Data standardization definition:
Data standardization is the process of transforming data into a common format that allows for easy comparison and analysis. This process is useful when working with data that is collected from different sources or has different units of measurement. Data standardization involves scaling the data to a common range, usually with a mean of 0 and standard deviation of 1.
Data standardization example using Python:
Please note that you need to have the necessary Python libraries installed in your Python environment to run this code.
In Python, we can use the StandardScaler
class from the sklearn.preprocessing module to perform data standardization. Here's an example:
import numpy as np
from sklearn.preprocessing import StandardScaler
# create a sample dataset
data = np.array([[1, 2], [3, 4], [5, 6]])
# create a StandardScaler object
scaler = StandardScaler()
# fit and transform the data
scaled_data = scaler.fit_transform(data)
print(scaled_data)
This code will generate the following output:
[[-1.22474487 -1.22474487]
[ 0. 0. ]
[ 1.22474487 1.22474487]]
In this example, we create a sample dataset with 3 rows and 2 columns. We then create a StandardScaler
object and fit it to the data. Finally, we transform the data using the fit_transform
method and print the scaled data. The output shows that each column has been scaled to have a mean of 0 and a standard deviation of 1.
Align
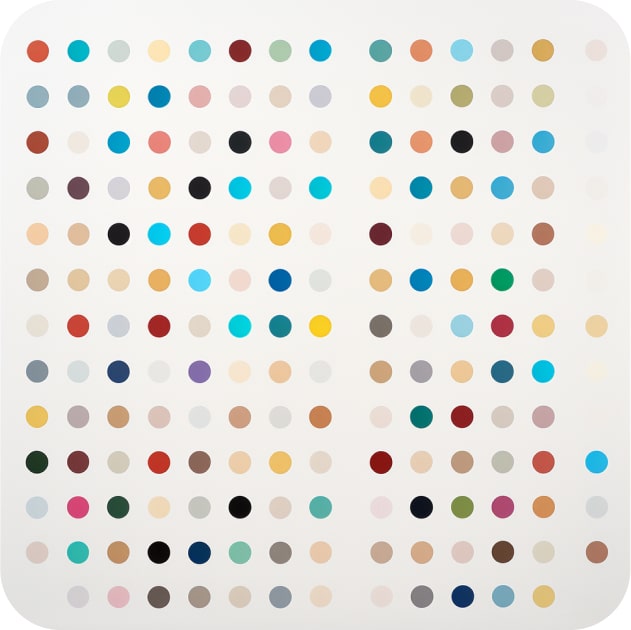
Clean or Cleanse
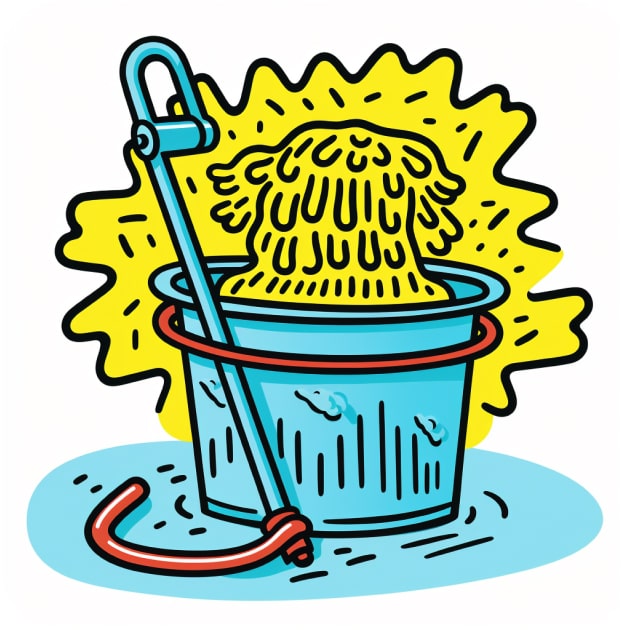
Cluster
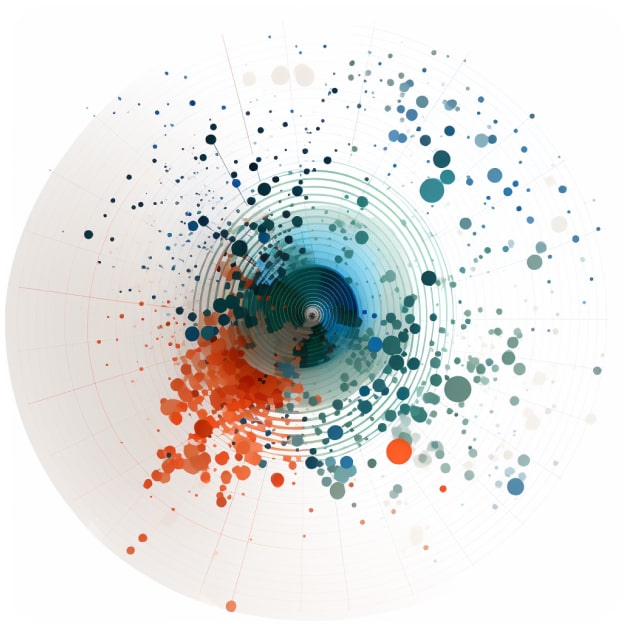
Curate
