Dagster Data Engineering Glossary:
Data Homogenization
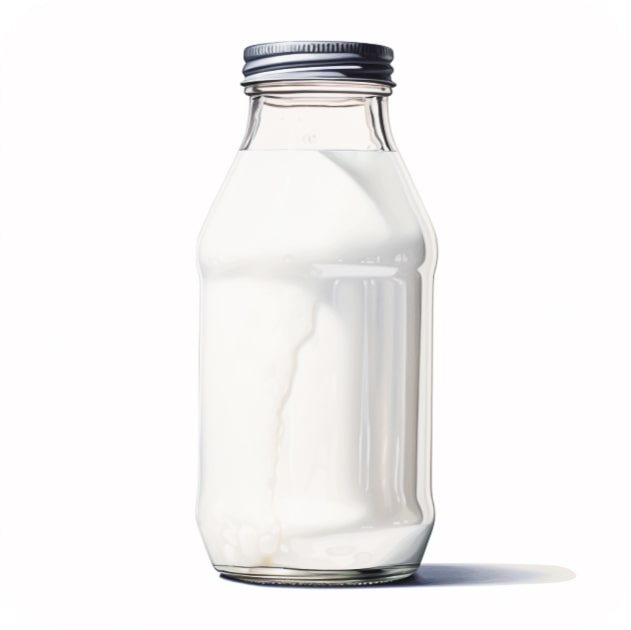
Data homogenization definition
Data homogenization is the process of making data uniform, consistent, and comparable. As data sources multiply and diversify, data can come in various formats, structures, and quality. When we talk about homogenizing data, we're talking about transforming and preparing this diverse data in a way that it becomes coherent and can be used seamlessly across applications, analyses, or systems.
Data homogenization is crucial in scenarios like data integration, business intelligence, analytics, and more, where coherent and consistent data is a requirement for accurate insights or smooth functioning of applications.
The many steps of data homogenization
Here's a breakdown of what this process might involve:
Standardization: This is about making sure data adheres to a common standard. For instance, date values from one system might be in the format
MM-DD-YYYY
while another usesYYYY-MM-DD
. Standardizing would involve picking one format and converting all dates to that format. See entry for standardizationNormalization: This is about scaling numerical data to fall within a specific range. For instance, one system might represent a percentage as a value between 0 and 1 (like 0.85) while another represents it between 0 and 100 (like 85). Normalizing would involve converting all these values to a chosen range. See entry for normalization.
Data Cleansing: This involves identifying and correcting (or removing) errors and inconsistencies in data to improve its quality. This could involve handling missing data, correcting typos or inaccuracies, and more. See entry for cleanse.
Schema Mapping: If you're integrating data from multiple databases, each with its schema, you might need to map fields between them. For example, one database might have a field called "mobilenumber", and another has "cell_phone". Homogenization would involve deciding on a consistent naming convention and structure. See entry for schema mapping_
Encoding and Categorization: Converting categorical data into a common format or encoding, like one-hot encoding, label encoding, etc. See entry for encode.
Addressing Variability: Sometimes data from different sources might refer to similar things but have slight variability. For instance, company names could be abbreviated in some systems and spelled out in others. Part of homogenization could involve making these consistent. See entry for integration.
Time Series Alignment: If you're dealing with time series data from different sources, they might have different granularities or time zones. Homogenization might involve resampling, aligning, and converting time zones to get consistent time series data. See entry for time series analysis.
Example of data homogenization in Python
Let's look at a scenario where you have to integrate sales data from two e-commerce platforms. Each platform provides data with various inconsistencies. We'll deal with:
- Different data formats
- Product categorization differences
- Currency conversions
- Data anomalies like outliers and missing values
We'll still use the pandas
library and also numpy
for some numerical operations.
