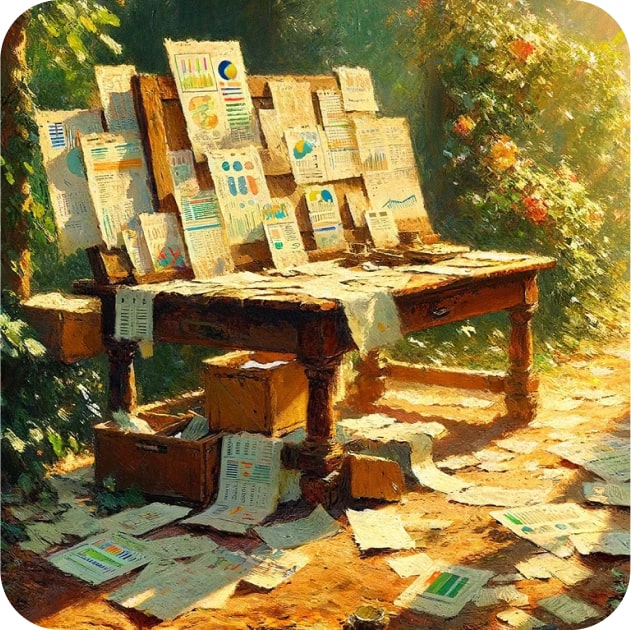
Reshape
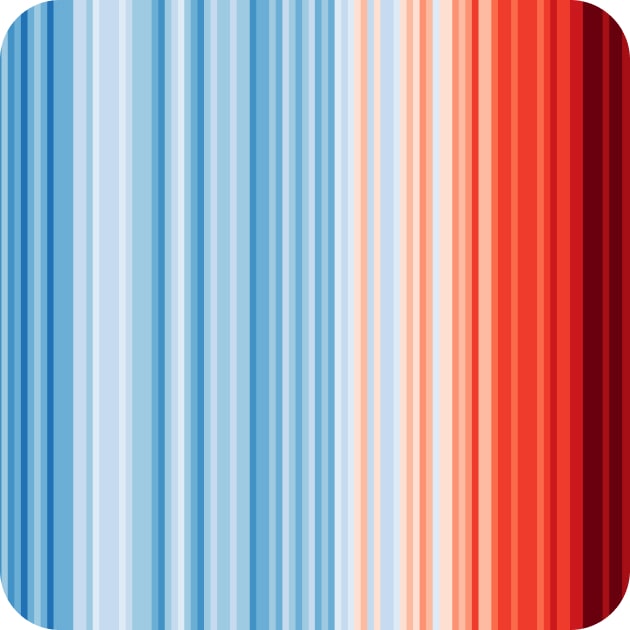
Serialize
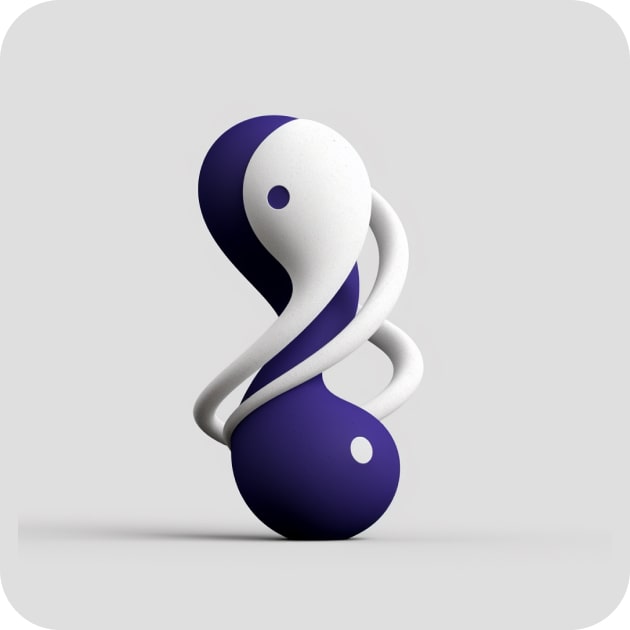
Shred
Skew
Split
