Dagster Data Engineering Glossary:
Data Encoding
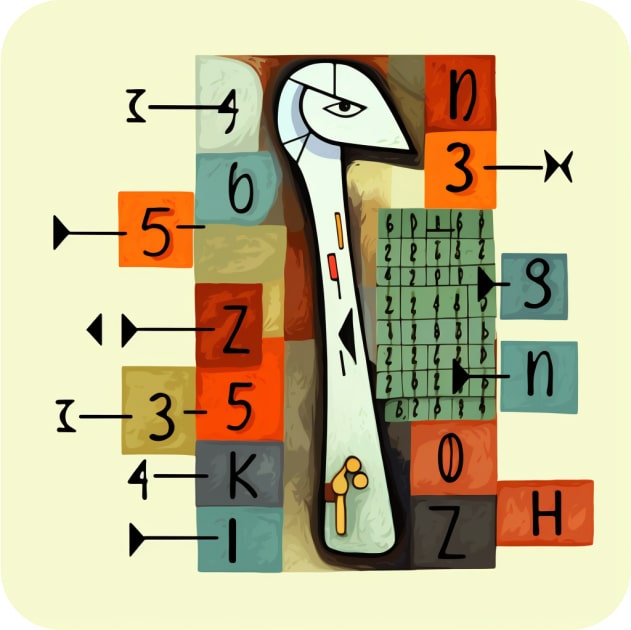
Data encoding definition:
Data Encoding refers to the converting of categorical variables into numerical representations that can be understood by machine learning algorithms. Common techniques include one-hot encoding, label encoding, or ordinal encoding.
Machine learning algorithms, especially traditional ones, are mathematical models that work on the basis of numeric computations and statistical operations. They require data to be represented in a numerical format to be able to establish any meaningful patterns or relationships within the data.
Categorical data, which might include labels like 'red', 'green', 'blue', can't be directly processed by these algorithms. To overcome this, categorical data is often encoded into a numerical form.
There are different encoding methods like one-hot encoding, label encoding, ordinal encoding, etc., each with its own pros and cons. The encoding technique to use depends on the categorical variable properties (e.g., nominal or ordinal) and the specific machine learning algorithm being used.
Furthermore, some machine learning models can handle categorical variables implicitly, such as decision trees and random forests. However, even in those cases, depending on the situation and the specific data set, it might be more efficient or yield better performance to manually encode categorical variables.
Lastly, categorical data often leads to high-dimensionality if not appropriately encoded. This is because a categorical variable with 'n' unique values might need to be transformed into 'n' binary variables, leading to an increase in the dimensionality of the data, a phenomenon known as "the curse of dimensionality", which can make learning more difficult for many algorithms.
Example of data encoding using Python
Let's look at the process of data encoding using one-hot encoding and label encoding, which are two commonly used encoding techniques in machine learning.
We'll be using the Python library pandas
for data manipulation and sklearn
for the encoding techniques. Please note that you may need to add the necessary Python libraries installed in your Python environment to run this code.
import pandas as pd
from sklearn.preprocessing import LabelEncoder, OneHotEncoder
# let's say we have some pipeline data like the following
data = {
'Region': ['North', 'West', 'East', 'South', 'North', 'West', 'West', 'East'],
'Product': ['Apples', 'Oranges', 'Bananas', 'Apples', 'Bananas', 'Bananas', 'Oranges', 'Apples'],
'Quantity': [100, 150, 200, 100, 300, 200, 150, 100]
}
df = pd.DataFrame(data)
# let's print the original dataframe
print("Original DataFrame:")
print(df)
# data encoding
# for simplicity, we'll use label encoding for the 'Region' column and one-hot encoding for the 'Product' column
# label encoding
le = LabelEncoder()
df['Region_encoded'] = le.fit_transform(df['Region'])
# one-hot encoding
ohe = OneHotEncoder(sparse_output=False)
ohe_results = ohe.fit_transform(df[['Product']])
ohe_df = pd.DataFrame(ohe_results, columns=ohe.get_feature_names_out(['Product']))
# concatenate original dataframe with the one-hot encoding dataframe
df = pd.concat([df, ohe_df], axis=1)
print("DataFrame after Encoding:")
print(df)
This Python script creates a simple dataframe from the given data. It then applies label encoding to the 'Region' column, where each unique region name is assigned an integer value. It also applies one-hot encoding to the 'Product' column, creating a new binary column for each unique product.