import pandas as pd
import numpy as np
# Sample data
data1 = {
'Date': ['01-01-2023', '01-02-2023', '01-03-2023', '01-04-2023'],
'Product': ['Smartphone', 'Laptop', 'Tablet', 'Laptop'],
'Category': ['Electronics', 'Computers', 'Electronics', 'Computers'],
'Sales': [100, 200, 150, np.nan],
'Currency': ['USD', 'USD', 'USD', 'USD']
}
data2 = {
'Date': ['2023-01-01', '2023-01-02', '2023-01-03', '2023-01-04'],
'Product': ['SPhone', 'Lap', 'Tab', 'Desk'],
'Category': ['Mobile', 'Laptop', 'Tablet', 'Computer'],
'Sales': [120, 2500, 160, -5], # Sales in a different currency & has an outlier
'Currency': ['EUR', 'EUR', 'EUR', 'EUR']
}
df1 = pd.DataFrame(data1)
df2 = pd.DataFrame(data2)
# Convert date format in df2 to match df1
df2['Date'] = pd.to_datetime(df2['Date']).dt.strftime('%m-%d-%Y')
# Standardize product names using a mapping dictionary
product_map = {
'SPhone': 'Smartphone',
'Lap': 'Laptop',
'Tab': 'Tablet',
'Desk': 'Desktop'
}
df2['Product'] = df2['Product'].map(product_map)
# Map categories
category_map = {
'Mobile': 'Electronics',
'Laptop': 'Computers',
'Tablet': 'Electronics',
'Computer': 'Computers'
}
