Dagster Data Engineering Glossary:
Data Reshaping
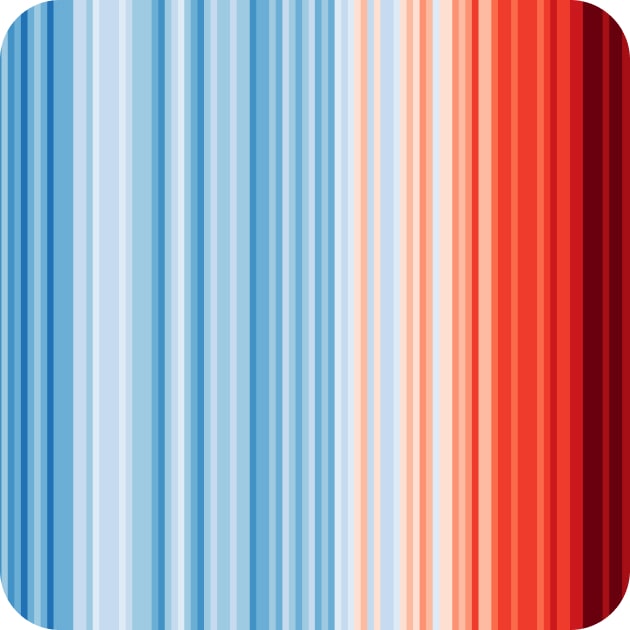
Data reshaping definition:
Data reshaping is the process of changing the layout or structure of data from one form to another, in order to better suit the needs of an analysis or downstream processing. This can involve rearranging rows and