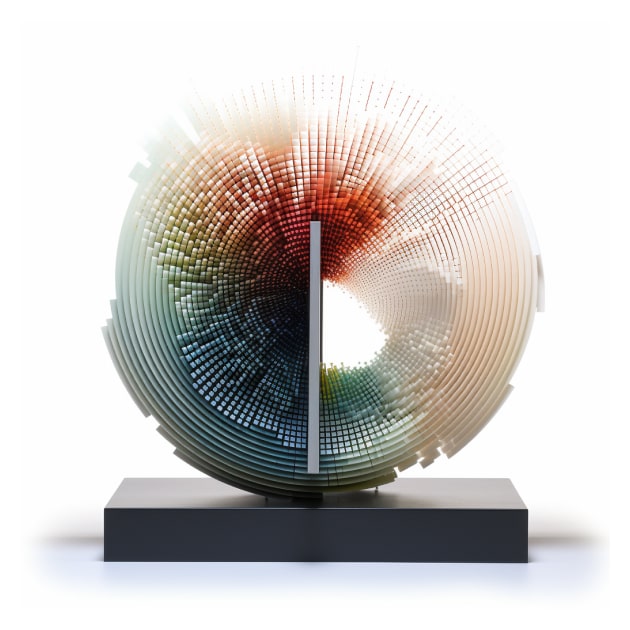
Denoise
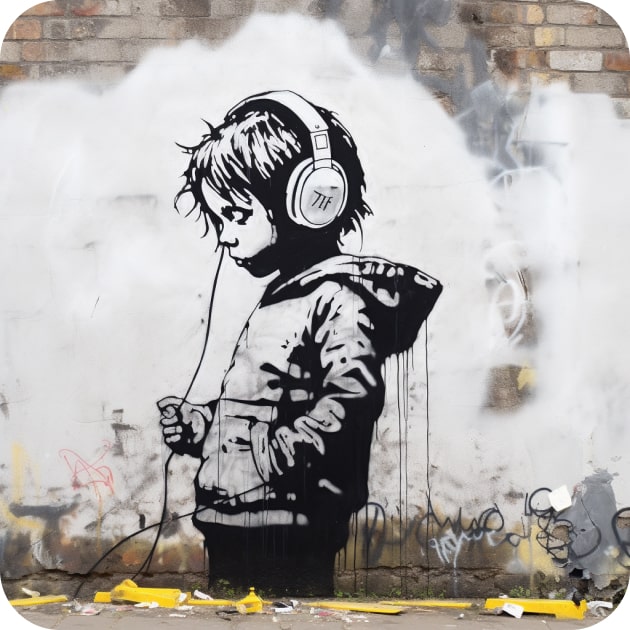
Denormalize
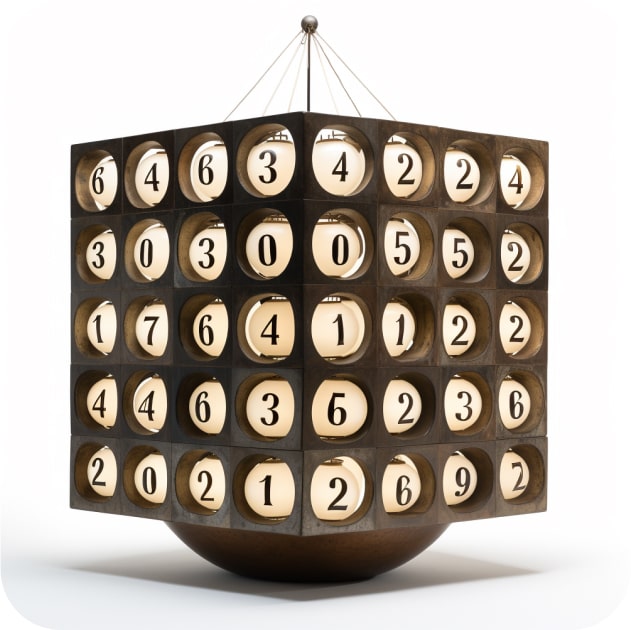
Derive
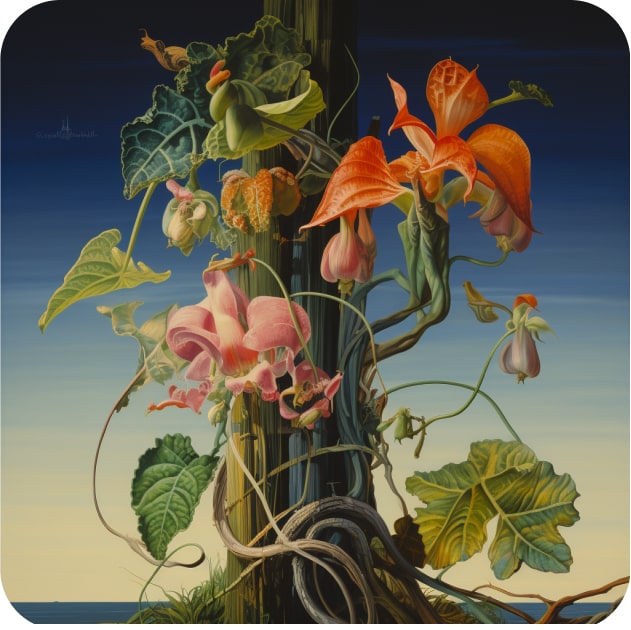
Discretize
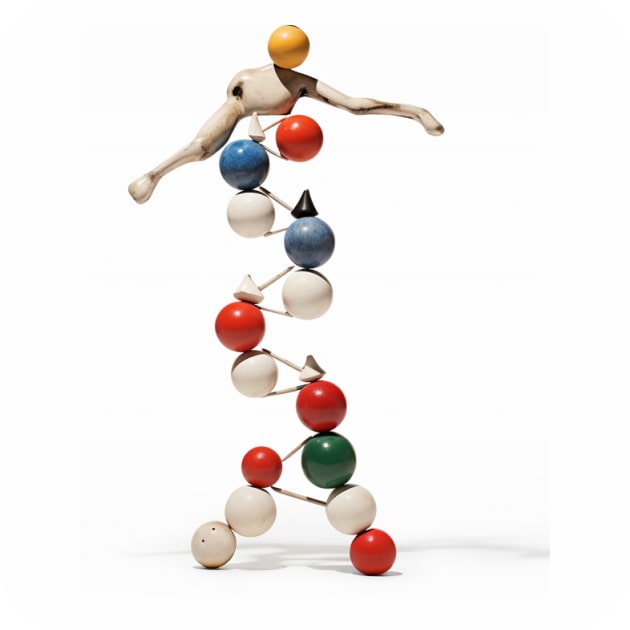
ETL
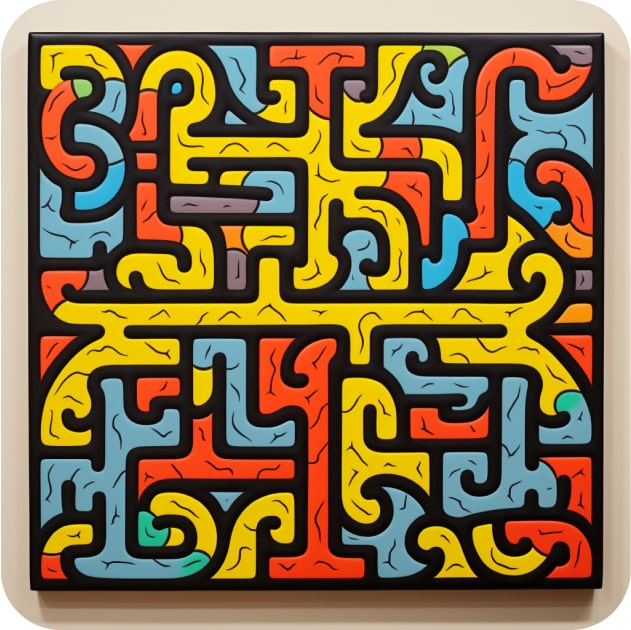
Encode
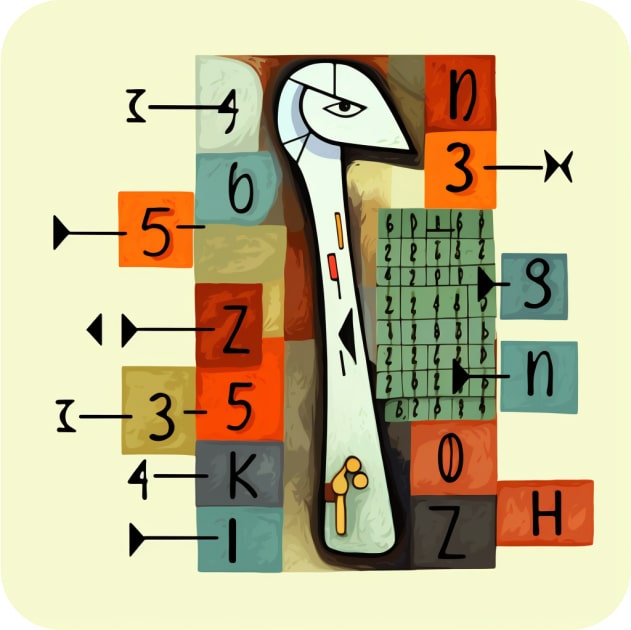