df2['Category'] = df2['Category'].map(category_map)
# Handling currency conversion
# Let's assume 1 EUR = 1.1 USD for simplicity
currency_conversion = {
'EUR': 1.1
}
df2['Sales'] = df2.apply(lambda row: row['Sales'] * currency_conversion[row['Currency']] if row['Currency'] in currency_conversion else row['Sales'], axis=1)
df2['Currency'] = 'USD'
# Handle missing values in df1 by forward filling for simplicity
df1['Sales'].fillna(method='ffill', inplace=True)
# Handle outliers (negative sales, which is an anomaly)
df2['Sales'] = df2['Sales'].apply(lambda x: np.nan if x < 0 else x)
df2['Sales'].fillna(method='ffill', inplace=True) # forward fill for simplicity
# Merge dataframes
combined_data = pd.concat([df1, df2]).reset_index(drop=True)
print(combined_data)
In this example:
- We've addressed different date formats.
- Standardized product names and categories.
- Converted EUR to USD.
- Handled missing values using forward fill.
- Managed sales anomalies by replacing them with NaN and then using forward fill.
Try running this code on your own machine and you will see this result - a homogenized dataset:
Date Product Category Sales Currency
0 01-01-2023 Smartphone Electronics 100.0 USD
1 01-02-2023 Laptop Computers 200.0 USD
2 01-03-2023 Tablet Electronics 150.0 USD
3 01-04-2023 Laptop Computers 150.0 USD
4 01-01-2023 Smartphone Electronics 132.0 USD
5 01-02-2023 Laptop Computers 2750.0 USD
6 01-03-2023 Tablet Electronics 176.0 USD
7 01-04-2023 Desktop Computers 176.0 USD
In a more real-world setting, you might leverage external APIs for up-to-date currency conversion rates, use sophisticated imputation methods for missing values, and perhaps integrate statistical methods or machine learning models to handle anomalies more intelligently.