columns, changing the data type, or converting between wide and long formats.
Data transformation example using Python:
Please note that you need to have the necessary Python libraries installed in your Python environment to run this code.
In Python, the reshape()
function in the NumPy library can be used for data reshaping. Let’s look at a simple example:
import numpy as np
# create a 3x4 array of data
data = np.array([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
# reshape the data into a 2x6 array
reshaped_data = data.reshape(2, 6)
print(reshaped_data)
This will print out the new array as follows:
[[ 1 2 3 4 5 6]
[ 7 8 9 10 11 12]]
This example reshapes a 3x4 array into a 2x6 array. The reshape
function takes the new dimensions of the array as arguments. Note that the total number of elements in the original array must equal the total number of elements in the reshaped array, or an error will occur.
Align
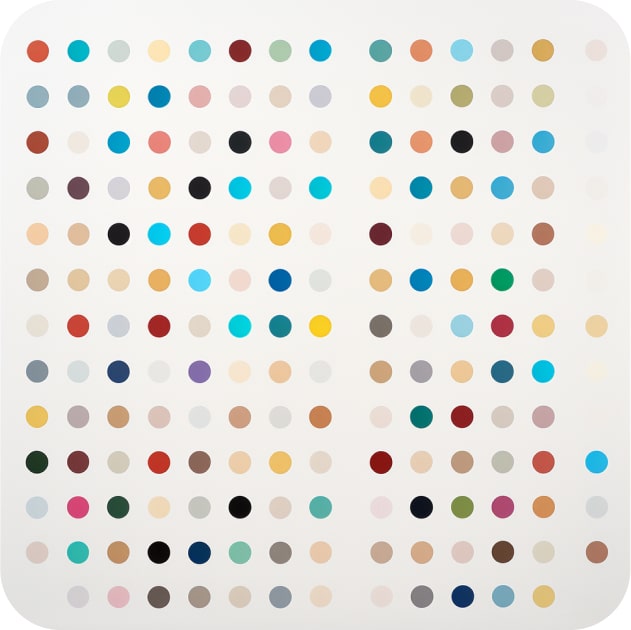
Clean or Cleanse