Filter
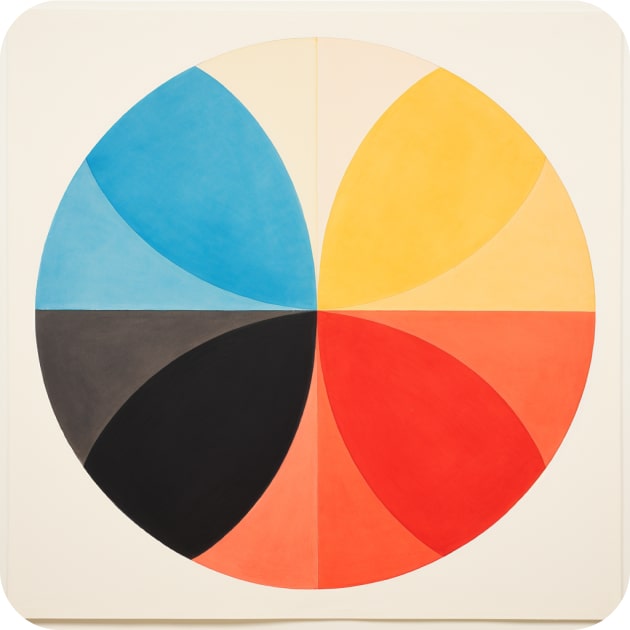
Fragment
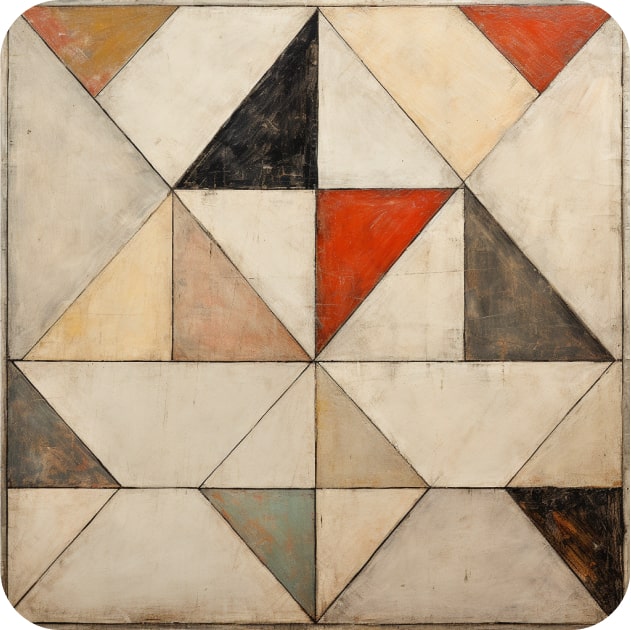
Homogenize
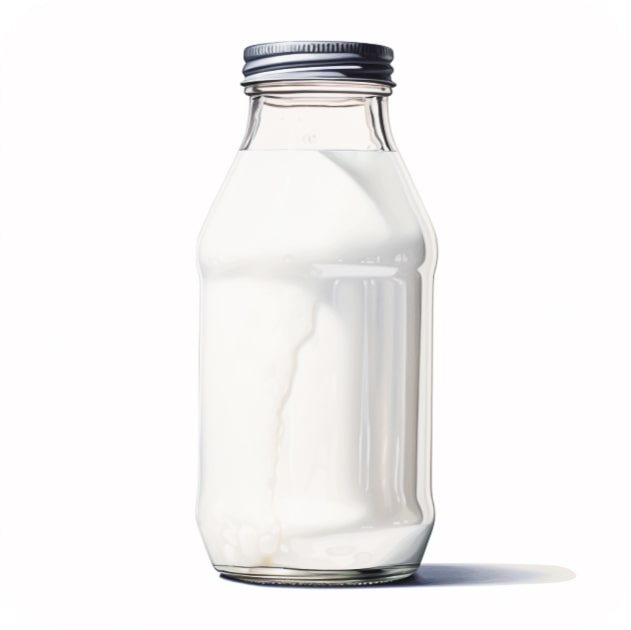
Impute
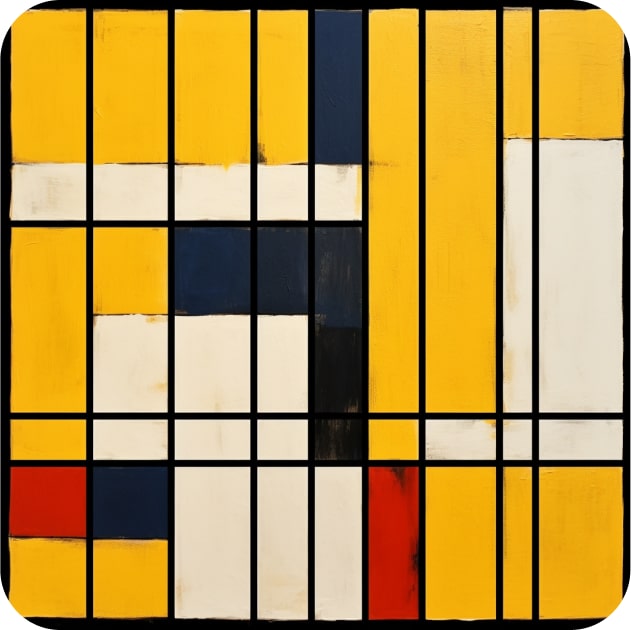
Linearize
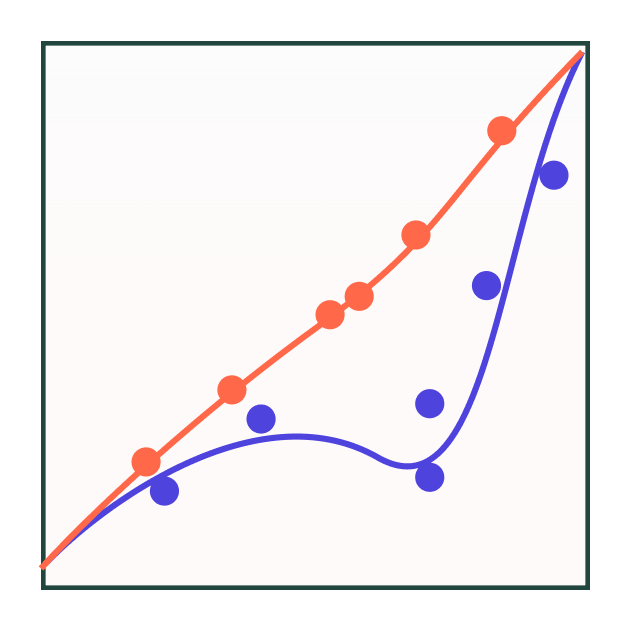
Munge
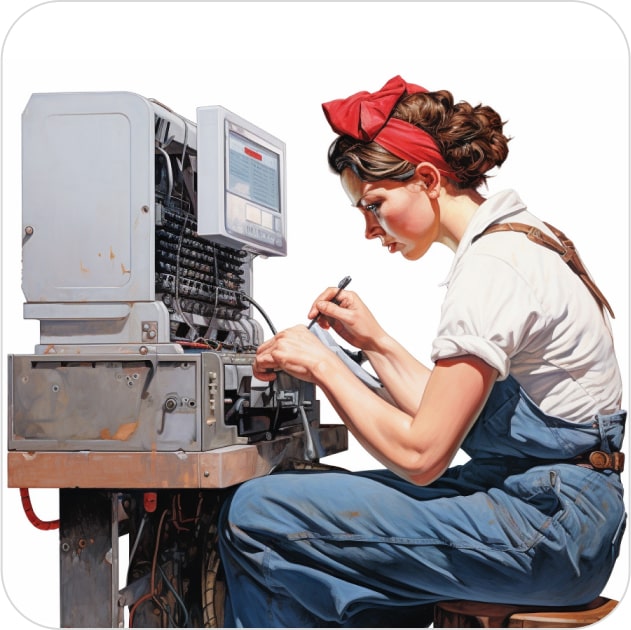
Normalize