Align
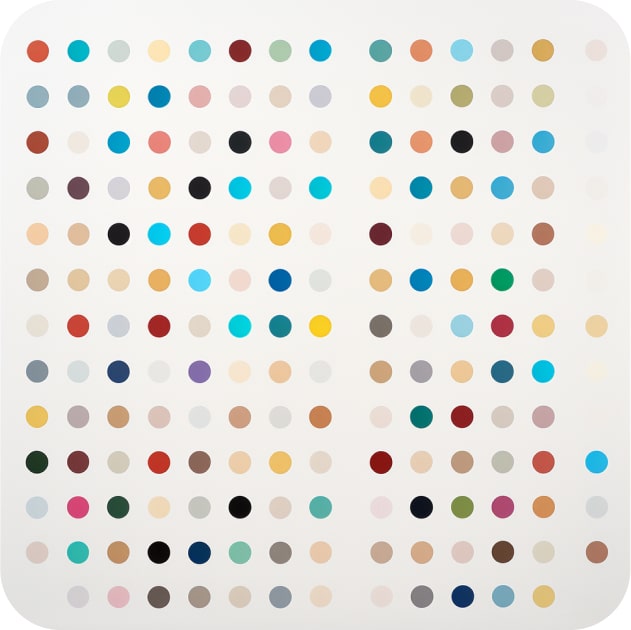
Clean or Cleanse
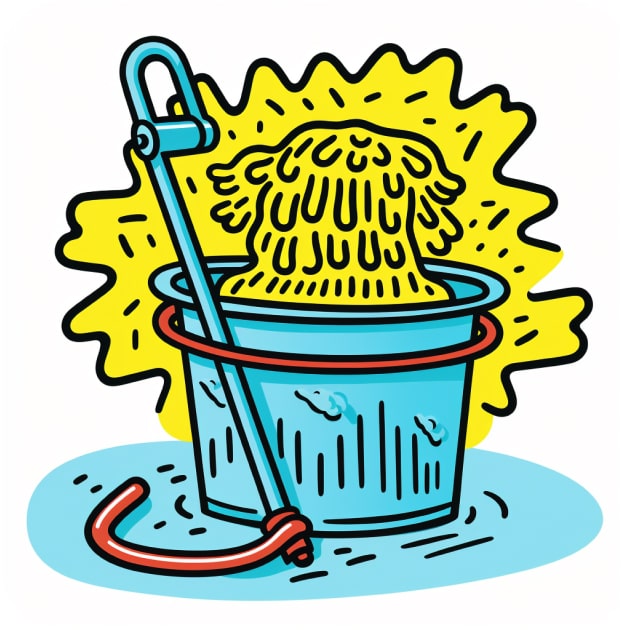
Cluster
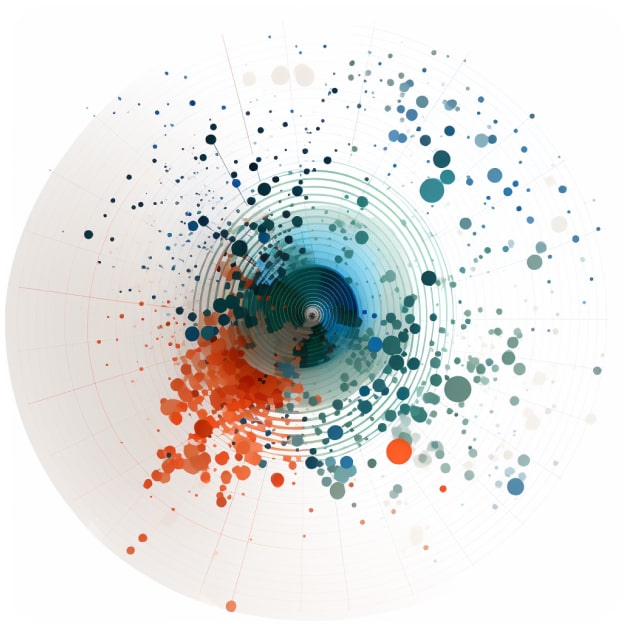
Curate
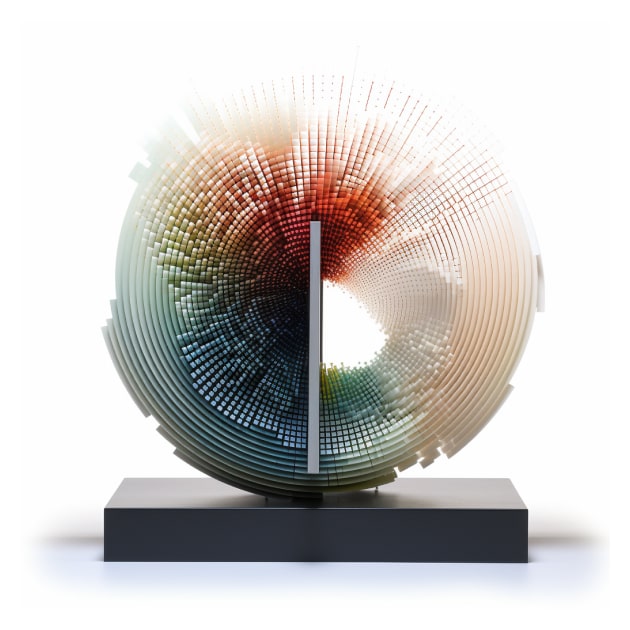
Denoise
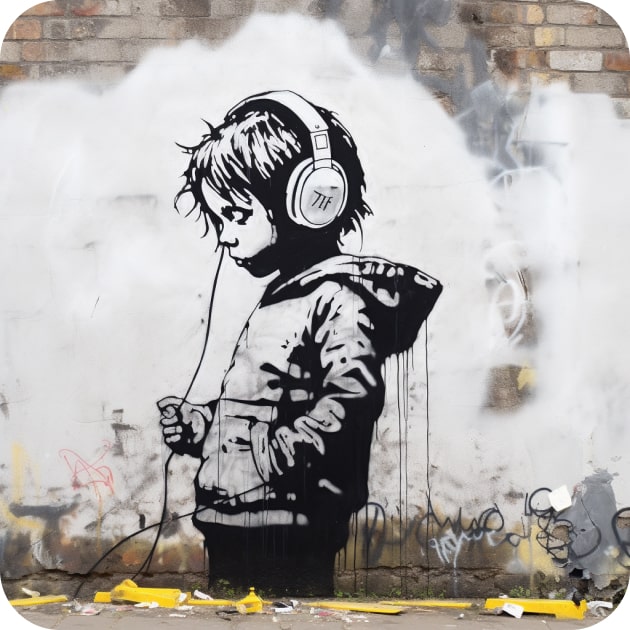
Denormalize
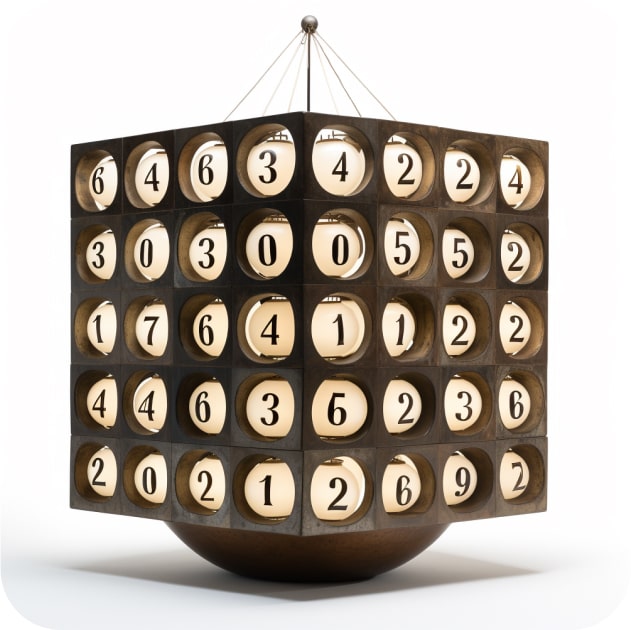
Derive
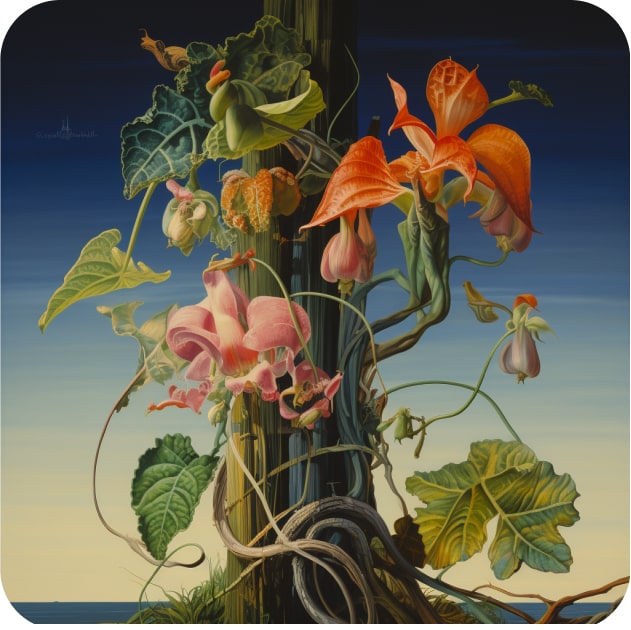
Discretize
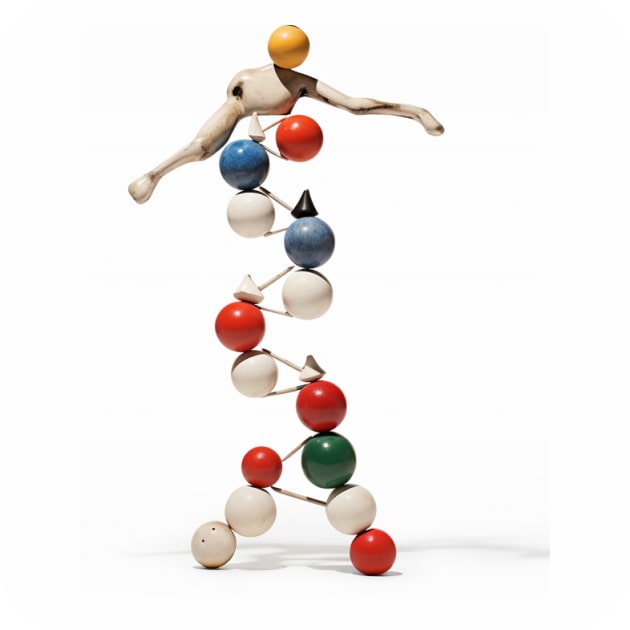
ETL
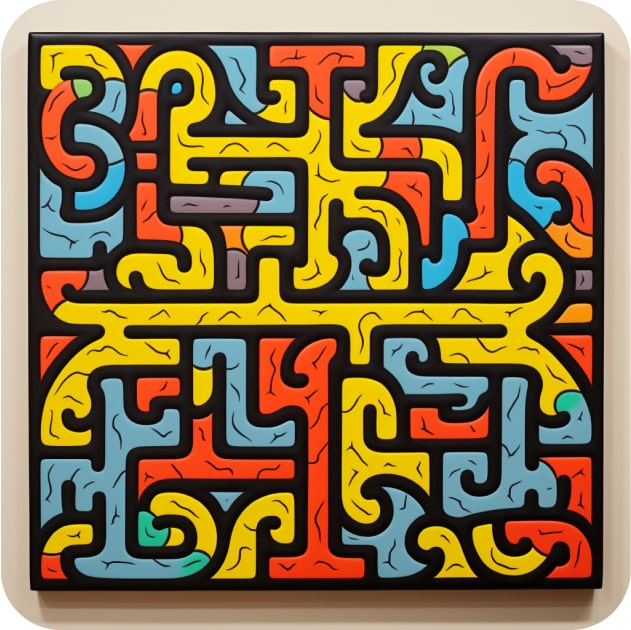
Encode
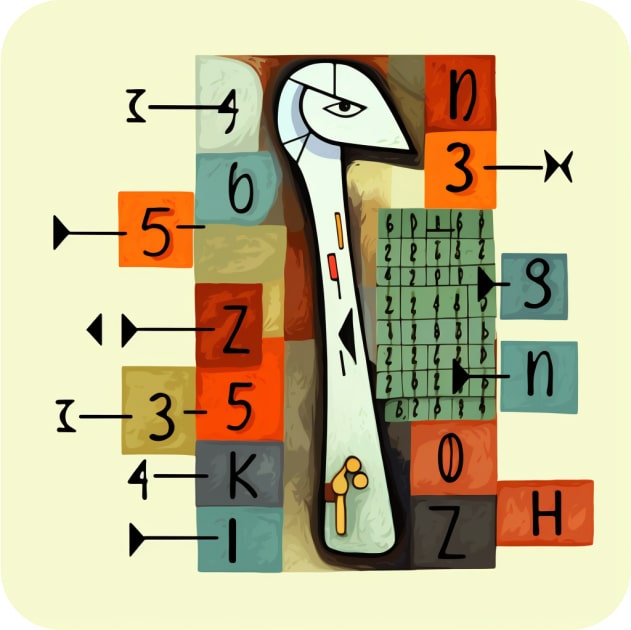
Filter