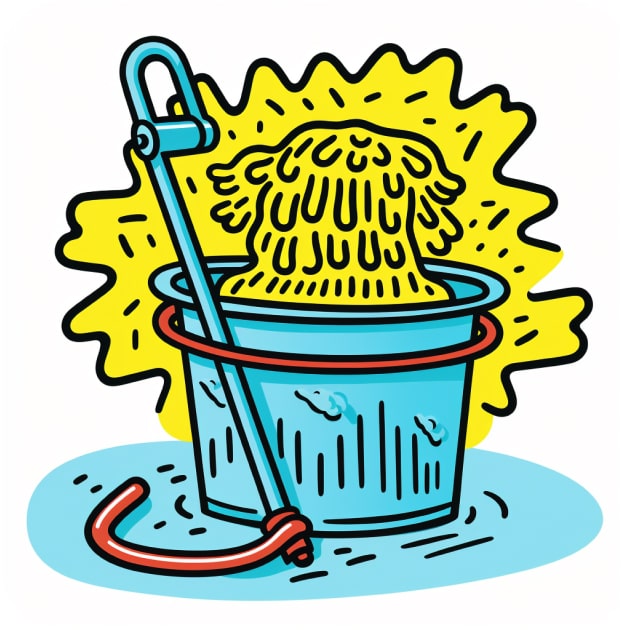
Cluster
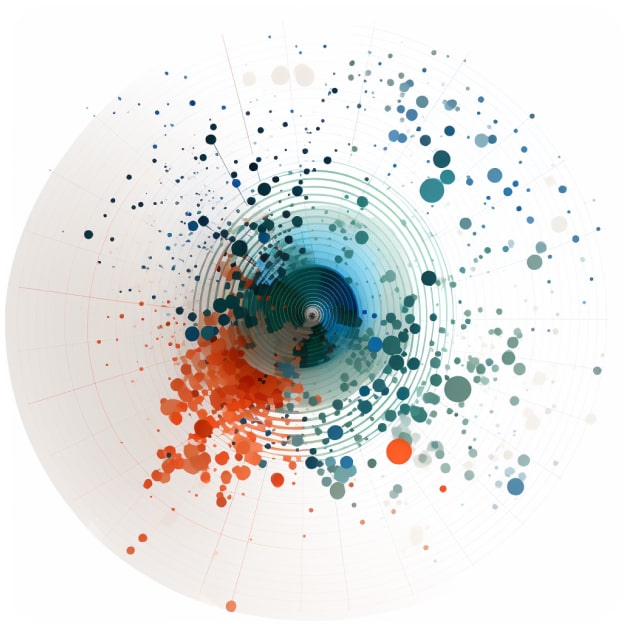
Curate
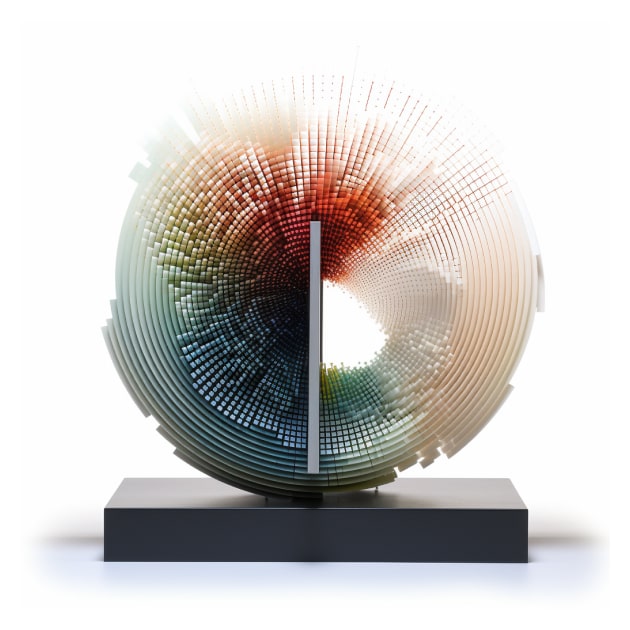
Denoise
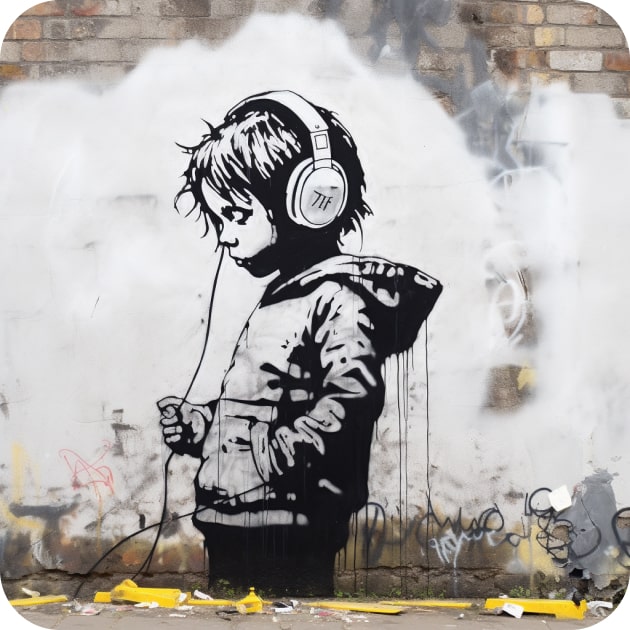
Denormalize
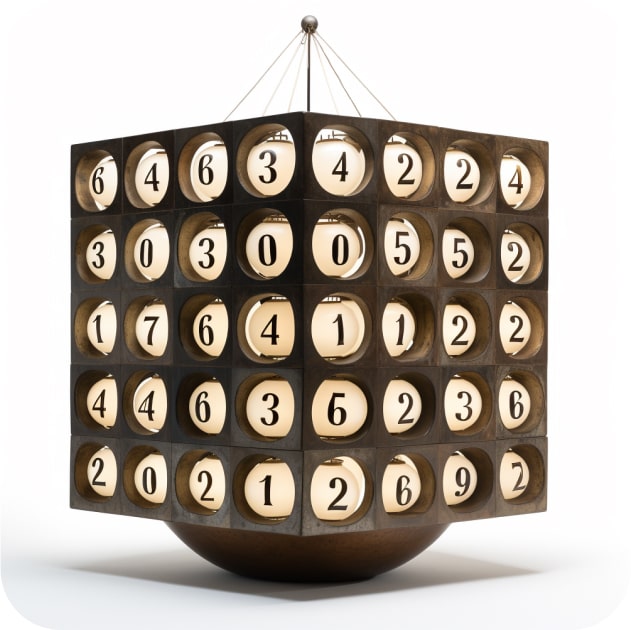
Derive
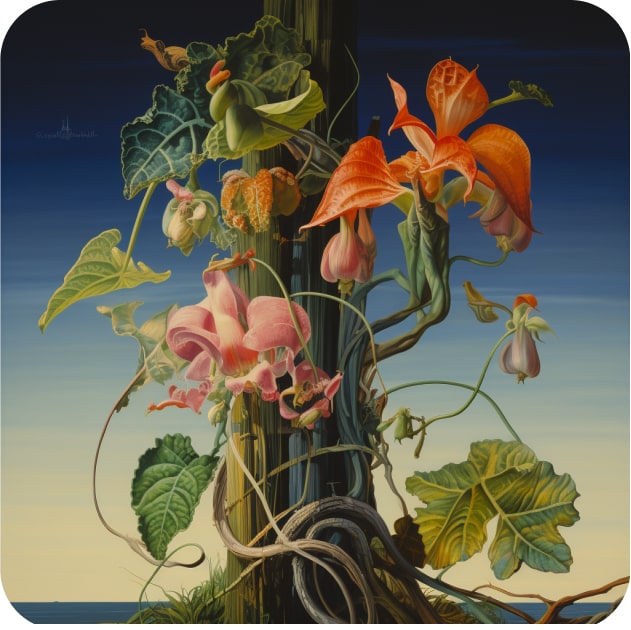
Discretize
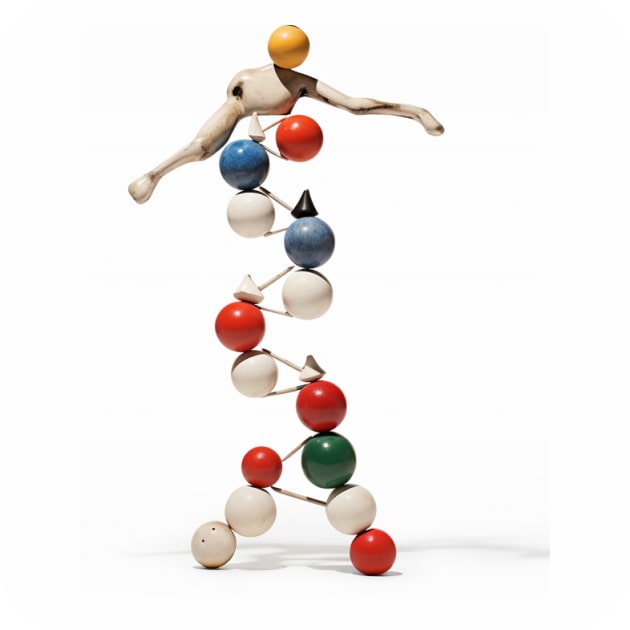
ETL