Reduce
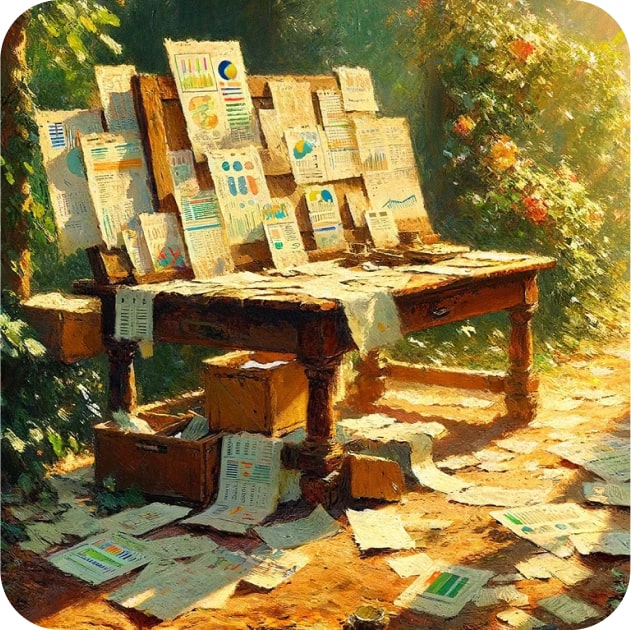
Reshape
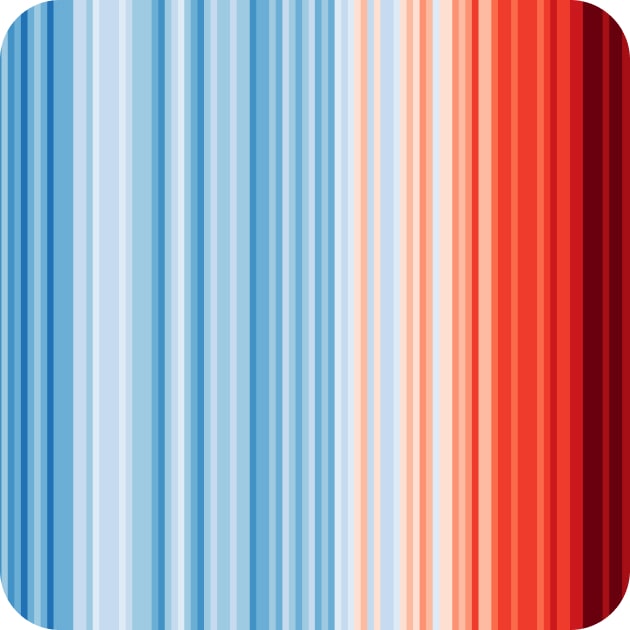
Serialize
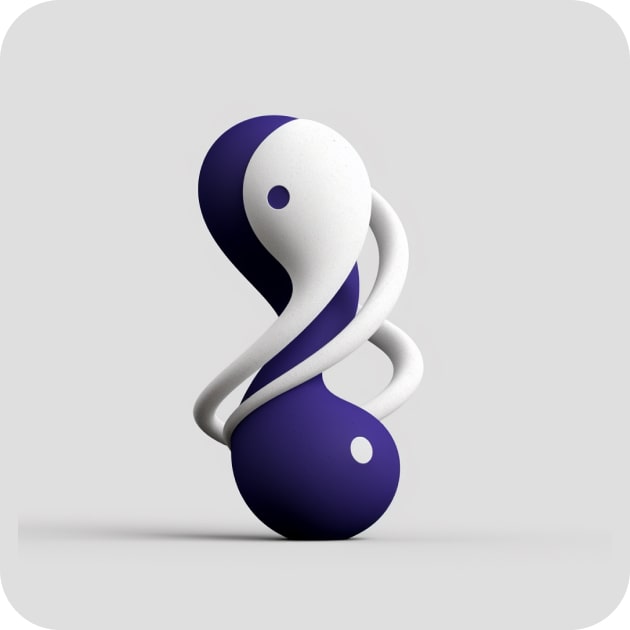
Shred
Skew
Split
Tokenize
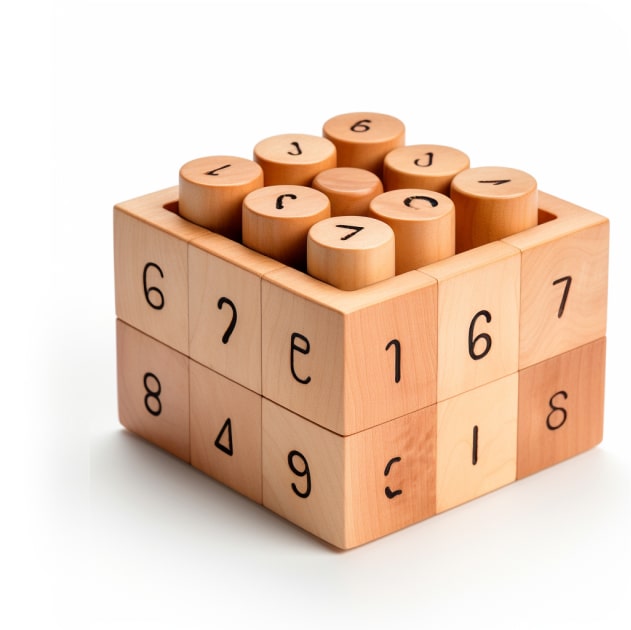
Transform
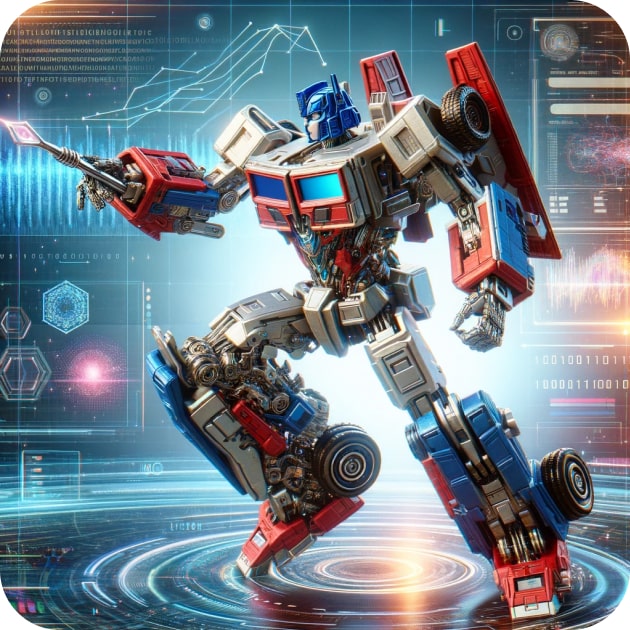
Wrangle
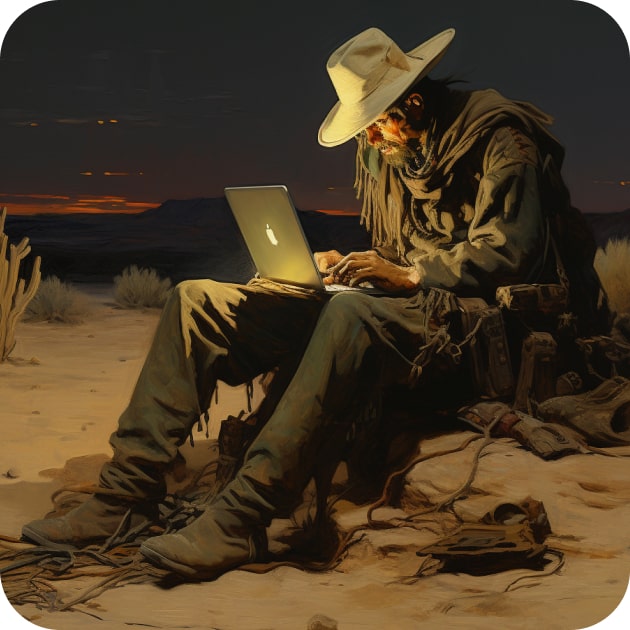