The resulting dataframe contains the original data, as well as the encoded 'Region' and 'Product' data.
Here is our output:
Original DataFrame:
Region Product Quantity
0 North Apples 100
1 West Oranges 150
2 East Bananas 200
3 South Apples 100
4 North Bananas 300
5 West Bananas 200
6 West Oranges 150
7 East Apples 100
DataFrame after Encoding:
Region Product Quantity Region_encoded Product_Apples Product_Bananas Product_Oranges
0 North Apples 100 1 1.0 0.0 0.0
1 West Oranges 150 3 0.0 0.0 1.0
2 East Bananas 200 0 0.0 1.0 0.0
3 South Apples 100 2 1.0 0.0 0.0
4 North Bananas 300 1 0.0 1.0 0.0
5 West Bananas 200 3 0.0 1.0 0.0
6 West Oranges 150 3 0.0 0.0 1.0
7 East Apples 100 0 1.0 0.0 0.0
Encoding data is a crucial step in preparing categorical data for use in machine learning algorithms, as these algorithms typically require numerical input.
Align
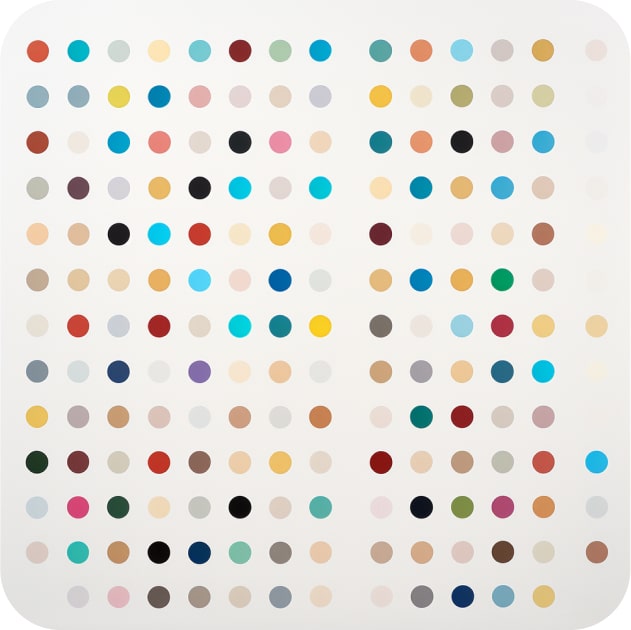
Clean or Cleanse
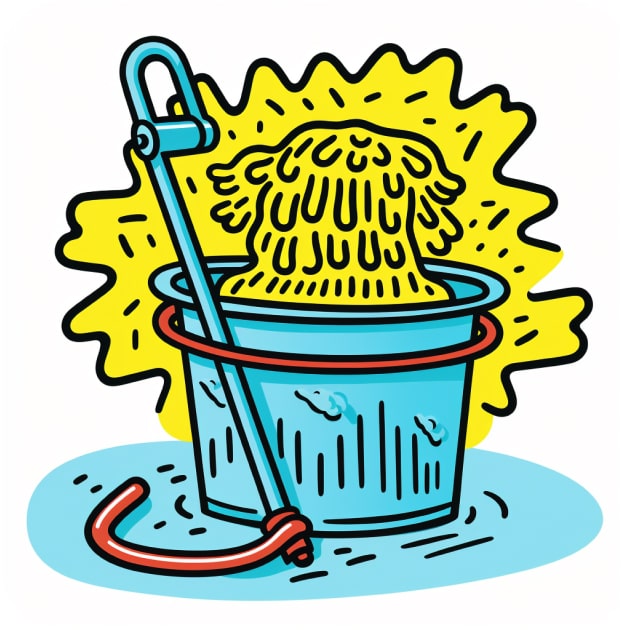
Cluster
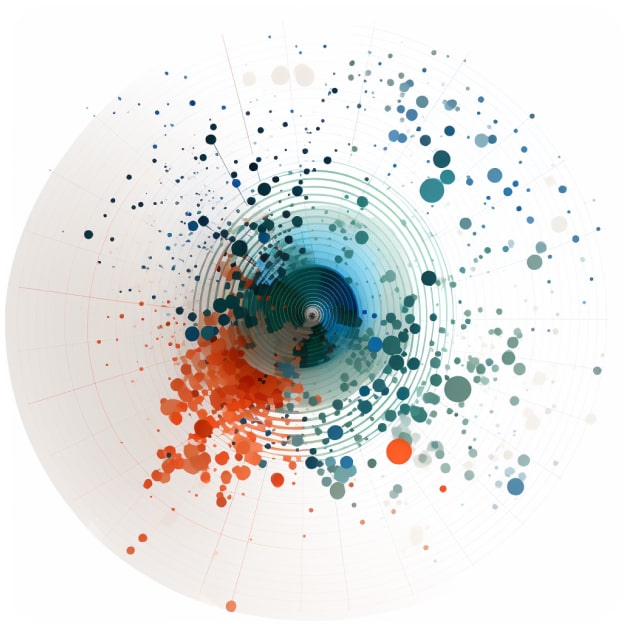
Curate
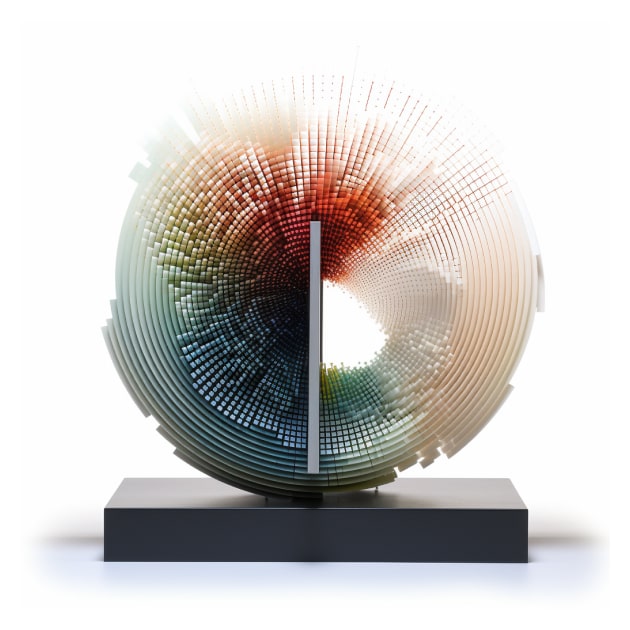
Denoise
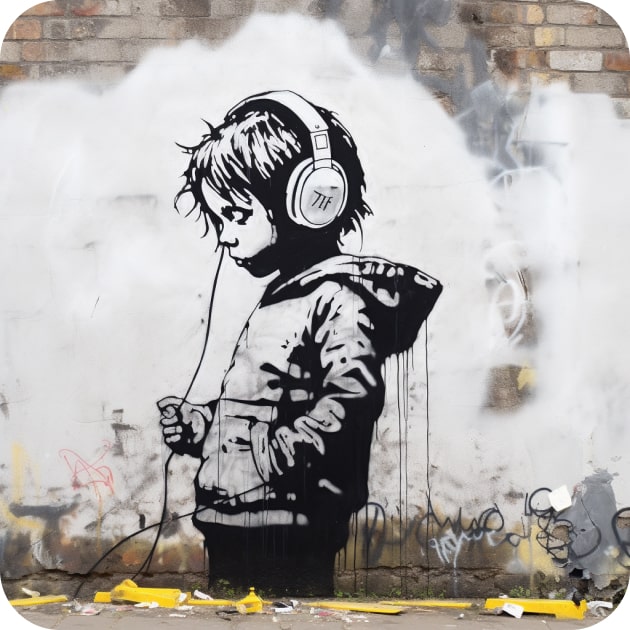