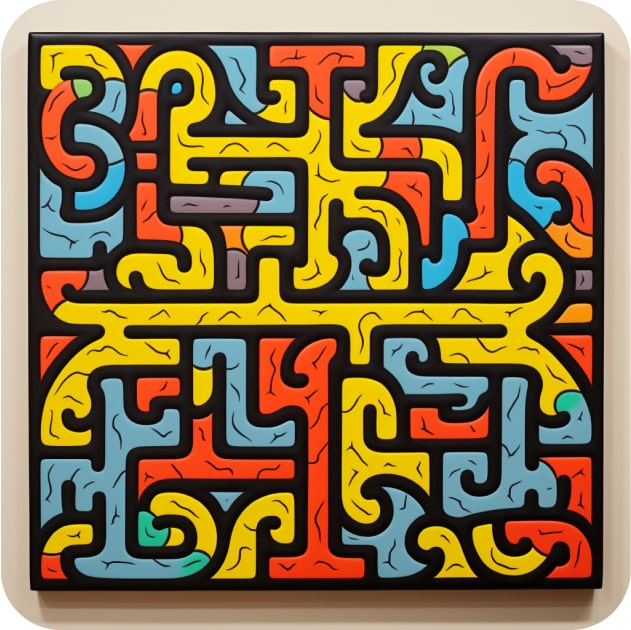
Encode
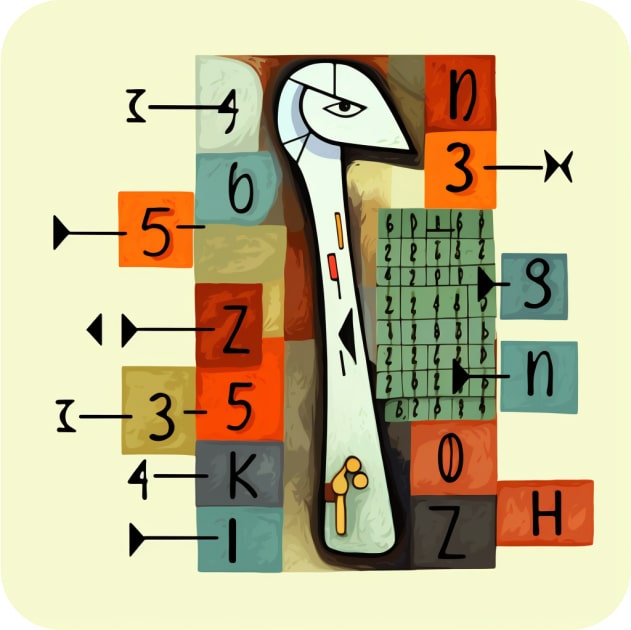
Filter
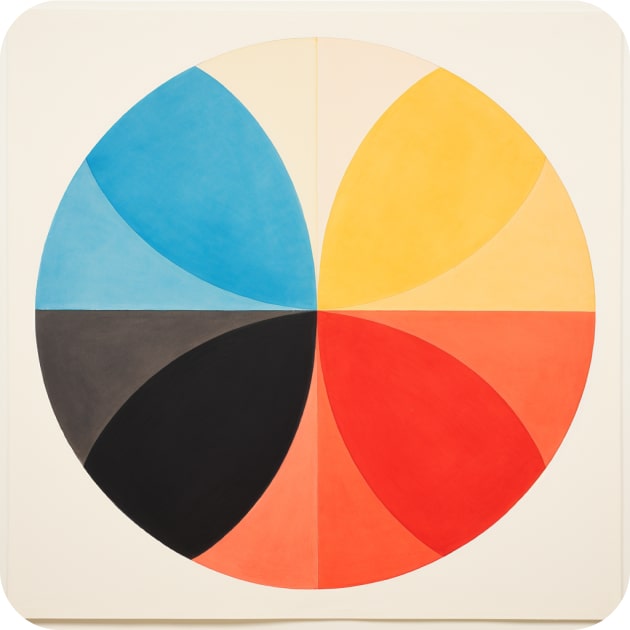
Fragment
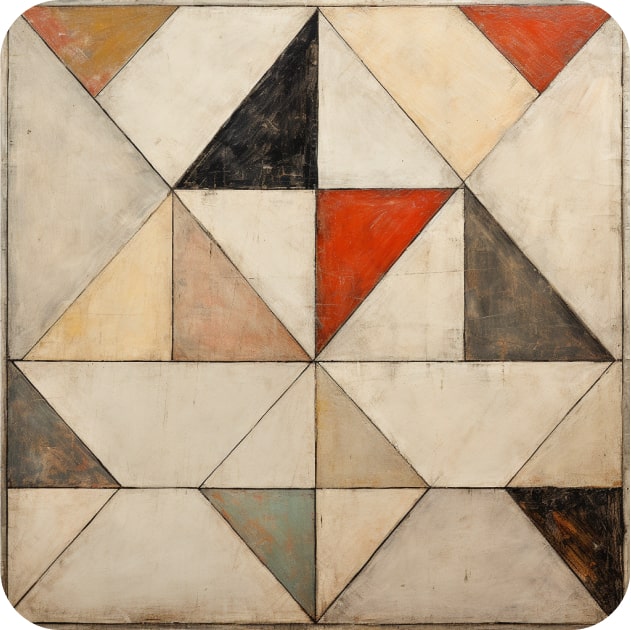
Homogenize
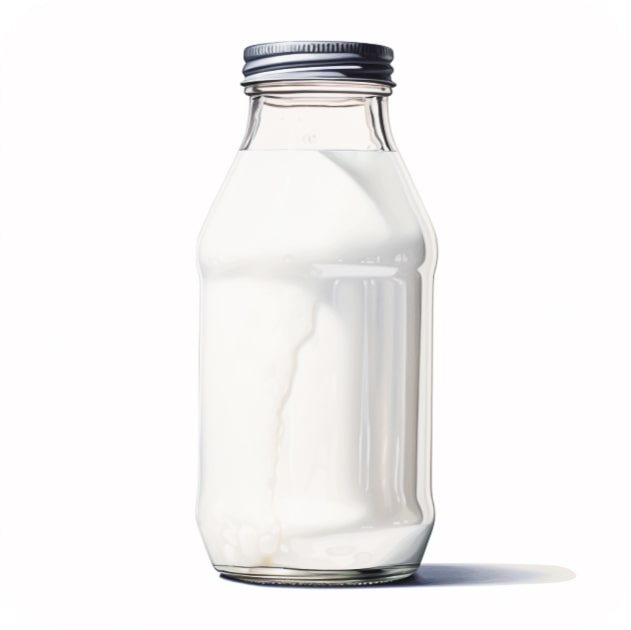
Impute
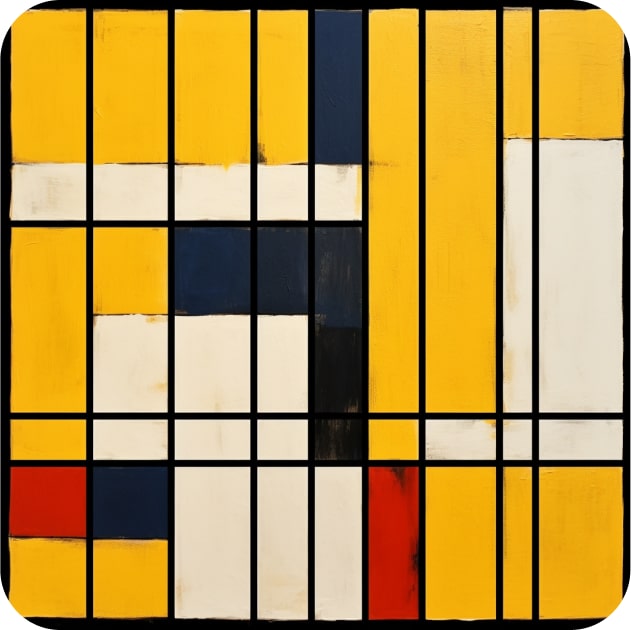
Linearize
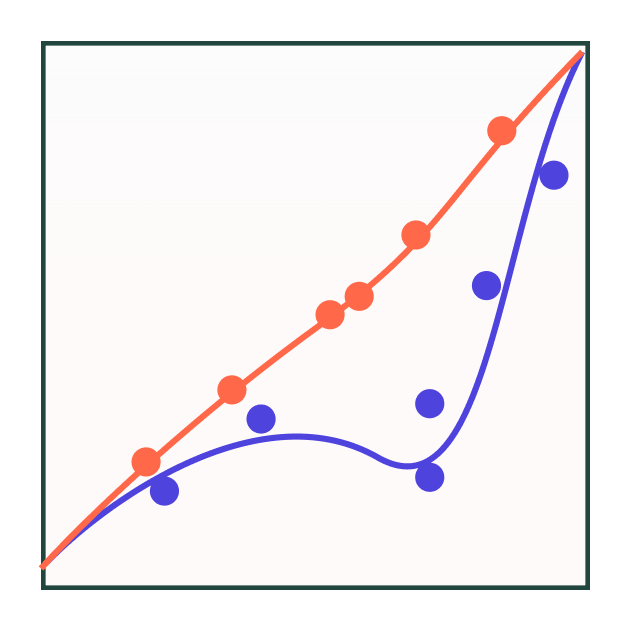