Standardize
Tokenize
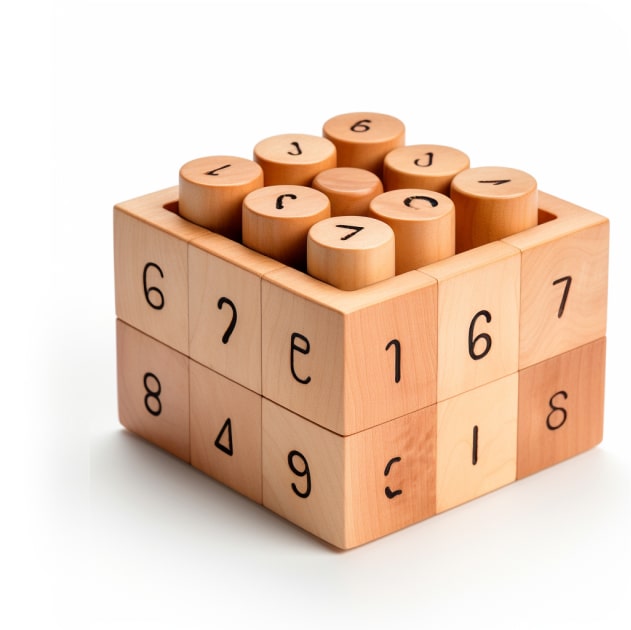
Transform
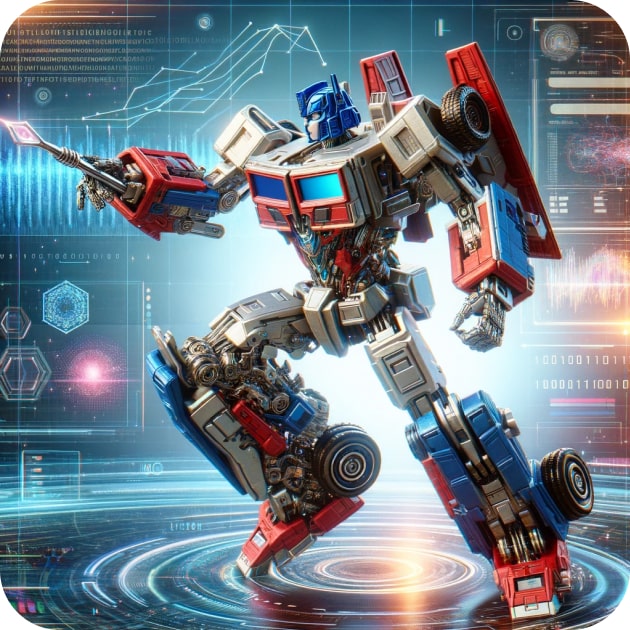
Wrangle
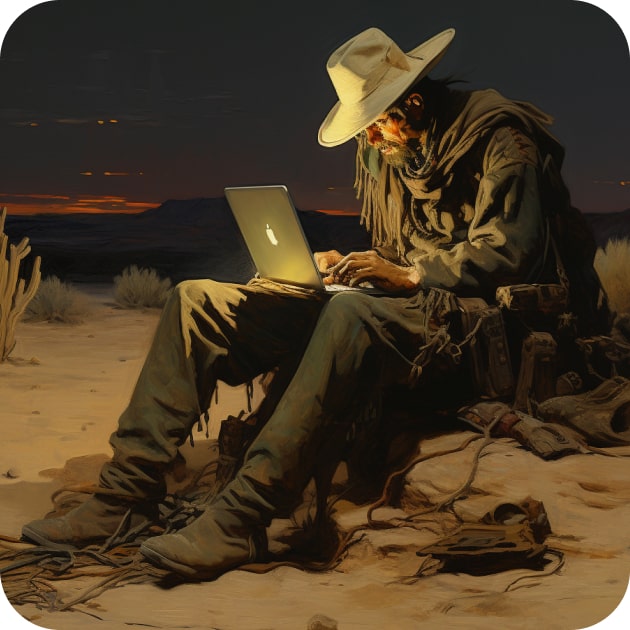