Denormalize
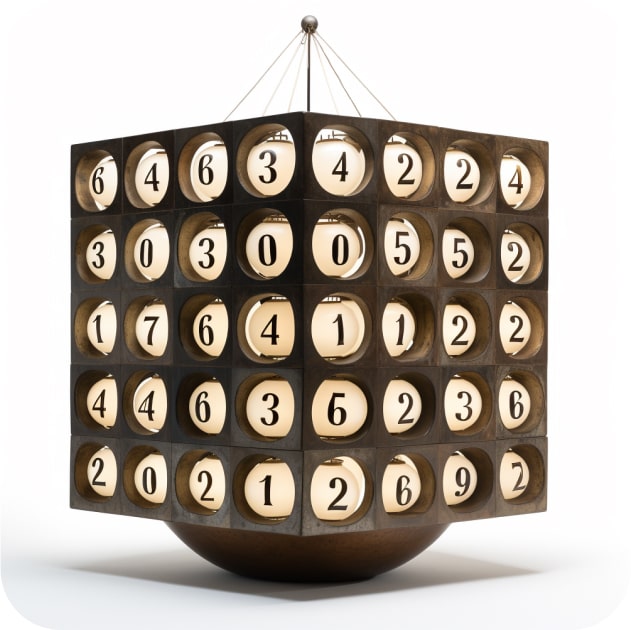
Derive
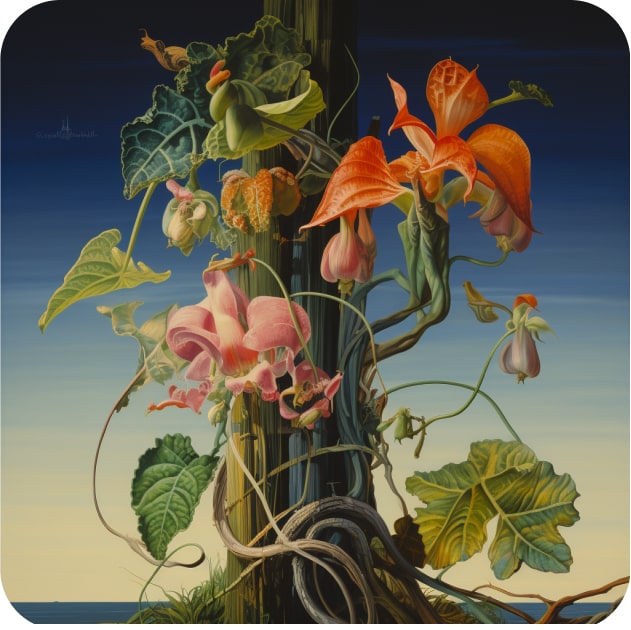
Discretize
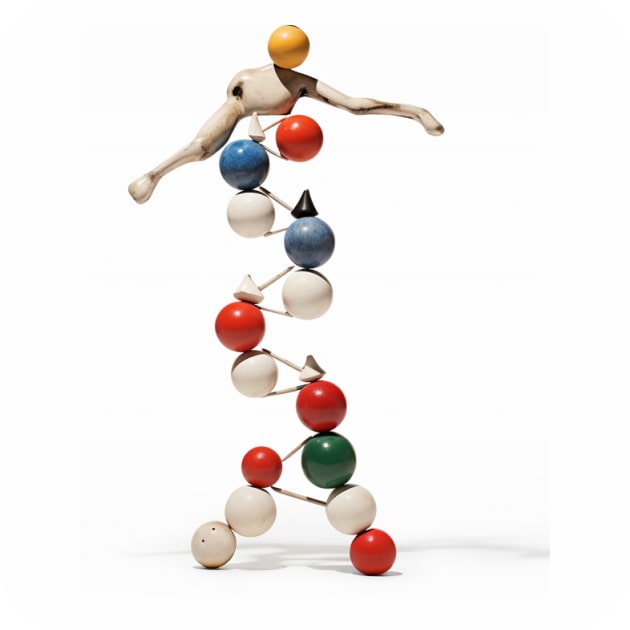
ETL
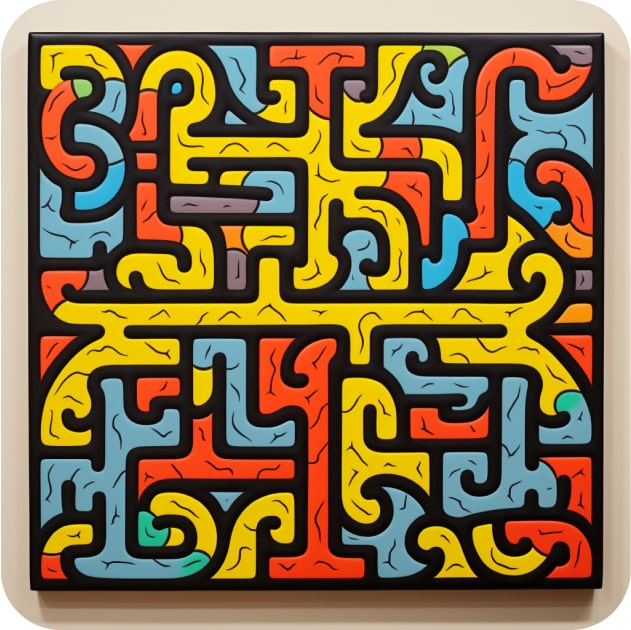
Filter
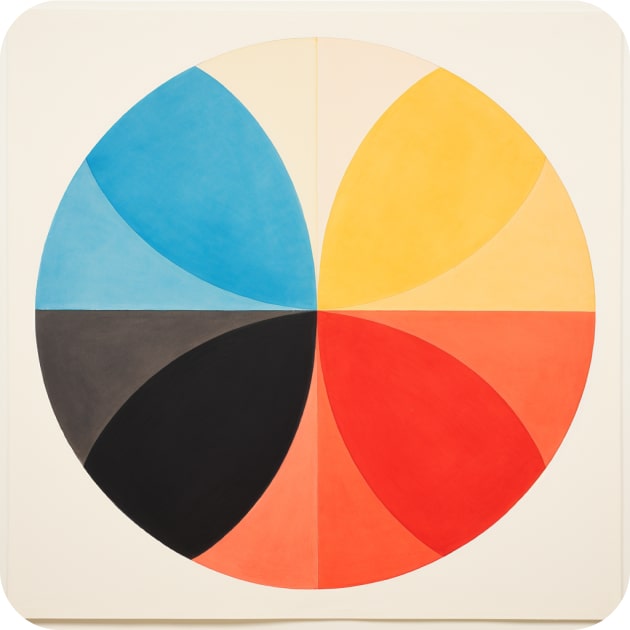
Fragment
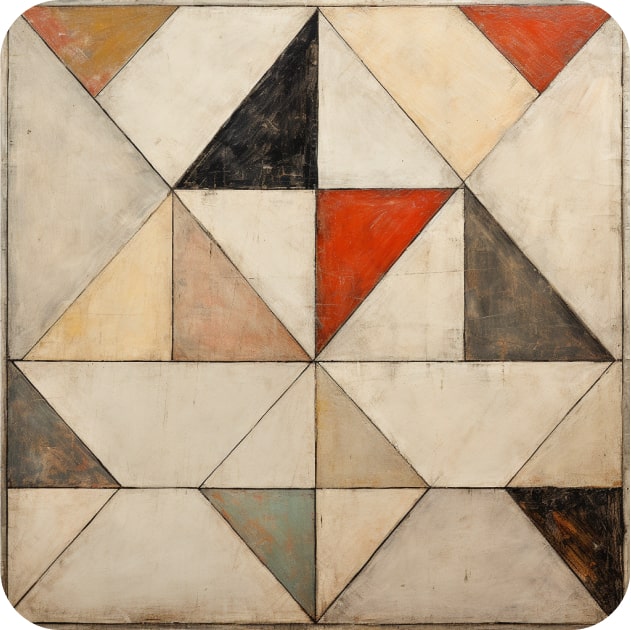