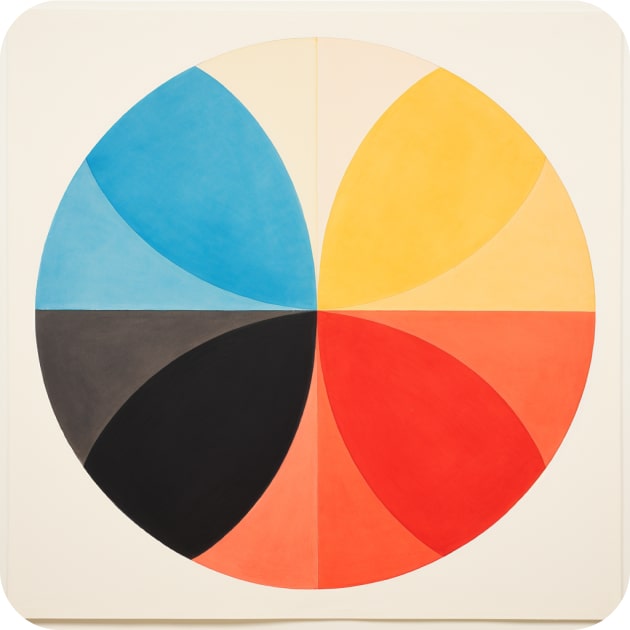
Fragment
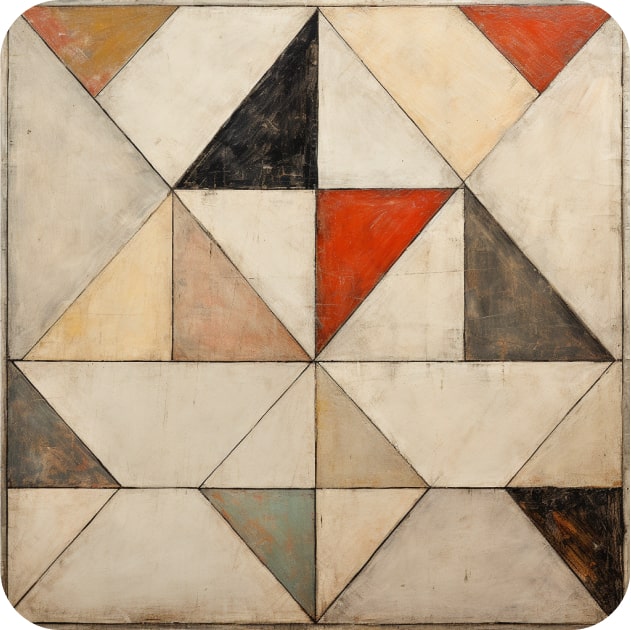
Impute
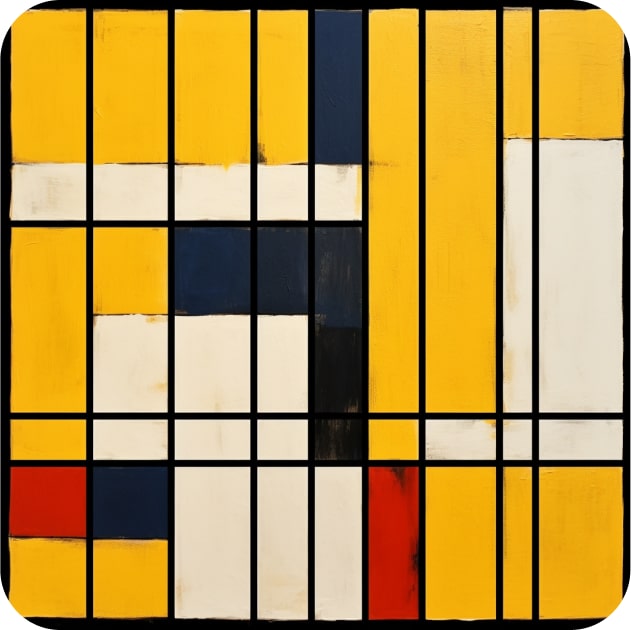
Linearize
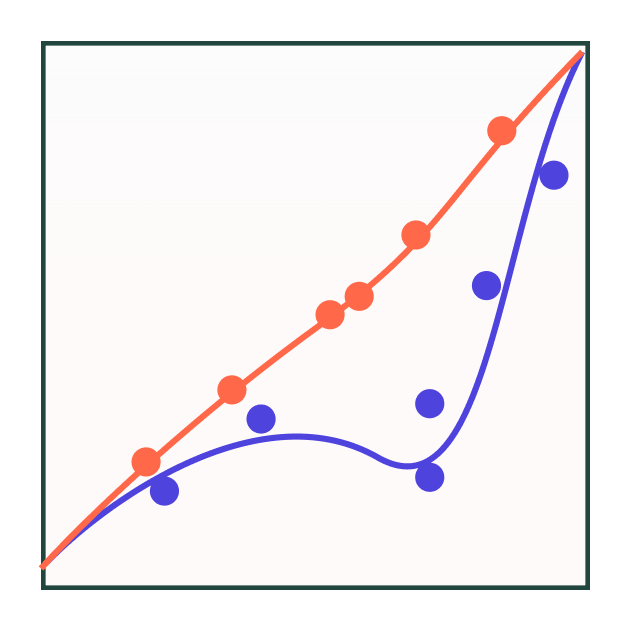
Munge
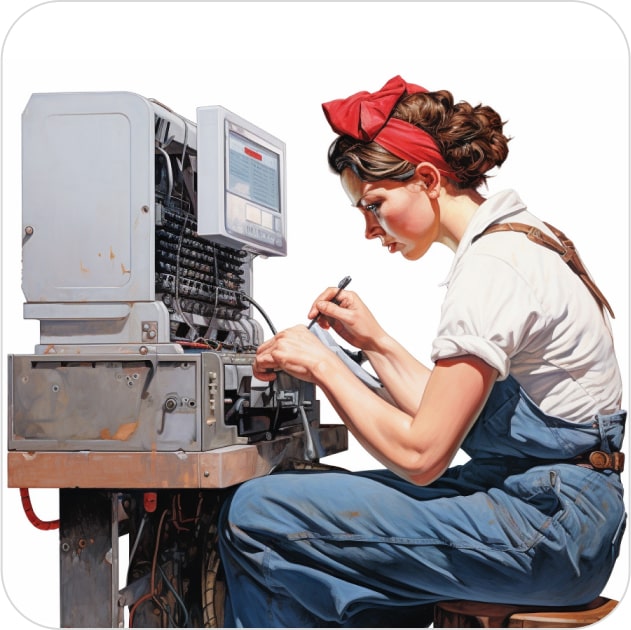
Normalize
Reduce
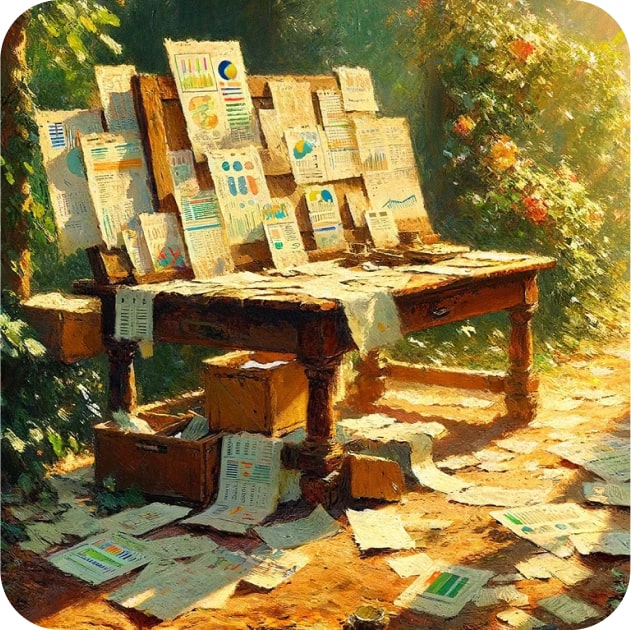
Reshape
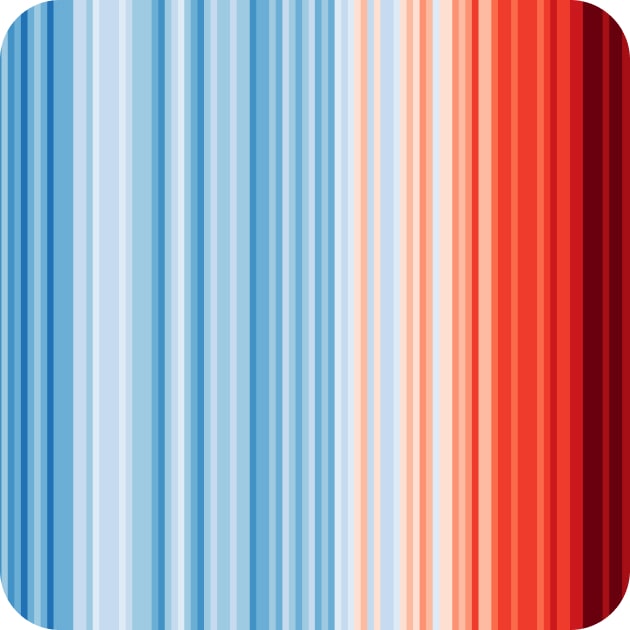
Serialize
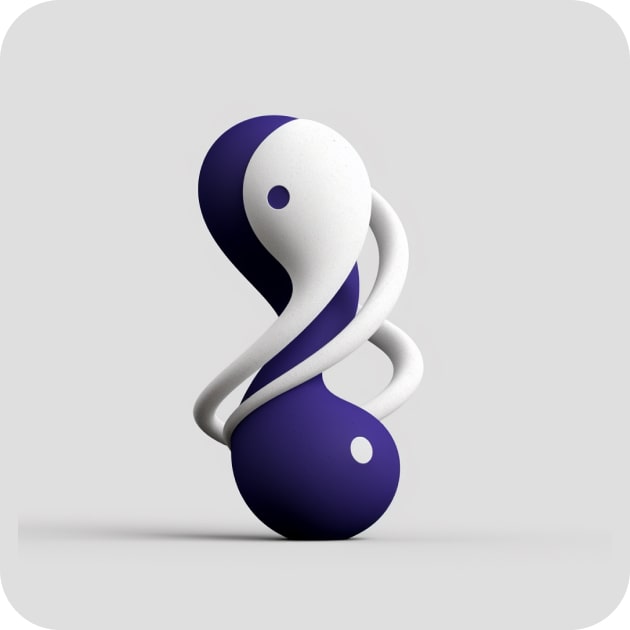
Shred
Skew
Split
Standardize
Tokenize
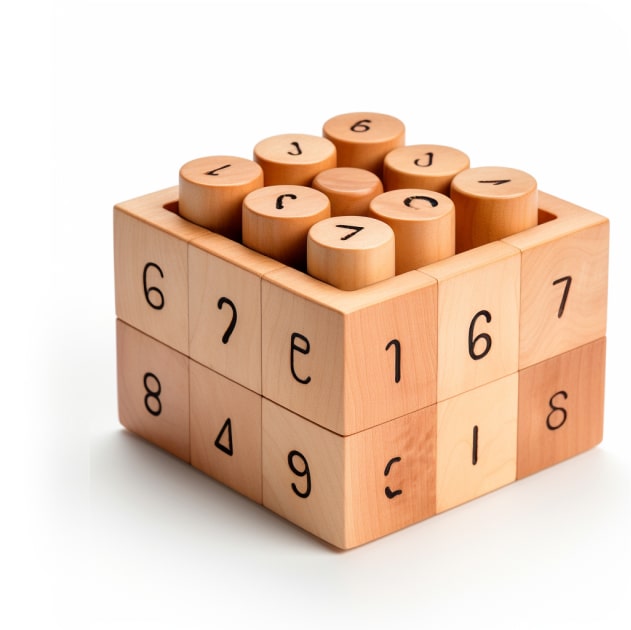
Transform
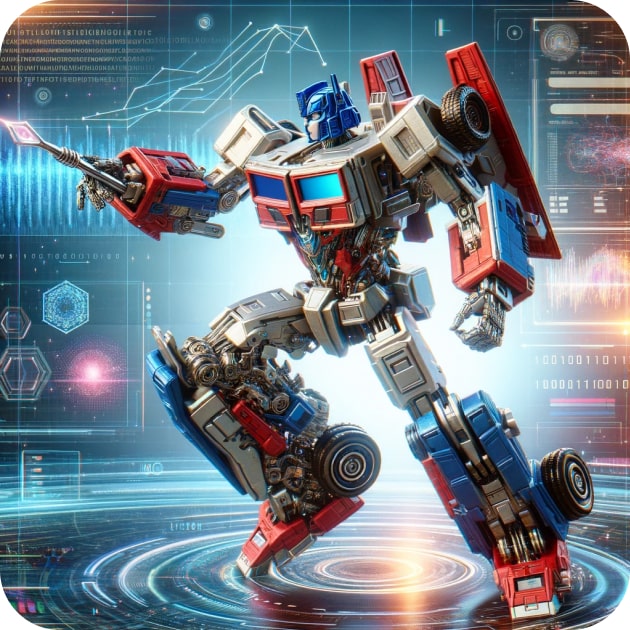
Wrangle
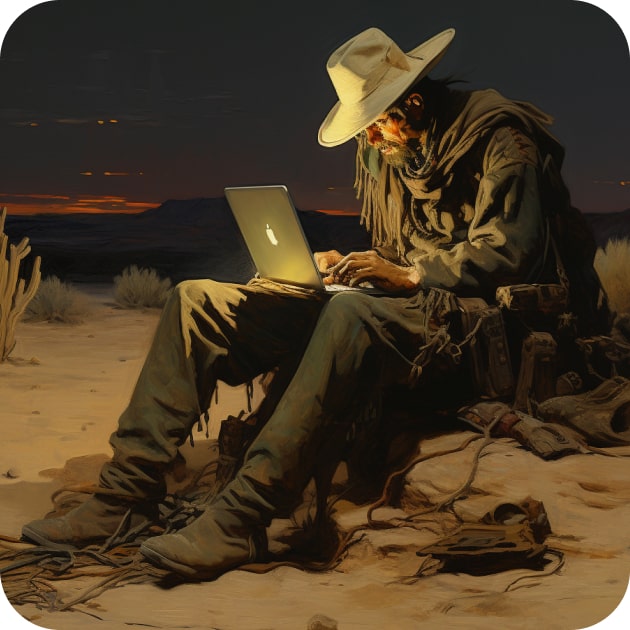