Munge
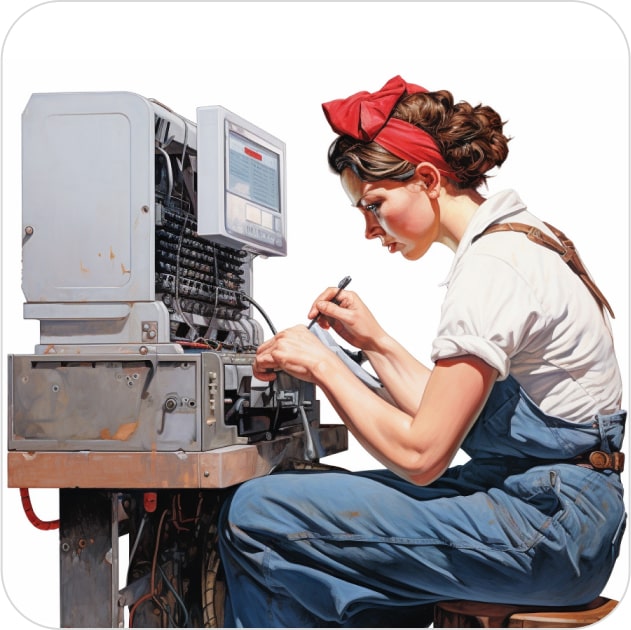
Normalize
Reduce
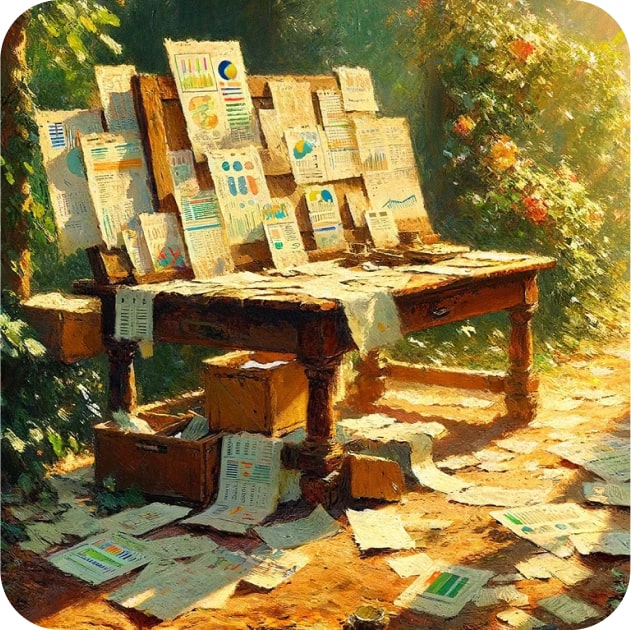
Serialize
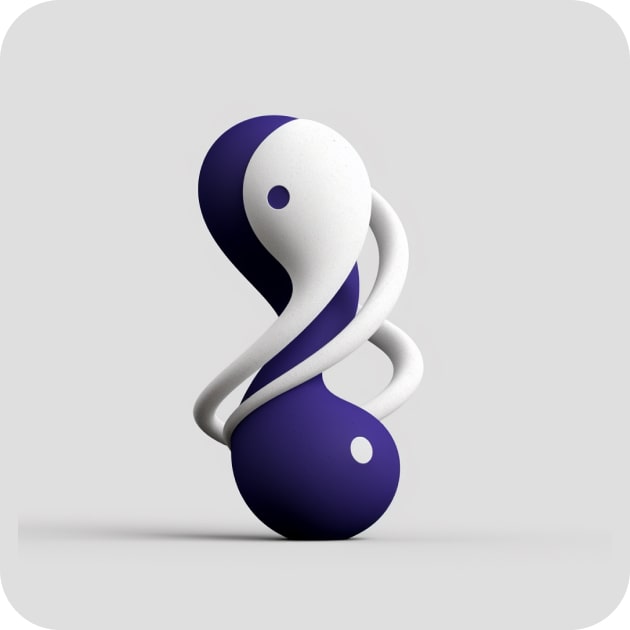
Shred
Skew
Split
Standardize
Tokenize
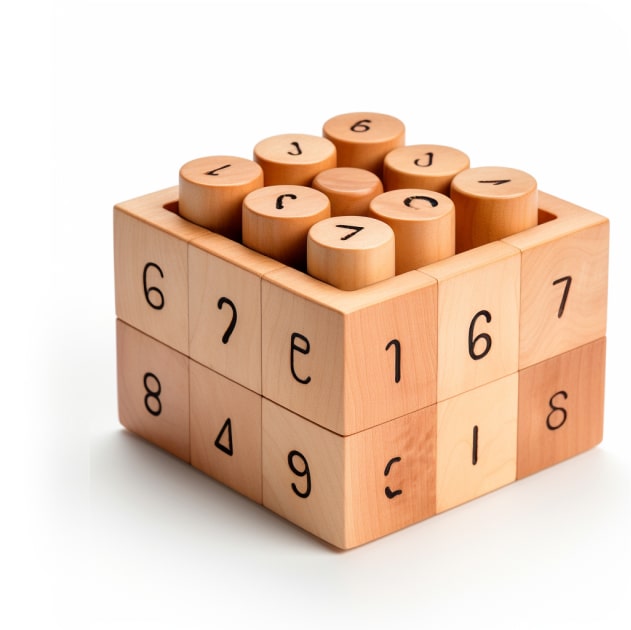
Transform
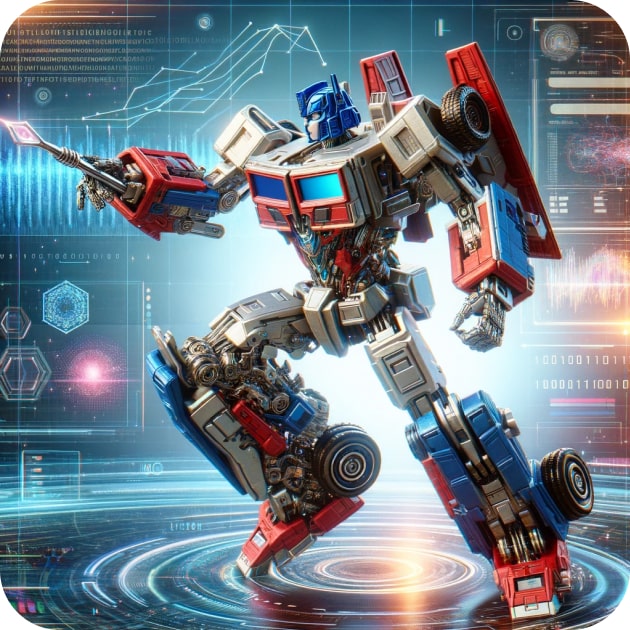
Wrangle
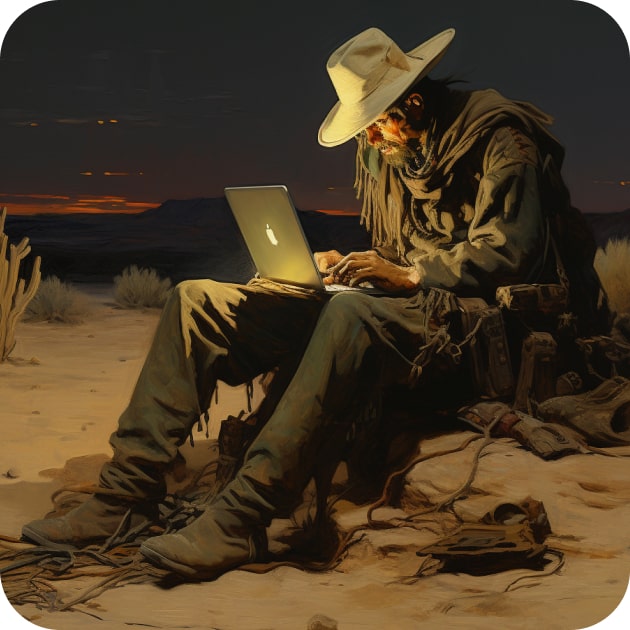