Homogenize
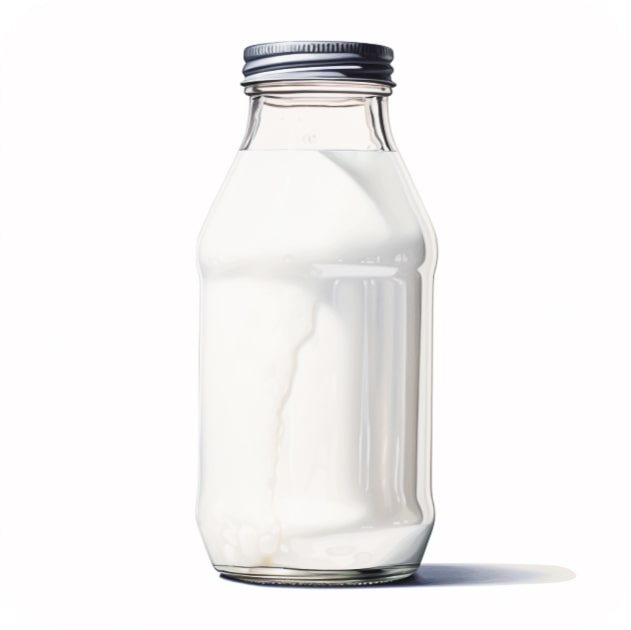
Impute
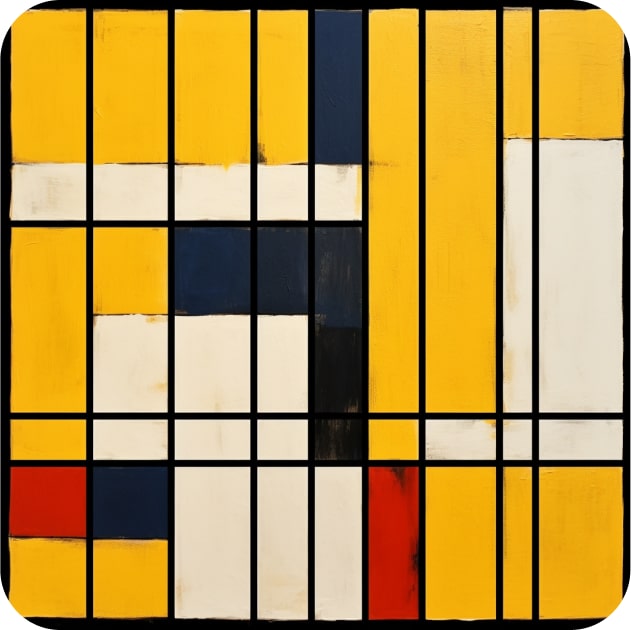
Linearize
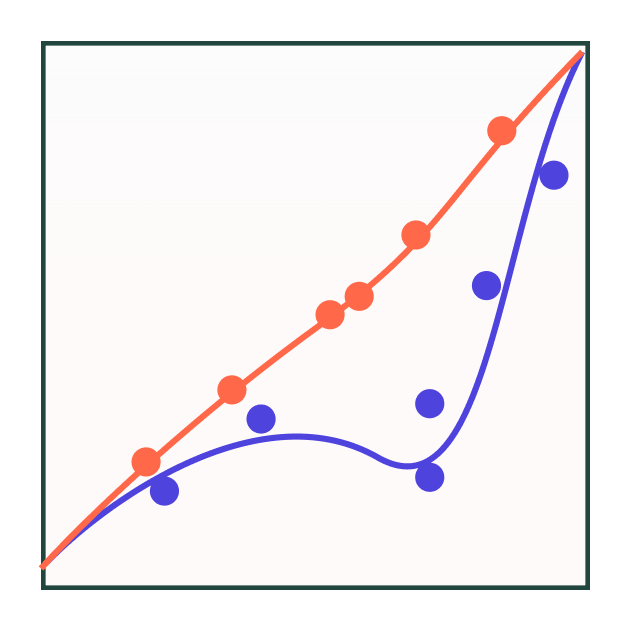
Munge
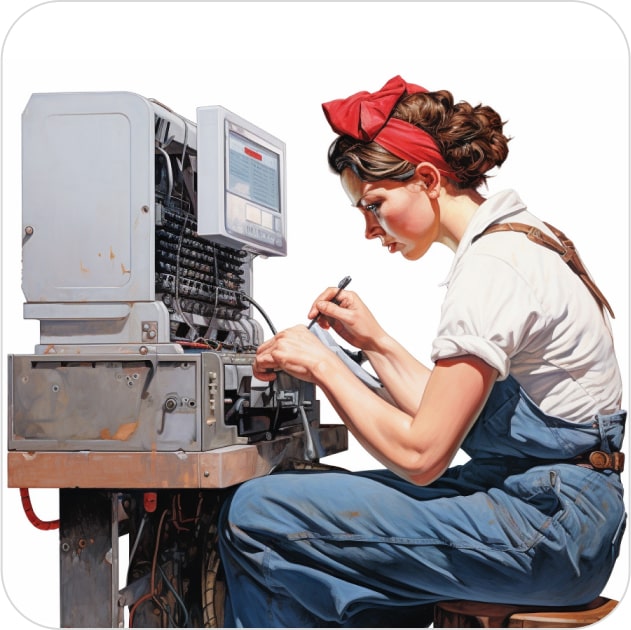
Normalize
Reduce
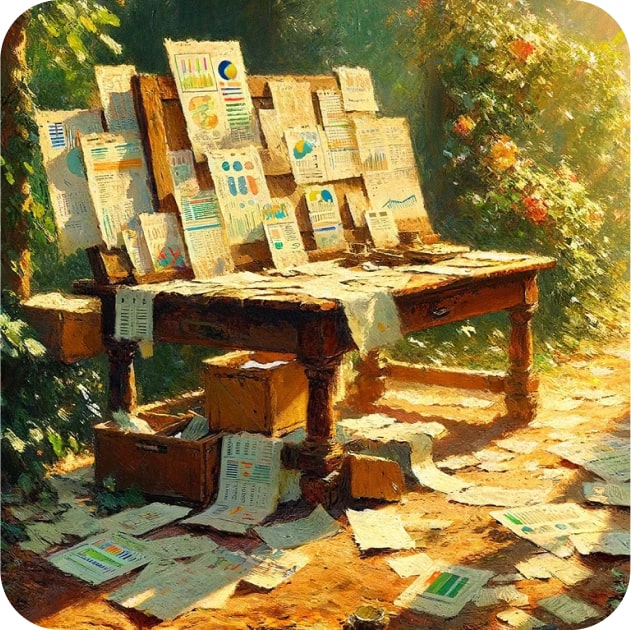
Reshape
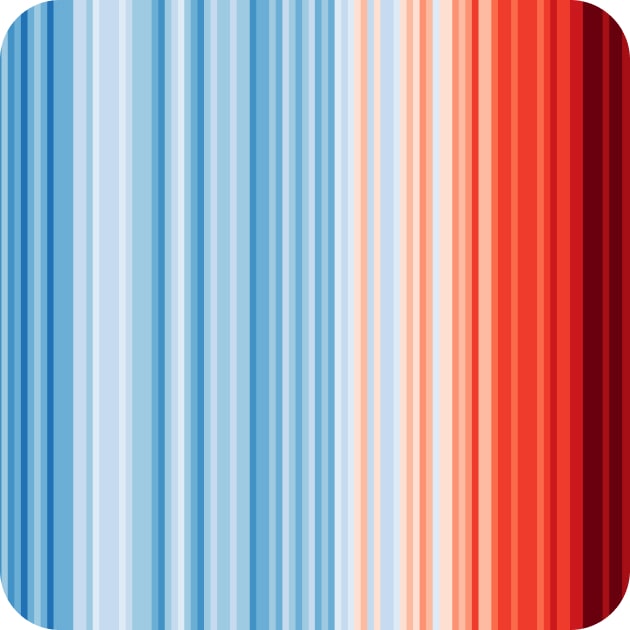
Serialize
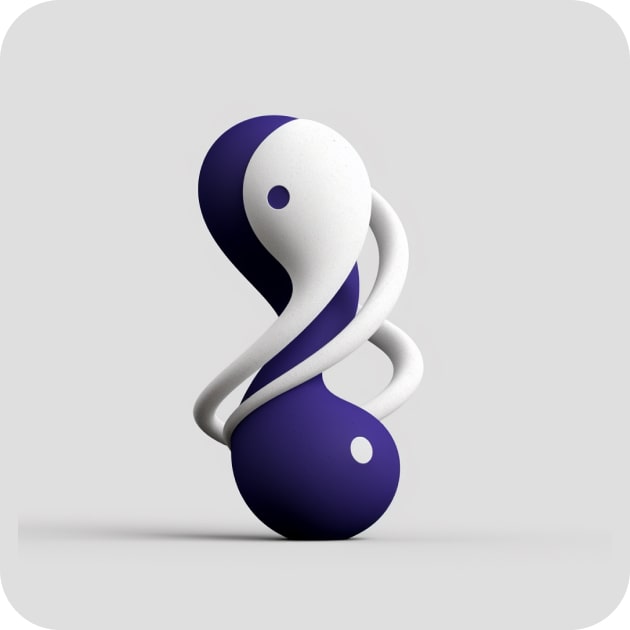
Shred
Skew
Split
Standardize
Tokenize
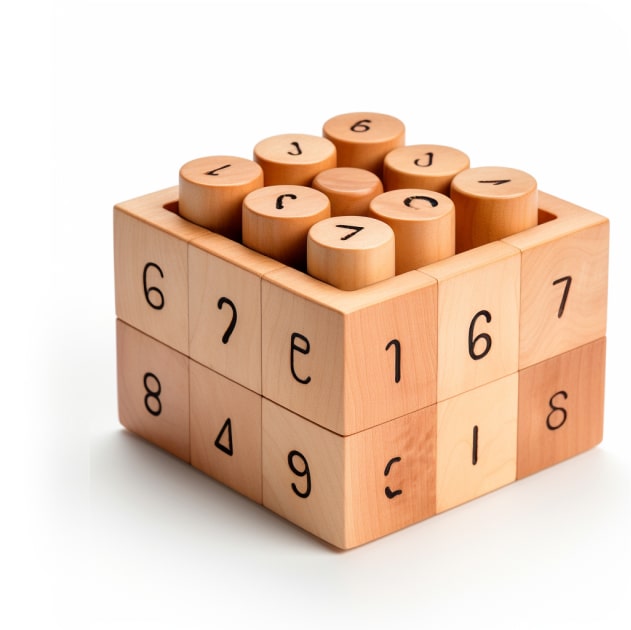
Transform
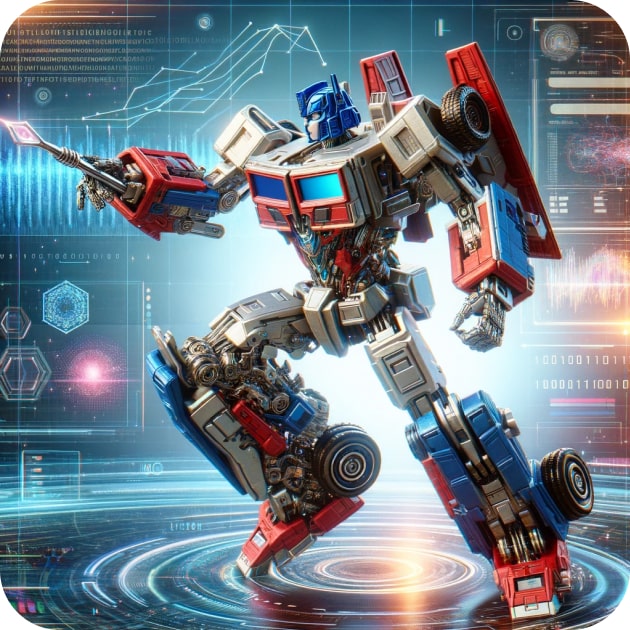
Wrangle
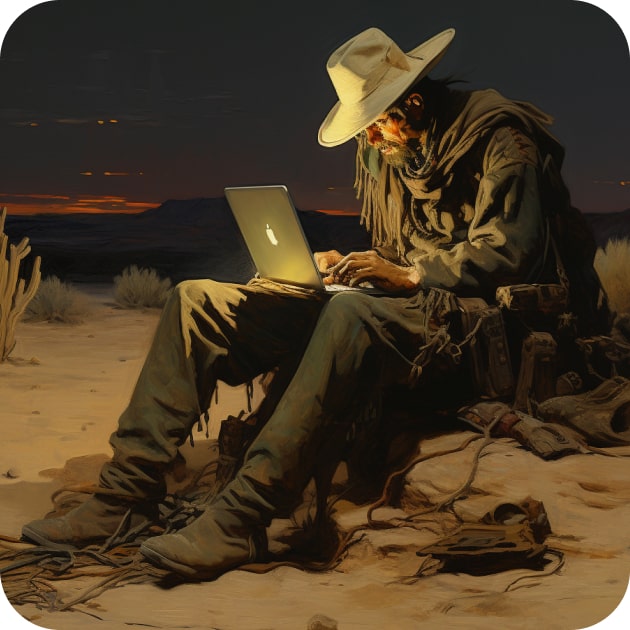